Resolve "Connect FindGameController to backend"
parent
26336913
No related branches found
No related tags found
Showing
- backend/src/index.ts 34 additions, 15 deletionsbackend/src/index.ts
- backend/src/interfaces/ILobby.ts 1 addition, 0 deletionsbackend/src/interfaces/ILobby.ts
- backend/src/lobby/Lobby.ts 4 additions, 0 deletionsbackend/src/lobby/Lobby.ts
- frontend/assets/menu-textures.atlas 8 additions, 1 deletionfrontend/assets/menu-textures.atlas
- frontend/assets/menu-textures.png 0 additions, 0 deletionsfrontend/assets/menu-textures.png
- frontend/core/src/com/game/tankwars/HTTPRequestHandler.java 74 additions, 0 deletionsfrontend/core/src/com/game/tankwars/HTTPRequestHandler.java
- frontend/core/src/com/game/tankwars/ReceiverHandler.java 4 additions, 0 deletionsfrontend/core/src/com/game/tankwars/ReceiverHandler.java
- frontend/core/src/com/game/tankwars/TankWarsGame.java 1 addition, 0 deletionsfrontend/core/src/com/game/tankwars/TankWarsGame.java
- frontend/core/src/com/game/tankwars/controller/FindGameController.java 257 additions, 38 deletions.../src/com/game/tankwars/controller/FindGameController.java
- frontend/core/src/com/game/tankwars/model/LobbyId.java 14 additions, 0 deletionsfrontend/core/src/com/game/tankwars/model/LobbyId.java
- frontend/core/src/com/game/tankwars/model/LobbyStatus.java 13 additions, 0 deletionsfrontend/core/src/com/game/tankwars/model/LobbyStatus.java
- frontend/core/src/com/game/tankwars/view/FindGameScreen.java 38 additions, 3 deletionsfrontend/core/src/com/game/tankwars/view/FindGameScreen.java
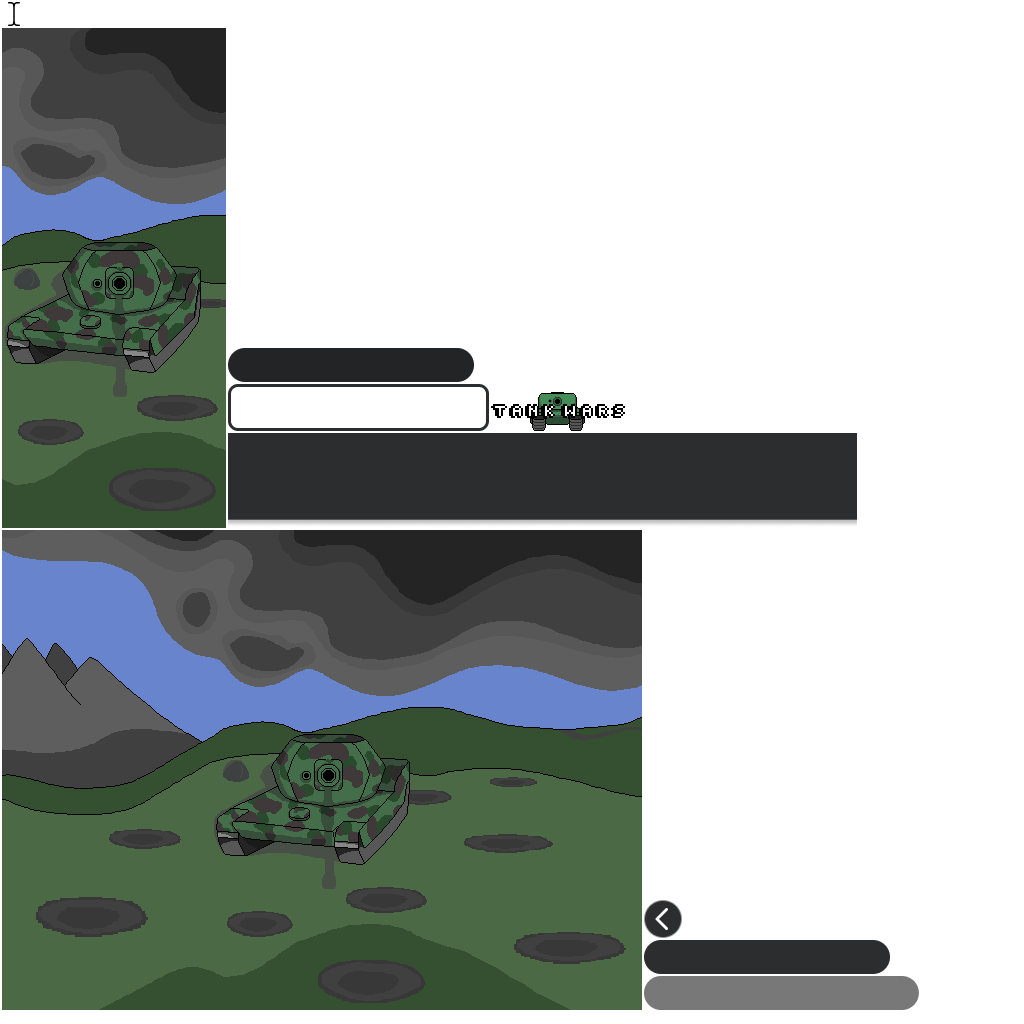
| W: | H:
| W: | H:
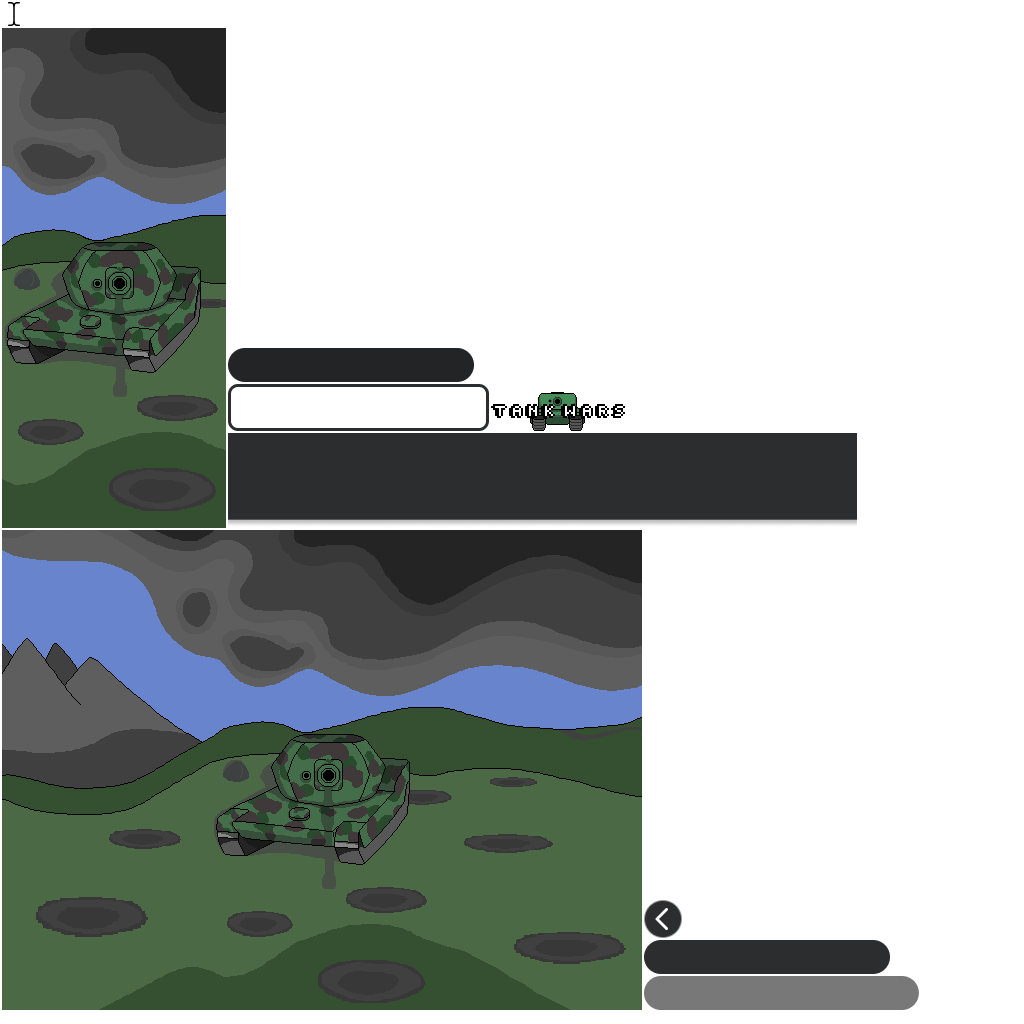
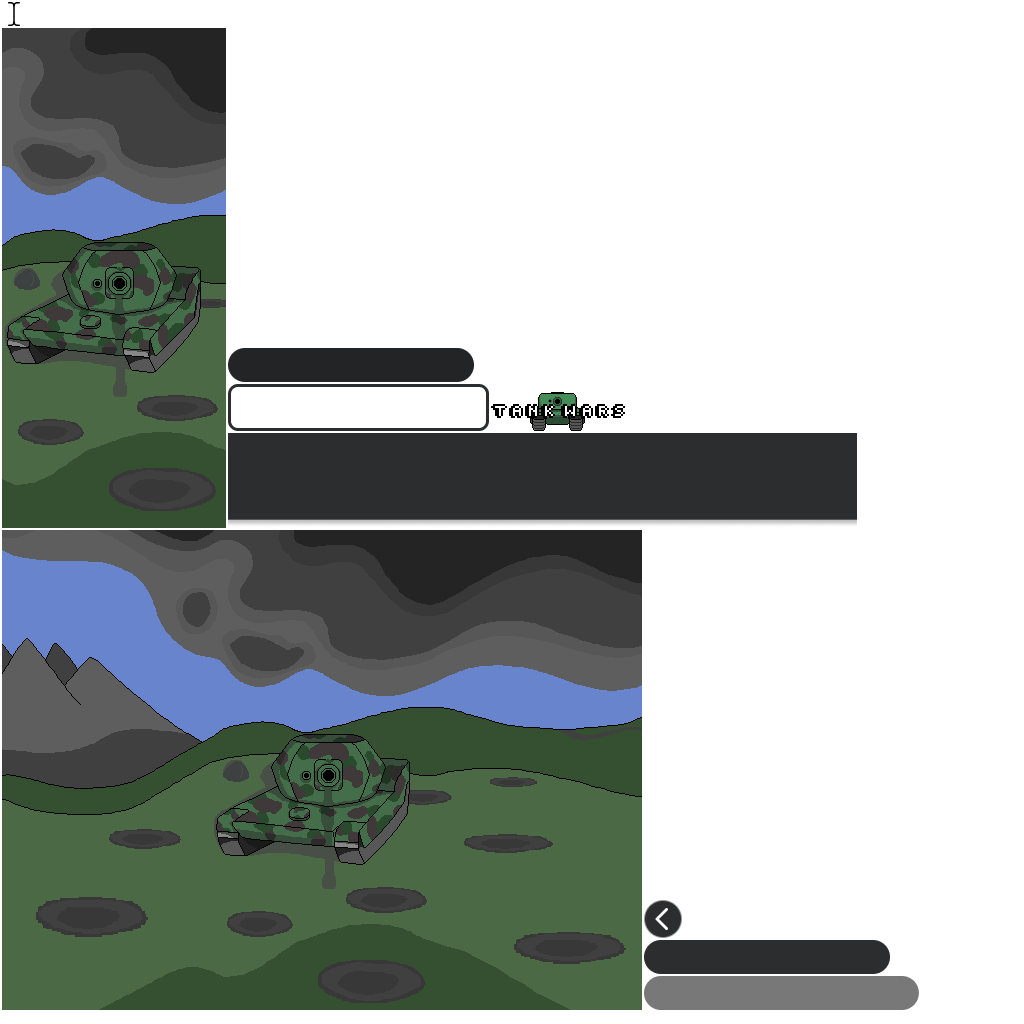