diff --git a/MrBigsock/.vscode/settings.json b/MrBigsock/.vscode/settings.json new file mode 100644 index 0000000000000000000000000000000000000000..e232cd65d2460442918b956f368fde49f1dda80c --- /dev/null +++ b/MrBigsock/.vscode/settings.json @@ -0,0 +1,55 @@ +{ + "files.exclude": + { + "**/.DS_Store":true, + "**/.git":true, + "**/.gitmodules":true, + "**/*.booproj":true, + "**/*.pidb":true, + "**/*.suo":true, + "**/*.user":true, + "**/*.userprefs":true, + "**/*.unityproj":true, + "**/*.dll":true, + "**/*.exe":true, + "**/*.pdf":true, + "**/*.mid":true, + "**/*.midi":true, + "**/*.wav":true, + "**/*.gif":true, + "**/*.ico":true, + "**/*.jpg":true, + "**/*.jpeg":true, + "**/*.png":true, + "**/*.psd":true, + "**/*.tga":true, + "**/*.tif":true, + "**/*.tiff":true, + "**/*.3ds":true, + "**/*.3DS":true, + "**/*.fbx":true, + "**/*.FBX":true, + "**/*.lxo":true, + "**/*.LXO":true, + "**/*.ma":true, + "**/*.MA":true, + "**/*.obj":true, + "**/*.OBJ":true, + "**/*.asset":true, + "**/*.cubemap":true, + "**/*.flare":true, + "**/*.mat":true, + "**/*.meta":true, + "**/*.prefab":true, + "**/*.unity":true, + "build/":true, + "Build/":true, + "Library/":true, + "library/":true, + "obj/":true, + "Obj/":true, + "ProjectSettings/":true, + "temp/":true, + "Temp/":true + } +} \ No newline at end of file diff --git a/MrBigsock/Assets/Code.meta b/MrBigsock/Assets/Code.meta new file mode 100644 index 0000000000000000000000000000000000000000..5443d5273b167d0587ff132b90845dd472574656 --- /dev/null +++ b/MrBigsock/Assets/Code.meta @@ -0,0 +1,8 @@ +fileFormatVersion: 2 +guid: 9aa5ae1ee13548344b59a85f90930f79 +folderAsset: yes +DefaultImporter: + externalObjects: {} + userData: + assetBundleName: + assetBundleVariant: diff --git a/MrBigsock/Assets/Code/PlayerController.cs b/MrBigsock/Assets/Code/PlayerController.cs new file mode 100644 index 0000000000000000000000000000000000000000..2ca3d8e8420b0f05a601c3599111270dfeb0e461 --- /dev/null +++ b/MrBigsock/Assets/Code/PlayerController.cs @@ -0,0 +1,93 @@ +using System.Collections; +using System.Collections.Generic; +using UnityEngine; +using UnityEngine.InputSystem; + +// Takes and handles input and movement for a player character +public class PlayerController : MonoBehaviour +{ + public float moveSpeed = 1f; + public float collisionOffset = 0.05f; + public ContactFilter2D movementFilter; + + Vector2 movementInput; + SpriteRenderer spriteRenderer; + Rigidbody2D rb; + Animator animator; + List<RaycastHit2D> castCollisions = new List<RaycastHit2D>(); + + bool canMove = true; + + // Start is called before the first frame update + void Start() + { + rb = GetComponent<Rigidbody2D>(); + animator = GetComponent<Animator>(); + spriteRenderer = GetComponent<SpriteRenderer>(); + } + + private void FixedUpdate() { + if(canMove) { + // If movement input is not 0, try to move + if(movementInput != Vector2.zero){ + + bool success = TryMove(movementInput); + + if(!success) { + success = TryMove(new Vector2(movementInput.x, 0)); + } + + if(!success) { + success = TryMove(new Vector2(0, movementInput.y)); + } + + animator.SetBool("isMoving", success); + } else { + animator.SetBool("isMoving", false); + } + + // Set direction of sprite to movement direction + if(movementInput.x < 0) { + spriteRenderer.flipX = true; + } else if (movementInput.x > 0) { + spriteRenderer.flipX = false; + } + } + } + + private bool TryMove(Vector2 direction) { + if(direction != Vector2.zero) { + // Check for potential collisions + int count = rb.Cast( + direction, // X and Y values between -1 and 1 that represent the direction from the body to look for collisions + movementFilter, // The settings that determine where a collision can occur on such as layers to collide with + castCollisions, // List of collisions to store the found collisions into after the Cast is finished + moveSpeed * Time.fixedDeltaTime + collisionOffset); // The amount to cast equal to the movement plus an offset + + if(count == 0){ + rb.MovePosition(rb.position + direction * moveSpeed * Time.fixedDeltaTime); + return true; + } else { + return false; + } + } else { + // Can't move if there's no direction to move in + return false; + } + + } + + void OnMove(InputValue movementValue) { + movementInput = movementValue.Get<Vector2>(); + } + + + public void LockMovement() { + canMove = false; + } + + public void UnlockMovement() { + canMove = true; + } +} + diff --git a/MrBigsock/Assets/Code/PlayerController.cs.meta b/MrBigsock/Assets/Code/PlayerController.cs.meta new file mode 100644 index 0000000000000000000000000000000000000000..9df3eaec06141ce3e7c11f08677f85d93e1f980f --- /dev/null +++ b/MrBigsock/Assets/Code/PlayerController.cs.meta @@ -0,0 +1,11 @@ +fileFormatVersion: 2 +guid: 7cac03e60e9d6f24591d5bdc111d211a +MonoImporter: + externalObjects: {} + serializedVersion: 2 + defaultReferences: [] + executionOrder: 0 + icon: {instanceID: 0} + userData: + assetBundleName: + assetBundleVariant: diff --git a/MrBigsock/Assets/Prefabs.meta b/MrBigsock/Assets/Prefabs.meta new file mode 100644 index 0000000000000000000000000000000000000000..db228d0f22fbaf164e1dd22304bb4e70b4314a66 --- /dev/null +++ b/MrBigsock/Assets/Prefabs.meta @@ -0,0 +1,8 @@ +fileFormatVersion: 2 +guid: fb3328750c2a1c64e922fc2f43691a8f +folderAsset: yes +DefaultImporter: + externalObjects: {} + userData: + assetBundleName: + assetBundleVariant: diff --git a/MrBigsock/Assets/Prefabs/BigSock.prefab b/MrBigsock/Assets/Prefabs/BigSock.prefab new file mode 100644 index 0000000000000000000000000000000000000000..900f967cb2d13ba57911004b3f081ebf01417bc5 --- /dev/null +++ b/MrBigsock/Assets/Prefabs/BigSock.prefab @@ -0,0 +1,216 @@ +%YAML 1.1 +%TAG !u! tag:unity3d.com,2011: +--- !u!1 &8799933624292384519 +GameObject: + m_ObjectHideFlags: 0 + m_CorrespondingSourceObject: {fileID: 0} + m_PrefabInstance: {fileID: 0} + m_PrefabAsset: {fileID: 0} + serializedVersion: 6 + m_Component: + - component: {fileID: 8799933624292384517} + - component: {fileID: 8799933624292384516} + - component: {fileID: 7996070617006012692} + - component: {fileID: 9039433759692224201} + - component: {fileID: 8339702841083125274} + - component: {fileID: 8616172023331984945} + - component: {fileID: 8280117626939289948} + m_Layer: 0 + m_Name: BigSock + m_TagString: Player + m_Icon: {fileID: 0} + m_NavMeshLayer: 0 + m_StaticEditorFlags: 0 + m_IsActive: 1 +--- !u!4 &8799933624292384517 +Transform: + m_ObjectHideFlags: 0 + m_CorrespondingSourceObject: {fileID: 0} + m_PrefabInstance: {fileID: 0} + m_PrefabAsset: {fileID: 0} + m_GameObject: {fileID: 8799933624292384519} + m_LocalRotation: {x: 0, y: 0, z: 0, w: 1} + m_LocalPosition: {x: 0, y: 0, z: 0} + m_LocalScale: {x: 1, y: 1, z: 1} + m_ConstrainProportionsScale: 0 + m_Children: [] + m_Father: {fileID: 0} + m_RootOrder: 0 + m_LocalEulerAnglesHint: {x: 0, y: 0, z: 0} +--- !u!212 &8799933624292384516 +SpriteRenderer: + m_ObjectHideFlags: 0 + m_CorrespondingSourceObject: {fileID: 0} + m_PrefabInstance: {fileID: 0} + m_PrefabAsset: {fileID: 0} + m_GameObject: {fileID: 8799933624292384519} + m_Enabled: 1 + m_CastShadows: 0 + m_ReceiveShadows: 0 + m_DynamicOccludee: 1 + m_StaticShadowCaster: 0 + m_MotionVectors: 1 + m_LightProbeUsage: 1 + m_ReflectionProbeUsage: 1 + m_RayTracingMode: 0 + m_RayTraceProcedural: 0 + m_RenderingLayerMask: 1 + m_RendererPriority: 0 + m_Materials: + - {fileID: 10754, guid: 0000000000000000f000000000000000, type: 0} + m_StaticBatchInfo: + firstSubMesh: 0 + subMeshCount: 0 + m_StaticBatchRoot: {fileID: 0} + m_ProbeAnchor: {fileID: 0} + m_LightProbeVolumeOverride: {fileID: 0} + m_ScaleInLightmap: 1 + m_ReceiveGI: 1 + m_PreserveUVs: 0 + m_IgnoreNormalsForChartDetection: 0 + m_ImportantGI: 0 + m_StitchLightmapSeams: 1 + m_SelectedEditorRenderState: 0 + m_MinimumChartSize: 4 + m_AutoUVMaxDistance: 0.5 + m_AutoUVMaxAngle: 89 + m_LightmapParameters: {fileID: 0} + m_SortingLayerID: 0 + m_SortingLayer: 0 + m_SortingOrder: 2 + m_Sprite: {fileID: 2440943779331152110, guid: 4c2297f5370fd0440a68aa88193024e7, type: 3} + m_Color: {r: 1, g: 1, b: 1, a: 1} + m_FlipX: 0 + m_FlipY: 0 + m_DrawMode: 0 + m_Size: {x: 2, y: 2} + m_AdaptiveModeThreshold: 0.5 + m_SpriteTileMode: 0 + m_WasSpriteAssigned: 1 + m_MaskInteraction: 0 + m_SpriteSortPoint: 0 +--- !u!114 &7996070617006012692 +MonoBehaviour: + m_ObjectHideFlags: 0 + m_CorrespondingSourceObject: {fileID: 0} + m_PrefabInstance: {fileID: 0} + m_PrefabAsset: {fileID: 0} + m_GameObject: {fileID: 8799933624292384519} + m_Enabled: 1 + m_EditorHideFlags: 0 + m_Script: {fileID: 11500000, guid: 62899f850307741f2a39c98a8b639597, type: 3} + m_Name: + m_EditorClassIdentifier: + m_Actions: {fileID: -944628639613478452, guid: cf470a27421e24644b34218d18002b82, type: 3} + m_NotificationBehavior: 0 + m_UIInputModule: {fileID: 0} + m_DeviceLostEvent: + m_PersistentCalls: + m_Calls: [] + m_DeviceRegainedEvent: + m_PersistentCalls: + m_Calls: [] + m_ControlsChangedEvent: + m_PersistentCalls: + m_Calls: [] + m_ActionEvents: [] + m_NeverAutoSwitchControlSchemes: 0 + m_DefaultControlScheme: + m_DefaultActionMap: Player + m_SplitScreenIndex: -1 + m_Camera: {fileID: 0} +--- !u!114 &9039433759692224201 +MonoBehaviour: + m_ObjectHideFlags: 0 + m_CorrespondingSourceObject: {fileID: 0} + m_PrefabInstance: {fileID: 0} + m_PrefabAsset: {fileID: 0} + m_GameObject: {fileID: 8799933624292384519} + m_Enabled: 1 + m_EditorHideFlags: 0 + m_Script: {fileID: 11500000, guid: 7cac03e60e9d6f24591d5bdc111d211a, type: 3} + m_Name: + m_EditorClassIdentifier: + moveSpeed: 5 + collisionOffset: 0.05 + movementFilter: + useTriggers: 0 + useLayerMask: 0 + useDepth: 0 + useOutsideDepth: 0 + useNormalAngle: 0 + useOutsideNormalAngle: 0 + layerMask: + serializedVersion: 2 + m_Bits: 0 + minDepth: 0 + maxDepth: 0 + minNormalAngle: 0 + maxNormalAngle: 0 +--- !u!50 &8339702841083125274 +Rigidbody2D: + serializedVersion: 4 + m_ObjectHideFlags: 0 + m_CorrespondingSourceObject: {fileID: 0} + m_PrefabInstance: {fileID: 0} + m_PrefabAsset: {fileID: 0} + m_GameObject: {fileID: 8799933624292384519} + m_BodyType: 1 + m_Simulated: 1 + m_UseFullKinematicContacts: 0 + m_UseAutoMass: 0 + m_Mass: 1 + m_LinearDrag: 0 + m_AngularDrag: 0.05 + m_GravityScale: 1 + m_Material: {fileID: 0} + m_Interpolate: 0 + m_SleepingMode: 1 + m_CollisionDetection: 0 + m_Constraints: 0 +--- !u!95 &8616172023331984945 +Animator: + serializedVersion: 4 + m_ObjectHideFlags: 0 + m_CorrespondingSourceObject: {fileID: 0} + m_PrefabInstance: {fileID: 0} + m_PrefabAsset: {fileID: 0} + m_GameObject: {fileID: 8799933624292384519} + m_Enabled: 1 + m_Avatar: {fileID: 0} + m_Controller: {fileID: 9100000, guid: 7bc7bec76fc4588429b332093f2416b9, type: 2} + m_CullingMode: 0 + m_UpdateMode: 0 + m_ApplyRootMotion: 0 + m_LinearVelocityBlending: 0 + m_StabilizeFeet: 0 + m_WarningMessage: + m_HasTransformHierarchy: 1 + m_AllowConstantClipSamplingOptimization: 1 + m_KeepAnimatorControllerStateOnDisable: 0 +--- !u!61 &8280117626939289948 +BoxCollider2D: + m_ObjectHideFlags: 0 + m_CorrespondingSourceObject: {fileID: 0} + m_PrefabInstance: {fileID: 0} + m_PrefabAsset: {fileID: 0} + m_GameObject: {fileID: 8799933624292384519} + m_Enabled: 1 + m_Density: 1 + m_Material: {fileID: 0} + m_IsTrigger: 0 + m_UsedByEffector: 0 + m_UsedByComposite: 0 + m_Offset: {x: -0.20459312, y: -0.77745444} + m_SpriteTilingProperty: + border: {x: 0, y: 0, z: 0, w: 0} + pivot: {x: 0.5, y: 0.5} + oldSize: {x: 2, y: 2} + newSize: {x: 2, y: 2} + adaptiveTilingThreshold: 0.5 + drawMode: 0 + adaptiveTiling: 0 + m_AutoTiling: 0 + serializedVersion: 2 + m_Size: {x: 0.60876524, y: 0.40417254} + m_EdgeRadius: 0 diff --git a/MrBigsock/Assets/Prefabs/BigSock.prefab.meta b/MrBigsock/Assets/Prefabs/BigSock.prefab.meta new file mode 100644 index 0000000000000000000000000000000000000000..27cad852111d01912170e13ef8c66e56f9849ae8 --- /dev/null +++ b/MrBigsock/Assets/Prefabs/BigSock.prefab.meta @@ -0,0 +1,7 @@ +fileFormatVersion: 2 +guid: 008ac26ba660ab94484970b17c589923 +PrefabImporter: + externalObjects: {} + userData: + assetBundleName: + assetBundleVariant: diff --git a/MrBigsock/Assets/Scenes/example.unity b/MrBigsock/Assets/Scenes/example.unity new file mode 100644 index 0000000000000000000000000000000000000000..a0d9b57fdd5201ba30efecf7651349a417d3ca06 --- /dev/null +++ b/MrBigsock/Assets/Scenes/example.unity @@ -0,0 +1,2187 @@ +%YAML 1.1 +%TAG !u! tag:unity3d.com,2011: +--- !u!29 &1 +OcclusionCullingSettings: + m_ObjectHideFlags: 0 + serializedVersion: 2 + m_OcclusionBakeSettings: + smallestOccluder: 5 + smallestHole: 0.25 + backfaceThreshold: 100 + m_SceneGUID: 00000000000000000000000000000000 + m_OcclusionCullingData: {fileID: 0} +--- !u!104 &2 +RenderSettings: + m_ObjectHideFlags: 0 + serializedVersion: 9 + m_Fog: 0 + m_FogColor: {r: 0.5, g: 0.5, b: 0.5, a: 1} + m_FogMode: 3 + m_FogDensity: 0.01 + m_LinearFogStart: 0 + m_LinearFogEnd: 300 + m_AmbientSkyColor: {r: 0.212, g: 0.227, b: 0.259, a: 1} + m_AmbientEquatorColor: {r: 0.114, g: 0.125, b: 0.133, a: 1} + m_AmbientGroundColor: {r: 0.047, g: 0.043, b: 0.035, a: 1} + m_AmbientIntensity: 1 + m_AmbientMode: 3 + m_SubtractiveShadowColor: {r: 0.42, g: 0.478, b: 0.627, a: 1} + m_SkyboxMaterial: {fileID: 0} + m_HaloStrength: 0.5 + m_FlareStrength: 1 + m_FlareFadeSpeed: 3 + m_HaloTexture: {fileID: 0} + m_SpotCookie: {fileID: 10001, guid: 0000000000000000e000000000000000, type: 0} + m_DefaultReflectionMode: 0 + m_DefaultReflectionResolution: 128 + m_ReflectionBounces: 1 + m_ReflectionIntensity: 1 + m_CustomReflection: {fileID: 0} + m_Sun: {fileID: 0} + m_IndirectSpecularColor: {r: 0, g: 0, b: 0, a: 1} + m_UseRadianceAmbientProbe: 0 +--- !u!157 &3 +LightmapSettings: + m_ObjectHideFlags: 0 + serializedVersion: 12 + m_GIWorkflowMode: 1 + m_GISettings: + serializedVersion: 2 + m_BounceScale: 1 + m_IndirectOutputScale: 1 + m_AlbedoBoost: 1 + m_EnvironmentLightingMode: 0 + m_EnableBakedLightmaps: 0 + m_EnableRealtimeLightmaps: 0 + m_LightmapEditorSettings: + serializedVersion: 12 + m_Resolution: 2 + m_BakeResolution: 40 + m_AtlasSize: 1024 + m_AO: 0 + m_AOMaxDistance: 1 + m_CompAOExponent: 1 + m_CompAOExponentDirect: 0 + m_ExtractAmbientOcclusion: 0 + m_Padding: 2 + m_LightmapParameters: {fileID: 0} + m_LightmapsBakeMode: 1 + m_TextureCompression: 1 + m_FinalGather: 0 + m_FinalGatherFiltering: 1 + m_FinalGatherRayCount: 256 + m_ReflectionCompression: 2 + m_MixedBakeMode: 2 + m_BakeBackend: 1 + m_PVRSampling: 1 + m_PVRDirectSampleCount: 32 + m_PVRSampleCount: 512 + m_PVRBounces: 2 + m_PVREnvironmentSampleCount: 256 + m_PVREnvironmentReferencePointCount: 2048 + m_PVRFilteringMode: 1 + m_PVRDenoiserTypeDirect: 1 + m_PVRDenoiserTypeIndirect: 1 + m_PVRDenoiserTypeAO: 1 + m_PVRFilterTypeDirect: 0 + m_PVRFilterTypeIndirect: 0 + m_PVRFilterTypeAO: 0 + m_PVREnvironmentMIS: 1 + m_PVRCulling: 1 + m_PVRFilteringGaussRadiusDirect: 1 + m_PVRFilteringGaussRadiusIndirect: 5 + m_PVRFilteringGaussRadiusAO: 2 + m_PVRFilteringAtrousPositionSigmaDirect: 0.5 + m_PVRFilteringAtrousPositionSigmaIndirect: 2 + m_PVRFilteringAtrousPositionSigmaAO: 1 + m_ExportTrainingData: 0 + m_TrainingDataDestination: TrainingData + m_LightProbeSampleCountMultiplier: 4 + m_LightingDataAsset: {fileID: 0} + m_LightingSettings: {fileID: 0} +--- !u!196 &4 +NavMeshSettings: + serializedVersion: 2 + m_ObjectHideFlags: 0 + m_BuildSettings: + serializedVersion: 2 + agentTypeID: 0 + agentRadius: 0.5 + agentHeight: 2 + agentSlope: 45 + agentClimb: 0.4 + ledgeDropHeight: 0 + maxJumpAcrossDistance: 0 + minRegionArea: 2 + manualCellSize: 0 + cellSize: 0.16666667 + manualTileSize: 0 + tileSize: 256 + accuratePlacement: 0 + maxJobWorkers: 0 + preserveTilesOutsideBounds: 0 + debug: + m_Flags: 0 + m_NavMeshData: {fileID: 0} +--- !u!1 &26578340 +GameObject: + m_ObjectHideFlags: 0 + m_CorrespondingSourceObject: {fileID: 0} + m_PrefabInstance: {fileID: 0} + m_PrefabAsset: {fileID: 0} + serializedVersion: 6 + m_Component: + - component: {fileID: 26578342} + - component: {fileID: 26578341} + m_Layer: 0 + m_Name: Grid + m_TagString: Untagged + m_Icon: {fileID: 0} + m_NavMeshLayer: 0 + m_StaticEditorFlags: 0 + m_IsActive: 1 +--- !u!156049354 &26578341 +Grid: + m_ObjectHideFlags: 0 + m_CorrespondingSourceObject: {fileID: 0} + m_PrefabInstance: {fileID: 0} + m_PrefabAsset: {fileID: 0} + m_GameObject: {fileID: 26578340} + m_Enabled: 1 + m_CellSize: {x: 1, y: 1, z: 0} + m_CellGap: {x: 0, y: 0, z: 0} + m_CellLayout: 0 + m_CellSwizzle: 0 +--- !u!4 &26578342 +Transform: + m_ObjectHideFlags: 0 + m_CorrespondingSourceObject: {fileID: 0} + m_PrefabInstance: {fileID: 0} + m_PrefabAsset: {fileID: 0} + m_GameObject: {fileID: 26578340} + m_LocalRotation: {x: 0, y: 0, z: 0, w: 1} + m_LocalPosition: {x: 0, y: 0, z: 0} + m_LocalScale: {x: 1, y: 1, z: 1} + m_ConstrainProportionsScale: 0 + m_Children: + - {fileID: 114663867} + - {fileID: 323648872} + - {fileID: 1483953644} + m_Father: {fileID: 0} + m_RootOrder: 2 + m_LocalEulerAnglesHint: {x: 0, y: 0, z: 0} +--- !u!1 &114663866 +GameObject: + m_ObjectHideFlags: 0 + m_CorrespondingSourceObject: {fileID: 0} + m_PrefabInstance: {fileID: 0} + m_PrefabAsset: {fileID: 0} + serializedVersion: 6 + m_Component: + - component: {fileID: 114663867} + - component: {fileID: 114663869} + - component: {fileID: 114663868} + m_Layer: 0 + m_Name: Floor + m_TagString: Untagged + m_Icon: {fileID: 0} + m_NavMeshLayer: 0 + m_StaticEditorFlags: 0 + m_IsActive: 1 +--- !u!4 &114663867 +Transform: + m_ObjectHideFlags: 0 + m_CorrespondingSourceObject: {fileID: 0} + m_PrefabInstance: {fileID: 0} + m_PrefabAsset: {fileID: 0} + m_GameObject: {fileID: 114663866} + m_LocalRotation: {x: -0, y: -0, z: -0, w: 1} + m_LocalPosition: {x: 0, y: 0, z: 0} + m_LocalScale: {x: 1, y: 1, z: 1} + m_ConstrainProportionsScale: 0 + m_Children: [] + m_Father: {fileID: 26578342} + m_RootOrder: 0 + m_LocalEulerAnglesHint: {x: 0, y: 0, z: 0} +--- !u!483693784 &114663868 +TilemapRenderer: + m_ObjectHideFlags: 0 + m_CorrespondingSourceObject: {fileID: 0} + m_PrefabInstance: {fileID: 0} + m_PrefabAsset: {fileID: 0} + m_GameObject: {fileID: 114663866} + m_Enabled: 1 + m_CastShadows: 0 + m_ReceiveShadows: 0 + m_DynamicOccludee: 1 + m_StaticShadowCaster: 0 + m_MotionVectors: 1 + m_LightProbeUsage: 0 + m_ReflectionProbeUsage: 0 + m_RayTracingMode: 0 + m_RayTraceProcedural: 0 + m_RenderingLayerMask: 1 + m_RendererPriority: 0 + m_Materials: + - {fileID: 10754, guid: 0000000000000000f000000000000000, type: 0} + m_StaticBatchInfo: + firstSubMesh: 0 + subMeshCount: 0 + m_StaticBatchRoot: {fileID: 0} + m_ProbeAnchor: {fileID: 0} + m_LightProbeVolumeOverride: {fileID: 0} + m_ScaleInLightmap: 1 + m_ReceiveGI: 1 + m_PreserveUVs: 0 + m_IgnoreNormalsForChartDetection: 0 + m_ImportantGI: 0 + m_StitchLightmapSeams: 1 + m_SelectedEditorRenderState: 0 + m_MinimumChartSize: 4 + m_AutoUVMaxDistance: 0.5 + m_AutoUVMaxAngle: 89 + m_LightmapParameters: {fileID: 0} + m_SortingLayerID: 0 + m_SortingLayer: 0 + m_SortingOrder: 0 + m_ChunkSize: {x: 32, y: 32, z: 32} + m_ChunkCullingBounds: {x: 0, y: 0, z: 0} + m_MaxChunkCount: 16 + m_MaxFrameAge: 16 + m_SortOrder: 0 + m_Mode: 0 + m_DetectChunkCullingBounds: 0 + m_MaskInteraction: 0 +--- !u!1839735485 &114663869 +Tilemap: + m_ObjectHideFlags: 0 + m_CorrespondingSourceObject: {fileID: 0} + m_PrefabInstance: {fileID: 0} + m_PrefabAsset: {fileID: 0} + m_GameObject: {fileID: 114663866} + m_Enabled: 1 + m_Tiles: + - first: {x: -6, y: -3, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 0 + m_TileSpriteIndex: 0 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: -5, y: -3, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 0 + m_TileSpriteIndex: 0 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: -4, y: -3, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 0 + m_TileSpriteIndex: 0 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: -3, y: -3, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 0 + m_TileSpriteIndex: 0 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: -2, y: -3, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 0 + m_TileSpriteIndex: 0 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: -1, y: -3, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 0 + m_TileSpriteIndex: 0 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: 0, y: -3, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 0 + m_TileSpriteIndex: 0 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: 1, y: -3, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 0 + m_TileSpriteIndex: 0 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: 2, y: -3, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 0 + m_TileSpriteIndex: 0 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: 3, y: -3, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 0 + m_TileSpriteIndex: 0 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: 4, y: -3, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 0 + m_TileSpriteIndex: 0 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: 5, y: -3, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 0 + m_TileSpriteIndex: 0 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: 6, y: -3, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 0 + m_TileSpriteIndex: 0 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: 7, y: -3, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 0 + m_TileSpriteIndex: 0 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: -6, y: -2, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 0 + m_TileSpriteIndex: 0 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: -5, y: -2, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 0 + m_TileSpriteIndex: 0 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: -4, y: -2, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 0 + m_TileSpriteIndex: 0 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: -3, y: -2, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 0 + m_TileSpriteIndex: 0 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: -2, y: -2, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 0 + m_TileSpriteIndex: 0 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: -1, y: -2, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 0 + m_TileSpriteIndex: 0 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: 0, y: -2, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 0 + m_TileSpriteIndex: 0 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: 1, y: -2, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 0 + m_TileSpriteIndex: 0 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: 2, y: -2, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 0 + m_TileSpriteIndex: 0 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: 3, y: -2, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 0 + m_TileSpriteIndex: 0 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: 4, y: -2, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 0 + m_TileSpriteIndex: 0 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: 5, y: -2, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 0 + m_TileSpriteIndex: 0 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: 6, y: -2, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 0 + m_TileSpriteIndex: 0 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: 7, y: -2, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 0 + m_TileSpriteIndex: 0 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: -6, y: -1, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 0 + m_TileSpriteIndex: 0 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: -5, y: -1, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 0 + m_TileSpriteIndex: 0 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: -4, y: -1, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 0 + m_TileSpriteIndex: 0 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: -3, y: -1, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 0 + m_TileSpriteIndex: 0 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: -2, y: -1, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 0 + m_TileSpriteIndex: 0 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: -1, y: -1, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 0 + m_TileSpriteIndex: 0 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: 0, y: -1, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 0 + m_TileSpriteIndex: 0 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: 1, y: -1, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 0 + m_TileSpriteIndex: 0 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: 2, y: -1, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 0 + m_TileSpriteIndex: 0 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: 3, y: -1, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 0 + m_TileSpriteIndex: 0 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: 4, y: -1, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 0 + m_TileSpriteIndex: 0 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: 5, y: -1, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 0 + m_TileSpriteIndex: 0 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: 6, y: -1, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 0 + m_TileSpriteIndex: 0 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: 7, y: -1, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 0 + m_TileSpriteIndex: 0 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: -6, y: 0, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 0 + m_TileSpriteIndex: 0 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: -5, y: 0, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 0 + m_TileSpriteIndex: 0 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: -4, y: 0, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 0 + m_TileSpriteIndex: 0 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: -3, y: 0, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 0 + m_TileSpriteIndex: 0 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: -2, y: 0, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 0 + m_TileSpriteIndex: 0 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: -1, y: 0, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 0 + m_TileSpriteIndex: 0 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: 0, y: 0, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 0 + m_TileSpriteIndex: 0 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: 1, y: 0, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 0 + m_TileSpriteIndex: 0 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: 2, y: 0, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 0 + m_TileSpriteIndex: 0 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: 3, y: 0, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 0 + m_TileSpriteIndex: 0 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: 4, y: 0, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 0 + m_TileSpriteIndex: 0 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: 5, y: 0, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 0 + m_TileSpriteIndex: 0 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: 6, y: 0, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 0 + m_TileSpriteIndex: 0 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: 7, y: 0, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 0 + m_TileSpriteIndex: 0 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: -6, y: 1, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 0 + m_TileSpriteIndex: 0 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: -5, y: 1, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 0 + m_TileSpriteIndex: 0 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: -4, y: 1, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 0 + m_TileSpriteIndex: 0 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: -3, y: 1, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 0 + m_TileSpriteIndex: 0 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: -2, y: 1, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 0 + m_TileSpriteIndex: 0 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: -1, y: 1, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 0 + m_TileSpriteIndex: 0 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: 0, y: 1, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 0 + m_TileSpriteIndex: 0 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: 1, y: 1, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 0 + m_TileSpriteIndex: 0 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: 2, y: 1, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 0 + m_TileSpriteIndex: 0 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: 3, y: 1, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 0 + m_TileSpriteIndex: 0 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: 4, y: 1, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 0 + m_TileSpriteIndex: 0 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: 5, y: 1, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 0 + m_TileSpriteIndex: 0 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: 6, y: 1, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 0 + m_TileSpriteIndex: 0 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: 7, y: 1, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 0 + m_TileSpriteIndex: 0 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + m_AnimatedTiles: {} + m_TileAssetArray: + - m_RefCount: 70 + m_Data: {fileID: 11400000, guid: decde4ccadd99f044a6a9f09c51a9077, type: 2} + m_TileSpriteArray: + - m_RefCount: 70 + m_Data: {fileID: 43680089, guid: 0a806eb0d25e11c48b5dee57fb9339b5, type: 3} + m_TileMatrixArray: + - m_RefCount: 70 + m_Data: + e00: 1 + e01: 0 + e02: 0 + e03: 0 + e10: 0 + e11: 1 + e12: 0 + e13: 0 + e20: 0 + e21: 0 + e22: 1 + e23: 0 + e30: 0 + e31: 0 + e32: 0 + e33: 1 + m_TileColorArray: + - m_RefCount: 70 + m_Data: {r: 1, g: 1, b: 1, a: 1} + m_TileObjectToInstantiateArray: [] + m_AnimationFrameRate: 1 + m_Color: {r: 1, g: 1, b: 1, a: 1} + m_Origin: {x: -8, y: -3, z: 0} + m_Size: {x: 16, y: 6, z: 1} + m_TileAnchor: {x: 0.5, y: 0.5, z: 0} + m_TileOrientation: 0 + m_TileOrientationMatrix: + e00: 1 + e01: 0 + e02: 0 + e03: 0 + e10: 0 + e11: 1 + e12: 0 + e13: 0 + e20: 0 + e21: 0 + e22: 1 + e23: 0 + e30: 0 + e31: 0 + e32: 0 + e33: 1 +--- !u!1 &323648871 +GameObject: + m_ObjectHideFlags: 0 + m_CorrespondingSourceObject: {fileID: 0} + m_PrefabInstance: {fileID: 0} + m_PrefabAsset: {fileID: 0} + serializedVersion: 6 + m_Component: + - component: {fileID: 323648872} + - component: {fileID: 323648874} + - component: {fileID: 323648873} + - component: {fileID: 323648875} + m_Layer: 0 + m_Name: Foreground_wall + m_TagString: Untagged + m_Icon: {fileID: 0} + m_NavMeshLayer: 0 + m_StaticEditorFlags: 0 + m_IsActive: 1 +--- !u!4 &323648872 +Transform: + m_ObjectHideFlags: 0 + m_CorrespondingSourceObject: {fileID: 0} + m_PrefabInstance: {fileID: 0} + m_PrefabAsset: {fileID: 0} + m_GameObject: {fileID: 323648871} + m_LocalRotation: {x: -0, y: -0, z: -0, w: 1} + m_LocalPosition: {x: 0, y: 0, z: 0} + m_LocalScale: {x: 1, y: 1, z: 1} + m_ConstrainProportionsScale: 0 + m_Children: [] + m_Father: {fileID: 26578342} + m_RootOrder: 1 + m_LocalEulerAnglesHint: {x: 0, y: 0, z: 0} +--- !u!483693784 &323648873 +TilemapRenderer: + m_ObjectHideFlags: 0 + m_CorrespondingSourceObject: {fileID: 0} + m_PrefabInstance: {fileID: 0} + m_PrefabAsset: {fileID: 0} + m_GameObject: {fileID: 323648871} + m_Enabled: 1 + m_CastShadows: 0 + m_ReceiveShadows: 0 + m_DynamicOccludee: 1 + m_StaticShadowCaster: 0 + m_MotionVectors: 1 + m_LightProbeUsage: 0 + m_ReflectionProbeUsage: 0 + m_RayTracingMode: 0 + m_RayTraceProcedural: 0 + m_RenderingLayerMask: 1 + m_RendererPriority: 0 + m_Materials: + - {fileID: 10754, guid: 0000000000000000f000000000000000, type: 0} + m_StaticBatchInfo: + firstSubMesh: 0 + subMeshCount: 0 + m_StaticBatchRoot: {fileID: 0} + m_ProbeAnchor: {fileID: 0} + m_LightProbeVolumeOverride: {fileID: 0} + m_ScaleInLightmap: 1 + m_ReceiveGI: 1 + m_PreserveUVs: 0 + m_IgnoreNormalsForChartDetection: 0 + m_ImportantGI: 0 + m_StitchLightmapSeams: 1 + m_SelectedEditorRenderState: 0 + m_MinimumChartSize: 4 + m_AutoUVMaxDistance: 0.5 + m_AutoUVMaxAngle: 89 + m_LightmapParameters: {fileID: 0} + m_SortingLayerID: 0 + m_SortingLayer: 0 + m_SortingOrder: 3 + m_ChunkSize: {x: 32, y: 32, z: 32} + m_ChunkCullingBounds: {x: 0, y: 0, z: 0} + m_MaxChunkCount: 16 + m_MaxFrameAge: 16 + m_SortOrder: 0 + m_Mode: 0 + m_DetectChunkCullingBounds: 0 + m_MaskInteraction: 0 +--- !u!1839735485 &323648874 +Tilemap: + m_ObjectHideFlags: 0 + m_CorrespondingSourceObject: {fileID: 0} + m_PrefabInstance: {fileID: 0} + m_PrefabAsset: {fileID: 0} + m_GameObject: {fileID: 323648871} + m_Enabled: 1 + m_Tiles: + - first: {x: -6, y: -4, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 0 + m_TileSpriteIndex: 0 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: -5, y: -4, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 0 + m_TileSpriteIndex: 0 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: -4, y: -4, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 0 + m_TileSpriteIndex: 0 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: -3, y: -4, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 0 + m_TileSpriteIndex: 0 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: -2, y: -4, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 0 + m_TileSpriteIndex: 0 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: -1, y: -4, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 0 + m_TileSpriteIndex: 0 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: 0, y: -4, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 0 + m_TileSpriteIndex: 0 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: 1, y: -4, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 0 + m_TileSpriteIndex: 0 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: 2, y: -4, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 0 + m_TileSpriteIndex: 0 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: 3, y: -4, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 0 + m_TileSpriteIndex: 0 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: 4, y: -4, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 0 + m_TileSpriteIndex: 0 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: 5, y: -4, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 0 + m_TileSpriteIndex: 0 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: 6, y: -4, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 0 + m_TileSpriteIndex: 0 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: 7, y: -4, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 0 + m_TileSpriteIndex: 0 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: -6, y: -3, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 2 + m_TileSpriteIndex: 2 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: -5, y: -3, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 1 + m_TileSpriteIndex: 1 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: -4, y: -3, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 1 + m_TileSpriteIndex: 1 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: -3, y: -3, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 1 + m_TileSpriteIndex: 1 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: -2, y: -3, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 1 + m_TileSpriteIndex: 1 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: -1, y: -3, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 1 + m_TileSpriteIndex: 1 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: 0, y: -3, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 1 + m_TileSpriteIndex: 1 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: 1, y: -3, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 1 + m_TileSpriteIndex: 1 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: 2, y: -3, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 1 + m_TileSpriteIndex: 1 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: 3, y: -3, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 1 + m_TileSpriteIndex: 1 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: 4, y: -3, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 1 + m_TileSpriteIndex: 1 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: 5, y: -3, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 1 + m_TileSpriteIndex: 1 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: 6, y: -3, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 1 + m_TileSpriteIndex: 1 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: 7, y: -3, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 3 + m_TileSpriteIndex: 3 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + m_AnimatedTiles: {} + m_TileAssetArray: + - m_RefCount: 14 + m_Data: {fileID: 11400000, guid: 2ead1fba8f3becd49aed19156d21e54c, type: 2} + - m_RefCount: 12 + m_Data: {fileID: 11400000, guid: 257657ca820b66741ab4695fbb49f83e, type: 2} + - m_RefCount: 1 + m_Data: {fileID: 11400000, guid: 91f123c55b83ed54ea8387bbb6250b9c, type: 2} + - m_RefCount: 1 + m_Data: {fileID: 11400000, guid: abf6b1252b746f744a5040d07340a8f4, type: 2} + m_TileSpriteArray: + - m_RefCount: 14 + m_Data: {fileID: 198299372, guid: 0a806eb0d25e11c48b5dee57fb9339b5, type: 3} + - m_RefCount: 12 + m_Data: {fileID: -1085996522, guid: 0a806eb0d25e11c48b5dee57fb9339b5, type: 3} + - m_RefCount: 1 + m_Data: {fileID: -509989529, guid: 0a806eb0d25e11c48b5dee57fb9339b5, type: 3} + - m_RefCount: 1 + m_Data: {fileID: 164431376, guid: 0a806eb0d25e11c48b5dee57fb9339b5, type: 3} + m_TileMatrixArray: + - m_RefCount: 28 + m_Data: + e00: 1 + e01: 0 + e02: 0 + e03: 0 + e10: 0 + e11: 1 + e12: 0 + e13: 0 + e20: 0 + e21: 0 + e22: 1 + e23: 0 + e30: 0 + e31: 0 + e32: 0 + e33: 1 + m_TileColorArray: + - m_RefCount: 28 + m_Data: {r: 1, g: 1, b: 1, a: 1} + m_TileObjectToInstantiateArray: [] + m_AnimationFrameRate: 1 + m_Color: {r: 1, g: 1, b: 1, a: 1} + m_Origin: {x: -6, y: -4, z: 0} + m_Size: {x: 14, y: 4, z: 1} + m_TileAnchor: {x: 0.5, y: 0.5, z: 0} + m_TileOrientation: 0 + m_TileOrientationMatrix: + e00: 1 + e01: 0 + e02: 0 + e03: 0 + e10: 0 + e11: 1 + e12: 0 + e13: 0 + e20: 0 + e21: 0 + e22: 1 + e23: 0 + e30: 0 + e31: 0 + e32: 0 + e33: 1 +--- !u!19719996 &323648875 +TilemapCollider2D: + m_ObjectHideFlags: 0 + m_CorrespondingSourceObject: {fileID: 0} + m_PrefabInstance: {fileID: 0} + m_PrefabAsset: {fileID: 0} + m_GameObject: {fileID: 323648871} + m_Enabled: 1 + m_Density: 1 + m_Material: {fileID: 0} + m_IsTrigger: 0 + m_UsedByEffector: 0 + m_UsedByComposite: 0 + m_Offset: {x: 0, y: 0} + m_MaximumTileChangeCount: 1000 + m_ExtrusionFactor: 0.00001 +--- !u!1 &1483953643 +GameObject: + m_ObjectHideFlags: 0 + m_CorrespondingSourceObject: {fileID: 0} + m_PrefabInstance: {fileID: 0} + m_PrefabAsset: {fileID: 0} + serializedVersion: 6 + m_Component: + - component: {fileID: 1483953644} + - component: {fileID: 1483953646} + - component: {fileID: 1483953645} + - component: {fileID: 1483953647} + m_Layer: 0 + m_Name: Background_wall + m_TagString: Untagged + m_Icon: {fileID: 0} + m_NavMeshLayer: 0 + m_StaticEditorFlags: 0 + m_IsActive: 1 +--- !u!4 &1483953644 +Transform: + m_ObjectHideFlags: 0 + m_CorrespondingSourceObject: {fileID: 0} + m_PrefabInstance: {fileID: 0} + m_PrefabAsset: {fileID: 0} + m_GameObject: {fileID: 1483953643} + m_LocalRotation: {x: -0, y: -0, z: -0, w: 1} + m_LocalPosition: {x: 0, y: 0, z: 0} + m_LocalScale: {x: 1, y: 1, z: 1} + m_ConstrainProportionsScale: 0 + m_Children: [] + m_Father: {fileID: 26578342} + m_RootOrder: 2 + m_LocalEulerAnglesHint: {x: 0, y: 0, z: 0} +--- !u!483693784 &1483953645 +TilemapRenderer: + m_ObjectHideFlags: 0 + m_CorrespondingSourceObject: {fileID: 0} + m_PrefabInstance: {fileID: 0} + m_PrefabAsset: {fileID: 0} + m_GameObject: {fileID: 1483953643} + m_Enabled: 1 + m_CastShadows: 0 + m_ReceiveShadows: 0 + m_DynamicOccludee: 1 + m_StaticShadowCaster: 0 + m_MotionVectors: 1 + m_LightProbeUsage: 0 + m_ReflectionProbeUsage: 0 + m_RayTracingMode: 0 + m_RayTraceProcedural: 0 + m_RenderingLayerMask: 1 + m_RendererPriority: 0 + m_Materials: + - {fileID: 10754, guid: 0000000000000000f000000000000000, type: 0} + m_StaticBatchInfo: + firstSubMesh: 0 + subMeshCount: 0 + m_StaticBatchRoot: {fileID: 0} + m_ProbeAnchor: {fileID: 0} + m_LightProbeVolumeOverride: {fileID: 0} + m_ScaleInLightmap: 1 + m_ReceiveGI: 1 + m_PreserveUVs: 0 + m_IgnoreNormalsForChartDetection: 0 + m_ImportantGI: 0 + m_StitchLightmapSeams: 1 + m_SelectedEditorRenderState: 0 + m_MinimumChartSize: 4 + m_AutoUVMaxDistance: 0.5 + m_AutoUVMaxAngle: 89 + m_LightmapParameters: {fileID: 0} + m_SortingLayerID: 0 + m_SortingLayer: 0 + m_SortingOrder: 1 + m_ChunkSize: {x: 32, y: 32, z: 32} + m_ChunkCullingBounds: {x: 0, y: 0, z: 0} + m_MaxChunkCount: 16 + m_MaxFrameAge: 16 + m_SortOrder: 0 + m_Mode: 0 + m_DetectChunkCullingBounds: 0 + m_MaskInteraction: 0 +--- !u!1839735485 &1483953646 +Tilemap: + m_ObjectHideFlags: 0 + m_CorrespondingSourceObject: {fileID: 0} + m_PrefabInstance: {fileID: 0} + m_PrefabAsset: {fileID: 0} + m_GameObject: {fileID: 1483953643} + m_Enabled: 1 + m_Tiles: + - first: {x: -6, y: -3, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 1 + m_TileSpriteIndex: 1 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: 7, y: -3, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 0 + m_TileSpriteIndex: 0 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: -6, y: -2, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 3 + m_TileSpriteIndex: 3 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: 7, y: -2, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 2 + m_TileSpriteIndex: 2 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: -6, y: -1, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 3 + m_TileSpriteIndex: 3 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: 7, y: -1, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 2 + m_TileSpriteIndex: 2 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: -6, y: 0, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 3 + m_TileSpriteIndex: 3 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: 7, y: 0, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 2 + m_TileSpriteIndex: 2 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: -6, y: 1, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 4 + m_TileSpriteIndex: 4 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: -5, y: 1, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 8 + m_TileSpriteIndex: 8 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: -4, y: 1, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 8 + m_TileSpriteIndex: 8 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: -3, y: 1, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 8 + m_TileSpriteIndex: 8 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: -2, y: 1, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 8 + m_TileSpriteIndex: 8 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: -1, y: 1, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 8 + m_TileSpriteIndex: 8 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: 0, y: 1, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 8 + m_TileSpriteIndex: 8 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: 1, y: 1, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 8 + m_TileSpriteIndex: 8 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: 2, y: 1, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 8 + m_TileSpriteIndex: 8 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: 3, y: 1, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 8 + m_TileSpriteIndex: 8 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: 4, y: 1, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 8 + m_TileSpriteIndex: 8 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: 5, y: 1, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 8 + m_TileSpriteIndex: 8 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: 6, y: 1, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 8 + m_TileSpriteIndex: 8 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: 7, y: 1, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 5 + m_TileSpriteIndex: 5 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: -6, y: 2, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 7 + m_TileSpriteIndex: 7 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: -5, y: 2, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 9 + m_TileSpriteIndex: 9 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: -4, y: 2, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 9 + m_TileSpriteIndex: 9 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: -3, y: 2, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 9 + m_TileSpriteIndex: 9 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: -2, y: 2, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 9 + m_TileSpriteIndex: 9 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: -1, y: 2, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 9 + m_TileSpriteIndex: 9 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: 0, y: 2, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 9 + m_TileSpriteIndex: 9 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: 1, y: 2, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 9 + m_TileSpriteIndex: 9 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: 2, y: 2, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 9 + m_TileSpriteIndex: 9 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: 3, y: 2, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 9 + m_TileSpriteIndex: 9 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: 4, y: 2, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 9 + m_TileSpriteIndex: 9 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: 5, y: 2, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 9 + m_TileSpriteIndex: 9 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: 6, y: 2, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 9 + m_TileSpriteIndex: 9 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: 7, y: 2, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 6 + m_TileSpriteIndex: 6 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + m_AnimatedTiles: {} + m_TileAssetArray: + - m_RefCount: 1 + m_Data: {fileID: 11400000, guid: 3093f1e128519824e844277fb6d3402a, type: 2} + - m_RefCount: 1 + m_Data: {fileID: 11400000, guid: 048dff00fd5a17946a7ba7b5541a2eb6, type: 2} + - m_RefCount: 3 + m_Data: {fileID: 11400000, guid: 1d382a2702adb7f4ebc3ca6a5110afb4, type: 2} + - m_RefCount: 3 + m_Data: {fileID: 11400000, guid: 482ddd0787e8e6845bf2715684b0eba3, type: 2} + - m_RefCount: 1 + m_Data: {fileID: 11400000, guid: 256c43bc5f4ab714eab7a6f360254d43, type: 2} + - m_RefCount: 1 + m_Data: {fileID: 11400000, guid: 92968a7b2c7327e4682d7d69d2082e75, type: 2} + - m_RefCount: 1 + m_Data: {fileID: 11400000, guid: 26e1dd6488abfb34584a76d2ee5021de, type: 2} + - m_RefCount: 1 + m_Data: {fileID: 11400000, guid: 75f9c18ba8ecb2d4b98ae64f7340474a, type: 2} + - m_RefCount: 12 + m_Data: {fileID: 11400000, guid: a23d356dc7ef26b42afb97e7a7e87330, type: 2} + - m_RefCount: 12 + m_Data: {fileID: 11400000, guid: 257657ca820b66741ab4695fbb49f83e, type: 2} + m_TileSpriteArray: + - m_RefCount: 1 + m_Data: {fileID: -2046453881, guid: 0a806eb0d25e11c48b5dee57fb9339b5, type: 3} + - m_RefCount: 1 + m_Data: {fileID: 1661568666, guid: 0a806eb0d25e11c48b5dee57fb9339b5, type: 3} + - m_RefCount: 3 + m_Data: {fileID: -395624179, guid: 0a806eb0d25e11c48b5dee57fb9339b5, type: 3} + - m_RefCount: 3 + m_Data: {fileID: 453119210, guid: 0a806eb0d25e11c48b5dee57fb9339b5, type: 3} + - m_RefCount: 1 + m_Data: {fileID: -1669469920, guid: 0a806eb0d25e11c48b5dee57fb9339b5, type: 3} + - m_RefCount: 1 + m_Data: {fileID: 79250496, guid: 0a806eb0d25e11c48b5dee57fb9339b5, type: 3} + - m_RefCount: 1 + m_Data: {fileID: 1802662682, guid: 0a806eb0d25e11c48b5dee57fb9339b5, type: 3} + - m_RefCount: 1 + m_Data: {fileID: -1396940042, guid: 0a806eb0d25e11c48b5dee57fb9339b5, type: 3} + - m_RefCount: 12 + m_Data: {fileID: -650063954, guid: 0a806eb0d25e11c48b5dee57fb9339b5, type: 3} + - m_RefCount: 12 + m_Data: {fileID: -1085996522, guid: 0a806eb0d25e11c48b5dee57fb9339b5, type: 3} + m_TileMatrixArray: + - m_RefCount: 36 + m_Data: + e00: 1 + e01: 0 + e02: 0 + e03: 0 + e10: 0 + e11: 1 + e12: 0 + e13: 0 + e20: 0 + e21: 0 + e22: 1 + e23: 0 + e30: 0 + e31: 0 + e32: 0 + e33: 1 + m_TileColorArray: + - m_RefCount: 36 + m_Data: {r: 1, g: 1, b: 1, a: 1} + m_TileObjectToInstantiateArray: [] + m_AnimationFrameRate: 1 + m_Color: {r: 1, g: 1, b: 1, a: 1} + m_Origin: {x: -6, y: -3, z: 0} + m_Size: {x: 14, y: 6, z: 1} + m_TileAnchor: {x: 0.5, y: 0.5, z: 0} + m_TileOrientation: 0 + m_TileOrientationMatrix: + e00: 1 + e01: 0 + e02: 0 + e03: 0 + e10: 0 + e11: 1 + e12: 0 + e13: 0 + e20: 0 + e21: 0 + e22: 1 + e23: 0 + e30: 0 + e31: 0 + e32: 0 + e33: 1 +--- !u!19719996 &1483953647 +TilemapCollider2D: + m_ObjectHideFlags: 0 + m_CorrespondingSourceObject: {fileID: 0} + m_PrefabInstance: {fileID: 0} + m_PrefabAsset: {fileID: 0} + m_GameObject: {fileID: 1483953643} + m_Enabled: 1 + m_Density: 1 + m_Material: {fileID: 0} + m_IsTrigger: 0 + m_UsedByEffector: 0 + m_UsedByComposite: 0 + m_Offset: {x: 0, y: 0} + m_MaximumTileChangeCount: 1000 + m_ExtrusionFactor: 0.00001 +--- !u!1 &1763586303 +GameObject: + m_ObjectHideFlags: 0 + m_CorrespondingSourceObject: {fileID: 0} + m_PrefabInstance: {fileID: 0} + m_PrefabAsset: {fileID: 0} + serializedVersion: 6 + m_Component: + - component: {fileID: 1763586306} + - component: {fileID: 1763586305} + - component: {fileID: 1763586304} + m_Layer: 0 + m_Name: Main Camera + m_TagString: MainCamera + m_Icon: {fileID: 0} + m_NavMeshLayer: 0 + m_StaticEditorFlags: 0 + m_IsActive: 1 +--- !u!81 &1763586304 +AudioListener: + m_ObjectHideFlags: 0 + m_CorrespondingSourceObject: {fileID: 0} + m_PrefabInstance: {fileID: 0} + m_PrefabAsset: {fileID: 0} + m_GameObject: {fileID: 1763586303} + m_Enabled: 1 +--- !u!20 &1763586305 +Camera: + m_ObjectHideFlags: 0 + m_CorrespondingSourceObject: {fileID: 0} + m_PrefabInstance: {fileID: 0} + m_PrefabAsset: {fileID: 0} + m_GameObject: {fileID: 1763586303} + m_Enabled: 1 + serializedVersion: 2 + m_ClearFlags: 1 + m_BackGroundColor: {r: 0.19215687, g: 0.3019608, b: 0.4745098, a: 0} + m_projectionMatrixMode: 1 + m_GateFitMode: 2 + m_FOVAxisMode: 0 + m_SensorSize: {x: 36, y: 24} + m_LensShift: {x: 0, y: 0} + m_FocalLength: 50 + m_NormalizedViewPortRect: + serializedVersion: 2 + x: 0 + y: 0 + width: 1 + height: 1 + near clip plane: 0.3 + far clip plane: 1000 + field of view: 60 + orthographic: 1 + orthographic size: 5 + m_Depth: -1 + m_CullingMask: + serializedVersion: 2 + m_Bits: 4294967295 + m_RenderingPath: -1 + m_TargetTexture: {fileID: 0} + m_TargetDisplay: 0 + m_TargetEye: 3 + m_HDR: 1 + m_AllowMSAA: 1 + m_AllowDynamicResolution: 0 + m_ForceIntoRT: 0 + m_OcclusionCulling: 1 + m_StereoConvergence: 10 + m_StereoSeparation: 0.022 +--- !u!4 &1763586306 +Transform: + m_ObjectHideFlags: 0 + m_CorrespondingSourceObject: {fileID: 0} + m_PrefabInstance: {fileID: 0} + m_PrefabAsset: {fileID: 0} + m_GameObject: {fileID: 1763586303} + m_LocalRotation: {x: 0, y: 0, z: 0, w: 1} + m_LocalPosition: {x: 0, y: 0, z: -10} + m_LocalScale: {x: 1, y: 1, z: 1} + m_ConstrainProportionsScale: 0 + m_Children: [] + m_Father: {fileID: 0} + m_RootOrder: 0 + m_LocalEulerAnglesHint: {x: 0, y: 0, z: 0} +--- !u!1001 &8799933624669862766 +PrefabInstance: + m_ObjectHideFlags: 0 + serializedVersion: 2 + m_Modification: + m_TransformParent: {fileID: 0} + m_Modifications: + - target: {fileID: 8280117626939289948, guid: 008ac26ba660ab94484970b17c589923, type: 3} + propertyPath: m_Size.x + value: 0.53090835 + objectReference: {fileID: 0} + - target: {fileID: 8280117626939289948, guid: 008ac26ba660ab94484970b17c589923, type: 3} + propertyPath: m_Size.y + value: 0.26792312 + objectReference: {fileID: 0} + - target: {fileID: 8280117626939289948, guid: 008ac26ba660ab94484970b17c589923, type: 3} + propertyPath: m_Offset.x + value: -0.10727203 + objectReference: {fileID: 0} + - target: {fileID: 8280117626939289948, guid: 008ac26ba660ab94484970b17c589923, type: 3} + propertyPath: m_Offset.y + value: -0.84557915 + objectReference: {fileID: 0} + - target: {fileID: 8616172023331984945, guid: 008ac26ba660ab94484970b17c589923, type: 3} + propertyPath: m_Controller + value: + objectReference: {fileID: 9100000, guid: 7bc7bec76fc4588429b332093f2416b9, type: 2} + - target: {fileID: 8799933624292384517, guid: 008ac26ba660ab94484970b17c589923, type: 3} + propertyPath: m_RootOrder + value: 1 + objectReference: {fileID: 0} + - target: {fileID: 8799933624292384517, guid: 008ac26ba660ab94484970b17c589923, type: 3} + propertyPath: m_LocalPosition.x + value: 0 + objectReference: {fileID: 0} + - target: {fileID: 8799933624292384517, guid: 008ac26ba660ab94484970b17c589923, type: 3} + propertyPath: m_LocalPosition.y + value: 0 + objectReference: {fileID: 0} + - target: {fileID: 8799933624292384517, guid: 008ac26ba660ab94484970b17c589923, type: 3} + propertyPath: m_LocalPosition.z + value: 0 + objectReference: {fileID: 0} + - target: {fileID: 8799933624292384517, guid: 008ac26ba660ab94484970b17c589923, type: 3} + propertyPath: m_LocalRotation.w + value: 1 + objectReference: {fileID: 0} + - target: {fileID: 8799933624292384517, guid: 008ac26ba660ab94484970b17c589923, type: 3} + propertyPath: m_LocalRotation.x + value: 0 + objectReference: {fileID: 0} + - target: {fileID: 8799933624292384517, guid: 008ac26ba660ab94484970b17c589923, type: 3} + propertyPath: m_LocalRotation.y + value: 0 + objectReference: {fileID: 0} + - target: {fileID: 8799933624292384517, guid: 008ac26ba660ab94484970b17c589923, type: 3} + propertyPath: m_LocalRotation.z + value: 0 + objectReference: {fileID: 0} + - target: {fileID: 8799933624292384517, guid: 008ac26ba660ab94484970b17c589923, type: 3} + propertyPath: m_LocalEulerAnglesHint.x + value: 0 + objectReference: {fileID: 0} + - target: {fileID: 8799933624292384517, guid: 008ac26ba660ab94484970b17c589923, type: 3} + propertyPath: m_LocalEulerAnglesHint.y + value: 0 + objectReference: {fileID: 0} + - target: {fileID: 8799933624292384517, guid: 008ac26ba660ab94484970b17c589923, type: 3} + propertyPath: m_LocalEulerAnglesHint.z + value: 0 + objectReference: {fileID: 0} + - target: {fileID: 8799933624292384519, guid: 008ac26ba660ab94484970b17c589923, type: 3} + propertyPath: m_Name + value: BigSock + objectReference: {fileID: 0} + m_RemovedComponents: [] + m_SourcePrefab: {fileID: 100100000, guid: 008ac26ba660ab94484970b17c589923, type: 3} diff --git a/MrBigsock/Assets/Scenes/example.unity.meta b/MrBigsock/Assets/Scenes/example.unity.meta new file mode 100644 index 0000000000000000000000000000000000000000..8818cbd47f3f22e0c543cb9af16c81f424c07c85 --- /dev/null +++ b/MrBigsock/Assets/Scenes/example.unity.meta @@ -0,0 +1,7 @@ +fileFormatVersion: 2 +guid: a87dad853a19cd448a702e0e3f1f69e0 +DefaultImporter: + externalObjects: {} + userData: + assetBundleName: + assetBundleVariant: diff --git a/MrBigsock/Assets/Sprites/Player/Animations/idle.meta b/MrBigsock/Assets/Sprites/Player/Animations/idle.meta new file mode 100644 index 0000000000000000000000000000000000000000..08ef8c68bd5a6ff983a49b67b93e73c8af24d860 --- /dev/null +++ b/MrBigsock/Assets/Sprites/Player/Animations/idle.meta @@ -0,0 +1,8 @@ +fileFormatVersion: 2 +guid: a55ae11a94ab30c4b8f97ba2a4eab797 +folderAsset: yes +DefaultImporter: + externalObjects: {} + userData: + assetBundleName: + assetBundleVariant: diff --git a/MrBigsock/Assets/Sprites/Player/Animations/idle/BigSock.controller b/MrBigsock/Assets/Sprites/Player/Animations/idle/BigSock.controller new file mode 100644 index 0000000000000000000000000000000000000000..cb62a0141820c75bf978c006d007b32bbc845a4d --- /dev/null +++ b/MrBigsock/Assets/Sprites/Player/Animations/idle/BigSock.controller @@ -0,0 +1,159 @@ +%YAML 1.1 +%TAG !u! tag:unity3d.com,2011: +--- !u!1102 &-4318511894538673849 +AnimatorState: + serializedVersion: 6 + m_ObjectHideFlags: 1 + m_CorrespondingSourceObject: {fileID: 0} + m_PrefabInstance: {fileID: 0} + m_PrefabAsset: {fileID: 0} + m_Name: BigSock_idle + m_Speed: 1 + m_CycleOffset: 0 + m_Transitions: + - {fileID: -2660658045118055878} + m_StateMachineBehaviours: [] + m_Position: {x: 50, y: 50, z: 0} + m_IKOnFeet: 0 + m_WriteDefaultValues: 1 + m_Mirror: 0 + m_SpeedParameterActive: 0 + m_MirrorParameterActive: 0 + m_CycleOffsetParameterActive: 0 + m_TimeParameterActive: 0 + m_Motion: {fileID: 7400000, guid: 00848c5d11c8da14c9d560112aef7536, type: 2} + m_Tag: + m_SpeedParameter: + m_MirrorParameter: + m_CycleOffsetParameter: + m_TimeParameter: +--- !u!1101 &-2723339157162918007 +AnimatorStateTransition: + m_ObjectHideFlags: 1 + m_CorrespondingSourceObject: {fileID: 0} + m_PrefabInstance: {fileID: 0} + m_PrefabAsset: {fileID: 0} + m_Name: + m_Conditions: + - m_ConditionMode: 2 + m_ConditionEvent: isMoving + m_EventTreshold: 0 + m_DstStateMachine: {fileID: 0} + m_DstState: {fileID: -4318511894538673849} + m_Solo: 0 + m_Mute: 0 + m_IsExit: 0 + serializedVersion: 3 + m_TransitionDuration: 0 + m_TransitionOffset: 0 + m_ExitTime: 0.63414633 + m_HasExitTime: 0 + m_HasFixedDuration: 1 + m_InterruptionSource: 0 + m_OrderedInterruption: 1 + m_CanTransitionToSelf: 1 +--- !u!1101 &-2660658045118055878 +AnimatorStateTransition: + m_ObjectHideFlags: 1 + m_CorrespondingSourceObject: {fileID: 0} + m_PrefabInstance: {fileID: 0} + m_PrefabAsset: {fileID: 0} + m_Name: + m_Conditions: + - m_ConditionMode: 1 + m_ConditionEvent: isMoving + m_EventTreshold: 0 + m_DstStateMachine: {fileID: 0} + m_DstState: {fileID: 7302482643755999671} + m_Solo: 0 + m_Mute: 0 + m_IsExit: 0 + serializedVersion: 3 + m_TransitionDuration: 0 + m_TransitionOffset: 0 + m_ExitTime: 0.75409836 + m_HasExitTime: 0 + m_HasFixedDuration: 1 + m_InterruptionSource: 0 + m_OrderedInterruption: 1 + m_CanTransitionToSelf: 1 +--- !u!91 &9100000 +AnimatorController: + m_ObjectHideFlags: 0 + m_CorrespondingSourceObject: {fileID: 0} + m_PrefabInstance: {fileID: 0} + m_PrefabAsset: {fileID: 0} + m_Name: BigSock + serializedVersion: 5 + m_AnimatorParameters: + - m_Name: isMoving + m_Type: 4 + m_DefaultFloat: 0 + m_DefaultInt: 0 + m_DefaultBool: 0 + m_Controller: {fileID: 0} + m_AnimatorLayers: + - serializedVersion: 5 + m_Name: Base Layer + m_StateMachine: {fileID: 3674710006329895736} + m_Mask: {fileID: 0} + m_Motions: [] + m_Behaviours: [] + m_BlendingMode: 0 + m_SyncedLayerIndex: -1 + m_DefaultWeight: 0 + m_IKPass: 0 + m_SyncedLayerAffectsTiming: 0 + m_Controller: {fileID: 9100000} +--- !u!1107 &3674710006329895736 +AnimatorStateMachine: + serializedVersion: 6 + m_ObjectHideFlags: 1 + m_CorrespondingSourceObject: {fileID: 0} + m_PrefabInstance: {fileID: 0} + m_PrefabAsset: {fileID: 0} + m_Name: Base Layer + m_ChildStates: + - serializedVersion: 1 + m_State: {fileID: -4318511894538673849} + m_Position: {x: 40, y: 200, z: 0} + - serializedVersion: 1 + m_State: {fileID: 7302482643755999671} + m_Position: {x: 50, y: 290, z: 0} + m_ChildStateMachines: [] + m_AnyStateTransitions: [] + m_EntryTransitions: [] + m_StateMachineTransitions: {} + m_StateMachineBehaviours: [] + m_AnyStatePosition: {x: 50, y: 20, z: 0} + m_EntryPosition: {x: 50, y: 120, z: 0} + m_ExitPosition: {x: 800, y: 120, z: 0} + m_ParentStateMachinePosition: {x: 800, y: 20, z: 0} + m_DefaultState: {fileID: -4318511894538673849} +--- !u!1102 &7302482643755999671 +AnimatorState: + serializedVersion: 6 + m_ObjectHideFlags: 1 + m_CorrespondingSourceObject: {fileID: 0} + m_PrefabInstance: {fileID: 0} + m_PrefabAsset: {fileID: 0} + m_Name: Bigsock_walk + m_Speed: 1 + m_CycleOffset: 0 + m_Transitions: + - {fileID: -2723339157162918007} + m_StateMachineBehaviours: [] + m_Position: {x: 50, y: 50, z: 0} + m_IKOnFeet: 0 + m_WriteDefaultValues: 1 + m_Mirror: 0 + m_SpeedParameterActive: 0 + m_MirrorParameterActive: 0 + m_CycleOffsetParameterActive: 0 + m_TimeParameterActive: 0 + m_Motion: {fileID: 7400000, guid: 574950c5abe3d744a81c8a02f6c66e70, type: 2} + m_Tag: + m_SpeedParameter: + m_MirrorParameter: + m_CycleOffsetParameter: + m_TimeParameter: diff --git a/MrBigsock/Assets/Sprites/Player/Animations/idle/BigSock.controller.meta b/MrBigsock/Assets/Sprites/Player/Animations/idle/BigSock.controller.meta new file mode 100644 index 0000000000000000000000000000000000000000..89eae04e375d279e8e22009d554013fff61d6ea0 --- /dev/null +++ b/MrBigsock/Assets/Sprites/Player/Animations/idle/BigSock.controller.meta @@ -0,0 +1,8 @@ +fileFormatVersion: 2 +guid: 7bc7bec76fc4588429b332093f2416b9 +NativeFormatImporter: + externalObjects: {} + mainObjectFileID: 9100000 + userData: + assetBundleName: + assetBundleVariant: diff --git a/MrBigsock/Assets/Sprites/Player/Animations/idle/BigSock_Idle_Right.png b/MrBigsock/Assets/Sprites/Player/Animations/idle/BigSock_Idle_Right.png new file mode 100644 index 0000000000000000000000000000000000000000..6992ffa6fed6cb3b870e5b7da7325157bfaf0cf7 Binary files /dev/null and b/MrBigsock/Assets/Sprites/Player/Animations/idle/BigSock_Idle_Right.png differ diff --git a/MrBigsock/Assets/Sprites/Player/Animations/idle/BigSock_Idle_Right.png.meta b/MrBigsock/Assets/Sprites/Player/Animations/idle/BigSock_Idle_Right.png.meta new file mode 100644 index 0000000000000000000000000000000000000000..85f513399f4d4e2cb9ff4e23a8cff1f757a5f2b6 --- /dev/null +++ b/MrBigsock/Assets/Sprites/Player/Animations/idle/BigSock_Idle_Right.png.meta @@ -0,0 +1,235 @@ +fileFormatVersion: 2 +guid: 4c2297f5370fd0440a68aa88193024e7 +TextureImporter: + internalIDToNameTable: + - first: + 213: 2440943779331152110 + second: BigSock_Idle_Right_0 + - first: + 213: -3136223072955814593 + second: BigSock_Idle_Right_1 + - first: + 213: -1189885765897968158 + second: BigSock_Idle_Right_2 + - first: + 213: -5815125922403245774 + second: BigSock_Idle_Right_3 + externalObjects: {} + serializedVersion: 12 + mipmaps: + mipMapMode: 0 + enableMipMap: 0 + sRGBTexture: 1 + linearTexture: 0 + fadeOut: 0 + borderMipMap: 0 + mipMapsPreserveCoverage: 0 + alphaTestReferenceValue: 0.5 + mipMapFadeDistanceStart: 1 + mipMapFadeDistanceEnd: 3 + bumpmap: + convertToNormalMap: 0 + externalNormalMap: 0 + heightScale: 0.25 + normalMapFilter: 0 + isReadable: 0 + streamingMipmaps: 0 + streamingMipmapsPriority: 0 + vTOnly: 0 + ignoreMasterTextureLimit: 0 + grayScaleToAlpha: 0 + generateCubemap: 6 + cubemapConvolution: 0 + seamlessCubemap: 0 + textureFormat: 1 + maxTextureSize: 2048 + textureSettings: + serializedVersion: 2 + filterMode: 0 + aniso: 1 + mipBias: 0 + wrapU: 1 + wrapV: 1 + wrapW: 1 + nPOTScale: 0 + lightmap: 0 + compressionQuality: 50 + spriteMode: 2 + spriteExtrude: 1 + spriteMeshType: 1 + alignment: 0 + spritePivot: {x: 0.5, y: 0.5} + spritePixelsToUnits: 16 + spriteBorder: {x: 0, y: 0, z: 0, w: 0} + spriteGenerateFallbackPhysicsShape: 1 + alphaUsage: 1 + alphaIsTransparency: 1 + spriteTessellationDetail: -1 + textureType: 8 + textureShape: 1 + singleChannelComponent: 0 + flipbookRows: 1 + flipbookColumns: 1 + maxTextureSizeSet: 0 + compressionQualitySet: 0 + textureFormatSet: 0 + ignorePngGamma: 0 + applyGammaDecoding: 0 + cookieLightType: 1 + platformSettings: + - serializedVersion: 3 + buildTarget: DefaultTexturePlatform + maxTextureSize: 2048 + resizeAlgorithm: 0 + textureFormat: -1 + textureCompression: 1 + compressionQuality: 50 + crunchedCompression: 0 + allowsAlphaSplitting: 0 + overridden: 0 + androidETC2FallbackOverride: 0 + forceMaximumCompressionQuality_BC6H_BC7: 0 + - serializedVersion: 3 + buildTarget: Standalone + maxTextureSize: 2048 + resizeAlgorithm: 0 + textureFormat: -1 + textureCompression: 1 + compressionQuality: 50 + crunchedCompression: 0 + allowsAlphaSplitting: 0 + overridden: 0 + androidETC2FallbackOverride: 0 + forceMaximumCompressionQuality_BC6H_BC7: 0 + - serializedVersion: 3 + buildTarget: WebGL + maxTextureSize: 2048 + resizeAlgorithm: 0 + textureFormat: -1 + textureCompression: 1 + compressionQuality: 50 + crunchedCompression: 0 + allowsAlphaSplitting: 0 + overridden: 0 + androidETC2FallbackOverride: 0 + forceMaximumCompressionQuality_BC6H_BC7: 0 + - serializedVersion: 3 + buildTarget: Server + maxTextureSize: 2048 + resizeAlgorithm: 0 + textureFormat: -1 + textureCompression: 1 + compressionQuality: 50 + crunchedCompression: 0 + allowsAlphaSplitting: 0 + overridden: 0 + androidETC2FallbackOverride: 0 + forceMaximumCompressionQuality_BC6H_BC7: 0 + spriteSheet: + serializedVersion: 2 + sprites: + - serializedVersion: 2 + name: BigSock_Idle_Right_0 + rect: + serializedVersion: 2 + x: 0 + y: 0 + width: 32 + height: 32 + alignment: 0 + pivot: {x: 0, y: 0} + border: {x: 0, y: 0, z: 0, w: 0} + outline: [] + physicsShape: [] + tessellationDetail: 0 + bones: [] + spriteID: ee89bb34f69ffd120800000000000000 + internalID: 2440943779331152110 + vertices: [] + indices: + edges: [] + weights: [] + - serializedVersion: 2 + name: BigSock_Idle_Right_1 + rect: + serializedVersion: 2 + x: 32 + y: 0 + width: 32 + height: 32 + alignment: 0 + pivot: {x: 0, y: 0} + border: {x: 0, y: 0, z: 0, w: 0} + outline: [] + physicsShape: [] + tessellationDetail: 0 + bones: [] + spriteID: f3da5184bb5e974d0800000000000000 + internalID: -3136223072955814593 + vertices: [] + indices: + edges: [] + weights: [] + - serializedVersion: 2 + name: BigSock_Idle_Right_2 + rect: + serializedVersion: 2 + x: 64 + y: 0 + width: 32 + height: 32 + alignment: 0 + pivot: {x: 0, y: 0} + border: {x: 0, y: 0, z: 0, w: 0} + outline: [] + physicsShape: [] + tessellationDetail: 0 + bones: [] + spriteID: 2e5ab50433dac7fe0800000000000000 + internalID: -1189885765897968158 + vertices: [] + indices: + edges: [] + weights: [] + - serializedVersion: 2 + name: BigSock_Idle_Right_3 + rect: + serializedVersion: 2 + x: 96 + y: 0 + width: 32 + height: 32 + alignment: 0 + pivot: {x: 0, y: 0} + border: {x: 0, y: 0, z: 0, w: 0} + outline: [] + physicsShape: [] + tessellationDetail: 0 + bones: [] + spriteID: 2312dfe88c58c4fa0800000000000000 + internalID: -5815125922403245774 + vertices: [] + indices: + edges: [] + weights: [] + outline: [] + physicsShape: [] + bones: [] + spriteID: 5e97eb03825dee720800000000000000 + internalID: 0 + vertices: [] + indices: + edges: [] + weights: [] + secondaryTextures: [] + nameFileIdTable: + BigSock_Idle_Right_0: 2440943779331152110 + BigSock_Idle_Right_1: -3136223072955814593 + BigSock_Idle_Right_2: -1189885765897968158 + BigSock_Idle_Right_3: -5815125922403245774 + spritePackingTag: + pSDRemoveMatte: 0 + pSDShowRemoveMatteOption: 0 + userData: + assetBundleName: + assetBundleVariant: diff --git a/MrBigsock/Assets/Sprites/Player/Animations/idle/BigSock_idle.anim b/MrBigsock/Assets/Sprites/Player/Animations/idle/BigSock_idle.anim new file mode 100644 index 0000000000000000000000000000000000000000..e78d99288ef1cee15c737f2bf4809adf1c55dfc8 --- /dev/null +++ b/MrBigsock/Assets/Sprites/Player/Animations/idle/BigSock_idle.anim @@ -0,0 +1,80 @@ +%YAML 1.1 +%TAG !u! tag:unity3d.com,2011: +--- !u!74 &7400000 +AnimationClip: + m_ObjectHideFlags: 0 + m_CorrespondingSourceObject: {fileID: 0} + m_PrefabInstance: {fileID: 0} + m_PrefabAsset: {fileID: 0} + m_Name: BigSock_idle + serializedVersion: 6 + m_Legacy: 0 + m_Compressed: 0 + m_UseHighQualityCurve: 1 + m_RotationCurves: [] + m_CompressedRotationCurves: [] + m_EulerCurves: [] + m_PositionCurves: [] + m_ScaleCurves: [] + m_FloatCurves: [] + m_PPtrCurves: + - curve: + - time: 0 + value: {fileID: 2440943779331152110, guid: 4c2297f5370fd0440a68aa88193024e7, type: 3} + - time: 0.25 + value: {fileID: -3136223072955814593, guid: 4c2297f5370fd0440a68aa88193024e7, type: 3} + - time: 0.5 + value: {fileID: -1189885765897968158, guid: 4c2297f5370fd0440a68aa88193024e7, type: 3} + - time: 0.75 + value: {fileID: -5815125922403245774, guid: 4c2297f5370fd0440a68aa88193024e7, type: 3} + - time: 1 + value: {fileID: -5815125922403245774, guid: 4c2297f5370fd0440a68aa88193024e7, type: 3} + attribute: m_Sprite + path: + classID: 212 + script: {fileID: 0} + m_SampleRate: 60 + m_WrapMode: 0 + m_Bounds: + m_Center: {x: 0, y: 0, z: 0} + m_Extent: {x: 0, y: 0, z: 0} + m_ClipBindingConstant: + genericBindings: + - serializedVersion: 2 + path: 0 + attribute: 0 + script: {fileID: 0} + typeID: 212 + customType: 23 + isPPtrCurve: 1 + pptrCurveMapping: + - {fileID: 2440943779331152110, guid: 4c2297f5370fd0440a68aa88193024e7, type: 3} + - {fileID: -3136223072955814593, guid: 4c2297f5370fd0440a68aa88193024e7, type: 3} + - {fileID: -1189885765897968158, guid: 4c2297f5370fd0440a68aa88193024e7, type: 3} + - {fileID: -5815125922403245774, guid: 4c2297f5370fd0440a68aa88193024e7, type: 3} + - {fileID: -5815125922403245774, guid: 4c2297f5370fd0440a68aa88193024e7, type: 3} + m_AnimationClipSettings: + serializedVersion: 2 + m_AdditiveReferencePoseClip: {fileID: 0} + m_AdditiveReferencePoseTime: 0 + m_StartTime: 0 + m_StopTime: 1.0166667 + m_OrientationOffsetY: 0 + m_Level: 0 + m_CycleOffset: 0 + m_HasAdditiveReferencePose: 0 + m_LoopTime: 1 + m_LoopBlend: 0 + m_LoopBlendOrientation: 0 + m_LoopBlendPositionY: 0 + m_LoopBlendPositionXZ: 0 + m_KeepOriginalOrientation: 0 + m_KeepOriginalPositionY: 1 + m_KeepOriginalPositionXZ: 0 + m_HeightFromFeet: 0 + m_Mirror: 0 + m_EditorCurves: [] + m_EulerEditorCurves: [] + m_HasGenericRootTransform: 0 + m_HasMotionFloatCurves: 0 + m_Events: [] diff --git a/MrBigsock/Assets/Sprites/Player/Animations/idle/BigSock_idle.anim.meta b/MrBigsock/Assets/Sprites/Player/Animations/idle/BigSock_idle.anim.meta new file mode 100644 index 0000000000000000000000000000000000000000..ea464c887a1ff129c28945d10771af48f3ce7e73 --- /dev/null +++ b/MrBigsock/Assets/Sprites/Player/Animations/idle/BigSock_idle.anim.meta @@ -0,0 +1,8 @@ +fileFormatVersion: 2 +guid: 00848c5d11c8da14c9d560112aef7536 +NativeFormatImporter: + externalObjects: {} + mainObjectFileID: 7400000 + userData: + assetBundleName: + assetBundleVariant: diff --git a/MrBigsock/Assets/Sprites/Player/Animations/walk.meta b/MrBigsock/Assets/Sprites/Player/Animations/walk.meta new file mode 100644 index 0000000000000000000000000000000000000000..93bab7887e58e248b0c5b329bd8738a49014433e --- /dev/null +++ b/MrBigsock/Assets/Sprites/Player/Animations/walk.meta @@ -0,0 +1,8 @@ +fileFormatVersion: 2 +guid: 68ee5279dea41b64e8e77892013a1087 +folderAsset: yes +DefaultImporter: + externalObjects: {} + userData: + assetBundleName: + assetBundleVariant: diff --git a/MrBigsock/Assets/Sprites/Player/Animations/walk/BigSock_Walk_Right.png b/MrBigsock/Assets/Sprites/Player/Animations/walk/BigSock_Walk_Right.png new file mode 100644 index 0000000000000000000000000000000000000000..f181a85cf1551a3745c7254f613f31d489f086e7 Binary files /dev/null and b/MrBigsock/Assets/Sprites/Player/Animations/walk/BigSock_Walk_Right.png differ diff --git a/MrBigsock/Assets/Sprites/Player/Animations/walk/BigSock_Walk_Right.png.meta b/MrBigsock/Assets/Sprites/Player/Animations/walk/BigSock_Walk_Right.png.meta new file mode 100644 index 0000000000000000000000000000000000000000..53baf56c608af0ec44e9c97b162d1afadc4f7f69 --- /dev/null +++ b/MrBigsock/Assets/Sprites/Player/Animations/walk/BigSock_Walk_Right.png.meta @@ -0,0 +1,235 @@ +fileFormatVersion: 2 +guid: 804a059e33d135d498c0cda23b933b36 +TextureImporter: + internalIDToNameTable: + - first: + 213: 8131826041949496069 + second: BigSock_Walk_Right_0 + - first: + 213: 5323494212205141862 + second: BigSock_Walk_Right_1 + - first: + 213: -3526397308662125963 + second: BigSock_Walk_Right_2 + - first: + 213: 743132843662613167 + second: BigSock_Walk_Right_3 + externalObjects: {} + serializedVersion: 12 + mipmaps: + mipMapMode: 0 + enableMipMap: 0 + sRGBTexture: 1 + linearTexture: 0 + fadeOut: 0 + borderMipMap: 0 + mipMapsPreserveCoverage: 0 + alphaTestReferenceValue: 0.5 + mipMapFadeDistanceStart: 1 + mipMapFadeDistanceEnd: 3 + bumpmap: + convertToNormalMap: 0 + externalNormalMap: 0 + heightScale: 0.25 + normalMapFilter: 0 + isReadable: 0 + streamingMipmaps: 0 + streamingMipmapsPriority: 0 + vTOnly: 0 + ignoreMasterTextureLimit: 0 + grayScaleToAlpha: 0 + generateCubemap: 6 + cubemapConvolution: 0 + seamlessCubemap: 0 + textureFormat: 1 + maxTextureSize: 2048 + textureSettings: + serializedVersion: 2 + filterMode: 0 + aniso: 1 + mipBias: 0 + wrapU: 1 + wrapV: 1 + wrapW: 1 + nPOTScale: 0 + lightmap: 0 + compressionQuality: 50 + spriteMode: 2 + spriteExtrude: 1 + spriteMeshType: 1 + alignment: 0 + spritePivot: {x: 0.5, y: 0.5} + spritePixelsToUnits: 16 + spriteBorder: {x: 0, y: 0, z: 0, w: 0} + spriteGenerateFallbackPhysicsShape: 1 + alphaUsage: 1 + alphaIsTransparency: 1 + spriteTessellationDetail: -1 + textureType: 8 + textureShape: 1 + singleChannelComponent: 0 + flipbookRows: 1 + flipbookColumns: 1 + maxTextureSizeSet: 0 + compressionQualitySet: 0 + textureFormatSet: 0 + ignorePngGamma: 0 + applyGammaDecoding: 0 + cookieLightType: 1 + platformSettings: + - serializedVersion: 3 + buildTarget: DefaultTexturePlatform + maxTextureSize: 2048 + resizeAlgorithm: 0 + textureFormat: -1 + textureCompression: 1 + compressionQuality: 50 + crunchedCompression: 0 + allowsAlphaSplitting: 0 + overridden: 0 + androidETC2FallbackOverride: 0 + forceMaximumCompressionQuality_BC6H_BC7: 0 + - serializedVersion: 3 + buildTarget: Standalone + maxTextureSize: 2048 + resizeAlgorithm: 0 + textureFormat: -1 + textureCompression: 1 + compressionQuality: 50 + crunchedCompression: 0 + allowsAlphaSplitting: 0 + overridden: 0 + androidETC2FallbackOverride: 0 + forceMaximumCompressionQuality_BC6H_BC7: 0 + - serializedVersion: 3 + buildTarget: WebGL + maxTextureSize: 2048 + resizeAlgorithm: 0 + textureFormat: -1 + textureCompression: 1 + compressionQuality: 50 + crunchedCompression: 0 + allowsAlphaSplitting: 0 + overridden: 0 + androidETC2FallbackOverride: 0 + forceMaximumCompressionQuality_BC6H_BC7: 0 + - serializedVersion: 3 + buildTarget: Server + maxTextureSize: 2048 + resizeAlgorithm: 0 + textureFormat: -1 + textureCompression: 1 + compressionQuality: 50 + crunchedCompression: 0 + allowsAlphaSplitting: 0 + overridden: 0 + androidETC2FallbackOverride: 0 + forceMaximumCompressionQuality_BC6H_BC7: 0 + spriteSheet: + serializedVersion: 2 + sprites: + - serializedVersion: 2 + name: BigSock_Walk_Right_0 + rect: + serializedVersion: 2 + x: 0 + y: 0 + width: 32 + height: 32 + alignment: 0 + pivot: {x: 0, y: 0} + border: {x: 0, y: 0, z: 0, w: 0} + outline: [] + physicsShape: [] + tessellationDetail: 0 + bones: [] + spriteID: 503abad63bc0ad070800000000000000 + internalID: 8131826041949496069 + vertices: [] + indices: + edges: [] + weights: [] + - serializedVersion: 2 + name: BigSock_Walk_Right_1 + rect: + serializedVersion: 2 + x: 32 + y: 0 + width: 32 + height: 32 + alignment: 0 + pivot: {x: 0, y: 0} + border: {x: 0, y: 0, z: 0, w: 0} + outline: [] + physicsShape: [] + tessellationDetail: 0 + bones: [] + spriteID: 66fd55ae7c9d0e940800000000000000 + internalID: 5323494212205141862 + vertices: [] + indices: + edges: [] + weights: [] + - serializedVersion: 2 + name: BigSock_Walk_Right_2 + rect: + serializedVersion: 2 + x: 64 + y: 0 + width: 32 + height: 32 + alignment: 0 + pivot: {x: 0, y: 0} + border: {x: 0, y: 0, z: 0, w: 0} + outline: [] + physicsShape: [] + tessellationDetail: 0 + bones: [] + spriteID: 57aec48d458bf0fc0800000000000000 + internalID: -3526397308662125963 + vertices: [] + indices: + edges: [] + weights: [] + - serializedVersion: 2 + name: BigSock_Walk_Right_3 + rect: + serializedVersion: 2 + x: 96 + y: 0 + width: 32 + height: 32 + alignment: 0 + pivot: {x: 0, y: 0} + border: {x: 0, y: 0, z: 0, w: 0} + outline: [] + physicsShape: [] + tessellationDetail: 0 + bones: [] + spriteID: faa9f6f4263205a00800000000000000 + internalID: 743132843662613167 + vertices: [] + indices: + edges: [] + weights: [] + outline: [] + physicsShape: [] + bones: [] + spriteID: 5e97eb03825dee720800000000000000 + internalID: 0 + vertices: [] + indices: + edges: [] + weights: [] + secondaryTextures: [] + nameFileIdTable: + BigSock_Walk_Right_3: 743132843662613167 + BigSock_Walk_Right_2: -3526397308662125963 + BigSock_Walk_Right_1: 5323494212205141862 + BigSock_Walk_Right_0: 8131826041949496069 + spritePackingTag: + pSDRemoveMatte: 0 + pSDShowRemoveMatteOption: 0 + userData: + assetBundleName: + assetBundleVariant: diff --git a/MrBigsock/Assets/Sprites/Player/Animations/walk/Bigsock_walk.anim b/MrBigsock/Assets/Sprites/Player/Animations/walk/Bigsock_walk.anim new file mode 100644 index 0000000000000000000000000000000000000000..81feaa360f3e04cd9c6def58511a7dfaebd9909f --- /dev/null +++ b/MrBigsock/Assets/Sprites/Player/Animations/walk/Bigsock_walk.anim @@ -0,0 +1,80 @@ +%YAML 1.1 +%TAG !u! tag:unity3d.com,2011: +--- !u!74 &7400000 +AnimationClip: + m_ObjectHideFlags: 0 + m_CorrespondingSourceObject: {fileID: 0} + m_PrefabInstance: {fileID: 0} + m_PrefabAsset: {fileID: 0} + m_Name: Bigsock_walk + serializedVersion: 6 + m_Legacy: 0 + m_Compressed: 0 + m_UseHighQualityCurve: 1 + m_RotationCurves: [] + m_CompressedRotationCurves: [] + m_EulerCurves: [] + m_PositionCurves: [] + m_ScaleCurves: [] + m_FloatCurves: [] + m_PPtrCurves: + - curve: + - time: 0 + value: {fileID: 8131826041949496069, guid: 804a059e33d135d498c0cda23b933b36, type: 3} + - time: 0.16666667 + value: {fileID: 5323494212205141862, guid: 804a059e33d135d498c0cda23b933b36, type: 3} + - time: 0.33333334 + value: {fileID: -3526397308662125963, guid: 804a059e33d135d498c0cda23b933b36, type: 3} + - time: 0.5 + value: {fileID: 743132843662613167, guid: 804a059e33d135d498c0cda23b933b36, type: 3} + - time: 0.6666667 + value: {fileID: 743132843662613167, guid: 804a059e33d135d498c0cda23b933b36, type: 3} + attribute: m_Sprite + path: + classID: 212 + script: {fileID: 0} + m_SampleRate: 60 + m_WrapMode: 0 + m_Bounds: + m_Center: {x: 0, y: 0, z: 0} + m_Extent: {x: 0, y: 0, z: 0} + m_ClipBindingConstant: + genericBindings: + - serializedVersion: 2 + path: 0 + attribute: 0 + script: {fileID: 0} + typeID: 212 + customType: 23 + isPPtrCurve: 1 + pptrCurveMapping: + - {fileID: 8131826041949496069, guid: 804a059e33d135d498c0cda23b933b36, type: 3} + - {fileID: 5323494212205141862, guid: 804a059e33d135d498c0cda23b933b36, type: 3} + - {fileID: -3526397308662125963, guid: 804a059e33d135d498c0cda23b933b36, type: 3} + - {fileID: 743132843662613167, guid: 804a059e33d135d498c0cda23b933b36, type: 3} + - {fileID: 743132843662613167, guid: 804a059e33d135d498c0cda23b933b36, type: 3} + m_AnimationClipSettings: + serializedVersion: 2 + m_AdditiveReferencePoseClip: {fileID: 0} + m_AdditiveReferencePoseTime: 0 + m_StartTime: 0 + m_StopTime: 0.68333334 + m_OrientationOffsetY: 0 + m_Level: 0 + m_CycleOffset: 0 + m_HasAdditiveReferencePose: 0 + m_LoopTime: 1 + m_LoopBlend: 0 + m_LoopBlendOrientation: 0 + m_LoopBlendPositionY: 0 + m_LoopBlendPositionXZ: 0 + m_KeepOriginalOrientation: 0 + m_KeepOriginalPositionY: 1 + m_KeepOriginalPositionXZ: 0 + m_HeightFromFeet: 0 + m_Mirror: 0 + m_EditorCurves: [] + m_EulerEditorCurves: [] + m_HasGenericRootTransform: 0 + m_HasMotionFloatCurves: 0 + m_Events: [] diff --git a/MrBigsock/Assets/Sprites/Player/Animations/walk/Bigsock_walk.anim.meta b/MrBigsock/Assets/Sprites/Player/Animations/walk/Bigsock_walk.anim.meta new file mode 100644 index 0000000000000000000000000000000000000000..a29b337097389435f4dfd2757e1c00e4fd554285 --- /dev/null +++ b/MrBigsock/Assets/Sprites/Player/Animations/walk/Bigsock_walk.anim.meta @@ -0,0 +1,8 @@ +fileFormatVersion: 2 +guid: 574950c5abe3d744a81c8a02f6c66e70 +NativeFormatImporter: + externalObjects: {} + mainObjectFileID: 7400000 + userData: + assetBundleName: + assetBundleVariant: diff --git a/MrBigsock/Assets/Sprites/Player/BigSock.meta b/MrBigsock/Assets/Sprites/Player/BigSock.meta new file mode 100644 index 0000000000000000000000000000000000000000..32b324741008b4dbe23e6d91f024a8f2304e6de9 --- /dev/null +++ b/MrBigsock/Assets/Sprites/Player/BigSock.meta @@ -0,0 +1,8 @@ +fileFormatVersion: 2 +guid: d4bdc9a1751165f44abd660b036f2e48 +folderAsset: yes +DefaultImporter: + externalObjects: {} + userData: + assetBundleName: + assetBundleVariant: diff --git a/MrBigsock/Assets/Sprites/Player/BigSock/PlayerInputActions.inputactions b/MrBigsock/Assets/Sprites/Player/BigSock/PlayerInputActions.inputactions new file mode 100644 index 0000000000000000000000000000000000000000..9408158e367b948d84ed56c80f20c728b8cdb49e --- /dev/null +++ b/MrBigsock/Assets/Sprites/Player/BigSock/PlayerInputActions.inputactions @@ -0,0 +1,838 @@ +{ + "name": "PlayerInputActions", + "maps": [ + { + "name": "Player", + "id": "0174e2a0-4bfe-4434-aced-415f3c2762d4", + "actions": [ + { + "name": "Move", + "type": "Value", + "id": "7b31de1e-b184-45d7-98f6-e6bc2e425999", + "expectedControlType": "Vector2", + "processors": "", + "interactions": "", + "initialStateCheck": true + }, + { + "name": "Look", + "type": "Value", + "id": "0c1f49d2-2106-4221-be7f-e14998b78632", + "expectedControlType": "Vector2", + "processors": "", + "interactions": "", + "initialStateCheck": true + }, + { + "name": "Fire", + "type": "Button", + "id": "81e78cc4-6a7c-4f89-8749-e8dc9d6a3d36", + "expectedControlType": "Button", + "processors": "", + "interactions": "", + "initialStateCheck": false + } + ], + "bindings": [ + { + "name": "", + "id": "978bfe49-cc26-4a3d-ab7b-7d7a29327403", + "path": "<Gamepad>/leftStick", + "interactions": "", + "processors": "", + "groups": ";Gamepad", + "action": "Move", + "isComposite": false, + "isPartOfComposite": false + }, + { + "name": "WASD", + "id": "00ca640b-d935-4593-8157-c05846ea39b3", + "path": "Dpad", + "interactions": "", + "processors": "", + "groups": "", + "action": "Move", + "isComposite": true, + "isPartOfComposite": false + }, + { + "name": "up", + "id": "e2062cb9-1b15-46a2-838c-2f8d72a0bdd9", + "path": "<Keyboard>/w", + "interactions": "", + "processors": "", + "groups": ";Keyboard&Mouse", + "action": "Move", + "isComposite": false, + "isPartOfComposite": true + }, + { + "name": "up", + "id": "8180e8bd-4097-4f4e-ab88-4523101a6ce9", + "path": "<Keyboard>/upArrow", + "interactions": "", + "processors": "", + "groups": ";Keyboard&Mouse", + "action": "Move", + "isComposite": false, + "isPartOfComposite": true + }, + { + "name": "down", + "id": "320bffee-a40b-4347-ac70-c210eb8bc73a", + "path": "<Keyboard>/s", + "interactions": "", + "processors": "", + "groups": ";Keyboard&Mouse", + "action": "Move", + "isComposite": false, + "isPartOfComposite": true + }, + { + "name": "down", + "id": "1c5327b5-f71c-4f60-99c7-4e737386f1d1", + "path": "<Keyboard>/downArrow", + "interactions": "", + "processors": "", + "groups": ";Keyboard&Mouse", + "action": "Move", + "isComposite": false, + "isPartOfComposite": true + }, + { + "name": "left", + "id": "d2581a9b-1d11-4566-b27d-b92aff5fabbc", + "path": "<Keyboard>/a", + "interactions": "", + "processors": "", + "groups": ";Keyboard&Mouse", + "action": "Move", + "isComposite": false, + "isPartOfComposite": true + }, + { + "name": "left", + "id": "2e46982e-44cc-431b-9f0b-c11910bf467a", + "path": "<Keyboard>/leftArrow", + "interactions": "", + "processors": "", + "groups": ";Keyboard&Mouse", + "action": "Move", + "isComposite": false, + "isPartOfComposite": true + }, + { + "name": "right", + "id": "fcfe95b8-67b9-4526-84b5-5d0bc98d6400", + "path": "<Keyboard>/d", + "interactions": "", + "processors": "", + "groups": ";Keyboard&Mouse", + "action": "Move", + "isComposite": false, + "isPartOfComposite": true + }, + { + "name": "right", + "id": "77bff152-3580-4b21-b6de-dcd0c7e41164", + "path": "<Keyboard>/rightArrow", + "interactions": "", + "processors": "", + "groups": ";Keyboard&Mouse", + "action": "Move", + "isComposite": false, + "isPartOfComposite": true + }, + { + "name": "", + "id": "1635d3fe-58b6-4ba9-a4e2-f4b964f6b5c8", + "path": "<XRController>/{Primary2DAxis}", + "interactions": "", + "processors": "", + "groups": "XR", + "action": "Move", + "isComposite": false, + "isPartOfComposite": false + }, + { + "name": "", + "id": "3ea4d645-4504-4529-b061-ab81934c3752", + "path": "<Joystick>/stick", + "interactions": "", + "processors": "", + "groups": "Joystick", + "action": "Move", + "isComposite": false, + "isPartOfComposite": false + }, + { + "name": "", + "id": "c1f7a91b-d0fd-4a62-997e-7fb9b69bf235", + "path": "<Gamepad>/rightStick", + "interactions": "", + "processors": "", + "groups": ";Gamepad", + "action": "Look", + "isComposite": false, + "isPartOfComposite": false + }, + { + "name": "", + "id": "8c8e490b-c610-4785-884f-f04217b23ca4", + "path": "<Pointer>/delta", + "interactions": "", + "processors": "", + "groups": ";Keyboard&Mouse;Touch", + "action": "Look", + "isComposite": false, + "isPartOfComposite": false + }, + { + "name": "", + "id": "3e5f5442-8668-4b27-a940-df99bad7e831", + "path": "<Joystick>/{Hatswitch}", + "interactions": "", + "processors": "", + "groups": "Joystick", + "action": "Look", + "isComposite": false, + "isPartOfComposite": false + }, + { + "name": "", + "id": "143bb1cd-cc10-4eca-a2f0-a3664166fe91", + "path": "<Gamepad>/rightTrigger", + "interactions": "", + "processors": "", + "groups": ";Gamepad", + "action": "Fire", + "isComposite": false, + "isPartOfComposite": false + }, + { + "name": "", + "id": "05f6913d-c316-48b2-a6bb-e225f14c7960", + "path": "<Mouse>/leftButton", + "interactions": "", + "processors": "", + "groups": ";Keyboard&Mouse", + "action": "Fire", + "isComposite": false, + "isPartOfComposite": false + }, + { + "name": "", + "id": "886e731e-7071-4ae4-95c0-e61739dad6fd", + "path": "<Touchscreen>/primaryTouch/tap", + "interactions": "", + "processors": "", + "groups": ";Touch", + "action": "Fire", + "isComposite": false, + "isPartOfComposite": false + }, + { + "name": "", + "id": "ee3d0cd2-254e-47a7-a8cb-bc94d9658c54", + "path": "<Joystick>/trigger", + "interactions": "", + "processors": "", + "groups": "Joystick", + "action": "Fire", + "isComposite": false, + "isPartOfComposite": false + }, + { + "name": "", + "id": "8255d333-5683-4943-a58a-ccb207ff1dce", + "path": "<XRController>/{PrimaryAction}", + "interactions": "", + "processors": "", + "groups": "XR", + "action": "Fire", + "isComposite": false, + "isPartOfComposite": false + } + ] + }, + { + "name": "UI", + "id": "43203311-9f24-4e6e-956a-639f57f1ae2c", + "actions": [ + { + "name": "Navigate", + "type": "PassThrough", + "id": "a3381194-0354-40bf-a5f5-6a32a80ed7c4", + "expectedControlType": "Vector2", + "processors": "", + "interactions": "", + "initialStateCheck": false + }, + { + "name": "Submit", + "type": "Button", + "id": "46eadbba-30c8-481b-9a5a-7d8350e4863d", + "expectedControlType": "Button", + "processors": "", + "interactions": "", + "initialStateCheck": false + }, + { + "name": "Cancel", + "type": "Button", + "id": "e726802b-967c-42a9-b98d-60d98039485e", + "expectedControlType": "Button", + "processors": "", + "interactions": "", + "initialStateCheck": false + }, + { + "name": "Point", + "type": "PassThrough", + "id": "a71f9f7f-dcdd-4bc1-b2e7-d4c363d73600", + "expectedControlType": "Vector2", + "processors": "", + "interactions": "", + "initialStateCheck": true + }, + { + "name": "Click", + "type": "PassThrough", + "id": "cb89aa30-fbd1-4f3b-af07-70e5b839d952", + "expectedControlType": "Button", + "processors": "", + "interactions": "", + "initialStateCheck": true + }, + { + "name": "ScrollWheel", + "type": "PassThrough", + "id": "07ea199d-f520-4074-8499-e722a8656bd4", + "expectedControlType": "Vector2", + "processors": "", + "interactions": "", + "initialStateCheck": false + }, + { + "name": "MiddleClick", + "type": "PassThrough", + "id": "c8c14e10-6666-490f-ae4c-2cc911748042", + "expectedControlType": "Button", + "processors": "", + "interactions": "", + "initialStateCheck": false + }, + { + "name": "RightClick", + "type": "PassThrough", + "id": "92276877-5a00-449f-ac05-4866c559f505", + "expectedControlType": "Button", + "processors": "", + "interactions": "", + "initialStateCheck": false + }, + { + "name": "TrackedDevicePosition", + "type": "PassThrough", + "id": "eee2c4f4-99da-4cbf-8e7e-e178daad9c2c", + "expectedControlType": "Vector3", + "processors": "", + "interactions": "", + "initialStateCheck": false + }, + { + "name": "TrackedDeviceOrientation", + "type": "PassThrough", + "id": "abd70394-0b83-42c1-ad25-63401f1d8e87", + "expectedControlType": "Quaternion", + "processors": "", + "interactions": "", + "initialStateCheck": false + } + ], + "bindings": [ + { + "name": "Gamepad", + "id": "809f371f-c5e2-4e7a-83a1-d867598f40dd", + "path": "2DVector", + "interactions": "", + "processors": "", + "groups": "", + "action": "Navigate", + "isComposite": true, + "isPartOfComposite": false + }, + { + "name": "up", + "id": "14a5d6e8-4aaf-4119-a9ef-34b8c2c548bf", + "path": "<Gamepad>/leftStick/up", + "interactions": "", + "processors": "", + "groups": ";Gamepad", + "action": "Navigate", + "isComposite": false, + "isPartOfComposite": true + }, + { + "name": "up", + "id": "9144cbe6-05e1-4687-a6d7-24f99d23dd81", + "path": "<Gamepad>/rightStick/up", + "interactions": "", + "processors": "", + "groups": ";Gamepad", + "action": "Navigate", + "isComposite": false, + "isPartOfComposite": true + }, + { + "name": "down", + "id": "2db08d65-c5fb-421b-983f-c71163608d67", + "path": "<Gamepad>/leftStick/down", + "interactions": "", + "processors": "", + "groups": ";Gamepad", + "action": "Navigate", + "isComposite": false, + "isPartOfComposite": true + }, + { + "name": "down", + "id": "58748904-2ea9-4a80-8579-b500e6a76df8", + "path": "<Gamepad>/rightStick/down", + "interactions": "", + "processors": "", + "groups": ";Gamepad", + "action": "Navigate", + "isComposite": false, + "isPartOfComposite": true + }, + { + "name": "left", + "id": "8ba04515-75aa-45de-966d-393d9bbd1c14", + "path": "<Gamepad>/leftStick/left", + "interactions": "", + "processors": "", + "groups": ";Gamepad", + "action": "Navigate", + "isComposite": false, + "isPartOfComposite": true + }, + { + "name": "left", + "id": "712e721c-bdfb-4b23-a86c-a0d9fcfea921", + "path": "<Gamepad>/rightStick/left", + "interactions": "", + "processors": "", + "groups": ";Gamepad", + "action": "Navigate", + "isComposite": false, + "isPartOfComposite": true + }, + { + "name": "right", + "id": "fcd248ae-a788-4676-a12e-f4d81205600b", + "path": "<Gamepad>/leftStick/right", + "interactions": "", + "processors": "", + "groups": ";Gamepad", + "action": "Navigate", + "isComposite": false, + "isPartOfComposite": true + }, + { + "name": "right", + "id": "1f04d9bc-c50b-41a1-bfcc-afb75475ec20", + "path": "<Gamepad>/rightStick/right", + "interactions": "", + "processors": "", + "groups": ";Gamepad", + "action": "Navigate", + "isComposite": false, + "isPartOfComposite": true + }, + { + "name": "", + "id": "fb8277d4-c5cd-4663-9dc7-ee3f0b506d90", + "path": "<Gamepad>/dpad", + "interactions": "", + "processors": "", + "groups": ";Gamepad", + "action": "Navigate", + "isComposite": false, + "isPartOfComposite": false + }, + { + "name": "Joystick", + "id": "e25d9774-381c-4a61-b47c-7b6b299ad9f9", + "path": "2DVector", + "interactions": "", + "processors": "", + "groups": "", + "action": "Navigate", + "isComposite": true, + "isPartOfComposite": false + }, + { + "name": "up", + "id": "3db53b26-6601-41be-9887-63ac74e79d19", + "path": "<Joystick>/stick/up", + "interactions": "", + "processors": "", + "groups": "Joystick", + "action": "Navigate", + "isComposite": false, + "isPartOfComposite": true + }, + { + "name": "down", + "id": "0cb3e13e-3d90-4178-8ae6-d9c5501d653f", + "path": "<Joystick>/stick/down", + "interactions": "", + "processors": "", + "groups": "Joystick", + "action": "Navigate", + "isComposite": false, + "isPartOfComposite": true + }, + { + "name": "left", + "id": "0392d399-f6dd-4c82-8062-c1e9c0d34835", + "path": "<Joystick>/stick/left", + "interactions": "", + "processors": "", + "groups": "Joystick", + "action": "Navigate", + "isComposite": false, + "isPartOfComposite": true + }, + { + "name": "right", + "id": "942a66d9-d42f-43d6-8d70-ecb4ba5363bc", + "path": "<Joystick>/stick/right", + "interactions": "", + "processors": "", + "groups": "Joystick", + "action": "Navigate", + "isComposite": false, + "isPartOfComposite": true + }, + { + "name": "Keyboard", + "id": "ff527021-f211-4c02-933e-5976594c46ed", + "path": "2DVector", + "interactions": "", + "processors": "", + "groups": "", + "action": "Navigate", + "isComposite": true, + "isPartOfComposite": false + }, + { + "name": "up", + "id": "563fbfdd-0f09-408d-aa75-8642c4f08ef0", + "path": "<Keyboard>/w", + "interactions": "", + "processors": "", + "groups": "Keyboard&Mouse", + "action": "Navigate", + "isComposite": false, + "isPartOfComposite": true + }, + { + "name": "up", + "id": "eb480147-c587-4a33-85ed-eb0ab9942c43", + "path": "<Keyboard>/upArrow", + "interactions": "", + "processors": "", + "groups": "Keyboard&Mouse", + "action": "Navigate", + "isComposite": false, + "isPartOfComposite": true + }, + { + "name": "down", + "id": "2bf42165-60bc-42ca-8072-8c13ab40239b", + "path": "<Keyboard>/s", + "interactions": "", + "processors": "", + "groups": "Keyboard&Mouse", + "action": "Navigate", + "isComposite": false, + "isPartOfComposite": true + }, + { + "name": "down", + "id": "85d264ad-e0a0-4565-b7ff-1a37edde51ac", + "path": "<Keyboard>/downArrow", + "interactions": "", + "processors": "", + "groups": "Keyboard&Mouse", + "action": "Navigate", + "isComposite": false, + "isPartOfComposite": true + }, + { + "name": "left", + "id": "74214943-c580-44e4-98eb-ad7eebe17902", + "path": "<Keyboard>/a", + "interactions": "", + "processors": "", + "groups": "Keyboard&Mouse", + "action": "Navigate", + "isComposite": false, + "isPartOfComposite": true + }, + { + "name": "left", + "id": "cea9b045-a000-445b-95b8-0c171af70a3b", + "path": "<Keyboard>/leftArrow", + "interactions": "", + "processors": "", + "groups": "Keyboard&Mouse", + "action": "Navigate", + "isComposite": false, + "isPartOfComposite": true + }, + { + "name": "right", + "id": "8607c725-d935-4808-84b1-8354e29bab63", + "path": "<Keyboard>/d", + "interactions": "", + "processors": "", + "groups": "Keyboard&Mouse", + "action": "Navigate", + "isComposite": false, + "isPartOfComposite": true + }, + { + "name": "right", + "id": "4cda81dc-9edd-4e03-9d7c-a71a14345d0b", + "path": "<Keyboard>/rightArrow", + "interactions": "", + "processors": "", + "groups": "Keyboard&Mouse", + "action": "Navigate", + "isComposite": false, + "isPartOfComposite": true + }, + { + "name": "", + "id": "9e92bb26-7e3b-4ec4-b06b-3c8f8e498ddc", + "path": "*/{Submit}", + "interactions": "", + "processors": "", + "groups": "Keyboard&Mouse;Gamepad;Touch;Joystick;XR", + "action": "Submit", + "isComposite": false, + "isPartOfComposite": false + }, + { + "name": "", + "id": "82627dcc-3b13-4ba9-841d-e4b746d6553e", + "path": "*/{Cancel}", + "interactions": "", + "processors": "", + "groups": "Keyboard&Mouse;Gamepad;Touch;Joystick;XR", + "action": "Cancel", + "isComposite": false, + "isPartOfComposite": false + }, + { + "name": "", + "id": "c52c8e0b-8179-41d3-b8a1-d149033bbe86", + "path": "<Mouse>/position", + "interactions": "", + "processors": "", + "groups": "Keyboard&Mouse", + "action": "Point", + "isComposite": false, + "isPartOfComposite": false + }, + { + "name": "", + "id": "e1394cbc-336e-44ce-9ea8-6007ed6193f7", + "path": "<Pen>/position", + "interactions": "", + "processors": "", + "groups": "Keyboard&Mouse", + "action": "Point", + "isComposite": false, + "isPartOfComposite": false + }, + { + "name": "", + "id": "5693e57a-238a-46ed-b5ae-e64e6e574302", + "path": "<Touchscreen>/touch*/position", + "interactions": "", + "processors": "", + "groups": "Touch", + "action": "Point", + "isComposite": false, + "isPartOfComposite": false + }, + { + "name": "", + "id": "4faf7dc9-b979-4210-aa8c-e808e1ef89f5", + "path": "<Mouse>/leftButton", + "interactions": "", + "processors": "", + "groups": ";Keyboard&Mouse", + "action": "Click", + "isComposite": false, + "isPartOfComposite": false + }, + { + "name": "", + "id": "8d66d5ba-88d7-48e6-b1cd-198bbfef7ace", + "path": "<Pen>/tip", + "interactions": "", + "processors": "", + "groups": ";Keyboard&Mouse", + "action": "Click", + "isComposite": false, + "isPartOfComposite": false + }, + { + "name": "", + "id": "47c2a644-3ebc-4dae-a106-589b7ca75b59", + "path": "<Touchscreen>/touch*/press", + "interactions": "", + "processors": "", + "groups": "Touch", + "action": "Click", + "isComposite": false, + "isPartOfComposite": false + }, + { + "name": "", + "id": "bb9e6b34-44bf-4381-ac63-5aa15d19f677", + "path": "<XRController>/trigger", + "interactions": "", + "processors": "", + "groups": "XR", + "action": "Click", + "isComposite": false, + "isPartOfComposite": false + }, + { + "name": "", + "id": "38c99815-14ea-4617-8627-164d27641299", + "path": "<Mouse>/scroll", + "interactions": "", + "processors": "", + "groups": ";Keyboard&Mouse", + "action": "ScrollWheel", + "isComposite": false, + "isPartOfComposite": false + }, + { + "name": "", + "id": "24066f69-da47-44f3-a07e-0015fb02eb2e", + "path": "<Mouse>/middleButton", + "interactions": "", + "processors": "", + "groups": ";Keyboard&Mouse", + "action": "MiddleClick", + "isComposite": false, + "isPartOfComposite": false + }, + { + "name": "", + "id": "4c191405-5738-4d4b-a523-c6a301dbf754", + "path": "<Mouse>/rightButton", + "interactions": "", + "processors": "", + "groups": ";Keyboard&Mouse", + "action": "RightClick", + "isComposite": false, + "isPartOfComposite": false + }, + { + "name": "", + "id": "7236c0d9-6ca3-47cf-a6ee-a97f5b59ea77", + "path": "<XRController>/devicePosition", + "interactions": "", + "processors": "", + "groups": "XR", + "action": "TrackedDevicePosition", + "isComposite": false, + "isPartOfComposite": false + }, + { + "name": "", + "id": "23e01e3a-f935-4948-8d8b-9bcac77714fb", + "path": "<XRController>/deviceRotation", + "interactions": "", + "processors": "", + "groups": "XR", + "action": "TrackedDeviceOrientation", + "isComposite": false, + "isPartOfComposite": false + } + ] + } + ], + "controlSchemes": [ + { + "name": "Keyboard&Mouse", + "bindingGroup": "Keyboard&Mouse", + "devices": [ + { + "devicePath": "<Keyboard>", + "isOptional": false, + "isOR": false + }, + { + "devicePath": "<Mouse>", + "isOptional": false, + "isOR": false + } + ] + }, + { + "name": "Gamepad", + "bindingGroup": "Gamepad", + "devices": [ + { + "devicePath": "<Gamepad>", + "isOptional": false, + "isOR": false + } + ] + }, + { + "name": "Touch", + "bindingGroup": "Touch", + "devices": [ + { + "devicePath": "<Touchscreen>", + "isOptional": false, + "isOR": false + } + ] + }, + { + "name": "Joystick", + "bindingGroup": "Joystick", + "devices": [ + { + "devicePath": "<Joystick>", + "isOptional": false, + "isOR": false + } + ] + }, + { + "name": "XR", + "bindingGroup": "XR", + "devices": [ + { + "devicePath": "<XRController>", + "isOptional": false, + "isOR": false + } + ] + } + ] +} \ No newline at end of file diff --git a/MrBigsock/Assets/Sprites/Player/BigSock/PlayerInputActions.inputactions.meta b/MrBigsock/Assets/Sprites/Player/BigSock/PlayerInputActions.inputactions.meta new file mode 100644 index 0000000000000000000000000000000000000000..9010dd8fc75f562c2ce32845074edbd077228efa --- /dev/null +++ b/MrBigsock/Assets/Sprites/Player/BigSock/PlayerInputActions.inputactions.meta @@ -0,0 +1,14 @@ +fileFormatVersion: 2 +guid: cf470a27421e24644b34218d18002b82 +ScriptedImporter: + internalIDToNameTable: [] + externalObjects: {} + serializedVersion: 2 + userData: + assetBundleName: + assetBundleVariant: + script: {fileID: 11500000, guid: 8404be70184654265930450def6a9037, type: 3} + generateWrapperCode: 0 + wrapperCodePath: + wrapperClassName: + wrapperCodeNamespace: diff --git a/MrBigsock/Assets/Tilemaps/Dungeon.meta b/MrBigsock/Assets/Tilemaps/Dungeon.meta new file mode 100644 index 0000000000000000000000000000000000000000..98a597643b078e0bccb5a9370ebb5d2d60dd9ab6 --- /dev/null +++ b/MrBigsock/Assets/Tilemaps/Dungeon.meta @@ -0,0 +1,8 @@ +fileFormatVersion: 2 +guid: e267343cd5d8f6745ac27a613cfb1bc2 +folderAsset: yes +DefaultImporter: + externalObjects: {} + userData: + assetBundleName: + assetBundleVariant: diff --git a/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon.png b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon.png new file mode 100644 index 0000000000000000000000000000000000000000..39d1293aa42df5f27bfb4f09da8c363af157b17f Binary files /dev/null and b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon.png differ diff --git a/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon.png.meta b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon.png.meta new file mode 100644 index 0000000000000000000000000000000000000000..89add8b39d0c8ae5d35045172766b19098173265 --- /dev/null +++ b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon.png.meta @@ -0,0 +1,2125 @@ +fileFormatVersion: 2 +guid: 0a806eb0d25e11c48b5dee57fb9339b5 +TextureImporter: + internalIDToNameTable: [] + externalObjects: {} + serializedVersion: 12 + mipmaps: + mipMapMode: 0 + enableMipMap: 0 + sRGBTexture: 1 + linearTexture: 0 + fadeOut: 0 + borderMipMap: 0 + mipMapsPreserveCoverage: 0 + alphaTestReferenceValue: 0.5 + mipMapFadeDistanceStart: 1 + mipMapFadeDistanceEnd: 3 + bumpmap: + convertToNormalMap: 0 + externalNormalMap: 0 + heightScale: 0.25 + normalMapFilter: 0 + isReadable: 0 + streamingMipmaps: 0 + streamingMipmapsPriority: 0 + vTOnly: 0 + ignoreMasterTextureLimit: 0 + grayScaleToAlpha: 0 + generateCubemap: 6 + cubemapConvolution: 0 + seamlessCubemap: 0 + textureFormat: 1 + maxTextureSize: 2048 + textureSettings: + serializedVersion: 2 + filterMode: 0 + aniso: 1 + mipBias: 0 + wrapU: 1 + wrapV: 1 + wrapW: 1 + nPOTScale: 0 + lightmap: 0 + compressionQuality: 50 + spriteMode: 2 + spriteExtrude: 1 + spriteMeshType: 1 + alignment: 0 + spritePivot: {x: 0.5, y: 0.5} + spritePixelsToUnits: 16 + spriteBorder: {x: 0, y: 0, z: 0, w: 0} + spriteGenerateFallbackPhysicsShape: 1 + alphaUsage: 1 + alphaIsTransparency: 1 + spriteTessellationDetail: -1 + textureType: 8 + textureShape: 1 + singleChannelComponent: 0 + flipbookRows: 1 + flipbookColumns: 1 + maxTextureSizeSet: 0 + compressionQualitySet: 0 + textureFormatSet: 0 + ignorePngGamma: 0 + applyGammaDecoding: 0 + cookieLightType: 0 + platformSettings: + - serializedVersion: 3 + buildTarget: DefaultTexturePlatform + maxTextureSize: 2048 + resizeAlgorithm: 0 + textureFormat: -1 + textureCompression: 1 + compressionQuality: 50 + crunchedCompression: 0 + allowsAlphaSplitting: 0 + overridden: 0 + androidETC2FallbackOverride: 0 + forceMaximumCompressionQuality_BC6H_BC7: 0 + - serializedVersion: 3 + buildTarget: Standalone + maxTextureSize: 2048 + resizeAlgorithm: 0 + textureFormat: -1 + textureCompression: 1 + compressionQuality: 50 + crunchedCompression: 0 + allowsAlphaSplitting: 0 + overridden: 0 + androidETC2FallbackOverride: 0 + forceMaximumCompressionQuality_BC6H_BC7: 0 + - serializedVersion: 3 + buildTarget: Server + maxTextureSize: 2048 + resizeAlgorithm: 0 + textureFormat: -1 + textureCompression: 1 + compressionQuality: 50 + crunchedCompression: 0 + allowsAlphaSplitting: 0 + overridden: 0 + androidETC2FallbackOverride: 0 + forceMaximumCompressionQuality_BC6H_BC7: 0 + spriteSheet: + serializedVersion: 2 + sprites: + - serializedVersion: 2 + name: Dungeon_0 + rect: + serializedVersion: 2 + x: 16 + y: 240 + width: 16 + height: 16 + alignment: 0 + pivot: {x: 0, y: 0} + border: {x: 0, y: 0, z: 0, w: 0} + outline: [] + physicsShape: [] + tessellationDetail: 0 + bones: [] + spriteID: bc0a1787ed5cb8342bd04f794cfb2a1a + internalID: -509989529 + vertices: [] + indices: + edges: [] + weights: [] + - serializedVersion: 2 + name: Dungeon_1 + rect: + serializedVersion: 2 + x: 32 + y: 240 + width: 16 + height: 16 + alignment: 0 + pivot: {x: 0, y: 0} + border: {x: 0, y: 0, z: 0, w: 0} + outline: [] + physicsShape: [] + tessellationDetail: 0 + bones: [] + spriteID: 68e3a54768e5ea045ac1c2dff3cab4e0 + internalID: -1085996522 + vertices: [] + indices: + edges: [] + weights: [] + - serializedVersion: 2 + name: Dungeon_2 + rect: + serializedVersion: 2 + x: 48 + y: 240 + width: 16 + height: 16 + alignment: 0 + pivot: {x: 0, y: 0} + border: {x: 0, y: 0, z: 0, w: 0} + outline: [] + physicsShape: [] + tessellationDetail: 0 + bones: [] + spriteID: f6fab432826c0d046a83e5a950ae3166 + internalID: 164431376 + vertices: [] + indices: + edges: [] + weights: [] + - serializedVersion: 2 + name: Dungeon_3 + rect: + serializedVersion: 2 + x: 64 + y: 240 + width: 16 + height: 16 + alignment: 0 + pivot: {x: 0, y: 0} + border: {x: 0, y: 0, z: 0, w: 0} + outline: [] + physicsShape: [] + tessellationDetail: 0 + bones: [] + spriteID: 623c94b859649554d8386dacf94718e8 + internalID: 326241045 + vertices: [] + indices: + edges: [] + weights: [] + - serializedVersion: 2 + name: Dungeon_4 + rect: + serializedVersion: 2 + x: 80 + y: 240 + width: 16 + height: 16 + alignment: 0 + pivot: {x: 0, y: 0} + border: {x: 0, y: 0, z: 0, w: 0} + outline: [] + physicsShape: [] + tessellationDetail: 0 + bones: [] + spriteID: d6264dc7f9bdb8e4791d6f6ee4a23b9b + internalID: -345571141 + vertices: [] + indices: + edges: [] + weights: [] + - serializedVersion: 2 + name: Dungeon_5 + rect: + serializedVersion: 2 + x: 96 + y: 240 + width: 16 + height: 16 + alignment: 0 + pivot: {x: 0, y: 0} + border: {x: 0, y: 0, z: 0, w: 0} + outline: [] + physicsShape: [] + tessellationDetail: 0 + bones: [] + spriteID: 910421940f3923c47a4d424416276e8c + internalID: 2094817791 + vertices: [] + indices: + edges: [] + weights: [] + - serializedVersion: 2 + name: Dungeon_6 + rect: + serializedVersion: 2 + x: 16 + y: 224 + width: 16 + height: 16 + alignment: 0 + pivot: {x: 0, y: 0} + border: {x: 0, y: 0, z: 0, w: 0} + outline: [] + physicsShape: [] + tessellationDetail: 0 + bones: [] + spriteID: ef873dd1b08df5c408574bc5914b17e0 + internalID: 198299372 + vertices: [] + indices: + edges: [] + weights: [] + - serializedVersion: 2 + name: Dungeon_7 + rect: + serializedVersion: 2 + x: 32 + y: 224 + width: 16 + height: 16 + alignment: 0 + pivot: {x: 0, y: 0} + border: {x: 0, y: 0, z: 0, w: 0} + outline: [] + physicsShape: [] + tessellationDetail: 0 + bones: [] + spriteID: 9de658625e7c1cd4a8191d53ccb75084 + internalID: -650063954 + vertices: [] + indices: + edges: [] + weights: [] + - serializedVersion: 2 + name: Dungeon_8 + rect: + serializedVersion: 2 + x: 48 + y: 224 + width: 16 + height: 16 + alignment: 0 + pivot: {x: 0, y: 0} + border: {x: 0, y: 0, z: 0, w: 0} + outline: [] + physicsShape: [] + tessellationDetail: 0 + bones: [] + spriteID: dcc2accc12d32d446aa486f2ef7d26c1 + internalID: -1536037944 + vertices: [] + indices: + edges: [] + weights: [] + - serializedVersion: 2 + name: Dungeon_9 + rect: + serializedVersion: 2 + x: 64 + y: 224 + width: 16 + height: 16 + alignment: 0 + pivot: {x: 0, y: 0} + border: {x: 0, y: 0, z: 0, w: 0} + outline: [] + physicsShape: [] + tessellationDetail: 0 + bones: [] + spriteID: b6aea8aebe729ea41b8a90bcf38b5ab2 + internalID: 136035454 + vertices: [] + indices: + edges: [] + weights: [] + - serializedVersion: 2 + name: Dungeon_10 + rect: + serializedVersion: 2 + x: 80 + y: 224 + width: 16 + height: 16 + alignment: 0 + pivot: {x: 0, y: 0} + border: {x: 0, y: 0, z: 0, w: 0} + outline: [] + physicsShape: [] + tessellationDetail: 0 + bones: [] + spriteID: 912c9ed811a15e84aa8447ed6757b979 + internalID: 1460478432 + vertices: [] + indices: + edges: [] + weights: [] + - serializedVersion: 2 + name: Dungeon_11 + rect: + serializedVersion: 2 + x: 96 + y: 224 + width: 16 + height: 16 + alignment: 0 + pivot: {x: 0, y: 0} + border: {x: 0, y: 0, z: 0, w: 0} + outline: [] + physicsShape: [] + tessellationDetail: 0 + bones: [] + spriteID: 701619140c2b7b84b889399924c7b7a8 + internalID: 586641414 + vertices: [] + indices: + edges: [] + weights: [] + - serializedVersion: 2 + name: Dungeon_12 + rect: + serializedVersion: 2 + x: 16 + y: 208 + width: 16 + height: 16 + alignment: 0 + pivot: {x: 0, y: 0} + border: {x: 0, y: 0, z: 0, w: 0} + outline: [] + physicsShape: [] + tessellationDetail: 0 + bones: [] + spriteID: 37dbfeef47f12f54fb6f18eccb4cd377 + internalID: 1613682724 + vertices: [] + indices: + edges: [] + weights: [] + - serializedVersion: 2 + name: Dungeon_13 + rect: + serializedVersion: 2 + x: 32 + y: 208 + width: 16 + height: 16 + alignment: 0 + pivot: {x: 0, y: 0} + border: {x: 0, y: 0, z: 0, w: 0} + outline: [] + physicsShape: [] + tessellationDetail: 0 + bones: [] + spriteID: a0b320b5ac6accb4fa12ca505739b768 + internalID: 489946030 + vertices: [] + indices: + edges: [] + weights: [] + - serializedVersion: 2 + name: Dungeon_14 + rect: + serializedVersion: 2 + x: 48 + y: 208 + width: 16 + height: 16 + alignment: 0 + pivot: {x: 0, y: 0} + border: {x: 0, y: 0, z: 0, w: 0} + outline: [] + physicsShape: [] + tessellationDetail: 0 + bones: [] + spriteID: 1064354597a5a2949aab21b47ac49e8c + internalID: -1758251442 + vertices: [] + indices: + edges: [] + weights: [] + - serializedVersion: 2 + name: Dungeon_15 + rect: + serializedVersion: 2 + x: 64 + y: 208 + width: 16 + height: 16 + alignment: 0 + pivot: {x: 0, y: 0} + border: {x: 0, y: 0, z: 0, w: 0} + outline: [] + physicsShape: [] + tessellationDetail: 0 + bones: [] + spriteID: a4239a45296b80f4a86faf11e0e5828c + internalID: -432912164 + vertices: [] + indices: + edges: [] + weights: [] + - serializedVersion: 2 + name: Dungeon_16 + rect: + serializedVersion: 2 + x: 80 + y: 208 + width: 16 + height: 16 + alignment: 0 + pivot: {x: 0, y: 0} + border: {x: 0, y: 0, z: 0, w: 0} + outline: [] + physicsShape: [] + tessellationDetail: 0 + bones: [] + spriteID: 480dec6ec0684a348a714326de31b451 + internalID: 1580949469 + vertices: [] + indices: + edges: [] + weights: [] + - serializedVersion: 2 + name: Dungeon_17 + rect: + serializedVersion: 2 + x: 96 + y: 208 + width: 16 + height: 16 + alignment: 0 + pivot: {x: 0, y: 0} + border: {x: 0, y: 0, z: 0, w: 0} + outline: [] + physicsShape: [] + tessellationDetail: 0 + bones: [] + spriteID: 495b21167f6b3fd4d922579972f1b6ab + internalID: 2016605797 + vertices: [] + indices: + edges: [] + weights: [] + - serializedVersion: 2 + name: Dungeon_18 + rect: + serializedVersion: 2 + x: 16 + y: 192 + width: 16 + height: 16 + alignment: 0 + pivot: {x: 0, y: 0} + border: {x: 0, y: 0, z: 0, w: 0} + outline: [] + physicsShape: [] + tessellationDetail: 0 + bones: [] + spriteID: 5f989ce5aa199694d97329e397f9f4d3 + internalID: -1798427165 + vertices: [] + indices: + edges: [] + weights: [] + - serializedVersion: 2 + name: Dungeon_19 + rect: + serializedVersion: 2 + x: 32 + y: 192 + width: 16 + height: 16 + alignment: 0 + pivot: {x: 0, y: 0} + border: {x: 0, y: 0, z: 0, w: 0} + outline: [] + physicsShape: [] + tessellationDetail: 0 + bones: [] + spriteID: 32bf7e252282bb24eab1d880eae66566 + internalID: 1917838317 + vertices: [] + indices: + edges: [] + weights: [] + - serializedVersion: 2 + name: Dungeon_20 + rect: + serializedVersion: 2 + x: 48 + y: 192 + width: 16 + height: 16 + alignment: 0 + pivot: {x: 0, y: 0} + border: {x: 0, y: 0, z: 0, w: 0} + outline: [] + physicsShape: [] + tessellationDetail: 0 + bones: [] + spriteID: ab5ca4eeea337614b9062d740e9861e2 + internalID: 1926522667 + vertices: [] + indices: + edges: [] + weights: [] + - serializedVersion: 2 + name: Dungeon_21 + rect: + serializedVersion: 2 + x: 64 + y: 192 + width: 16 + height: 16 + alignment: 0 + pivot: {x: 0, y: 0} + border: {x: 0, y: 0, z: 0, w: 0} + outline: [] + physicsShape: [] + tessellationDetail: 0 + bones: [] + spriteID: 4d9775677c7808348966ab2998286035 + internalID: -672668870 + vertices: [] + indices: + edges: [] + weights: [] + - serializedVersion: 2 + name: Dungeon_22 + rect: + serializedVersion: 2 + x: 80 + y: 192 + width: 16 + height: 16 + alignment: 0 + pivot: {x: 0, y: 0} + border: {x: 0, y: 0, z: 0, w: 0} + outline: [] + physicsShape: [] + tessellationDetail: 0 + bones: [] + spriteID: 40dfa8fc29de55b498d08d60a7ca586c + internalID: -2113988543 + vertices: [] + indices: + edges: [] + weights: [] + - serializedVersion: 2 + name: Dungeon_23 + rect: + serializedVersion: 2 + x: 96 + y: 192 + width: 16 + height: 16 + alignment: 0 + pivot: {x: 0, y: 0} + border: {x: 0, y: 0, z: 0, w: 0} + outline: [] + physicsShape: [] + tessellationDetail: 0 + bones: [] + spriteID: 9c884ff0e218b8840a54d265ef53676b + internalID: 1655838605 + vertices: [] + indices: + edges: [] + weights: [] + - serializedVersion: 2 + name: Dungeon_24 + rect: + serializedVersion: 2 + x: 16 + y: 176 + width: 16 + height: 16 + alignment: 0 + pivot: {x: 0, y: 0} + border: {x: 0, y: 0, z: 0, w: 0} + outline: [] + physicsShape: [] + tessellationDetail: 0 + bones: [] + spriteID: 27b1385b703500246a71a995ad1620ef + internalID: 43680089 + vertices: [] + indices: + edges: [] + weights: [] + - serializedVersion: 2 + name: Dungeon_25 + rect: + serializedVersion: 2 + x: 32 + y: 176 + width: 16 + height: 16 + alignment: 0 + pivot: {x: 0, y: 0} + border: {x: 0, y: 0, z: 0, w: 0} + outline: [] + physicsShape: [] + tessellationDetail: 0 + bones: [] + spriteID: 3f464f3839745f04186125a036c714a6 + internalID: 1995419026 + vertices: [] + indices: + edges: [] + weights: [] + - serializedVersion: 2 + name: Dungeon_26 + rect: + serializedVersion: 2 + x: 48 + y: 176 + width: 16 + height: 16 + alignment: 0 + pivot: {x: 0, y: 0} + border: {x: 0, y: 0, z: 0, w: 0} + outline: [] + physicsShape: [] + tessellationDetail: 0 + bones: [] + spriteID: ed7b19798c589f645aceb64879e18f85 + internalID: 1277448032 + vertices: [] + indices: + edges: [] + weights: [] + - serializedVersion: 2 + name: Dungeon_27 + rect: + serializedVersion: 2 + x: 64 + y: 176 + width: 16 + height: 16 + alignment: 0 + pivot: {x: 0, y: 0} + border: {x: 0, y: 0, z: 0, w: 0} + outline: [] + physicsShape: [] + tessellationDetail: 0 + bones: [] + spriteID: c79bbfe12c65fb24696d2fa62468b4be + internalID: -412475550 + vertices: [] + indices: + edges: [] + weights: [] + - serializedVersion: 2 + name: Dungeon_28 + rect: + serializedVersion: 2 + x: 80 + y: 176 + width: 16 + height: 16 + alignment: 0 + pivot: {x: 0, y: 0} + border: {x: 0, y: 0, z: 0, w: 0} + outline: [] + physicsShape: [] + tessellationDetail: 0 + bones: [] + spriteID: cfaa11c808708be41a8227a53c94c7bb + internalID: 1065039898 + vertices: [] + indices: + edges: [] + weights: [] + - serializedVersion: 2 + name: Dungeon_29 + rect: + serializedVersion: 2 + x: 96 + y: 176 + width: 16 + height: 16 + alignment: 0 + pivot: {x: 0, y: 0} + border: {x: 0, y: 0, z: 0, w: 0} + outline: [] + physicsShape: [] + tessellationDetail: 0 + bones: [] + spriteID: 4d8069dc6a9194144b8a749b36bf9362 + internalID: 1956140949 + vertices: [] + indices: + edges: [] + weights: [] + - serializedVersion: 2 + name: Dungeon_30 + rect: + serializedVersion: 2 + x: 16 + y: 160 + width: 16 + height: 16 + alignment: 0 + pivot: {x: 0, y: 0} + border: {x: 0, y: 0, z: 0, w: 0} + outline: [] + physicsShape: [] + tessellationDetail: 0 + bones: [] + spriteID: 8a298191a82df2049a8b8256af5adc56 + internalID: -164313747 + vertices: [] + indices: + edges: [] + weights: [] + - serializedVersion: 2 + name: Dungeon_31 + rect: + serializedVersion: 2 + x: 32 + y: 160 + width: 16 + height: 16 + alignment: 0 + pivot: {x: 0, y: 0} + border: {x: 0, y: 0, z: 0, w: 0} + outline: [] + physicsShape: [] + tessellationDetail: 0 + bones: [] + spriteID: 891a3e4b901d46b42baabd1fe2d6883e + internalID: 1376823085 + vertices: [] + indices: + edges: [] + weights: [] + - serializedVersion: 2 + name: Dungeon_32 + rect: + serializedVersion: 2 + x: 48 + y: 160 + width: 16 + height: 16 + alignment: 0 + pivot: {x: 0, y: 0} + border: {x: 0, y: 0, z: 0, w: 0} + outline: [] + physicsShape: [] + tessellationDetail: 0 + bones: [] + spriteID: 1e81cae7afad8e54f89f51e183cb6bf4 + internalID: 2009541612 + vertices: [] + indices: + edges: [] + weights: [] + - serializedVersion: 2 + name: Dungeon_33 + rect: + serializedVersion: 2 + x: 64 + y: 160 + width: 16 + height: 16 + alignment: 0 + pivot: {x: 0, y: 0} + border: {x: 0, y: 0, z: 0, w: 0} + outline: [] + physicsShape: [] + tessellationDetail: 0 + bones: [] + spriteID: 9da1752ee6bd97b439b0c61093d337ba + internalID: -1028069899 + vertices: [] + indices: + edges: [] + weights: [] + - serializedVersion: 2 + name: Dungeon_34 + rect: + serializedVersion: 2 + x: 80 + y: 160 + width: 16 + height: 16 + alignment: 0 + pivot: {x: 0, y: 0} + border: {x: 0, y: 0, z: 0, w: 0} + outline: [] + physicsShape: [] + tessellationDetail: 0 + bones: [] + spriteID: 2db58ad66d0b4434eb0e410c00981d47 + internalID: 1099301450 + vertices: [] + indices: + edges: [] + weights: [] + - serializedVersion: 2 + name: Dungeon_35 + rect: + serializedVersion: 2 + x: 96 + y: 160 + width: 16 + height: 16 + alignment: 0 + pivot: {x: 0, y: 0} + border: {x: 0, y: 0, z: 0, w: 0} + outline: [] + physicsShape: [] + tessellationDetail: 0 + bones: [] + spriteID: c4b74b6988f6453429a7f54c4f850f6d + internalID: 81769002 + vertices: [] + indices: + edges: [] + weights: [] + - serializedVersion: 2 + name: Dungeon_36 + rect: + serializedVersion: 2 + x: 16 + y: 144 + width: 16 + height: 16 + alignment: 0 + pivot: {x: 0, y: 0} + border: {x: 0, y: 0, z: 0, w: 0} + outline: [] + physicsShape: [] + tessellationDetail: 0 + bones: [] + spriteID: fd67c9234f5a3fa409d57ee4fd1cf090 + internalID: 1050266288 + vertices: [] + indices: + edges: [] + weights: [] + - serializedVersion: 2 + name: Dungeon_37 + rect: + serializedVersion: 2 + x: 32 + y: 144 + width: 16 + height: 16 + alignment: 0 + pivot: {x: 0, y: 0} + border: {x: 0, y: 0, z: 0, w: 0} + outline: [] + physicsShape: [] + tessellationDetail: 0 + bones: [] + spriteID: e521a0b1c49cda84e8aa52d6d5c40f82 + internalID: 1708775233 + vertices: [] + indices: + edges: [] + weights: [] + - serializedVersion: 2 + name: Dungeon_38 + rect: + serializedVersion: 2 + x: 48 + y: 144 + width: 16 + height: 16 + alignment: 0 + pivot: {x: 0, y: 0} + border: {x: 0, y: 0, z: 0, w: 0} + outline: [] + physicsShape: [] + tessellationDetail: 0 + bones: [] + spriteID: 76cd98032eb5d7f43ae55b384e4d8be0 + internalID: 660103562 + vertices: [] + indices: + edges: [] + weights: [] + - serializedVersion: 2 + name: Dungeon_39 + rect: + serializedVersion: 2 + x: 64 + y: 144 + width: 16 + height: 16 + alignment: 0 + pivot: {x: 0, y: 0} + border: {x: 0, y: 0, z: 0, w: 0} + outline: [] + physicsShape: [] + tessellationDetail: 0 + bones: [] + spriteID: e601dcc244fa62b4ba2d7f997b036789 + internalID: 1397179706 + vertices: [] + indices: + edges: [] + weights: [] + - serializedVersion: 2 + name: Dungeon_40 + rect: + serializedVersion: 2 + x: 80 + y: 144 + width: 16 + height: 16 + alignment: 0 + pivot: {x: 0, y: 0} + border: {x: 0, y: 0, z: 0, w: 0} + outline: [] + physicsShape: [] + tessellationDetail: 0 + bones: [] + spriteID: 578c063b0737ae645b71148570121f70 + internalID: 1158887391 + vertices: [] + indices: + edges: [] + weights: [] + - serializedVersion: 2 + name: Dungeon_41 + rect: + serializedVersion: 2 + x: 96 + y: 144 + width: 16 + height: 16 + alignment: 0 + pivot: {x: 0, y: 0} + border: {x: 0, y: 0, z: 0, w: 0} + outline: [] + physicsShape: [] + tessellationDetail: 0 + bones: [] + spriteID: c9fb5cd67f7dabd4f9e2753e51f34694 + internalID: 615089749 + vertices: [] + indices: + edges: [] + weights: [] + - serializedVersion: 2 + name: Dungeon_42 + rect: + serializedVersion: 2 + x: 0 + y: 128 + width: 16 + height: 16 + alignment: 0 + pivot: {x: 0, y: 0} + border: {x: 0, y: 0, z: 0, w: 0} + outline: [] + physicsShape: [] + tessellationDetail: 0 + bones: [] + spriteID: 302f114cdf21bcf41bb067a33eac78ad + internalID: -777615196 + vertices: [] + indices: + edges: [] + weights: [] + - serializedVersion: 2 + name: Dungeon_43 + rect: + serializedVersion: 2 + x: 16 + y: 128 + width: 16 + height: 16 + alignment: 0 + pivot: {x: 0, y: 0} + border: {x: 0, y: 0, z: 0, w: 0} + outline: [] + physicsShape: [] + tessellationDetail: 0 + bones: [] + spriteID: f76ba334a7a6333449e2755ccdcea25f + internalID: 197855793 + vertices: [] + indices: + edges: [] + weights: [] + - serializedVersion: 2 + name: Dungeon_44 + rect: + serializedVersion: 2 + x: 32 + y: 128 + width: 16 + height: 16 + alignment: 0 + pivot: {x: 0, y: 0} + border: {x: 0, y: 0, z: 0, w: 0} + outline: [] + physicsShape: [] + tessellationDetail: 0 + bones: [] + spriteID: 9881fa91a8e725240b67935319713c09 + internalID: -1396940042 + vertices: [] + indices: + edges: [] + weights: [] + - serializedVersion: 2 + name: Dungeon_45 + rect: + serializedVersion: 2 + x: 48 + y: 128 + width: 16 + height: 16 + alignment: 0 + pivot: {x: 0, y: 0} + border: {x: 0, y: 0, z: 0, w: 0} + outline: [] + physicsShape: [] + tessellationDetail: 0 + bones: [] + spriteID: f6d97ddf698b1ac47b708682454d08a5 + internalID: 1802662682 + vertices: [] + indices: + edges: [] + weights: [] + - serializedVersion: 2 + name: Dungeon_46 + rect: + serializedVersion: 2 + x: 80 + y: 128 + width: 16 + height: 16 + alignment: 0 + pivot: {x: 0, y: 0} + border: {x: 0, y: 0, z: 0, w: 0} + outline: [] + physicsShape: [] + tessellationDetail: 0 + bones: [] + spriteID: 808eea3cd265fc84bbe544158a5dad0a + internalID: -549131558 + vertices: [] + indices: + edges: [] + weights: [] + - serializedVersion: 2 + name: Dungeon_47 + rect: + serializedVersion: 2 + x: 96 + y: 128 + width: 16 + height: 16 + alignment: 0 + pivot: {x: 0, y: 0} + border: {x: 0, y: 0, z: 0, w: 0} + outline: [] + physicsShape: [] + tessellationDetail: 0 + bones: [] + spriteID: 730499fc0651a2347ab744a7b9eca7b8 + internalID: -1668792333 + vertices: [] + indices: + edges: [] + weights: [] + - serializedVersion: 2 + name: Dungeon_48 + rect: + serializedVersion: 2 + x: 0 + y: 112 + width: 16 + height: 16 + alignment: 0 + pivot: {x: 0, y: 0} + border: {x: 0, y: 0, z: 0, w: 0} + outline: [] + physicsShape: [] + tessellationDetail: 0 + bones: [] + spriteID: a563291a358926b45b4d66ae59d25fca + internalID: -395624179 + vertices: [] + indices: + edges: [] + weights: [] + - serializedVersion: 2 + name: Dungeon_49 + rect: + serializedVersion: 2 + x: 16 + y: 112 + width: 16 + height: 16 + alignment: 0 + pivot: {x: 0, y: 0} + border: {x: 0, y: 0, z: 0, w: 0} + outline: [] + physicsShape: [] + tessellationDetail: 0 + bones: [] + spriteID: 1e30bb0edb68627499dcd04ef9884eac + internalID: 453119210 + vertices: [] + indices: + edges: [] + weights: [] + - serializedVersion: 2 + name: Dungeon_50 + rect: + serializedVersion: 2 + x: 32 + y: 112 + width: 16 + height: 16 + alignment: 0 + pivot: {x: 0, y: 0} + border: {x: 0, y: 0, z: 0, w: 0} + outline: [] + physicsShape: [] + tessellationDetail: 0 + bones: [] + spriteID: 8084f783a64e896458d865cd3b51072b + internalID: -1669469920 + vertices: [] + indices: + edges: [] + weights: [] + - serializedVersion: 2 + name: Dungeon_51 + rect: + serializedVersion: 2 + x: 48 + y: 112 + width: 16 + height: 16 + alignment: 0 + pivot: {x: 0, y: 0} + border: {x: 0, y: 0, z: 0, w: 0} + outline: [] + physicsShape: [] + tessellationDetail: 0 + bones: [] + spriteID: 9b65adaf4dbdbae478d322d52783e2ed + internalID: 79250496 + vertices: [] + indices: + edges: [] + weights: [] + - serializedVersion: 2 + name: Dungeon_52 + rect: + serializedVersion: 2 + x: 64 + y: 112 + width: 16 + height: 16 + alignment: 0 + pivot: {x: 0, y: 0} + border: {x: 0, y: 0, z: 0, w: 0} + outline: [] + physicsShape: [] + tessellationDetail: 0 + bones: [] + spriteID: 052d5d89c450f9a42878932054a50c19 + internalID: 2075798291 + vertices: [] + indices: + edges: [] + weights: [] + - serializedVersion: 2 + name: Dungeon_53 + rect: + serializedVersion: 2 + x: 80 + y: 112 + width: 16 + height: 16 + alignment: 0 + pivot: {x: 0, y: 0} + border: {x: 0, y: 0, z: 0, w: 0} + outline: [] + physicsShape: [] + tessellationDetail: 0 + bones: [] + spriteID: 3fea9c9692a4fc24e9a31a9ba5056ba0 + internalID: -282376894 + vertices: [] + indices: + edges: [] + weights: [] + - serializedVersion: 2 + name: Dungeon_54 + rect: + serializedVersion: 2 + x: 96 + y: 112 + width: 16 + height: 16 + alignment: 0 + pivot: {x: 0, y: 0} + border: {x: 0, y: 0, z: 0, w: 0} + outline: [] + physicsShape: [] + tessellationDetail: 0 + bones: [] + spriteID: 97439090f9b702445a0572987f4c98ab + internalID: 1862703900 + vertices: [] + indices: + edges: [] + weights: [] + - serializedVersion: 2 + name: Dungeon_55 + rect: + serializedVersion: 2 + x: 0 + y: 96 + width: 16 + height: 16 + alignment: 0 + pivot: {x: 0, y: 0} + border: {x: 0, y: 0, z: 0, w: 0} + outline: [] + physicsShape: [] + tessellationDetail: 0 + bones: [] + spriteID: d442e7bcd1f277e44a247494fa851675 + internalID: 536411375 + vertices: [] + indices: + edges: [] + weights: [] + - serializedVersion: 2 + name: Dungeon_56 + rect: + serializedVersion: 2 + x: 16 + y: 96 + width: 16 + height: 16 + alignment: 0 + pivot: {x: 0, y: 0} + border: {x: 0, y: 0, z: 0, w: 0} + outline: [] + physicsShape: [] + tessellationDetail: 0 + bones: [] + spriteID: 15407da73b9c97443bc11a04287e0180 + internalID: 796962211 + vertices: [] + indices: + edges: [] + weights: [] + - serializedVersion: 2 + name: Dungeon_57 + rect: + serializedVersion: 2 + x: 32 + y: 96 + width: 16 + height: 16 + alignment: 0 + pivot: {x: 0, y: 0} + border: {x: 0, y: 0, z: 0, w: 0} + outline: [] + physicsShape: [] + tessellationDetail: 0 + bones: [] + spriteID: fd087ee39935470409472dd50b9f7410 + internalID: 1661568666 + vertices: [] + indices: + edges: [] + weights: [] + - serializedVersion: 2 + name: Dungeon_58 + rect: + serializedVersion: 2 + x: 48 + y: 96 + width: 16 + height: 16 + alignment: 0 + pivot: {x: 0, y: 0} + border: {x: 0, y: 0, z: 0, w: 0} + outline: [] + physicsShape: [] + tessellationDetail: 0 + bones: [] + spriteID: b255fcab2b253ef46b7d5bd54074536b + internalID: -2046453881 + vertices: [] + indices: + edges: [] + weights: [] + - serializedVersion: 2 + name: Dungeon_59 + rect: + serializedVersion: 2 + x: 64 + y: 96 + width: 16 + height: 16 + alignment: 0 + pivot: {x: 0, y: 0} + border: {x: 0, y: 0, z: 0, w: 0} + outline: [] + physicsShape: [] + tessellationDetail: 0 + bones: [] + spriteID: abb1a5b87ce10fd47a7149107ffb57de + internalID: 2038936553 + vertices: [] + indices: + edges: [] + weights: [] + - serializedVersion: 2 + name: Dungeon_60 + rect: + serializedVersion: 2 + x: 80 + y: 96 + width: 16 + height: 16 + alignment: 0 + pivot: {x: 0, y: 0} + border: {x: 0, y: 0, z: 0, w: 0} + outline: [] + physicsShape: [] + tessellationDetail: 0 + bones: [] + spriteID: 7a477f36df9ca9a4e9885ae8d66eac01 + internalID: 1660072189 + vertices: [] + indices: + edges: [] + weights: [] + - serializedVersion: 2 + name: Dungeon_61 + rect: + serializedVersion: 2 + x: 96 + y: 96 + width: 16 + height: 16 + alignment: 0 + pivot: {x: 0, y: 0} + border: {x: 0, y: 0, z: 0, w: 0} + outline: [] + physicsShape: [] + tessellationDetail: 0 + bones: [] + spriteID: 5b97b0fc47b4a834cbc3c3519a266b5a + internalID: -1999030480 + vertices: [] + indices: + edges: [] + weights: [] + - serializedVersion: 2 + name: Dungeon_62 + rect: + serializedVersion: 2 + x: 32 + y: 80 + width: 16 + height: 16 + alignment: 0 + pivot: {x: 0, y: 0} + border: {x: 0, y: 0, z: 0, w: 0} + outline: [] + physicsShape: [] + tessellationDetail: 0 + bones: [] + spriteID: d2187b6778dfb624680cd9da4f353226 + internalID: -62922940 + vertices: [] + indices: + edges: [] + weights: [] + - serializedVersion: 2 + name: Dungeon_63 + rect: + serializedVersion: 2 + x: 48 + y: 80 + width: 16 + height: 16 + alignment: 0 + pivot: {x: 0, y: 0} + border: {x: 0, y: 0, z: 0, w: 0} + outline: [] + physicsShape: [] + tessellationDetail: 0 + bones: [] + spriteID: 0191ea08adeff5b46ad4fa52c24b46b6 + internalID: 154182392 + vertices: [] + indices: + edges: [] + weights: [] + - serializedVersion: 2 + name: Dungeon_64 + rect: + serializedVersion: 2 + x: 64 + y: 80 + width: 16 + height: 16 + alignment: 0 + pivot: {x: 0, y: 0} + border: {x: 0, y: 0, z: 0, w: 0} + outline: [] + physicsShape: [] + tessellationDetail: 0 + bones: [] + spriteID: f89aaff1ea7ccd844a0e0ba5603af8d0 + internalID: -38314673 + vertices: [] + indices: + edges: [] + weights: [] + - serializedVersion: 2 + name: Dungeon_65 + rect: + serializedVersion: 2 + x: 80 + y: 80 + width: 16 + height: 16 + alignment: 0 + pivot: {x: 0, y: 0} + border: {x: 0, y: 0, z: 0, w: 0} + outline: [] + physicsShape: [] + tessellationDetail: 0 + bones: [] + spriteID: 4080ed98fd7a6354aaeb0dbc74da61d6 + internalID: 442919366 + vertices: [] + indices: + edges: [] + weights: [] + - serializedVersion: 2 + name: Dungeon_66 + rect: + serializedVersion: 2 + x: 16 + y: 64 + width: 16 + height: 16 + alignment: 0 + pivot: {x: 0, y: 0} + border: {x: 0, y: 0, z: 0, w: 0} + outline: [] + physicsShape: [] + tessellationDetail: 0 + bones: [] + spriteID: 5a1b45265ba432f41991153f2330f8c6 + internalID: -521188253 + vertices: [] + indices: + edges: [] + weights: [] + - serializedVersion: 2 + name: Dungeon_67 + rect: + serializedVersion: 2 + x: 32 + y: 64 + width: 16 + height: 16 + alignment: 0 + pivot: {x: 0, y: 0} + border: {x: 0, y: 0, z: 0, w: 0} + outline: [] + physicsShape: [] + tessellationDetail: 0 + bones: [] + spriteID: 9fcea01c09abc44429fd10817af93eaa + internalID: -428477728 + vertices: [] + indices: + edges: [] + weights: [] + - serializedVersion: 2 + name: Dungeon_68 + rect: + serializedVersion: 2 + x: 48 + y: 64 + width: 16 + height: 16 + alignment: 0 + pivot: {x: 0, y: 0} + border: {x: 0, y: 0, z: 0, w: 0} + outline: [] + physicsShape: [] + tessellationDetail: 0 + bones: [] + spriteID: 1e0f8e856a9362942ac7aed0b997669c + internalID: -2045571822 + vertices: [] + indices: + edges: [] + weights: [] + - serializedVersion: 2 + name: Dungeon_69 + rect: + serializedVersion: 2 + x: 64 + y: 64 + width: 16 + height: 16 + alignment: 0 + pivot: {x: 0, y: 0} + border: {x: 0, y: 0, z: 0, w: 0} + outline: [] + physicsShape: [] + tessellationDetail: 0 + bones: [] + spriteID: c80d93ad7ad5cd54fba876df2ed7d574 + internalID: -839279710 + vertices: [] + indices: + edges: [] + weights: [] + - serializedVersion: 2 + name: Dungeon_70 + rect: + serializedVersion: 2 + x: 80 + y: 64 + width: 16 + height: 16 + alignment: 0 + pivot: {x: 0, y: 0} + border: {x: 0, y: 0, z: 0, w: 0} + outline: [] + physicsShape: [] + tessellationDetail: 0 + bones: [] + spriteID: 9fd7d51465f30aa41b3892237fdcc9f6 + internalID: 2090772569 + vertices: [] + indices: + edges: [] + weights: [] + - serializedVersion: 2 + name: Dungeon_71 + rect: + serializedVersion: 2 + x: 16 + y: 48 + width: 16 + height: 16 + alignment: 0 + pivot: {x: 0, y: 0} + border: {x: 0, y: 0, z: 0, w: 0} + outline: [] + physicsShape: [] + tessellationDetail: 0 + bones: [] + spriteID: 96ad5c0baa8abd4449220e7c363b6afb + internalID: -1297728808 + vertices: [] + indices: + edges: [] + weights: [] + - serializedVersion: 2 + name: Dungeon_72 + rect: + serializedVersion: 2 + x: 32 + y: 48 + width: 16 + height: 16 + alignment: 0 + pivot: {x: 0, y: 0} + border: {x: 0, y: 0, z: 0, w: 0} + outline: [] + physicsShape: [] + tessellationDetail: 0 + bones: [] + spriteID: bafc5256d552ca54c917f0060096a4a1 + internalID: -1473822626 + vertices: [] + indices: + edges: [] + weights: [] + - serializedVersion: 2 + name: Dungeon_73 + rect: + serializedVersion: 2 + x: 48 + y: 48 + width: 16 + height: 16 + alignment: 0 + pivot: {x: 0, y: 0} + border: {x: 0, y: 0, z: 0, w: 0} + outline: [] + physicsShape: [] + tessellationDetail: 0 + bones: [] + spriteID: c9f2a101c0dc0dd48966d09c8eacbae2 + internalID: -1760836496 + vertices: [] + indices: + edges: [] + weights: [] + - serializedVersion: 2 + name: Dungeon_74 + rect: + serializedVersion: 2 + x: 64 + y: 48 + width: 16 + height: 16 + alignment: 0 + pivot: {x: 0, y: 0} + border: {x: 0, y: 0, z: 0, w: 0} + outline: [] + physicsShape: [] + tessellationDetail: 0 + bones: [] + spriteID: c09640ba7ce5fa94ab4f02b17a9d2558 + internalID: 1547048006 + vertices: [] + indices: + edges: [] + weights: [] + - serializedVersion: 2 + name: Dungeon_75 + rect: + serializedVersion: 2 + x: 80 + y: 48 + width: 16 + height: 16 + alignment: 0 + pivot: {x: 0, y: 0} + border: {x: 0, y: 0, z: 0, w: 0} + outline: [] + physicsShape: [] + tessellationDetail: 0 + bones: [] + spriteID: 543b4eaf26b094849bfe9dba24094631 + internalID: 613834084 + vertices: [] + indices: + edges: [] + weights: [] + - serializedVersion: 2 + name: Dungeon_76 + rect: + serializedVersion: 2 + x: 96 + y: 48 + width: 16 + height: 16 + alignment: 0 + pivot: {x: 0, y: 0} + border: {x: 0, y: 0, z: 0, w: 0} + outline: [] + physicsShape: [] + tessellationDetail: 0 + bones: [] + spriteID: 9576cb2301ab23449836e77215037559 + internalID: 1270900073 + vertices: [] + indices: + edges: [] + weights: [] + - serializedVersion: 2 + name: Dungeon_77 + rect: + serializedVersion: 2 + x: 32 + y: 32 + width: 16 + height: 16 + alignment: 0 + pivot: {x: 0, y: 0} + border: {x: 0, y: 0, z: 0, w: 0} + outline: [] + physicsShape: [] + tessellationDetail: 0 + bones: [] + spriteID: 8869ea4a2f670b7409cde3bcfe761b73 + internalID: 2137237813 + vertices: [] + indices: + edges: [] + weights: [] + - serializedVersion: 2 + name: Dungeon_78 + rect: + serializedVersion: 2 + x: 48 + y: 32 + width: 16 + height: 16 + alignment: 0 + pivot: {x: 0, y: 0} + border: {x: 0, y: 0, z: 0, w: 0} + outline: [] + physicsShape: [] + tessellationDetail: 0 + bones: [] + spriteID: f7169a7302d74734fa7e02af66c5ab5b + internalID: 1460241374 + vertices: [] + indices: + edges: [] + weights: [] + - serializedVersion: 2 + name: Dungeon_79 + rect: + serializedVersion: 2 + x: 16 + y: 16 + width: 16 + height: 16 + alignment: 0 + pivot: {x: 0, y: 0} + border: {x: 0, y: 0, z: 0, w: 0} + outline: [] + physicsShape: [] + tessellationDetail: 0 + bones: [] + spriteID: e8753b2ce8551154dac73185900a57fa + internalID: -1614669064 + vertices: [] + indices: + edges: [] + weights: [] + - serializedVersion: 2 + name: Dungeon_80 + rect: + serializedVersion: 2 + x: 32 + y: 16 + width: 16 + height: 16 + alignment: 0 + pivot: {x: 0, y: 0} + border: {x: 0, y: 0, z: 0, w: 0} + outline: [] + physicsShape: [] + tessellationDetail: 0 + bones: [] + spriteID: 3fb64f1629d468a45a69f5f2d03e236f + internalID: 1642675801 + vertices: [] + indices: + edges: [] + weights: [] + - serializedVersion: 2 + name: Dungeon_81 + rect: + serializedVersion: 2 + x: 48 + y: 16 + width: 16 + height: 16 + alignment: 0 + pivot: {x: 0, y: 0} + border: {x: 0, y: 0, z: 0, w: 0} + outline: [] + physicsShape: [] + tessellationDetail: 0 + bones: [] + spriteID: a8d64ec0744f3b64cac5cea0c22b25ac + internalID: -953407387 + vertices: [] + indices: + edges: [] + weights: [] + - serializedVersion: 2 + name: Dungeon_82 + rect: + serializedVersion: 2 + x: 64 + y: 16 + width: 16 + height: 16 + alignment: 0 + pivot: {x: 0, y: 0} + border: {x: 0, y: 0, z: 0, w: 0} + outline: [] + physicsShape: [] + tessellationDetail: 0 + bones: [] + spriteID: 1dd3af11184c04345ae15d2626c3fe5d + internalID: -1181825705 + vertices: [] + indices: + edges: [] + weights: [] + - serializedVersion: 2 + name: Dungeon_83 + rect: + serializedVersion: 2 + x: 80 + y: 16 + width: 16 + height: 16 + alignment: 0 + pivot: {x: 0, y: 0} + border: {x: 0, y: 0, z: 0, w: 0} + outline: [] + physicsShape: [] + tessellationDetail: 0 + bones: [] + spriteID: 21233572fdc354640a8bd268ea347480 + internalID: -1892450189 + vertices: [] + indices: + edges: [] + weights: [] + - serializedVersion: 2 + name: Dungeon_84 + rect: + serializedVersion: 2 + x: 96 + y: 16 + width: 16 + height: 16 + alignment: 0 + pivot: {x: 0, y: 0} + border: {x: 0, y: 0, z: 0, w: 0} + outline: [] + physicsShape: [] + tessellationDetail: 0 + bones: [] + spriteID: 4f777125b847a264b98de302daca646e + internalID: -1649980043 + vertices: [] + indices: + edges: [] + weights: [] + - serializedVersion: 2 + name: Dungeon_85 + rect: + serializedVersion: 2 + x: 16 + y: 0 + width: 16 + height: 16 + alignment: 0 + pivot: {x: 0, y: 0} + border: {x: 0, y: 0, z: 0, w: 0} + outline: [] + physicsShape: [] + tessellationDetail: 0 + bones: [] + spriteID: 31cd7e0a3953ce84c8ef51be8c745e83 + internalID: 811441136 + vertices: [] + indices: + edges: [] + weights: [] + - serializedVersion: 2 + name: Dungeon_86 + rect: + serializedVersion: 2 + x: 32 + y: 0 + width: 16 + height: 16 + alignment: 0 + pivot: {x: 0, y: 0} + border: {x: 0, y: 0, z: 0, w: 0} + outline: [] + physicsShape: [] + tessellationDetail: 0 + bones: [] + spriteID: 94006db4bdfd19d4e954b46df8c70dcc + internalID: 207130727 + vertices: [] + indices: + edges: [] + weights: [] + - serializedVersion: 2 + name: Dungeon_87 + rect: + serializedVersion: 2 + x: 48 + y: 0 + width: 16 + height: 16 + alignment: 0 + pivot: {x: 0, y: 0} + border: {x: 0, y: 0, z: 0, w: 0} + outline: [] + physicsShape: [] + tessellationDetail: 0 + bones: [] + spriteID: f8cbc00824210c54091c01aa25c8f0f3 + internalID: -857797333 + vertices: [] + indices: + edges: [] + weights: [] + - serializedVersion: 2 + name: Dungeon_88 + rect: + serializedVersion: 2 + x: 64 + y: 0 + width: 16 + height: 16 + alignment: 0 + pivot: {x: 0, y: 0} + border: {x: 0, y: 0, z: 0, w: 0} + outline: [] + physicsShape: [] + tessellationDetail: 0 + bones: [] + spriteID: 14e24fd6fc1f5ba41ac9bf332c783f08 + internalID: 306057277 + vertices: [] + indices: + edges: [] + weights: [] + - serializedVersion: 2 + name: Dungeon_89 + rect: + serializedVersion: 2 + x: 80 + y: 0 + width: 16 + height: 16 + alignment: 0 + pivot: {x: 0, y: 0} + border: {x: 0, y: 0, z: 0, w: 0} + outline: [] + physicsShape: [] + tessellationDetail: 0 + bones: [] + spriteID: 479d5663452909c4c9848b728acb8eb0 + internalID: -1558256172 + vertices: [] + indices: + edges: [] + weights: [] + - serializedVersion: 2 + name: Dungeon_90 + rect: + serializedVersion: 2 + x: 96 + y: 0 + width: 16 + height: 16 + alignment: 0 + pivot: {x: 0, y: 0} + border: {x: 0, y: 0, z: 0, w: 0} + outline: [] + physicsShape: [] + tessellationDetail: 0 + bones: [] + spriteID: 95a0645b5a03669488f134a8f8509b95 + internalID: 1466099207 + vertices: [] + indices: + edges: [] + weights: [] + outline: [] + physicsShape: [] + bones: [] + spriteID: 5e97eb03825dee720800000000000000 + internalID: 0 + vertices: [] + indices: + edges: [] + weights: [] + secondaryTextures: [] + nameFileIdTable: + Dungeon_39: 1397179706 + Dungeon_46: -549131558 + Dungeon_47: -1668792333 + Dungeon_4: -345571141 + Dungeon_78: 1460241374 + Dungeon_87: -857797333 + Dungeon_16: 1580949469 + Dungeon_62: -62922940 + Dungeon_90: 1466099207 + Dungeon_50: -1669469920 + Dungeon_67: -428477728 + Dungeon_55: 536411375 + Dungeon_72: -1473822626 + Dungeon_73: -1760836496 + Dungeon_21: -672668870 + Dungeon_34: 1099301450 + Dungeon_79: -1614669064 + Dungeon_58: -2046453881 + Dungeon_7: -650063954 + Dungeon_19: 1917838317 + Dungeon_30: -164313747 + Dungeon_68: -2045571822 + Dungeon_14: -1758251442 + Dungeon_27: -412475550 + Dungeon_69: -839279710 + Dungeon_76: 1270900073 + Dungeon_48: -395624179 + Dungeon_77: 2137237813 + Dungeon_31: 1376823085 + Dungeon_88: 306057277 + Dungeon_53: -282376894 + Dungeon_29: 1956140949 + Dungeon_57: 1661568666 + Dungeon_81: -953407387 + Dungeon_28: 1065039898 + Dungeon_51: 79250496 + Dungeon_9: 136035454 + Dungeon_59: 2038936553 + Dungeon_15: -432912164 + Dungeon_41: 615089749 + Dungeon_61: -1999030480 + Dungeon_80: 1642675801 + Dungeon_35: 81769002 + Dungeon_83: -1892450189 + Dungeon_22: -2113988543 + Dungeon_5: 2094817791 + Dungeon_18: -1798427165 + Dungeon_49: 453119210 + Dungeon_63: 154182392 + Dungeon_11: 586641414 + Dungeon_44: -1396940042 + Dungeon_86: 207130727 + Dungeon_66: -521188253 + Dungeon_40: 1158887391 + Dungeon_12: 1613682724 + Dungeon_8: -1536037944 + Dungeon_82: -1181825705 + Dungeon_10: 1460478432 + Dungeon_75: 613834084 + Dungeon_60: 1660072189 + Dungeon_33: -1028069899 + Dungeon_20: 1926522667 + Dungeon_6: 198299372 + Dungeon_0: -509989529 + Dungeon_1: -1085996522 + Dungeon_3: 326241045 + Dungeon_45: 1802662682 + Dungeon_71: -1297728808 + Dungeon_17: 2016605797 + Dungeon_85: 811441136 + Dungeon_70: 2090772569 + Dungeon_84: -1649980043 + Dungeon_89: -1558256172 + Dungeon_2: 164431376 + Dungeon_13: 489946030 + Dungeon_64: -38314673 + Dungeon_36: 1050266288 + Dungeon_56: 796962211 + Dungeon_23: 1655838605 + Dungeon_25: 1995419026 + Dungeon_37: 1708775233 + Dungeon_52: 2075798291 + Dungeon_54: 1862703900 + Dungeon_43: 197855793 + Dungeon_38: 660103562 + Dungeon_42: -777615196 + Dungeon_32: 2009541612 + Dungeon_24: 43680089 + Dungeon_74: 1547048006 + Dungeon_26: 1277448032 + Dungeon_65: 442919366 + spritePackingTag: + pSDRemoveMatte: 0 + pSDShowRemoveMatteOption: 0 + userData: + assetBundleName: + assetBundleVariant: diff --git a/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon.prefab b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon.prefab new file mode 100644 index 0000000000000000000000000000000000000000..55b54489c0a55d5afc5139a1b8fbfa6f640b9c19 --- /dev/null +++ b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon.prefab @@ -0,0 +1,1476 @@ +%YAML 1.1 +%TAG !u! tag:unity3d.com,2011: +--- !u!1 &910361770656200846 +GameObject: + m_ObjectHideFlags: 0 + m_CorrespondingSourceObject: {fileID: 0} + m_PrefabInstance: {fileID: 0} + m_PrefabAsset: {fileID: 0} + serializedVersion: 6 + m_Component: + - component: {fileID: 1644156093156590069} + - component: {fileID: 8165535013688410873} + - component: {fileID: 2026011780236248997} + m_Layer: 0 + m_Name: Layer1 + m_TagString: Untagged + m_Icon: {fileID: 0} + m_NavMeshLayer: 0 + m_StaticEditorFlags: 0 + m_IsActive: 1 +--- !u!4 &1644156093156590069 +Transform: + m_ObjectHideFlags: 0 + m_CorrespondingSourceObject: {fileID: 0} + m_PrefabInstance: {fileID: 0} + m_PrefabAsset: {fileID: 0} + m_GameObject: {fileID: 910361770656200846} + m_LocalRotation: {x: -0, y: -0, z: -0, w: 1} + m_LocalPosition: {x: 0, y: 0, z: 0} + m_LocalScale: {x: 1, y: 1, z: 1} + m_ConstrainProportionsScale: 0 + m_Children: [] + m_Father: {fileID: 1100878670463798578} + m_RootOrder: 0 + m_LocalEulerAnglesHint: {x: 0, y: 0, z: 0} +--- !u!1839735485 &8165535013688410873 +Tilemap: + m_ObjectHideFlags: 0 + m_CorrespondingSourceObject: {fileID: 0} + m_PrefabInstance: {fileID: 0} + m_PrefabAsset: {fileID: 0} + m_GameObject: {fileID: 910361770656200846} + m_Enabled: 1 + m_Tiles: + - first: {x: -1, y: -14, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 85 + m_TileSpriteIndex: 85 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: 0, y: -14, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 86 + m_TileSpriteIndex: 86 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: 1, y: -14, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 87 + m_TileSpriteIndex: 87 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: 2, y: -14, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 88 + m_TileSpriteIndex: 88 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: 3, y: -14, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 89 + m_TileSpriteIndex: 89 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: 4, y: -14, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 90 + m_TileSpriteIndex: 90 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: -1, y: -13, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 79 + m_TileSpriteIndex: 79 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: 0, y: -13, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 80 + m_TileSpriteIndex: 80 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: 1, y: -13, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 81 + m_TileSpriteIndex: 81 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: 2, y: -13, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 82 + m_TileSpriteIndex: 82 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: 3, y: -13, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 83 + m_TileSpriteIndex: 83 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: 4, y: -13, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 84 + m_TileSpriteIndex: 84 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: 0, y: -12, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 77 + m_TileSpriteIndex: 77 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: 1, y: -12, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 78 + m_TileSpriteIndex: 78 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: -1, y: -11, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 71 + m_TileSpriteIndex: 71 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: 0, y: -11, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 72 + m_TileSpriteIndex: 72 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: 1, y: -11, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 73 + m_TileSpriteIndex: 73 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: 2, y: -11, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 74 + m_TileSpriteIndex: 74 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: 3, y: -11, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 75 + m_TileSpriteIndex: 75 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: 4, y: -11, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 76 + m_TileSpriteIndex: 76 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: -1, y: -10, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 66 + m_TileSpriteIndex: 66 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: 0, y: -10, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 67 + m_TileSpriteIndex: 67 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: 1, y: -10, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 68 + m_TileSpriteIndex: 68 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: 2, y: -10, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 69 + m_TileSpriteIndex: 69 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: 3, y: -10, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 70 + m_TileSpriteIndex: 70 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: 0, y: -9, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 62 + m_TileSpriteIndex: 62 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: 1, y: -9, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 63 + m_TileSpriteIndex: 63 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: 2, y: -9, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 64 + m_TileSpriteIndex: 64 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: 3, y: -9, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 65 + m_TileSpriteIndex: 65 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: -2, y: -8, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 55 + m_TileSpriteIndex: 55 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: -1, y: -8, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 56 + m_TileSpriteIndex: 56 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: 0, y: -8, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 57 + m_TileSpriteIndex: 57 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: 1, y: -8, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 58 + m_TileSpriteIndex: 58 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: 2, y: -8, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 59 + m_TileSpriteIndex: 59 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: 3, y: -8, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 60 + m_TileSpriteIndex: 60 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: 4, y: -8, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 61 + m_TileSpriteIndex: 61 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: -2, y: -7, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 48 + m_TileSpriteIndex: 48 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: -1, y: -7, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 49 + m_TileSpriteIndex: 49 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: 0, y: -7, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 50 + m_TileSpriteIndex: 50 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: 1, y: -7, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 51 + m_TileSpriteIndex: 51 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: 2, y: -7, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 52 + m_TileSpriteIndex: 52 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: 3, y: -7, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 53 + m_TileSpriteIndex: 53 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: 4, y: -7, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 54 + m_TileSpriteIndex: 54 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: -2, y: -6, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 42 + m_TileSpriteIndex: 42 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: -1, y: -6, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 43 + m_TileSpriteIndex: 43 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: 0, y: -6, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 44 + m_TileSpriteIndex: 44 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: 1, y: -6, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 45 + m_TileSpriteIndex: 45 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: 3, y: -6, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 46 + m_TileSpriteIndex: 46 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: 4, y: -6, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 47 + m_TileSpriteIndex: 47 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: -1, y: -5, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 36 + m_TileSpriteIndex: 36 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: 0, y: -5, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 37 + m_TileSpriteIndex: 37 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: 1, y: -5, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 38 + m_TileSpriteIndex: 38 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: 2, y: -5, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 39 + m_TileSpriteIndex: 39 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: 3, y: -5, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 40 + m_TileSpriteIndex: 40 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: 4, y: -5, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 41 + m_TileSpriteIndex: 41 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: -1, y: -4, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 30 + m_TileSpriteIndex: 30 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: 0, y: -4, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 31 + m_TileSpriteIndex: 31 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: 1, y: -4, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 32 + m_TileSpriteIndex: 32 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: 2, y: -4, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 33 + m_TileSpriteIndex: 33 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: 3, y: -4, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 34 + m_TileSpriteIndex: 34 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: 4, y: -4, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 35 + m_TileSpriteIndex: 35 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: -1, y: -3, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 24 + m_TileSpriteIndex: 24 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: 0, y: -3, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 25 + m_TileSpriteIndex: 25 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: 1, y: -3, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 26 + m_TileSpriteIndex: 26 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: 2, y: -3, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 27 + m_TileSpriteIndex: 27 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: 3, y: -3, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 28 + m_TileSpriteIndex: 28 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: 4, y: -3, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 29 + m_TileSpriteIndex: 29 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: -1, y: -2, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 18 + m_TileSpriteIndex: 18 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: 0, y: -2, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 19 + m_TileSpriteIndex: 19 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: 1, y: -2, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 20 + m_TileSpriteIndex: 20 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: 2, y: -2, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 21 + m_TileSpriteIndex: 21 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: 3, y: -2, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 22 + m_TileSpriteIndex: 22 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: 4, y: -2, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 23 + m_TileSpriteIndex: 23 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: -1, y: -1, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 12 + m_TileSpriteIndex: 12 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: 0, y: -1, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 13 + m_TileSpriteIndex: 13 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: 1, y: -1, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 14 + m_TileSpriteIndex: 14 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: 2, y: -1, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 15 + m_TileSpriteIndex: 15 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: 3, y: -1, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 16 + m_TileSpriteIndex: 16 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: 4, y: -1, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 17 + m_TileSpriteIndex: 17 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: -1, y: 0, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 6 + m_TileSpriteIndex: 6 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: 0, y: 0, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 7 + m_TileSpriteIndex: 7 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: 1, y: 0, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 8 + m_TileSpriteIndex: 8 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: 2, y: 0, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 9 + m_TileSpriteIndex: 9 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: 3, y: 0, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 10 + m_TileSpriteIndex: 10 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: 4, y: 0, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 11 + m_TileSpriteIndex: 11 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: -1, y: 1, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 0 + m_TileSpriteIndex: 0 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: 0, y: 1, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 1 + m_TileSpriteIndex: 1 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: 1, y: 1, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 2 + m_TileSpriteIndex: 2 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: 2, y: 1, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 3 + m_TileSpriteIndex: 3 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: 3, y: 1, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 4 + m_TileSpriteIndex: 4 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + - first: {x: 4, y: 1, z: 0} + second: + serializedVersion: 2 + m_TileIndex: 5 + m_TileSpriteIndex: 5 + m_TileMatrixIndex: 0 + m_TileColorIndex: 0 + m_TileObjectToInstantiateIndex: 65535 + dummyAlignment: 0 + m_AllTileFlags: 1073741825 + m_AnimatedTiles: {} + m_TileAssetArray: + - m_RefCount: 1 + m_Data: {fileID: 11400000, guid: 91f123c55b83ed54ea8387bbb6250b9c, type: 2} + - m_RefCount: 1 + m_Data: {fileID: 11400000, guid: 257657ca820b66741ab4695fbb49f83e, type: 2} + - m_RefCount: 1 + m_Data: {fileID: 11400000, guid: abf6b1252b746f744a5040d07340a8f4, type: 2} + - m_RefCount: 1 + m_Data: {fileID: 11400000, guid: 609866c82a109c746b2814487ebbef66, type: 2} + - m_RefCount: 1 + m_Data: {fileID: 11400000, guid: 95fb445777b235b4fba02befc77382dd, type: 2} + - m_RefCount: 1 + m_Data: {fileID: 11400000, guid: 15279f4515799164b81e6d2645edb2b8, type: 2} + - m_RefCount: 1 + m_Data: {fileID: 11400000, guid: 2ead1fba8f3becd49aed19156d21e54c, type: 2} + - m_RefCount: 1 + m_Data: {fileID: 11400000, guid: a23d356dc7ef26b42afb97e7a7e87330, type: 2} + - m_RefCount: 1 + m_Data: {fileID: 11400000, guid: 13e364b0eaf450c4095d73340ee6a58c, type: 2} + - m_RefCount: 1 + m_Data: {fileID: 11400000, guid: 146696a0f0461d9439de4b790c6e083b, type: 2} + - m_RefCount: 1 + m_Data: {fileID: 11400000, guid: e56c374d1cab17f4aabbff2d5aa48bf6, type: 2} + - m_RefCount: 1 + m_Data: {fileID: 11400000, guid: d594b739b3e29ee468ebc200a8b966a4, type: 2} + - m_RefCount: 1 + m_Data: {fileID: 11400000, guid: f9f70339e2318c94988b284e7fb563e1, type: 2} + - m_RefCount: 1 + m_Data: {fileID: 11400000, guid: c0a9a81d8f984974caea2de0685f4691, type: 2} + - m_RefCount: 1 + m_Data: {fileID: 11400000, guid: 604fb5a5936131f42b7ed995b90b27f5, type: 2} + - m_RefCount: 1 + m_Data: {fileID: 11400000, guid: de3388181831cdc46a01a7486e223f94, type: 2} + - m_RefCount: 1 + m_Data: {fileID: 11400000, guid: eb057963df5e999448defa44fca62171, type: 2} + - m_RefCount: 1 + m_Data: {fileID: 11400000, guid: 08940e2753a7a074a9301f324a0422cc, type: 2} + - m_RefCount: 1 + m_Data: {fileID: 11400000, guid: 6c8ea1298beba484d81a637df26e49f2, type: 2} + - m_RefCount: 1 + m_Data: {fileID: 11400000, guid: f2976e05ee95b31469443f58b6e4e2b5, type: 2} + - m_RefCount: 1 + m_Data: {fileID: 11400000, guid: 78df622e549350e419f33628d6de5bec, type: 2} + - m_RefCount: 1 + m_Data: {fileID: 11400000, guid: abce38af38bd21149b37233db41fc261, type: 2} + - m_RefCount: 1 + m_Data: {fileID: 11400000, guid: 399a27777263f1345bbdcd02ac25a63e, type: 2} + - m_RefCount: 1 + m_Data: {fileID: 11400000, guid: 7acbe6c401ddccd448f62bb0b4a4d679, type: 2} + - m_RefCount: 1 + m_Data: {fileID: 11400000, guid: decde4ccadd99f044a6a9f09c51a9077, type: 2} + - m_RefCount: 1 + m_Data: {fileID: 11400000, guid: 8938aa05be2283741866f9cb212cbfb2, type: 2} + - m_RefCount: 1 + m_Data: {fileID: 11400000, guid: 45520d2e837024f4583428bb378123a0, type: 2} + - m_RefCount: 1 + m_Data: {fileID: 11400000, guid: 8a1fcfba9dfd3e143ac55d8750b645fe, type: 2} + - m_RefCount: 1 + m_Data: {fileID: 11400000, guid: 34005f47333a0b54dbb9fdfbadac413b, type: 2} + - m_RefCount: 1 + m_Data: {fileID: 11400000, guid: 12de738e24082e946b800cc99f9c8299, type: 2} + - m_RefCount: 1 + m_Data: {fileID: 11400000, guid: 184d2ff80e48f4b4d82d61458a6756c1, type: 2} + - m_RefCount: 1 + m_Data: {fileID: 11400000, guid: f04a8e256fee52940a0cb96ea72163a0, type: 2} + - m_RefCount: 1 + m_Data: {fileID: 11400000, guid: 60bfa98e2ec021b4abd020ea77da127b, type: 2} + - m_RefCount: 1 + m_Data: {fileID: 11400000, guid: e9b00c5e96e845a46abe228c5988ced7, type: 2} + - m_RefCount: 1 + m_Data: {fileID: 11400000, guid: e5cdf30eaad5fce488688512e6e582ae, type: 2} + - m_RefCount: 1 + m_Data: {fileID: 11400000, guid: 68f8bdbdf51aa454f9412df899ac312f, type: 2} + - m_RefCount: 1 + m_Data: {fileID: 11400000, guid: 471a1ffc9aeb1c04bb8bbe643f7ac9a1, type: 2} + - m_RefCount: 1 + m_Data: {fileID: 11400000, guid: bfe73897392c1ee49b9d6dee3d174820, type: 2} + - m_RefCount: 1 + m_Data: {fileID: 11400000, guid: d0be3b3859731d54f808b245d6b107ab, type: 2} + - m_RefCount: 1 + m_Data: {fileID: 11400000, guid: 0acf0b0434f3b5f4caef76921c697191, type: 2} + - m_RefCount: 1 + m_Data: {fileID: 11400000, guid: 590bc9cada8177b488e10eba7b11719e, type: 2} + - m_RefCount: 1 + m_Data: {fileID: 11400000, guid: ffa3bf238b4515344b377b5da194f7c8, type: 2} + - m_RefCount: 1 + m_Data: {fileID: 11400000, guid: b7af5ab325f607041a625895c85c22fb, type: 2} + - m_RefCount: 1 + m_Data: {fileID: 11400000, guid: 710a5785df889584887ce56dc689b5b7, type: 2} + - m_RefCount: 1 + m_Data: {fileID: 11400000, guid: 75f9c18ba8ecb2d4b98ae64f7340474a, type: 2} + - m_RefCount: 1 + m_Data: {fileID: 11400000, guid: 26e1dd6488abfb34584a76d2ee5021de, type: 2} + - m_RefCount: 1 + m_Data: {fileID: 11400000, guid: fc6124d66efc3524f8f35e4468c78100, type: 2} + - m_RefCount: 1 + m_Data: {fileID: 11400000, guid: 8b5f706aa5654f94c874ae8e383f3dfc, type: 2} + - m_RefCount: 1 + m_Data: {fileID: 11400000, guid: 1d382a2702adb7f4ebc3ca6a5110afb4, type: 2} + - m_RefCount: 1 + m_Data: {fileID: 11400000, guid: 482ddd0787e8e6845bf2715684b0eba3, type: 2} + - m_RefCount: 1 + m_Data: {fileID: 11400000, guid: 256c43bc5f4ab714eab7a6f360254d43, type: 2} + - m_RefCount: 1 + m_Data: {fileID: 11400000, guid: 92968a7b2c7327e4682d7d69d2082e75, type: 2} + - m_RefCount: 1 + m_Data: {fileID: 11400000, guid: 5cfa31067f8707e42874ef4f4ba4de79, type: 2} + - m_RefCount: 1 + m_Data: {fileID: 11400000, guid: 19db1f411a19ff44ab9a0b06e1811747, type: 2} + - m_RefCount: 1 + m_Data: {fileID: 11400000, guid: 1204e5a6fef20cb4bbc137b1b66869ab, type: 2} + - m_RefCount: 1 + m_Data: {fileID: 11400000, guid: 5a946ab25fbde3e4c840ad3f178f6137, type: 2} + - m_RefCount: 1 + m_Data: {fileID: 11400000, guid: ba2773a24a8457f44b4706314bd25a73, type: 2} + - m_RefCount: 1 + m_Data: {fileID: 11400000, guid: 048dff00fd5a17946a7ba7b5541a2eb6, type: 2} + - m_RefCount: 1 + m_Data: {fileID: 11400000, guid: 3093f1e128519824e844277fb6d3402a, type: 2} + - m_RefCount: 1 + m_Data: {fileID: 11400000, guid: 5387ff301b194ea46a0138787c1906d2, type: 2} + - m_RefCount: 1 + m_Data: {fileID: 11400000, guid: 1da059d2ebc6f354494f465adfaaedb8, type: 2} + - m_RefCount: 1 + m_Data: {fileID: 11400000, guid: 8fb6991985720cc408646a54158626f8, type: 2} + - m_RefCount: 1 + m_Data: {fileID: 11400000, guid: a31dae3bd70797649a110851518ca16f, type: 2} + - m_RefCount: 1 + m_Data: {fileID: 11400000, guid: f6adb266f61c3d44fb326964d0f44ea9, type: 2} + - m_RefCount: 1 + m_Data: {fileID: 11400000, guid: c366a769491273347aa4de6d628d37af, type: 2} + - m_RefCount: 1 + m_Data: {fileID: 11400000, guid: ade8c7170fa6c0145a49e498ac98644e, type: 2} + - m_RefCount: 1 + m_Data: {fileID: 11400000, guid: 75e564577f6e73441be6bf6e336d07f7, type: 2} + - m_RefCount: 1 + m_Data: {fileID: 11400000, guid: 2411437a6c35d8944bc9bea58d0e1c09, type: 2} + - m_RefCount: 1 + m_Data: {fileID: 11400000, guid: a1643267c869f4a4b9c3f1aabc8156a2, type: 2} + - m_RefCount: 1 + m_Data: {fileID: 11400000, guid: 59f56605b41b89c46bab93964ba3efd7, type: 2} + - m_RefCount: 1 + m_Data: {fileID: 11400000, guid: ef1e419e402ec864dbe97f93e50fc7bb, type: 2} + - m_RefCount: 1 + m_Data: {fileID: 11400000, guid: b521b926ffbbcaf42a960d4b96b8e0c9, type: 2} + - m_RefCount: 1 + m_Data: {fileID: 11400000, guid: e61fae997815a4a4b8d2e6cc611327f8, type: 2} + - m_RefCount: 1 + m_Data: {fileID: 11400000, guid: 69d9c223832b9954c8371363f9fe9d72, type: 2} + - m_RefCount: 1 + m_Data: {fileID: 11400000, guid: 81dbd4adc8f925741b8d2aee5f076335, type: 2} + - m_RefCount: 1 + m_Data: {fileID: 11400000, guid: 9b83c35937e6ba542b5162858b841d57, type: 2} + - m_RefCount: 1 + m_Data: {fileID: 11400000, guid: 37c818adc58b19a4a9e160f4e0c289d1, type: 2} + - m_RefCount: 1 + m_Data: {fileID: 11400000, guid: 725ac7f68af6b7646922285011a3f343, type: 2} + - m_RefCount: 1 + m_Data: {fileID: 11400000, guid: 89f154c4c20759a43b960c725c916389, type: 2} + - m_RefCount: 1 + m_Data: {fileID: 11400000, guid: 2451da60eae72804ea1516793136c130, type: 2} + - m_RefCount: 1 + m_Data: {fileID: 11400000, guid: 008ec0ae646e84841a3bcd3753ab7fa3, type: 2} + - m_RefCount: 1 + m_Data: {fileID: 11400000, guid: c3b5291c318038e4bbb6f1b39772db8a, type: 2} + - m_RefCount: 1 + m_Data: {fileID: 11400000, guid: 8607fee69c003254086318ea700356bc, type: 2} + - m_RefCount: 1 + m_Data: {fileID: 11400000, guid: 3342c11b5fc52bd4c89d97026d53d56b, type: 2} + - m_RefCount: 1 + m_Data: {fileID: 11400000, guid: daf96d2a3dc80a244b41477f24e9c9f3, type: 2} + - m_RefCount: 1 + m_Data: {fileID: 11400000, guid: 1d79f2a2dd2a98142ab3dee5022b6812, type: 2} + - m_RefCount: 1 + m_Data: {fileID: 11400000, guid: d4ff608324b75a24693fb931623e9d02, type: 2} + - m_RefCount: 1 + m_Data: {fileID: 11400000, guid: 731304ae8a725e34a8c382e0b0dd3467, type: 2} + - m_RefCount: 1 + m_Data: {fileID: 11400000, guid: 794cb64b1bb867c438f45faf4c809e60, type: 2} + - m_RefCount: 1 + m_Data: {fileID: 11400000, guid: 6242f92cd02e8a84093a2c3aaa0141f8, type: 2} + - m_RefCount: 1 + m_Data: {fileID: 11400000, guid: 80e70deb48d53a241be6ed8f133a67db, type: 2} + m_TileSpriteArray: + - m_RefCount: 1 + m_Data: {fileID: -509989529, guid: 0a806eb0d25e11c48b5dee57fb9339b5, type: 3} + - m_RefCount: 1 + m_Data: {fileID: -1085996522, guid: 0a806eb0d25e11c48b5dee57fb9339b5, type: 3} + - m_RefCount: 1 + m_Data: {fileID: 164431376, guid: 0a806eb0d25e11c48b5dee57fb9339b5, type: 3} + - m_RefCount: 1 + m_Data: {fileID: 326241045, guid: 0a806eb0d25e11c48b5dee57fb9339b5, type: 3} + - m_RefCount: 1 + m_Data: {fileID: -345571141, guid: 0a806eb0d25e11c48b5dee57fb9339b5, type: 3} + - m_RefCount: 1 + m_Data: {fileID: 2094817791, guid: 0a806eb0d25e11c48b5dee57fb9339b5, type: 3} + - m_RefCount: 1 + m_Data: {fileID: 198299372, guid: 0a806eb0d25e11c48b5dee57fb9339b5, type: 3} + - m_RefCount: 1 + m_Data: {fileID: -650063954, guid: 0a806eb0d25e11c48b5dee57fb9339b5, type: 3} + - m_RefCount: 1 + m_Data: {fileID: -1536037944, guid: 0a806eb0d25e11c48b5dee57fb9339b5, type: 3} + - m_RefCount: 1 + m_Data: {fileID: 136035454, guid: 0a806eb0d25e11c48b5dee57fb9339b5, type: 3} + - m_RefCount: 1 + m_Data: {fileID: 1460478432, guid: 0a806eb0d25e11c48b5dee57fb9339b5, type: 3} + - m_RefCount: 1 + m_Data: {fileID: 586641414, guid: 0a806eb0d25e11c48b5dee57fb9339b5, type: 3} + - m_RefCount: 1 + m_Data: {fileID: 1613682724, guid: 0a806eb0d25e11c48b5dee57fb9339b5, type: 3} + - m_RefCount: 1 + m_Data: {fileID: 489946030, guid: 0a806eb0d25e11c48b5dee57fb9339b5, type: 3} + - m_RefCount: 1 + m_Data: {fileID: -1758251442, guid: 0a806eb0d25e11c48b5dee57fb9339b5, type: 3} + - m_RefCount: 1 + m_Data: {fileID: -432912164, guid: 0a806eb0d25e11c48b5dee57fb9339b5, type: 3} + - m_RefCount: 1 + m_Data: {fileID: 1580949469, guid: 0a806eb0d25e11c48b5dee57fb9339b5, type: 3} + - m_RefCount: 1 + m_Data: {fileID: 2016605797, guid: 0a806eb0d25e11c48b5dee57fb9339b5, type: 3} + - m_RefCount: 1 + m_Data: {fileID: -1798427165, guid: 0a806eb0d25e11c48b5dee57fb9339b5, type: 3} + - m_RefCount: 1 + m_Data: {fileID: 1917838317, guid: 0a806eb0d25e11c48b5dee57fb9339b5, type: 3} + - m_RefCount: 1 + m_Data: {fileID: 1926522667, guid: 0a806eb0d25e11c48b5dee57fb9339b5, type: 3} + - m_RefCount: 1 + m_Data: {fileID: -672668870, guid: 0a806eb0d25e11c48b5dee57fb9339b5, type: 3} + - m_RefCount: 1 + m_Data: {fileID: -2113988543, guid: 0a806eb0d25e11c48b5dee57fb9339b5, type: 3} + - m_RefCount: 1 + m_Data: {fileID: 1655838605, guid: 0a806eb0d25e11c48b5dee57fb9339b5, type: 3} + - m_RefCount: 1 + m_Data: {fileID: 43680089, guid: 0a806eb0d25e11c48b5dee57fb9339b5, type: 3} + - m_RefCount: 1 + m_Data: {fileID: 1995419026, guid: 0a806eb0d25e11c48b5dee57fb9339b5, type: 3} + - m_RefCount: 1 + m_Data: {fileID: 1277448032, guid: 0a806eb0d25e11c48b5dee57fb9339b5, type: 3} + - m_RefCount: 1 + m_Data: {fileID: -412475550, guid: 0a806eb0d25e11c48b5dee57fb9339b5, type: 3} + - m_RefCount: 1 + m_Data: {fileID: 1065039898, guid: 0a806eb0d25e11c48b5dee57fb9339b5, type: 3} + - m_RefCount: 1 + m_Data: {fileID: 1956140949, guid: 0a806eb0d25e11c48b5dee57fb9339b5, type: 3} + - m_RefCount: 1 + m_Data: {fileID: -164313747, guid: 0a806eb0d25e11c48b5dee57fb9339b5, type: 3} + - m_RefCount: 1 + m_Data: {fileID: 1376823085, guid: 0a806eb0d25e11c48b5dee57fb9339b5, type: 3} + - m_RefCount: 1 + m_Data: {fileID: 2009541612, guid: 0a806eb0d25e11c48b5dee57fb9339b5, type: 3} + - m_RefCount: 1 + m_Data: {fileID: -1028069899, guid: 0a806eb0d25e11c48b5dee57fb9339b5, type: 3} + - m_RefCount: 1 + m_Data: {fileID: 1099301450, guid: 0a806eb0d25e11c48b5dee57fb9339b5, type: 3} + - m_RefCount: 1 + m_Data: {fileID: 81769002, guid: 0a806eb0d25e11c48b5dee57fb9339b5, type: 3} + - m_RefCount: 1 + m_Data: {fileID: 1050266288, guid: 0a806eb0d25e11c48b5dee57fb9339b5, type: 3} + - m_RefCount: 1 + m_Data: {fileID: 1708775233, guid: 0a806eb0d25e11c48b5dee57fb9339b5, type: 3} + - m_RefCount: 1 + m_Data: {fileID: 660103562, guid: 0a806eb0d25e11c48b5dee57fb9339b5, type: 3} + - m_RefCount: 1 + m_Data: {fileID: 1397179706, guid: 0a806eb0d25e11c48b5dee57fb9339b5, type: 3} + - m_RefCount: 1 + m_Data: {fileID: 1158887391, guid: 0a806eb0d25e11c48b5dee57fb9339b5, type: 3} + - m_RefCount: 1 + m_Data: {fileID: 615089749, guid: 0a806eb0d25e11c48b5dee57fb9339b5, type: 3} + - m_RefCount: 1 + m_Data: {fileID: -777615196, guid: 0a806eb0d25e11c48b5dee57fb9339b5, type: 3} + - m_RefCount: 1 + m_Data: {fileID: 197855793, guid: 0a806eb0d25e11c48b5dee57fb9339b5, type: 3} + - m_RefCount: 1 + m_Data: {fileID: -1396940042, guid: 0a806eb0d25e11c48b5dee57fb9339b5, type: 3} + - m_RefCount: 1 + m_Data: {fileID: 1802662682, guid: 0a806eb0d25e11c48b5dee57fb9339b5, type: 3} + - m_RefCount: 1 + m_Data: {fileID: -549131558, guid: 0a806eb0d25e11c48b5dee57fb9339b5, type: 3} + - m_RefCount: 1 + m_Data: {fileID: -1668792333, guid: 0a806eb0d25e11c48b5dee57fb9339b5, type: 3} + - m_RefCount: 1 + m_Data: {fileID: -395624179, guid: 0a806eb0d25e11c48b5dee57fb9339b5, type: 3} + - m_RefCount: 1 + m_Data: {fileID: 453119210, guid: 0a806eb0d25e11c48b5dee57fb9339b5, type: 3} + - m_RefCount: 1 + m_Data: {fileID: -1669469920, guid: 0a806eb0d25e11c48b5dee57fb9339b5, type: 3} + - m_RefCount: 1 + m_Data: {fileID: 79250496, guid: 0a806eb0d25e11c48b5dee57fb9339b5, type: 3} + - m_RefCount: 1 + m_Data: {fileID: 2075798291, guid: 0a806eb0d25e11c48b5dee57fb9339b5, type: 3} + - m_RefCount: 1 + m_Data: {fileID: -282376894, guid: 0a806eb0d25e11c48b5dee57fb9339b5, type: 3} + - m_RefCount: 1 + m_Data: {fileID: 1862703900, guid: 0a806eb0d25e11c48b5dee57fb9339b5, type: 3} + - m_RefCount: 1 + m_Data: {fileID: 536411375, guid: 0a806eb0d25e11c48b5dee57fb9339b5, type: 3} + - m_RefCount: 1 + m_Data: {fileID: 796962211, guid: 0a806eb0d25e11c48b5dee57fb9339b5, type: 3} + - m_RefCount: 1 + m_Data: {fileID: 1661568666, guid: 0a806eb0d25e11c48b5dee57fb9339b5, type: 3} + - m_RefCount: 1 + m_Data: {fileID: -2046453881, guid: 0a806eb0d25e11c48b5dee57fb9339b5, type: 3} + - m_RefCount: 1 + m_Data: {fileID: 2038936553, guid: 0a806eb0d25e11c48b5dee57fb9339b5, type: 3} + - m_RefCount: 1 + m_Data: {fileID: 1660072189, guid: 0a806eb0d25e11c48b5dee57fb9339b5, type: 3} + - m_RefCount: 1 + m_Data: {fileID: -1999030480, guid: 0a806eb0d25e11c48b5dee57fb9339b5, type: 3} + - m_RefCount: 1 + m_Data: {fileID: -62922940, guid: 0a806eb0d25e11c48b5dee57fb9339b5, type: 3} + - m_RefCount: 1 + m_Data: {fileID: 154182392, guid: 0a806eb0d25e11c48b5dee57fb9339b5, type: 3} + - m_RefCount: 1 + m_Data: {fileID: -38314673, guid: 0a806eb0d25e11c48b5dee57fb9339b5, type: 3} + - m_RefCount: 1 + m_Data: {fileID: 442919366, guid: 0a806eb0d25e11c48b5dee57fb9339b5, type: 3} + - m_RefCount: 1 + m_Data: {fileID: -521188253, guid: 0a806eb0d25e11c48b5dee57fb9339b5, type: 3} + - m_RefCount: 1 + m_Data: {fileID: -428477728, guid: 0a806eb0d25e11c48b5dee57fb9339b5, type: 3} + - m_RefCount: 1 + m_Data: {fileID: -2045571822, guid: 0a806eb0d25e11c48b5dee57fb9339b5, type: 3} + - m_RefCount: 1 + m_Data: {fileID: -839279710, guid: 0a806eb0d25e11c48b5dee57fb9339b5, type: 3} + - m_RefCount: 1 + m_Data: {fileID: 2090772569, guid: 0a806eb0d25e11c48b5dee57fb9339b5, type: 3} + - m_RefCount: 1 + m_Data: {fileID: -1297728808, guid: 0a806eb0d25e11c48b5dee57fb9339b5, type: 3} + - m_RefCount: 1 + m_Data: {fileID: -1473822626, guid: 0a806eb0d25e11c48b5dee57fb9339b5, type: 3} + - m_RefCount: 1 + m_Data: {fileID: -1760836496, guid: 0a806eb0d25e11c48b5dee57fb9339b5, type: 3} + - m_RefCount: 1 + m_Data: {fileID: 1547048006, guid: 0a806eb0d25e11c48b5dee57fb9339b5, type: 3} + - m_RefCount: 1 + m_Data: {fileID: 613834084, guid: 0a806eb0d25e11c48b5dee57fb9339b5, type: 3} + - m_RefCount: 1 + m_Data: {fileID: 1270900073, guid: 0a806eb0d25e11c48b5dee57fb9339b5, type: 3} + - m_RefCount: 1 + m_Data: {fileID: 2137237813, guid: 0a806eb0d25e11c48b5dee57fb9339b5, type: 3} + - m_RefCount: 1 + m_Data: {fileID: 1460241374, guid: 0a806eb0d25e11c48b5dee57fb9339b5, type: 3} + - m_RefCount: 1 + m_Data: {fileID: -1614669064, guid: 0a806eb0d25e11c48b5dee57fb9339b5, type: 3} + - m_RefCount: 1 + m_Data: {fileID: 1642675801, guid: 0a806eb0d25e11c48b5dee57fb9339b5, type: 3} + - m_RefCount: 1 + m_Data: {fileID: -953407387, guid: 0a806eb0d25e11c48b5dee57fb9339b5, type: 3} + - m_RefCount: 1 + m_Data: {fileID: -1181825705, guid: 0a806eb0d25e11c48b5dee57fb9339b5, type: 3} + - m_RefCount: 1 + m_Data: {fileID: -1892450189, guid: 0a806eb0d25e11c48b5dee57fb9339b5, type: 3} + - m_RefCount: 1 + m_Data: {fileID: -1649980043, guid: 0a806eb0d25e11c48b5dee57fb9339b5, type: 3} + - m_RefCount: 1 + m_Data: {fileID: 811441136, guid: 0a806eb0d25e11c48b5dee57fb9339b5, type: 3} + - m_RefCount: 1 + m_Data: {fileID: 207130727, guid: 0a806eb0d25e11c48b5dee57fb9339b5, type: 3} + - m_RefCount: 1 + m_Data: {fileID: -857797333, guid: 0a806eb0d25e11c48b5dee57fb9339b5, type: 3} + - m_RefCount: 1 + m_Data: {fileID: 306057277, guid: 0a806eb0d25e11c48b5dee57fb9339b5, type: 3} + - m_RefCount: 1 + m_Data: {fileID: -1558256172, guid: 0a806eb0d25e11c48b5dee57fb9339b5, type: 3} + - m_RefCount: 1 + m_Data: {fileID: 1466099207, guid: 0a806eb0d25e11c48b5dee57fb9339b5, type: 3} + m_TileMatrixArray: + - m_RefCount: 91 + m_Data: + e00: 1 + e01: 0 + e02: 0 + e03: 0 + e10: 0 + e11: 1 + e12: 0 + e13: 0 + e20: 0 + e21: 0 + e22: 1 + e23: 0 + e30: 0 + e31: 0 + e32: 0 + e33: 1 + m_TileColorArray: + - m_RefCount: 91 + m_Data: {r: 1, g: 1, b: 1, a: 1} + m_TileObjectToInstantiateArray: [] + m_AnimationFrameRate: 1 + m_Color: {r: 1, g: 1, b: 1, a: 1} + m_Origin: {x: -2, y: -14, z: 0} + m_Size: {x: 7, y: 16, z: 1} + m_TileAnchor: {x: 0.5, y: 0.5, z: 0} + m_TileOrientation: 0 + m_TileOrientationMatrix: + e00: 1 + e01: 0 + e02: 0 + e03: 0 + e10: 0 + e11: 1 + e12: 0 + e13: 0 + e20: 0 + e21: 0 + e22: 1 + e23: 0 + e30: 0 + e31: 0 + e32: 0 + e33: 1 +--- !u!483693784 &2026011780236248997 +TilemapRenderer: + m_ObjectHideFlags: 0 + m_CorrespondingSourceObject: {fileID: 0} + m_PrefabInstance: {fileID: 0} + m_PrefabAsset: {fileID: 0} + m_GameObject: {fileID: 910361770656200846} + m_Enabled: 1 + m_CastShadows: 0 + m_ReceiveShadows: 0 + m_DynamicOccludee: 0 + m_StaticShadowCaster: 0 + m_MotionVectors: 1 + m_LightProbeUsage: 0 + m_ReflectionProbeUsage: 0 + m_RayTracingMode: 0 + m_RayTraceProcedural: 0 + m_RenderingLayerMask: 1 + m_RendererPriority: 0 + m_Materials: + - {fileID: 10754, guid: 0000000000000000f000000000000000, type: 0} + m_StaticBatchInfo: + firstSubMesh: 0 + subMeshCount: 0 + m_StaticBatchRoot: {fileID: 0} + m_ProbeAnchor: {fileID: 0} + m_LightProbeVolumeOverride: {fileID: 0} + m_ScaleInLightmap: 1 + m_ReceiveGI: 1 + m_PreserveUVs: 0 + m_IgnoreNormalsForChartDetection: 0 + m_ImportantGI: 0 + m_StitchLightmapSeams: 1 + m_SelectedEditorRenderState: 0 + m_MinimumChartSize: 4 + m_AutoUVMaxDistance: 0.5 + m_AutoUVMaxAngle: 89 + m_LightmapParameters: {fileID: 0} + m_SortingLayerID: 0 + m_SortingLayer: 0 + m_SortingOrder: 0 + m_ChunkSize: {x: 32, y: 32, z: 32} + m_ChunkCullingBounds: {x: 0, y: 0, z: 0} + m_MaxChunkCount: 16 + m_MaxFrameAge: 16 + m_SortOrder: 0 + m_Mode: 0 + m_DetectChunkCullingBounds: 0 + m_MaskInteraction: 0 +--- !u!1 &5977100872266195667 +GameObject: + m_ObjectHideFlags: 0 + m_CorrespondingSourceObject: {fileID: 0} + m_PrefabInstance: {fileID: 0} + m_PrefabAsset: {fileID: 0} + serializedVersion: 6 + m_Component: + - component: {fileID: 1100878670463798578} + - component: {fileID: 2924924599886432406} + m_Layer: 0 + m_Name: Dungeon + m_TagString: Untagged + m_Icon: {fileID: 0} + m_NavMeshLayer: 0 + m_StaticEditorFlags: 0 + m_IsActive: 1 +--- !u!4 &1100878670463798578 +Transform: + m_ObjectHideFlags: 0 + m_CorrespondingSourceObject: {fileID: 0} + m_PrefabInstance: {fileID: 0} + m_PrefabAsset: {fileID: 0} + m_GameObject: {fileID: 5977100872266195667} + m_LocalRotation: {x: 0, y: 0, z: 0, w: 1} + m_LocalPosition: {x: 0, y: 0, z: 0} + m_LocalScale: {x: 1, y: 1, z: 1} + m_ConstrainProportionsScale: 0 + m_Children: + - {fileID: 1644156093156590069} + m_Father: {fileID: 0} + m_RootOrder: 0 + m_LocalEulerAnglesHint: {x: 0, y: 0, z: 0} +--- !u!156049354 &2924924599886432406 +Grid: + m_ObjectHideFlags: 0 + m_CorrespondingSourceObject: {fileID: 0} + m_PrefabInstance: {fileID: 0} + m_PrefabAsset: {fileID: 0} + m_GameObject: {fileID: 5977100872266195667} + m_Enabled: 1 + m_CellSize: {x: 1, y: 1, z: 0} + m_CellGap: {x: 0, y: 0, z: 0} + m_CellLayout: 0 + m_CellSwizzle: 0 +--- !u!114 &1769567913174593949 +MonoBehaviour: + m_ObjectHideFlags: 0 + m_CorrespondingSourceObject: {fileID: 0} + m_PrefabInstance: {fileID: 0} + m_PrefabAsset: {fileID: 0} + m_GameObject: {fileID: 0} + m_Enabled: 1 + m_EditorHideFlags: 0 + m_Script: {fileID: 12395, guid: 0000000000000000e000000000000000, type: 0} + m_Name: Palette Settings + m_EditorClassIdentifier: + cellSizing: 0 + m_TransparencySortMode: 0 + m_TransparencySortAxis: {x: 0, y: 0, z: 1} diff --git a/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon.prefab.meta b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon.prefab.meta new file mode 100644 index 0000000000000000000000000000000000000000..149e7712db0fd865eb0237adccf4683289db1627 --- /dev/null +++ b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon.prefab.meta @@ -0,0 +1,7 @@ +fileFormatVersion: 2 +guid: ee6cef3e461225145bf80571ff1f96be +PrefabImporter: + externalObjects: {} + userData: + assetBundleName: + assetBundleVariant: diff --git a/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_0.asset b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_0.asset new file mode 100644 index 0000000000000000000000000000000000000000..6913e3705f2753e3fc1286b79287358e5597273d --- /dev/null +++ b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_0.asset @@ -0,0 +1,36 @@ +%YAML 1.1 +%TAG !u! tag:unity3d.com,2011: +--- !u!114 &11400000 +MonoBehaviour: + m_ObjectHideFlags: 0 + m_CorrespondingSourceObject: {fileID: 0} + m_PrefabInstance: {fileID: 0} + m_PrefabAsset: {fileID: 0} + m_GameObject: {fileID: 0} + m_Enabled: 1 + m_EditorHideFlags: 0 + m_Script: {fileID: 13312, guid: 0000000000000000e000000000000000, type: 0} + m_Name: Dungeon_0 + m_EditorClassIdentifier: + m_Sprite: {fileID: -509989529, guid: 0a806eb0d25e11c48b5dee57fb9339b5, type: 3} + m_Color: {r: 1, g: 1, b: 1, a: 1} + m_Transform: + e00: 1 + e01: 0 + e02: 0 + e03: 0 + e10: 0 + e11: 1 + e12: 0 + e13: 0 + e20: 0 + e21: 0 + e22: 1 + e23: 0 + e30: 0 + e31: 0 + e32: 0 + e33: 1 + m_InstancedGameObject: {fileID: 0} + m_Flags: 1 + m_ColliderType: 1 diff --git a/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_0.asset.meta b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_0.asset.meta new file mode 100644 index 0000000000000000000000000000000000000000..9aa1e76d870586f9d1d4d3f0d8df1d550525a62c --- /dev/null +++ b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_0.asset.meta @@ -0,0 +1,8 @@ +fileFormatVersion: 2 +guid: 91f123c55b83ed54ea8387bbb6250b9c +NativeFormatImporter: + externalObjects: {} + mainObjectFileID: 11400000 + userData: + assetBundleName: + assetBundleVariant: diff --git a/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_1.asset b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_1.asset new file mode 100644 index 0000000000000000000000000000000000000000..23e5505f9f2567802d6d2ea663cb36cd4f3f17ab --- /dev/null +++ b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_1.asset @@ -0,0 +1,36 @@ +%YAML 1.1 +%TAG !u! tag:unity3d.com,2011: +--- !u!114 &11400000 +MonoBehaviour: + m_ObjectHideFlags: 0 + m_CorrespondingSourceObject: {fileID: 0} + m_PrefabInstance: {fileID: 0} + m_PrefabAsset: {fileID: 0} + m_GameObject: {fileID: 0} + m_Enabled: 1 + m_EditorHideFlags: 0 + m_Script: {fileID: 13312, guid: 0000000000000000e000000000000000, type: 0} + m_Name: Dungeon_1 + m_EditorClassIdentifier: + m_Sprite: {fileID: -1085996522, guid: 0a806eb0d25e11c48b5dee57fb9339b5, type: 3} + m_Color: {r: 1, g: 1, b: 1, a: 1} + m_Transform: + e00: 1 + e01: 0 + e02: 0 + e03: 0 + e10: 0 + e11: 1 + e12: 0 + e13: 0 + e20: 0 + e21: 0 + e22: 1 + e23: 0 + e30: 0 + e31: 0 + e32: 0 + e33: 1 + m_InstancedGameObject: {fileID: 0} + m_Flags: 1 + m_ColliderType: 1 diff --git a/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_1.asset.meta b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_1.asset.meta new file mode 100644 index 0000000000000000000000000000000000000000..2a1cec41296b0f9d2514b57085b326727cef7b19 --- /dev/null +++ b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_1.asset.meta @@ -0,0 +1,8 @@ +fileFormatVersion: 2 +guid: 257657ca820b66741ab4695fbb49f83e +NativeFormatImporter: + externalObjects: {} + mainObjectFileID: 11400000 + userData: + assetBundleName: + assetBundleVariant: diff --git a/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_10.asset b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_10.asset new file mode 100644 index 0000000000000000000000000000000000000000..17825849b1d7882c0d93ce04a148bbd719a73c21 --- /dev/null +++ b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_10.asset @@ -0,0 +1,36 @@ +%YAML 1.1 +%TAG !u! tag:unity3d.com,2011: +--- !u!114 &11400000 +MonoBehaviour: + m_ObjectHideFlags: 0 + m_CorrespondingSourceObject: {fileID: 0} + m_PrefabInstance: {fileID: 0} + m_PrefabAsset: {fileID: 0} + m_GameObject: {fileID: 0} + m_Enabled: 1 + m_EditorHideFlags: 0 + m_Script: {fileID: 13312, guid: 0000000000000000e000000000000000, type: 0} + m_Name: Dungeon_10 + m_EditorClassIdentifier: + m_Sprite: {fileID: 1460478432, guid: 0a806eb0d25e11c48b5dee57fb9339b5, type: 3} + m_Color: {r: 1, g: 1, b: 1, a: 1} + m_Transform: + e00: 1 + e01: 0 + e02: 0 + e03: 0 + e10: 0 + e11: 1 + e12: 0 + e13: 0 + e20: 0 + e21: 0 + e22: 1 + e23: 0 + e30: 0 + e31: 0 + e32: 0 + e33: 1 + m_InstancedGameObject: {fileID: 0} + m_Flags: 1 + m_ColliderType: 1 diff --git a/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_10.asset.meta b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_10.asset.meta new file mode 100644 index 0000000000000000000000000000000000000000..9f4f1f78e1119e103a86a15ff77e9745be3c18db --- /dev/null +++ b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_10.asset.meta @@ -0,0 +1,8 @@ +fileFormatVersion: 2 +guid: e56c374d1cab17f4aabbff2d5aa48bf6 +NativeFormatImporter: + externalObjects: {} + mainObjectFileID: 11400000 + userData: + assetBundleName: + assetBundleVariant: diff --git a/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_11.asset b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_11.asset new file mode 100644 index 0000000000000000000000000000000000000000..6678f8b907db244a7bbe3c9f7616903f34750d6b --- /dev/null +++ b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_11.asset @@ -0,0 +1,36 @@ +%YAML 1.1 +%TAG !u! tag:unity3d.com,2011: +--- !u!114 &11400000 +MonoBehaviour: + m_ObjectHideFlags: 0 + m_CorrespondingSourceObject: {fileID: 0} + m_PrefabInstance: {fileID: 0} + m_PrefabAsset: {fileID: 0} + m_GameObject: {fileID: 0} + m_Enabled: 1 + m_EditorHideFlags: 0 + m_Script: {fileID: 13312, guid: 0000000000000000e000000000000000, type: 0} + m_Name: Dungeon_11 + m_EditorClassIdentifier: + m_Sprite: {fileID: 586641414, guid: 0a806eb0d25e11c48b5dee57fb9339b5, type: 3} + m_Color: {r: 1, g: 1, b: 1, a: 1} + m_Transform: + e00: 1 + e01: 0 + e02: 0 + e03: 0 + e10: 0 + e11: 1 + e12: 0 + e13: 0 + e20: 0 + e21: 0 + e22: 1 + e23: 0 + e30: 0 + e31: 0 + e32: 0 + e33: 1 + m_InstancedGameObject: {fileID: 0} + m_Flags: 1 + m_ColliderType: 1 diff --git a/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_11.asset.meta b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_11.asset.meta new file mode 100644 index 0000000000000000000000000000000000000000..e55e948c41d8e12a1db53bb92e46f5320ee1595e --- /dev/null +++ b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_11.asset.meta @@ -0,0 +1,8 @@ +fileFormatVersion: 2 +guid: d594b739b3e29ee468ebc200a8b966a4 +NativeFormatImporter: + externalObjects: {} + mainObjectFileID: 11400000 + userData: + assetBundleName: + assetBundleVariant: diff --git a/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_12.asset b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_12.asset new file mode 100644 index 0000000000000000000000000000000000000000..d0d385c690781c478797f51676e740046e55be46 --- /dev/null +++ b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_12.asset @@ -0,0 +1,36 @@ +%YAML 1.1 +%TAG !u! tag:unity3d.com,2011: +--- !u!114 &11400000 +MonoBehaviour: + m_ObjectHideFlags: 0 + m_CorrespondingSourceObject: {fileID: 0} + m_PrefabInstance: {fileID: 0} + m_PrefabAsset: {fileID: 0} + m_GameObject: {fileID: 0} + m_Enabled: 1 + m_EditorHideFlags: 0 + m_Script: {fileID: 13312, guid: 0000000000000000e000000000000000, type: 0} + m_Name: Dungeon_12 + m_EditorClassIdentifier: + m_Sprite: {fileID: 1613682724, guid: 0a806eb0d25e11c48b5dee57fb9339b5, type: 3} + m_Color: {r: 1, g: 1, b: 1, a: 1} + m_Transform: + e00: 1 + e01: 0 + e02: 0 + e03: 0 + e10: 0 + e11: 1 + e12: 0 + e13: 0 + e20: 0 + e21: 0 + e22: 1 + e23: 0 + e30: 0 + e31: 0 + e32: 0 + e33: 1 + m_InstancedGameObject: {fileID: 0} + m_Flags: 1 + m_ColliderType: 1 diff --git a/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_12.asset.meta b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_12.asset.meta new file mode 100644 index 0000000000000000000000000000000000000000..2355bca7deddce9741b055f92cda0f8b970532fb --- /dev/null +++ b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_12.asset.meta @@ -0,0 +1,8 @@ +fileFormatVersion: 2 +guid: f9f70339e2318c94988b284e7fb563e1 +NativeFormatImporter: + externalObjects: {} + mainObjectFileID: 11400000 + userData: + assetBundleName: + assetBundleVariant: diff --git a/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_13.asset b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_13.asset new file mode 100644 index 0000000000000000000000000000000000000000..9386d66c23150dca3e2e479eab7bf9eb9da0c115 --- /dev/null +++ b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_13.asset @@ -0,0 +1,36 @@ +%YAML 1.1 +%TAG !u! tag:unity3d.com,2011: +--- !u!114 &11400000 +MonoBehaviour: + m_ObjectHideFlags: 0 + m_CorrespondingSourceObject: {fileID: 0} + m_PrefabInstance: {fileID: 0} + m_PrefabAsset: {fileID: 0} + m_GameObject: {fileID: 0} + m_Enabled: 1 + m_EditorHideFlags: 0 + m_Script: {fileID: 13312, guid: 0000000000000000e000000000000000, type: 0} + m_Name: Dungeon_13 + m_EditorClassIdentifier: + m_Sprite: {fileID: 489946030, guid: 0a806eb0d25e11c48b5dee57fb9339b5, type: 3} + m_Color: {r: 1, g: 1, b: 1, a: 1} + m_Transform: + e00: 1 + e01: 0 + e02: 0 + e03: 0 + e10: 0 + e11: 1 + e12: 0 + e13: 0 + e20: 0 + e21: 0 + e22: 1 + e23: 0 + e30: 0 + e31: 0 + e32: 0 + e33: 1 + m_InstancedGameObject: {fileID: 0} + m_Flags: 1 + m_ColliderType: 1 diff --git a/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_13.asset.meta b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_13.asset.meta new file mode 100644 index 0000000000000000000000000000000000000000..0c502ea0da74e6a0614ead34b77e3b7617a6c432 --- /dev/null +++ b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_13.asset.meta @@ -0,0 +1,8 @@ +fileFormatVersion: 2 +guid: c0a9a81d8f984974caea2de0685f4691 +NativeFormatImporter: + externalObjects: {} + mainObjectFileID: 11400000 + userData: + assetBundleName: + assetBundleVariant: diff --git a/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_14.asset b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_14.asset new file mode 100644 index 0000000000000000000000000000000000000000..bfaca90bfcf5f778c602d10d189fe6a6a69e45f1 --- /dev/null +++ b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_14.asset @@ -0,0 +1,36 @@ +%YAML 1.1 +%TAG !u! tag:unity3d.com,2011: +--- !u!114 &11400000 +MonoBehaviour: + m_ObjectHideFlags: 0 + m_CorrespondingSourceObject: {fileID: 0} + m_PrefabInstance: {fileID: 0} + m_PrefabAsset: {fileID: 0} + m_GameObject: {fileID: 0} + m_Enabled: 1 + m_EditorHideFlags: 0 + m_Script: {fileID: 13312, guid: 0000000000000000e000000000000000, type: 0} + m_Name: Dungeon_14 + m_EditorClassIdentifier: + m_Sprite: {fileID: -1758251442, guid: 0a806eb0d25e11c48b5dee57fb9339b5, type: 3} + m_Color: {r: 1, g: 1, b: 1, a: 1} + m_Transform: + e00: 1 + e01: 0 + e02: 0 + e03: 0 + e10: 0 + e11: 1 + e12: 0 + e13: 0 + e20: 0 + e21: 0 + e22: 1 + e23: 0 + e30: 0 + e31: 0 + e32: 0 + e33: 1 + m_InstancedGameObject: {fileID: 0} + m_Flags: 1 + m_ColliderType: 1 diff --git a/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_14.asset.meta b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_14.asset.meta new file mode 100644 index 0000000000000000000000000000000000000000..869b0fcfff2c0d13bcbbafacafb13bffeaed1428 --- /dev/null +++ b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_14.asset.meta @@ -0,0 +1,8 @@ +fileFormatVersion: 2 +guid: 604fb5a5936131f42b7ed995b90b27f5 +NativeFormatImporter: + externalObjects: {} + mainObjectFileID: 11400000 + userData: + assetBundleName: + assetBundleVariant: diff --git a/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_15.asset b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_15.asset new file mode 100644 index 0000000000000000000000000000000000000000..ea1beb13d537f18f394aa7ee000cf1eab5f330ba --- /dev/null +++ b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_15.asset @@ -0,0 +1,36 @@ +%YAML 1.1 +%TAG !u! tag:unity3d.com,2011: +--- !u!114 &11400000 +MonoBehaviour: + m_ObjectHideFlags: 0 + m_CorrespondingSourceObject: {fileID: 0} + m_PrefabInstance: {fileID: 0} + m_PrefabAsset: {fileID: 0} + m_GameObject: {fileID: 0} + m_Enabled: 1 + m_EditorHideFlags: 0 + m_Script: {fileID: 13312, guid: 0000000000000000e000000000000000, type: 0} + m_Name: Dungeon_15 + m_EditorClassIdentifier: + m_Sprite: {fileID: -432912164, guid: 0a806eb0d25e11c48b5dee57fb9339b5, type: 3} + m_Color: {r: 1, g: 1, b: 1, a: 1} + m_Transform: + e00: 1 + e01: 0 + e02: 0 + e03: 0 + e10: 0 + e11: 1 + e12: 0 + e13: 0 + e20: 0 + e21: 0 + e22: 1 + e23: 0 + e30: 0 + e31: 0 + e32: 0 + e33: 1 + m_InstancedGameObject: {fileID: 0} + m_Flags: 1 + m_ColliderType: 1 diff --git a/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_15.asset.meta b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_15.asset.meta new file mode 100644 index 0000000000000000000000000000000000000000..f434ff3282fe4c3e45249ad0f876098be64da204 --- /dev/null +++ b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_15.asset.meta @@ -0,0 +1,8 @@ +fileFormatVersion: 2 +guid: de3388181831cdc46a01a7486e223f94 +NativeFormatImporter: + externalObjects: {} + mainObjectFileID: 11400000 + userData: + assetBundleName: + assetBundleVariant: diff --git a/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_16.asset b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_16.asset new file mode 100644 index 0000000000000000000000000000000000000000..d8008a26cef987fbd66d6426fc87c5a607cc3201 --- /dev/null +++ b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_16.asset @@ -0,0 +1,36 @@ +%YAML 1.1 +%TAG !u! tag:unity3d.com,2011: +--- !u!114 &11400000 +MonoBehaviour: + m_ObjectHideFlags: 0 + m_CorrespondingSourceObject: {fileID: 0} + m_PrefabInstance: {fileID: 0} + m_PrefabAsset: {fileID: 0} + m_GameObject: {fileID: 0} + m_Enabled: 1 + m_EditorHideFlags: 0 + m_Script: {fileID: 13312, guid: 0000000000000000e000000000000000, type: 0} + m_Name: Dungeon_16 + m_EditorClassIdentifier: + m_Sprite: {fileID: 1580949469, guid: 0a806eb0d25e11c48b5dee57fb9339b5, type: 3} + m_Color: {r: 1, g: 1, b: 1, a: 1} + m_Transform: + e00: 1 + e01: 0 + e02: 0 + e03: 0 + e10: 0 + e11: 1 + e12: 0 + e13: 0 + e20: 0 + e21: 0 + e22: 1 + e23: 0 + e30: 0 + e31: 0 + e32: 0 + e33: 1 + m_InstancedGameObject: {fileID: 0} + m_Flags: 1 + m_ColliderType: 1 diff --git a/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_16.asset.meta b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_16.asset.meta new file mode 100644 index 0000000000000000000000000000000000000000..ffa8d75bf9bdc045cac28d6044b23456081061f9 --- /dev/null +++ b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_16.asset.meta @@ -0,0 +1,8 @@ +fileFormatVersion: 2 +guid: eb057963df5e999448defa44fca62171 +NativeFormatImporter: + externalObjects: {} + mainObjectFileID: 11400000 + userData: + assetBundleName: + assetBundleVariant: diff --git a/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_17.asset b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_17.asset new file mode 100644 index 0000000000000000000000000000000000000000..0bf0f9da0c0ed38c952cb48776219c3543c7caac --- /dev/null +++ b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_17.asset @@ -0,0 +1,36 @@ +%YAML 1.1 +%TAG !u! tag:unity3d.com,2011: +--- !u!114 &11400000 +MonoBehaviour: + m_ObjectHideFlags: 0 + m_CorrespondingSourceObject: {fileID: 0} + m_PrefabInstance: {fileID: 0} + m_PrefabAsset: {fileID: 0} + m_GameObject: {fileID: 0} + m_Enabled: 1 + m_EditorHideFlags: 0 + m_Script: {fileID: 13312, guid: 0000000000000000e000000000000000, type: 0} + m_Name: Dungeon_17 + m_EditorClassIdentifier: + m_Sprite: {fileID: 2016605797, guid: 0a806eb0d25e11c48b5dee57fb9339b5, type: 3} + m_Color: {r: 1, g: 1, b: 1, a: 1} + m_Transform: + e00: 1 + e01: 0 + e02: 0 + e03: 0 + e10: 0 + e11: 1 + e12: 0 + e13: 0 + e20: 0 + e21: 0 + e22: 1 + e23: 0 + e30: 0 + e31: 0 + e32: 0 + e33: 1 + m_InstancedGameObject: {fileID: 0} + m_Flags: 1 + m_ColliderType: 1 diff --git a/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_17.asset.meta b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_17.asset.meta new file mode 100644 index 0000000000000000000000000000000000000000..1b795367dfcb6119384423fa03d48cea37762fc2 --- /dev/null +++ b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_17.asset.meta @@ -0,0 +1,8 @@ +fileFormatVersion: 2 +guid: 08940e2753a7a074a9301f324a0422cc +NativeFormatImporter: + externalObjects: {} + mainObjectFileID: 11400000 + userData: + assetBundleName: + assetBundleVariant: diff --git a/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_18.asset b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_18.asset new file mode 100644 index 0000000000000000000000000000000000000000..b0966e776c30b42e8d95a5120bdc7c990a34e0e5 --- /dev/null +++ b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_18.asset @@ -0,0 +1,36 @@ +%YAML 1.1 +%TAG !u! tag:unity3d.com,2011: +--- !u!114 &11400000 +MonoBehaviour: + m_ObjectHideFlags: 0 + m_CorrespondingSourceObject: {fileID: 0} + m_PrefabInstance: {fileID: 0} + m_PrefabAsset: {fileID: 0} + m_GameObject: {fileID: 0} + m_Enabled: 1 + m_EditorHideFlags: 0 + m_Script: {fileID: 13312, guid: 0000000000000000e000000000000000, type: 0} + m_Name: Dungeon_18 + m_EditorClassIdentifier: + m_Sprite: {fileID: -1798427165, guid: 0a806eb0d25e11c48b5dee57fb9339b5, type: 3} + m_Color: {r: 1, g: 1, b: 1, a: 1} + m_Transform: + e00: 1 + e01: 0 + e02: 0 + e03: 0 + e10: 0 + e11: 1 + e12: 0 + e13: 0 + e20: 0 + e21: 0 + e22: 1 + e23: 0 + e30: 0 + e31: 0 + e32: 0 + e33: 1 + m_InstancedGameObject: {fileID: 0} + m_Flags: 1 + m_ColliderType: 1 diff --git a/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_18.asset.meta b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_18.asset.meta new file mode 100644 index 0000000000000000000000000000000000000000..cb8500d73a20050a04aa964f37d8cf5207e8bbab --- /dev/null +++ b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_18.asset.meta @@ -0,0 +1,8 @@ +fileFormatVersion: 2 +guid: 6c8ea1298beba484d81a637df26e49f2 +NativeFormatImporter: + externalObjects: {} + mainObjectFileID: 11400000 + userData: + assetBundleName: + assetBundleVariant: diff --git a/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_19.asset b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_19.asset new file mode 100644 index 0000000000000000000000000000000000000000..160be95ac4d3fd62e74a5242f003dead68175355 --- /dev/null +++ b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_19.asset @@ -0,0 +1,36 @@ +%YAML 1.1 +%TAG !u! tag:unity3d.com,2011: +--- !u!114 &11400000 +MonoBehaviour: + m_ObjectHideFlags: 0 + m_CorrespondingSourceObject: {fileID: 0} + m_PrefabInstance: {fileID: 0} + m_PrefabAsset: {fileID: 0} + m_GameObject: {fileID: 0} + m_Enabled: 1 + m_EditorHideFlags: 0 + m_Script: {fileID: 13312, guid: 0000000000000000e000000000000000, type: 0} + m_Name: Dungeon_19 + m_EditorClassIdentifier: + m_Sprite: {fileID: 1917838317, guid: 0a806eb0d25e11c48b5dee57fb9339b5, type: 3} + m_Color: {r: 1, g: 1, b: 1, a: 1} + m_Transform: + e00: 1 + e01: 0 + e02: 0 + e03: 0 + e10: 0 + e11: 1 + e12: 0 + e13: 0 + e20: 0 + e21: 0 + e22: 1 + e23: 0 + e30: 0 + e31: 0 + e32: 0 + e33: 1 + m_InstancedGameObject: {fileID: 0} + m_Flags: 1 + m_ColliderType: 1 diff --git a/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_19.asset.meta b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_19.asset.meta new file mode 100644 index 0000000000000000000000000000000000000000..75e20b7aa6f7095dc9e71b339e7eda21acadfc12 --- /dev/null +++ b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_19.asset.meta @@ -0,0 +1,8 @@ +fileFormatVersion: 2 +guid: f2976e05ee95b31469443f58b6e4e2b5 +NativeFormatImporter: + externalObjects: {} + mainObjectFileID: 11400000 + userData: + assetBundleName: + assetBundleVariant: diff --git a/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_2.asset b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_2.asset new file mode 100644 index 0000000000000000000000000000000000000000..ecbcdd3f3d0e70a9957d6794ac26c77678ef25d1 --- /dev/null +++ b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_2.asset @@ -0,0 +1,36 @@ +%YAML 1.1 +%TAG !u! tag:unity3d.com,2011: +--- !u!114 &11400000 +MonoBehaviour: + m_ObjectHideFlags: 0 + m_CorrespondingSourceObject: {fileID: 0} + m_PrefabInstance: {fileID: 0} + m_PrefabAsset: {fileID: 0} + m_GameObject: {fileID: 0} + m_Enabled: 1 + m_EditorHideFlags: 0 + m_Script: {fileID: 13312, guid: 0000000000000000e000000000000000, type: 0} + m_Name: Dungeon_2 + m_EditorClassIdentifier: + m_Sprite: {fileID: 164431376, guid: 0a806eb0d25e11c48b5dee57fb9339b5, type: 3} + m_Color: {r: 1, g: 1, b: 1, a: 1} + m_Transform: + e00: 1 + e01: 0 + e02: 0 + e03: 0 + e10: 0 + e11: 1 + e12: 0 + e13: 0 + e20: 0 + e21: 0 + e22: 1 + e23: 0 + e30: 0 + e31: 0 + e32: 0 + e33: 1 + m_InstancedGameObject: {fileID: 0} + m_Flags: 1 + m_ColliderType: 1 diff --git a/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_2.asset.meta b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_2.asset.meta new file mode 100644 index 0000000000000000000000000000000000000000..9abbad79ae6c38e61a32bc35aa5097044edd94e4 --- /dev/null +++ b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_2.asset.meta @@ -0,0 +1,8 @@ +fileFormatVersion: 2 +guid: abf6b1252b746f744a5040d07340a8f4 +NativeFormatImporter: + externalObjects: {} + mainObjectFileID: 11400000 + userData: + assetBundleName: + assetBundleVariant: diff --git a/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_20.asset b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_20.asset new file mode 100644 index 0000000000000000000000000000000000000000..7707dec8e791f66a11ef0acab280024d7fe9710c --- /dev/null +++ b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_20.asset @@ -0,0 +1,36 @@ +%YAML 1.1 +%TAG !u! tag:unity3d.com,2011: +--- !u!114 &11400000 +MonoBehaviour: + m_ObjectHideFlags: 0 + m_CorrespondingSourceObject: {fileID: 0} + m_PrefabInstance: {fileID: 0} + m_PrefabAsset: {fileID: 0} + m_GameObject: {fileID: 0} + m_Enabled: 1 + m_EditorHideFlags: 0 + m_Script: {fileID: 13312, guid: 0000000000000000e000000000000000, type: 0} + m_Name: Dungeon_20 + m_EditorClassIdentifier: + m_Sprite: {fileID: 1926522667, guid: 0a806eb0d25e11c48b5dee57fb9339b5, type: 3} + m_Color: {r: 1, g: 1, b: 1, a: 1} + m_Transform: + e00: 1 + e01: 0 + e02: 0 + e03: 0 + e10: 0 + e11: 1 + e12: 0 + e13: 0 + e20: 0 + e21: 0 + e22: 1 + e23: 0 + e30: 0 + e31: 0 + e32: 0 + e33: 1 + m_InstancedGameObject: {fileID: 0} + m_Flags: 1 + m_ColliderType: 1 diff --git a/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_20.asset.meta b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_20.asset.meta new file mode 100644 index 0000000000000000000000000000000000000000..139cd88604d65f2a8466c59c944d789c96d296c2 --- /dev/null +++ b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_20.asset.meta @@ -0,0 +1,8 @@ +fileFormatVersion: 2 +guid: 78df622e549350e419f33628d6de5bec +NativeFormatImporter: + externalObjects: {} + mainObjectFileID: 11400000 + userData: + assetBundleName: + assetBundleVariant: diff --git a/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_21.asset b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_21.asset new file mode 100644 index 0000000000000000000000000000000000000000..71e34b1799508fdca399a7498f912400e225c8f4 --- /dev/null +++ b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_21.asset @@ -0,0 +1,36 @@ +%YAML 1.1 +%TAG !u! tag:unity3d.com,2011: +--- !u!114 &11400000 +MonoBehaviour: + m_ObjectHideFlags: 0 + m_CorrespondingSourceObject: {fileID: 0} + m_PrefabInstance: {fileID: 0} + m_PrefabAsset: {fileID: 0} + m_GameObject: {fileID: 0} + m_Enabled: 1 + m_EditorHideFlags: 0 + m_Script: {fileID: 13312, guid: 0000000000000000e000000000000000, type: 0} + m_Name: Dungeon_21 + m_EditorClassIdentifier: + m_Sprite: {fileID: -672668870, guid: 0a806eb0d25e11c48b5dee57fb9339b5, type: 3} + m_Color: {r: 1, g: 1, b: 1, a: 1} + m_Transform: + e00: 1 + e01: 0 + e02: 0 + e03: 0 + e10: 0 + e11: 1 + e12: 0 + e13: 0 + e20: 0 + e21: 0 + e22: 1 + e23: 0 + e30: 0 + e31: 0 + e32: 0 + e33: 1 + m_InstancedGameObject: {fileID: 0} + m_Flags: 1 + m_ColliderType: 1 diff --git a/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_21.asset.meta b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_21.asset.meta new file mode 100644 index 0000000000000000000000000000000000000000..32a4c4eea0908aaccf9773acc9dee9ac3f1f7c67 --- /dev/null +++ b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_21.asset.meta @@ -0,0 +1,8 @@ +fileFormatVersion: 2 +guid: abce38af38bd21149b37233db41fc261 +NativeFormatImporter: + externalObjects: {} + mainObjectFileID: 11400000 + userData: + assetBundleName: + assetBundleVariant: diff --git a/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_22.asset b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_22.asset new file mode 100644 index 0000000000000000000000000000000000000000..888a11de1a58fc4cf5e1a4175ee0b6b2e14342fb --- /dev/null +++ b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_22.asset @@ -0,0 +1,36 @@ +%YAML 1.1 +%TAG !u! tag:unity3d.com,2011: +--- !u!114 &11400000 +MonoBehaviour: + m_ObjectHideFlags: 0 + m_CorrespondingSourceObject: {fileID: 0} + m_PrefabInstance: {fileID: 0} + m_PrefabAsset: {fileID: 0} + m_GameObject: {fileID: 0} + m_Enabled: 1 + m_EditorHideFlags: 0 + m_Script: {fileID: 13312, guid: 0000000000000000e000000000000000, type: 0} + m_Name: Dungeon_22 + m_EditorClassIdentifier: + m_Sprite: {fileID: -2113988543, guid: 0a806eb0d25e11c48b5dee57fb9339b5, type: 3} + m_Color: {r: 1, g: 1, b: 1, a: 1} + m_Transform: + e00: 1 + e01: 0 + e02: 0 + e03: 0 + e10: 0 + e11: 1 + e12: 0 + e13: 0 + e20: 0 + e21: 0 + e22: 1 + e23: 0 + e30: 0 + e31: 0 + e32: 0 + e33: 1 + m_InstancedGameObject: {fileID: 0} + m_Flags: 1 + m_ColliderType: 1 diff --git a/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_22.asset.meta b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_22.asset.meta new file mode 100644 index 0000000000000000000000000000000000000000..471ef5b6c2770dbd5bd37e64f4db2be45e87c089 --- /dev/null +++ b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_22.asset.meta @@ -0,0 +1,8 @@ +fileFormatVersion: 2 +guid: 399a27777263f1345bbdcd02ac25a63e +NativeFormatImporter: + externalObjects: {} + mainObjectFileID: 11400000 + userData: + assetBundleName: + assetBundleVariant: diff --git a/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_23.asset b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_23.asset new file mode 100644 index 0000000000000000000000000000000000000000..c9cf879a0b8ab5a3d4a629016adaf70cb8dfd431 --- /dev/null +++ b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_23.asset @@ -0,0 +1,36 @@ +%YAML 1.1 +%TAG !u! tag:unity3d.com,2011: +--- !u!114 &11400000 +MonoBehaviour: + m_ObjectHideFlags: 0 + m_CorrespondingSourceObject: {fileID: 0} + m_PrefabInstance: {fileID: 0} + m_PrefabAsset: {fileID: 0} + m_GameObject: {fileID: 0} + m_Enabled: 1 + m_EditorHideFlags: 0 + m_Script: {fileID: 13312, guid: 0000000000000000e000000000000000, type: 0} + m_Name: Dungeon_23 + m_EditorClassIdentifier: + m_Sprite: {fileID: 1655838605, guid: 0a806eb0d25e11c48b5dee57fb9339b5, type: 3} + m_Color: {r: 1, g: 1, b: 1, a: 1} + m_Transform: + e00: 1 + e01: 0 + e02: 0 + e03: 0 + e10: 0 + e11: 1 + e12: 0 + e13: 0 + e20: 0 + e21: 0 + e22: 1 + e23: 0 + e30: 0 + e31: 0 + e32: 0 + e33: 1 + m_InstancedGameObject: {fileID: 0} + m_Flags: 1 + m_ColliderType: 1 diff --git a/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_23.asset.meta b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_23.asset.meta new file mode 100644 index 0000000000000000000000000000000000000000..5c05a3dcc0d9cecc79f2799f070a9c1c3773d502 --- /dev/null +++ b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_23.asset.meta @@ -0,0 +1,8 @@ +fileFormatVersion: 2 +guid: 7acbe6c401ddccd448f62bb0b4a4d679 +NativeFormatImporter: + externalObjects: {} + mainObjectFileID: 11400000 + userData: + assetBundleName: + assetBundleVariant: diff --git a/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_24.asset b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_24.asset new file mode 100644 index 0000000000000000000000000000000000000000..fda41827330be5a548d486a7dadb7ff9dc413df2 --- /dev/null +++ b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_24.asset @@ -0,0 +1,36 @@ +%YAML 1.1 +%TAG !u! tag:unity3d.com,2011: +--- !u!114 &11400000 +MonoBehaviour: + m_ObjectHideFlags: 0 + m_CorrespondingSourceObject: {fileID: 0} + m_PrefabInstance: {fileID: 0} + m_PrefabAsset: {fileID: 0} + m_GameObject: {fileID: 0} + m_Enabled: 1 + m_EditorHideFlags: 0 + m_Script: {fileID: 13312, guid: 0000000000000000e000000000000000, type: 0} + m_Name: Dungeon_24 + m_EditorClassIdentifier: + m_Sprite: {fileID: 43680089, guid: 0a806eb0d25e11c48b5dee57fb9339b5, type: 3} + m_Color: {r: 1, g: 1, b: 1, a: 1} + m_Transform: + e00: 1 + e01: 0 + e02: 0 + e03: 0 + e10: 0 + e11: 1 + e12: 0 + e13: 0 + e20: 0 + e21: 0 + e22: 1 + e23: 0 + e30: 0 + e31: 0 + e32: 0 + e33: 1 + m_InstancedGameObject: {fileID: 0} + m_Flags: 1 + m_ColliderType: 1 diff --git a/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_24.asset.meta b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_24.asset.meta new file mode 100644 index 0000000000000000000000000000000000000000..67fb5e4c2506eac7d0021da116ab89717ecce3d0 --- /dev/null +++ b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_24.asset.meta @@ -0,0 +1,8 @@ +fileFormatVersion: 2 +guid: decde4ccadd99f044a6a9f09c51a9077 +NativeFormatImporter: + externalObjects: {} + mainObjectFileID: 11400000 + userData: + assetBundleName: + assetBundleVariant: diff --git a/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_25.asset b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_25.asset new file mode 100644 index 0000000000000000000000000000000000000000..80163929e843e6e42a72d88e43e461db3a7edc6e --- /dev/null +++ b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_25.asset @@ -0,0 +1,36 @@ +%YAML 1.1 +%TAG !u! tag:unity3d.com,2011: +--- !u!114 &11400000 +MonoBehaviour: + m_ObjectHideFlags: 0 + m_CorrespondingSourceObject: {fileID: 0} + m_PrefabInstance: {fileID: 0} + m_PrefabAsset: {fileID: 0} + m_GameObject: {fileID: 0} + m_Enabled: 1 + m_EditorHideFlags: 0 + m_Script: {fileID: 13312, guid: 0000000000000000e000000000000000, type: 0} + m_Name: Dungeon_25 + m_EditorClassIdentifier: + m_Sprite: {fileID: 1995419026, guid: 0a806eb0d25e11c48b5dee57fb9339b5, type: 3} + m_Color: {r: 1, g: 1, b: 1, a: 1} + m_Transform: + e00: 1 + e01: 0 + e02: 0 + e03: 0 + e10: 0 + e11: 1 + e12: 0 + e13: 0 + e20: 0 + e21: 0 + e22: 1 + e23: 0 + e30: 0 + e31: 0 + e32: 0 + e33: 1 + m_InstancedGameObject: {fileID: 0} + m_Flags: 1 + m_ColliderType: 1 diff --git a/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_25.asset.meta b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_25.asset.meta new file mode 100644 index 0000000000000000000000000000000000000000..30cb5476d60c32c1a951879810f7f938f7b921e7 --- /dev/null +++ b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_25.asset.meta @@ -0,0 +1,8 @@ +fileFormatVersion: 2 +guid: 8938aa05be2283741866f9cb212cbfb2 +NativeFormatImporter: + externalObjects: {} + mainObjectFileID: 11400000 + userData: + assetBundleName: + assetBundleVariant: diff --git a/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_26.asset b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_26.asset new file mode 100644 index 0000000000000000000000000000000000000000..cbe85769318fef238201293c51354aace741b128 --- /dev/null +++ b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_26.asset @@ -0,0 +1,36 @@ +%YAML 1.1 +%TAG !u! tag:unity3d.com,2011: +--- !u!114 &11400000 +MonoBehaviour: + m_ObjectHideFlags: 0 + m_CorrespondingSourceObject: {fileID: 0} + m_PrefabInstance: {fileID: 0} + m_PrefabAsset: {fileID: 0} + m_GameObject: {fileID: 0} + m_Enabled: 1 + m_EditorHideFlags: 0 + m_Script: {fileID: 13312, guid: 0000000000000000e000000000000000, type: 0} + m_Name: Dungeon_26 + m_EditorClassIdentifier: + m_Sprite: {fileID: 1277448032, guid: 0a806eb0d25e11c48b5dee57fb9339b5, type: 3} + m_Color: {r: 1, g: 1, b: 1, a: 1} + m_Transform: + e00: 1 + e01: 0 + e02: 0 + e03: 0 + e10: 0 + e11: 1 + e12: 0 + e13: 0 + e20: 0 + e21: 0 + e22: 1 + e23: 0 + e30: 0 + e31: 0 + e32: 0 + e33: 1 + m_InstancedGameObject: {fileID: 0} + m_Flags: 1 + m_ColliderType: 1 diff --git a/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_26.asset.meta b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_26.asset.meta new file mode 100644 index 0000000000000000000000000000000000000000..b4bc0760774d70b91ea37ceea6f7974e2ff4650b --- /dev/null +++ b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_26.asset.meta @@ -0,0 +1,8 @@ +fileFormatVersion: 2 +guid: 45520d2e837024f4583428bb378123a0 +NativeFormatImporter: + externalObjects: {} + mainObjectFileID: 11400000 + userData: + assetBundleName: + assetBundleVariant: diff --git a/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_27.asset b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_27.asset new file mode 100644 index 0000000000000000000000000000000000000000..a545668803df91459ac46f3989bd87b3c6d70fef --- /dev/null +++ b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_27.asset @@ -0,0 +1,36 @@ +%YAML 1.1 +%TAG !u! tag:unity3d.com,2011: +--- !u!114 &11400000 +MonoBehaviour: + m_ObjectHideFlags: 0 + m_CorrespondingSourceObject: {fileID: 0} + m_PrefabInstance: {fileID: 0} + m_PrefabAsset: {fileID: 0} + m_GameObject: {fileID: 0} + m_Enabled: 1 + m_EditorHideFlags: 0 + m_Script: {fileID: 13312, guid: 0000000000000000e000000000000000, type: 0} + m_Name: Dungeon_27 + m_EditorClassIdentifier: + m_Sprite: {fileID: -412475550, guid: 0a806eb0d25e11c48b5dee57fb9339b5, type: 3} + m_Color: {r: 1, g: 1, b: 1, a: 1} + m_Transform: + e00: 1 + e01: 0 + e02: 0 + e03: 0 + e10: 0 + e11: 1 + e12: 0 + e13: 0 + e20: 0 + e21: 0 + e22: 1 + e23: 0 + e30: 0 + e31: 0 + e32: 0 + e33: 1 + m_InstancedGameObject: {fileID: 0} + m_Flags: 1 + m_ColliderType: 1 diff --git a/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_27.asset.meta b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_27.asset.meta new file mode 100644 index 0000000000000000000000000000000000000000..0f8f8c0aac061c411d377ffbf89b74230ce16a0e --- /dev/null +++ b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_27.asset.meta @@ -0,0 +1,8 @@ +fileFormatVersion: 2 +guid: 8a1fcfba9dfd3e143ac55d8750b645fe +NativeFormatImporter: + externalObjects: {} + mainObjectFileID: 11400000 + userData: + assetBundleName: + assetBundleVariant: diff --git a/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_28.asset b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_28.asset new file mode 100644 index 0000000000000000000000000000000000000000..45d3fd45b63af112f560e441222f67601b5da572 --- /dev/null +++ b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_28.asset @@ -0,0 +1,36 @@ +%YAML 1.1 +%TAG !u! tag:unity3d.com,2011: +--- !u!114 &11400000 +MonoBehaviour: + m_ObjectHideFlags: 0 + m_CorrespondingSourceObject: {fileID: 0} + m_PrefabInstance: {fileID: 0} + m_PrefabAsset: {fileID: 0} + m_GameObject: {fileID: 0} + m_Enabled: 1 + m_EditorHideFlags: 0 + m_Script: {fileID: 13312, guid: 0000000000000000e000000000000000, type: 0} + m_Name: Dungeon_28 + m_EditorClassIdentifier: + m_Sprite: {fileID: 1065039898, guid: 0a806eb0d25e11c48b5dee57fb9339b5, type: 3} + m_Color: {r: 1, g: 1, b: 1, a: 1} + m_Transform: + e00: 1 + e01: 0 + e02: 0 + e03: 0 + e10: 0 + e11: 1 + e12: 0 + e13: 0 + e20: 0 + e21: 0 + e22: 1 + e23: 0 + e30: 0 + e31: 0 + e32: 0 + e33: 1 + m_InstancedGameObject: {fileID: 0} + m_Flags: 1 + m_ColliderType: 1 diff --git a/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_28.asset.meta b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_28.asset.meta new file mode 100644 index 0000000000000000000000000000000000000000..100611553494dbd89bc38763a0e8cab7e43a752c --- /dev/null +++ b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_28.asset.meta @@ -0,0 +1,8 @@ +fileFormatVersion: 2 +guid: 34005f47333a0b54dbb9fdfbadac413b +NativeFormatImporter: + externalObjects: {} + mainObjectFileID: 11400000 + userData: + assetBundleName: + assetBundleVariant: diff --git a/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_29.asset b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_29.asset new file mode 100644 index 0000000000000000000000000000000000000000..a67d7634962691bdabd71d1269603b46ef721b17 --- /dev/null +++ b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_29.asset @@ -0,0 +1,36 @@ +%YAML 1.1 +%TAG !u! tag:unity3d.com,2011: +--- !u!114 &11400000 +MonoBehaviour: + m_ObjectHideFlags: 0 + m_CorrespondingSourceObject: {fileID: 0} + m_PrefabInstance: {fileID: 0} + m_PrefabAsset: {fileID: 0} + m_GameObject: {fileID: 0} + m_Enabled: 1 + m_EditorHideFlags: 0 + m_Script: {fileID: 13312, guid: 0000000000000000e000000000000000, type: 0} + m_Name: Dungeon_29 + m_EditorClassIdentifier: + m_Sprite: {fileID: 1956140949, guid: 0a806eb0d25e11c48b5dee57fb9339b5, type: 3} + m_Color: {r: 1, g: 1, b: 1, a: 1} + m_Transform: + e00: 1 + e01: 0 + e02: 0 + e03: 0 + e10: 0 + e11: 1 + e12: 0 + e13: 0 + e20: 0 + e21: 0 + e22: 1 + e23: 0 + e30: 0 + e31: 0 + e32: 0 + e33: 1 + m_InstancedGameObject: {fileID: 0} + m_Flags: 1 + m_ColliderType: 1 diff --git a/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_29.asset.meta b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_29.asset.meta new file mode 100644 index 0000000000000000000000000000000000000000..c8d41a7ef8fcd801de63c28d0598a3d0e7239932 --- /dev/null +++ b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_29.asset.meta @@ -0,0 +1,8 @@ +fileFormatVersion: 2 +guid: 12de738e24082e946b800cc99f9c8299 +NativeFormatImporter: + externalObjects: {} + mainObjectFileID: 11400000 + userData: + assetBundleName: + assetBundleVariant: diff --git a/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_3.asset b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_3.asset new file mode 100644 index 0000000000000000000000000000000000000000..34e4eb8e4cf89b67487a55bb25efd299083bafea --- /dev/null +++ b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_3.asset @@ -0,0 +1,36 @@ +%YAML 1.1 +%TAG !u! tag:unity3d.com,2011: +--- !u!114 &11400000 +MonoBehaviour: + m_ObjectHideFlags: 0 + m_CorrespondingSourceObject: {fileID: 0} + m_PrefabInstance: {fileID: 0} + m_PrefabAsset: {fileID: 0} + m_GameObject: {fileID: 0} + m_Enabled: 1 + m_EditorHideFlags: 0 + m_Script: {fileID: 13312, guid: 0000000000000000e000000000000000, type: 0} + m_Name: Dungeon_3 + m_EditorClassIdentifier: + m_Sprite: {fileID: 326241045, guid: 0a806eb0d25e11c48b5dee57fb9339b5, type: 3} + m_Color: {r: 1, g: 1, b: 1, a: 1} + m_Transform: + e00: 1 + e01: 0 + e02: 0 + e03: 0 + e10: 0 + e11: 1 + e12: 0 + e13: 0 + e20: 0 + e21: 0 + e22: 1 + e23: 0 + e30: 0 + e31: 0 + e32: 0 + e33: 1 + m_InstancedGameObject: {fileID: 0} + m_Flags: 1 + m_ColliderType: 1 diff --git a/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_3.asset.meta b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_3.asset.meta new file mode 100644 index 0000000000000000000000000000000000000000..45944feabc468427636917728078e05b6355fb3f --- /dev/null +++ b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_3.asset.meta @@ -0,0 +1,8 @@ +fileFormatVersion: 2 +guid: 609866c82a109c746b2814487ebbef66 +NativeFormatImporter: + externalObjects: {} + mainObjectFileID: 11400000 + userData: + assetBundleName: + assetBundleVariant: diff --git a/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_30.asset b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_30.asset new file mode 100644 index 0000000000000000000000000000000000000000..3cd88a400bb468311508d8958d5c0f6d5e490d23 --- /dev/null +++ b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_30.asset @@ -0,0 +1,36 @@ +%YAML 1.1 +%TAG !u! tag:unity3d.com,2011: +--- !u!114 &11400000 +MonoBehaviour: + m_ObjectHideFlags: 0 + m_CorrespondingSourceObject: {fileID: 0} + m_PrefabInstance: {fileID: 0} + m_PrefabAsset: {fileID: 0} + m_GameObject: {fileID: 0} + m_Enabled: 1 + m_EditorHideFlags: 0 + m_Script: {fileID: 13312, guid: 0000000000000000e000000000000000, type: 0} + m_Name: Dungeon_30 + m_EditorClassIdentifier: + m_Sprite: {fileID: -164313747, guid: 0a806eb0d25e11c48b5dee57fb9339b5, type: 3} + m_Color: {r: 1, g: 1, b: 1, a: 1} + m_Transform: + e00: 1 + e01: 0 + e02: 0 + e03: 0 + e10: 0 + e11: 1 + e12: 0 + e13: 0 + e20: 0 + e21: 0 + e22: 1 + e23: 0 + e30: 0 + e31: 0 + e32: 0 + e33: 1 + m_InstancedGameObject: {fileID: 0} + m_Flags: 1 + m_ColliderType: 1 diff --git a/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_30.asset.meta b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_30.asset.meta new file mode 100644 index 0000000000000000000000000000000000000000..b7741a8a15341439b0f44de574b39d88c004639a --- /dev/null +++ b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_30.asset.meta @@ -0,0 +1,8 @@ +fileFormatVersion: 2 +guid: 184d2ff80e48f4b4d82d61458a6756c1 +NativeFormatImporter: + externalObjects: {} + mainObjectFileID: 11400000 + userData: + assetBundleName: + assetBundleVariant: diff --git a/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_31.asset b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_31.asset new file mode 100644 index 0000000000000000000000000000000000000000..458254ec7d35a68d82f4c580623c4aafaa3e3d3a --- /dev/null +++ b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_31.asset @@ -0,0 +1,36 @@ +%YAML 1.1 +%TAG !u! tag:unity3d.com,2011: +--- !u!114 &11400000 +MonoBehaviour: + m_ObjectHideFlags: 0 + m_CorrespondingSourceObject: {fileID: 0} + m_PrefabInstance: {fileID: 0} + m_PrefabAsset: {fileID: 0} + m_GameObject: {fileID: 0} + m_Enabled: 1 + m_EditorHideFlags: 0 + m_Script: {fileID: 13312, guid: 0000000000000000e000000000000000, type: 0} + m_Name: Dungeon_31 + m_EditorClassIdentifier: + m_Sprite: {fileID: 1376823085, guid: 0a806eb0d25e11c48b5dee57fb9339b5, type: 3} + m_Color: {r: 1, g: 1, b: 1, a: 1} + m_Transform: + e00: 1 + e01: 0 + e02: 0 + e03: 0 + e10: 0 + e11: 1 + e12: 0 + e13: 0 + e20: 0 + e21: 0 + e22: 1 + e23: 0 + e30: 0 + e31: 0 + e32: 0 + e33: 1 + m_InstancedGameObject: {fileID: 0} + m_Flags: 1 + m_ColliderType: 1 diff --git a/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_31.asset.meta b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_31.asset.meta new file mode 100644 index 0000000000000000000000000000000000000000..e5217cb17903d1aa56fe1cb18858b7b6b8ba7536 --- /dev/null +++ b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_31.asset.meta @@ -0,0 +1,8 @@ +fileFormatVersion: 2 +guid: f04a8e256fee52940a0cb96ea72163a0 +NativeFormatImporter: + externalObjects: {} + mainObjectFileID: 11400000 + userData: + assetBundleName: + assetBundleVariant: diff --git a/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_32.asset b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_32.asset new file mode 100644 index 0000000000000000000000000000000000000000..c2926ab0bf57ddf176309d2f51d8214eb7e79220 --- /dev/null +++ b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_32.asset @@ -0,0 +1,36 @@ +%YAML 1.1 +%TAG !u! tag:unity3d.com,2011: +--- !u!114 &11400000 +MonoBehaviour: + m_ObjectHideFlags: 0 + m_CorrespondingSourceObject: {fileID: 0} + m_PrefabInstance: {fileID: 0} + m_PrefabAsset: {fileID: 0} + m_GameObject: {fileID: 0} + m_Enabled: 1 + m_EditorHideFlags: 0 + m_Script: {fileID: 13312, guid: 0000000000000000e000000000000000, type: 0} + m_Name: Dungeon_32 + m_EditorClassIdentifier: + m_Sprite: {fileID: 2009541612, guid: 0a806eb0d25e11c48b5dee57fb9339b5, type: 3} + m_Color: {r: 1, g: 1, b: 1, a: 1} + m_Transform: + e00: 1 + e01: 0 + e02: 0 + e03: 0 + e10: 0 + e11: 1 + e12: 0 + e13: 0 + e20: 0 + e21: 0 + e22: 1 + e23: 0 + e30: 0 + e31: 0 + e32: 0 + e33: 1 + m_InstancedGameObject: {fileID: 0} + m_Flags: 1 + m_ColliderType: 1 diff --git a/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_32.asset.meta b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_32.asset.meta new file mode 100644 index 0000000000000000000000000000000000000000..acf2d17d1b68638966b5d2b6b477e264681fbcfb --- /dev/null +++ b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_32.asset.meta @@ -0,0 +1,8 @@ +fileFormatVersion: 2 +guid: 60bfa98e2ec021b4abd020ea77da127b +NativeFormatImporter: + externalObjects: {} + mainObjectFileID: 11400000 + userData: + assetBundleName: + assetBundleVariant: diff --git a/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_33.asset b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_33.asset new file mode 100644 index 0000000000000000000000000000000000000000..4f5e3c64662e0029ed84d67f7294acbbff7c8e2f --- /dev/null +++ b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_33.asset @@ -0,0 +1,36 @@ +%YAML 1.1 +%TAG !u! tag:unity3d.com,2011: +--- !u!114 &11400000 +MonoBehaviour: + m_ObjectHideFlags: 0 + m_CorrespondingSourceObject: {fileID: 0} + m_PrefabInstance: {fileID: 0} + m_PrefabAsset: {fileID: 0} + m_GameObject: {fileID: 0} + m_Enabled: 1 + m_EditorHideFlags: 0 + m_Script: {fileID: 13312, guid: 0000000000000000e000000000000000, type: 0} + m_Name: Dungeon_33 + m_EditorClassIdentifier: + m_Sprite: {fileID: -1028069899, guid: 0a806eb0d25e11c48b5dee57fb9339b5, type: 3} + m_Color: {r: 1, g: 1, b: 1, a: 1} + m_Transform: + e00: 1 + e01: 0 + e02: 0 + e03: 0 + e10: 0 + e11: 1 + e12: 0 + e13: 0 + e20: 0 + e21: 0 + e22: 1 + e23: 0 + e30: 0 + e31: 0 + e32: 0 + e33: 1 + m_InstancedGameObject: {fileID: 0} + m_Flags: 1 + m_ColliderType: 1 diff --git a/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_33.asset.meta b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_33.asset.meta new file mode 100644 index 0000000000000000000000000000000000000000..297a9c8d6e04189e78cb01eceb48994a3b457270 --- /dev/null +++ b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_33.asset.meta @@ -0,0 +1,8 @@ +fileFormatVersion: 2 +guid: e9b00c5e96e845a46abe228c5988ced7 +NativeFormatImporter: + externalObjects: {} + mainObjectFileID: 11400000 + userData: + assetBundleName: + assetBundleVariant: diff --git a/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_34.asset b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_34.asset new file mode 100644 index 0000000000000000000000000000000000000000..6fc378438b82234ac6f969cd8fe992774c8b0f5d --- /dev/null +++ b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_34.asset @@ -0,0 +1,36 @@ +%YAML 1.1 +%TAG !u! tag:unity3d.com,2011: +--- !u!114 &11400000 +MonoBehaviour: + m_ObjectHideFlags: 0 + m_CorrespondingSourceObject: {fileID: 0} + m_PrefabInstance: {fileID: 0} + m_PrefabAsset: {fileID: 0} + m_GameObject: {fileID: 0} + m_Enabled: 1 + m_EditorHideFlags: 0 + m_Script: {fileID: 13312, guid: 0000000000000000e000000000000000, type: 0} + m_Name: Dungeon_34 + m_EditorClassIdentifier: + m_Sprite: {fileID: 1099301450, guid: 0a806eb0d25e11c48b5dee57fb9339b5, type: 3} + m_Color: {r: 1, g: 1, b: 1, a: 1} + m_Transform: + e00: 1 + e01: 0 + e02: 0 + e03: 0 + e10: 0 + e11: 1 + e12: 0 + e13: 0 + e20: 0 + e21: 0 + e22: 1 + e23: 0 + e30: 0 + e31: 0 + e32: 0 + e33: 1 + m_InstancedGameObject: {fileID: 0} + m_Flags: 1 + m_ColliderType: 1 diff --git a/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_34.asset.meta b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_34.asset.meta new file mode 100644 index 0000000000000000000000000000000000000000..523ebc6a96b92d593a3aebfadd82bf4ae4db2bf3 --- /dev/null +++ b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_34.asset.meta @@ -0,0 +1,8 @@ +fileFormatVersion: 2 +guid: e5cdf30eaad5fce488688512e6e582ae +NativeFormatImporter: + externalObjects: {} + mainObjectFileID: 11400000 + userData: + assetBundleName: + assetBundleVariant: diff --git a/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_35.asset b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_35.asset new file mode 100644 index 0000000000000000000000000000000000000000..1d1d3c317f11a8c4a846e583305b7137730bffeb --- /dev/null +++ b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_35.asset @@ -0,0 +1,36 @@ +%YAML 1.1 +%TAG !u! tag:unity3d.com,2011: +--- !u!114 &11400000 +MonoBehaviour: + m_ObjectHideFlags: 0 + m_CorrespondingSourceObject: {fileID: 0} + m_PrefabInstance: {fileID: 0} + m_PrefabAsset: {fileID: 0} + m_GameObject: {fileID: 0} + m_Enabled: 1 + m_EditorHideFlags: 0 + m_Script: {fileID: 13312, guid: 0000000000000000e000000000000000, type: 0} + m_Name: Dungeon_35 + m_EditorClassIdentifier: + m_Sprite: {fileID: 81769002, guid: 0a806eb0d25e11c48b5dee57fb9339b5, type: 3} + m_Color: {r: 1, g: 1, b: 1, a: 1} + m_Transform: + e00: 1 + e01: 0 + e02: 0 + e03: 0 + e10: 0 + e11: 1 + e12: 0 + e13: 0 + e20: 0 + e21: 0 + e22: 1 + e23: 0 + e30: 0 + e31: 0 + e32: 0 + e33: 1 + m_InstancedGameObject: {fileID: 0} + m_Flags: 1 + m_ColliderType: 1 diff --git a/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_35.asset.meta b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_35.asset.meta new file mode 100644 index 0000000000000000000000000000000000000000..8995b3ff5e5fdf031e0177f3ad504164de061897 --- /dev/null +++ b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_35.asset.meta @@ -0,0 +1,8 @@ +fileFormatVersion: 2 +guid: 68f8bdbdf51aa454f9412df899ac312f +NativeFormatImporter: + externalObjects: {} + mainObjectFileID: 11400000 + userData: + assetBundleName: + assetBundleVariant: diff --git a/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_36.asset b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_36.asset new file mode 100644 index 0000000000000000000000000000000000000000..790dad1b6aa76a6e2c227342f24e91ef94969473 --- /dev/null +++ b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_36.asset @@ -0,0 +1,36 @@ +%YAML 1.1 +%TAG !u! tag:unity3d.com,2011: +--- !u!114 &11400000 +MonoBehaviour: + m_ObjectHideFlags: 0 + m_CorrespondingSourceObject: {fileID: 0} + m_PrefabInstance: {fileID: 0} + m_PrefabAsset: {fileID: 0} + m_GameObject: {fileID: 0} + m_Enabled: 1 + m_EditorHideFlags: 0 + m_Script: {fileID: 13312, guid: 0000000000000000e000000000000000, type: 0} + m_Name: Dungeon_36 + m_EditorClassIdentifier: + m_Sprite: {fileID: 1050266288, guid: 0a806eb0d25e11c48b5dee57fb9339b5, type: 3} + m_Color: {r: 1, g: 1, b: 1, a: 1} + m_Transform: + e00: 1 + e01: 0 + e02: 0 + e03: 0 + e10: 0 + e11: 1 + e12: 0 + e13: 0 + e20: 0 + e21: 0 + e22: 1 + e23: 0 + e30: 0 + e31: 0 + e32: 0 + e33: 1 + m_InstancedGameObject: {fileID: 0} + m_Flags: 1 + m_ColliderType: 1 diff --git a/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_36.asset.meta b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_36.asset.meta new file mode 100644 index 0000000000000000000000000000000000000000..ddd087917008551a081e3e1c050c1a5ffd02e665 --- /dev/null +++ b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_36.asset.meta @@ -0,0 +1,8 @@ +fileFormatVersion: 2 +guid: 471a1ffc9aeb1c04bb8bbe643f7ac9a1 +NativeFormatImporter: + externalObjects: {} + mainObjectFileID: 11400000 + userData: + assetBundleName: + assetBundleVariant: diff --git a/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_37.asset b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_37.asset new file mode 100644 index 0000000000000000000000000000000000000000..88111ea178daaef5d08512fdc3b626c3ef27db99 --- /dev/null +++ b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_37.asset @@ -0,0 +1,36 @@ +%YAML 1.1 +%TAG !u! tag:unity3d.com,2011: +--- !u!114 &11400000 +MonoBehaviour: + m_ObjectHideFlags: 0 + m_CorrespondingSourceObject: {fileID: 0} + m_PrefabInstance: {fileID: 0} + m_PrefabAsset: {fileID: 0} + m_GameObject: {fileID: 0} + m_Enabled: 1 + m_EditorHideFlags: 0 + m_Script: {fileID: 13312, guid: 0000000000000000e000000000000000, type: 0} + m_Name: Dungeon_37 + m_EditorClassIdentifier: + m_Sprite: {fileID: 1708775233, guid: 0a806eb0d25e11c48b5dee57fb9339b5, type: 3} + m_Color: {r: 1, g: 1, b: 1, a: 1} + m_Transform: + e00: 1 + e01: 0 + e02: 0 + e03: 0 + e10: 0 + e11: 1 + e12: 0 + e13: 0 + e20: 0 + e21: 0 + e22: 1 + e23: 0 + e30: 0 + e31: 0 + e32: 0 + e33: 1 + m_InstancedGameObject: {fileID: 0} + m_Flags: 1 + m_ColliderType: 1 diff --git a/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_37.asset.meta b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_37.asset.meta new file mode 100644 index 0000000000000000000000000000000000000000..a7d545f3787dc0b1a2e1cbbf1428cca918c84ab9 --- /dev/null +++ b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_37.asset.meta @@ -0,0 +1,8 @@ +fileFormatVersion: 2 +guid: bfe73897392c1ee49b9d6dee3d174820 +NativeFormatImporter: + externalObjects: {} + mainObjectFileID: 11400000 + userData: + assetBundleName: + assetBundleVariant: diff --git a/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_38.asset b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_38.asset new file mode 100644 index 0000000000000000000000000000000000000000..6e4cc5abc9d40e1767ffd6e7b9e3a18b9ba82fa0 --- /dev/null +++ b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_38.asset @@ -0,0 +1,36 @@ +%YAML 1.1 +%TAG !u! tag:unity3d.com,2011: +--- !u!114 &11400000 +MonoBehaviour: + m_ObjectHideFlags: 0 + m_CorrespondingSourceObject: {fileID: 0} + m_PrefabInstance: {fileID: 0} + m_PrefabAsset: {fileID: 0} + m_GameObject: {fileID: 0} + m_Enabled: 1 + m_EditorHideFlags: 0 + m_Script: {fileID: 13312, guid: 0000000000000000e000000000000000, type: 0} + m_Name: Dungeon_38 + m_EditorClassIdentifier: + m_Sprite: {fileID: 660103562, guid: 0a806eb0d25e11c48b5dee57fb9339b5, type: 3} + m_Color: {r: 1, g: 1, b: 1, a: 1} + m_Transform: + e00: 1 + e01: 0 + e02: 0 + e03: 0 + e10: 0 + e11: 1 + e12: 0 + e13: 0 + e20: 0 + e21: 0 + e22: 1 + e23: 0 + e30: 0 + e31: 0 + e32: 0 + e33: 1 + m_InstancedGameObject: {fileID: 0} + m_Flags: 1 + m_ColliderType: 1 diff --git a/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_38.asset.meta b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_38.asset.meta new file mode 100644 index 0000000000000000000000000000000000000000..d63a6668fd24d60e850b144120d22813b713054d --- /dev/null +++ b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_38.asset.meta @@ -0,0 +1,8 @@ +fileFormatVersion: 2 +guid: d0be3b3859731d54f808b245d6b107ab +NativeFormatImporter: + externalObjects: {} + mainObjectFileID: 11400000 + userData: + assetBundleName: + assetBundleVariant: diff --git a/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_39.asset b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_39.asset new file mode 100644 index 0000000000000000000000000000000000000000..616de4f8048b9121183895b1f2fe71a7e18fe324 --- /dev/null +++ b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_39.asset @@ -0,0 +1,36 @@ +%YAML 1.1 +%TAG !u! tag:unity3d.com,2011: +--- !u!114 &11400000 +MonoBehaviour: + m_ObjectHideFlags: 0 + m_CorrespondingSourceObject: {fileID: 0} + m_PrefabInstance: {fileID: 0} + m_PrefabAsset: {fileID: 0} + m_GameObject: {fileID: 0} + m_Enabled: 1 + m_EditorHideFlags: 0 + m_Script: {fileID: 13312, guid: 0000000000000000e000000000000000, type: 0} + m_Name: Dungeon_39 + m_EditorClassIdentifier: + m_Sprite: {fileID: 1397179706, guid: 0a806eb0d25e11c48b5dee57fb9339b5, type: 3} + m_Color: {r: 1, g: 1, b: 1, a: 1} + m_Transform: + e00: 1 + e01: 0 + e02: 0 + e03: 0 + e10: 0 + e11: 1 + e12: 0 + e13: 0 + e20: 0 + e21: 0 + e22: 1 + e23: 0 + e30: 0 + e31: 0 + e32: 0 + e33: 1 + m_InstancedGameObject: {fileID: 0} + m_Flags: 1 + m_ColliderType: 1 diff --git a/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_39.asset.meta b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_39.asset.meta new file mode 100644 index 0000000000000000000000000000000000000000..0090c3cbbb4f9dfb508441b30c484dfdb201c8ec --- /dev/null +++ b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_39.asset.meta @@ -0,0 +1,8 @@ +fileFormatVersion: 2 +guid: 0acf0b0434f3b5f4caef76921c697191 +NativeFormatImporter: + externalObjects: {} + mainObjectFileID: 11400000 + userData: + assetBundleName: + assetBundleVariant: diff --git a/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_4.asset b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_4.asset new file mode 100644 index 0000000000000000000000000000000000000000..ce6f473a7cf252cc9ef335d5c791b7e743eebe52 --- /dev/null +++ b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_4.asset @@ -0,0 +1,36 @@ +%YAML 1.1 +%TAG !u! tag:unity3d.com,2011: +--- !u!114 &11400000 +MonoBehaviour: + m_ObjectHideFlags: 0 + m_CorrespondingSourceObject: {fileID: 0} + m_PrefabInstance: {fileID: 0} + m_PrefabAsset: {fileID: 0} + m_GameObject: {fileID: 0} + m_Enabled: 1 + m_EditorHideFlags: 0 + m_Script: {fileID: 13312, guid: 0000000000000000e000000000000000, type: 0} + m_Name: Dungeon_4 + m_EditorClassIdentifier: + m_Sprite: {fileID: -345571141, guid: 0a806eb0d25e11c48b5dee57fb9339b5, type: 3} + m_Color: {r: 1, g: 1, b: 1, a: 1} + m_Transform: + e00: 1 + e01: 0 + e02: 0 + e03: 0 + e10: 0 + e11: 1 + e12: 0 + e13: 0 + e20: 0 + e21: 0 + e22: 1 + e23: 0 + e30: 0 + e31: 0 + e32: 0 + e33: 1 + m_InstancedGameObject: {fileID: 0} + m_Flags: 1 + m_ColliderType: 1 diff --git a/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_4.asset.meta b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_4.asset.meta new file mode 100644 index 0000000000000000000000000000000000000000..a488fb3521762100e47085a7007f43b14cedbd75 --- /dev/null +++ b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_4.asset.meta @@ -0,0 +1,8 @@ +fileFormatVersion: 2 +guid: 95fb445777b235b4fba02befc77382dd +NativeFormatImporter: + externalObjects: {} + mainObjectFileID: 11400000 + userData: + assetBundleName: + assetBundleVariant: diff --git a/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_40.asset b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_40.asset new file mode 100644 index 0000000000000000000000000000000000000000..0bdece2628c02bca36fc4b82dc9c1a700a165e18 --- /dev/null +++ b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_40.asset @@ -0,0 +1,36 @@ +%YAML 1.1 +%TAG !u! tag:unity3d.com,2011: +--- !u!114 &11400000 +MonoBehaviour: + m_ObjectHideFlags: 0 + m_CorrespondingSourceObject: {fileID: 0} + m_PrefabInstance: {fileID: 0} + m_PrefabAsset: {fileID: 0} + m_GameObject: {fileID: 0} + m_Enabled: 1 + m_EditorHideFlags: 0 + m_Script: {fileID: 13312, guid: 0000000000000000e000000000000000, type: 0} + m_Name: Dungeon_40 + m_EditorClassIdentifier: + m_Sprite: {fileID: 1158887391, guid: 0a806eb0d25e11c48b5dee57fb9339b5, type: 3} + m_Color: {r: 1, g: 1, b: 1, a: 1} + m_Transform: + e00: 1 + e01: 0 + e02: 0 + e03: 0 + e10: 0 + e11: 1 + e12: 0 + e13: 0 + e20: 0 + e21: 0 + e22: 1 + e23: 0 + e30: 0 + e31: 0 + e32: 0 + e33: 1 + m_InstancedGameObject: {fileID: 0} + m_Flags: 1 + m_ColliderType: 1 diff --git a/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_40.asset.meta b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_40.asset.meta new file mode 100644 index 0000000000000000000000000000000000000000..5a71002e6269b6644b1d32f171aad8ab24602ae0 --- /dev/null +++ b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_40.asset.meta @@ -0,0 +1,8 @@ +fileFormatVersion: 2 +guid: 590bc9cada8177b488e10eba7b11719e +NativeFormatImporter: + externalObjects: {} + mainObjectFileID: 11400000 + userData: + assetBundleName: + assetBundleVariant: diff --git a/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_41.asset b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_41.asset new file mode 100644 index 0000000000000000000000000000000000000000..6c2d2373fb8b191756458ba831a83ae59dfa6cd0 --- /dev/null +++ b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_41.asset @@ -0,0 +1,36 @@ +%YAML 1.1 +%TAG !u! tag:unity3d.com,2011: +--- !u!114 &11400000 +MonoBehaviour: + m_ObjectHideFlags: 0 + m_CorrespondingSourceObject: {fileID: 0} + m_PrefabInstance: {fileID: 0} + m_PrefabAsset: {fileID: 0} + m_GameObject: {fileID: 0} + m_Enabled: 1 + m_EditorHideFlags: 0 + m_Script: {fileID: 13312, guid: 0000000000000000e000000000000000, type: 0} + m_Name: Dungeon_41 + m_EditorClassIdentifier: + m_Sprite: {fileID: 615089749, guid: 0a806eb0d25e11c48b5dee57fb9339b5, type: 3} + m_Color: {r: 1, g: 1, b: 1, a: 1} + m_Transform: + e00: 1 + e01: 0 + e02: 0 + e03: 0 + e10: 0 + e11: 1 + e12: 0 + e13: 0 + e20: 0 + e21: 0 + e22: 1 + e23: 0 + e30: 0 + e31: 0 + e32: 0 + e33: 1 + m_InstancedGameObject: {fileID: 0} + m_Flags: 1 + m_ColliderType: 1 diff --git a/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_41.asset.meta b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_41.asset.meta new file mode 100644 index 0000000000000000000000000000000000000000..8d0956b1ec4890bb6123c2f1586d764e4b3fcfc7 --- /dev/null +++ b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_41.asset.meta @@ -0,0 +1,8 @@ +fileFormatVersion: 2 +guid: ffa3bf238b4515344b377b5da194f7c8 +NativeFormatImporter: + externalObjects: {} + mainObjectFileID: 11400000 + userData: + assetBundleName: + assetBundleVariant: diff --git a/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_42.asset b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_42.asset new file mode 100644 index 0000000000000000000000000000000000000000..3b10001b5bec351b2bf3054b21cec2e02f119d7b --- /dev/null +++ b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_42.asset @@ -0,0 +1,36 @@ +%YAML 1.1 +%TAG !u! tag:unity3d.com,2011: +--- !u!114 &11400000 +MonoBehaviour: + m_ObjectHideFlags: 0 + m_CorrespondingSourceObject: {fileID: 0} + m_PrefabInstance: {fileID: 0} + m_PrefabAsset: {fileID: 0} + m_GameObject: {fileID: 0} + m_Enabled: 1 + m_EditorHideFlags: 0 + m_Script: {fileID: 13312, guid: 0000000000000000e000000000000000, type: 0} + m_Name: Dungeon_42 + m_EditorClassIdentifier: + m_Sprite: {fileID: -777615196, guid: 0a806eb0d25e11c48b5dee57fb9339b5, type: 3} + m_Color: {r: 1, g: 1, b: 1, a: 1} + m_Transform: + e00: 1 + e01: 0 + e02: 0 + e03: 0 + e10: 0 + e11: 1 + e12: 0 + e13: 0 + e20: 0 + e21: 0 + e22: 1 + e23: 0 + e30: 0 + e31: 0 + e32: 0 + e33: 1 + m_InstancedGameObject: {fileID: 0} + m_Flags: 1 + m_ColliderType: 1 diff --git a/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_42.asset.meta b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_42.asset.meta new file mode 100644 index 0000000000000000000000000000000000000000..4b51659ed8b4338e06c13e43088f56980a9a507e --- /dev/null +++ b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_42.asset.meta @@ -0,0 +1,8 @@ +fileFormatVersion: 2 +guid: b7af5ab325f607041a625895c85c22fb +NativeFormatImporter: + externalObjects: {} + mainObjectFileID: 11400000 + userData: + assetBundleName: + assetBundleVariant: diff --git a/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_43.asset b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_43.asset new file mode 100644 index 0000000000000000000000000000000000000000..448ec681ba4e6ef893e94c69a9631f7e724ee590 --- /dev/null +++ b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_43.asset @@ -0,0 +1,36 @@ +%YAML 1.1 +%TAG !u! tag:unity3d.com,2011: +--- !u!114 &11400000 +MonoBehaviour: + m_ObjectHideFlags: 0 + m_CorrespondingSourceObject: {fileID: 0} + m_PrefabInstance: {fileID: 0} + m_PrefabAsset: {fileID: 0} + m_GameObject: {fileID: 0} + m_Enabled: 1 + m_EditorHideFlags: 0 + m_Script: {fileID: 13312, guid: 0000000000000000e000000000000000, type: 0} + m_Name: Dungeon_43 + m_EditorClassIdentifier: + m_Sprite: {fileID: 197855793, guid: 0a806eb0d25e11c48b5dee57fb9339b5, type: 3} + m_Color: {r: 1, g: 1, b: 1, a: 1} + m_Transform: + e00: 1 + e01: 0 + e02: 0 + e03: 0 + e10: 0 + e11: 1 + e12: 0 + e13: 0 + e20: 0 + e21: 0 + e22: 1 + e23: 0 + e30: 0 + e31: 0 + e32: 0 + e33: 1 + m_InstancedGameObject: {fileID: 0} + m_Flags: 1 + m_ColliderType: 1 diff --git a/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_43.asset.meta b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_43.asset.meta new file mode 100644 index 0000000000000000000000000000000000000000..0526b6ebefdf60e16b62b339c93fe2a580a865b9 --- /dev/null +++ b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_43.asset.meta @@ -0,0 +1,8 @@ +fileFormatVersion: 2 +guid: 710a5785df889584887ce56dc689b5b7 +NativeFormatImporter: + externalObjects: {} + mainObjectFileID: 11400000 + userData: + assetBundleName: + assetBundleVariant: diff --git a/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_44.asset b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_44.asset new file mode 100644 index 0000000000000000000000000000000000000000..ef100d644882d61b9df0ee00df3016fab0e9060b --- /dev/null +++ b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_44.asset @@ -0,0 +1,36 @@ +%YAML 1.1 +%TAG !u! tag:unity3d.com,2011: +--- !u!114 &11400000 +MonoBehaviour: + m_ObjectHideFlags: 0 + m_CorrespondingSourceObject: {fileID: 0} + m_PrefabInstance: {fileID: 0} + m_PrefabAsset: {fileID: 0} + m_GameObject: {fileID: 0} + m_Enabled: 1 + m_EditorHideFlags: 0 + m_Script: {fileID: 13312, guid: 0000000000000000e000000000000000, type: 0} + m_Name: Dungeon_44 + m_EditorClassIdentifier: + m_Sprite: {fileID: -1396940042, guid: 0a806eb0d25e11c48b5dee57fb9339b5, type: 3} + m_Color: {r: 1, g: 1, b: 1, a: 1} + m_Transform: + e00: 1 + e01: 0 + e02: 0 + e03: 0 + e10: 0 + e11: 1 + e12: 0 + e13: 0 + e20: 0 + e21: 0 + e22: 1 + e23: 0 + e30: 0 + e31: 0 + e32: 0 + e33: 1 + m_InstancedGameObject: {fileID: 0} + m_Flags: 1 + m_ColliderType: 1 diff --git a/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_44.asset.meta b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_44.asset.meta new file mode 100644 index 0000000000000000000000000000000000000000..86c96b205fd62ee9c467560357a94f7aa51e5968 --- /dev/null +++ b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_44.asset.meta @@ -0,0 +1,8 @@ +fileFormatVersion: 2 +guid: 75f9c18ba8ecb2d4b98ae64f7340474a +NativeFormatImporter: + externalObjects: {} + mainObjectFileID: 11400000 + userData: + assetBundleName: + assetBundleVariant: diff --git a/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_45.asset b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_45.asset new file mode 100644 index 0000000000000000000000000000000000000000..9546a0d122e5e503a513ea8e5aff8a092297014e --- /dev/null +++ b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_45.asset @@ -0,0 +1,36 @@ +%YAML 1.1 +%TAG !u! tag:unity3d.com,2011: +--- !u!114 &11400000 +MonoBehaviour: + m_ObjectHideFlags: 0 + m_CorrespondingSourceObject: {fileID: 0} + m_PrefabInstance: {fileID: 0} + m_PrefabAsset: {fileID: 0} + m_GameObject: {fileID: 0} + m_Enabled: 1 + m_EditorHideFlags: 0 + m_Script: {fileID: 13312, guid: 0000000000000000e000000000000000, type: 0} + m_Name: Dungeon_45 + m_EditorClassIdentifier: + m_Sprite: {fileID: 1802662682, guid: 0a806eb0d25e11c48b5dee57fb9339b5, type: 3} + m_Color: {r: 1, g: 1, b: 1, a: 1} + m_Transform: + e00: 1 + e01: 0 + e02: 0 + e03: 0 + e10: 0 + e11: 1 + e12: 0 + e13: 0 + e20: 0 + e21: 0 + e22: 1 + e23: 0 + e30: 0 + e31: 0 + e32: 0 + e33: 1 + m_InstancedGameObject: {fileID: 0} + m_Flags: 1 + m_ColliderType: 1 diff --git a/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_45.asset.meta b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_45.asset.meta new file mode 100644 index 0000000000000000000000000000000000000000..e89b0c84688f7fb7f503a84db50e84451e3dbb44 --- /dev/null +++ b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_45.asset.meta @@ -0,0 +1,8 @@ +fileFormatVersion: 2 +guid: 26e1dd6488abfb34584a76d2ee5021de +NativeFormatImporter: + externalObjects: {} + mainObjectFileID: 11400000 + userData: + assetBundleName: + assetBundleVariant: diff --git a/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_46.asset b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_46.asset new file mode 100644 index 0000000000000000000000000000000000000000..8229a80289636900522e116ed4cfca25c4f43501 --- /dev/null +++ b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_46.asset @@ -0,0 +1,36 @@ +%YAML 1.1 +%TAG !u! tag:unity3d.com,2011: +--- !u!114 &11400000 +MonoBehaviour: + m_ObjectHideFlags: 0 + m_CorrespondingSourceObject: {fileID: 0} + m_PrefabInstance: {fileID: 0} + m_PrefabAsset: {fileID: 0} + m_GameObject: {fileID: 0} + m_Enabled: 1 + m_EditorHideFlags: 0 + m_Script: {fileID: 13312, guid: 0000000000000000e000000000000000, type: 0} + m_Name: Dungeon_46 + m_EditorClassIdentifier: + m_Sprite: {fileID: -549131558, guid: 0a806eb0d25e11c48b5dee57fb9339b5, type: 3} + m_Color: {r: 1, g: 1, b: 1, a: 1} + m_Transform: + e00: 1 + e01: 0 + e02: 0 + e03: 0 + e10: 0 + e11: 1 + e12: 0 + e13: 0 + e20: 0 + e21: 0 + e22: 1 + e23: 0 + e30: 0 + e31: 0 + e32: 0 + e33: 1 + m_InstancedGameObject: {fileID: 0} + m_Flags: 1 + m_ColliderType: 1 diff --git a/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_46.asset.meta b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_46.asset.meta new file mode 100644 index 0000000000000000000000000000000000000000..4349a5e3d02dd0f3cd434259ec161270ca6bb676 --- /dev/null +++ b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_46.asset.meta @@ -0,0 +1,8 @@ +fileFormatVersion: 2 +guid: fc6124d66efc3524f8f35e4468c78100 +NativeFormatImporter: + externalObjects: {} + mainObjectFileID: 11400000 + userData: + assetBundleName: + assetBundleVariant: diff --git a/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_47.asset b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_47.asset new file mode 100644 index 0000000000000000000000000000000000000000..408d5df9e495120163f37c93c1ece41df29b1444 --- /dev/null +++ b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_47.asset @@ -0,0 +1,36 @@ +%YAML 1.1 +%TAG !u! tag:unity3d.com,2011: +--- !u!114 &11400000 +MonoBehaviour: + m_ObjectHideFlags: 0 + m_CorrespondingSourceObject: {fileID: 0} + m_PrefabInstance: {fileID: 0} + m_PrefabAsset: {fileID: 0} + m_GameObject: {fileID: 0} + m_Enabled: 1 + m_EditorHideFlags: 0 + m_Script: {fileID: 13312, guid: 0000000000000000e000000000000000, type: 0} + m_Name: Dungeon_47 + m_EditorClassIdentifier: + m_Sprite: {fileID: -1668792333, guid: 0a806eb0d25e11c48b5dee57fb9339b5, type: 3} + m_Color: {r: 1, g: 1, b: 1, a: 1} + m_Transform: + e00: 1 + e01: 0 + e02: 0 + e03: 0 + e10: 0 + e11: 1 + e12: 0 + e13: 0 + e20: 0 + e21: 0 + e22: 1 + e23: 0 + e30: 0 + e31: 0 + e32: 0 + e33: 1 + m_InstancedGameObject: {fileID: 0} + m_Flags: 1 + m_ColliderType: 1 diff --git a/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_47.asset.meta b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_47.asset.meta new file mode 100644 index 0000000000000000000000000000000000000000..5a3fdac3febfa97c66b3269dadeb73789426fed3 --- /dev/null +++ b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_47.asset.meta @@ -0,0 +1,8 @@ +fileFormatVersion: 2 +guid: 8b5f706aa5654f94c874ae8e383f3dfc +NativeFormatImporter: + externalObjects: {} + mainObjectFileID: 11400000 + userData: + assetBundleName: + assetBundleVariant: diff --git a/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_48.asset b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_48.asset new file mode 100644 index 0000000000000000000000000000000000000000..a9a29c8e5ca78272657bf475a15d26b541853aa3 --- /dev/null +++ b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_48.asset @@ -0,0 +1,36 @@ +%YAML 1.1 +%TAG !u! tag:unity3d.com,2011: +--- !u!114 &11400000 +MonoBehaviour: + m_ObjectHideFlags: 0 + m_CorrespondingSourceObject: {fileID: 0} + m_PrefabInstance: {fileID: 0} + m_PrefabAsset: {fileID: 0} + m_GameObject: {fileID: 0} + m_Enabled: 1 + m_EditorHideFlags: 0 + m_Script: {fileID: 13312, guid: 0000000000000000e000000000000000, type: 0} + m_Name: Dungeon_48 + m_EditorClassIdentifier: + m_Sprite: {fileID: -395624179, guid: 0a806eb0d25e11c48b5dee57fb9339b5, type: 3} + m_Color: {r: 1, g: 1, b: 1, a: 1} + m_Transform: + e00: 1 + e01: 0 + e02: 0 + e03: 0 + e10: 0 + e11: 1 + e12: 0 + e13: 0 + e20: 0 + e21: 0 + e22: 1 + e23: 0 + e30: 0 + e31: 0 + e32: 0 + e33: 1 + m_InstancedGameObject: {fileID: 0} + m_Flags: 1 + m_ColliderType: 1 diff --git a/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_48.asset.meta b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_48.asset.meta new file mode 100644 index 0000000000000000000000000000000000000000..b6dff954df8f4acdd42d30c360c3672f7920af9e --- /dev/null +++ b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_48.asset.meta @@ -0,0 +1,8 @@ +fileFormatVersion: 2 +guid: 1d382a2702adb7f4ebc3ca6a5110afb4 +NativeFormatImporter: + externalObjects: {} + mainObjectFileID: 11400000 + userData: + assetBundleName: + assetBundleVariant: diff --git a/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_49.asset b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_49.asset new file mode 100644 index 0000000000000000000000000000000000000000..e1e55a871349ddb74f994f805ffbd178ecc299af --- /dev/null +++ b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_49.asset @@ -0,0 +1,36 @@ +%YAML 1.1 +%TAG !u! tag:unity3d.com,2011: +--- !u!114 &11400000 +MonoBehaviour: + m_ObjectHideFlags: 0 + m_CorrespondingSourceObject: {fileID: 0} + m_PrefabInstance: {fileID: 0} + m_PrefabAsset: {fileID: 0} + m_GameObject: {fileID: 0} + m_Enabled: 1 + m_EditorHideFlags: 0 + m_Script: {fileID: 13312, guid: 0000000000000000e000000000000000, type: 0} + m_Name: Dungeon_49 + m_EditorClassIdentifier: + m_Sprite: {fileID: 453119210, guid: 0a806eb0d25e11c48b5dee57fb9339b5, type: 3} + m_Color: {r: 1, g: 1, b: 1, a: 1} + m_Transform: + e00: 1 + e01: 0 + e02: 0 + e03: 0 + e10: 0 + e11: 1 + e12: 0 + e13: 0 + e20: 0 + e21: 0 + e22: 1 + e23: 0 + e30: 0 + e31: 0 + e32: 0 + e33: 1 + m_InstancedGameObject: {fileID: 0} + m_Flags: 1 + m_ColliderType: 1 diff --git a/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_49.asset.meta b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_49.asset.meta new file mode 100644 index 0000000000000000000000000000000000000000..a44670870599a9ba7da9f0a8a65afc7ce43d7d27 --- /dev/null +++ b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_49.asset.meta @@ -0,0 +1,8 @@ +fileFormatVersion: 2 +guid: 482ddd0787e8e6845bf2715684b0eba3 +NativeFormatImporter: + externalObjects: {} + mainObjectFileID: 11400000 + userData: + assetBundleName: + assetBundleVariant: diff --git a/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_5.asset b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_5.asset new file mode 100644 index 0000000000000000000000000000000000000000..1a11db8d2f3562e04ef8551bc94f77b828b2ef66 --- /dev/null +++ b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_5.asset @@ -0,0 +1,36 @@ +%YAML 1.1 +%TAG !u! tag:unity3d.com,2011: +--- !u!114 &11400000 +MonoBehaviour: + m_ObjectHideFlags: 0 + m_CorrespondingSourceObject: {fileID: 0} + m_PrefabInstance: {fileID: 0} + m_PrefabAsset: {fileID: 0} + m_GameObject: {fileID: 0} + m_Enabled: 1 + m_EditorHideFlags: 0 + m_Script: {fileID: 13312, guid: 0000000000000000e000000000000000, type: 0} + m_Name: Dungeon_5 + m_EditorClassIdentifier: + m_Sprite: {fileID: 2094817791, guid: 0a806eb0d25e11c48b5dee57fb9339b5, type: 3} + m_Color: {r: 1, g: 1, b: 1, a: 1} + m_Transform: + e00: 1 + e01: 0 + e02: 0 + e03: 0 + e10: 0 + e11: 1 + e12: 0 + e13: 0 + e20: 0 + e21: 0 + e22: 1 + e23: 0 + e30: 0 + e31: 0 + e32: 0 + e33: 1 + m_InstancedGameObject: {fileID: 0} + m_Flags: 1 + m_ColliderType: 1 diff --git a/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_5.asset.meta b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_5.asset.meta new file mode 100644 index 0000000000000000000000000000000000000000..1821786bb03721a204b08b5b163bae2077b6b6c6 --- /dev/null +++ b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_5.asset.meta @@ -0,0 +1,8 @@ +fileFormatVersion: 2 +guid: 15279f4515799164b81e6d2645edb2b8 +NativeFormatImporter: + externalObjects: {} + mainObjectFileID: 11400000 + userData: + assetBundleName: + assetBundleVariant: diff --git a/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_50.asset b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_50.asset new file mode 100644 index 0000000000000000000000000000000000000000..f20d0901836332b5caba830fe6ffee701a6351ee --- /dev/null +++ b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_50.asset @@ -0,0 +1,36 @@ +%YAML 1.1 +%TAG !u! tag:unity3d.com,2011: +--- !u!114 &11400000 +MonoBehaviour: + m_ObjectHideFlags: 0 + m_CorrespondingSourceObject: {fileID: 0} + m_PrefabInstance: {fileID: 0} + m_PrefabAsset: {fileID: 0} + m_GameObject: {fileID: 0} + m_Enabled: 1 + m_EditorHideFlags: 0 + m_Script: {fileID: 13312, guid: 0000000000000000e000000000000000, type: 0} + m_Name: Dungeon_50 + m_EditorClassIdentifier: + m_Sprite: {fileID: -1669469920, guid: 0a806eb0d25e11c48b5dee57fb9339b5, type: 3} + m_Color: {r: 1, g: 1, b: 1, a: 1} + m_Transform: + e00: 1 + e01: 0 + e02: 0 + e03: 0 + e10: 0 + e11: 1 + e12: 0 + e13: 0 + e20: 0 + e21: 0 + e22: 1 + e23: 0 + e30: 0 + e31: 0 + e32: 0 + e33: 1 + m_InstancedGameObject: {fileID: 0} + m_Flags: 1 + m_ColliderType: 1 diff --git a/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_50.asset.meta b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_50.asset.meta new file mode 100644 index 0000000000000000000000000000000000000000..521b62f8c232e73c2102eb01642a6f03401540eb --- /dev/null +++ b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_50.asset.meta @@ -0,0 +1,8 @@ +fileFormatVersion: 2 +guid: 256c43bc5f4ab714eab7a6f360254d43 +NativeFormatImporter: + externalObjects: {} + mainObjectFileID: 11400000 + userData: + assetBundleName: + assetBundleVariant: diff --git a/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_51.asset b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_51.asset new file mode 100644 index 0000000000000000000000000000000000000000..6487a22a6ceb3c9f5afb8c429699d02f70f1a402 --- /dev/null +++ b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_51.asset @@ -0,0 +1,36 @@ +%YAML 1.1 +%TAG !u! tag:unity3d.com,2011: +--- !u!114 &11400000 +MonoBehaviour: + m_ObjectHideFlags: 0 + m_CorrespondingSourceObject: {fileID: 0} + m_PrefabInstance: {fileID: 0} + m_PrefabAsset: {fileID: 0} + m_GameObject: {fileID: 0} + m_Enabled: 1 + m_EditorHideFlags: 0 + m_Script: {fileID: 13312, guid: 0000000000000000e000000000000000, type: 0} + m_Name: Dungeon_51 + m_EditorClassIdentifier: + m_Sprite: {fileID: 79250496, guid: 0a806eb0d25e11c48b5dee57fb9339b5, type: 3} + m_Color: {r: 1, g: 1, b: 1, a: 1} + m_Transform: + e00: 1 + e01: 0 + e02: 0 + e03: 0 + e10: 0 + e11: 1 + e12: 0 + e13: 0 + e20: 0 + e21: 0 + e22: 1 + e23: 0 + e30: 0 + e31: 0 + e32: 0 + e33: 1 + m_InstancedGameObject: {fileID: 0} + m_Flags: 1 + m_ColliderType: 1 diff --git a/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_51.asset.meta b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_51.asset.meta new file mode 100644 index 0000000000000000000000000000000000000000..76b2ab36fef4757579b74cc8df255b14ee8f2ee5 --- /dev/null +++ b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_51.asset.meta @@ -0,0 +1,8 @@ +fileFormatVersion: 2 +guid: 92968a7b2c7327e4682d7d69d2082e75 +NativeFormatImporter: + externalObjects: {} + mainObjectFileID: 11400000 + userData: + assetBundleName: + assetBundleVariant: diff --git a/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_52.asset b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_52.asset new file mode 100644 index 0000000000000000000000000000000000000000..66f14d6a8176c14f6110d9d53efe90922fb3438a --- /dev/null +++ b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_52.asset @@ -0,0 +1,36 @@ +%YAML 1.1 +%TAG !u! tag:unity3d.com,2011: +--- !u!114 &11400000 +MonoBehaviour: + m_ObjectHideFlags: 0 + m_CorrespondingSourceObject: {fileID: 0} + m_PrefabInstance: {fileID: 0} + m_PrefabAsset: {fileID: 0} + m_GameObject: {fileID: 0} + m_Enabled: 1 + m_EditorHideFlags: 0 + m_Script: {fileID: 13312, guid: 0000000000000000e000000000000000, type: 0} + m_Name: Dungeon_52 + m_EditorClassIdentifier: + m_Sprite: {fileID: 2075798291, guid: 0a806eb0d25e11c48b5dee57fb9339b5, type: 3} + m_Color: {r: 1, g: 1, b: 1, a: 1} + m_Transform: + e00: 1 + e01: 0 + e02: 0 + e03: 0 + e10: 0 + e11: 1 + e12: 0 + e13: 0 + e20: 0 + e21: 0 + e22: 1 + e23: 0 + e30: 0 + e31: 0 + e32: 0 + e33: 1 + m_InstancedGameObject: {fileID: 0} + m_Flags: 1 + m_ColliderType: 1 diff --git a/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_52.asset.meta b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_52.asset.meta new file mode 100644 index 0000000000000000000000000000000000000000..d475d297b3a572dcf5a5026042184089e1f2479f --- /dev/null +++ b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_52.asset.meta @@ -0,0 +1,8 @@ +fileFormatVersion: 2 +guid: 5cfa31067f8707e42874ef4f4ba4de79 +NativeFormatImporter: + externalObjects: {} + mainObjectFileID: 11400000 + userData: + assetBundleName: + assetBundleVariant: diff --git a/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_53.asset b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_53.asset new file mode 100644 index 0000000000000000000000000000000000000000..7ee09dbb37fb72b831aa114fb4b416633764744c --- /dev/null +++ b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_53.asset @@ -0,0 +1,36 @@ +%YAML 1.1 +%TAG !u! tag:unity3d.com,2011: +--- !u!114 &11400000 +MonoBehaviour: + m_ObjectHideFlags: 0 + m_CorrespondingSourceObject: {fileID: 0} + m_PrefabInstance: {fileID: 0} + m_PrefabAsset: {fileID: 0} + m_GameObject: {fileID: 0} + m_Enabled: 1 + m_EditorHideFlags: 0 + m_Script: {fileID: 13312, guid: 0000000000000000e000000000000000, type: 0} + m_Name: Dungeon_53 + m_EditorClassIdentifier: + m_Sprite: {fileID: -282376894, guid: 0a806eb0d25e11c48b5dee57fb9339b5, type: 3} + m_Color: {r: 1, g: 1, b: 1, a: 1} + m_Transform: + e00: 1 + e01: 0 + e02: 0 + e03: 0 + e10: 0 + e11: 1 + e12: 0 + e13: 0 + e20: 0 + e21: 0 + e22: 1 + e23: 0 + e30: 0 + e31: 0 + e32: 0 + e33: 1 + m_InstancedGameObject: {fileID: 0} + m_Flags: 1 + m_ColliderType: 1 diff --git a/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_53.asset.meta b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_53.asset.meta new file mode 100644 index 0000000000000000000000000000000000000000..c2dc95d830af3711ccb4133649d41da80c8e2564 --- /dev/null +++ b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_53.asset.meta @@ -0,0 +1,8 @@ +fileFormatVersion: 2 +guid: 19db1f411a19ff44ab9a0b06e1811747 +NativeFormatImporter: + externalObjects: {} + mainObjectFileID: 11400000 + userData: + assetBundleName: + assetBundleVariant: diff --git a/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_54.asset b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_54.asset new file mode 100644 index 0000000000000000000000000000000000000000..a7b9df1136101025ea7bba1e093c079b206a3b7b --- /dev/null +++ b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_54.asset @@ -0,0 +1,36 @@ +%YAML 1.1 +%TAG !u! tag:unity3d.com,2011: +--- !u!114 &11400000 +MonoBehaviour: + m_ObjectHideFlags: 0 + m_CorrespondingSourceObject: {fileID: 0} + m_PrefabInstance: {fileID: 0} + m_PrefabAsset: {fileID: 0} + m_GameObject: {fileID: 0} + m_Enabled: 1 + m_EditorHideFlags: 0 + m_Script: {fileID: 13312, guid: 0000000000000000e000000000000000, type: 0} + m_Name: Dungeon_54 + m_EditorClassIdentifier: + m_Sprite: {fileID: 1862703900, guid: 0a806eb0d25e11c48b5dee57fb9339b5, type: 3} + m_Color: {r: 1, g: 1, b: 1, a: 1} + m_Transform: + e00: 1 + e01: 0 + e02: 0 + e03: 0 + e10: 0 + e11: 1 + e12: 0 + e13: 0 + e20: 0 + e21: 0 + e22: 1 + e23: 0 + e30: 0 + e31: 0 + e32: 0 + e33: 1 + m_InstancedGameObject: {fileID: 0} + m_Flags: 1 + m_ColliderType: 1 diff --git a/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_54.asset.meta b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_54.asset.meta new file mode 100644 index 0000000000000000000000000000000000000000..b332b27992cab2d20c2ba9f024baa5bfb0f4bb69 --- /dev/null +++ b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_54.asset.meta @@ -0,0 +1,8 @@ +fileFormatVersion: 2 +guid: 1204e5a6fef20cb4bbc137b1b66869ab +NativeFormatImporter: + externalObjects: {} + mainObjectFileID: 11400000 + userData: + assetBundleName: + assetBundleVariant: diff --git a/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_55.asset b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_55.asset new file mode 100644 index 0000000000000000000000000000000000000000..9a8f37adbc8c0c95123a91e5e254d2d943e6b096 --- /dev/null +++ b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_55.asset @@ -0,0 +1,36 @@ +%YAML 1.1 +%TAG !u! tag:unity3d.com,2011: +--- !u!114 &11400000 +MonoBehaviour: + m_ObjectHideFlags: 0 + m_CorrespondingSourceObject: {fileID: 0} + m_PrefabInstance: {fileID: 0} + m_PrefabAsset: {fileID: 0} + m_GameObject: {fileID: 0} + m_Enabled: 1 + m_EditorHideFlags: 0 + m_Script: {fileID: 13312, guid: 0000000000000000e000000000000000, type: 0} + m_Name: Dungeon_55 + m_EditorClassIdentifier: + m_Sprite: {fileID: 536411375, guid: 0a806eb0d25e11c48b5dee57fb9339b5, type: 3} + m_Color: {r: 1, g: 1, b: 1, a: 1} + m_Transform: + e00: 1 + e01: 0 + e02: 0 + e03: 0 + e10: 0 + e11: 1 + e12: 0 + e13: 0 + e20: 0 + e21: 0 + e22: 1 + e23: 0 + e30: 0 + e31: 0 + e32: 0 + e33: 1 + m_InstancedGameObject: {fileID: 0} + m_Flags: 1 + m_ColliderType: 1 diff --git a/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_55.asset.meta b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_55.asset.meta new file mode 100644 index 0000000000000000000000000000000000000000..e2855c414a2f59d37aad55a786199b7b834b371d --- /dev/null +++ b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_55.asset.meta @@ -0,0 +1,8 @@ +fileFormatVersion: 2 +guid: 5a946ab25fbde3e4c840ad3f178f6137 +NativeFormatImporter: + externalObjects: {} + mainObjectFileID: 11400000 + userData: + assetBundleName: + assetBundleVariant: diff --git a/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_56.asset b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_56.asset new file mode 100644 index 0000000000000000000000000000000000000000..64a8f69429ac238978b6a2117965ef8cbc68f3f8 --- /dev/null +++ b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_56.asset @@ -0,0 +1,36 @@ +%YAML 1.1 +%TAG !u! tag:unity3d.com,2011: +--- !u!114 &11400000 +MonoBehaviour: + m_ObjectHideFlags: 0 + m_CorrespondingSourceObject: {fileID: 0} + m_PrefabInstance: {fileID: 0} + m_PrefabAsset: {fileID: 0} + m_GameObject: {fileID: 0} + m_Enabled: 1 + m_EditorHideFlags: 0 + m_Script: {fileID: 13312, guid: 0000000000000000e000000000000000, type: 0} + m_Name: Dungeon_56 + m_EditorClassIdentifier: + m_Sprite: {fileID: 796962211, guid: 0a806eb0d25e11c48b5dee57fb9339b5, type: 3} + m_Color: {r: 1, g: 1, b: 1, a: 1} + m_Transform: + e00: 1 + e01: 0 + e02: 0 + e03: 0 + e10: 0 + e11: 1 + e12: 0 + e13: 0 + e20: 0 + e21: 0 + e22: 1 + e23: 0 + e30: 0 + e31: 0 + e32: 0 + e33: 1 + m_InstancedGameObject: {fileID: 0} + m_Flags: 1 + m_ColliderType: 1 diff --git a/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_56.asset.meta b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_56.asset.meta new file mode 100644 index 0000000000000000000000000000000000000000..de5608e4c327dc42cc84fdacde96501864748643 --- /dev/null +++ b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_56.asset.meta @@ -0,0 +1,8 @@ +fileFormatVersion: 2 +guid: ba2773a24a8457f44b4706314bd25a73 +NativeFormatImporter: + externalObjects: {} + mainObjectFileID: 11400000 + userData: + assetBundleName: + assetBundleVariant: diff --git a/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_57.asset b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_57.asset new file mode 100644 index 0000000000000000000000000000000000000000..a8afd66f33a6564672a9418ac1b7c57556c1cfa6 --- /dev/null +++ b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_57.asset @@ -0,0 +1,36 @@ +%YAML 1.1 +%TAG !u! tag:unity3d.com,2011: +--- !u!114 &11400000 +MonoBehaviour: + m_ObjectHideFlags: 0 + m_CorrespondingSourceObject: {fileID: 0} + m_PrefabInstance: {fileID: 0} + m_PrefabAsset: {fileID: 0} + m_GameObject: {fileID: 0} + m_Enabled: 1 + m_EditorHideFlags: 0 + m_Script: {fileID: 13312, guid: 0000000000000000e000000000000000, type: 0} + m_Name: Dungeon_57 + m_EditorClassIdentifier: + m_Sprite: {fileID: 1661568666, guid: 0a806eb0d25e11c48b5dee57fb9339b5, type: 3} + m_Color: {r: 1, g: 1, b: 1, a: 1} + m_Transform: + e00: 1 + e01: 0 + e02: 0 + e03: 0 + e10: 0 + e11: 1 + e12: 0 + e13: 0 + e20: 0 + e21: 0 + e22: 1 + e23: 0 + e30: 0 + e31: 0 + e32: 0 + e33: 1 + m_InstancedGameObject: {fileID: 0} + m_Flags: 1 + m_ColliderType: 1 diff --git a/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_57.asset.meta b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_57.asset.meta new file mode 100644 index 0000000000000000000000000000000000000000..3ea4b4cc5e19c7036a8c21c100beeaf56a9c1c16 --- /dev/null +++ b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_57.asset.meta @@ -0,0 +1,8 @@ +fileFormatVersion: 2 +guid: 048dff00fd5a17946a7ba7b5541a2eb6 +NativeFormatImporter: + externalObjects: {} + mainObjectFileID: 11400000 + userData: + assetBundleName: + assetBundleVariant: diff --git a/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_58.asset b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_58.asset new file mode 100644 index 0000000000000000000000000000000000000000..bc5765a6db17b75f2b162aa79039026d8740fb72 --- /dev/null +++ b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_58.asset @@ -0,0 +1,36 @@ +%YAML 1.1 +%TAG !u! tag:unity3d.com,2011: +--- !u!114 &11400000 +MonoBehaviour: + m_ObjectHideFlags: 0 + m_CorrespondingSourceObject: {fileID: 0} + m_PrefabInstance: {fileID: 0} + m_PrefabAsset: {fileID: 0} + m_GameObject: {fileID: 0} + m_Enabled: 1 + m_EditorHideFlags: 0 + m_Script: {fileID: 13312, guid: 0000000000000000e000000000000000, type: 0} + m_Name: Dungeon_58 + m_EditorClassIdentifier: + m_Sprite: {fileID: -2046453881, guid: 0a806eb0d25e11c48b5dee57fb9339b5, type: 3} + m_Color: {r: 1, g: 1, b: 1, a: 1} + m_Transform: + e00: 1 + e01: 0 + e02: 0 + e03: 0 + e10: 0 + e11: 1 + e12: 0 + e13: 0 + e20: 0 + e21: 0 + e22: 1 + e23: 0 + e30: 0 + e31: 0 + e32: 0 + e33: 1 + m_InstancedGameObject: {fileID: 0} + m_Flags: 1 + m_ColliderType: 1 diff --git a/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_58.asset.meta b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_58.asset.meta new file mode 100644 index 0000000000000000000000000000000000000000..fccffb75b4fb85c9154e3ffc300ed13330a02341 --- /dev/null +++ b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_58.asset.meta @@ -0,0 +1,8 @@ +fileFormatVersion: 2 +guid: 3093f1e128519824e844277fb6d3402a +NativeFormatImporter: + externalObjects: {} + mainObjectFileID: 11400000 + userData: + assetBundleName: + assetBundleVariant: diff --git a/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_59.asset b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_59.asset new file mode 100644 index 0000000000000000000000000000000000000000..f99ebead81f1a2034f6f6c0e00972d069b5a2cdd --- /dev/null +++ b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_59.asset @@ -0,0 +1,36 @@ +%YAML 1.1 +%TAG !u! tag:unity3d.com,2011: +--- !u!114 &11400000 +MonoBehaviour: + m_ObjectHideFlags: 0 + m_CorrespondingSourceObject: {fileID: 0} + m_PrefabInstance: {fileID: 0} + m_PrefabAsset: {fileID: 0} + m_GameObject: {fileID: 0} + m_Enabled: 1 + m_EditorHideFlags: 0 + m_Script: {fileID: 13312, guid: 0000000000000000e000000000000000, type: 0} + m_Name: Dungeon_59 + m_EditorClassIdentifier: + m_Sprite: {fileID: 2038936553, guid: 0a806eb0d25e11c48b5dee57fb9339b5, type: 3} + m_Color: {r: 1, g: 1, b: 1, a: 1} + m_Transform: + e00: 1 + e01: 0 + e02: 0 + e03: 0 + e10: 0 + e11: 1 + e12: 0 + e13: 0 + e20: 0 + e21: 0 + e22: 1 + e23: 0 + e30: 0 + e31: 0 + e32: 0 + e33: 1 + m_InstancedGameObject: {fileID: 0} + m_Flags: 1 + m_ColliderType: 1 diff --git a/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_59.asset.meta b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_59.asset.meta new file mode 100644 index 0000000000000000000000000000000000000000..f0e81752cde9e9e51ccb7f0c7b1e9c0edf3787b6 --- /dev/null +++ b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_59.asset.meta @@ -0,0 +1,8 @@ +fileFormatVersion: 2 +guid: 5387ff301b194ea46a0138787c1906d2 +NativeFormatImporter: + externalObjects: {} + mainObjectFileID: 11400000 + userData: + assetBundleName: + assetBundleVariant: diff --git a/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_6.asset b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_6.asset new file mode 100644 index 0000000000000000000000000000000000000000..f664f65b2300560a4e88beb0f717b06f2a6442a7 --- /dev/null +++ b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_6.asset @@ -0,0 +1,36 @@ +%YAML 1.1 +%TAG !u! tag:unity3d.com,2011: +--- !u!114 &11400000 +MonoBehaviour: + m_ObjectHideFlags: 0 + m_CorrespondingSourceObject: {fileID: 0} + m_PrefabInstance: {fileID: 0} + m_PrefabAsset: {fileID: 0} + m_GameObject: {fileID: 0} + m_Enabled: 1 + m_EditorHideFlags: 0 + m_Script: {fileID: 13312, guid: 0000000000000000e000000000000000, type: 0} + m_Name: Dungeon_6 + m_EditorClassIdentifier: + m_Sprite: {fileID: 198299372, guid: 0a806eb0d25e11c48b5dee57fb9339b5, type: 3} + m_Color: {r: 1, g: 1, b: 1, a: 1} + m_Transform: + e00: 1 + e01: 0 + e02: 0 + e03: 0 + e10: 0 + e11: 1 + e12: 0 + e13: 0 + e20: 0 + e21: 0 + e22: 1 + e23: 0 + e30: 0 + e31: 0 + e32: 0 + e33: 1 + m_InstancedGameObject: {fileID: 0} + m_Flags: 1 + m_ColliderType: 1 diff --git a/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_6.asset.meta b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_6.asset.meta new file mode 100644 index 0000000000000000000000000000000000000000..8e64672de28b2d02fd8d37eeef2f33b53a4ca1ee --- /dev/null +++ b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_6.asset.meta @@ -0,0 +1,8 @@ +fileFormatVersion: 2 +guid: 2ead1fba8f3becd49aed19156d21e54c +NativeFormatImporter: + externalObjects: {} + mainObjectFileID: 11400000 + userData: + assetBundleName: + assetBundleVariant: diff --git a/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_60.asset b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_60.asset new file mode 100644 index 0000000000000000000000000000000000000000..ab36ef81c02d474c97c97c1b1d85674ef68a4df9 --- /dev/null +++ b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_60.asset @@ -0,0 +1,36 @@ +%YAML 1.1 +%TAG !u! tag:unity3d.com,2011: +--- !u!114 &11400000 +MonoBehaviour: + m_ObjectHideFlags: 0 + m_CorrespondingSourceObject: {fileID: 0} + m_PrefabInstance: {fileID: 0} + m_PrefabAsset: {fileID: 0} + m_GameObject: {fileID: 0} + m_Enabled: 1 + m_EditorHideFlags: 0 + m_Script: {fileID: 13312, guid: 0000000000000000e000000000000000, type: 0} + m_Name: Dungeon_60 + m_EditorClassIdentifier: + m_Sprite: {fileID: 1660072189, guid: 0a806eb0d25e11c48b5dee57fb9339b5, type: 3} + m_Color: {r: 1, g: 1, b: 1, a: 1} + m_Transform: + e00: 1 + e01: 0 + e02: 0 + e03: 0 + e10: 0 + e11: 1 + e12: 0 + e13: 0 + e20: 0 + e21: 0 + e22: 1 + e23: 0 + e30: 0 + e31: 0 + e32: 0 + e33: 1 + m_InstancedGameObject: {fileID: 0} + m_Flags: 1 + m_ColliderType: 1 diff --git a/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_60.asset.meta b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_60.asset.meta new file mode 100644 index 0000000000000000000000000000000000000000..94aa3098d424c3a6df1ba07d6e4d1e6bfc7626a3 --- /dev/null +++ b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_60.asset.meta @@ -0,0 +1,8 @@ +fileFormatVersion: 2 +guid: 1da059d2ebc6f354494f465adfaaedb8 +NativeFormatImporter: + externalObjects: {} + mainObjectFileID: 11400000 + userData: + assetBundleName: + assetBundleVariant: diff --git a/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_61.asset b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_61.asset new file mode 100644 index 0000000000000000000000000000000000000000..b67c20f87e1509113556b0350733a823008bd8a5 --- /dev/null +++ b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_61.asset @@ -0,0 +1,36 @@ +%YAML 1.1 +%TAG !u! tag:unity3d.com,2011: +--- !u!114 &11400000 +MonoBehaviour: + m_ObjectHideFlags: 0 + m_CorrespondingSourceObject: {fileID: 0} + m_PrefabInstance: {fileID: 0} + m_PrefabAsset: {fileID: 0} + m_GameObject: {fileID: 0} + m_Enabled: 1 + m_EditorHideFlags: 0 + m_Script: {fileID: 13312, guid: 0000000000000000e000000000000000, type: 0} + m_Name: Dungeon_61 + m_EditorClassIdentifier: + m_Sprite: {fileID: -1999030480, guid: 0a806eb0d25e11c48b5dee57fb9339b5, type: 3} + m_Color: {r: 1, g: 1, b: 1, a: 1} + m_Transform: + e00: 1 + e01: 0 + e02: 0 + e03: 0 + e10: 0 + e11: 1 + e12: 0 + e13: 0 + e20: 0 + e21: 0 + e22: 1 + e23: 0 + e30: 0 + e31: 0 + e32: 0 + e33: 1 + m_InstancedGameObject: {fileID: 0} + m_Flags: 1 + m_ColliderType: 1 diff --git a/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_61.asset.meta b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_61.asset.meta new file mode 100644 index 0000000000000000000000000000000000000000..19c080fe6bb86459a35594a152197c7fa9f3befe --- /dev/null +++ b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_61.asset.meta @@ -0,0 +1,8 @@ +fileFormatVersion: 2 +guid: 8fb6991985720cc408646a54158626f8 +NativeFormatImporter: + externalObjects: {} + mainObjectFileID: 11400000 + userData: + assetBundleName: + assetBundleVariant: diff --git a/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_62.asset b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_62.asset new file mode 100644 index 0000000000000000000000000000000000000000..c89c13bc5ad61b0f3d2a978345a6c6bed2900030 --- /dev/null +++ b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_62.asset @@ -0,0 +1,36 @@ +%YAML 1.1 +%TAG !u! tag:unity3d.com,2011: +--- !u!114 &11400000 +MonoBehaviour: + m_ObjectHideFlags: 0 + m_CorrespondingSourceObject: {fileID: 0} + m_PrefabInstance: {fileID: 0} + m_PrefabAsset: {fileID: 0} + m_GameObject: {fileID: 0} + m_Enabled: 1 + m_EditorHideFlags: 0 + m_Script: {fileID: 13312, guid: 0000000000000000e000000000000000, type: 0} + m_Name: Dungeon_62 + m_EditorClassIdentifier: + m_Sprite: {fileID: -62922940, guid: 0a806eb0d25e11c48b5dee57fb9339b5, type: 3} + m_Color: {r: 1, g: 1, b: 1, a: 1} + m_Transform: + e00: 1 + e01: 0 + e02: 0 + e03: 0 + e10: 0 + e11: 1 + e12: 0 + e13: 0 + e20: 0 + e21: 0 + e22: 1 + e23: 0 + e30: 0 + e31: 0 + e32: 0 + e33: 1 + m_InstancedGameObject: {fileID: 0} + m_Flags: 1 + m_ColliderType: 1 diff --git a/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_62.asset.meta b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_62.asset.meta new file mode 100644 index 0000000000000000000000000000000000000000..07d8f91ca834871b07a2f3d7d1fbe0c0cd74cbad --- /dev/null +++ b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_62.asset.meta @@ -0,0 +1,8 @@ +fileFormatVersion: 2 +guid: a31dae3bd70797649a110851518ca16f +NativeFormatImporter: + externalObjects: {} + mainObjectFileID: 11400000 + userData: + assetBundleName: + assetBundleVariant: diff --git a/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_63.asset b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_63.asset new file mode 100644 index 0000000000000000000000000000000000000000..e2cc6fca29f6312bcf874444b29876c27dc5840b --- /dev/null +++ b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_63.asset @@ -0,0 +1,36 @@ +%YAML 1.1 +%TAG !u! tag:unity3d.com,2011: +--- !u!114 &11400000 +MonoBehaviour: + m_ObjectHideFlags: 0 + m_CorrespondingSourceObject: {fileID: 0} + m_PrefabInstance: {fileID: 0} + m_PrefabAsset: {fileID: 0} + m_GameObject: {fileID: 0} + m_Enabled: 1 + m_EditorHideFlags: 0 + m_Script: {fileID: 13312, guid: 0000000000000000e000000000000000, type: 0} + m_Name: Dungeon_63 + m_EditorClassIdentifier: + m_Sprite: {fileID: 154182392, guid: 0a806eb0d25e11c48b5dee57fb9339b5, type: 3} + m_Color: {r: 1, g: 1, b: 1, a: 1} + m_Transform: + e00: 1 + e01: 0 + e02: 0 + e03: 0 + e10: 0 + e11: 1 + e12: 0 + e13: 0 + e20: 0 + e21: 0 + e22: 1 + e23: 0 + e30: 0 + e31: 0 + e32: 0 + e33: 1 + m_InstancedGameObject: {fileID: 0} + m_Flags: 1 + m_ColliderType: 1 diff --git a/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_63.asset.meta b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_63.asset.meta new file mode 100644 index 0000000000000000000000000000000000000000..4471d908570c8c1b9ac7a8f987c8de63d40fb072 --- /dev/null +++ b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_63.asset.meta @@ -0,0 +1,8 @@ +fileFormatVersion: 2 +guid: f6adb266f61c3d44fb326964d0f44ea9 +NativeFormatImporter: + externalObjects: {} + mainObjectFileID: 11400000 + userData: + assetBundleName: + assetBundleVariant: diff --git a/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_64.asset b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_64.asset new file mode 100644 index 0000000000000000000000000000000000000000..6e2204002be596a7d3a7f83f8d4694097407b4a3 --- /dev/null +++ b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_64.asset @@ -0,0 +1,36 @@ +%YAML 1.1 +%TAG !u! tag:unity3d.com,2011: +--- !u!114 &11400000 +MonoBehaviour: + m_ObjectHideFlags: 0 + m_CorrespondingSourceObject: {fileID: 0} + m_PrefabInstance: {fileID: 0} + m_PrefabAsset: {fileID: 0} + m_GameObject: {fileID: 0} + m_Enabled: 1 + m_EditorHideFlags: 0 + m_Script: {fileID: 13312, guid: 0000000000000000e000000000000000, type: 0} + m_Name: Dungeon_64 + m_EditorClassIdentifier: + m_Sprite: {fileID: -38314673, guid: 0a806eb0d25e11c48b5dee57fb9339b5, type: 3} + m_Color: {r: 1, g: 1, b: 1, a: 1} + m_Transform: + e00: 1 + e01: 0 + e02: 0 + e03: 0 + e10: 0 + e11: 1 + e12: 0 + e13: 0 + e20: 0 + e21: 0 + e22: 1 + e23: 0 + e30: 0 + e31: 0 + e32: 0 + e33: 1 + m_InstancedGameObject: {fileID: 0} + m_Flags: 1 + m_ColliderType: 1 diff --git a/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_64.asset.meta b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_64.asset.meta new file mode 100644 index 0000000000000000000000000000000000000000..3a5668cad9d31e60a2a101af7c5a226de2b0ad40 --- /dev/null +++ b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_64.asset.meta @@ -0,0 +1,8 @@ +fileFormatVersion: 2 +guid: c366a769491273347aa4de6d628d37af +NativeFormatImporter: + externalObjects: {} + mainObjectFileID: 11400000 + userData: + assetBundleName: + assetBundleVariant: diff --git a/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_65.asset b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_65.asset new file mode 100644 index 0000000000000000000000000000000000000000..c1074d93cb68993aa5146f22c0ced9f9150a23bd --- /dev/null +++ b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_65.asset @@ -0,0 +1,36 @@ +%YAML 1.1 +%TAG !u! tag:unity3d.com,2011: +--- !u!114 &11400000 +MonoBehaviour: + m_ObjectHideFlags: 0 + m_CorrespondingSourceObject: {fileID: 0} + m_PrefabInstance: {fileID: 0} + m_PrefabAsset: {fileID: 0} + m_GameObject: {fileID: 0} + m_Enabled: 1 + m_EditorHideFlags: 0 + m_Script: {fileID: 13312, guid: 0000000000000000e000000000000000, type: 0} + m_Name: Dungeon_65 + m_EditorClassIdentifier: + m_Sprite: {fileID: 442919366, guid: 0a806eb0d25e11c48b5dee57fb9339b5, type: 3} + m_Color: {r: 1, g: 1, b: 1, a: 1} + m_Transform: + e00: 1 + e01: 0 + e02: 0 + e03: 0 + e10: 0 + e11: 1 + e12: 0 + e13: 0 + e20: 0 + e21: 0 + e22: 1 + e23: 0 + e30: 0 + e31: 0 + e32: 0 + e33: 1 + m_InstancedGameObject: {fileID: 0} + m_Flags: 1 + m_ColliderType: 1 diff --git a/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_65.asset.meta b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_65.asset.meta new file mode 100644 index 0000000000000000000000000000000000000000..0801d940fce1444405e39bfe50a8c184e749cf47 --- /dev/null +++ b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_65.asset.meta @@ -0,0 +1,8 @@ +fileFormatVersion: 2 +guid: ade8c7170fa6c0145a49e498ac98644e +NativeFormatImporter: + externalObjects: {} + mainObjectFileID: 11400000 + userData: + assetBundleName: + assetBundleVariant: diff --git a/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_66.asset b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_66.asset new file mode 100644 index 0000000000000000000000000000000000000000..063bdf7a442435af14673cb0014c3f9b4276636b --- /dev/null +++ b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_66.asset @@ -0,0 +1,36 @@ +%YAML 1.1 +%TAG !u! tag:unity3d.com,2011: +--- !u!114 &11400000 +MonoBehaviour: + m_ObjectHideFlags: 0 + m_CorrespondingSourceObject: {fileID: 0} + m_PrefabInstance: {fileID: 0} + m_PrefabAsset: {fileID: 0} + m_GameObject: {fileID: 0} + m_Enabled: 1 + m_EditorHideFlags: 0 + m_Script: {fileID: 13312, guid: 0000000000000000e000000000000000, type: 0} + m_Name: Dungeon_66 + m_EditorClassIdentifier: + m_Sprite: {fileID: -521188253, guid: 0a806eb0d25e11c48b5dee57fb9339b5, type: 3} + m_Color: {r: 1, g: 1, b: 1, a: 1} + m_Transform: + e00: 1 + e01: 0 + e02: 0 + e03: 0 + e10: 0 + e11: 1 + e12: 0 + e13: 0 + e20: 0 + e21: 0 + e22: 1 + e23: 0 + e30: 0 + e31: 0 + e32: 0 + e33: 1 + m_InstancedGameObject: {fileID: 0} + m_Flags: 1 + m_ColliderType: 1 diff --git a/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_66.asset.meta b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_66.asset.meta new file mode 100644 index 0000000000000000000000000000000000000000..ace08ef4692c7c931c17bcbbc27fe20884c26b5a --- /dev/null +++ b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_66.asset.meta @@ -0,0 +1,8 @@ +fileFormatVersion: 2 +guid: 75e564577f6e73441be6bf6e336d07f7 +NativeFormatImporter: + externalObjects: {} + mainObjectFileID: 11400000 + userData: + assetBundleName: + assetBundleVariant: diff --git a/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_67.asset b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_67.asset new file mode 100644 index 0000000000000000000000000000000000000000..1f126c81f7dae103aa4dd77ab0924f5cd188b613 --- /dev/null +++ b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_67.asset @@ -0,0 +1,36 @@ +%YAML 1.1 +%TAG !u! tag:unity3d.com,2011: +--- !u!114 &11400000 +MonoBehaviour: + m_ObjectHideFlags: 0 + m_CorrespondingSourceObject: {fileID: 0} + m_PrefabInstance: {fileID: 0} + m_PrefabAsset: {fileID: 0} + m_GameObject: {fileID: 0} + m_Enabled: 1 + m_EditorHideFlags: 0 + m_Script: {fileID: 13312, guid: 0000000000000000e000000000000000, type: 0} + m_Name: Dungeon_67 + m_EditorClassIdentifier: + m_Sprite: {fileID: -428477728, guid: 0a806eb0d25e11c48b5dee57fb9339b5, type: 3} + m_Color: {r: 1, g: 1, b: 1, a: 1} + m_Transform: + e00: 1 + e01: 0 + e02: 0 + e03: 0 + e10: 0 + e11: 1 + e12: 0 + e13: 0 + e20: 0 + e21: 0 + e22: 1 + e23: 0 + e30: 0 + e31: 0 + e32: 0 + e33: 1 + m_InstancedGameObject: {fileID: 0} + m_Flags: 1 + m_ColliderType: 1 diff --git a/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_67.asset.meta b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_67.asset.meta new file mode 100644 index 0000000000000000000000000000000000000000..188192d894cb4e4d032da8d4c62ecd7dddef45ab --- /dev/null +++ b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_67.asset.meta @@ -0,0 +1,8 @@ +fileFormatVersion: 2 +guid: 2411437a6c35d8944bc9bea58d0e1c09 +NativeFormatImporter: + externalObjects: {} + mainObjectFileID: 11400000 + userData: + assetBundleName: + assetBundleVariant: diff --git a/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_68.asset b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_68.asset new file mode 100644 index 0000000000000000000000000000000000000000..33c13e2145163ea4e98dd2d95187e245b037df7e --- /dev/null +++ b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_68.asset @@ -0,0 +1,36 @@ +%YAML 1.1 +%TAG !u! tag:unity3d.com,2011: +--- !u!114 &11400000 +MonoBehaviour: + m_ObjectHideFlags: 0 + m_CorrespondingSourceObject: {fileID: 0} + m_PrefabInstance: {fileID: 0} + m_PrefabAsset: {fileID: 0} + m_GameObject: {fileID: 0} + m_Enabled: 1 + m_EditorHideFlags: 0 + m_Script: {fileID: 13312, guid: 0000000000000000e000000000000000, type: 0} + m_Name: Dungeon_68 + m_EditorClassIdentifier: + m_Sprite: {fileID: -2045571822, guid: 0a806eb0d25e11c48b5dee57fb9339b5, type: 3} + m_Color: {r: 1, g: 1, b: 1, a: 1} + m_Transform: + e00: 1 + e01: 0 + e02: 0 + e03: 0 + e10: 0 + e11: 1 + e12: 0 + e13: 0 + e20: 0 + e21: 0 + e22: 1 + e23: 0 + e30: 0 + e31: 0 + e32: 0 + e33: 1 + m_InstancedGameObject: {fileID: 0} + m_Flags: 1 + m_ColliderType: 1 diff --git a/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_68.asset.meta b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_68.asset.meta new file mode 100644 index 0000000000000000000000000000000000000000..d1d8e2a6de4573641b11e2416dfba75c63e12686 --- /dev/null +++ b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_68.asset.meta @@ -0,0 +1,8 @@ +fileFormatVersion: 2 +guid: a1643267c869f4a4b9c3f1aabc8156a2 +NativeFormatImporter: + externalObjects: {} + mainObjectFileID: 11400000 + userData: + assetBundleName: + assetBundleVariant: diff --git a/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_69.asset b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_69.asset new file mode 100644 index 0000000000000000000000000000000000000000..82aad9eb39a6787dee87e7a175c51b7eb1903220 --- /dev/null +++ b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_69.asset @@ -0,0 +1,36 @@ +%YAML 1.1 +%TAG !u! tag:unity3d.com,2011: +--- !u!114 &11400000 +MonoBehaviour: + m_ObjectHideFlags: 0 + m_CorrespondingSourceObject: {fileID: 0} + m_PrefabInstance: {fileID: 0} + m_PrefabAsset: {fileID: 0} + m_GameObject: {fileID: 0} + m_Enabled: 1 + m_EditorHideFlags: 0 + m_Script: {fileID: 13312, guid: 0000000000000000e000000000000000, type: 0} + m_Name: Dungeon_69 + m_EditorClassIdentifier: + m_Sprite: {fileID: -839279710, guid: 0a806eb0d25e11c48b5dee57fb9339b5, type: 3} + m_Color: {r: 1, g: 1, b: 1, a: 1} + m_Transform: + e00: 1 + e01: 0 + e02: 0 + e03: 0 + e10: 0 + e11: 1 + e12: 0 + e13: 0 + e20: 0 + e21: 0 + e22: 1 + e23: 0 + e30: 0 + e31: 0 + e32: 0 + e33: 1 + m_InstancedGameObject: {fileID: 0} + m_Flags: 1 + m_ColliderType: 1 diff --git a/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_69.asset.meta b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_69.asset.meta new file mode 100644 index 0000000000000000000000000000000000000000..b8d0852b68b7d1f1340fbb77e96de3a5fa6b0eac --- /dev/null +++ b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_69.asset.meta @@ -0,0 +1,8 @@ +fileFormatVersion: 2 +guid: 59f56605b41b89c46bab93964ba3efd7 +NativeFormatImporter: + externalObjects: {} + mainObjectFileID: 11400000 + userData: + assetBundleName: + assetBundleVariant: diff --git a/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_7.asset b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_7.asset new file mode 100644 index 0000000000000000000000000000000000000000..a3564f1bba475960c815f6b0fb7019de07b94639 --- /dev/null +++ b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_7.asset @@ -0,0 +1,36 @@ +%YAML 1.1 +%TAG !u! tag:unity3d.com,2011: +--- !u!114 &11400000 +MonoBehaviour: + m_ObjectHideFlags: 0 + m_CorrespondingSourceObject: {fileID: 0} + m_PrefabInstance: {fileID: 0} + m_PrefabAsset: {fileID: 0} + m_GameObject: {fileID: 0} + m_Enabled: 1 + m_EditorHideFlags: 0 + m_Script: {fileID: 13312, guid: 0000000000000000e000000000000000, type: 0} + m_Name: Dungeon_7 + m_EditorClassIdentifier: + m_Sprite: {fileID: -650063954, guid: 0a806eb0d25e11c48b5dee57fb9339b5, type: 3} + m_Color: {r: 1, g: 1, b: 1, a: 1} + m_Transform: + e00: 1 + e01: 0 + e02: 0 + e03: 0 + e10: 0 + e11: 1 + e12: 0 + e13: 0 + e20: 0 + e21: 0 + e22: 1 + e23: 0 + e30: 0 + e31: 0 + e32: 0 + e33: 1 + m_InstancedGameObject: {fileID: 0} + m_Flags: 1 + m_ColliderType: 1 diff --git a/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_7.asset.meta b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_7.asset.meta new file mode 100644 index 0000000000000000000000000000000000000000..21824552a78628ae143d35fbf350ec178cb5650b --- /dev/null +++ b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_7.asset.meta @@ -0,0 +1,8 @@ +fileFormatVersion: 2 +guid: a23d356dc7ef26b42afb97e7a7e87330 +NativeFormatImporter: + externalObjects: {} + mainObjectFileID: 11400000 + userData: + assetBundleName: + assetBundleVariant: diff --git a/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_70.asset b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_70.asset new file mode 100644 index 0000000000000000000000000000000000000000..93e8a7f94449b8e36805aa9d5259509efa09f8ab --- /dev/null +++ b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_70.asset @@ -0,0 +1,36 @@ +%YAML 1.1 +%TAG !u! tag:unity3d.com,2011: +--- !u!114 &11400000 +MonoBehaviour: + m_ObjectHideFlags: 0 + m_CorrespondingSourceObject: {fileID: 0} + m_PrefabInstance: {fileID: 0} + m_PrefabAsset: {fileID: 0} + m_GameObject: {fileID: 0} + m_Enabled: 1 + m_EditorHideFlags: 0 + m_Script: {fileID: 13312, guid: 0000000000000000e000000000000000, type: 0} + m_Name: Dungeon_70 + m_EditorClassIdentifier: + m_Sprite: {fileID: 2090772569, guid: 0a806eb0d25e11c48b5dee57fb9339b5, type: 3} + m_Color: {r: 1, g: 1, b: 1, a: 1} + m_Transform: + e00: 1 + e01: 0 + e02: 0 + e03: 0 + e10: 0 + e11: 1 + e12: 0 + e13: 0 + e20: 0 + e21: 0 + e22: 1 + e23: 0 + e30: 0 + e31: 0 + e32: 0 + e33: 1 + m_InstancedGameObject: {fileID: 0} + m_Flags: 1 + m_ColliderType: 1 diff --git a/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_70.asset.meta b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_70.asset.meta new file mode 100644 index 0000000000000000000000000000000000000000..77c38ef84ab91d0a9b3a3d583824d0eb13590115 --- /dev/null +++ b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_70.asset.meta @@ -0,0 +1,8 @@ +fileFormatVersion: 2 +guid: ef1e419e402ec864dbe97f93e50fc7bb +NativeFormatImporter: + externalObjects: {} + mainObjectFileID: 11400000 + userData: + assetBundleName: + assetBundleVariant: diff --git a/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_71.asset b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_71.asset new file mode 100644 index 0000000000000000000000000000000000000000..487de34d12eb1f36136ce862297690fe5921b266 --- /dev/null +++ b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_71.asset @@ -0,0 +1,36 @@ +%YAML 1.1 +%TAG !u! tag:unity3d.com,2011: +--- !u!114 &11400000 +MonoBehaviour: + m_ObjectHideFlags: 0 + m_CorrespondingSourceObject: {fileID: 0} + m_PrefabInstance: {fileID: 0} + m_PrefabAsset: {fileID: 0} + m_GameObject: {fileID: 0} + m_Enabled: 1 + m_EditorHideFlags: 0 + m_Script: {fileID: 13312, guid: 0000000000000000e000000000000000, type: 0} + m_Name: Dungeon_71 + m_EditorClassIdentifier: + m_Sprite: {fileID: -1297728808, guid: 0a806eb0d25e11c48b5dee57fb9339b5, type: 3} + m_Color: {r: 1, g: 1, b: 1, a: 1} + m_Transform: + e00: 1 + e01: 0 + e02: 0 + e03: 0 + e10: 0 + e11: 1 + e12: 0 + e13: 0 + e20: 0 + e21: 0 + e22: 1 + e23: 0 + e30: 0 + e31: 0 + e32: 0 + e33: 1 + m_InstancedGameObject: {fileID: 0} + m_Flags: 1 + m_ColliderType: 1 diff --git a/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_71.asset.meta b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_71.asset.meta new file mode 100644 index 0000000000000000000000000000000000000000..ac6826e6a29722c9b026683f081134a3c0d7d3f9 --- /dev/null +++ b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_71.asset.meta @@ -0,0 +1,8 @@ +fileFormatVersion: 2 +guid: b521b926ffbbcaf42a960d4b96b8e0c9 +NativeFormatImporter: + externalObjects: {} + mainObjectFileID: 11400000 + userData: + assetBundleName: + assetBundleVariant: diff --git a/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_72.asset b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_72.asset new file mode 100644 index 0000000000000000000000000000000000000000..85aa54f4977ef69e5142468fd55027148b2a8dd8 --- /dev/null +++ b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_72.asset @@ -0,0 +1,36 @@ +%YAML 1.1 +%TAG !u! tag:unity3d.com,2011: +--- !u!114 &11400000 +MonoBehaviour: + m_ObjectHideFlags: 0 + m_CorrespondingSourceObject: {fileID: 0} + m_PrefabInstance: {fileID: 0} + m_PrefabAsset: {fileID: 0} + m_GameObject: {fileID: 0} + m_Enabled: 1 + m_EditorHideFlags: 0 + m_Script: {fileID: 13312, guid: 0000000000000000e000000000000000, type: 0} + m_Name: Dungeon_72 + m_EditorClassIdentifier: + m_Sprite: {fileID: -1473822626, guid: 0a806eb0d25e11c48b5dee57fb9339b5, type: 3} + m_Color: {r: 1, g: 1, b: 1, a: 1} + m_Transform: + e00: 1 + e01: 0 + e02: 0 + e03: 0 + e10: 0 + e11: 1 + e12: 0 + e13: 0 + e20: 0 + e21: 0 + e22: 1 + e23: 0 + e30: 0 + e31: 0 + e32: 0 + e33: 1 + m_InstancedGameObject: {fileID: 0} + m_Flags: 1 + m_ColliderType: 1 diff --git a/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_72.asset.meta b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_72.asset.meta new file mode 100644 index 0000000000000000000000000000000000000000..733c368433a556c115d0930471fde7ae87340550 --- /dev/null +++ b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_72.asset.meta @@ -0,0 +1,8 @@ +fileFormatVersion: 2 +guid: e61fae997815a4a4b8d2e6cc611327f8 +NativeFormatImporter: + externalObjects: {} + mainObjectFileID: 11400000 + userData: + assetBundleName: + assetBundleVariant: diff --git a/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_73.asset b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_73.asset new file mode 100644 index 0000000000000000000000000000000000000000..280414bd1553012059a8d2a6d19f0d84bb7af1ac --- /dev/null +++ b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_73.asset @@ -0,0 +1,36 @@ +%YAML 1.1 +%TAG !u! tag:unity3d.com,2011: +--- !u!114 &11400000 +MonoBehaviour: + m_ObjectHideFlags: 0 + m_CorrespondingSourceObject: {fileID: 0} + m_PrefabInstance: {fileID: 0} + m_PrefabAsset: {fileID: 0} + m_GameObject: {fileID: 0} + m_Enabled: 1 + m_EditorHideFlags: 0 + m_Script: {fileID: 13312, guid: 0000000000000000e000000000000000, type: 0} + m_Name: Dungeon_73 + m_EditorClassIdentifier: + m_Sprite: {fileID: -1760836496, guid: 0a806eb0d25e11c48b5dee57fb9339b5, type: 3} + m_Color: {r: 1, g: 1, b: 1, a: 1} + m_Transform: + e00: 1 + e01: 0 + e02: 0 + e03: 0 + e10: 0 + e11: 1 + e12: 0 + e13: 0 + e20: 0 + e21: 0 + e22: 1 + e23: 0 + e30: 0 + e31: 0 + e32: 0 + e33: 1 + m_InstancedGameObject: {fileID: 0} + m_Flags: 1 + m_ColliderType: 1 diff --git a/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_73.asset.meta b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_73.asset.meta new file mode 100644 index 0000000000000000000000000000000000000000..8c47e739f0da19ac109f561226d45583605a89a0 --- /dev/null +++ b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_73.asset.meta @@ -0,0 +1,8 @@ +fileFormatVersion: 2 +guid: 69d9c223832b9954c8371363f9fe9d72 +NativeFormatImporter: + externalObjects: {} + mainObjectFileID: 11400000 + userData: + assetBundleName: + assetBundleVariant: diff --git a/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_74.asset b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_74.asset new file mode 100644 index 0000000000000000000000000000000000000000..6485b270734e764b0262cf0b14d4a5636d563874 --- /dev/null +++ b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_74.asset @@ -0,0 +1,36 @@ +%YAML 1.1 +%TAG !u! tag:unity3d.com,2011: +--- !u!114 &11400000 +MonoBehaviour: + m_ObjectHideFlags: 0 + m_CorrespondingSourceObject: {fileID: 0} + m_PrefabInstance: {fileID: 0} + m_PrefabAsset: {fileID: 0} + m_GameObject: {fileID: 0} + m_Enabled: 1 + m_EditorHideFlags: 0 + m_Script: {fileID: 13312, guid: 0000000000000000e000000000000000, type: 0} + m_Name: Dungeon_74 + m_EditorClassIdentifier: + m_Sprite: {fileID: 1547048006, guid: 0a806eb0d25e11c48b5dee57fb9339b5, type: 3} + m_Color: {r: 1, g: 1, b: 1, a: 1} + m_Transform: + e00: 1 + e01: 0 + e02: 0 + e03: 0 + e10: 0 + e11: 1 + e12: 0 + e13: 0 + e20: 0 + e21: 0 + e22: 1 + e23: 0 + e30: 0 + e31: 0 + e32: 0 + e33: 1 + m_InstancedGameObject: {fileID: 0} + m_Flags: 1 + m_ColliderType: 1 diff --git a/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_74.asset.meta b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_74.asset.meta new file mode 100644 index 0000000000000000000000000000000000000000..d6a09a4395cbfb273589b5fae8ff427428c6fd34 --- /dev/null +++ b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_74.asset.meta @@ -0,0 +1,8 @@ +fileFormatVersion: 2 +guid: 81dbd4adc8f925741b8d2aee5f076335 +NativeFormatImporter: + externalObjects: {} + mainObjectFileID: 11400000 + userData: + assetBundleName: + assetBundleVariant: diff --git a/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_75.asset b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_75.asset new file mode 100644 index 0000000000000000000000000000000000000000..ddecf6dfaad4a63742d8fe1d805258810c078d2e --- /dev/null +++ b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_75.asset @@ -0,0 +1,36 @@ +%YAML 1.1 +%TAG !u! tag:unity3d.com,2011: +--- !u!114 &11400000 +MonoBehaviour: + m_ObjectHideFlags: 0 + m_CorrespondingSourceObject: {fileID: 0} + m_PrefabInstance: {fileID: 0} + m_PrefabAsset: {fileID: 0} + m_GameObject: {fileID: 0} + m_Enabled: 1 + m_EditorHideFlags: 0 + m_Script: {fileID: 13312, guid: 0000000000000000e000000000000000, type: 0} + m_Name: Dungeon_75 + m_EditorClassIdentifier: + m_Sprite: {fileID: 613834084, guid: 0a806eb0d25e11c48b5dee57fb9339b5, type: 3} + m_Color: {r: 1, g: 1, b: 1, a: 1} + m_Transform: + e00: 1 + e01: 0 + e02: 0 + e03: 0 + e10: 0 + e11: 1 + e12: 0 + e13: 0 + e20: 0 + e21: 0 + e22: 1 + e23: 0 + e30: 0 + e31: 0 + e32: 0 + e33: 1 + m_InstancedGameObject: {fileID: 0} + m_Flags: 1 + m_ColliderType: 1 diff --git a/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_75.asset.meta b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_75.asset.meta new file mode 100644 index 0000000000000000000000000000000000000000..c9e854b84042f64876526c57d4982b8c41e7e109 --- /dev/null +++ b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_75.asset.meta @@ -0,0 +1,8 @@ +fileFormatVersion: 2 +guid: 9b83c35937e6ba542b5162858b841d57 +NativeFormatImporter: + externalObjects: {} + mainObjectFileID: 11400000 + userData: + assetBundleName: + assetBundleVariant: diff --git a/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_76.asset b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_76.asset new file mode 100644 index 0000000000000000000000000000000000000000..283231b3d9276502c691e8b9a56ed70bed1292e3 --- /dev/null +++ b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_76.asset @@ -0,0 +1,36 @@ +%YAML 1.1 +%TAG !u! tag:unity3d.com,2011: +--- !u!114 &11400000 +MonoBehaviour: + m_ObjectHideFlags: 0 + m_CorrespondingSourceObject: {fileID: 0} + m_PrefabInstance: {fileID: 0} + m_PrefabAsset: {fileID: 0} + m_GameObject: {fileID: 0} + m_Enabled: 1 + m_EditorHideFlags: 0 + m_Script: {fileID: 13312, guid: 0000000000000000e000000000000000, type: 0} + m_Name: Dungeon_76 + m_EditorClassIdentifier: + m_Sprite: {fileID: 1270900073, guid: 0a806eb0d25e11c48b5dee57fb9339b5, type: 3} + m_Color: {r: 1, g: 1, b: 1, a: 1} + m_Transform: + e00: 1 + e01: 0 + e02: 0 + e03: 0 + e10: 0 + e11: 1 + e12: 0 + e13: 0 + e20: 0 + e21: 0 + e22: 1 + e23: 0 + e30: 0 + e31: 0 + e32: 0 + e33: 1 + m_InstancedGameObject: {fileID: 0} + m_Flags: 1 + m_ColliderType: 1 diff --git a/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_76.asset.meta b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_76.asset.meta new file mode 100644 index 0000000000000000000000000000000000000000..a6199391abca53005a3733d856e2ea99825a2fa0 --- /dev/null +++ b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_76.asset.meta @@ -0,0 +1,8 @@ +fileFormatVersion: 2 +guid: 37c818adc58b19a4a9e160f4e0c289d1 +NativeFormatImporter: + externalObjects: {} + mainObjectFileID: 11400000 + userData: + assetBundleName: + assetBundleVariant: diff --git a/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_77.asset b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_77.asset new file mode 100644 index 0000000000000000000000000000000000000000..51d53638ca4f0a4a74fe8c1fc0037a8251a9b528 --- /dev/null +++ b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_77.asset @@ -0,0 +1,36 @@ +%YAML 1.1 +%TAG !u! tag:unity3d.com,2011: +--- !u!114 &11400000 +MonoBehaviour: + m_ObjectHideFlags: 0 + m_CorrespondingSourceObject: {fileID: 0} + m_PrefabInstance: {fileID: 0} + m_PrefabAsset: {fileID: 0} + m_GameObject: {fileID: 0} + m_Enabled: 1 + m_EditorHideFlags: 0 + m_Script: {fileID: 13312, guid: 0000000000000000e000000000000000, type: 0} + m_Name: Dungeon_77 + m_EditorClassIdentifier: + m_Sprite: {fileID: 2137237813, guid: 0a806eb0d25e11c48b5dee57fb9339b5, type: 3} + m_Color: {r: 1, g: 1, b: 1, a: 1} + m_Transform: + e00: 1 + e01: 0 + e02: 0 + e03: 0 + e10: 0 + e11: 1 + e12: 0 + e13: 0 + e20: 0 + e21: 0 + e22: 1 + e23: 0 + e30: 0 + e31: 0 + e32: 0 + e33: 1 + m_InstancedGameObject: {fileID: 0} + m_Flags: 1 + m_ColliderType: 1 diff --git a/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_77.asset.meta b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_77.asset.meta new file mode 100644 index 0000000000000000000000000000000000000000..244b1068fecd9ac03d9ab07a28154848e7e8ecad --- /dev/null +++ b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_77.asset.meta @@ -0,0 +1,8 @@ +fileFormatVersion: 2 +guid: 725ac7f68af6b7646922285011a3f343 +NativeFormatImporter: + externalObjects: {} + mainObjectFileID: 11400000 + userData: + assetBundleName: + assetBundleVariant: diff --git a/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_78.asset b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_78.asset new file mode 100644 index 0000000000000000000000000000000000000000..8ef55b27a2a0d56ac7cec9a5fea6b2a420c5d2cf --- /dev/null +++ b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_78.asset @@ -0,0 +1,36 @@ +%YAML 1.1 +%TAG !u! tag:unity3d.com,2011: +--- !u!114 &11400000 +MonoBehaviour: + m_ObjectHideFlags: 0 + m_CorrespondingSourceObject: {fileID: 0} + m_PrefabInstance: {fileID: 0} + m_PrefabAsset: {fileID: 0} + m_GameObject: {fileID: 0} + m_Enabled: 1 + m_EditorHideFlags: 0 + m_Script: {fileID: 13312, guid: 0000000000000000e000000000000000, type: 0} + m_Name: Dungeon_78 + m_EditorClassIdentifier: + m_Sprite: {fileID: 1460241374, guid: 0a806eb0d25e11c48b5dee57fb9339b5, type: 3} + m_Color: {r: 1, g: 1, b: 1, a: 1} + m_Transform: + e00: 1 + e01: 0 + e02: 0 + e03: 0 + e10: 0 + e11: 1 + e12: 0 + e13: 0 + e20: 0 + e21: 0 + e22: 1 + e23: 0 + e30: 0 + e31: 0 + e32: 0 + e33: 1 + m_InstancedGameObject: {fileID: 0} + m_Flags: 1 + m_ColliderType: 1 diff --git a/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_78.asset.meta b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_78.asset.meta new file mode 100644 index 0000000000000000000000000000000000000000..9be50c28a0d1581a9059ab65035fdc1e0df45030 --- /dev/null +++ b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_78.asset.meta @@ -0,0 +1,8 @@ +fileFormatVersion: 2 +guid: 89f154c4c20759a43b960c725c916389 +NativeFormatImporter: + externalObjects: {} + mainObjectFileID: 11400000 + userData: + assetBundleName: + assetBundleVariant: diff --git a/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_79.asset b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_79.asset new file mode 100644 index 0000000000000000000000000000000000000000..eb67be0c6e752d1e21dd03c62fbf3ec5dc9db7da --- /dev/null +++ b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_79.asset @@ -0,0 +1,36 @@ +%YAML 1.1 +%TAG !u! tag:unity3d.com,2011: +--- !u!114 &11400000 +MonoBehaviour: + m_ObjectHideFlags: 0 + m_CorrespondingSourceObject: {fileID: 0} + m_PrefabInstance: {fileID: 0} + m_PrefabAsset: {fileID: 0} + m_GameObject: {fileID: 0} + m_Enabled: 1 + m_EditorHideFlags: 0 + m_Script: {fileID: 13312, guid: 0000000000000000e000000000000000, type: 0} + m_Name: Dungeon_79 + m_EditorClassIdentifier: + m_Sprite: {fileID: -1614669064, guid: 0a806eb0d25e11c48b5dee57fb9339b5, type: 3} + m_Color: {r: 1, g: 1, b: 1, a: 1} + m_Transform: + e00: 1 + e01: 0 + e02: 0 + e03: 0 + e10: 0 + e11: 1 + e12: 0 + e13: 0 + e20: 0 + e21: 0 + e22: 1 + e23: 0 + e30: 0 + e31: 0 + e32: 0 + e33: 1 + m_InstancedGameObject: {fileID: 0} + m_Flags: 1 + m_ColliderType: 1 diff --git a/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_79.asset.meta b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_79.asset.meta new file mode 100644 index 0000000000000000000000000000000000000000..c566b9fc16895e720f536ab01963c30600978e2b --- /dev/null +++ b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_79.asset.meta @@ -0,0 +1,8 @@ +fileFormatVersion: 2 +guid: 2451da60eae72804ea1516793136c130 +NativeFormatImporter: + externalObjects: {} + mainObjectFileID: 11400000 + userData: + assetBundleName: + assetBundleVariant: diff --git a/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_8.asset b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_8.asset new file mode 100644 index 0000000000000000000000000000000000000000..57a3db38b5b915045451ed73db1432f2d1379663 --- /dev/null +++ b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_8.asset @@ -0,0 +1,36 @@ +%YAML 1.1 +%TAG !u! tag:unity3d.com,2011: +--- !u!114 &11400000 +MonoBehaviour: + m_ObjectHideFlags: 0 + m_CorrespondingSourceObject: {fileID: 0} + m_PrefabInstance: {fileID: 0} + m_PrefabAsset: {fileID: 0} + m_GameObject: {fileID: 0} + m_Enabled: 1 + m_EditorHideFlags: 0 + m_Script: {fileID: 13312, guid: 0000000000000000e000000000000000, type: 0} + m_Name: Dungeon_8 + m_EditorClassIdentifier: + m_Sprite: {fileID: -1536037944, guid: 0a806eb0d25e11c48b5dee57fb9339b5, type: 3} + m_Color: {r: 1, g: 1, b: 1, a: 1} + m_Transform: + e00: 1 + e01: 0 + e02: 0 + e03: 0 + e10: 0 + e11: 1 + e12: 0 + e13: 0 + e20: 0 + e21: 0 + e22: 1 + e23: 0 + e30: 0 + e31: 0 + e32: 0 + e33: 1 + m_InstancedGameObject: {fileID: 0} + m_Flags: 1 + m_ColliderType: 1 diff --git a/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_8.asset.meta b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_8.asset.meta new file mode 100644 index 0000000000000000000000000000000000000000..adb8ebb743a45de6a8de0fcd15e2d7d93d228356 --- /dev/null +++ b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_8.asset.meta @@ -0,0 +1,8 @@ +fileFormatVersion: 2 +guid: 13e364b0eaf450c4095d73340ee6a58c +NativeFormatImporter: + externalObjects: {} + mainObjectFileID: 11400000 + userData: + assetBundleName: + assetBundleVariant: diff --git a/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_80.asset b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_80.asset new file mode 100644 index 0000000000000000000000000000000000000000..58c35e64b74448d176bed330485a739453b0b50b --- /dev/null +++ b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_80.asset @@ -0,0 +1,36 @@ +%YAML 1.1 +%TAG !u! tag:unity3d.com,2011: +--- !u!114 &11400000 +MonoBehaviour: + m_ObjectHideFlags: 0 + m_CorrespondingSourceObject: {fileID: 0} + m_PrefabInstance: {fileID: 0} + m_PrefabAsset: {fileID: 0} + m_GameObject: {fileID: 0} + m_Enabled: 1 + m_EditorHideFlags: 0 + m_Script: {fileID: 13312, guid: 0000000000000000e000000000000000, type: 0} + m_Name: Dungeon_80 + m_EditorClassIdentifier: + m_Sprite: {fileID: 1642675801, guid: 0a806eb0d25e11c48b5dee57fb9339b5, type: 3} + m_Color: {r: 1, g: 1, b: 1, a: 1} + m_Transform: + e00: 1 + e01: 0 + e02: 0 + e03: 0 + e10: 0 + e11: 1 + e12: 0 + e13: 0 + e20: 0 + e21: 0 + e22: 1 + e23: 0 + e30: 0 + e31: 0 + e32: 0 + e33: 1 + m_InstancedGameObject: {fileID: 0} + m_Flags: 1 + m_ColliderType: 1 diff --git a/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_80.asset.meta b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_80.asset.meta new file mode 100644 index 0000000000000000000000000000000000000000..f927194f3a77a1a37d24154b6b8f50c4806b4b87 --- /dev/null +++ b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_80.asset.meta @@ -0,0 +1,8 @@ +fileFormatVersion: 2 +guid: 008ec0ae646e84841a3bcd3753ab7fa3 +NativeFormatImporter: + externalObjects: {} + mainObjectFileID: 11400000 + userData: + assetBundleName: + assetBundleVariant: diff --git a/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_81.asset b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_81.asset new file mode 100644 index 0000000000000000000000000000000000000000..33ff65d533e736bd9b10b853103ea60f9a6b3c47 --- /dev/null +++ b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_81.asset @@ -0,0 +1,36 @@ +%YAML 1.1 +%TAG !u! tag:unity3d.com,2011: +--- !u!114 &11400000 +MonoBehaviour: + m_ObjectHideFlags: 0 + m_CorrespondingSourceObject: {fileID: 0} + m_PrefabInstance: {fileID: 0} + m_PrefabAsset: {fileID: 0} + m_GameObject: {fileID: 0} + m_Enabled: 1 + m_EditorHideFlags: 0 + m_Script: {fileID: 13312, guid: 0000000000000000e000000000000000, type: 0} + m_Name: Dungeon_81 + m_EditorClassIdentifier: + m_Sprite: {fileID: -953407387, guid: 0a806eb0d25e11c48b5dee57fb9339b5, type: 3} + m_Color: {r: 1, g: 1, b: 1, a: 1} + m_Transform: + e00: 1 + e01: 0 + e02: 0 + e03: 0 + e10: 0 + e11: 1 + e12: 0 + e13: 0 + e20: 0 + e21: 0 + e22: 1 + e23: 0 + e30: 0 + e31: 0 + e32: 0 + e33: 1 + m_InstancedGameObject: {fileID: 0} + m_Flags: 1 + m_ColliderType: 1 diff --git a/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_81.asset.meta b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_81.asset.meta new file mode 100644 index 0000000000000000000000000000000000000000..a50fa48255d358fd71d2e890f4ef1afafce44513 --- /dev/null +++ b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_81.asset.meta @@ -0,0 +1,8 @@ +fileFormatVersion: 2 +guid: c3b5291c318038e4bbb6f1b39772db8a +NativeFormatImporter: + externalObjects: {} + mainObjectFileID: 11400000 + userData: + assetBundleName: + assetBundleVariant: diff --git a/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_82.asset b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_82.asset new file mode 100644 index 0000000000000000000000000000000000000000..ff7a4b604722c6ad7172c4a8cc686b179f572269 --- /dev/null +++ b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_82.asset @@ -0,0 +1,36 @@ +%YAML 1.1 +%TAG !u! tag:unity3d.com,2011: +--- !u!114 &11400000 +MonoBehaviour: + m_ObjectHideFlags: 0 + m_CorrespondingSourceObject: {fileID: 0} + m_PrefabInstance: {fileID: 0} + m_PrefabAsset: {fileID: 0} + m_GameObject: {fileID: 0} + m_Enabled: 1 + m_EditorHideFlags: 0 + m_Script: {fileID: 13312, guid: 0000000000000000e000000000000000, type: 0} + m_Name: Dungeon_82 + m_EditorClassIdentifier: + m_Sprite: {fileID: -1181825705, guid: 0a806eb0d25e11c48b5dee57fb9339b5, type: 3} + m_Color: {r: 1, g: 1, b: 1, a: 1} + m_Transform: + e00: 1 + e01: 0 + e02: 0 + e03: 0 + e10: 0 + e11: 1 + e12: 0 + e13: 0 + e20: 0 + e21: 0 + e22: 1 + e23: 0 + e30: 0 + e31: 0 + e32: 0 + e33: 1 + m_InstancedGameObject: {fileID: 0} + m_Flags: 1 + m_ColliderType: 1 diff --git a/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_82.asset.meta b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_82.asset.meta new file mode 100644 index 0000000000000000000000000000000000000000..1cc3131d0fbf8b2a10c61740015d3fe60b06637b --- /dev/null +++ b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_82.asset.meta @@ -0,0 +1,8 @@ +fileFormatVersion: 2 +guid: 8607fee69c003254086318ea700356bc +NativeFormatImporter: + externalObjects: {} + mainObjectFileID: 11400000 + userData: + assetBundleName: + assetBundleVariant: diff --git a/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_83.asset b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_83.asset new file mode 100644 index 0000000000000000000000000000000000000000..43c1c127cf0665f30a9e3e3ee27a27b652a4bdde --- /dev/null +++ b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_83.asset @@ -0,0 +1,36 @@ +%YAML 1.1 +%TAG !u! tag:unity3d.com,2011: +--- !u!114 &11400000 +MonoBehaviour: + m_ObjectHideFlags: 0 + m_CorrespondingSourceObject: {fileID: 0} + m_PrefabInstance: {fileID: 0} + m_PrefabAsset: {fileID: 0} + m_GameObject: {fileID: 0} + m_Enabled: 1 + m_EditorHideFlags: 0 + m_Script: {fileID: 13312, guid: 0000000000000000e000000000000000, type: 0} + m_Name: Dungeon_83 + m_EditorClassIdentifier: + m_Sprite: {fileID: -1892450189, guid: 0a806eb0d25e11c48b5dee57fb9339b5, type: 3} + m_Color: {r: 1, g: 1, b: 1, a: 1} + m_Transform: + e00: 1 + e01: 0 + e02: 0 + e03: 0 + e10: 0 + e11: 1 + e12: 0 + e13: 0 + e20: 0 + e21: 0 + e22: 1 + e23: 0 + e30: 0 + e31: 0 + e32: 0 + e33: 1 + m_InstancedGameObject: {fileID: 0} + m_Flags: 1 + m_ColliderType: 1 diff --git a/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_83.asset.meta b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_83.asset.meta new file mode 100644 index 0000000000000000000000000000000000000000..2e2c39f5ad1bb88d3068149f6b828ead05db5953 --- /dev/null +++ b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_83.asset.meta @@ -0,0 +1,8 @@ +fileFormatVersion: 2 +guid: 3342c11b5fc52bd4c89d97026d53d56b +NativeFormatImporter: + externalObjects: {} + mainObjectFileID: 11400000 + userData: + assetBundleName: + assetBundleVariant: diff --git a/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_84.asset b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_84.asset new file mode 100644 index 0000000000000000000000000000000000000000..8d8151899ec05ac87434ae1c35a1175ce1486093 --- /dev/null +++ b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_84.asset @@ -0,0 +1,36 @@ +%YAML 1.1 +%TAG !u! tag:unity3d.com,2011: +--- !u!114 &11400000 +MonoBehaviour: + m_ObjectHideFlags: 0 + m_CorrespondingSourceObject: {fileID: 0} + m_PrefabInstance: {fileID: 0} + m_PrefabAsset: {fileID: 0} + m_GameObject: {fileID: 0} + m_Enabled: 1 + m_EditorHideFlags: 0 + m_Script: {fileID: 13312, guid: 0000000000000000e000000000000000, type: 0} + m_Name: Dungeon_84 + m_EditorClassIdentifier: + m_Sprite: {fileID: -1649980043, guid: 0a806eb0d25e11c48b5dee57fb9339b5, type: 3} + m_Color: {r: 1, g: 1, b: 1, a: 1} + m_Transform: + e00: 1 + e01: 0 + e02: 0 + e03: 0 + e10: 0 + e11: 1 + e12: 0 + e13: 0 + e20: 0 + e21: 0 + e22: 1 + e23: 0 + e30: 0 + e31: 0 + e32: 0 + e33: 1 + m_InstancedGameObject: {fileID: 0} + m_Flags: 1 + m_ColliderType: 1 diff --git a/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_84.asset.meta b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_84.asset.meta new file mode 100644 index 0000000000000000000000000000000000000000..855d596f6df1c9caa3ac6701adc19742ccca019b --- /dev/null +++ b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_84.asset.meta @@ -0,0 +1,8 @@ +fileFormatVersion: 2 +guid: daf96d2a3dc80a244b41477f24e9c9f3 +NativeFormatImporter: + externalObjects: {} + mainObjectFileID: 11400000 + userData: + assetBundleName: + assetBundleVariant: diff --git a/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_85.asset b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_85.asset new file mode 100644 index 0000000000000000000000000000000000000000..6233239950d2b33b8159c096f6a11f78c40a8c84 --- /dev/null +++ b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_85.asset @@ -0,0 +1,36 @@ +%YAML 1.1 +%TAG !u! tag:unity3d.com,2011: +--- !u!114 &11400000 +MonoBehaviour: + m_ObjectHideFlags: 0 + m_CorrespondingSourceObject: {fileID: 0} + m_PrefabInstance: {fileID: 0} + m_PrefabAsset: {fileID: 0} + m_GameObject: {fileID: 0} + m_Enabled: 1 + m_EditorHideFlags: 0 + m_Script: {fileID: 13312, guid: 0000000000000000e000000000000000, type: 0} + m_Name: Dungeon_85 + m_EditorClassIdentifier: + m_Sprite: {fileID: 811441136, guid: 0a806eb0d25e11c48b5dee57fb9339b5, type: 3} + m_Color: {r: 1, g: 1, b: 1, a: 1} + m_Transform: + e00: 1 + e01: 0 + e02: 0 + e03: 0 + e10: 0 + e11: 1 + e12: 0 + e13: 0 + e20: 0 + e21: 0 + e22: 1 + e23: 0 + e30: 0 + e31: 0 + e32: 0 + e33: 1 + m_InstancedGameObject: {fileID: 0} + m_Flags: 1 + m_ColliderType: 1 diff --git a/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_85.asset.meta b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_85.asset.meta new file mode 100644 index 0000000000000000000000000000000000000000..72b21ecbab476f4dc288a6f7c3a401217b377227 --- /dev/null +++ b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_85.asset.meta @@ -0,0 +1,8 @@ +fileFormatVersion: 2 +guid: 1d79f2a2dd2a98142ab3dee5022b6812 +NativeFormatImporter: + externalObjects: {} + mainObjectFileID: 11400000 + userData: + assetBundleName: + assetBundleVariant: diff --git a/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_86.asset b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_86.asset new file mode 100644 index 0000000000000000000000000000000000000000..38878703e8e0bfdde69eb1654d613ba61098baa7 --- /dev/null +++ b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_86.asset @@ -0,0 +1,36 @@ +%YAML 1.1 +%TAG !u! tag:unity3d.com,2011: +--- !u!114 &11400000 +MonoBehaviour: + m_ObjectHideFlags: 0 + m_CorrespondingSourceObject: {fileID: 0} + m_PrefabInstance: {fileID: 0} + m_PrefabAsset: {fileID: 0} + m_GameObject: {fileID: 0} + m_Enabled: 1 + m_EditorHideFlags: 0 + m_Script: {fileID: 13312, guid: 0000000000000000e000000000000000, type: 0} + m_Name: Dungeon_86 + m_EditorClassIdentifier: + m_Sprite: {fileID: 207130727, guid: 0a806eb0d25e11c48b5dee57fb9339b5, type: 3} + m_Color: {r: 1, g: 1, b: 1, a: 1} + m_Transform: + e00: 1 + e01: 0 + e02: 0 + e03: 0 + e10: 0 + e11: 1 + e12: 0 + e13: 0 + e20: 0 + e21: 0 + e22: 1 + e23: 0 + e30: 0 + e31: 0 + e32: 0 + e33: 1 + m_InstancedGameObject: {fileID: 0} + m_Flags: 1 + m_ColliderType: 1 diff --git a/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_86.asset.meta b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_86.asset.meta new file mode 100644 index 0000000000000000000000000000000000000000..e02c7fada814b62ea252aee1dcd73f25f8e13dca --- /dev/null +++ b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_86.asset.meta @@ -0,0 +1,8 @@ +fileFormatVersion: 2 +guid: d4ff608324b75a24693fb931623e9d02 +NativeFormatImporter: + externalObjects: {} + mainObjectFileID: 11400000 + userData: + assetBundleName: + assetBundleVariant: diff --git a/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_87.asset b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_87.asset new file mode 100644 index 0000000000000000000000000000000000000000..c7e39c9305a3fa0d5721762c2c8578b21d8fb971 --- /dev/null +++ b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_87.asset @@ -0,0 +1,36 @@ +%YAML 1.1 +%TAG !u! tag:unity3d.com,2011: +--- !u!114 &11400000 +MonoBehaviour: + m_ObjectHideFlags: 0 + m_CorrespondingSourceObject: {fileID: 0} + m_PrefabInstance: {fileID: 0} + m_PrefabAsset: {fileID: 0} + m_GameObject: {fileID: 0} + m_Enabled: 1 + m_EditorHideFlags: 0 + m_Script: {fileID: 13312, guid: 0000000000000000e000000000000000, type: 0} + m_Name: Dungeon_87 + m_EditorClassIdentifier: + m_Sprite: {fileID: -857797333, guid: 0a806eb0d25e11c48b5dee57fb9339b5, type: 3} + m_Color: {r: 1, g: 1, b: 1, a: 1} + m_Transform: + e00: 1 + e01: 0 + e02: 0 + e03: 0 + e10: 0 + e11: 1 + e12: 0 + e13: 0 + e20: 0 + e21: 0 + e22: 1 + e23: 0 + e30: 0 + e31: 0 + e32: 0 + e33: 1 + m_InstancedGameObject: {fileID: 0} + m_Flags: 1 + m_ColliderType: 1 diff --git a/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_87.asset.meta b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_87.asset.meta new file mode 100644 index 0000000000000000000000000000000000000000..9ec9060142b38f84b8350dfe2e8ce9231111151b --- /dev/null +++ b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_87.asset.meta @@ -0,0 +1,8 @@ +fileFormatVersion: 2 +guid: 731304ae8a725e34a8c382e0b0dd3467 +NativeFormatImporter: + externalObjects: {} + mainObjectFileID: 11400000 + userData: + assetBundleName: + assetBundleVariant: diff --git a/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_88.asset b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_88.asset new file mode 100644 index 0000000000000000000000000000000000000000..435d106107da652b68a8b7b9d39aa89fd0f6da89 --- /dev/null +++ b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_88.asset @@ -0,0 +1,36 @@ +%YAML 1.1 +%TAG !u! tag:unity3d.com,2011: +--- !u!114 &11400000 +MonoBehaviour: + m_ObjectHideFlags: 0 + m_CorrespondingSourceObject: {fileID: 0} + m_PrefabInstance: {fileID: 0} + m_PrefabAsset: {fileID: 0} + m_GameObject: {fileID: 0} + m_Enabled: 1 + m_EditorHideFlags: 0 + m_Script: {fileID: 13312, guid: 0000000000000000e000000000000000, type: 0} + m_Name: Dungeon_88 + m_EditorClassIdentifier: + m_Sprite: {fileID: 306057277, guid: 0a806eb0d25e11c48b5dee57fb9339b5, type: 3} + m_Color: {r: 1, g: 1, b: 1, a: 1} + m_Transform: + e00: 1 + e01: 0 + e02: 0 + e03: 0 + e10: 0 + e11: 1 + e12: 0 + e13: 0 + e20: 0 + e21: 0 + e22: 1 + e23: 0 + e30: 0 + e31: 0 + e32: 0 + e33: 1 + m_InstancedGameObject: {fileID: 0} + m_Flags: 1 + m_ColliderType: 1 diff --git a/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_88.asset.meta b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_88.asset.meta new file mode 100644 index 0000000000000000000000000000000000000000..db865d4a2f58464f5615b3b2b0352872afec3968 --- /dev/null +++ b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_88.asset.meta @@ -0,0 +1,8 @@ +fileFormatVersion: 2 +guid: 794cb64b1bb867c438f45faf4c809e60 +NativeFormatImporter: + externalObjects: {} + mainObjectFileID: 11400000 + userData: + assetBundleName: + assetBundleVariant: diff --git a/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_89.asset b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_89.asset new file mode 100644 index 0000000000000000000000000000000000000000..7daaf36d366289bd323cd72450f84df355efa024 --- /dev/null +++ b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_89.asset @@ -0,0 +1,36 @@ +%YAML 1.1 +%TAG !u! tag:unity3d.com,2011: +--- !u!114 &11400000 +MonoBehaviour: + m_ObjectHideFlags: 0 + m_CorrespondingSourceObject: {fileID: 0} + m_PrefabInstance: {fileID: 0} + m_PrefabAsset: {fileID: 0} + m_GameObject: {fileID: 0} + m_Enabled: 1 + m_EditorHideFlags: 0 + m_Script: {fileID: 13312, guid: 0000000000000000e000000000000000, type: 0} + m_Name: Dungeon_89 + m_EditorClassIdentifier: + m_Sprite: {fileID: -1558256172, guid: 0a806eb0d25e11c48b5dee57fb9339b5, type: 3} + m_Color: {r: 1, g: 1, b: 1, a: 1} + m_Transform: + e00: 1 + e01: 0 + e02: 0 + e03: 0 + e10: 0 + e11: 1 + e12: 0 + e13: 0 + e20: 0 + e21: 0 + e22: 1 + e23: 0 + e30: 0 + e31: 0 + e32: 0 + e33: 1 + m_InstancedGameObject: {fileID: 0} + m_Flags: 1 + m_ColliderType: 1 diff --git a/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_89.asset.meta b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_89.asset.meta new file mode 100644 index 0000000000000000000000000000000000000000..f4133615487c1727372e31ee5ce06a4c19442c34 --- /dev/null +++ b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_89.asset.meta @@ -0,0 +1,8 @@ +fileFormatVersion: 2 +guid: 6242f92cd02e8a84093a2c3aaa0141f8 +NativeFormatImporter: + externalObjects: {} + mainObjectFileID: 11400000 + userData: + assetBundleName: + assetBundleVariant: diff --git a/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_9.asset b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_9.asset new file mode 100644 index 0000000000000000000000000000000000000000..a64c03e3d0c32dbb4fb8a8a187357836e076a2bc --- /dev/null +++ b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_9.asset @@ -0,0 +1,36 @@ +%YAML 1.1 +%TAG !u! tag:unity3d.com,2011: +--- !u!114 &11400000 +MonoBehaviour: + m_ObjectHideFlags: 0 + m_CorrespondingSourceObject: {fileID: 0} + m_PrefabInstance: {fileID: 0} + m_PrefabAsset: {fileID: 0} + m_GameObject: {fileID: 0} + m_Enabled: 1 + m_EditorHideFlags: 0 + m_Script: {fileID: 13312, guid: 0000000000000000e000000000000000, type: 0} + m_Name: Dungeon_9 + m_EditorClassIdentifier: + m_Sprite: {fileID: 136035454, guid: 0a806eb0d25e11c48b5dee57fb9339b5, type: 3} + m_Color: {r: 1, g: 1, b: 1, a: 1} + m_Transform: + e00: 1 + e01: 0 + e02: 0 + e03: 0 + e10: 0 + e11: 1 + e12: 0 + e13: 0 + e20: 0 + e21: 0 + e22: 1 + e23: 0 + e30: 0 + e31: 0 + e32: 0 + e33: 1 + m_InstancedGameObject: {fileID: 0} + m_Flags: 1 + m_ColliderType: 1 diff --git a/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_9.asset.meta b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_9.asset.meta new file mode 100644 index 0000000000000000000000000000000000000000..38711fbc8f867bb733e30e8e2340d8524c0b55f7 --- /dev/null +++ b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_9.asset.meta @@ -0,0 +1,8 @@ +fileFormatVersion: 2 +guid: 146696a0f0461d9439de4b790c6e083b +NativeFormatImporter: + externalObjects: {} + mainObjectFileID: 11400000 + userData: + assetBundleName: + assetBundleVariant: diff --git a/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_90.asset b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_90.asset new file mode 100644 index 0000000000000000000000000000000000000000..8b760798de39d37ea835577b8dfe086f7f288a42 --- /dev/null +++ b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_90.asset @@ -0,0 +1,36 @@ +%YAML 1.1 +%TAG !u! tag:unity3d.com,2011: +--- !u!114 &11400000 +MonoBehaviour: + m_ObjectHideFlags: 0 + m_CorrespondingSourceObject: {fileID: 0} + m_PrefabInstance: {fileID: 0} + m_PrefabAsset: {fileID: 0} + m_GameObject: {fileID: 0} + m_Enabled: 1 + m_EditorHideFlags: 0 + m_Script: {fileID: 13312, guid: 0000000000000000e000000000000000, type: 0} + m_Name: Dungeon_90 + m_EditorClassIdentifier: + m_Sprite: {fileID: 1466099207, guid: 0a806eb0d25e11c48b5dee57fb9339b5, type: 3} + m_Color: {r: 1, g: 1, b: 1, a: 1} + m_Transform: + e00: 1 + e01: 0 + e02: 0 + e03: 0 + e10: 0 + e11: 1 + e12: 0 + e13: 0 + e20: 0 + e21: 0 + e22: 1 + e23: 0 + e30: 0 + e31: 0 + e32: 0 + e33: 1 + m_InstancedGameObject: {fileID: 0} + m_Flags: 1 + m_ColliderType: 1 diff --git a/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_90.asset.meta b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_90.asset.meta new file mode 100644 index 0000000000000000000000000000000000000000..97d9e88b5979d1bab8418c396b2f148988e5b3d3 --- /dev/null +++ b/MrBigsock/Assets/Tilemaps/Dungeon/Dungeon_90.asset.meta @@ -0,0 +1,8 @@ +fileFormatVersion: 2 +guid: 80e70deb48d53a241be6ed8f133a67db +NativeFormatImporter: + externalObjects: {} + mainObjectFileID: 11400000 + userData: + assetBundleName: + assetBundleVariant: diff --git a/MrBigsock/Packages/com.unity.2d.tilemap.extras/.npmignore b/MrBigsock/Packages/com.unity.2d.tilemap.extras/.npmignore new file mode 100644 index 0000000000000000000000000000000000000000..9bb8a065fd39b9645045e45628e5ce5df7116104 --- /dev/null +++ b/MrBigsock/Packages/com.unity.2d.tilemap.extras/.npmignore @@ -0,0 +1,11 @@ +artifacts/** +build/** +.DS_Store +.npmrc +.npmignore +.gitignore +QAReport.md +QAReport.md.meta +.gitlab-ci.yml +build.bat +build.sh \ No newline at end of file diff --git a/MrBigsock/Packages/com.unity.2d.tilemap.extras/CHANGELOG.md b/MrBigsock/Packages/com.unity.2d.tilemap.extras/CHANGELOG.md new file mode 100644 index 0000000000000000000000000000000000000000..1ce27e905841b739940ca223bafa8a9649539fcb --- /dev/null +++ b/MrBigsock/Packages/com.unity.2d.tilemap.extras/CHANGELOG.md @@ -0,0 +1,190 @@ +# Changelog +All notable changes to this package will be documented in this file. + +The format is based on [Keep a Changelog](http://keepachangelog.com/en/1.0.0/) + +## [2.2.3] - 2022-04-01 +### Fixed +- [RuleOverrideTile] -Mark RuleOverrideTile InstanceTile as dirty when overriding RuleTile for the RuleOverrideTile changes +- [RuleOverrideTile] -Fix undo for RuleOverrideTile when overriding RuleTile has changed +- [RuleTileEditor] -Fix height for ReorderableList when extending the view for marking Rules + +## [2.2.2] - 2022-03-03 +### Fixed +- [AnimatedTileEditor] -Fix undo when setting number of Sprites for Animated Tile +- [RuleTile] -Fix data for custom container fields not being transferred in RuleOverrideTiles overriding a Custom Rule Tile +- [RuleTileEditor] -Fix undo when setting number of Rules for Rule Tile +- [RuleTileEditor] -Use different text color for Extend Neighbors with dark and light skin + +## [2.2.1] - 2021-08-24 +### Changed +- [GameObjectBrush] Add canChangePosition +- [GameObjectBrush] Use GridLayout from BrushTarget if it has one +- [HexagonalRuleTile] Fix GetOffsetPositionReverse +- [RuleOverrideTile] Create instance Tile on override +- [RuleTile] Add scripting documentation +- [RuleTileEditor] Add drag and drop rect for Sprites to create initial TilingRules +- [RuleTileEditor] Add field to change number of TilingRules +- [RuleTileEditor] Add blank space to the end of the Rule list +- [RuleTileEditor] Add undo for changes +- [AnimatedTileEditor] Add undo for changes +- [TintBrush] Convert cell positions to world positions based on the Grid used +- [TintBrush] Add k_ScaleFactor for better precision when painting on non-rectangular Tilemaps + +### Fixed +- [RuleTile] Fixed error in RuleTileEditor when removing all Rules and adding a new Rule + +## [2.2.0] - 2021-06-01 +- [RuleTileEditor] Add tooltips to fields +- Add required package dependencies + +## [2.1.0] - 2021-05-06 +### Changed +- [RuleTile] Improve performance of RuleTile caching +- [RuleTileEditor] Allow non-public fields with the SerializeField attribute as custom fields for RuleTile +- Make U2DExtrasPlaceholder internal + +### Fixed +- [RuleTileEditor] Fix exception when adding a new Rule when no Rule is selected + +## [2.0.0] - 2021-03-17 +- Update version to 2.0.0 + +## [2.0.0-pre.3] - 2021-02-19 +- [HexagonalRuleTile] Fix issue with mirror rule +- [RuleTile] Add min and max animation speedup +- [RuleOverrideTile] Fix import issue when upgrading from a previous version of RuleOverrideTile +- [RuleTileEditor] Add new rule below selected rule in RuleTileEditor +- [RuleTileEditor] Add dropdown to duplicate Rule + +## [2.0.0-pre.2] - 2020-11-26 +### Changed +- Update documentation +- Add contribution notice in README.md +- Update Third Party Notices.md +- [PrefabBush] Add pick +- [PrefabBush] Add tooltip for "Erase Any Objects" field +- [PrefabBrush][GameObjectBrush] Account for Anchor when using GetObjectsInCell in PrefabBrush and GameObjectBrush +- [CustomRuleTileScript] Allow Custom Rule Tile template script to be created regardless of where template script is installed (from a package or in the project) + +## [2.0.0-pre.1] - 2020-10-14 +- Update version to 2.0.0-pre.1 + +## [1.6.2-preview] - 2020-09-25 +### Changed +- [RuleTile/RuleOverrideTile/AdvancedRuleOverrideTile] Renamed Game Object to GameObject +- [RuleTile] Fix menu order for RuleOverrideTile +- [RuleOverrideTile] Fix menu order for RuleOverrideTile +- [AdvancedRuleOverrideTile] Fix Rule toggle for AdvancedRuleOverrideTile +- [GameObjectBrush] Use correct position when ClearSceneCell +- [GameObjectBrush] Update cells when size changes +- [GameObjectBrush] Clear cell for Prefabs +- [LineBrush] Clear previews from base.OnPaintSceneGUI +- [PrefabBrush] Fix box erase + +## [1.6.1-preview] - 2020-08-11 +### Changed +- Update samples + +## [1.6.0-preview] - 2020-05-27 +### Changed +- Updated for Unity 2020.1 +- [GameObjectBrush] Allow painting, erasing and picking on Tile Palette +- [GameObjectBrush] Add Paint on Scene view to GameObjectBrush +- [PrefabBush] Add BoxFill to PrefabBrush +- [PrefabBush] Add Rotation to PrefabBrush +- Consolidated menu items + +## [1.5.0-preview] - 2020-02-14 +### Added +- Added CONTRIBUTING.md +- Updated LICENSE.md + +### Added +- [PrefabRandomBrush] Split functionality of PrefabBrush to PrefabBrush and PrefabRandomBrush +- [PrefabBrush/PrefabRandomBrush] Add Erase Any Objects toggle to choose between erasing any Objects or Objects in the Brush + +### Changed +- Consolidated menu items + +### Fixed +- [WeightedRandomTile] Fixed WeightedRandomTile messing up Random.seed! + +## [1.4.0] - 2020-01-07 +### Added +- [RuleTile / HexagonalRuleTile / IsometricRuleTile / RuleOverrideTile] Added Asset Preview for TilingRules +- [RuleTile] Hidden Rule field +- [CustomRuleTile] Support custom field of Object type +- [CustomRuleTile] Support HideInInspector, DontOverride attributes +- [RuleOverrideTile] Move advanced mode to AdvancedRuleOverrideTile +- [RuleOverrideTile] Add GameObject overrides +- [RuleOverrideTile] List height lessen +- [RuleOverrideTile] Don't override null sprite +- [RuleOverrideTile] Add static preview +- [AdvancedRuleOverrideTile] List GUI simplify +- [RuleOverrideTile / AdvancedRuleOverrideTile] Show unused overrides +- [RuleOverrideTile / AdvancedRuleOverrideTile] Support multiple inheritance +- [RuleOverrideTile / AdvancedRuleOverrideTile] Prevent circular reference +- [AnimatedTile] Added Animation Start Frame which helps to calculate the Animation Start Time for a given Tilemap + +### Fixed +- [RuleTile] Fixed RuleTile InstantiatedGameObject rotation/scale +- [RuleTile] Fixed override tiles have not update when default properties changed +- [AdvancedRuleOverrideTile] Fix override rule lost reference when source rule reorder +- [PrefabBrush] Use WorldToCell comparison when getting GameObjects using PrefabBrush + +## [1.3.1] - 2019-11-06 +### Changed +- [RuleTile] Simplified +- [RuleTile] Caching all RuleTile neighbor positions for Tilemap to speedup refresh affected tiles + +### Fixed +- [RuleTile] Fix remote positions missing of MirrorXY (#148) +- [HexagonalRuleTile] Fix ApplyRandomTransform() of HexagonalRuleTile missing MirrorXY case +- [RuleOverrideTile] Fix RuleOverrideTile does not refresh when add/remove rule +- [RuleTile] Fix random rotation calculation mistake +- [RuleTile] Fix cache data will not update when rule change + +## [1.3.0] - 2019-11-01 +### Changed +- [RuleTile] changed from using index to using position. +- [RuleTile] Additional storage rule position. +- [RuleTile] Delete DontCare rule. +- [RuleTile] Rule list increased Extend Neighbor toggle. When selected, it will increase the rule range that can be set. +- [RuleTile] No longer fixed to checking around 8 rules. +- [RuleTile] RefreshTile() will refresh affected remote Tiles. +- [RuleTile] Delete GetMatchingNeighboringTiles(), no longer get nearby Tiles in advance, the performance is affected. (may be changed to cache later) +- [IsometricRuleTile] Rewrite. +- [HexagonalRuleTile] Rewrite. +- [LineBrush] Fix for Tiles disappear after selection and drag with LineBrush +- [RuleTile] Add MirrorXY Transform Rule + +## [1.2.0] - 2019-10-17 +### Changed +- [PrefabBrush] Erase GameObjects at target position before painting +- [RuleTileEditor] Made RuleTileEditor and children public +- [RuleTile] Roll back m_Self to this. +- [RuleOverrideTile] Remove m_OverrideSelf property. +- [RuleOverrideTile] Inherit custom properties from custom RuleTile. +- [RuleOverrideTile] Change m_RuntimeTile to m_InstanceTile. + +## [1.1.0] - 2019-08-23 +### Changed +- Validate Gap and Limit for GroupBrush +- Fix z iterator for RandomBrush +- Check randomTileSets on addToRandomTiles +- Add Anchor to GameObjectBrush and PrefabBrush + +## [1.1.0] - 2019-03-22 +### Changed +- Copy GameObject when copying TilingRule in RuleOverrideTile + +## [1.1.0] - 2019-03-08 +### Added +- Added com.unity.2d.tilemap as a dependency of com.unity.2d.tilemap.extras + +### Changed +- Custom Grid Brushes have been updated to the UnityEditor.Tilemaps namespace + +## [1.0.0] - 2019-01-02 +### This is the first release of Tilemap Extras, as a Package diff --git a/MrBigsock/Packages/com.unity.2d.tilemap.extras/CHANGELOG.md.meta b/MrBigsock/Packages/com.unity.2d.tilemap.extras/CHANGELOG.md.meta new file mode 100644 index 0000000000000000000000000000000000000000..155d50baa84d0694c70266a93f8b63cde7ff86a5 --- /dev/null +++ b/MrBigsock/Packages/com.unity.2d.tilemap.extras/CHANGELOG.md.meta @@ -0,0 +1,7 @@ +fileFormatVersion: 2 +guid: e2b8acf174765f141a47de79f2db4b26 +TextScriptImporter: + externalObjects: {} + userData: + assetBundleName: + assetBundleVariant: diff --git a/MrBigsock/Packages/com.unity.2d.tilemap.extras/CONTRIBUTING.md b/MrBigsock/Packages/com.unity.2d.tilemap.extras/CONTRIBUTING.md new file mode 100644 index 0000000000000000000000000000000000000000..0c3a58b8f9c6141b4559272ef50135cdabdeac6b --- /dev/null +++ b/MrBigsock/Packages/com.unity.2d.tilemap.extras/CONTRIBUTING.md @@ -0,0 +1,6 @@ +# Contributing + +## All contributions are subject to the [Unity Contribution Agreement(UCA)](https://unity3d.com/legal/licenses/Unity_Contribution_Agreement) +By making a pull request, you are confirming agreement to the terms and conditions of the UCA, including that your Contributions are your original creation and that you have complete right and authority to make your Contributions. + +## Once you have a change ready following these ground rules. Simply make a pull request! \ No newline at end of file diff --git a/MrBigsock/Packages/com.unity.2d.tilemap.extras/CONTRIBUTING.md.meta b/MrBigsock/Packages/com.unity.2d.tilemap.extras/CONTRIBUTING.md.meta new file mode 100644 index 0000000000000000000000000000000000000000..ee2ec1b74902d70cb1f16a4cbcdcc0892fddab82 --- /dev/null +++ b/MrBigsock/Packages/com.unity.2d.tilemap.extras/CONTRIBUTING.md.meta @@ -0,0 +1,7 @@ +fileFormatVersion: 2 +guid: 6227377cf86ed2341ba50b1d9889f2f7 +TextScriptImporter: + externalObjects: {} + userData: + assetBundleName: + assetBundleVariant: diff --git a/MrBigsock/Packages/com.unity.2d.tilemap.extras/Documentation~/AdvancedRuleOverrideTile.md b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Documentation~/AdvancedRuleOverrideTile.md new file mode 100644 index 0000000000000000000000000000000000000000..915633afe984a7315c7b280a815a36da7285ac46 --- /dev/null +++ b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Documentation~/AdvancedRuleOverrideTile.md @@ -0,0 +1,25 @@ +# Advanced Rule Override Tile + +__Contributions by:__ [johnsoncodehk](https://github.com/johnsoncodehk), [Autofire](https://github.com/Autofire) + +__Advanced Rule Override Tiles__ are Tiles which can override a subset of Rules for a given [Rule Tile](RuleTile.md) while maintaining most of the other set Rules of the Rule Tile. This allows you to create Tiles that provide specialized behavior in specific scenarios. + +## Properties + +| Property | Function | +| ----------------- | ------------------------------------------------------------ | +| __Tile__ | The Rule Tile to override. | + +Depending on the Rule Tile that is overridden, there may be further properties which you can override here. Any public property in the Rule Tile that does not have a `RuleTile.DontOverride` attribute will be shown here and can be overridden. + +## Usage + +First select the Rule Tile to be overridden in the __Tile__ property. The Rule Override Tile editor then displays the different rules in the selected Rule Tile which you can override. + +Select the Rule which you want to override by toggling the Rule. This will allow you to modify the output of the Rule, such as the Sprite, the GameObject or the Collider Type. The outputs are the same as the original [Rule Tile](RuleTile.md) and are detailed there. The matching Rule itself cannot be changed here and is displayed here to help identify it. + + + +Paint with the Advanced Rule Override Tile using the [Tile Palette](https://docs.unity3d.com/Manual/Tilemap-Painting.html) tools. + + \ No newline at end of file diff --git a/MrBigsock/Packages/com.unity.2d.tilemap.extras/Documentation~/AnimatedTile.md b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Documentation~/AnimatedTile.md new file mode 100644 index 0000000000000000000000000000000000000000..ae3aa2a013d8bac4d9ff55f7b5b9b9a3d69a1920 --- /dev/null +++ b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Documentation~/AnimatedTile.md @@ -0,0 +1,25 @@ +# Animated Tile + +__Contribution by:__ MahdiMahzuni + +An Animated Tile runs through and displays a list of Sprites in sequence to create a frame-by-frame animation. + +<br/>Animated Tile editor window + +### Properties + +| Property | Function | +| ------------------------------ | ------------------------------------------------------------ | +| __Number of Animated Sprites__ | Number of Animated Sprites in the Animated Tile. | +| __Sprite list__ | The list displaying the current order of Sprites for this Animated Tile’s animation which plays in sequence. Set a Sprite by selecting the **Select** button at the bottom right of the Sprite preview, then choosing the Sprite from the dialog box. Select and hold the **=** next to each Sprite to reorder their place in the animation sequence. | +| __Minimum Speed__ | The minimum possible speed at which the Animation of the Tile is played. A speed value will be randomly chosen between the minimum and maximum speed. | +| __Maximum Speed__ | The maximum possible speed at which the Animation of the Tile is played. A speed value will be randomly chosen between the minimum and maximum speed. | +| __Start Time__ | The starting time of this Animated Tile. This allows you to start the Animation from a particular time. | +| __Start Frame__ | The starting frame of this Animated Tile. This allows you to start the Animation from a particular Sprite in the list of Animated Sprites. | +| __Collider Type__ | The [Collider](https://docs.unity3d.com/Manual/Collider2D.html) shape generated by the Tile. | + +### Usage + +Create the Animated Tile by selecting and ordering the Sprites that makes up its animation sequence in the Animated Tile editor, then paint the Animated Tile with the [Tile Palette tools](https://docs.unity3d.com/Manual/Tilemap-Painting.html). + +<br/>Game view, painted with the [Group Brush](GroupBrush.md). \ No newline at end of file diff --git a/MrBigsock/Packages/com.unity.2d.tilemap.extras/Documentation~/Brushes.md b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Documentation~/Brushes.md new file mode 100644 index 0000000000000000000000000000000000000000..1f7241c38f76dddd2a27eee34c4c6c9f1db9a809 --- /dev/null +++ b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Documentation~/Brushes.md @@ -0,0 +1,13 @@ +# Scriptable Brushes + +You can script Brushes to paint items based on the position and conditions of the cell it targets on the Grid Layout. Brush paint behavior can be further modified by the selected editing Tool, such as __Erase__ or __Floodfill__. + +Here are some implementations of **Scriptable Brushes** which can help save time when designing your Tilemap: + +- [GameObject Brush](GameObjectBrush.md) +- [Random Brush](RandomBrush.md) +- [Line Brush](LineBrush.md) +- [Group Brush](GroupBrush.md) + +Refer to the [Scriptable Brushes](https://docs.unity3d.com/Manual/Tilemap-ScriptableBrushes.html) documentation for more information. + diff --git a/MrBigsock/Packages/com.unity.2d.tilemap.extras/Documentation~/Contributors.md b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Documentation~/Contributors.md new file mode 100644 index 0000000000000000000000000000000000000000..a97c234efda2a758784de7f29ff80f9fb505b044 --- /dev/null +++ b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Documentation~/Contributors.md @@ -0,0 +1,29 @@ +# Contributors + +Thank you to all who have contributed to this repository! + +- [johnsoncodehk](https://github.com/johnsoncodehk) +- [nicovain](https://github.com/nicovain) +- [superkerokero](https://github.com/superkerokero) +- [pmurph0305](https://github.com/pmurph0305) +- [janissimsons](https://github.com/janissimsons) +- [distantcam](https://github.com/distantcam) +- [Pepperized](https://github.com/Pepperized) +- MahdiMahzuni +- [DreadBoy](https://github.com/DreadBoy) +- [DoctorShinobi](https://github.com/DoctorShinobi) +- [CraigGraff](https://github.com/CraigGraff) +- [Autofire](https://github.com/Autofire) +- [AVChemodanov](https://github.com/AVChemodanov) +- [ream88](https://github.com/ream88) +- [Quickz](https://github.com/Quickz) +- [capnslipp](https://github.com/capnslipp) +- [TrentSterling](https://github.com/TrentSterling) +- [vladderb](https://github.com/vladderb) +- [trobol](https://github.com/trobol) +- [HyagoOliveira](https://github.com/HyagoOliveira) +- [RyotaMurohoshi](https://github.com/RyotaMurohoshi) +- [ManickYoj](https://github.com/ManickYoj) +- [n4n0lix](https://githubb.com/n4n0lix) + +If anybody has been missed, please do let us know! \ No newline at end of file diff --git a/MrBigsock/Packages/com.unity.2d.tilemap.extras/Documentation~/CoordinateBrush.md b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Documentation~/CoordinateBrush.md new file mode 100644 index 0000000000000000000000000000000000000000..62868025fa0e006ad91c2278dbd85aa766d7f1ad --- /dev/null +++ b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Documentation~/CoordinateBrush.md @@ -0,0 +1,8 @@ +# Coordinate Brush + +__Contributions by :__ [nicovain](https://github.com/nicovain), [pmurph0305](https://github.com/pmurph0305) + +This Brush displays the coordinates of the it is currently targeting in the Scene view. Use this Brush as an example for creating custom Brushes that display extra visual features, such as cell coordinates or other information, while painting a Tilemap with the Brush. + +<br/>When painting with the Coordinate Brush in the Scene view, the position of the currently highlighted cell is displayed. + diff --git a/MrBigsock/Packages/com.unity.2d.tilemap.extras/Documentation~/CustomRulesForRuleTile.md b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Documentation~/CustomRulesForRuleTile.md new file mode 100644 index 0000000000000000000000000000000000000000..401edbfb43704b295a242b491fea86cb2680ed45 --- /dev/null +++ b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Documentation~/CustomRulesForRuleTile.md @@ -0,0 +1,102 @@ +# Custom Rules for Rule Tile + +__Contribution by:__ [johnsoncodehk](https://github.com/johnsoncodehk) + +Use this template script to create new custom [Rule Tiles](RuleTile.md) with matching options that differ from the Rule Tile’s [default options](RuleTile.md#Usage) (namely **This** and **Not This**). This creates selectable options for each Rule in your custom __Rule Tile__. + +## Template features + +- Inheritable Rule Tile. +- Customizable properties. +- Expand or rewrite both neighbor Rules and the GUI display of the Rules. +- Usable with by [RuleOverrideTile](RuleOverrideTile.md) +- Create from a template script. +- Neighbor Rules tooltips. +- Backward compatible. + +## Creating a custom Rule Tile script + +Create a Custom Rule Tile script by going to __Assets > Create > Custom Rule Tile Script__. Name the newly created file when prompted. After creating the file, you can edit it to add new matching options and custom algorithms for testing matches. + +### Examples + +- Custom properties: + +```csharp +public class MyTile : RuleTile { + public string tileId; + public bool isWater; +} +``` + +- Custom rules: + +```csharp +public class MyTile : RuleTile<MyTile.Neighbor> { + public class Neighbor { + public const int MyRule1 = 0; + public const int MyRule2 = 1; + } + public override bool RuleMatch(int neighbor, TileBase tile) { + switch (neighbor) { + case Neighbor.MyRule1: return false; + case Neighbor.MyRule2: return true; + } + return true; + } +} +``` + +- Expansion rules + +```csharp +public class MyTile : RuleTile<MyTile.Neighbor> { + public class Neighbor : RuleTile.TilingRule.Neighbor { + // 0, 1, 2 is using in RuleTile.TilingRule.Neighbor + public const int MyRule1 = 3; + public const int MyRule2 = 4; + } + public override bool RuleMatch(int neighbor, TileBase tile) { + switch (neighbor) { + case Neighbor.MyRule1: return false; + case Neighbor.MyRule2: return true; + } + return base.RuleMatch(neighbor, tile); + } +} +``` + +- Siblings Tile 1 + +```csharp +public class MyTile : RuleTile<MyTile.Neighbor> { + public List<TileBase> sibings = new List<TileBase>(); + public class Neighbor : RuleTile.TilingRule.Neighbor { + public const int Sibing = 3; + } + public override bool RuleMatch(int neighbor, TileBase tile) { + switch (neighbor) { + case Neighbor.Sibing: return sibings.Contains(tile); + } + return base.RuleMatch(neighbor, tile); + } +} +``` + +- Siblings Tile 2 + +```csharp +public class MyTile : RuleTile<MyTile.Neighbor> { + public int siblingGroup; + public class Neighbor : RuleTile.TilingRule.Neighbor { + public const int Sibing = 3; + } + public override bool RuleMatch(int neighbor, TileBase tile) { + MyTile myTile = tile as MyTile; + switch (neighbor) { + case Neighbor.Sibing: return myTile && myTile.siblingGroup == siblingGroup; + } + return base.RuleMatch(neighbor, tile); + } +} +``` \ No newline at end of file diff --git a/MrBigsock/Packages/com.unity.2d.tilemap.extras/Documentation~/GameObjectBrush.md b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Documentation~/GameObjectBrush.md new file mode 100644 index 0000000000000000000000000000000000000000..2edc9c8ba2731d91e4c0ce5f5be25789018a6334 --- /dev/null +++ b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Documentation~/GameObjectBrush.md @@ -0,0 +1,21 @@ +# GameObject Brush + +This Brush instances, places and manipulates GameObjects onto the Scene. Use this Brush as an example for creating custom Brushes which can target and manipulate other GameObjects beside Tiles. + +## Usage + +First select the GameObject Brush from the Brush drop-down menu. With the Brush selected, then select the [Picker Tool](https://docs.unity3d.com/Manual/Tilemap-Painting.html#Picker) from the [Tile Palette](https://docs.unity3d.com/Manual/Tilemap-Painting.html) toolbar. Use the [Select Tool](https://docs.unity3d.com/Manual/Tilemap-Painting.html#Select) to select GameObjects from the Scene that you want the GameObject Brush to paint with. Note that these GameObjects must be a child of the active Grid to be selectable with this Brush. + +When painting with the GameObject Brush, the Brush will instantiate GameObjects picked onto the Scene. + + + +## Implementation + +The GameObjectBrush inherits from the GridBrush and overrides several methods when implemented. The following methods are overridden: + +- It overrides the `Paint` method to paint a GameObject. +- It overrides the `Erase` method to erase the GameObjects from the Scene. +- It overrides the `BoxFill` method to paint a GameObject in each cell defined by the [Box Tool](https://docs.unity3d.com/Manual/Tilemap-Painting.html#Rec). +- It overrides the `Move` methods to move GameObjects in the Scene. +- It overrides the `Flip` methods to flip GameObjects in the picked selection. \ No newline at end of file diff --git a/MrBigsock/Packages/com.unity.2d.tilemap.extras/Documentation~/GridInformation.md b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Documentation~/GridInformation.md new file mode 100644 index 0000000000000000000000000000000000000000..5571eba3bf493de99eecee9e02acc8de27bc6533 --- /dev/null +++ b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Documentation~/GridInformation.md @@ -0,0 +1,41 @@ +# Grid Information + +This is a simple component that stores and provides information based on Grid positions and keywords. + +## Usage + +Add this Component to a GameObject with a Grid component. To store information on to the Grid Information component, use the following APIs: + +```C# +public bool SetPositionProperty(Vector3Int position, String name, int positionProperty) + +public bool SetPositionProperty(Vector3Int position, String name, string positionProperty) + +public bool SetPositionProperty(Vector3Int position, String name, float positionProperty) + +public bool SetPositionProperty(Vector3Int position, String name, double positionProperty) + +public bool SetPositionProperty(Vector3Int position, String name, UnityEngine.Object positionProperty) + +public bool SetPositionProperty(Vector3Int position, String name, Color positionProperty) +``` + + + +To retrieve information from the Grid Information component, use the following APIs: + +```C# +public T GetPositionProperty<T>(Vector3Int position, String name, T defaultValue) where T : UnityEngine.Object + +public int GetPositionProperty(Vector3Int position, String name, int defaultValue) + +public string GetPositionProperty(Vector3Int position, String name, string defaultValue) + +public float GetPositionProperty(Vector3Int position, String name, float defaultValue) + +public double GetPositionProperty(Vector3Int position, String name, double defaultValue) + +public Color GetPositionProperty(Vector3Int position, String name, Color defaultValue) +``` + +You can use this in combination with [Scriptable Tiles](https://docs.unity3d.com/Manual/Tilemap-ScriptableTiles.html) to get the right [TileData](https://docs.unity3d.com/Manual/Tilemap-ScriptableTiles-TileData.html) when creating the layout of your Tilemap. \ No newline at end of file diff --git a/MrBigsock/Packages/com.unity.2d.tilemap.extras/Documentation~/GroupBrush.md b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Documentation~/GroupBrush.md new file mode 100644 index 0000000000000000000000000000000000000000..6efd399d9add6d7cde9ca71fee9d3af9baec9c33 --- /dev/null +++ b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Documentation~/GroupBrush.md @@ -0,0 +1,20 @@ +# Group Brush + +This Brush picks Tiles which are grouped together according to their position and its set properties. Set the __Gap__ value to identify which Tiles belong to the group, and set the __Limit__ value to ensure that the picked group remains within the desired size. Use this Brush as an example to create your own Brushes that can choose and pick specific Tiles + +## Properties + +| Property | Function | +| --------- | ------------------------------------------------------------ | +| __Gap__ | This value represents the minimum number of cells that must be in between picked Tiles. Only Tiles that are at least this many cells apart are picked by the Brush and placed in the group. Set this value to 0 to pick up all Tiles that are directly adjacent to each other in the group. | +| __Limit__ | This value represents the maximum number of cells around the initial picked position. Only Tiles within this range of cells are picked by the Brush and placed in the group. | + +## Usage + +Select the Group Brush, and use the [Picker Tool](https://docs.unity3d.com/Manual/Tilemap-Painting.html#Picker) and pick a position on the Tilemap. The Group Brush selects a group of Tiles based on its set properties and creates a Group. + + + +## Implementation + +The Group Brush inherits from the Grid Brush. It overrides the `Pick` method when picking a group of Tiles based on their position and its set properties. \ No newline at end of file diff --git a/MrBigsock/Packages/com.unity.2d.tilemap.extras/Documentation~/LineBrush.md b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Documentation~/LineBrush.md new file mode 100644 index 0000000000000000000000000000000000000000..ebd289eb3f3e3c3b5901885bd7633810e2f150de --- /dev/null +++ b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Documentation~/LineBrush.md @@ -0,0 +1,28 @@ +# Line Brush + +__Contribution by :__ [CraigGraff](https://github.com/CraigGraff) + +This Brush draws a line of Tiles onto a Tilemap. With this Brush selected, click once to set the starting point of the line and click again at another position to set the ending point of the line. This Brush then draws a line of Tiles between the two points. + +Use this as an example to create custom Brush behavior to make painting more efficient. + +## Properties + +| Property | Function | +| --------------------- | ------------------------------------------------------------ | +| __Line Start Active__ | Indicates whether the Line Brush has started drawing a line. | +| __Fill Gaps__ | Ensures that there are orthogonal connections between all Tiles that connect the start and end of the line. | +| __Line Start__ | The current starting point of the line. | + +## Usage +Select the Line Brush, then click once on a cell of the Tilemap to set the starting point of the line, then click on a second cell to set the ending point of the line. The Brush then draws the line of Tiles between the two set points. When the Line Brush is active, a blue outline will indicate the starting point of the line. + + + +To have Tiles which are orthogonally connected from start to end, enable the __Fill Gaps__ property in the Brush Editor. + + + +## Implementation + +The Line Brush inherits from the Grid Brush and overrides the `Paint` method to implement the line painting functionality. \ No newline at end of file diff --git a/MrBigsock/Packages/com.unity.2d.tilemap.extras/Documentation~/Other.md b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Documentation~/Other.md new file mode 100644 index 0000000000000000000000000000000000000000..6d71bcd334db4ab4149bc610bb4582f05cb0ffd2 --- /dev/null +++ b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Documentation~/Other.md @@ -0,0 +1,4 @@ +# Other + +This section will contain other useful scripts which you can use and other details in the future. + diff --git a/MrBigsock/Packages/com.unity.2d.tilemap.extras/Documentation~/PipelineTile.md b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Documentation~/PipelineTile.md new file mode 100644 index 0000000000000000000000000000000000000000..c73b4ea600eca8dfdcdd06ccc887e6f459edadec --- /dev/null +++ b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Documentation~/PipelineTile.md @@ -0,0 +1,23 @@ +# Pipeline Tile + +A Pipeline Tile take into consideration its orthogonal neighboring tiles and displays a Sprite depending on whether the neighboring Tile is the same Tile as itself and the number of Tiles bordering it. + +## Properties + +| Property | Function | +| --------- | ------------------------------------------------------------ | +| __None__ | Displays this selected Sprite when no other Tiles border the Tile. | +| __One__ | Displays this selected Sprite when one Tile borders the Tile. | +| __Two__ | Displays this selected Sprite when two Tiles border the Tile. | +| __Three__ | Displays this selected Sprite when three Tiles border the Tile. | +| __Four__ | Displays this selected Sprite when four Tiles border the Tile. | + +## Usage + +In the Pipeline Tile editor window, set up the Pipeline Tile by selecting the Sprites that are displayed depending on the number of Tiles bordering the Sprite. + + + +Then [paint](https://docs.unity3d.com/Manual/Tilemap-Painting.html) the Pipeline Tile onto the Tilemap with the [Tile Palette](https://docs.unity3d.com/Manual/Tilemap-Palette.html) tools. + + \ No newline at end of file diff --git a/MrBigsock/Packages/com.unity.2d.tilemap.extras/Documentation~/PrefabBrush.md b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Documentation~/PrefabBrush.md new file mode 100644 index 0000000000000000000000000000000000000000..f9d787127e4163171d8f1cd597474a209b2513f5 --- /dev/null +++ b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Documentation~/PrefabBrush.md @@ -0,0 +1,53 @@ +# Prefab Brush + +__Contributions by:__ [Pepperized](https://github.com/Pepperized), [superkerokero](https://github.com/superkerokero), [vladderb](https://github.com/vladderb), [RyotaMurohoshi](https://github.com/RyotaMurohoshi), [ManickYoj](https://github.com/ManickYoj), [Quickz](https://github.com/Quickz) + +This Brush instances and places randomly selected Prefabs onto the target location and parents the instanced object to the [paint target](https://docs.unity3d.com/Manual/Tilemap-Painting.htm). Use this Brush as an example to create custom Brushes which can quickly place an assortment of GameObjects onto structured locations. + +## Properties + +<table> +<thead> +<tr> +<th><strong>Property</strong></th> +<th colspan="2"><strong>Function</strong></th> +</tr> +</thead> +<tbody> +<tr> +<td><strong>Perlin Scale</strong></td> +<td colspan="2">The factor using the <a href="https://en.wikipedia.org/wiki/Perlin_noise">Perlin noise</a> algorithm for the random distribution of Prefabs chosen when painting.</td> +</tr> +<tr> +<td><strong>Prefabs</strong></td> +<td colspan="2">Set the number of and selection of Prefabs to paint from.</td> +</tr> +<tr> +<td></td> +<td><strong>Size</strong></td> +<td>Set the number of Prefabs that the Brush selects from.</td> +</tr> +<tr> +<td></td> +<td><strong>Element *</strong></td> +<td>Set one of the Prefabs to select from for each entry. The number of <b>Element</b> entries matches the value set in <b>Size</b>.</td> +</tr> +</tbody> +</table> + +## Usage + +First set the number of Prefabs to select from in the __Prefabs__ property, then add Prefab Assets to the list. Adjust the __Perlin Scale__ property to adjust the distribution of Prefabs painted onto a particular cell. + + + +When painting with the Prefab Brush, the Prefab Brush picks from the available Prefabs based on the __Perlin Scale__ set, and instantiate the Prefabs to the Scene. + + + +## Implementation + +The Prefab Brush inherits from the Grid Brush and implements the following overrides: + +- It overrides the `Paint` method to paint a Prefab from the Prefab selection. +- It overrides the `Erase` method to be able to erase the instantiated Prefabs or other GameObjects from the Scene. \ No newline at end of file diff --git a/MrBigsock/Packages/com.unity.2d.tilemap.extras/Documentation~/RandomBrush.md b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Documentation~/RandomBrush.md new file mode 100644 index 0000000000000000000000000000000000000000..b93b19ea9b77ff0f6ded5be222a561bf5f822ba5 --- /dev/null +++ b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Documentation~/RandomBrush.md @@ -0,0 +1,33 @@ +# Random Brush + +This Brush places random Tiles onto a Tilemap by selecting from defined **Tile Sets** while painting onto the the Tilemap. Use this as an example to create custom Brushes which store specific data per Brush, and to make Brushes with randomized painting behavior. + +## Properties + +| Property | Function | +| ----------------------- | ------------------------------------------------------------ | +| __Pick Random Tiles__ | Enable this property to pick the Tiles from the current selection as a random Tile Set. | +| __Add To Random Tiles__ | Enable this property to add the picked Tile Sets to existing Tile Sets instead of replacing them. | +| __Tile Set Size__ | Set the size of the Tile Set that is painted by this Brush. | +| __Number of Tiles__ | The number of Tile Sets. | +| __Tile Set__ | The Tile Set to randomize from | +| __Tiles__ | The Tiles in the Tile Set. | + +## Usage + +To create a **Tile Set**, first define the size of the Tile Set you want to paint by setting its size values in the **Tile Set Size** property. Then you can add Tile Sets manually with the Brush Editor or select them from an existing Tile Palette. + +To select Tile Sets from an existing [Tile Palette](https://docs.unity3d.com/Manual/Tilemap-Palette.html), enable the __Pick Random Tiles__ property and select the Tile Sets using the [Picker Tool](https://docs.unity3d.com/Manual/Tilemap-Painting.html#Picker). This will create a Tile Set, or multiple Sets if the picked size is larger than the size set in the **Tile Set Size** property. Enable the __Add To Random Tiles__ property to add a picked selection of Tiles onto new or existing Tile Sets instead of replacing them. + +</br> In this example, 3 Tile Sets of 1x2 are created. + +When painting with the Random Brush, the Random Brush will randomly pick from the available Tile Sets while painting the Tiles. + + + +## Implementation + +The Random Brush inherits from the Grid Brush and implements the following overrides: + +- It overrides the Paint method to paint random selections of Tiles from chosen Tile Sets. +- It overrides the Pick method to be able to pick selections of Tiles for the random Tile Sets. diff --git a/MrBigsock/Packages/com.unity.2d.tilemap.extras/Documentation~/RandomTile.md b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Documentation~/RandomTile.md new file mode 100644 index 0000000000000000000000000000000000000000..66c32470b0aae68b61589eec3804dae6eaf19730 --- /dev/null +++ b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Documentation~/RandomTile.md @@ -0,0 +1,22 @@ +# Random Tile + +Random Tiles are Tiles which pick a Sprite from a given list of Sprites and a target location pseudo-randomly, and then displays that Sprite. The Sprite displayed for the Tile is randomized based on its location and remains fixed for that specific location. + +### Properties + +| Property | Function | +| --------------------- | ------------------------------------------------------------ | +| __Number of Sprites__ | Set the number of Sprites to randomize from here. | +| __Sprite *__ | Select the Sprite the Tile can randomly place. | +| __Color__ | Set the color of the Tile. | +| __Collider Type__ | Select the type of [Collider Shape](https://docs.unity3d.com/Manual/class-TilemapCollider2D.html) the Tile will generate. | + +### Usage + +First set the size of the list of Sprites to choose from by setting the value to the __Number of Sprites__ property in the Random Tile editor window. Then select the Sprites that the Tiles chooses from for each Sprite entry. + + + +Paint the Random Tile onto the Tilemap with the [Tile Palette](https://docs.unity3d.com/Manual/Tilemap-Painting.html) tools. + + \ No newline at end of file diff --git a/MrBigsock/Packages/com.unity.2d.tilemap.extras/Documentation~/RuleOverrideTile.md b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Documentation~/RuleOverrideTile.md new file mode 100644 index 0000000000000000000000000000000000000000..912b99450d56c940d7497094206e9a1eb414e95c --- /dev/null +++ b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Documentation~/RuleOverrideTile.md @@ -0,0 +1,31 @@ +# Rule Override Tile + +__Contributions by:__ [johnsoncodehk](https://github.com/johnsoncodehk), [Autofire](https://github.com/Autofire) + +__Rule Override Tiles__ are Tiles which can override the Sprites and GameObjects for a given [Rule Tile](RuleTile.md) while maintaining the Rule set of the Rule Tile. This allows you to create Tiles that provide variations of a Rule Tile without setting new Rules. + +## Properties + +| Property | Function | +| ----------------- | ------------------------------------------------------------ | +| __Tile__ | The Rule Tile to override. | + +Depending on the Rule Tile that is overridden, there may be further properties which you can override here. Any public property in the Rule Tile that does not have a `RuleTile.DontOverride` attribute will be shown here and can be overridden. + +## Usage + +First select the Rule Tile to be overridden in the __Tile__ property. The Editor then displays the different Sprites and GameObjects in the selected Rule Tile which you can override. + +The editor displays the original Sprites that are used in the Rule Tile in the left column. Select the Sprites that override each of the respective original Sprites on the right ‘Override’ column. When the Rule Tile has a match that would usually output the original Sprite, it will instead output the override Sprite. + +Below that, the editor displays the original GameObjects that are used in the Rule Tile in the left column. Select the GameObjects that override each of the respective original GameObjects on the right ‘Override’ column. When the Rule Tile has a match that would usually output the original GameObject, it will instead output the override GameObject. + + + +If you have modified the original Rule Tile and changed the Sprites there, the Rule Override Tile will note that the original Sprites are missing. You can check the original Rule Tile to see if it is set up correctly or set the Override Sprites to None to remove the override. + + + +Paint with the Rule Override Tile using the [Tile Palette](https://docs.unity3d.com/Manual/Tilemap-Painting.html) tools. + + \ No newline at end of file diff --git a/MrBigsock/Packages/com.unity.2d.tilemap.extras/Documentation~/RuleTile.md b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Documentation~/RuleTile.md new file mode 100644 index 0000000000000000000000000000000000000000..ade85f40bfc13d4edc9a29d215a1348ed7920243 --- /dev/null +++ b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Documentation~/RuleTile.md @@ -0,0 +1,106 @@ +# Rule Tile + +__Contributions by:__ [johnsoncodehk](https://github.com/johnsoncodehk), [DreadBoy](https://github.com/DreadBoy), [AVChemodanov](https://github.com/AVChemodanov), [DoctorShinobi](https://github.com/DoctorShinobi), [n4n0lix](https://github.com/n4n0lix) + +This is a generic visual Tile that other Tiles such as the [Terrain Tiles](TerrainTile.md), [Pipeline Tile](PipelineTile.md), [Random Tile](RandomTile.md) or [Animated Tiles](AnimatedTile.md) are based on. There are specific types of Rule Tiles for each of the [Tilemap grid types](https://docs.unity3d.com/Manual/class-Grid.html). The default Rule Tile is for the default [Rectangle Grid](https://docs.unity3d.com/Manual/Tilemap-CreatingTilemaps.html) type; the Hexagonal Rule Tile is for the [Hexagonal Grid](https://docs.unity3d.com/Manual/Tilemap-Hexagonal.html) type; and the Isometric Rule Tile is for the [Isometric Grid](https://docs.unity3d.com/Manual/Tilemap-Isometric.html) types. The different types of Rule Tiles all possess the same properties. + +## Properties + +<br/>The Rule Tile editor of a Terrain Tile. + +| Property | Function | +| ----------------------- | ------------------------------------------------------- | +| __Default Sprite__ | The default Sprite set when creating a new Rule. | +| __Default GameObject__ | The default GameObject set when creating a new Rule. | +| __Default Collider__ | The default Collider Type set when creating a new Rule. | + +### Tiling Rules + +<br/>Tiling Rules properties + +| Property | Function | +| -------------- | ------------------------------------------------------------ | +| __Rule__ | The Rule Type for this Rule. | +| __GameObject__ | The GameObject for the Tile which fits this Rule. | +| __Collider__ | The Collider Type for the Tile which fits this Rule | +| __Output__ | The Output for the Tile which fits this Rule. Each Output type has its own properties. | + +### Output: Fixed + +| Property | Function | +| ---------- | -------------------------------------------------- | +| __Sprite__ | Display this Sprite for Tiles which fit this Rule. | + +### Output: Random + +| Property | Function | +| ----------- | ------------------------------------------------------------ | +| __Noise__ | The [Perlin noise](https://en.wikipedia.org/wiki/Perlin_noise) factor when placing the Tile. | +| __Shuffle__ | The randomized transform given to the Tile when placing it. | +| __Size__ | The number of Sprites to randomize from. | +| __Sprite__ | The Sprite for the Tile which fits this Rule. A random Sprite will be chosen out of this when placing the Tile. | + +### Output: Animation + +| Property | Function | +| ---------- | ------------------------------------------------------------ | +| __MinSpeed__ | The minimum speed at which the animation is played. | +| __MaxSpeed__ | The maximum speed at which the animation is played. | +| __Size__ | The number of Sprites in the animation. | +| __Sprite__ | The Sprite for the Tile which fits this Rule. Sprites will be shown in sequence based on the order of the list. | + +## Editor Properties + +| Property | Function | +| ----------------------- | ------------------------------------------------------- | +| __Extend Neighbor__ | Enabling this allows you to increase the range of neighbors beyond the 3x3 box. | + +## <a name="Usage"></a>Setting up a Rule Tile + +Set up the Rule Tile with the required rules with the __Rule Tile editor__. In the Rule Tile editor, you can change, add, duplicate or remove Rules in the **Tiling Rules** list. Click on the + or - buttons to add or remove Rules. If you have a Rule selected, clicking on the + button will allow you to choose between adding a new Rule or duplicating the selected Rule. The newly created Rule will be placed after the current selected Rule. Select and hold the top left corner of each row to drag them up or down to change the order of the Rules in the list. + +<br/>Rule Tile Editor + +When you add a new Rule, the Rule editor displays the following: the list of Rule properties, a 3x3 box that visualizes the behavior of the set Rules, and a Sprite selector that displays a preview of the selected Sprite. + + + +The 3x3 box represents the neighbors a Tile can have, where the center represents the Tile itself, and the eight bordering cells are its neighboring Tiles in their relative positions to the Tile. Each of the neighboring cells can be set with one of three options: **Don't Care**, **This** and **Not This**. These define the behavior of the Rule Tile towards these Tiles. Edit the 3x3 box to set up the Rule the Tile must match. + +| Options | Rule Tile behavior | +| -------------- | ------------------------------------------------------------ | +| __Don't Care__ | The Rule Tile ignores the contents in this cell. | +| __This__ | The Rule Tile checks if the contents of this cell is an instance of this Rule Tile. If it is an instance, the rule passes. If it is not an instance, the rule fails. | +| __Not This__ | The Rule Tile checks if the contents of this cell is not an instance of this Rule Tile. If it is not an instance, the rule passes. If it is an instance, the rule fails. | + +If all of the neighbors of the Rule Tile match the options set for their respective directions, then the Rule is considered matched and the rest of the Rule properties are applied. + +When the Rule is set to Fixed, the Rule will only match exactly the conditions set for its neighbors. The example below will only match if there are the same Rule Tiles to the left and right of it. + + + +When the Rule is set to ‘Rotated’, the 3x3 box will be rotated 90 degrees each time the Rule fails to match and it will try to match again with this rotated 3x3 box. If the Rule now matches, the contents of this Rule will be applied as well as the rotation required to match the Rule. Use this if you want the Rule to match for the four 90 degree rotations if rotation is possible. + + + +When the Rule is set to Mirror X, Mirror Y or Mirror XY, the 3x3 box will be mirrored in that axis each time the Rule fails to match and it will try to match again with this mirrored 3x3 box. If the Rule now matches, the contents of this Rule will be applied as well as the mirroring required to match the Rule. Use this if you want the Rule to match for the mirrored locations if mirroring is possible. + + + +If you want the Rule Tile to have a Random output, you can set the Output to Random. This will allow you to specify a number of input Sprites to randomize from. The rotation of the Sprites can be randomized as well by changing the __Shuffle__ property. + + + +If you want the Rule Tile to output a Sprite Animation, you can set the Output to Animation. This will allow you to specify a number of Sprites to animate sequentially. The speed of the Animation can be randomized as well by changing the __Speed__ property. + + + +When <b>Extend Neighbors</b> is enabled, the 3x3 box can be extended to allow for more specific neighbor matching. The Transform rule matching (eg. Rotated, Mirror) will apply for the extended neighbors set. + + + +Paint with the Rule Tile in the same way as other Tiles by using the Tile Palette tools. + + + +For optimization, please set the most common Rule at the top of the list of Rules and follow with next most common Rule and so on. When matching Rules during the placement of the Tile, the Rule Tile algorithm will check the first Rule first, before proceeding with the next Rules. \ No newline at end of file diff --git a/MrBigsock/Packages/com.unity.2d.tilemap.extras/Documentation~/TableOfContents.md b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Documentation~/TableOfContents.md new file mode 100644 index 0000000000000000000000000000000000000000..a8fef84cebfb712f881327163ca9ca82aa919ab5 --- /dev/null +++ b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Documentation~/TableOfContents.md @@ -0,0 +1,15 @@ +* [Overview](index.md) +* [Scriptable Brushes](Brushes.md) + * [GameObject Brush](GameObjectBrush.md) + * [Group Brush](GroupBrush.md) + * [Line Brush](LineBrush.md) + * [Random Brush](RandomBrush.md) +* [Scriptable Tiles](Tiles.md) + * [Animated Tile](AnimatedTile.md) + * [Rule Tile](RuleTile.md) + * [Rule Override Tile](RuleOverrideTile.md) +* [Other](Other.md) + * [Grid Information](GridInformation.md) + * [Custom Rules for Rule Tile](CustomRulesForRuleTile.md) + * [Contributors](Contributors.md) + diff --git a/MrBigsock/Packages/com.unity.2d.tilemap.extras/Documentation~/TerrainTile.md b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Documentation~/TerrainTile.md new file mode 100644 index 0000000000000000000000000000000000000000..5dc21d46e7fc209ec0b4bca50a6f7224e400f2b8 --- /dev/null +++ b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Documentation~/TerrainTile.md @@ -0,0 +1,35 @@ +# Terrain Tile + +Terrain Tiles take into consideration their orthogonal and diagonal neighbors and displays a Sprite depending on whether the neighboring Tile is the same Tile as itself. This is a similar behavior to [Pipeline Tiles](PipelineTile.md). + +## Properties + + + + + +The following properties describe the appearance of Sprites representing terrain or walls. Assign a Sprite that matches the description to each of these properties. + +| Property | Function | +| --------------------------------- | ------------------------------------------------------------ | +| __Filled__ | A Sprite with all sides filled. | +| __Three Sides__ | A Sprite with three sides. | +| __Two Sides and One Corner__ | A Sprite with two sides and one corner without adjacent sides. | +| __Two Adjacent Sides__ | A Sprite with two adjacent sides. | +| __Two Opposite Sides__ | A Sprite with two opposite sides across each other. | +| __One Side and Two Corners__ | A Sprite with a single side and two corners without adjacent sides. | +| __One Side and One Lower Corner__ | A Sprite with one side and a corner in the lower half of the Sprite. | +| __One Side and One Upper Corner__ | A Sprite with one side and a corner in the upper half of the Sprite. | +| __One Side__ | A Sprite with a single side. | +| __Four Corners__ | A Sprite with four unconnected corners without adjacent sides. | +| __Three Corners__ | A Sprite with three corners without adjacent sides. | +| __Two Adjacent Corners__ | A Sprite with two adjacent corners. | +| __Two Opposite Corners__ | A Sprite with two opposite corners across each other. | +| __One Corner__ | A Sprite with a single corner with no adjacent sides. | +| __Empty__ | A Sprite without any terrain. | + +## Usage + +Set up a Terrain Tile by select Sprites which fit the characteristics stated on the left of the Terrain Tile editor. As you paint with the Terrain Tile using the [Tile Palette](https://docs.unity3d.com/Manual/Tilemap-Palette.html) tools, the Tile Sprite automatically adjusts to the appropriate one relative to its position with neighboring Tiles. + + \ No newline at end of file diff --git a/MrBigsock/Packages/com.unity.2d.tilemap.extras/Documentation~/Tiles.md b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Documentation~/Tiles.md new file mode 100644 index 0000000000000000000000000000000000000000..30445b48d82ccfd84b91132b00e6f23bc904c8fa --- /dev/null +++ b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Documentation~/Tiles.md @@ -0,0 +1,11 @@ +# Scriptable Tiles + +You can script Tiles to adapt to different criteria and conditions, such as its position on the Tilemap. It then displays the Sprite which meets its scripted requirements. This allows you to create different Tiles that can help you save time and be more efficient when creating Tilemaps. Refer to the [Scriptable Tiles](https://docs.unity3d.com/Manual/Tilemap-ScriptableTiles.html) page for more information. + +The following **Scriptable Tiles** are included in this package, with examples of how they are implemented. You can use these Tiles as the base for your own custom Tiles as well. + + +- [Animated Tile](AnimatedTile.md) +- [Rule Tile](RuleTile.md) +- [Rule Override Tile](RuleOverrideTile.md) + diff --git a/MrBigsock/Packages/com.unity.2d.tilemap.extras/Documentation~/TintBrush.md b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Documentation~/TintBrush.md new file mode 100644 index 0000000000000000000000000000000000000000..3b58c9ccd101ce38bd113b26ef007f5f3a0f3e16 --- /dev/null +++ b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Documentation~/TintBrush.md @@ -0,0 +1,22 @@ +# Tint Brush + +This Brush changes the color of Tiles placed on a Tilemap to the color selected. + +## Properties + +| Property | Function | +| --------- | ----------------------------- | +| __Color__ | Select the Color of the tint. | + +## Usage + +Select the color to tint a Tile with in the Brush properties. Then use the Paint tool with the Brush to change the color of the Tiles you paint over. + + + +## Implementation + +The Tint Brush inherits from the Grid Brush and implements the following overrides: + +- It overrides the `Paint` method to set the color of a Tile. +- It overrides the `Erase` method to be able to set the color of a Tile back to the default white color. \ No newline at end of file diff --git a/MrBigsock/Packages/com.unity.2d.tilemap.extras/Documentation~/TintBrushSmooth.md b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Documentation~/TintBrushSmooth.md new file mode 100644 index 0000000000000000000000000000000000000000..568902b41f8bcdb87c4f6c92812ad049b8b2f4ae --- /dev/null +++ b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Documentation~/TintBrushSmooth.md @@ -0,0 +1,28 @@ +# Tint Brush (Smooth) + +This advanced Tint Brush interpolates tint color per-cell. This requires the use of a custom Shader (see TintedTilemap.shader in the package contents) and the helper component **TileTextureGenerator**. + +## Properties + +| Property | Function | +| --------- | ------------------------------------------------------------ | +| __Color__ | Select the color of the tint. | +| __Blend__ | Factor to blend the color of a Tile with this Brush's __Color__. | + +## Usage + +First set the [Tilemap Renderer](https://docs.unity3d.com/Manual/class-TilemapRenderer.html)'s __Material__ to the TintedTilemap.shader. Add the __Tile Texture Generator__ component to the Tilemap Renderer as well. Then select the desired tint color in the __Color__ property or pick the color from an existing Tile. + +Adjust the __Blend__ property to blend the chosen color with the original color of the Tile. A lower value weighs the new color with the existing color of the Tile, while a higher value weighs the new color with the color of the Brush. + +With the Brush selected, paint Tiles with the [Paintbbrush tool](https://docs.unity3d.com/Manual/Tilemap-Painting.html#Brush) to change their color. + + + +## Implementation + +The TintBrushSmooth inherits from the GridBrushBase and implements the following overrides: + +- It overrides the `Paint` method to set the color of a Tile. +- It overrides the `Erase` method to be able to set the color of a Tile back to the default white color. +- It overrides the `Pick` method to pick the color of a Tile. \ No newline at end of file diff --git a/MrBigsock/Packages/com.unity.2d.tilemap.extras/Documentation~/WeightedRandomTile.md b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Documentation~/WeightedRandomTile.md new file mode 100644 index 0000000000000000000000000000000000000000..c8b038e9fafa452b8052a96a6fdd0880cd81e6c2 --- /dev/null +++ b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Documentation~/WeightedRandomTile.md @@ -0,0 +1,23 @@ +# Weighted Random Tile + +__Contributions by:__ [nicovain](https://github.com/nicovain), [distantcam](https://github.com/distantcam) + +Weighted Random Tiles are Tiles which randomly pick a Sprite from a given list of Sprites and displays that Sprite at the target location. Adjusting the __Weight__ value of the Sprites changes their probability of appearing. The Sprite displayed for the Tile is randomized based on its location and remains fixed for that particular location. + +## Properties + + + +| Property | Function | +| --------------------- | ------------------------------------------------------------ | +| __Number of Sprites__ | The number of Sprites to randomize from. | +| __Sprite__ | A Sprite that is in the randomize pool. | +| __Weight__ | A Weight value of the Sprite that affects its probability of appearing. | +| __Color__ | The color of the Tile. | +| __Collider Type__ | The __Collider Shape__ generated by the Tile. | + +## Usage + +Select the Sprites to randomize from in the Weighted Random Tile editor. The Weight of each Sprite determines the probability of the appearance of the Sprite where the higher the weight, the higher the probability of appearance. Paint the Weighted Random Tile onto the Tilemap with the [Tile Palette](https://docs.unity3d.com/Manual/Tilemap-Palette.html) tools. + + \ No newline at end of file diff --git a/MrBigsock/Packages/com.unity.2d.tilemap.extras/Documentation~/images/AdvancedRuleOverrideTile.png b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Documentation~/images/AdvancedRuleOverrideTile.png new file mode 100644 index 0000000000000000000000000000000000000000..bfbc158309384bb004971ffe091ef6c99d10922c Binary files /dev/null and b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Documentation~/images/AdvancedRuleOverrideTile.png differ diff --git a/MrBigsock/Packages/com.unity.2d.tilemap.extras/Documentation~/images/AdvancedRuleOverrideTileEditor.png b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Documentation~/images/AdvancedRuleOverrideTileEditor.png new file mode 100644 index 0000000000000000000000000000000000000000..4e9d1e5511b1e8e8cc292336c1ef9abc768ca9a2 Binary files /dev/null and b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Documentation~/images/AdvancedRuleOverrideTileEditor.png differ diff --git a/MrBigsock/Packages/com.unity.2d.tilemap.extras/Documentation~/images/AnimatedTile.png b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Documentation~/images/AnimatedTile.png new file mode 100644 index 0000000000000000000000000000000000000000..0ed909ebefc6aa7b08558251773073f94f7e9ed5 Binary files /dev/null and b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Documentation~/images/AnimatedTile.png differ diff --git a/MrBigsock/Packages/com.unity.2d.tilemap.extras/Documentation~/images/AnimatedTileEditor.png b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Documentation~/images/AnimatedTileEditor.png new file mode 100644 index 0000000000000000000000000000000000000000..2c31ab2208ed395534c449508044fbad7e3bb8b7 Binary files /dev/null and b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Documentation~/images/AnimatedTileEditor.png differ diff --git a/MrBigsock/Packages/com.unity.2d.tilemap.extras/Documentation~/images/BrushDropdown.png b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Documentation~/images/BrushDropdown.png new file mode 100644 index 0000000000000000000000000000000000000000..eec74ccfa8b19603f1b114a4177863cc85523be6 Binary files /dev/null and b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Documentation~/images/BrushDropdown.png differ diff --git a/MrBigsock/Packages/com.unity.2d.tilemap.extras/Documentation~/images/CoordinateBrush.png b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Documentation~/images/CoordinateBrush.png new file mode 100644 index 0000000000000000000000000000000000000000..8abea74571cefce90f54acbd0f8b47b0f531c6e6 Binary files /dev/null and b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Documentation~/images/CoordinateBrush.png differ diff --git a/MrBigsock/Packages/com.unity.2d.tilemap.extras/Documentation~/images/GameObjectBrush.png b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Documentation~/images/GameObjectBrush.png new file mode 100644 index 0000000000000000000000000000000000000000..0a6e31b6fdea54c6f289114d07b3d8b7dcf865a8 Binary files /dev/null and b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Documentation~/images/GameObjectBrush.png differ diff --git a/MrBigsock/Packages/com.unity.2d.tilemap.extras/Documentation~/images/GroupBrush.png b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Documentation~/images/GroupBrush.png new file mode 100644 index 0000000000000000000000000000000000000000..6a9d6bef13ddf3758be85b108468c2e63c50ee32 Binary files /dev/null and b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Documentation~/images/GroupBrush.png differ diff --git a/MrBigsock/Packages/com.unity.2d.tilemap.extras/Documentation~/images/LineBrush.png b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Documentation~/images/LineBrush.png new file mode 100644 index 0000000000000000000000000000000000000000..bdb69c042ed9ba9c3878485bdbad482524acb7ad Binary files /dev/null and b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Documentation~/images/LineBrush.png differ diff --git a/MrBigsock/Packages/com.unity.2d.tilemap.extras/Documentation~/images/LineBrushFillGaps.png b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Documentation~/images/LineBrushFillGaps.png new file mode 100644 index 0000000000000000000000000000000000000000..f90dd37d67b12adb3f79d88e21670bce3e974932 Binary files /dev/null and b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Documentation~/images/LineBrushFillGaps.png differ diff --git a/MrBigsock/Packages/com.unity.2d.tilemap.extras/Documentation~/images/PipelineTile.png b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Documentation~/images/PipelineTile.png new file mode 100644 index 0000000000000000000000000000000000000000..81904682cb9fceeba5b873aa3fe46972d686f688 Binary files /dev/null and b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Documentation~/images/PipelineTile.png differ diff --git a/MrBigsock/Packages/com.unity.2d.tilemap.extras/Documentation~/images/PipelineTileEditor.png b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Documentation~/images/PipelineTileEditor.png new file mode 100644 index 0000000000000000000000000000000000000000..78a6b2264087f9187c2f9b736fe72e8bd2287243 Binary files /dev/null and b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Documentation~/images/PipelineTileEditor.png differ diff --git a/MrBigsock/Packages/com.unity.2d.tilemap.extras/Documentation~/images/PrefabBrush.png b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Documentation~/images/PrefabBrush.png new file mode 100644 index 0000000000000000000000000000000000000000..5ba8b14ab94b81e66c7be03ba9e6cfc6ba1e51ba Binary files /dev/null and b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Documentation~/images/PrefabBrush.png differ diff --git a/MrBigsock/Packages/com.unity.2d.tilemap.extras/Documentation~/images/PrefabBrushEditor.png b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Documentation~/images/PrefabBrushEditor.png new file mode 100644 index 0000000000000000000000000000000000000000..7d85f4f8a8072cd79a65376e86a210b73baa3525 Binary files /dev/null and b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Documentation~/images/PrefabBrushEditor.png differ diff --git a/MrBigsock/Packages/com.unity.2d.tilemap.extras/Documentation~/images/RandomBrush.png b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Documentation~/images/RandomBrush.png new file mode 100644 index 0000000000000000000000000000000000000000..0d7ea8880df469a2cf2cb523bf3cbc6c2dae6a5b Binary files /dev/null and b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Documentation~/images/RandomBrush.png differ diff --git a/MrBigsock/Packages/com.unity.2d.tilemap.extras/Documentation~/images/RandomBrushTileSet.png b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Documentation~/images/RandomBrushTileSet.png new file mode 100644 index 0000000000000000000000000000000000000000..8348118c7c9055fbdfd5b169808634c4bff9f251 Binary files /dev/null and b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Documentation~/images/RandomBrushTileSet.png differ diff --git a/MrBigsock/Packages/com.unity.2d.tilemap.extras/Documentation~/images/RandomTile.png b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Documentation~/images/RandomTile.png new file mode 100644 index 0000000000000000000000000000000000000000..537608f4f7bc6e4d9d04fe7a1d7ea647cbecf94f Binary files /dev/null and b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Documentation~/images/RandomTile.png differ diff --git a/MrBigsock/Packages/com.unity.2d.tilemap.extras/Documentation~/images/RandomTileEditor.png b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Documentation~/images/RandomTileEditor.png new file mode 100644 index 0000000000000000000000000000000000000000..797e6f3afc015f76a4d532fcef978410a39d2b5d Binary files /dev/null and b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Documentation~/images/RandomTileEditor.png differ diff --git a/MrBigsock/Packages/com.unity.2d.tilemap.extras/Documentation~/images/RuleOverrideTile.png b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Documentation~/images/RuleOverrideTile.png new file mode 100644 index 0000000000000000000000000000000000000000..19bffd721cceee363525900e431a85f267788ea6 Binary files /dev/null and b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Documentation~/images/RuleOverrideTile.png differ diff --git a/MrBigsock/Packages/com.unity.2d.tilemap.extras/Documentation~/images/RuleOverrideTileEditor.png b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Documentation~/images/RuleOverrideTileEditor.png new file mode 100644 index 0000000000000000000000000000000000000000..7f53aafaa1f339acd48348a199a7fdce6cda940d Binary files /dev/null and b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Documentation~/images/RuleOverrideTileEditor.png differ diff --git a/MrBigsock/Packages/com.unity.2d.tilemap.extras/Documentation~/images/RuleOverrideTileEditorAdvanced.png b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Documentation~/images/RuleOverrideTileEditorAdvanced.png new file mode 100644 index 0000000000000000000000000000000000000000..98911b4ecfd4d7ab4b6be5d983fb260fead3a7e1 Binary files /dev/null and b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Documentation~/images/RuleOverrideTileEditorAdvanced.png differ diff --git a/MrBigsock/Packages/com.unity.2d.tilemap.extras/Documentation~/images/RuleOverrideTileEditorMissing.png b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Documentation~/images/RuleOverrideTileEditorMissing.png new file mode 100644 index 0000000000000000000000000000000000000000..1265c9c03df5622275bb888c4a88df5943288605 Binary files /dev/null and b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Documentation~/images/RuleOverrideTileEditorMissing.png differ diff --git a/MrBigsock/Packages/com.unity.2d.tilemap.extras/Documentation~/images/RuleTile.png b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Documentation~/images/RuleTile.png new file mode 100644 index 0000000000000000000000000000000000000000..a19574efceb9682cf3c68c9da75c1f3cc2165ab7 Binary files /dev/null and b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Documentation~/images/RuleTile.png differ diff --git a/MrBigsock/Packages/com.unity.2d.tilemap.extras/Documentation~/images/RuleTileEditor.png b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Documentation~/images/RuleTileEditor.png new file mode 100644 index 0000000000000000000000000000000000000000..7e05a64cdb9fecc6d1f947c25123ad4146507ff6 Binary files /dev/null and b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Documentation~/images/RuleTileEditor.png differ diff --git a/MrBigsock/Packages/com.unity.2d.tilemap.extras/Documentation~/images/RuleTileOutputAnimation.png b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Documentation~/images/RuleTileOutputAnimation.png new file mode 100644 index 0000000000000000000000000000000000000000..816f14e1cc6e5b5ce933c08c0802b93d3b4b8854 Binary files /dev/null and b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Documentation~/images/RuleTileOutputAnimation.png differ diff --git a/MrBigsock/Packages/com.unity.2d.tilemap.extras/Documentation~/images/RuleTileOutputRandom.png b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Documentation~/images/RuleTileOutputRandom.png new file mode 100644 index 0000000000000000000000000000000000000000..8a7b49008e695a07ccbd39d3dce185fdf679e9eb Binary files /dev/null and b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Documentation~/images/RuleTileOutputRandom.png differ diff --git a/MrBigsock/Packages/com.unity.2d.tilemap.extras/Documentation~/images/RuleTileRule.png b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Documentation~/images/RuleTileRule.png new file mode 100644 index 0000000000000000000000000000000000000000..e8bbfb88c15c41a0c3af8384bdd58597392d5741 Binary files /dev/null and b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Documentation~/images/RuleTileRule.png differ diff --git a/MrBigsock/Packages/com.unity.2d.tilemap.extras/Documentation~/images/RuleTileRuleExtendNeighbor.png b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Documentation~/images/RuleTileRuleExtendNeighbor.png new file mode 100644 index 0000000000000000000000000000000000000000..d3ed1a9732f858d8ee9cccb28ed291267846e5a8 Binary files /dev/null and b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Documentation~/images/RuleTileRuleExtendNeighbor.png differ diff --git a/MrBigsock/Packages/com.unity.2d.tilemap.extras/Documentation~/images/RuleTileRuleFixed.png b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Documentation~/images/RuleTileRuleFixed.png new file mode 100644 index 0000000000000000000000000000000000000000..dcdd57d9c2eacc11f0ae5d0e77d88803cbc3b8a5 Binary files /dev/null and b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Documentation~/images/RuleTileRuleFixed.png differ diff --git a/MrBigsock/Packages/com.unity.2d.tilemap.extras/Documentation~/images/RuleTileRuleMirror.png b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Documentation~/images/RuleTileRuleMirror.png new file mode 100644 index 0000000000000000000000000000000000000000..c33fa275a01158fba7ab0e862d17c7b8b295657c Binary files /dev/null and b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Documentation~/images/RuleTileRuleMirror.png differ diff --git a/MrBigsock/Packages/com.unity.2d.tilemap.extras/Documentation~/images/RuleTileRuleRotated.png b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Documentation~/images/RuleTileRuleRotated.png new file mode 100644 index 0000000000000000000000000000000000000000..08e7976c1364b6ba6dd86b4f16d090a41a65595e Binary files /dev/null and b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Documentation~/images/RuleTileRuleRotated.png differ diff --git a/MrBigsock/Packages/com.unity.2d.tilemap.extras/Documentation~/images/RuleTileRuleTip.png b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Documentation~/images/RuleTileRuleTip.png new file mode 100644 index 0000000000000000000000000000000000000000..a737ea5bac8fcee137e4a1bc1a3ed59a08f912fc Binary files /dev/null and b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Documentation~/images/RuleTileRuleTip.png differ diff --git a/MrBigsock/Packages/com.unity.2d.tilemap.extras/Documentation~/images/TerrainTile.png b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Documentation~/images/TerrainTile.png new file mode 100644 index 0000000000000000000000000000000000000000..bb265f8df4dbf653607b62010929ea2e275f28df Binary files /dev/null and b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Documentation~/images/TerrainTile.png differ diff --git a/MrBigsock/Packages/com.unity.2d.tilemap.extras/Documentation~/images/TerrainTileEditor.png b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Documentation~/images/TerrainTileEditor.png new file mode 100644 index 0000000000000000000000000000000000000000..14734032a108e5f00604ed9d2f0a8cc9e5fb209d Binary files /dev/null and b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Documentation~/images/TerrainTileEditor.png differ diff --git a/MrBigsock/Packages/com.unity.2d.tilemap.extras/Documentation~/images/TintBrush.png b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Documentation~/images/TintBrush.png new file mode 100644 index 0000000000000000000000000000000000000000..18b2d2fef52cea4ac96602445e068fa92dd23243 Binary files /dev/null and b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Documentation~/images/TintBrush.png differ diff --git a/MrBigsock/Packages/com.unity.2d.tilemap.extras/Documentation~/images/TintBrushSmooth.png b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Documentation~/images/TintBrushSmooth.png new file mode 100644 index 0000000000000000000000000000000000000000..38eda91ae10deafea07768c1cc1b9611c4ebdbcb Binary files /dev/null and b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Documentation~/images/TintBrushSmooth.png differ diff --git a/MrBigsock/Packages/com.unity.2d.tilemap.extras/Documentation~/images/WeightedRandomTile.png b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Documentation~/images/WeightedRandomTile.png new file mode 100644 index 0000000000000000000000000000000000000000..d8f413b4dbd021f98eeba5620ce0bf1ac67961f1 Binary files /dev/null and b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Documentation~/images/WeightedRandomTile.png differ diff --git a/MrBigsock/Packages/com.unity.2d.tilemap.extras/Documentation~/images/WeightedRandomTileEditor.png b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Documentation~/images/WeightedRandomTileEditor.png new file mode 100644 index 0000000000000000000000000000000000000000..f216942228eb59e620dcf83395dde367c5a17d12 Binary files /dev/null and b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Documentation~/images/WeightedRandomTileEditor.png differ diff --git a/MrBigsock/Packages/com.unity.2d.tilemap.extras/Documentation~/index.md b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Documentation~/index.md new file mode 100644 index 0000000000000000000000000000000000000000..105e0e3c49948b490fecb3b68525759f179593e5 --- /dev/null +++ b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Documentation~/index.md @@ -0,0 +1,33 @@ +# 2D Tilemap Extras + +The 2D Tilemap Extras package contains reusable 2D and Tilemap editor scripts which you can use for your own Projects, and as the basis for your own custom Brushes and Tiles. You can freely customize the behavior of the scripts to create new Brushes that suit different uses or scenarios. To find these additional Brushes, open the Tile Palette window (menu: __Window > 2D > Tile Palette__) and open the Brush drop-down menu near the bottom of the editor. Select from the available Brush options for different effects. + + + +The source code for these scripts can be found in the repository [2d-extras](https://github.com/Unity-Technologies/2d-extras "2d-extras: Extras for 2d features"), and examples of the implemented scripts can be found in the sister repository [2d-techdemos](https://github.com/Unity-Technologies/2d-techdemos "2d-techdemos: Examples for 2d features"). + +## Scriptable Brushes + +- [GameObject](GameObjectBrush.md): This Brush instances, places and manipulates GameObjects onto the Scene. Use this as an example to create Brushes which targets GameObjects, other than Tiles, for instancing and manipulation. + +- [Group](GroupBrush.md): This Brush picks groups of Tiles based on their positions relative to each other. Adjust the size of groups the Brush picks by setting the Gap and Limit properties. Use this Brush as an example to create Brushes that pick Tiles based on specific criteria. + +- [Line](LineBrush.md): This Brush draws a line of Tiles between two points onto a Tilemap. Use this as an example to modify Brush painting behavior to make painting more efficient. + +- [Random](RandomBrush.md): This Brush places random Tiles onto a Tilemap. Use this as an example to create Brushes which store specific data per Brush and to make Brushes which randomize behavior. + +## Scriptable Tiles + +The following are the [Scriptable Tiles](Tiles.md) included in this package. You can create (menu: __Create > Tiles__ ) the following additional Tile types that are included with this package. + +- [Animated](AnimatedTile.md): This Tile runs through and displays a list of Sprites in sequence to create a frame-by-frame animation. +- [Rule Tile](RuleTile.md): This is a generic visual Tile that accepts rules you create with the __Tiling Rules__ editor to create different Tilesets. Rule Tiles are the basis of the Terrain, Pipeline, Random or Animated Tiles. There are different types of Rule Tiles for each of the [Tilemap grid types](https://docs.unity3d.com/Manual/class-Grid.html). The default Rule Tile is only used with the Rectangle Grid type Tilemap, while the Hexagonal and Isometric Rule Tiles are used with their respective Grid types. +- __Hexagonal Rule Tile__: A Rule Tile for [Hexagonal Grids](https://docs.unity3d.com/Documentation/Manual/Tilemap-Hexagonal.html). Enable the **Flat Top** property for a Flat Top Hexagonal Grid, or clear it for a Pointed Top Hexagonal Grid. +- __Isometric Rule Tile__: A Rule Tile for use with [Isometric Grids](https://docs.unity3d.com/Documentation/Manual/Tilemap-Isometric-CreateIso.html). +- [Rule Override Tile](RuleOverrideTile.md): This Tile can override Sprites and GameObjects for a given [Rule Tile](RuleTile.md) to provide different behaviour without changing the original Rules. +- [Advanced Rule Override Tile](AdvancedRuleOverrideTile.md): This Tile can override a subset of Rules for a given [Rule Tile](RuleTile.md) to provide specialized behavior, while keeping the rest of the original Rules intact. + +## Other + +- [GridInformation](GridInformation.md): A simple MonoBehavior that stores and provides information based on Grid positions and keywords. +- [Custom Rules for RuleTile](CustomRulesForRuleTile.md): This helps to create new custom Rules for the Rule Tile with more options. \ No newline at end of file diff --git a/MrBigsock/Packages/com.unity.2d.tilemap.extras/Editor.meta b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Editor.meta new file mode 100644 index 0000000000000000000000000000000000000000..a05502822b9ec196defbe5245223d2f152dc5ac9 --- /dev/null +++ b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Editor.meta @@ -0,0 +1,8 @@ +fileFormatVersion: 2 +guid: 58147b5e82a62ad4aacd2cfa2ef653bc +folderAsset: yes +DefaultImporter: + externalObjects: {} + userData: + assetBundleName: + assetBundleVariant: diff --git a/MrBigsock/Packages/com.unity.2d.tilemap.extras/Editor/Brushes.meta b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Editor/Brushes.meta new file mode 100644 index 0000000000000000000000000000000000000000..3c05102f342285255665743dcd70b5ecc5ccdd81 --- /dev/null +++ b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Editor/Brushes.meta @@ -0,0 +1,8 @@ +fileFormatVersion: 2 +guid: b56ed00274c3c354593c893dbb7264c6 +folderAsset: yes +DefaultImporter: + externalObjects: {} + userData: + assetBundleName: + assetBundleVariant: diff --git a/MrBigsock/Packages/com.unity.2d.tilemap.extras/Editor/Brushes/CoordinateBrush.meta b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Editor/Brushes/CoordinateBrush.meta new file mode 100644 index 0000000000000000000000000000000000000000..94601d6c624dbda7416d7dc134eb2058b1401673 --- /dev/null +++ b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Editor/Brushes/CoordinateBrush.meta @@ -0,0 +1,8 @@ +fileFormatVersion: 2 +guid: f770bfc3e11779c4dac1955be8899365 +folderAsset: yes +DefaultImporter: + externalObjects: {} + userData: + assetBundleName: + assetBundleVariant: diff --git a/MrBigsock/Packages/com.unity.2d.tilemap.extras/Editor/Brushes/CoordinateBrush/CoordinateBrush.cs b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Editor/Brushes/CoordinateBrush/CoordinateBrush.cs new file mode 100644 index 0000000000000000000000000000000000000000..76e64ca2c75a60a05fa5558f379c63b687473a5b --- /dev/null +++ b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Editor/Brushes/CoordinateBrush/CoordinateBrush.cs @@ -0,0 +1,41 @@ +using UnityEngine; + +namespace UnityEditor.Tilemaps +{ + /// <summary> + /// This Brush displays the cell coordinates it is targeting in the SceneView. + /// Use this as an example to create brushes which have extra visualization features when painting onto a Tilemap. + /// </summary> + [CustomGridBrush(true, false, false, "Coordinate Brush")] + public class CoordinateBrush : GridBrush + { + } + + /// <summary> + /// The Brush Editor for a Coordinate Brush. + /// </summary> + [CustomEditor(typeof(CoordinateBrush))] + public class CoordinateBrushEditor : GridBrushEditor + { + /// <summary> + /// Callback for painting the GUI for the GridBrush in the Scene View. + /// The CoordinateBrush Editor overrides this to draw the current coordinates of the brush. + /// </summary> + /// <param name="grid">Grid that the brush is being used on.</param> + /// <param name="brushTarget">Target of the GridBrushBase::ref::Tool operation. By default the currently selected GameObject.</param> + /// <param name="position">Current selected location of the brush.</param> + /// <param name="tool">Current GridBrushBase::ref::Tool selected.</param> + /// <param name="executing">Whether brush is being used.</param> + public override void OnPaintSceneGUI(GridLayout grid, GameObject brushTarget, BoundsInt position, GridBrushBase.Tool tool, bool executing) + { + base.OnPaintSceneGUI(grid, brushTarget, position, tool, executing); + + var labelText = "Pos: " + position.position; + if (position.size.x > 1 || position.size.y > 1) { + labelText += " Size: " + position.size; + } + + Handles.Label(grid.CellToWorld(position.position), labelText); + } + } +} diff --git a/MrBigsock/Packages/com.unity.2d.tilemap.extras/Editor/Brushes/CoordinateBrush/CoordinateBrush.cs.meta b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Editor/Brushes/CoordinateBrush/CoordinateBrush.cs.meta new file mode 100644 index 0000000000000000000000000000000000000000..a143de247ed37f3ed3a249f5bd752de4cfd87e8b --- /dev/null +++ b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Editor/Brushes/CoordinateBrush/CoordinateBrush.cs.meta @@ -0,0 +1,13 @@ +fileFormatVersion: 2 +guid: d283e353fe1f4c34f8ac458281740fb4 +timeCreated: 1499149770 +licenseType: Pro +MonoImporter: + externalObjects: {} + serializedVersion: 2 + defaultReferences: [] + executionOrder: 0 + icon: {instanceID: 0} + userData: + assetBundleName: + assetBundleVariant: diff --git a/MrBigsock/Packages/com.unity.2d.tilemap.extras/Editor/Brushes/GameObjectBrush.meta b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Editor/Brushes/GameObjectBrush.meta new file mode 100644 index 0000000000000000000000000000000000000000..07421cd287be3520ae0dadbb38b1012e08abc0e3 --- /dev/null +++ b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Editor/Brushes/GameObjectBrush.meta @@ -0,0 +1,8 @@ +fileFormatVersion: 2 +guid: 122c3790d8f747345a2c265e1d0b6fc2 +folderAsset: yes +DefaultImporter: + externalObjects: {} + userData: + assetBundleName: + assetBundleVariant: diff --git a/MrBigsock/Packages/com.unity.2d.tilemap.extras/Editor/Brushes/GameObjectBrush/GameObjectBrush.cs b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Editor/Brushes/GameObjectBrush/GameObjectBrush.cs new file mode 100644 index 0000000000000000000000000000000000000000..ab05029b88b5d3f220b27d83fe0386586ba2a201 --- /dev/null +++ b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Editor/Brushes/GameObjectBrush/GameObjectBrush.cs @@ -0,0 +1,780 @@ +using System; +using System.Linq; +using UnityEditor.SceneManagement; +using UnityEngine; +using UnityEngine.SceneManagement; +using Object = UnityEngine.Object; + +namespace UnityEditor.Tilemaps +{ + /// <summary> + /// This Brush instances, places and manipulates GameObjects onto the scene. + /// Use this as an example to create brushes which targets objects other than tiles for manipulation. + /// </summary> + [HelpURL("https://docs.unity3d.com/Packages/com.unity.2d.tilemap.extras@latest/index.html?subfolder=/manual/GameObjectBrush.html")] + [CustomGridBrush(true, false, false, "GameObject Brush")] + public class GameObjectBrush : GridBrushBase + { + [Serializable] + internal class HiddenGridLayout + { + public Vector3 cellSize = Vector3.one; + public Vector3 cellGap = Vector3.zero; + public GridLayout.CellLayout cellLayout = GridLayout.CellLayout.Rectangle; + public GridLayout.CellSwizzle cellSwizzle = GridLayout.CellSwizzle.XYZ; + } + + [SerializeField] + private BrushCell[] m_Cells; + + [SerializeField] + private Vector3Int m_Size; + + [SerializeField] + private Vector3Int m_Pivot; + + [SerializeField] + [HideInInspector] + private bool m_CanChangeZPosition; + + [SerializeField] + [HideInInspector] + internal HiddenGridLayout hiddenGridLayout = new HiddenGridLayout(); + + /// <summary> + /// GameObject used for painting onto the Scene root + /// </summary> + [HideInInspector] + public GameObject hiddenGrid; + + /// <summary> + /// Anchor Point of the Instantiated GameObject in the cell when painting + /// </summary> + public Vector3 m_Anchor = new Vector3(0.5f, 0.5f, 0.5f); + /// <summary>Size of the brush in cells. </summary> + public Vector3Int size { get { return m_Size; } set { m_Size = value; SizeUpdated(); } } + /// <summary>Pivot of the brush. </summary> + public Vector3Int pivot { get { return m_Pivot; } set { m_Pivot = value; } } + /// <summary>All the brush cells the brush holds. </summary> + public BrushCell[] cells { get { return m_Cells; } } + /// <summary>Number of brush cells in the brush.</summary> + public int cellCount { get { return m_Cells != null ? m_Cells.Length : 0; } } + /// <summary>Number of brush cells based on size.</summary> + public int sizeCount + { + get { return m_Size.x * m_Size.y * m_Size.z; } + } + /// <summary>Whether the brush can change Z Position</summary> + public bool canChangeZPosition + { + get { return m_CanChangeZPosition; } + set { m_CanChangeZPosition = value; } + } + + /// <summary> + /// This Brush instances, places and manipulates GameObjects onto the scene. + /// </summary> + public GameObjectBrush() + { + Init(Vector3Int.one, Vector3Int.zero); + SizeUpdated(); + } + + private void OnEnable() + { + hiddenGrid = new GameObject(); + hiddenGrid.name = "(Paint on SceneRoot)"; + hiddenGrid.hideFlags = HideFlags.HideAndDontSave; + hiddenGrid.transform.position = Vector3.zero; + var grid = hiddenGrid.AddComponent<Grid>(); + grid.cellSize = hiddenGridLayout.cellSize; + grid.cellGap = hiddenGridLayout.cellGap; + grid.cellSwizzle = hiddenGridLayout.cellSwizzle; + grid.cellLayout = hiddenGridLayout.cellLayout; + } + + private void OnDisable() + { + DestroyImmediate(hiddenGrid); + } + + /// <summary> + /// Initializes the content of the GameObjectBrush. + /// </summary> + /// <param name="size">Size of the GameObjectBrush.</param> + public void Init(Vector3Int size) + { + Init(size, Vector3Int.zero); + SizeUpdated(); + } + + /// <summary>Initializes the content of the GameObjectBrush.</summary> + /// <param name="size">Size of the GameObjectBrush.</param> + /// <param name="pivot">Pivot point of the GameObjectBrush.</param> + public void Init(Vector3Int size, Vector3Int pivot) + { + m_Size = size; + m_Pivot = pivot; + SizeUpdated(); + } + + /// <summary> + /// Paints GameObjects into a given position within the selected layers. + /// The GameObjectBrush overrides this to provide GameObject painting functionality. + /// </summary> + /// <param name="gridLayout">Grid used for layout.</param> + /// <param name="brushTarget">Target of the paint operation. By default the currently selected GameObject.</param> + /// <param name="position">The coordinates of the cell to paint data to.</param> + public override void Paint(GridLayout gridLayout, GameObject brushTarget, Vector3Int position) + { + Vector3Int min = position - pivot; + BoundsInt bounds = new BoundsInt(min, m_Size); + + GetGrid(ref gridLayout, ref brushTarget); + BoxFill(gridLayout, brushTarget, bounds); + } + + private void PaintCell(GridLayout grid, Vector3Int position, Transform parent, BrushCell cell) + { + if (cell.gameObject == null) + return; + + var existingGO = GetObjectInCell(grid, parent, position); + if (existingGO == null) + { + SetSceneCell(grid, parent, position, cell.gameObject, cell.offset, cell.scale, cell.orientation, m_Anchor); + } + } + + /// <summary> + /// Erases GameObjects in a given position within the selected layers. + /// The GameObjectBrush overrides this to provide GameObject erasing functionality. + /// </summary> + /// <param name="gridLayout">Grid used for layout.</param> + /// <param name="brushTarget">Target of the erase operation. By default the currently selected GameObject.</param> + /// <param name="position">The coordinates of the cell to erase data from.</param> + public override void Erase(GridLayout gridLayout, GameObject brushTarget, Vector3Int position) + { + Vector3Int min = position - pivot; + BoundsInt bounds = new BoundsInt(min, m_Size); + + GetGrid(ref gridLayout, ref brushTarget); + BoxErase(gridLayout, brushTarget, bounds); + } + + private void EraseCell(GridLayout grid, Vector3Int position, Transform parent) + { + ClearSceneCell(grid, parent, position); + } + + /// <summary> + /// Box fills GameObjects into given bounds within the selected layers. + /// The GameObjectBrush overrides this to provide GameObject box-filling functionality. + /// </summary> + /// <param name="gridLayout">Grid to box fill data to.</param> + /// <param name="brushTarget">Target of the box fill operation. By default the currently selected GameObject.</param> + /// <param name="position">The bounds to box fill data into.</param> + public override void BoxFill(GridLayout gridLayout, GameObject brushTarget, BoundsInt position) + { + GetGrid(ref gridLayout, ref brushTarget); + + foreach (Vector3Int location in position.allPositionsWithin) + { + Vector3Int local = location - position.min; + BrushCell cell = m_Cells[GetCellIndexWrapAround(local.x, local.y, local.z)]; + PaintCell(gridLayout, location, brushTarget != null ? brushTarget.transform : null, cell); + } + } + + /// <summary> + /// Erases GameObjects from given bounds within the selected layers. + /// The GameObjectBrush overrides this to provide GameObject box-erasing functionality. + /// </summary> + /// <param name="gridLayout">Grid to erase data from.</param> + /// <param name="brushTarget">Target of the erase operation. By default the currently selected GameObject.</param> + /// <param name="position">The bounds to erase data from.</param> + public override void BoxErase(GridLayout gridLayout, GameObject brushTarget, BoundsInt position) + { + GetGrid(ref gridLayout, ref brushTarget); + + foreach (Vector3Int location in position.allPositionsWithin) + { + EraseCell(gridLayout, location, brushTarget != null ? brushTarget.transform : null); + } + } + + /// <summary> + /// This is not supported but it should floodfill GameObjects starting from a given position within the selected layers. + /// </summary> + /// <param name="gridLayout">Grid used for layout.</param> + /// <param name="brushTarget">Target of the flood fill operation. By default the currently selected GameObject.</param> + /// <param name="position">Starting position of the flood fill.</param> + public override void FloodFill(GridLayout gridLayout, GameObject brushTarget, Vector3Int position) + { + Debug.LogWarning("FloodFill not supported"); + } + + /// <summary> + /// Rotates the brush by 90 degrees in the given direction. + /// </summary> + /// <param name="direction">Direction to rotate by.</param> + /// <param name="layout">Cell Layout for rotating.</param> + public override void Rotate(RotationDirection direction, GridLayout.CellLayout layout) + { + Vector3Int oldSize = m_Size; + BrushCell[] oldCells = m_Cells.Clone() as BrushCell[]; + size = new Vector3Int(oldSize.y, oldSize.x, oldSize.z); + BoundsInt oldBounds = new BoundsInt(Vector3Int.zero, oldSize); + + foreach (Vector3Int oldPos in oldBounds.allPositionsWithin) + { + int newX = direction == RotationDirection.Clockwise ? oldSize.y - oldPos.y - 1 : oldPos.y; + int newY = direction == RotationDirection.Clockwise ? oldPos.x : oldSize.x - oldPos.x - 1; + int toIndex = GetCellIndex(newX, newY, oldPos.z); + int fromIndex = GetCellIndex(oldPos.x, oldPos.y, oldPos.z, oldSize.x, oldSize.y, oldSize.z); + m_Cells[toIndex] = oldCells[fromIndex]; + } + + int newPivotX = direction == RotationDirection.Clockwise ? oldSize.y - pivot.y - 1 : pivot.y; + int newPivotY = direction == RotationDirection.Clockwise ? pivot.x : oldSize.x - pivot.x - 1; + pivot = new Vector3Int(newPivotX, newPivotY, pivot.z); + + Matrix4x4 rotation = Matrix4x4.TRS(Vector3.zero, Quaternion.Euler(0f, 0f, direction == RotationDirection.Clockwise ? 90f : -90f), Vector3.one); + Quaternion orientation = Quaternion.Euler(0f, 0f, direction == RotationDirection.Clockwise ? 90f : -90f); + foreach (BrushCell cell in m_Cells) + { + cell.offset = rotation * cell.offset; + cell.orientation = cell.orientation * orientation; + } + } + + /// <summary>Flips the brush in the given axis.</summary> + /// <param name="flip">Axis to flip by.</param> + /// <param name="layout">Cell Layout for flipping.</param> + public override void Flip(FlipAxis flip, GridLayout.CellLayout layout) + { + if (flip == FlipAxis.X) + FlipX(); + else + FlipY(); + } + + /// <summary> + /// Picks child GameObjects given the coordinates of the cells. + /// The GameObjectBrush overrides this to provide GameObject picking functionality. + /// </summary> + /// <param name="gridLayout">Grid to pick data from.</param> + /// <param name="brushTarget">Target of the picking operation. By default the currently selected GameObject.</param> + /// <param name="position">The coordinates of the cells to paint data from.</param> + /// <param name="pivot">Pivot of the picking brush.</param> + public override void Pick(GridLayout gridLayout, GameObject brushTarget, BoundsInt position, Vector3Int pivot) + { + Reset(); + UpdateSizeAndPivot(new Vector3Int(position.size.x, position.size.y, 1), new Vector3Int(pivot.x, pivot.y, 0)); + + GetGrid(ref gridLayout, ref brushTarget); + + foreach (Vector3Int pos in position.allPositionsWithin) + { + Vector3Int brushPosition = new Vector3Int(pos.x - position.x, pos.y - position.y, 0); + PickCell(pos, brushPosition, gridLayout, brushTarget != null ? brushTarget.transform : null); + } + } + + private void PickCell(Vector3Int position, Vector3Int brushPosition, GridLayout grid, Transform parent) + { + Vector3 cellCenter = grid.LocalToWorld(grid.CellToLocalInterpolated(position + m_Anchor)); + GameObject go = GetObjectInCell(grid, parent, position); + + if (go != null) + { + Object prefab = PrefabUtility.GetCorrespondingObjectFromSource(go); + if (prefab) + { + SetGameObject(brushPosition, (GameObject) prefab); + } + else + { + GameObject newInstance = Instantiate(go); + newInstance.hideFlags = HideFlags.HideAndDontSave; + newInstance.SetActive(false); + SetGameObject(brushPosition, newInstance); + } + + SetOffset(brushPosition, go.transform.position - cellCenter); + SetScale(brushPosition, go.transform.localScale); + SetOrientation(brushPosition, go.transform.localRotation); + } + } + + /// <summary> + /// MoveStart is called when user starts moving the area previously selected with the selection marquee. + /// The GameObjectBrush overrides this to provide GameObject moving functionality. + /// </summary> + /// <param name="gridLayout">Grid used for layout.</param> + /// <param name="brushTarget">Target of the move operation. By default the currently selected GameObject.</param> + /// <param name="position">Position where the move operation has started.</param> + public override void MoveStart(GridLayout gridLayout, GameObject brushTarget, BoundsInt position) + { + Reset(); + UpdateSizeAndPivot(new Vector3Int(position.size.x, position.size.y, 1), Vector3Int.zero); + + GetGrid(ref gridLayout, ref brushTarget); + + var targetTransform = brushTarget != null ? brushTarget.transform : null; + foreach (Vector3Int pos in position.allPositionsWithin) + { + Vector3Int brushPosition = new Vector3Int(pos.x - position.x, pos.y - position.y, 0); + PickCell(pos, brushPosition, gridLayout, targetTransform); + ClearSceneCell(gridLayout, targetTransform, pos); + } + } + + /// <summary> + /// MoveEnd is called when user has ended the move of the area previously selected with the selection marquee. + /// The GameObjectBrush overrides this to provide GameObject moving functionality. + /// </summary> + /// <param name="gridLayout">Grid used for layout.</param> + /// <param name="brushTarget">Target of the move operation. By default the currently selected GameObject.</param> + /// <param name="position">Position where the move operation has ended.</param> + public override void MoveEnd(GridLayout gridLayout, GameObject brushTarget, BoundsInt position) + { + GetGrid(ref gridLayout, ref brushTarget); + Paint(gridLayout, brushTarget, position.min); + Reset(); + } + + private void GetGrid(ref GridLayout gridLayout, ref GameObject brushTarget) + { + if (brushTarget == hiddenGrid) + brushTarget = null; + if (brushTarget != null) + { + var targetGridLayout = brushTarget.GetComponent<GridLayout>(); + if (targetGridLayout != null) + gridLayout = targetGridLayout; + } + } + + /// <summary>Clears all data of the brush.</summary> + public void Reset() + { + foreach (var cell in m_Cells) + { + if (cell.gameObject != null && !EditorUtility.IsPersistent(cell.gameObject)) + { + DestroyImmediate(cell.gameObject); + } + cell.gameObject = null; + } + UpdateSizeAndPivot(Vector3Int.one, Vector3Int.zero); + } + + private void FlipX() + { + BrushCell[] oldCells = m_Cells.Clone() as BrushCell[]; + BoundsInt oldBounds = new BoundsInt(Vector3Int.zero, m_Size); + + foreach (Vector3Int oldPos in oldBounds.allPositionsWithin) + { + int newX = m_Size.x - oldPos.x - 1; + int toIndex = GetCellIndex(newX, oldPos.y, oldPos.z); + int fromIndex = GetCellIndex(oldPos); + m_Cells[toIndex] = oldCells[fromIndex]; + } + + int newPivotX = m_Size.x - pivot.x - 1; + pivot = new Vector3Int(newPivotX, pivot.y, pivot.z); + Matrix4x4 flip = Matrix4x4.TRS(Vector3.zero, Quaternion.identity, new Vector3(-1f, 1f, 1f)); + Quaternion orientation = Quaternion.Euler(0f, 0f, -180f); + + foreach (BrushCell cell in m_Cells) + { + Vector3 oldOffset = cell.offset; + cell.offset = flip * oldOffset; + cell.orientation = cell.orientation*orientation; + } + } + + private void FlipY() + { + BrushCell[] oldCells = m_Cells.Clone() as BrushCell[]; + BoundsInt oldBounds = new BoundsInt(Vector3Int.zero, m_Size); + + foreach (Vector3Int oldPos in oldBounds.allPositionsWithin) + { + int newY = m_Size.y - oldPos.y - 1; + int toIndex = GetCellIndex(oldPos.x, newY, oldPos.z); + int fromIndex = GetCellIndex(oldPos); + m_Cells[toIndex] = oldCells[fromIndex]; + } + + int newPivotY = m_Size.y - pivot.y - 1; + pivot = new Vector3Int(pivot.x, newPivotY, pivot.z); + Matrix4x4 flip = Matrix4x4.TRS(Vector3.zero, Quaternion.identity, new Vector3(1f, -1f, 1f)); + Quaternion orientation = Quaternion.Euler(0f, 0f, -180f); + foreach (BrushCell cell in m_Cells) + { + Vector3 oldOffset = cell.offset; + cell.offset = flip * oldOffset; + cell.orientation = cell.orientation * orientation; + } + } + + /// <summary>Updates the size, pivot and the number of layers of the brush.</summary> + /// <param name="size">New size of the brush.</param> + /// <param name="pivot">New pivot of the brush.</param> + public void UpdateSizeAndPivot(Vector3Int size, Vector3Int pivot) + { + m_Size = size; + m_Pivot = pivot; + SizeUpdated(); + } + + /// <summary> + /// Sets a GameObject at the position in the brush. + /// </summary> + /// <param name="position">Position to set the GameObject in the brush.</param> + /// <param name="go">GameObject to set in the brush.</param> + public void SetGameObject(Vector3Int position, GameObject go) + { + if (ValidateCellPosition(position)) + m_Cells[GetCellIndex(position)].gameObject = go; + } + + /// <summary> + /// Sets a position offset at the position in the brush. + /// </summary> + /// <param name="position">Position to set the offset in the brush.</param> + /// <param name="offset">Offset to set in the brush.</param> + public void SetOffset(Vector3Int position, Vector3 offset) + { + if (ValidateCellPosition(position)) + m_Cells[GetCellIndex(position)].offset = offset; + } + + /// <summary> + /// Sets an orientation at the position in the brush. + /// </summary> + /// <param name="position">Position to set the orientation in the brush.</param> + /// <param name="orientation">Orientation to set in the brush.</param> + public void SetOrientation(Vector3Int position, Quaternion orientation) + { + if (ValidateCellPosition(position)) + m_Cells[GetCellIndex(position)].orientation = orientation; + } + + /// <summary> + /// Sets a scale at the position in the brush. + /// </summary> + /// <param name="position">Position to set the scale in the brush.</param> + /// <param name="scale">Scale to set in the brush.</param> + public void SetScale(Vector3Int position, Vector3 scale) + { + if (ValidateCellPosition(position)) + m_Cells[GetCellIndex(position)].scale = scale; + } + + /// <summary>Gets the index to the GameObjectBrush::ref::BrushCell based on the position of the BrushCell.</summary> + /// <param name="brushPosition">Position of the BrushCell.</param> + /// <returns>The cell index for the position of the BrushCell.</returns> + public int GetCellIndex(Vector3Int brushPosition) + { + return GetCellIndex(brushPosition.x, brushPosition.y, brushPosition.z); + } + + /// <summary>Gets the index to the GameObjectBrush::ref::BrushCell based on the position of the BrushCell.</summary> + /// <param name="x">X Position of the BrushCell.</param> + /// <param name="y">Y Position of the BrushCell.</param> + /// <param name="z">Z Position of the BrushCell.</param> + /// <returns>The cell index for the position of the BrushCell.</returns> + public int GetCellIndex(int x, int y, int z) + { + return x + m_Size.x * y + m_Size.x * m_Size.y * z; + } + + /// <summary>Gets the index to the GameObjectBrush::ref::BrushCell based on the position of the BrushCell.</summary> + /// <param name="x">X Position of the BrushCell.</param> + /// <param name="y">Y Position of the BrushCell.</param> + /// <param name="z">Z Position of the BrushCell.</param> + /// <param name="sizex">X Size of Brush.</param> + /// <param name="sizey">Y Size of Brush.</param> + /// <param name="sizez">Z Size of Brush.</param> + /// <returns>The cell index for the position of the BrushCell.</returns> + public int GetCellIndex(int x, int y, int z, int sizex, int sizey, int sizez) + { + return x + sizex * y + sizex * sizey * z; + } + + /// <summary>Gets the index to the GameObjectBrush::ref::BrushCell based on the position of the BrushCell. Wraps each coordinate if it is larger than the size of the GameObjectBrush.</summary> + /// <param name="x">X Position of the BrushCell.</param> + /// <param name="y">Y Position of the BrushCell.</param> + /// <param name="z">Z Position of the BrushCell.</param> + /// <returns>The cell index for the position of the BrushCell.</returns> + public int GetCellIndexWrapAround(int x, int y, int z) + { + return (x % m_Size.x) + m_Size.x * (y % m_Size.y) + m_Size.x * m_Size.y * (z % m_Size.z); + } + + private GameObject GetObjectInCell(GridLayout grid, Transform parent, Vector3Int position) + { + int childCount; + GameObject[] sceneChildren = null; + if (parent == null) + { + var scene = SceneManager.GetActiveScene(); + sceneChildren = scene.GetRootGameObjects(); + childCount = scene.rootCount; + } + else + { + childCount = parent.childCount; + } + var anchorCellOffset = Vector3Int.FloorToInt(m_Anchor); + var cellSize = grid.cellSize; + anchorCellOffset.x = cellSize.x == 0 ? 0 : anchorCellOffset.x; + anchorCellOffset.y = cellSize.y == 0 ? 0 : anchorCellOffset.y; + anchorCellOffset.z = cellSize.z == 0 ? 0 : anchorCellOffset.z; + + for (var i = 0; i < childCount; i++) + { + var child = sceneChildren == null ? parent.GetChild(i) : sceneChildren[i].transform; + if (position == grid.WorldToCell(child.position) - anchorCellOffset) + return child.gameObject; + } + return null; + } + + private bool ValidateCellPosition(Vector3Int position) + { + var valid = + position.x >= 0 && position.x < size.x && + position.y >= 0 && position.y < size.y && + position.z >= 0 && position.z < size.z; + if (!valid) + throw new ArgumentException(string.Format("Position {0} is an invalid cell position. Valid range is between [{1}, {2}).", position, Vector3Int.zero, size)); + return true; + } + + internal void SizeUpdated(bool keepContents = false) + { + Array.Resize(ref m_Cells, sizeCount); + BoundsInt bounds = new BoundsInt(Vector3Int.zero, m_Size); + foreach (Vector3Int pos in bounds.allPositionsWithin) + { + if (keepContents || m_Cells[GetCellIndex(pos)] == null) + m_Cells[GetCellIndex(pos)] = new BrushCell(); + } + } + + private static void SetSceneCell(GridLayout grid, Transform parent, Vector3Int position, GameObject go, Vector3 offset, Vector3 scale, Quaternion orientation, Vector3 anchor) + { + if (go == null) + return; + + GameObject instance; + if (PrefabUtility.IsPartOfPrefabAsset(go)) + { + instance = (GameObject) PrefabUtility.InstantiatePrefab(go, parent != null ? parent.root.gameObject.scene : SceneManager.GetActiveScene()); + instance.transform.parent = parent; + } + else + { + instance = Instantiate(go, parent); + instance.name = go.name; + instance.SetActive(true); + foreach (var renderer in instance.GetComponentsInChildren<Renderer>()) + { + renderer.enabled = true; + } + } + + Undo.RegisterCreatedObjectUndo(instance, "Paint GameObject"); + instance.transform.position = grid.LocalToWorld(grid.CellToLocalInterpolated(new Vector3Int(position.x, position.y, position.z) + anchor)); + instance.transform.localRotation = orientation; + instance.transform.localScale = scale; + instance.transform.Translate(offset); + } + + private void ClearSceneCell(GridLayout grid, Transform parent, Vector3Int position) + { + GameObject erased = GetObjectInCell(grid, parent, new Vector3Int(position.x, position.y, position.z)); + if (erased != null) + Undo.DestroyObjectImmediate(erased); + } + + /// <summary> + /// Hashes the contents of the brush. + /// </summary> + /// <returns>A hash code of the brush</returns> + public override int GetHashCode() + { + int hash = 0; + unchecked + { + foreach (var cell in cells) + { + hash = hash * 33 + cell.GetHashCode(); + } + } + return hash; + } + + internal void UpdateHiddenGridLayout() + { + var grid = hiddenGrid.GetComponent<Grid>(); + hiddenGridLayout.cellSize = grid.cellSize; + hiddenGridLayout.cellGap = grid.cellGap; + hiddenGridLayout.cellSwizzle = grid.cellSwizzle; + hiddenGridLayout.cellLayout = grid.cellLayout; + } + + /// <summary> + ///Brush Cell stores the data to be painted in a grid cell. + /// </summary> + [Serializable] + public class BrushCell + { + /// <summary> + /// GameObject to be placed when painting. + /// </summary> + public GameObject gameObject { get { return m_GameObject; } set { m_GameObject = value; } } + /// <summary> + /// Position offset of the GameObject when painted. + /// </summary> + public Vector3 offset { get { return m_Offset; } set { m_Offset = value; } } + /// <summary> + /// Scale of the GameObject when painted. + /// </summary> + public Vector3 scale { get { return m_Scale; } set { m_Scale = value; } } + /// <summary> + /// Orientatio of the GameObject when painted. + /// </summary> + public Quaternion orientation { get { return m_Orientation; } set { m_Orientation = value; } } + + [SerializeField] + private GameObject m_GameObject; + [SerializeField] + Vector3 m_Offset = Vector3.zero; + [SerializeField] + Vector3 m_Scale = Vector3.one; + [SerializeField] + Quaternion m_Orientation = Quaternion.identity; + + /// <summary> + /// Hashes the contents of the brush cell. + /// </summary> + /// <returns>A hash code of the brush cell.</returns> + public override int GetHashCode() + { + int hash; + unchecked + { + hash = gameObject != null ? gameObject.GetInstanceID() : 0; + hash = hash * 33 + offset.GetHashCode(); + hash = hash * 33 + scale.GetHashCode(); + hash = hash * 33 + orientation.GetHashCode(); + } + return hash; + } + } + } + + /// <summary> + /// The Brush Editor for a GameObject Brush. + /// </summary> + [CustomEditor(typeof(GameObjectBrush))] + public class GameObjectBrushEditor : GridBrushEditorBase + { + private bool hiddenGridFoldout; + private Editor hiddenGridEditor; + + /// <summary> + /// The GameObjectBrush for this Editor + /// </summary> + public GameObjectBrush brush { get { return target as GameObjectBrush; } } + + /// <summary> Whether the GridBrush can change Z Position. </summary> + public override bool canChangeZPosition + { + get { return brush.canChangeZPosition; } + set { brush.canChangeZPosition = value; } + } + + /// <summary> + /// Callback for painting the GUI for the GridBrush in the Scene View. + /// The GameObjectBrush Editor overrides this to draw the preview of the brush when drawing lines. + /// </summary> + /// <param name="gridLayout">Grid that the brush is being used on.</param> + /// <param name="brushTarget">Target of the GameObjectBrush::ref::Tool operation. By default the currently selected GameObject.</param> + /// <param name="position">Current selected location of the brush.</param> + /// <param name="tool">Current GameObjectBrush::ref::Tool selected.</param> + /// <param name="executing">Whether brush is being used.</param> + public override void OnPaintSceneGUI(GridLayout gridLayout, GameObject brushTarget, BoundsInt position, GridBrushBase.Tool tool, bool executing) + { + BoundsInt gizmoRect = position; + + if (tool == GridBrushBase.Tool.Paint || tool == GridBrushBase.Tool.Erase) + gizmoRect = new BoundsInt(position.min - brush.pivot, brush.size); + + base.OnPaintSceneGUI(gridLayout, brushTarget, gizmoRect, tool, executing); + } + + /// <summary> + /// Callback for painting the inspector GUI for the GameObjectBrush in the tilemap palette. + /// The GameObjectBrush Editor overrides this to show the usage of this Brush. + /// </summary> + public override void OnPaintInspectorGUI() + { + EditorGUI.BeginChangeCheck(); + base.OnInspectorGUI(); + if (EditorGUI.EndChangeCheck() && brush.cellCount != brush.sizeCount) + { + brush.SizeUpdated(true); + } + + hiddenGridFoldout = EditorGUILayout.Foldout(hiddenGridFoldout, "SceneRoot Grid"); + if (hiddenGridFoldout) + { + EditorGUI.indentLevel++; + using (new EditorGUI.DisabledScope(GridPaintingState.scenePaintTarget != brush.hiddenGrid)) + { + if (hiddenGridEditor == null) + { + hiddenGridEditor = Editor.CreateEditor(brush.hiddenGrid.GetComponent<Grid>()); + } + brush.hiddenGrid.hideFlags = HideFlags.None; + EditorGUI.BeginChangeCheck(); + hiddenGridEditor.OnInspectorGUI(); + if (EditorGUI.EndChangeCheck()) + { + brush.UpdateHiddenGridLayout(); + EditorUtility.SetDirty(brush); + SceneView.RepaintAll(); + } + brush.hiddenGrid.hideFlags = HideFlags.HideAndDontSave; + } + EditorGUI.indentLevel--; + } + } + + /// <summary> + /// The targets that the GameObjectBrush can paint on + /// </summary> + public override GameObject[] validTargets + { + get + { + StageHandle currentStageHandle = StageUtility.GetCurrentStageHandle(); + var results = currentStageHandle.FindComponentsOfType<GridLayout>().Where(x => + { + GameObject gameObject; + return (gameObject = x.gameObject).scene.isLoaded + && gameObject.activeInHierarchy; + }).Select(x => x.gameObject); + return results.Prepend(brush.hiddenGrid).ToArray(); + } + } + + } +} \ No newline at end of file diff --git a/MrBigsock/Packages/com.unity.2d.tilemap.extras/Editor/Brushes/GameObjectBrush/GameObjectBrush.cs.meta b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Editor/Brushes/GameObjectBrush/GameObjectBrush.cs.meta new file mode 100644 index 0000000000000000000000000000000000000000..1674345036f8554e31cefe7681503ebbf8baff64 --- /dev/null +++ b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Editor/Brushes/GameObjectBrush/GameObjectBrush.cs.meta @@ -0,0 +1,13 @@ +fileFormatVersion: 2 +guid: 0abded712ad706044a53ef292972edbb +timeCreated: 1501700935 +licenseType: Pro +MonoImporter: + externalObjects: {} + serializedVersion: 2 + defaultReferences: [] + executionOrder: 0 + icon: {instanceID: 0} + userData: + assetBundleName: + assetBundleVariant: diff --git a/MrBigsock/Packages/com.unity.2d.tilemap.extras/Editor/Brushes/GroupBrush.meta b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Editor/Brushes/GroupBrush.meta new file mode 100644 index 0000000000000000000000000000000000000000..5f43352180851a8e2fb25daf3bfed0004e08fdfc --- /dev/null +++ b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Editor/Brushes/GroupBrush.meta @@ -0,0 +1,8 @@ +fileFormatVersion: 2 +guid: 7780fefa5c524be4d9f1151a0cd89ae1 +folderAsset: yes +DefaultImporter: + externalObjects: {} + userData: + assetBundleName: + assetBundleVariant: diff --git a/MrBigsock/Packages/com.unity.2d.tilemap.extras/Editor/Brushes/GroupBrush/GroupBrush.cs b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Editor/Brushes/GroupBrush/GroupBrush.cs new file mode 100644 index 0000000000000000000000000000000000000000..001d3e067bc65023b2df206acdb674aeb5ab3605 --- /dev/null +++ b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Editor/Brushes/GroupBrush/GroupBrush.cs @@ -0,0 +1,205 @@ +using System.Collections; +using System.Collections.Generic; +using UnityEngine; +using UnityEngine.Tilemaps; + +namespace UnityEditor.Tilemaps +{ + /// <summary> + /// This Brush helps to pick Tiles which are grouped together by position. Gaps can be set to identify if Tiles belong to a Group. Limits can be set to ensure that an over-sized Group will not be picked. Use this as an example to create brushes that have the ability to choose and pick whichever Tiles it is interested in. + /// </summary> + [HelpURL("https://docs.unity3d.com/Packages/com.unity.2d.tilemap.extras@latest/index.html?subfolder=/manual/GroupBrush.html")] + [CustomGridBrush(true, false, false, "Group Brush")] + public class GroupBrush : GridBrush + { + /// <summary> + /// The gap in cell count before stopping to consider a Tile in a Group + /// </summary> + public Vector3Int gap + { + get { return m_Gap; } + set + { + m_Gap = value; + OnValidate(); + } + } + + /// <summary> + /// The count in cells beyond the initial position before stopping to consider a Tile in a Group + /// </summary> + public Vector3Int limit + { + get { return m_Limit; } + set + { + m_Limit = value; + OnValidate(); + } + } + + private int visitedLocationsSize + { + get { return (m_Limit.x * 2 + 1) * (m_Limit.y * 2 + 1) * (m_Limit.z * 2 + 1); } + } + + [SerializeField] + private Vector3Int m_Gap = Vector3Int.one; + [SerializeField] + private Vector3Int m_Limit = Vector3Int.one * 3; + [SerializeField] + private BitArray m_VisitedLocations = new BitArray(7 * 7 * 7); + [SerializeField] + private Stack<Vector3Int> m_NextPosition = new Stack<Vector3Int>(); + + private void OnValidate() + { + if (m_Gap.x < 0) + m_Gap.x = 0; + if (m_Gap.y < 0) + m_Gap.y = 0; + if (m_Gap.z < 0) + m_Gap.z = 0; + if (m_Limit.x < 0) + m_Limit.x = 0; + if (m_Limit.y < 0) + m_Limit.y = 0; + if (m_Limit.z < 0) + m_Limit.z = 0; + if (m_VisitedLocations.Length != visitedLocationsSize) + m_VisitedLocations = new BitArray(visitedLocationsSize); + } + + /// <summary> + /// Picks tiles from selected Tilemaps and child GameObjects, given the coordinates of the cells. + /// The GroupBrush overrides this to locate groups of Tiles from the picking position. + /// </summary> + /// <param name="grid">Grid to pick data from.</param> + /// <param name="brushTarget">Target of the picking operation. By default the currently selected GameObject.</param> + /// <param name="position">The coordinates of the cells to paint data from.</param> + /// <param name="pickStart">Pivot of the picking brush.</param> + public override void Pick(GridLayout grid, GameObject brushTarget, BoundsInt position, Vector3Int pickStart) + { + // Do standard pick if user has selected a custom bounds + if (position.size.x > 1 || position.size.y > 1 || position.size.z > 1) + { + base.Pick(grid, brushTarget, position, pickStart); + return; + } + + Tilemap tilemap = brushTarget.GetComponent<Tilemap>(); + if (tilemap == null) + return; + + Reset(); + + // Determine size of picked locations based on gap and limit + Vector3Int limitOrigin = position.position - limit; + Vector3Int limitSize = Vector3Int.one + limit * 2; + BoundsInt limitBounds = new BoundsInt(limitOrigin, limitSize); + BoundsInt pickBounds = new BoundsInt(position.position, Vector3Int.one); + + m_VisitedLocations.SetAll(false); + m_VisitedLocations.Set(GetIndex(position.position, limitOrigin, limitSize), true); + m_NextPosition.Clear(); + m_NextPosition.Push(position.position); + + while (m_NextPosition.Count > 0) + { + Vector3Int next = m_NextPosition.Pop(); + if (tilemap.GetTile(next) != null) + { + Encapsulate(ref pickBounds, next); + BoundsInt gapBounds = new BoundsInt(next - gap, Vector3Int.one + gap * 2); + foreach (var gapPosition in gapBounds.allPositionsWithin) + { + if (!limitBounds.Contains(gapPosition)) + continue; + int index = GetIndex(gapPosition, limitOrigin, limitSize); + if (!m_VisitedLocations.Get(index)) + { + m_NextPosition.Push(gapPosition); + m_VisitedLocations.Set(index, true); + } + } + } + } + + UpdateSizeAndPivot(pickBounds.size, position.position - pickBounds.position); + + foreach (Vector3Int pos in pickBounds.allPositionsWithin) + { + Vector3Int brushPosition = new Vector3Int(pos.x - pickBounds.x, pos.y - pickBounds.y, pos.z - pickBounds.z); + if (m_VisitedLocations.Get(GetIndex(pos, limitOrigin, limitSize))) + { + PickCell(pos, brushPosition, tilemap); + } + } + } + + private void Encapsulate(ref BoundsInt bounds, Vector3Int position) + { + if (bounds.Contains(position)) + return; + + if (position.x < bounds.position.x) + { + var increase = bounds.x - position.x; + bounds.position = new Vector3Int(position.x, bounds.y, bounds.z); + bounds.size = new Vector3Int(bounds.size.x + increase, bounds.size.y, bounds.size.z); + } + if (position.x >= bounds.xMax) + { + var increase = position.x - bounds.xMax + 1; + bounds.size = new Vector3Int(bounds.size.x + increase, bounds.size.y, bounds.size.z); + } + if (position.y < bounds.position.y) + { + var increase = bounds.y - position.y; + bounds.position = new Vector3Int(bounds.x, position.y, bounds.z); + bounds.size = new Vector3Int(bounds.size.x, bounds.size.y + increase, bounds.size.z); + } + if (position.y >= bounds.yMax) + { + var increase = position.y - bounds.yMax + 1; + bounds.size = new Vector3Int(bounds.size.x, bounds.size.y + increase, bounds.size.z); + } + if (position.z < bounds.position.z) + { + var increase = bounds.z - position.z; + bounds.position = new Vector3Int(bounds.x, bounds.y, position.z); + bounds.size = new Vector3Int(bounds.size.x, bounds.size.y, bounds.size.z + increase); + } + if (position.z >= bounds.zMax) + { + var increase = position.z - bounds.zMax + 1; + bounds.size = new Vector3Int(bounds.size.x, bounds.size.y, bounds.size.z + increase); + } + } + + private int GetIndex(Vector3Int position, Vector3Int origin, Vector3Int size) + { + return (position.z - origin.z) * size.y * size.x + + (position.y - origin.y) * size.x + + (position.x - origin.x); + } + + private void PickCell(Vector3Int position, Vector3Int brushPosition, Tilemap tilemap) + { + if (tilemap != null) + { + SetTile(brushPosition, tilemap.GetTile(position)); + SetMatrix(brushPosition, tilemap.GetTransformMatrix(position)); + SetColor(brushPosition, tilemap.GetColor(position)); + } + } + } + + /// <summary> + /// The Brush Editor for a Group Brush. + /// </summary> + [CustomEditor(typeof(GroupBrush))] + public class GroupBrushEditor : GridBrushEditor + { + } +} \ No newline at end of file diff --git a/MrBigsock/Packages/com.unity.2d.tilemap.extras/Editor/Brushes/GroupBrush/GroupBrush.cs.meta b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Editor/Brushes/GroupBrush/GroupBrush.cs.meta new file mode 100644 index 0000000000000000000000000000000000000000..7b72c1a6a344a44c8d854bc0282d34ef0447564c --- /dev/null +++ b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Editor/Brushes/GroupBrush/GroupBrush.cs.meta @@ -0,0 +1,11 @@ +fileFormatVersion: 2 +guid: eec4c0c21e439e04083398ccd1928db0 +MonoImporter: + externalObjects: {} + serializedVersion: 2 + defaultReferences: [] + executionOrder: 0 + icon: {instanceID: 0} + userData: + assetBundleName: + assetBundleVariant: diff --git a/MrBigsock/Packages/com.unity.2d.tilemap.extras/Editor/Brushes/LineBrush.meta b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Editor/Brushes/LineBrush.meta new file mode 100644 index 0000000000000000000000000000000000000000..40d0e15ce972ae70cec84e55858365dc18192bb9 --- /dev/null +++ b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Editor/Brushes/LineBrush.meta @@ -0,0 +1,8 @@ +fileFormatVersion: 2 +guid: aebb69cf5dff0af40889258ae645be7a +folderAsset: yes +DefaultImporter: + externalObjects: {} + userData: + assetBundleName: + assetBundleVariant: diff --git a/MrBigsock/Packages/com.unity.2d.tilemap.extras/Editor/Brushes/LineBrush/LineBrush.cs b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Editor/Brushes/LineBrush/LineBrush.cs new file mode 100644 index 0000000000000000000000000000000000000000..577f3a2611140bcf26f63ddf364958bb3bd9ef56 --- /dev/null +++ b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Editor/Brushes/LineBrush/LineBrush.cs @@ -0,0 +1,296 @@ +using System; +using System.Collections.Generic; +using UnityEngine; +using UnityEngine.Tilemaps; +using System.Linq; + +namespace UnityEditor.Tilemaps +{ + /// <summary> + /// This Brush helps draw lines of Tiles onto a Tilemap. + /// Use this as an example to modify brush painting behaviour to making painting quicker with less actions. + /// </summary> + [HelpURL("https://docs.unity3d.com/Packages/com.unity.2d.tilemap.extras@latest/index.html?subfolder=/manual/LineBrush.html")] + [CustomGridBrush(true, false, false, "Line Brush")] + public class LineBrush : GridBrush + { + /// <summary> + /// Whether the Line Brush has started drawing a line. + /// </summary> + public bool lineStartActive; + /// <summary> + /// Ensures that there are orthogonal connections of Tiles from the start of the line to the end. + /// </summary> + public bool fillGaps; + /// <summary> + /// The current starting point of the line. + /// </summary> + public Vector3Int lineStart = Vector3Int.zero; + + /// <summary> + /// Indicates whether the brush is currently + /// moving something using the "Move selection with active brush" tool. + /// </summary> + public bool IsMoving { get; private set; } + + /// <summary> + /// Paints tiles and GameObjects into a given position within the selected layers. + /// The LineBrush overrides this to provide line painting functionality. + /// The first paint action sets the starting point of the line. + /// The next paint action sets the ending point of the line and paints Tile from start to end. + /// </summary> + /// <param name="grid">Grid used for layout.</param> + /// <param name="brushTarget">Target of the paint operation. By default the currently selected GameObject.</param> + /// <param name="position">The coordinates of the cell to paint data to.</param> + public override void Paint(GridLayout grid, GameObject brushTarget, Vector3Int position) + { + if (lineStartActive) + { + Vector2Int startPos = new Vector2Int(lineStart.x, lineStart.y); + Vector2Int endPos = new Vector2Int(position.x, position.y); + if (startPos == endPos) + base.Paint(grid, brushTarget, position); + else + { + foreach (var point in GetPointsOnLine(startPos, endPos, fillGaps)) + { + Vector3Int paintPos = new Vector3Int(point.x, point.y, position.z); + base.Paint(grid, brushTarget, paintPos); + } + } + lineStartActive = false; + } + else if (IsMoving) + { + base.Paint(grid, brushTarget, position); + } + else + { + lineStart = position; + lineStartActive = true; + } + } + + /// <summary> + /// Starts the movement of tiles and GameObjects from a given position within the selected layers. + /// </summary> + /// <param name="grid">Grid used for layout.</param> + /// <param name="brushTarget">Target of the Move operation. By default the currently selected GameObject.</param> + /// <param name="position">The coordinates of the cell to move data from.</param> + public override void MoveStart(GridLayout grid, GameObject brushTarget, BoundsInt position) + { + base.MoveStart(grid, brushTarget, position); + IsMoving = true; + } + + /// <summary> + /// Ends the movement of tiles and GameObjects to a given position within the selected layers. + /// </summary> + /// <param name="grid">Grid used for layout.</param> + /// <param name="brushTarget">Target of the Move operation. By default the currently selected GameObject.</param> + /// <param name="position">The coordinates of the cell to move data to.</param> + public override void MoveEnd(GridLayout grid, GameObject brushTarget, BoundsInt position) + { + base.MoveEnd(grid, brushTarget, position); + IsMoving = false; + } + + /// <summary> + /// Enumerates all the points between the start and end position which are + /// linked diagonally or orthogonally. + /// </summary> + /// <param name="startPos">Start position of the line.</param> + /// <param name="endPos">End position of the line.</param> + /// <param name="fillGaps">Fills any gaps between the start and end position so that + /// all points are linked only orthogonally.</param> + /// <returns>Returns an IEnumerable which enumerates all the points between the start and end position which are + /// linked diagonally or orthogonally.</returns> + public static IEnumerable<Vector2Int> GetPointsOnLine(Vector2Int startPos, Vector2Int endPos, bool fillGaps) + { + var points = GetPointsOnLine(startPos, endPos); + if (fillGaps) + { + var rise = endPos.y - startPos.y; + var run = endPos.x - startPos.x; + + if (rise != 0 || run != 0) + { + var extraStart = startPos; + var extraEnd = endPos; + + + if (Mathf.Abs(rise) >= Mathf.Abs(run)) + { + // up + if (rise > 0) + { + extraStart.y += 1; + extraEnd.y += 1; + } + // down + else // rise < 0 + { + + extraStart.y -= 1; + extraEnd.y -= 1; + } + } + else // Mathf.Abs(rise) < Mathf.Abs(run) + { + + // right + if (run > 0) + { + extraStart.x += 1; + extraEnd.x += 1; + } + // left + else // run < 0 + { + extraStart.x -= 1; + extraEnd.x -= 1; + } + } + + var extraPoints = GetPointsOnLine(extraStart, extraEnd); + extraPoints = extraPoints.Except(new[] { extraEnd }); + points = points.Union(extraPoints); + } + + } + + return points; + } + + /// <summary> + /// Gets an enumerable for all the cells directly between two points + /// http://ericw.ca/notes/bresenhams-line-algorithm-in-csharp.html + /// </summary> + /// <param name="p1">A starting point of a line</param> + /// <param name="p2">An ending point of a line</param> + /// <returns>Gets an enumerable for all the cells directly between two points</returns> + public static IEnumerable<Vector2Int> GetPointsOnLine(Vector2Int p1, Vector2Int p2) + { + int x0 = p1.x; + int y0 = p1.y; + int x1 = p2.x; + int y1 = p2.y; + + bool steep = Math.Abs(y1 - y0) > Math.Abs(x1 - x0); + if (steep) + { + int t; + t = x0; // swap x0 and y0 + x0 = y0; + y0 = t; + t = x1; // swap x1 and y1 + x1 = y1; + y1 = t; + } + if (x0 > x1) + { + int t; + t = x0; // swap x0 and x1 + x0 = x1; + x1 = t; + t = y0; // swap y0 and y1 + y0 = y1; + y1 = t; + } + int dx = x1 - x0; + int dy = Math.Abs(y1 - y0); + int error = dx / 2; + int ystep = (y0 < y1) ? 1 : -1; + int y = y0; + for (int x = x0; x <= x1; x++) + { + yield return new Vector2Int((steep ? y : x), (steep ? x : y)); + error = error - dy; + if (error < 0) + { + y += ystep; + error += dx; + } + } + yield break; + } + } + + /// <summary> + /// The Brush Editor for a Line Brush. + /// </summary> + [CustomEditor(typeof(LineBrush))] + public class LineBrushEditor : GridBrushEditor + { + private LineBrush lineBrush { get { return target as LineBrush; } } + private Tilemap lastTilemap; + + /// <summary> + /// Callback for painting the GUI for the GridBrush in the Scene View. + /// The CoordinateBrush Editor overrides this to draw the preview of the brush when drawing lines. + /// </summary> + /// <param name="grid">Grid that the brush is being used on.</param> + /// <param name="brushTarget">Target of the GridBrushBase::ref::Tool operation. By default the currently selected GameObject.</param> + /// <param name="position">Current selected location of the brush.</param> + /// <param name="tool">Current GridBrushBase::ref::Tool selected.</param> + /// <param name="executing">Whether brush is being used.</param> + public override void OnPaintSceneGUI(GridLayout grid, GameObject brushTarget, BoundsInt position, GridBrushBase.Tool tool, bool executing) + { + base.OnPaintSceneGUI(grid, brushTarget, position, tool, executing); + if (lineBrush.lineStartActive && brushTarget != null) + { + Tilemap tilemap = brushTarget.GetComponent<Tilemap>(); + if (tilemap != null) + { + tilemap.ClearAllEditorPreviewTiles(); + lastTilemap = tilemap; + } + + // Draw preview tiles for tilemap + Vector2Int startPos = new Vector2Int(lineBrush.lineStart.x, lineBrush.lineStart.y); + Vector2Int endPos = new Vector2Int(position.x, position.y); + if (startPos == endPos) + PaintPreview(grid, brushTarget, position.min); + else + { + foreach (var point in LineBrush.GetPointsOnLine(startPos, endPos, lineBrush.fillGaps)) + { + Vector3Int paintPos = new Vector3Int(point.x, point.y, position.z); + PaintPreview(grid, brushTarget, paintPos); + } + } + + if (Event.current.type == EventType.Repaint) + { + var min = lineBrush.lineStart; + var max = lineBrush.lineStart + position.size; + + // Draws a box on the picked starting position + GL.PushMatrix(); + GL.MultMatrix(GUI.matrix); + GL.Begin(GL.LINES); + Handles.color = Color.blue; + Handles.DrawLine(new Vector3(min.x, min.y, min.z), new Vector3(max.x, min.y, min.z)); + Handles.DrawLine(new Vector3(max.x, min.y, min.z), new Vector3(max.x, max.y, min.z)); + Handles.DrawLine(new Vector3(max.x, max.y, min.z), new Vector3(min.x, max.y, min.z)); + Handles.DrawLine(new Vector3(min.x, max.y, min.z), new Vector3(min.x, min.y, min.z)); + GL.End(); + GL.PopMatrix(); + } + } + } + + /// <summary> + /// Clears all line previews. + /// </summary> + public override void ClearPreview() + { + base.ClearPreview(); + if (lastTilemap != null) + { + lastTilemap.ClearAllEditorPreviewTiles(); + lastTilemap = null; + } + } + } +} diff --git a/MrBigsock/Packages/com.unity.2d.tilemap.extras/Editor/Brushes/LineBrush/LineBrush.cs.meta b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Editor/Brushes/LineBrush/LineBrush.cs.meta new file mode 100644 index 0000000000000000000000000000000000000000..40513695e3d5419c3d4d3d8e42339fe5db8c6b29 --- /dev/null +++ b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Editor/Brushes/LineBrush/LineBrush.cs.meta @@ -0,0 +1,11 @@ +fileFormatVersion: 2 +guid: 6210598a979f8724a8dac4531c428889 +MonoImporter: + externalObjects: {} + serializedVersion: 2 + defaultReferences: [] + executionOrder: 0 + icon: {instanceID: 0} + userData: + assetBundleName: + assetBundleVariant: diff --git a/MrBigsock/Packages/com.unity.2d.tilemap.extras/Editor/Brushes/PrefabBrushes.meta b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Editor/Brushes/PrefabBrushes.meta new file mode 100644 index 0000000000000000000000000000000000000000..076a2f2cbf11a87cb2d4f1e959772ab6b596b7ab --- /dev/null +++ b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Editor/Brushes/PrefabBrushes.meta @@ -0,0 +1,8 @@ +fileFormatVersion: 2 +guid: a616f75a0128f4349bfbedb608a30c03 +folderAsset: yes +DefaultImporter: + externalObjects: {} + userData: + assetBundleName: + assetBundleVariant: diff --git a/MrBigsock/Packages/com.unity.2d.tilemap.extras/Editor/Brushes/PrefabBrushes/BasePrefabBrush.cs b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Editor/Brushes/PrefabBrushes/BasePrefabBrush.cs new file mode 100644 index 0000000000000000000000000000000000000000..697ebfc1b1b746679b6654ba23e3781ac1578af4 --- /dev/null +++ b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Editor/Brushes/PrefabBrushes/BasePrefabBrush.cs @@ -0,0 +1,96 @@ +using System.Collections.Generic; +using UnityEngine; + +namespace UnityEditor.Tilemaps +{ + /// <summary> + /// This base class for PrefabBrushes that contains common functionality + /// </summary> + public class BasePrefabBrush : GridBrush + { /// <summary> + /// Anchor Point of the Instantiated Prefab in the cell when painting + /// </summary> + public Vector3 m_Anchor = new Vector3(0.5f, 0.5f, 0.5f); + + /// <summary> + /// Gets all children of the parent Transform which are within the given Grid's cell position. + /// </summary> + /// <param name="grid">Grid to determine cell position.</param> + /// <param name="parent">Parent transform to get child Objects from.</param> + /// <param name="position">Cell position to get Objects from.</param> + /// <returns>A list of GameObjects within the given Grid's cell position.</returns> + protected List<GameObject> GetObjectsInCell(GridLayout grid, Transform parent, Vector3Int position) + { + var results = new List<GameObject>(); + var childCount = parent.childCount; + var anchorCellOffset = Vector3Int.FloorToInt(m_Anchor); + var cellSize = grid.cellSize; + anchorCellOffset.x = cellSize.x == 0 ? 0 : anchorCellOffset.x; + anchorCellOffset.y = cellSize.y == 0 ? 0 : anchorCellOffset.y; + anchorCellOffset.z = cellSize.z == 0 ? 0 : anchorCellOffset.z; + for (var i = 0; i < childCount; i++) + { + var child = parent.GetChild(i); + if (position == grid.WorldToCell(child.position) - anchorCellOffset) + { + results.Add(child.gameObject); + } + } + return results; + } + + /// <summary> + /// Instantiates a Prefab into the given Grid's cell position parented to the brush target. + /// </summary> + /// <param name="grid">Grid to determine cell position.</param> + /// <param name="brushTarget">Target to instantiate child to.</param> + /// <param name="position">Cell position to instantiate to.</param> + /// <param name="prefab">Prefab to instantiate.</param> + protected void InstantiatePrefabInCell(GridLayout grid, GameObject brushTarget, Vector3Int position, GameObject prefab, Quaternion rotation = default) + { + var instance = (GameObject)PrefabUtility.InstantiatePrefab(prefab); + if (instance != null) + { + Undo.MoveGameObjectToScene(instance, brushTarget.scene, "Paint Prefabs"); + Undo.RegisterCreatedObjectUndo((Object)instance, "Paint Prefabs"); + instance.transform.SetParent(brushTarget.transform); + instance.transform.position = grid.LocalToWorld(grid.CellToLocalInterpolated(position + m_Anchor)); + instance.transform.rotation = rotation; + } + } + } + + /// <summary> + /// The Base Brush Editor for a Prefab Brush. + /// </summary> + public class BasePrefabBrushEditor : GridBrushEditor + { + private SerializedProperty m_Anchor; + + /// <summary> + /// SerializedObject representation of the target Prefab Brush + /// </summary> + protected SerializedObject m_SerializedObject; + + /// <summary> + /// OnEnable for the BasePrefabBrushEditor + /// </summary> + protected override void OnEnable() + { + base.OnEnable(); + m_SerializedObject = new SerializedObject(target); + m_Anchor = m_SerializedObject.FindProperty("m_Anchor"); + } + + /// <summary> + /// Callback for painting the inspector GUI for the PrefabBrush in the Tile Palette. + /// The PrefabBrush Editor overrides this to have a custom inspector for this Brush. + /// </summary> + public override void OnPaintInspectorGUI() + { + m_SerializedObject.UpdateIfRequiredOrScript(); + EditorGUILayout.PropertyField(m_Anchor); + m_SerializedObject.ApplyModifiedPropertiesWithoutUndo(); + } + } +} \ No newline at end of file diff --git a/MrBigsock/Packages/com.unity.2d.tilemap.extras/Editor/Brushes/PrefabBrushes/BasePrefabBrush.cs.meta b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Editor/Brushes/PrefabBrushes/BasePrefabBrush.cs.meta new file mode 100644 index 0000000000000000000000000000000000000000..5b0c8a0a14d87f0e9f29c67ea9b8156d3d08d65a --- /dev/null +++ b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Editor/Brushes/PrefabBrushes/BasePrefabBrush.cs.meta @@ -0,0 +1,3 @@ +fileFormatVersion: 2 +guid: 71e49d8cb93a4e46b07eea7c8dd2186b +timeCreated: 1584030887 \ No newline at end of file diff --git a/MrBigsock/Packages/com.unity.2d.tilemap.extras/Editor/Brushes/PrefabBrushes/BrushMenuItem.cs b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Editor/Brushes/PrefabBrushes/BrushMenuItem.cs new file mode 100644 index 0000000000000000000000000000000000000000..f40862cddcdba357c3e0e4799a5af8bd391f6aee --- /dev/null +++ b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Editor/Brushes/PrefabBrushes/BrushMenuItem.cs @@ -0,0 +1,27 @@ +using UnityEngine; + +namespace UnityEditor.Tilemaps +{ + static internal partial class AssetCreation + { + [MenuItem("Assets/Create/2D/Brushes/Prefab Brush", priority = (int) EBrushMenuItemOrder.PrefabBrush)] + static void CreatePrefabBrush() + { + ProjectWindowUtil.CreateAsset(ScriptableObject.CreateInstance<PrefabBrush>(), "New Prefab Brush.asset"); + } + + [MenuItem("Assets/Create/2D/Brushes/Prefab Random Brush", + priority = (int) EBrushMenuItemOrder.PrefabRandomBrush)] + static void CreatePrefabRandomBrush() + { + ProjectWindowUtil.CreateAsset(ScriptableObject.CreateInstance<PrefabRandomBrush>(), + "New Prefab Random Brush.asset"); + } + + [MenuItem("Assets/Create/2D/Brushes/Random Brush", priority = (int) EBrushMenuItemOrder.RandomBrush)] + static void CreateRandomBrush() + { + ProjectWindowUtil.CreateAsset(ScriptableObject.CreateInstance<RandomBrush>(), "New Random Brush.asset"); + } + } +} \ No newline at end of file diff --git a/MrBigsock/Packages/com.unity.2d.tilemap.extras/Editor/Brushes/PrefabBrushes/BrushMenuItem.cs.meta b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Editor/Brushes/PrefabBrushes/BrushMenuItem.cs.meta new file mode 100644 index 0000000000000000000000000000000000000000..9e48b3b6b7e22c9795245c54a28f2501d4d9180d --- /dev/null +++ b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Editor/Brushes/PrefabBrushes/BrushMenuItem.cs.meta @@ -0,0 +1,11 @@ +fileFormatVersion: 2 +guid: 083cc01539b3849bea2ad657b333981d +MonoImporter: + externalObjects: {} + serializedVersion: 2 + defaultReferences: [] + executionOrder: 0 + icon: {instanceID: 0} + userData: + assetBundleName: + assetBundleVariant: diff --git a/MrBigsock/Packages/com.unity.2d.tilemap.extras/Editor/Brushes/PrefabBrushes/PrefabBrush.meta b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Editor/Brushes/PrefabBrushes/PrefabBrush.meta new file mode 100644 index 0000000000000000000000000000000000000000..717837dea9b40eb08cec845ce8638a2f2c84e8df --- /dev/null +++ b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Editor/Brushes/PrefabBrushes/PrefabBrush.meta @@ -0,0 +1,3 @@ +fileFormatVersion: 2 +guid: 2d6d2cdd834b42ac8b0666be436db917 +timeCreated: 1584030876 \ No newline at end of file diff --git a/MrBigsock/Packages/com.unity.2d.tilemap.extras/Editor/Brushes/PrefabBrushes/PrefabBrush/PrefabBrush.cs b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Editor/Brushes/PrefabBrushes/PrefabBrush/PrefabBrush.cs new file mode 100644 index 0000000000000000000000000000000000000000..0d1f9a3d44ff91be779d0eb2bde8617ec04ada6b --- /dev/null +++ b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Editor/Brushes/PrefabBrushes/PrefabBrush/PrefabBrush.cs @@ -0,0 +1,153 @@ +using System.Linq; +using UnityEngine; + +namespace UnityEditor.Tilemaps +{ + /// <summary> + /// This Brush instances and places a containing prefab onto the targeted location and parents the instanced object to the paint target. + /// </summary> + [CustomGridBrush(false, true, false, "Prefab Brush")] + public class PrefabBrush : BasePrefabBrush + { + #pragma warning disable 0649 + /// <summary> + /// The selection of Prefab to paint from + /// </summary> + [SerializeField] GameObject m_Prefab; + Quaternion m_Rotation = default; + + #pragma warning restore 0649 + + /// <summary> + /// If true, erases any GameObjects that are in a given position within the selected layers with Erasing. + /// Otherwise, erases only GameObjects that are created from owned Prefab in a given position within the selected layers with Erasing. + /// </summary> + bool m_EraseAnyObjects; + + /// <summary> + /// Rotates the brush in the given direction. + /// </summary> + /// <param name="direction">Direction to rotate by.</param> + /// <param name="layout">Cell Layout for rotating.</param> + public override void Rotate(RotationDirection direction, GridLayout.CellLayout layout) + { + var angle = layout == GridLayout.CellLayout.Hexagon ? 60f : 90f; + m_Rotation = Quaternion.Euler(0f, 0f, direction == RotationDirection.Clockwise ? m_Rotation.eulerAngles.z + angle : m_Rotation.eulerAngles.z - angle); + } + + /// <summary> + /// Paints GameObject from containing Prefab into a given position within the selected layers. + /// The PrefabBrush overrides this to provide Prefab painting functionality. + /// </summary> + /// <param name="grid">Grid used for layout.</param> + /// <param name="brushTarget">Target of the paint operation. By default the currently selected GameObject.</param> + /// <param name="position">The coordinates of the cell to paint data to.</param> + public override void Paint(GridLayout grid, GameObject brushTarget, Vector3Int position) + { + var objectsInCell = GetObjectsInCell(grid, brushTarget.transform, position); + var existPrefabObjectInCell = objectsInCell.Any(objectInCell => PrefabUtility.GetCorrespondingObjectFromSource(objectInCell) == m_Prefab); + + if (!existPrefabObjectInCell) + { + base.InstantiatePrefabInCell(grid, brushTarget, position, m_Prefab, m_Rotation); + } + } + + /// <summary> + /// Paints the PrefabBrush instance's prefab into all positions specified by the box fill tool. + /// </summary> + /// <param name="grid">Grid used for layout.</param> + /// <param name="brushTarget">Target of the box fill operation. By default the currently selected GameObject.</param> + /// <param name="bounds">The cooridnate boundries to fill.</param> + public override void BoxFill(GridLayout grid, GameObject brushTarget, BoundsInt bounds) + { + foreach(Vector3Int tilePosition in bounds.allPositionsWithin) + Paint(grid, brushTarget, tilePosition); + } + + public override void BoxErase(GridLayout grid, GameObject brushTarget, BoundsInt bounds) + { + foreach (Vector3Int tilePosition in bounds.allPositionsWithin) + Erase(grid, brushTarget, tilePosition); + } + + /// <summary> + /// If "Erase Any Objects" is true, erases any GameObjects that are in a given position within the selected layers. + /// If "Erase Any Objects" is false, erases only GameObjects that are created from owned Prefab in a given position within the selected layers. + /// The PrefabBrush overrides this to provide Prefab erasing functionality. + /// </summary> + /// <param name="grid">Grid used for layout.</param> + /// <param name="brushTarget">Target of the erase operation. By default the currently selected GameObject.</param> + /// <param name="position">The coordinates of the cell to erase data from.</param> + public override void Erase(GridLayout grid, GameObject brushTarget, Vector3Int position) + { + foreach (var objectInCell in GetObjectsInCell(grid, brushTarget.transform, position)) + { + if (m_EraseAnyObjects || PrefabUtility.GetCorrespondingObjectFromSource(objectInCell) == m_Prefab) + { + Undo.DestroyObjectImmediate(objectInCell); + } + } + } + + /// <summary> + /// Pick prefab from selected Tilemap, given the coordinates of the cells. + /// </summary> + public override void Pick(GridLayout gridLayout, GameObject brushTarget, BoundsInt position, Vector3Int pickStart) + { + if (brushTarget == null) + { + return; + } + foreach (var objectInCell in GetObjectsInCell(gridLayout, brushTarget.transform, position.position)) + { + if (objectInCell) + { + m_Prefab = PrefabUtility.GetCorrespondingObjectFromSource(objectInCell); + } + } + } + + /// <summary> + /// The Brush Editor for a Prefab Brush. + /// </summary> + [CustomEditor(typeof(PrefabBrush))] + public class PrefabBrushEditor : BasePrefabBrushEditor + { + private PrefabBrush prefabBrush => target as PrefabBrush; + private SerializedProperty m_Prefab; + + /// <summary> + /// OnEnable for the PrefabBrushEditor + /// </summary> + protected override void OnEnable() + { + base.OnEnable(); + m_Prefab = m_SerializedObject.FindProperty(nameof(m_Prefab)); + } + + /// <summary> + /// Callback for painting the inspector GUI for the PrefabBrush in the Tile Palette. + /// The PrefabBrush Editor overrides this to have a custom inspector for this Brush. + /// </summary> + public override void OnPaintInspectorGUI() + { + const string eraseAnyObjectsTooltip = + "If true, erases any GameObjects that are in a given position " + + "within the selected layers with Erasing. " + + "Otherwise, erases only GameObjects that are created " + + "from owned Prefab in a given position within the selected layers with Erasing."; + + base.OnPaintInspectorGUI(); + + m_SerializedObject.UpdateIfRequiredOrScript(); + EditorGUILayout.PropertyField(m_Prefab, true); + prefabBrush.m_EraseAnyObjects = EditorGUILayout.Toggle( + new GUIContent("Erase Any Objects", eraseAnyObjectsTooltip), + prefabBrush.m_EraseAnyObjects); + + m_SerializedObject.ApplyModifiedPropertiesWithoutUndo(); + } + } + } +} diff --git a/MrBigsock/Packages/com.unity.2d.tilemap.extras/Editor/Brushes/PrefabBrushes/PrefabBrush/PrefabBrush.cs.meta b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Editor/Brushes/PrefabBrushes/PrefabBrush/PrefabBrush.cs.meta new file mode 100644 index 0000000000000000000000000000000000000000..4b5ede0aee02f464eb398780b52d3b975b2794bc --- /dev/null +++ b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Editor/Brushes/PrefabBrushes/PrefabBrush/PrefabBrush.cs.meta @@ -0,0 +1,13 @@ +fileFormatVersion: 2 +guid: 2d5751a2c961df945a34295ccf5e576d +timeCreated: 1501681786 +licenseType: Pro +MonoImporter: + externalObjects: {} + serializedVersion: 2 + defaultReferences: [] + executionOrder: 0 + icon: {instanceID: 0} + userData: + assetBundleName: + assetBundleVariant: diff --git a/MrBigsock/Packages/com.unity.2d.tilemap.extras/Editor/Brushes/PrefabBrushes/PrefabRandomBrush.meta b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Editor/Brushes/PrefabBrushes/PrefabRandomBrush.meta new file mode 100644 index 0000000000000000000000000000000000000000..769a8495859205009e5a267cc81ba918613450e3 --- /dev/null +++ b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Editor/Brushes/PrefabBrushes/PrefabRandomBrush.meta @@ -0,0 +1,8 @@ +fileFormatVersion: 2 +guid: f8a67fc3786cfca4baa247e99c9c77ad +folderAsset: yes +DefaultImporter: + externalObjects: {} + userData: + assetBundleName: + assetBundleVariant: diff --git a/MrBigsock/Packages/com.unity.2d.tilemap.extras/Editor/Brushes/PrefabBrushes/PrefabRandomBrush/PrefabRandomBrush.cs b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Editor/Brushes/PrefabBrushes/PrefabRandomBrush/PrefabRandomBrush.cs new file mode 100644 index 0000000000000000000000000000000000000000..24391491d03c2b606f9d53c75d9c622aad484b50 --- /dev/null +++ b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Editor/Brushes/PrefabBrushes/PrefabRandomBrush/PrefabRandomBrush.cs @@ -0,0 +1,127 @@ +using System.Linq; +using UnityEngine; + +namespace UnityEditor.Tilemaps +{ + /// <summary> + /// This Brush instances and places a randomly selected Prefabs onto the targeted location and parents the instanced object to the paint target. Use this as an example to quickly place an assorted type of GameObjects onto structured locations. + /// </summary> + [CustomGridBrush(false, true, false, "Prefab Random Brush")] + public class PrefabRandomBrush : BasePrefabBrush + { + private const float k_PerlinOffset = 100000f; + + #pragma warning disable 0649 + /// <summary> + /// The selection of Prefabs to paint from + /// </summary> + [SerializeField] GameObject[] m_Prefabs; + + /// <summary> + /// Factor for distribution of choice of Prefabs to paint + /// </summary> + [SerializeField] float m_PerlinScale = 0.5f; + #pragma warning restore 0649 + + /// <summary> + /// If true, erases any GameObjects that are in a given position within the selected layers with Erasing. + /// Otherwise, erases only GameObjects that are created from owned Prefab in a given position within the selected layers with Erasing. + /// </summary> + bool m_EraseAnyObjects; + + /// <summary> + /// Paints GameObject from containg Prefabs with randomly into a given position within the selected layers. + /// The PrefabRandomBrush overrides this to provide Prefab painting functionality. + /// </summary> + /// <param name="grid">Grid used for layout.</param> + /// <param name="brushTarget">Target of the paint operation. By default the currently selected GameObject.</param> + /// <param name="position">The coordinates of the cell to paint data to.</param> + public override void Paint(GridLayout grid, GameObject brushTarget, Vector3Int position) + { + // Do not allow editing palettes + if (brushTarget == null || brushTarget.layer == 31) + { + return; + } + + var objectsInCell = GetObjectsInCell(grid, brushTarget.transform, position); + var existPrefabObjectInCell = objectsInCell.Any(objectInCell => + { + return m_Prefabs.Any(prefab => PrefabUtility.GetCorrespondingObjectFromSource(objectInCell) == prefab); + }); + + if (!existPrefabObjectInCell) + { + var index = Mathf.Clamp(Mathf.FloorToInt(GetPerlinValue(position, m_PerlinScale, k_PerlinOffset) * m_Prefabs.Length), 0, m_Prefabs.Length - 1); + var prefab = m_Prefabs[index]; + base.InstantiatePrefabInCell(grid, brushTarget, position, prefab); + } + } + + /// <summary> + /// If "Erase Any Objects" is true, erases any GameObjects that are in a given position within the selected layers. + /// If "Erase Any Objects" is false, erases only GameObjects that are created from owned Prefab in a given position within the selected layers. + /// The PrefabRandomBrush overrides this to provide Prefab erasing functionality. + /// </summary> + /// <param name="grid">Grid used for layout.</param> + /// <param name="brushTarget">Target of the erase operation. By default the currently selected GameObject.</param> + /// <param name="position">The coordinates of the cell to erase data from.</param> + public override void Erase(GridLayout grid, GameObject brushTarget, Vector3Int position) + { + if (brushTarget.layer == 31 || brushTarget.transform == null) + { + return; + } + + foreach (var objectInCell in GetObjectsInCell(grid, brushTarget.transform, position)) + { + foreach (var prefab in m_Prefabs) + { + if (objectInCell != null && (m_EraseAnyObjects || PrefabUtility.GetCorrespondingObjectFromSource(objectInCell) == prefab)) + { + Undo.DestroyObjectImmediate(objectInCell); + } + } + } + } + + private static float GetPerlinValue(Vector3Int position, float scale, float offset) + { + return Mathf.PerlinNoise((position.x + offset)*scale, (position.y + offset)*scale); + } + + /// <summary> + /// The Brush Editor for a Prefab Brush. + /// </summary> + [CustomEditor(typeof(PrefabRandomBrush))] + public class PrefabRandomBrushEditor : BasePrefabBrushEditor + { + private PrefabRandomBrush prefabRandomBrush => target as PrefabRandomBrush; + + private SerializedProperty m_Prefabs; + + /// <summary> + /// OnEnable for the PrefabRandomBrushEditor + /// </summary> + protected override void OnEnable() + { + base.OnEnable(); + m_Prefabs = m_SerializedObject.FindProperty("m_Prefabs"); + } + + /// <summary> + /// Callback for painting the inspector GUI for the PrefabBrush in the Tile Palette. + /// The PrefabBrush Editor overrides this to have a custom inspector for this Brush. + /// </summary> + public override void OnPaintInspectorGUI() + { + base.OnPaintInspectorGUI(); + m_SerializedObject.UpdateIfRequiredOrScript(); + prefabRandomBrush.m_PerlinScale = EditorGUILayout.Slider("Perlin Scale", prefabRandomBrush.m_PerlinScale, 0.001f, 0.999f); + EditorGUILayout.PropertyField(m_Prefabs, true); + prefabRandomBrush.m_EraseAnyObjects = EditorGUILayout.Toggle("Erase Any Objects", prefabRandomBrush.m_EraseAnyObjects); + m_SerializedObject.ApplyModifiedPropertiesWithoutUndo(); + } + } + } +} diff --git a/MrBigsock/Packages/com.unity.2d.tilemap.extras/Editor/Brushes/PrefabBrushes/PrefabRandomBrush/PrefabRandomBrush.cs.meta b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Editor/Brushes/PrefabBrushes/PrefabRandomBrush/PrefabRandomBrush.cs.meta new file mode 100644 index 0000000000000000000000000000000000000000..96642a820bacb2f835eb3dd6eaacbd8f484b0d4b --- /dev/null +++ b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Editor/Brushes/PrefabBrushes/PrefabRandomBrush/PrefabRandomBrush.cs.meta @@ -0,0 +1,13 @@ +fileFormatVersion: 2 +guid: fdc5e1eccedb29e4894bbfab99032590 +timeCreated: 1501681786 +licenseType: Pro +MonoImporter: + externalObjects: {} + serializedVersion: 2 + defaultReferences: [] + executionOrder: 0 + icon: {instanceID: 0} + userData: + assetBundleName: + assetBundleVariant: diff --git a/MrBigsock/Packages/com.unity.2d.tilemap.extras/Editor/Brushes/RandomBrush.meta b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Editor/Brushes/RandomBrush.meta new file mode 100644 index 0000000000000000000000000000000000000000..fb7b74605d9f79352e169338f02bb3ee2e1067ff --- /dev/null +++ b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Editor/Brushes/RandomBrush.meta @@ -0,0 +1,8 @@ +fileFormatVersion: 2 +guid: e4007e34ec02b614b894ce8e20b48644 +folderAsset: yes +DefaultImporter: + externalObjects: {} + userData: + assetBundleName: + assetBundleVariant: diff --git a/MrBigsock/Packages/com.unity.2d.tilemap.extras/Editor/Brushes/RandomBrush/RandomBrush.cs b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Editor/Brushes/RandomBrush/RandomBrush.cs new file mode 100644 index 0000000000000000000000000000000000000000..e16781993b4482b630fafed9104f97a80b86a9d0 --- /dev/null +++ b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Editor/Brushes/RandomBrush/RandomBrush.cs @@ -0,0 +1,306 @@ +using System; +using System.Collections; +using System.Collections.Generic; +using UnityEngine; +using UnityEngine.Tilemaps; + +namespace UnityEditor.Tilemaps +{ + /// <summary> + /// This Brush helps to place random Tiles onto a Tilemap. + /// Use this as an example to create brushes which store specific data per brush and to make brushes which randomize behaviour. + /// </summary> + [HelpURL("https://docs.unity3d.com/Packages/com.unity.2d.tilemap.extras@latest/index.html?subfolder=/manual/RandomBrush.html")] + [CustomGridBrush(false, false, false, "Random Brush")] + public class RandomBrush : GridBrush + { + internal struct SizeEnumerator : IEnumerator<Vector3Int> + { + private readonly Vector3Int _min, _max, _delta; + private Vector3Int _current; + + public SizeEnumerator(Vector3Int min, Vector3Int max, Vector3Int delta) + { + _min = _current = min; + _max = max; + _delta = delta; + Reset(); + } + + public SizeEnumerator GetEnumerator() + { + return this; + } + + public bool MoveNext() + { + if (_current.z >= _max.z) + return false; + + _current.x += _delta.x; + if (_current.x >= _max.x) + { + _current.x = _min.x; + _current.y += _delta.y; + if (_current.y >= _max.y) + { + _current.y = _min.y; + _current.z += _delta.z; + if (_current.z >= _max.z) + return false; + } + } + return true; + } + + public void Reset() + { + _current = _min; + _current.x -= _delta.x; + } + + public Vector3Int Current { get { return _current; } } + + object IEnumerator.Current { get { return Current; } } + + void IDisposable.Dispose() {} + } + + /// <summary> + /// A data structure for storing a set of Tiles used for randomization + /// </summary> + [Serializable] + public struct RandomTileSet + { + /// <summary> + /// A set of tiles to be painted as a set + /// </summary> + public TileBase[] randomTiles; + } + + /// <summary> + /// The size of a RandomTileSet + /// </summary> + public Vector3Int randomTileSetSize = Vector3Int.one; + + /// <summary> + /// An array of RandomTileSets to choose from when randomizing + /// </summary> + public RandomTileSet[] randomTileSets; + + /// <summary> + /// A flag to determine if picking will add new RandomTileSets + /// </summary> + public bool pickRandomTiles; + + /// <summary> + /// A flag to determine if picking will add to existing RandomTileSets + /// </summary> + public bool addToRandomTiles; + + /// <summary> + /// Paints RandomTileSets into a given position within the selected layers. + /// The RandomBrush overrides this to provide randomized painting functionality. + /// </summary> + /// <param name="grid">Grid used for layout.</param> + /// <param name="brushTarget">Target of the paint operation. By default the currently selected GameObject.</param> + /// <param name="position">The coordinates of the cell to paint data to.</param> + public override void Paint(GridLayout grid, GameObject brushTarget, Vector3Int position) + { + if (randomTileSets != null && randomTileSets.Length > 0) + { + if (brushTarget == null) + return; + + var tilemap = brushTarget.GetComponent<Tilemap>(); + if (tilemap == null) + return; + + Vector3Int min = position - pivot; + foreach (var startLocation in new SizeEnumerator(min, min + size, randomTileSetSize)) + { + var randomTileSet = randomTileSets[(int) (randomTileSets.Length * UnityEngine.Random.value)]; + var randomBounds = new BoundsInt(startLocation, randomTileSetSize); + tilemap.SetTilesBlock(randomBounds, randomTileSet.randomTiles); + } + } + else + { + base.Paint(grid, brushTarget, position); + } + } + + /// <summary> + /// Picks RandomTileSets given the coordinates of the cells. + /// The RandomBrush overrides this to provide picking functionality for RandomTileSets. + /// </summary> + /// <param name="gridLayout">Grid to pick data from.</param> + /// <param name="brushTarget">Target of the picking operation. By default the currently selected GameObject.</param> + /// <param name="bounds">The coordinates of the cells to paint data from.</param> + /// <param name="pickStart">Pivot of the picking brush.</param> + public override void Pick(GridLayout gridLayout, GameObject brushTarget, BoundsInt bounds, Vector3Int pickStart) + { + base.Pick(gridLayout, brushTarget, bounds, pickStart); + if (!pickRandomTiles) + return; + + Tilemap tilemap = brushTarget.GetComponent<Tilemap>(); + if (tilemap == null) + return; + + int i = 0; + int count = ((bounds.size.x + randomTileSetSize.x - 1) / randomTileSetSize.x) + * ((bounds.size.y + randomTileSetSize.y - 1) / randomTileSetSize.y) + * ((bounds.size.z + randomTileSetSize.z - 1) / randomTileSetSize.z); + if (addToRandomTiles) + { + i = randomTileSets != null ? randomTileSets.Length : 0; + count += i; + } + Array.Resize(ref randomTileSets, count); + + foreach (var startLocation in new SizeEnumerator(bounds.min, bounds.max, randomTileSetSize)) + { + randomTileSets[i].randomTiles = new TileBase[randomTileSetSize.x * randomTileSetSize.y * randomTileSetSize.z]; + var randomBounds = new BoundsInt(startLocation, randomTileSetSize); + int j = 0; + foreach (Vector3Int pos in randomBounds.allPositionsWithin) + { + var tile = (pos.x < bounds.max.x && pos.y < bounds.max.y && pos.z < bounds.max.z) + ? tilemap.GetTile(pos) + : null; + randomTileSets[i].randomTiles[j++] = tile; + } + i++; + } + } + } + + /// <summary> + /// The Brush Editor for a Random Brush. + /// </summary> + [CustomEditor(typeof(RandomBrush))] + public class RandomBrushEditor : GridBrushEditor + { + private RandomBrush randomBrush { get { return target as RandomBrush; } } + private GameObject lastBrushTarget; + + /// <summary> + /// Paints preview data into a cell of a grid given the coordinates of the cell. + /// The RandomBrush Editor overrides this to draw the preview of the brush for RandomTileSets + /// </summary> + /// <param name="grid">Grid to paint data to.</param> + /// <param name="brushTarget">Target of the paint operation. By default the currently selected GameObject.</param> + /// <param name="position">The coordinates of the cell to paint data to.</param> + public override void PaintPreview(GridLayout grid, GameObject brushTarget, Vector3Int position) + { + if (randomBrush.randomTileSets != null && randomBrush.randomTileSets.Length > 0) + { + base.PaintPreview(grid, null, position); + if (brushTarget == null) + return; + + var tilemap = brushTarget.GetComponent<Tilemap>(); + if (tilemap == null) + return; + + Vector3Int min = position - randomBrush.pivot; + foreach (var startLocation in new RandomBrush.SizeEnumerator(min, min + randomBrush.size, randomBrush.randomTileSetSize)) + { + var randomTileSet = randomBrush.randomTileSets[(int) (randomBrush.randomTileSets.Length * UnityEngine.Random.value)]; + var randomBounds = new BoundsInt(startLocation, randomBrush.randomTileSetSize); + int j = 0; + foreach (Vector3Int pos in randomBounds.allPositionsWithin) + { + tilemap.SetEditorPreviewTile(pos, randomTileSet.randomTiles[j++]); + } + } + lastBrushTarget = brushTarget; + } + else + { + base.PaintPreview(grid, brushTarget, position); + } + } + + /// <summary> + /// Clears all RandomTileSet previews. + /// </summary> + public override void ClearPreview() + { + if (lastBrushTarget != null) + { + var tilemap = lastBrushTarget.GetComponent<Tilemap>(); + if (tilemap == null) + return; + + tilemap.ClearAllEditorPreviewTiles(); + + lastBrushTarget = null; + } + else + { + base.ClearPreview(); + } + } + + /// <summary> + /// Callback for painting the inspector GUI for the RandomBrush in the Tile Palette. + /// The RandomBrush Editor overrides this to have a custom inspector for this Brush. + /// </summary> + public override void OnPaintInspectorGUI() + { + EditorGUI.BeginChangeCheck(); + randomBrush.pickRandomTiles = EditorGUILayout.Toggle("Pick Random Tiles", randomBrush.pickRandomTiles); + using (new EditorGUI.DisabledScope(!randomBrush.pickRandomTiles)) + { + randomBrush.addToRandomTiles = EditorGUILayout.Toggle("Add To Random Tiles", randomBrush.addToRandomTiles); + } + + EditorGUI.BeginChangeCheck(); + randomBrush.randomTileSetSize = EditorGUILayout.Vector3IntField("Tile Set Size", randomBrush.randomTileSetSize); + if (EditorGUI.EndChangeCheck()) + { + for (int i = 0; i < randomBrush.randomTileSets.Length; ++i) + { + int sizeCount = randomBrush.randomTileSetSize.x * randomBrush.randomTileSetSize.y * + randomBrush.randomTileSetSize.z; + randomBrush.randomTileSets[i].randomTiles = new TileBase[sizeCount]; + } + } + int randomTileSetCount = EditorGUILayout.DelayedIntField("Number of Tiles", randomBrush.randomTileSets != null ? randomBrush.randomTileSets.Length : 0); + if (randomTileSetCount < 0) + randomTileSetCount = 0; + if (randomBrush.randomTileSets == null || randomBrush.randomTileSets.Length != randomTileSetCount) + { + Array.Resize(ref randomBrush.randomTileSets, randomTileSetCount); + for (int i = 0; i < randomBrush.randomTileSets.Length; ++i) + { + int sizeCount = randomBrush.randomTileSetSize.x * randomBrush.randomTileSetSize.y * + randomBrush.randomTileSetSize.z; + if (randomBrush.randomTileSets[i].randomTiles == null + || randomBrush.randomTileSets[i].randomTiles.Length != sizeCount) + randomBrush.randomTileSets[i].randomTiles = new TileBase[sizeCount]; + } + } + + if (randomTileSetCount > 0) + { + EditorGUILayout.Space(); + EditorGUILayout.LabelField("Place random tiles."); + + for (int i = 0; i < randomTileSetCount; i++) + { + EditorGUILayout.LabelField("Tile Set " + (i+1)); + for (int j = 0; j < randomBrush.randomTileSets[i].randomTiles.Length; ++j) + { + randomBrush.randomTileSets[i].randomTiles[j] = (TileBase) EditorGUILayout.ObjectField("Tile " + (j+1), randomBrush.randomTileSets[i].randomTiles[j], typeof(TileBase), false, null); + } + } + } + + if (EditorGUI.EndChangeCheck()) + EditorUtility.SetDirty(randomBrush); + } + } +} \ No newline at end of file diff --git a/MrBigsock/Packages/com.unity.2d.tilemap.extras/Editor/Brushes/RandomBrush/RandomBrush.cs.meta b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Editor/Brushes/RandomBrush/RandomBrush.cs.meta new file mode 100644 index 0000000000000000000000000000000000000000..0204289eebabcb91277b10b1cbc21d6955473eaa --- /dev/null +++ b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Editor/Brushes/RandomBrush/RandomBrush.cs.meta @@ -0,0 +1,13 @@ +fileFormatVersion: 2 +guid: d80edd6caba93514eb01722041fe50b4 +timeCreated: 1499223220 +licenseType: Pro +MonoImporter: + externalObjects: {} + serializedVersion: 2 + defaultReferences: [] + executionOrder: 0 + icon: {instanceID: 0} + userData: + assetBundleName: + assetBundleVariant: diff --git a/MrBigsock/Packages/com.unity.2d.tilemap.extras/Editor/Brushes/TintBrush.meta b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Editor/Brushes/TintBrush.meta new file mode 100644 index 0000000000000000000000000000000000000000..6c0ab25a6e6df10dd56a3c6381c4b731fab20f75 --- /dev/null +++ b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Editor/Brushes/TintBrush.meta @@ -0,0 +1,8 @@ +fileFormatVersion: 2 +guid: fa3049f8883fd3d418d8a008505b489e +folderAsset: yes +DefaultImporter: + externalObjects: {} + userData: + assetBundleName: + assetBundleVariant: diff --git a/MrBigsock/Packages/com.unity.2d.tilemap.extras/Editor/Brushes/TintBrush/TintBrush.cs b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Editor/Brushes/TintBrush/TintBrush.cs new file mode 100644 index 0000000000000000000000000000000000000000..d10add8e44c7facbf5c7ff28019fc489b6510d72 --- /dev/null +++ b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Editor/Brushes/TintBrush/TintBrush.cs @@ -0,0 +1,88 @@ +using System.Linq; +using UnityEngine; +using UnityEngine.Tilemaps; + +namespace UnityEditor.Tilemaps +{ + /// <summary> + /// This Brush changes the color of Tiles placed on a Tilemap. + /// </summary> + [CustomGridBrush(true, false, false, "Tint Brush")] + public class TintBrush : GridBrushBase + { + /// <summary> + /// Color of the Tile to tint + /// </summary> + public Color m_Color = Color.white; + + /// <summary> + /// Tints tiles into a given position within the selected layers. + /// The TintBrush overrides this to set the color of the Tile to tint it. + /// </summary> + /// <param name="grid">Grid used for layout.</param> + /// <param name="brushTarget">Target of the paint operation. By default the currently selected GameObject.</param> + /// <param name="position">The coordinates of the cell to paint data to.</param> + public override void Paint(GridLayout grid, GameObject brushTarget, Vector3Int position) + { + Tilemap tilemap = brushTarget.GetComponent<Tilemap>(); + if (tilemap != null) + { + SetColor(tilemap, position, m_Color); + } + } + + /// <summary> + /// Resets the color of the tiles in a given position within the selected layers to White. + /// The TintBrush overrides this to set the color of the Tile to White. + /// </summary> + /// <param name="grid">Grid used for layout.</param> + /// <param name="brushTarget">Target of the erase operation. By default the currently selected GameObject.</param> + /// <param name="position">The coordinates of the cell to erase data from.</param> + public override void Erase(GridLayout grid, GameObject brushTarget, Vector3Int position) + { + Tilemap tilemap = brushTarget.GetComponent<Tilemap>(); + if (tilemap != null) + { + SetColor(tilemap, position, Color.white); + } + } + + private static void SetColor(Tilemap tilemap, Vector3Int position, Color color) + { + TileBase tile = tilemap.GetTile(position); + if (tile != null) + { + if ((tilemap.GetTileFlags(position) & TileFlags.LockColor) != 0) + { + if (tile is Tile) + { + Debug.LogWarning("Tint brush cancelled, because Tile (" + tile.name + ") has TileFlags.LockColor set. Unlock it from the Tile asset debug inspector."); + } + else + { + Debug.LogWarning("Tint brush cancelled. because Tile (" + tile.name + ") has TileFlags.LockColor set. Unset it in GetTileData()."); + } + } + + tilemap.SetColor(position, color); + } + } + } + + /// <summary> + /// The Brush Editor for a Tint Brush. + /// </summary> + [CustomEditor(typeof(TintBrush))] + public class TintBrushEditor : GridBrushEditorBase + { + /// <summary>Returns all valid targets that the brush can edit.</summary> + /// <remarks>Valid targets for the TintBrush are any GameObjects with a Tilemap component.</remarks> + public override GameObject[] validTargets + { + get + { + return GameObject.FindObjectsOfType<Tilemap>().Select(x => x.gameObject).ToArray(); + } + } + } +} diff --git a/MrBigsock/Packages/com.unity.2d.tilemap.extras/Editor/Brushes/TintBrush/TintBrush.cs.meta b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Editor/Brushes/TintBrush/TintBrush.cs.meta new file mode 100644 index 0000000000000000000000000000000000000000..d4331e3038f7b0c59ee95fa7dec1dc1f23f854a2 --- /dev/null +++ b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Editor/Brushes/TintBrush/TintBrush.cs.meta @@ -0,0 +1,13 @@ +fileFormatVersion: 2 +guid: 5461c6f43c33cae4e8367042630017f7 +timeCreated: 1502200727 +licenseType: Pro +MonoImporter: + externalObjects: {} + serializedVersion: 2 + defaultReferences: [] + executionOrder: 0 + icon: {instanceID: 0} + userData: + assetBundleName: + assetBundleVariant: diff --git a/MrBigsock/Packages/com.unity.2d.tilemap.extras/Editor/Brushes/TintBrushSmooth.meta b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Editor/Brushes/TintBrushSmooth.meta new file mode 100644 index 0000000000000000000000000000000000000000..052de42251d4db004d339c9c385ea649998b2591 --- /dev/null +++ b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Editor/Brushes/TintBrushSmooth.meta @@ -0,0 +1,8 @@ +fileFormatVersion: 2 +guid: d170c661919d6654faecafa5625d9cd6 +folderAsset: yes +DefaultImporter: + externalObjects: {} + userData: + assetBundleName: + assetBundleVariant: diff --git a/MrBigsock/Packages/com.unity.2d.tilemap.extras/Editor/Brushes/TintBrushSmooth/TintBrushSmooth.cs b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Editor/Brushes/TintBrushSmooth/TintBrushSmooth.cs new file mode 100644 index 0000000000000000000000000000000000000000..294650d1c7fc60fdd7d2ad9110b217609d44fce9 --- /dev/null +++ b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Editor/Brushes/TintBrushSmooth/TintBrushSmooth.cs @@ -0,0 +1,119 @@ +using System.Linq; +using UnityEngine; +using UnityEngine.Tilemaps; + +namespace UnityEditor.Tilemaps +{ + /// <summary> + /// Advanced tint brush for interpolated tint color per-cell. Requires the use of custom shader (see TintedTilemap.shader) and helper component TileTextureGenerator. + /// </summary> + [CustomGridBrush(false, false, false, "Tint Brush (Smooth)")] + public class TintBrushSmooth : GridBrushBase + { + /// <summary> + /// Factor to blend the Color of Tile with its color and this Brush's color + /// </summary> + public float m_Blend = 1f; + /// <summary> + /// Color of the Tile to tint + /// </summary> + public Color m_Color = Color.white; + + /// <summary> + /// Tints tiles into a given position within the selected layers. + /// The TintBrushSmooth overrides this to set the color of the Grid position to tint it. + /// </summary> + /// <param name="grid">Grid used for layout.</param> + /// <param name="brushTarget">Target of the paint operation. By default the currently selected GameObject.</param> + /// <param name="position">The coordinates of the cell to paint data to.</param> + public override void Paint(GridLayout grid, GameObject brushTarget, Vector3Int position) + { + TintTextureGenerator generator = GetGenerator(grid); + if (generator != null) + { + var oldColor = generator.GetColor(grid as Grid, position); + var blendColor = oldColor * (1 - m_Blend) + m_Color * m_Blend; + generator.SetColor(grid as Grid, position, blendColor); + } + } + + /// <summary> + /// Resets the color of the tiles in a given position within the selected layers to White. + /// The TintBrushSmooth overrides this to set the color of the Grid position to White. + /// </summary> + /// <param name="grid">Grid used for layout.</param> + /// <param name="brushTarget">Target of the erase operation. By default the currently selected GameObject.</param> + /// <param name="position">The coordinates of the cell to erase data from.</param> + public override void Erase(GridLayout grid, GameObject brushTarget, Vector3Int position) + { + TintTextureGenerator generator = GetGenerator(grid); + if (generator != null) + { + generator.SetColor(grid as Grid, position, Color.white); + } + } + + /// <summary> + /// Picks the tint color given the coordinates of the cells. + /// The TintBrushSmoot overrides this to provide color picking functionality. + /// </summary> + /// <param name="grid">Grid to pick data from.</param> + /// <param name="brushTarget">Target of the picking operation. By default the currently selected GameObject.</param> + /// <param name="position">The coordinates of the cells to paint data from.</param> + /// <param name="pivot">Pivot of the picking brush.</param> + public override void Pick(GridLayout grid, GameObject brushTarget, BoundsInt position, Vector3Int pivot) + { + TintTextureGenerator generator = GetGenerator(grid); + if (generator != null) + { + m_Color = generator.GetColor(grid as Grid, position.min); + } + } + + private TintTextureGenerator GetGenerator(GridLayout grid) + { + TintTextureGenerator generator = FindObjectOfType<TintTextureGenerator>(); + if (generator == null) + { + if (grid != null) + { + generator = grid.gameObject.AddComponent<TintTextureGenerator>(); + } + } + return generator; + } + } + + /// <summary> + /// The Brush Editor for a Tint Brush Smooth. + /// </summary> + [CustomEditor(typeof(TintBrushSmooth))] + public class TintBrushSmoothEditor : GridBrushEditorBase + { + /// <summary> + /// The TintBrushSmooth for this Editor + /// </summary> + public TintBrushSmooth brush { get { return target as TintBrushSmooth; } } + + /// <summary>Returns all valid targets that the brush can edit.</summary> + /// <remarks>Valid targets for the TintBrushSmooth are any GameObjects with a Tilemap component.</remarks> + public override GameObject[] validTargets + { + get + { + return GameObject.FindObjectsOfType<Tilemap>().Select(x => x.gameObject).ToArray(); + } + } + + /// <summary> + /// Callback for painting the inspector GUI for the TintBrushSmooth in the Tile Palette. + /// The TintBrushSmooth Editor overrides this to have a custom inspector for this Brush. + /// </summary> + public override void OnPaintInspectorGUI() + { + brush.m_Color = EditorGUILayout.ColorField("Color", brush.m_Color); + brush.m_Blend = EditorGUILayout.Slider("Blend", brush.m_Blend, 0f, 1f); + GUILayout.Label("Note: Tilemap needs to use TintedTilemap.shader!"); + } + } +} diff --git a/MrBigsock/Packages/com.unity.2d.tilemap.extras/Editor/Brushes/TintBrushSmooth/TintBrushSmooth.cs.meta b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Editor/Brushes/TintBrushSmooth/TintBrushSmooth.cs.meta new file mode 100644 index 0000000000000000000000000000000000000000..8a19afbb464a198c8f6bdb5b7a355e25bb48870c --- /dev/null +++ b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Editor/Brushes/TintBrushSmooth/TintBrushSmooth.cs.meta @@ -0,0 +1,13 @@ +fileFormatVersion: 2 +guid: ed363ce3b4856fa408111529bc784318 +timeCreated: 1502800385 +licenseType: Pro +MonoImporter: + externalObjects: {} + serializedVersion: 2 + defaultReferences: [] + executionOrder: 0 + icon: {instanceID: 0} + userData: + assetBundleName: + assetBundleVariant: diff --git a/MrBigsock/Packages/com.unity.2d.tilemap.extras/Editor/Brushes/TintBrushSmooth/TintTextureGeneratorEditor.cs b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Editor/Brushes/TintBrushSmooth/TintTextureGeneratorEditor.cs new file mode 100644 index 0000000000000000000000000000000000000000..796ad66a42100015d2ef08109d84071e525c0902 --- /dev/null +++ b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Editor/Brushes/TintBrushSmooth/TintTextureGeneratorEditor.cs @@ -0,0 +1,34 @@ +using System; +using UnityEngine; + +namespace UnityEditor.Tilemaps +{ + [CustomEditor(typeof(TintTextureGenerator))] + public class TintTextureGeneratorEditor : Editor + { + private static class Styles + { + public static GUIContent tintMapSize = new GUIContent("Tint Map Size", "Size of the Tint Map in cells"); + public static GUIContent scaleFactor = new GUIContent("Scale Factor", "Mapping scale for cells to Tint map texture. Adjust to get better definition."); + } + + private const int k_TextureSizeLimit = 4096; + + private SerializedProperty m_TintMapSize; + private SerializedProperty m_ScaleFactor; + + public void OnEnable() + { + m_TintMapSize = serializedObject.FindProperty("k_TintMapSize"); + m_ScaleFactor = serializedObject.FindProperty("k_ScaleFactor"); + } + + public override void OnInspectorGUI() + { + serializedObject.Update(); + EditorGUILayout.IntSlider(m_TintMapSize, 256, k_TextureSizeLimit, Styles.tintMapSize); + EditorGUILayout.IntSlider(m_ScaleFactor, 1, Math.Min(8, Math.Max(1, k_TextureSizeLimit / m_TintMapSize.intValue)), Styles.scaleFactor); + serializedObject.ApplyModifiedProperties(); + } + } +} \ No newline at end of file diff --git a/MrBigsock/Packages/com.unity.2d.tilemap.extras/Editor/Brushes/TintBrushSmooth/TintTextureGeneratorEditor.cs.meta b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Editor/Brushes/TintBrushSmooth/TintTextureGeneratorEditor.cs.meta new file mode 100644 index 0000000000000000000000000000000000000000..39ca417c214fdac90cf1223d078301eec91bc8e0 --- /dev/null +++ b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Editor/Brushes/TintBrushSmooth/TintTextureGeneratorEditor.cs.meta @@ -0,0 +1,11 @@ +fileFormatVersion: 2 +guid: 86a60437712ccf741bcf537b0d1c9bfe +MonoImporter: + externalObjects: {} + serializedVersion: 2 + defaultReferences: [] + executionOrder: 0 + icon: {instanceID: 0} + userData: + assetBundleName: + assetBundleVariant: diff --git a/MrBigsock/Packages/com.unity.2d.tilemap.extras/Editor/Menu.meta b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Editor/Menu.meta new file mode 100644 index 0000000000000000000000000000000000000000..c0588b7231c2c3272ca900654a9e182e57d660af --- /dev/null +++ b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Editor/Menu.meta @@ -0,0 +1,8 @@ +fileFormatVersion: 2 +guid: 123abb5d8f7434106bb182008cbd5f07 +folderAsset: yes +DefaultImporter: + externalObjects: {} + userData: + assetBundleName: + assetBundleVariant: diff --git a/MrBigsock/Packages/com.unity.2d.tilemap.extras/Editor/Menu/CustomRuleTileMenu.cs b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Editor/Menu/CustomRuleTileMenu.cs new file mode 100644 index 0000000000000000000000000000000000000000..a57a60d942dc6d248c3a94b5e4c8ec27b89e288e --- /dev/null +++ b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Editor/Menu/CustomRuleTileMenu.cs @@ -0,0 +1,44 @@ +using System; +using System.IO; +using UnityEditor.Tilemaps; +using UnityEngine; + +namespace UnityEditor +{ + static class CustomRuleTileMenu + { + private static string tempCustomRuleTilePath; + private const string customRuleTileScript = +@"using System.Collections; +using System.Collections.Generic; +using UnityEngine; +using UnityEngine.Tilemaps; + +[CreateAssetMenu] +public class #SCRIPTNAME# : RuleTile<#SCRIPTNAME#.Neighbor> { + public bool customField; + + public class Neighbor : RuleTile.TilingRule.Neighbor { + public const int Null = 3; + public const int NotNull = 4; + } + + public override bool RuleMatch(int neighbor, TileBase tile) { + switch (neighbor) { + case Neighbor.Null: return tile == null; + case Neighbor.NotNull: return tile != null; + } + return base.RuleMatch(neighbor, tile); + } +}"; + + [MenuItem("Assets/Create/2D/Tiles/Custom Rule Tile Script", false, (int)ETilesMenuItemOrder.CustomRuleTile)] + static void CreateCustomRuleTile() + { + if (String.IsNullOrEmpty(tempCustomRuleTilePath) || !File.Exists(tempCustomRuleTilePath)) + tempCustomRuleTilePath = FileUtil.GetUniqueTempPathInProject(); + File.WriteAllText(tempCustomRuleTilePath, customRuleTileScript); + ProjectWindowUtil.CreateScriptAssetFromTemplateFile(tempCustomRuleTilePath, "NewCustomRuleTile.cs"); + } + } +} diff --git a/MrBigsock/Packages/com.unity.2d.tilemap.extras/Editor/Menu/CustomRuleTileMenu.cs.meta b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Editor/Menu/CustomRuleTileMenu.cs.meta new file mode 100644 index 0000000000000000000000000000000000000000..c939715141a3fa743dfaa67d14033abd2dd4802d --- /dev/null +++ b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Editor/Menu/CustomRuleTileMenu.cs.meta @@ -0,0 +1,11 @@ +fileFormatVersion: 2 +guid: 1a641ca7aea56f8428c8c6efc8e618cb +MonoImporter: + externalObjects: {} + serializedVersion: 2 + defaultReferences: [] + executionOrder: 0 + icon: {instanceID: 0} + userData: + assetBundleName: + assetBundleVariant: diff --git a/MrBigsock/Packages/com.unity.2d.tilemap.extras/Editor/Menu/MenuItemOrder.cs b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Editor/Menu/MenuItemOrder.cs new file mode 100644 index 0000000000000000000000000000000000000000..41dd3e47068d9e80bf58344a248416f43e4b1869 --- /dev/null +++ b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Editor/Menu/MenuItemOrder.cs @@ -0,0 +1,66 @@ +using UnityEngine; +using UnityEngine.Tilemaps; + +namespace UnityEditor.Tilemaps +{ + internal enum ETilesMenuItemOrder + { + AnimatedTile = 2, + RuleTile = 100, + IsometricRuleTile, + HexagonalRuleTile, + RuleOverrideTile, + AdvanceRuleOverrideTile, + CustomRuleTile, + RandomTile = 200, + WeightedRandomTile, + PipelineTile, + TerrainTile, + } + internal enum EBrushMenuItemOrder + { + RandomBrush = 3, + PrefabBrush, + PrefabRandomBrush + } + + static internal partial class AssetCreation + { + + [MenuItem("Assets/Create/2D/Tiles/Animated Tile", priority = (int) ETilesMenuItemOrder.AnimatedTile)] + static void CreateAnimatedTile() + { + ProjectWindowUtil.CreateAsset(ScriptableObject.CreateInstance<AnimatedTile>(), "New Animated Tile.asset"); + } + + [MenuItem( "Assets/Create/2D/Tiles/Hexagonal Rule Tile", priority = (int)ETilesMenuItemOrder.HexagonalRuleTile)] + static void CreateHexagonalRuleTile() + { + ProjectWindowUtil.CreateAsset(ScriptableObject.CreateInstance<HexagonalRuleTile>(), "New Hexagonal Rule Tile.asset"); + } + + [MenuItem("Assets/Create/2D/Tiles/Isometric Rule Tile", priority = (int)ETilesMenuItemOrder.IsometricRuleTile)] + static void CreateIsometricRuleTile() + { + ProjectWindowUtil.CreateAsset(ScriptableObject.CreateInstance<IsometricRuleTile>(), "New Isometric Rule Tile.asset"); + } + + [MenuItem("Assets/Create/2D/Tiles/Advanced Rule Override Tile", priority = (int)ETilesMenuItemOrder.AdvanceRuleOverrideTile)] + static void CreateAdvancedRuleOverrideTile() + { + ProjectWindowUtil.CreateAsset(ScriptableObject.CreateInstance<AdvancedRuleOverrideTile>(), "New Advanced Rule Override Tile.asset"); + } + + [MenuItem("Assets/Create/2D/Tiles/Rule Override Tile", priority = (int)ETilesMenuItemOrder.RuleOverrideTile)] + static void CreateRuleOverrideTile() + { + ProjectWindowUtil.CreateAsset(ScriptableObject.CreateInstance<RuleOverrideTile>(), "New Rule Override Tile.asset"); + } + + [MenuItem("Assets/Create/2D/Tiles/Rule Tile", priority = (int)ETilesMenuItemOrder.RuleTile)] + static void CreateRuleTile() + { + ProjectWindowUtil.CreateAsset(ScriptableObject.CreateInstance<RuleTile>(), "New Rule Tile.asset"); + } + } +} diff --git a/MrBigsock/Packages/com.unity.2d.tilemap.extras/Editor/Menu/MenuItemOrder.cs.meta b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Editor/Menu/MenuItemOrder.cs.meta new file mode 100644 index 0000000000000000000000000000000000000000..d4c30665914a2906be20fcb18fc263c3660fd939 --- /dev/null +++ b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Editor/Menu/MenuItemOrder.cs.meta @@ -0,0 +1,11 @@ +fileFormatVersion: 2 +guid: 0f11ba7bd032140bfbcd546d93db1548 +MonoImporter: + externalObjects: {} + serializedVersion: 2 + defaultReferences: [] + executionOrder: 0 + icon: {instanceID: 0} + userData: + assetBundleName: + assetBundleVariant: diff --git a/MrBigsock/Packages/com.unity.2d.tilemap.extras/Editor/Tiles.meta b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Editor/Tiles.meta new file mode 100644 index 0000000000000000000000000000000000000000..07b0e988518c5b3553e037ddb82fe24a8a1a17f5 --- /dev/null +++ b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Editor/Tiles.meta @@ -0,0 +1,8 @@ +fileFormatVersion: 2 +guid: f03b3016bcc582043b27ae775025ef88 +folderAsset: yes +DefaultImporter: + externalObjects: {} + userData: + assetBundleName: + assetBundleVariant: diff --git a/MrBigsock/Packages/com.unity.2d.tilemap.extras/Editor/Tiles/HexagonalRuleTile.meta b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Editor/Tiles/HexagonalRuleTile.meta new file mode 100644 index 0000000000000000000000000000000000000000..4cb01ac109aa8fb70f823a3feaa07eed077189f0 --- /dev/null +++ b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Editor/Tiles/HexagonalRuleTile.meta @@ -0,0 +1,8 @@ +fileFormatVersion: 2 +guid: 3b68386deea7c2246bd5fe7bd6fa13c6 +folderAsset: yes +DefaultImporter: + externalObjects: {} + userData: + assetBundleName: + assetBundleVariant: diff --git a/MrBigsock/Packages/com.unity.2d.tilemap.extras/Editor/Tiles/HexagonalRuleTile/HexagonalRuleTileEditor.cs b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Editor/Tiles/HexagonalRuleTile/HexagonalRuleTileEditor.cs new file mode 100644 index 0000000000000000000000000000000000000000..9a5eea9f93ad126dc6ccd1de3e81f4eebf3b70c9 --- /dev/null +++ b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Editor/Tiles/HexagonalRuleTile/HexagonalRuleTileEditor.cs @@ -0,0 +1,223 @@ +using UnityEngine; +using UnityEngine.Tilemaps; + +namespace UnityEditor +{ + /// <summary> + /// The Editor for a HexagonalRuleTile. + /// </summary> + [CustomEditor(typeof(HexagonalRuleTile), true)] + [CanEditMultipleObjects] + public class HexagonalRuleTileEditor : RuleTileEditor + { + /// <summary> + /// The HexagonalRuleTile being edited. + /// </summary> + public HexagonalRuleTile hexTile => target as HexagonalRuleTile; + + /// <summary> + /// Gets the index for a Rule with the HexagonalRuleTile to display an arrow. + /// </summary> + /// <param name="position">The adjacent position of the arrow.</param> + /// <returns>Returns the index for a Rule with the HexagonalRuleTile to display an arrow.</returns> + public override int GetArrowIndex(Vector3Int position) + { + if (position.y % 2 != 0) + { + position *= 2; + position.x += 1; + } + + if (position.x == 0) + { + if (position.y > 0) + return hexTile.m_FlatTop ? 5 : 1; + else + return hexTile.m_FlatTop ? 3 : 7; + } + else if (position.y == 0) + { + if (position.x > 0) + return hexTile.m_FlatTop ? 1 : 5; + else + return hexTile.m_FlatTop ? 7 : 3; + } + else + { + if (position.x < 0 && position.y > 0) + return hexTile.m_FlatTop ? 8 : 0; + else if (position.x > 0 && position.y > 0) + return hexTile.m_FlatTop ? 2 : 2; + else if (position.x < 0 && position.y < 0) + return hexTile.m_FlatTop ? 6 : 6; + else if (position.x > 0 && position.y < 0) + return hexTile.m_FlatTop ? 0 : 8; + } + + return -1; + } + + /// <summary> + /// Get the GUI bounds for a Rule. + /// </summary> + /// <param name="bounds">Cell bounds of the Rule.</param> + /// <param name="rule">Rule to get GUI bounds for.</param> + /// <returns>The GUI bounds for a rule.</returns> + public override BoundsInt GetRuleGUIBounds(BoundsInt bounds, RuleTile.TilingRule rule) + { + foreach (var n in rule.GetNeighbors()) + { + if (n.Key.x == bounds.xMax - 1 && n.Key.y % 2 != 0) + { + bounds.xMax++; + break; + } + } + return base.GetRuleGUIBounds(bounds, rule); + } + + /// <summary> + /// Gets the GUI matrix size for a Rule of a HexagonalRuleTile + /// </summary> + /// <param name="bounds">Cell bounds of the Rule.</param> + /// <returns>Returns the GUI matrix size for a Rule of a HexagonalRuleTile.</returns> + public override Vector2 GetMatrixSize(BoundsInt bounds) + { + Vector2 size = base.GetMatrixSize(bounds); + return hexTile.m_FlatTop ? new Vector2(size.y, size.x) : size; + } + + /// <summary> + /// Draws a Rule Matrix for the given Rule for a HexagonalRuleTile. + /// </summary> + /// <param name="tile">Tile to draw rule for.</param> + /// <param name="rect">GUI Rect to draw rule at.</param> + /// <param name="bounds">Cell bounds of the Rule.</param> + /// <param name="tilingRule">Rule to draw Rule Matrix for.</param> + public override void RuleMatrixOnGUI(RuleTile tile, Rect rect, BoundsInt bounds, RuleTile.TilingRule tilingRule) + { + bool flatTop = hexTile.m_FlatTop; + + Handles.color = EditorGUIUtility.isProSkin ? new Color(1f, 1f, 1f, 0.2f) : new Color(0f, 0f, 0f, 0.2f); + float w = rect.width / (flatTop ? bounds.size.y : bounds.size.x); + float h = rect.height / (flatTop ? bounds.size.x : bounds.size.y); + + // Grid + if (flatTop) + { + for (int y = 0; y <= bounds.size.y; y++) + { + float left = rect.xMin + y * w; + float offset = 0; + + if (y == 0 && bounds.yMax % 2 == 0) + offset = h / 2; + else if (y == bounds.size.y && bounds.yMin % 2 != 0) + offset = h / 2; + + Handles.DrawLine(new Vector3(left, rect.yMin + offset), new Vector3(left, rect.yMax - offset)); + + if (y < bounds.size.y) + { + bool noOffset = (y + bounds.yMax) % 2 != 0; + for (int x = 0; x < (noOffset ? (bounds.size.x + 1) : bounds.size.x); x++) + { + float top = rect.yMin + x * h + (noOffset ? 0 : h / 2); + Handles.DrawLine(new Vector3(left, top), new Vector3(left + w, top)); + } + } + } + } + else + { + for (int y = 0; y <= bounds.size.y; y++) + { + float top = rect.yMin + y * h; + float offset = 0; + + if (y == 0 && bounds.yMax % 2 == 0) + offset = w / 2; + else if (y == bounds.size.y && bounds.yMin % 2 != 0) + offset = w / 2; + + Handles.DrawLine(new Vector3(rect.xMin + offset, top), new Vector3(rect.xMax - offset, top)); + + if (y < bounds.size.y) + { + bool noOffset = (y + bounds.yMax) % 2 != 0; + for (int x = 0; x < (noOffset ? (bounds.size.x + 1) : bounds.size.x); x++) + { + float left = rect.xMin + x * w + (noOffset ? 0 : w / 2); + Handles.DrawLine(new Vector3(left, top), new Vector3(left, top + h)); + } + } + } + } + + var neighbors = tilingRule.GetNeighbors(); + + // Icons + Handles.color = Color.white; + for (int y = bounds.yMin; y < bounds.yMax; y++) + { + int xMax = y % 2 == 0 ? bounds.xMax : (bounds.xMax - 1); + for (int x = bounds.xMin; x < xMax; x++) + { + Vector3Int pos = new Vector3Int(x, y, 0); + Vector2 offset = new Vector2(x - bounds.xMin, -y + bounds.yMax - 1); + Rect r = flatTop ? new Rect(rect.xMax - offset.y * w - w, rect.yMax - offset.x * h - h, w - 1, h - 1) + : new Rect(rect.xMin + offset.x * w, rect.yMin + offset.y * h, w - 1, h - 1); + + if (y % 2 != 0) + { + if (flatTop) + r.y -= h / 2; + else + r.x += w / 2; + } + + RuleMatrixIconOnGUI(tilingRule, neighbors, pos, r); + } + } + } + + /// <summary> + /// Creates a Preview for the HexagonalRuleTile. + /// </summary> + protected override void CreatePreview() + { + base.CreatePreview(); + + m_PreviewGrid.cellLayout = GridLayout.CellLayout.Hexagon; + m_PreviewGrid.cellSize = new Vector3(0.8659766f, 1.0f, 1.0f); + m_PreviewGrid.cellSwizzle = hexTile.m_FlatTop ? GridLayout.CellSwizzle.YXZ : GridLayout.CellSwizzle.XYZ; + + foreach (var tilemap in m_PreviewTilemaps) + { + tilemap.tileAnchor = Vector3.zero; + tilemap.ClearAllTiles(); + } + + for (int x = -1; x <= 0; ++x) + for (int y = -1; y <= 1; ++y) + m_PreviewTilemaps[0].SetTile(new Vector3Int(x, y, 0), tile); + + m_PreviewTilemaps[1].SetTile(new Vector3Int(1, -1, 0), tile); + m_PreviewTilemaps[1].SetTile(new Vector3Int(2, 0, 0), tile); + m_PreviewTilemaps[1].SetTile(new Vector3Int(2, 1, 0), tile); + + for (int x = -1; x <= 1; x++) + m_PreviewTilemaps[2].SetTile(new Vector3Int(x, -2, 0), tile); + + m_PreviewTilemaps[3].SetTile(new Vector3Int(1, 1, 0), tile); + + foreach (var tilemapRenderer in m_PreviewTilemapRenderers) + tilemapRenderer.sortOrder = TilemapRenderer.SortOrder.TopRight; + + m_PreviewTilemapRenderers[0].sortingOrder = 0; + m_PreviewTilemapRenderers[1].sortingOrder = -1; + m_PreviewTilemapRenderers[2].sortingOrder = 1; + m_PreviewTilemapRenderers[3].sortingOrder = 0; + } + } +} diff --git a/MrBigsock/Packages/com.unity.2d.tilemap.extras/Editor/Tiles/HexagonalRuleTile/HexagonalRuleTileEditor.cs.meta b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Editor/Tiles/HexagonalRuleTile/HexagonalRuleTileEditor.cs.meta new file mode 100644 index 0000000000000000000000000000000000000000..27d86a7fb5c59293a67fa1f4a89aa82de8fb8b1c --- /dev/null +++ b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Editor/Tiles/HexagonalRuleTile/HexagonalRuleTileEditor.cs.meta @@ -0,0 +1,11 @@ +fileFormatVersion: 2 +guid: a573a189e85fcd243a32fa6ac742ca44 +MonoImporter: + externalObjects: {} + serializedVersion: 2 + defaultReferences: [] + executionOrder: 0 + icon: {instanceID: 0} + userData: + assetBundleName: + assetBundleVariant: diff --git a/MrBigsock/Packages/com.unity.2d.tilemap.extras/Editor/Tiles/IsometricRuleTile.meta b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Editor/Tiles/IsometricRuleTile.meta new file mode 100644 index 0000000000000000000000000000000000000000..e6f80a7d5d6dd3de2e6a71e17b7789abd2556af4 --- /dev/null +++ b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Editor/Tiles/IsometricRuleTile.meta @@ -0,0 +1,8 @@ +fileFormatVersion: 2 +guid: 8ee1b1583a594cf47baebcd4a5d46cb9 +folderAsset: yes +DefaultImporter: + externalObjects: {} + userData: + assetBundleName: + assetBundleVariant: diff --git a/MrBigsock/Packages/com.unity.2d.tilemap.extras/Editor/Tiles/IsometricRuleTile/IsometricRuleTileEditor.cs b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Editor/Tiles/IsometricRuleTile/IsometricRuleTileEditor.cs new file mode 100644 index 0000000000000000000000000000000000000000..ad6cf100401bb0cd11497788a4e7e0c804b6dcfa --- /dev/null +++ b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Editor/Tiles/IsometricRuleTile/IsometricRuleTileEditor.cs @@ -0,0 +1,150 @@ +using UnityEngine; +using UnityEngine.Tilemaps; + +namespace UnityEditor +{ + /// <summary> + /// The Editor for an IsometricRuleTile. + /// </summary> + [CustomEditor(typeof(IsometricRuleTile), true)] + [CanEditMultipleObjects] + public class IsometricRuleTileEditor : RuleTileEditor + { + /// <summary> + /// Gets the index for a Rule with the IsometricRuleTileEditor to display an arrow. + /// </summary> + /// <param name="position">The adjacent position of the arrow.</param> + /// <returns>Returns the index for a Rule with the IsometricRuleTileEditor to display an arrow</returns> + private static readonly int[] s_Arrows = { 3, 0, 1, 6, -1, 2, 7, 8, 5 }; + + /// <summary> + /// Gets the index for a Rule with the HexagonalRuleTile to display an arrow. + /// </summary> + /// <param name="position">The adjacent position of the arrow.</param> + /// <returns>Returns the index for a Rule with the HexagonalRuleTile to display an arrow.</returns> + public override int GetArrowIndex(Vector3Int position) + { + return s_Arrows[base.GetArrowIndex(position)]; + } + + /// <summary> + /// Gets the GUI matrix size for a Rule of a IsometricRuleTile + /// </summary> + /// <param name="bounds">Cell bounds of the Rule.</param> + /// <returns>Returns the GUI matrix size for a Rule of a IsometricRuleTile.</returns> + public override Vector2 GetMatrixSize(BoundsInt bounds) + { + float p = Mathf.Pow(2, 0.5f); + float w = (bounds.size.x / p + bounds.size.y / p) * k_SingleLineHeight; + return new Vector2(w, w); + } + + /// <summary> + /// Draws a Rule Matrix for the given Rule for a IsometricRuleTile. + /// </summary> + /// <param name="tile">Tile to draw rule for.</param> + /// <param name="rect">GUI Rect to draw rule at.</param> + /// <param name="bounds">Cell bounds of the Rule.</param> + /// <param name="tilingRule">Rule to draw Rule Matrix for.</param> + public override void RuleMatrixOnGUI(RuleTile tile, Rect rect, BoundsInt bounds, RuleTile.TilingRule tilingRule) + { + Handles.color = EditorGUIUtility.isProSkin ? new Color(1f, 1f, 1f, 0.2f) : new Color(0f, 0f, 0f, 0.2f); + float w = rect.width / bounds.size.x; + float h = rect.height / bounds.size.y; + + // Grid + float d = rect.width / (bounds.size.x + bounds.size.y); + for (int y = 0; y <= bounds.size.y; y++) + { + float left = rect.xMin + d * y; + float top = rect.yMin + d * y; + float right = rect.xMax - d * (bounds.size.y - y); + float bottom = rect.yMax - d * (bounds.size.y - y); + Handles.DrawLine(new Vector3(left, bottom), new Vector3(right, top)); + } + for (int x = 0; x <= bounds.size.x; x++) + { + float left = rect.xMin + d * x; + float top = rect.yMax - d * x; + float right = rect.xMax - d * (bounds.size.x - x); + float bottom = rect.yMin + d * (bounds.size.x - x); + Handles.DrawLine(new Vector3(left, bottom), new Vector3(right, top)); + } + Handles.color = Color.white; + + var neighbors = tilingRule.GetNeighbors(); + + // Icons + float iconSize = (rect.width - d) / (bounds.size.x + bounds.size.y - 1); + float iconScale = Mathf.Pow(2, 0.5f); + + for (int y = bounds.yMin; y < bounds.yMax; y++) + { + for (int x = bounds.xMin; x < bounds.xMax; x++) + { + Vector3Int pos = new Vector3Int(x, y, 0); + Vector3Int offset = new Vector3Int(pos.x - bounds.xMin, pos.y - bounds.yMin, 0); + Rect r = new Rect( + rect.xMin + rect.size.x - iconSize * (offset.y - offset.x + 0.5f + bounds.size.x), + rect.yMin + rect.size.y - iconSize * (offset.y + offset.x + 1.5f), + iconSize, iconSize + ); + Vector2 center = r.center; + r.size *= iconScale; + r.center = center; + + RuleMatrixIconOnGUI(tilingRule, neighbors, pos, r); + } + } + } + + /// <summary> + /// Determines the current mouse position is within the given Rect. + /// </summary> + /// <param name="rect">Rect to test mouse position for.</param> + /// <returns>True if the current mouse position is within the given Rect. False otherwise.</returns> + public override bool ContainsMousePosition(Rect rect) + { + var center = rect.center; + var halfWidth = rect.width / 2; + var halfHeight = rect.height / 2; + var mouseFromCenter = Event.current.mousePosition - center; + var xAbs = Mathf.Abs(Vector2.Dot(mouseFromCenter, Vector2.right)); + var yAbs = Mathf.Abs(Vector2.Dot(mouseFromCenter, Vector2.up)); + return (xAbs / halfWidth + yAbs / halfHeight) <= 1; + } + + /// <summary> + /// Updates preview settings for the IsometricRuleTile. + /// </summary> + public override void OnPreviewSettings() + { + base.OnPreviewSettings(); + + if (m_PreviewGrid) + { + float height = EditorGUILayout.FloatField("Cell Height", m_PreviewGrid.cellSize.y); + m_PreviewGrid.cellSize = new Vector3(1f, Mathf.Max(height, 0), 1f); + } + } + + /// <summary> + /// Creates a Preview for the IsometricRuleTile. + /// </summary> + protected override void CreatePreview() + { + base.CreatePreview(); + + m_PreviewGrid.cellSize = new Vector3(1f, 0.5f, 1f); + m_PreviewGrid.cellLayout = GridLayout.CellLayout.Isometric; + + foreach (var tilemapRenderer in m_PreviewTilemapRenderers) + tilemapRenderer.sortOrder = TilemapRenderer.SortOrder.TopRight; + + m_PreviewTilemapRenderers[0].sortingOrder = 0; + m_PreviewTilemapRenderers[1].sortingOrder = -1; + m_PreviewTilemapRenderers[2].sortingOrder = 1; + m_PreviewTilemapRenderers[3].sortingOrder = 0; + } + } +} diff --git a/MrBigsock/Packages/com.unity.2d.tilemap.extras/Editor/Tiles/IsometricRuleTile/IsometricRuleTileEditor.cs.meta b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Editor/Tiles/IsometricRuleTile/IsometricRuleTileEditor.cs.meta new file mode 100644 index 0000000000000000000000000000000000000000..4eb03fad79d0f3ecc295ad47a210d5cfc27db624 --- /dev/null +++ b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Editor/Tiles/IsometricRuleTile/IsometricRuleTileEditor.cs.meta @@ -0,0 +1,11 @@ +fileFormatVersion: 2 +guid: 35a64db21fde55046a6d60c50f766aa7 +MonoImporter: + externalObjects: {} + serializedVersion: 2 + defaultReferences: [] + executionOrder: 0 + icon: {instanceID: 0} + userData: + assetBundleName: + assetBundleVariant: diff --git a/MrBigsock/Packages/com.unity.2d.tilemap.extras/Editor/Tiles/Menu.meta b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Editor/Tiles/Menu.meta new file mode 100644 index 0000000000000000000000000000000000000000..ee270133089370904d3f0fbcae8a8ceea36a2e67 --- /dev/null +++ b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Editor/Tiles/Menu.meta @@ -0,0 +1,8 @@ +fileFormatVersion: 2 +guid: 50c7c1a06cd3c42629d150afe8235b81 +folderAsset: yes +DefaultImporter: + externalObjects: {} + userData: + assetBundleName: + assetBundleVariant: diff --git a/MrBigsock/Packages/com.unity.2d.tilemap.extras/Editor/Tiles/Menu/PipelineTileMenu.cs b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Editor/Tiles/Menu/PipelineTileMenu.cs new file mode 100644 index 0000000000000000000000000000000000000000..8f189b9504f96def56bb8ab2470f9907fa03e918 --- /dev/null +++ b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Editor/Tiles/Menu/PipelineTileMenu.cs @@ -0,0 +1,14 @@ +using UnityEngine; +using UnityEngine.Tilemaps; + +namespace UnityEditor.Tilemaps +{ + static internal partial class AssetCreation + { + [MenuItem("Assets/Create/2D/Tiles/Pipeline Tile", priority = (int)ETilesMenuItemOrder.PipelineTile)] + static void CreatePipelineTile() + { + ProjectWindowUtil.CreateAsset(ScriptableObject.CreateInstance<PipelineTile>(), "New Pipeline Tile.asset"); + } + } +} \ No newline at end of file diff --git a/MrBigsock/Packages/com.unity.2d.tilemap.extras/Editor/Tiles/Menu/PipelineTileMenu.cs.meta b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Editor/Tiles/Menu/PipelineTileMenu.cs.meta new file mode 100644 index 0000000000000000000000000000000000000000..a6ca666313301b1b671c26bc034c161ecefec844 --- /dev/null +++ b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Editor/Tiles/Menu/PipelineTileMenu.cs.meta @@ -0,0 +1,11 @@ +fileFormatVersion: 2 +guid: 3cca55e39f80d432d8f73394b1210999 +MonoImporter: + externalObjects: {} + serializedVersion: 2 + defaultReferences: [] + executionOrder: 0 + icon: {instanceID: 0} + userData: + assetBundleName: + assetBundleVariant: diff --git a/MrBigsock/Packages/com.unity.2d.tilemap.extras/Editor/Tiles/Menu/RandomTileMenu.cs b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Editor/Tiles/Menu/RandomTileMenu.cs new file mode 100644 index 0000000000000000000000000000000000000000..e73d44653c4eda92a06721195f8962396eae87a6 --- /dev/null +++ b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Editor/Tiles/Menu/RandomTileMenu.cs @@ -0,0 +1,14 @@ +using UnityEngine; +using UnityEngine.Tilemaps; + +namespace UnityEditor.Tilemaps +{ + static internal partial class AssetCreation + { + [MenuItem("Assets/Create/2D/Tiles/Random Tile", priority = (int)ETilesMenuItemOrder.RandomTile)] + static void CreateRandomTile() + { + ProjectWindowUtil.CreateAsset(ScriptableObject.CreateInstance<RandomTile>(), "New Random Tile.asset"); + } + } +} \ No newline at end of file diff --git a/MrBigsock/Packages/com.unity.2d.tilemap.extras/Editor/Tiles/Menu/RandomTileMenu.cs.meta b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Editor/Tiles/Menu/RandomTileMenu.cs.meta new file mode 100644 index 0000000000000000000000000000000000000000..4b234e209721b7c511ff39232dac20a81c418dea --- /dev/null +++ b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Editor/Tiles/Menu/RandomTileMenu.cs.meta @@ -0,0 +1,11 @@ +fileFormatVersion: 2 +guid: fa22e74a1cea546e2abfc3606a959191 +MonoImporter: + externalObjects: {} + serializedVersion: 2 + defaultReferences: [] + executionOrder: 0 + icon: {instanceID: 0} + userData: + assetBundleName: + assetBundleVariant: diff --git a/MrBigsock/Packages/com.unity.2d.tilemap.extras/Editor/Tiles/Menu/TerrainTileMenu.cs b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Editor/Tiles/Menu/TerrainTileMenu.cs new file mode 100644 index 0000000000000000000000000000000000000000..a9ccc9aeedabb1673fa90430f11ebebf65c2225b --- /dev/null +++ b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Editor/Tiles/Menu/TerrainTileMenu.cs @@ -0,0 +1,15 @@ +using UnityEngine; +using UnityEngine.Tilemaps; + +namespace UnityEditor.Tilemaps +{ + static internal partial class AssetCreation + { + + [MenuItem("Assets/Create/2D/Tiles/Terrain Tile", priority = (int)ETilesMenuItemOrder.TerrainTile)] + static void CreateTerrainTile() + { + ProjectWindowUtil.CreateAsset(ScriptableObject.CreateInstance<TerrainTile>(), "New Terrain Tile.asset"); + } + } +} \ No newline at end of file diff --git a/MrBigsock/Packages/com.unity.2d.tilemap.extras/Editor/Tiles/Menu/TerrainTileMenu.cs.meta b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Editor/Tiles/Menu/TerrainTileMenu.cs.meta new file mode 100644 index 0000000000000000000000000000000000000000..6c220b05d76344682dd170f828048968b570f463 --- /dev/null +++ b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Editor/Tiles/Menu/TerrainTileMenu.cs.meta @@ -0,0 +1,11 @@ +fileFormatVersion: 2 +guid: a3bb18a640e6c45f7b90b85de9b43926 +MonoImporter: + externalObjects: {} + serializedVersion: 2 + defaultReferences: [] + executionOrder: 0 + icon: {instanceID: 0} + userData: + assetBundleName: + assetBundleVariant: diff --git a/MrBigsock/Packages/com.unity.2d.tilemap.extras/Editor/Tiles/Menu/WeightedRandomTileMenu.cs b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Editor/Tiles/Menu/WeightedRandomTileMenu.cs new file mode 100644 index 0000000000000000000000000000000000000000..ba6031519dfbdaf99868c3634ac2e375a2a6b0fd --- /dev/null +++ b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Editor/Tiles/Menu/WeightedRandomTileMenu.cs @@ -0,0 +1,15 @@ +using UnityEngine; +using UnityEngine.Tilemaps; + +namespace UnityEditor.Tilemaps +{ + static internal partial class AssetCreation + { + + [MenuItem("Assets/Create/2D/Tiles/Weighted Random Tile", priority = (int)ETilesMenuItemOrder.WeightedRandomTile)] + static void CreateWeightedRandomTile() + { + ProjectWindowUtil.CreateAsset(ScriptableObject.CreateInstance<WeightedRandomTile>(), "New Weighted Random Tile.asset"); + } + } +} \ No newline at end of file diff --git a/MrBigsock/Packages/com.unity.2d.tilemap.extras/Editor/Tiles/Menu/WeightedRandomTileMenu.cs.meta b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Editor/Tiles/Menu/WeightedRandomTileMenu.cs.meta new file mode 100644 index 0000000000000000000000000000000000000000..9f9592bf06cff3afa32fc019238a6c81e30a82c3 --- /dev/null +++ b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Editor/Tiles/Menu/WeightedRandomTileMenu.cs.meta @@ -0,0 +1,11 @@ +fileFormatVersion: 2 +guid: f1cc1aa0485d0449faca8f1ce01ec015 +MonoImporter: + externalObjects: {} + serializedVersion: 2 + defaultReferences: [] + executionOrder: 0 + icon: {instanceID: 0} + userData: + assetBundleName: + assetBundleVariant: diff --git a/MrBigsock/Packages/com.unity.2d.tilemap.extras/Editor/Tiles/RuleOverrideTile.meta b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Editor/Tiles/RuleOverrideTile.meta new file mode 100644 index 0000000000000000000000000000000000000000..92557e6c2f220dca54724ef12117e4fc27257f14 --- /dev/null +++ b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Editor/Tiles/RuleOverrideTile.meta @@ -0,0 +1,8 @@ +fileFormatVersion: 2 +guid: 0a1ce61609bfe5541aea5f933f4a25de +folderAsset: yes +DefaultImporter: + externalObjects: {} + userData: + assetBundleName: + assetBundleVariant: diff --git a/MrBigsock/Packages/com.unity.2d.tilemap.extras/Editor/Tiles/RuleOverrideTile/AdvancedRuleOverrideTileEditor.cs b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Editor/Tiles/RuleOverrideTile/AdvancedRuleOverrideTileEditor.cs new file mode 100644 index 0000000000000000000000000000000000000000..bf26fc526b93d3827210c11732cdd17ed52ffdde --- /dev/null +++ b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Editor/Tiles/RuleOverrideTile/AdvancedRuleOverrideTileEditor.cs @@ -0,0 +1,215 @@ +using UnityEngine; +using UnityEngine.Tilemaps; +using UnityEditorInternal; +using System.Collections.Generic; + +namespace UnityEditor +{ + /// <summary> + /// The Editor for an AdvancedRuleOverrideTileEditor. + /// </summary> + [CustomEditor(typeof(AdvancedRuleOverrideTile))] + public class AdvancedRuleOverrideTileEditor : RuleOverrideTileEditor + { + private static class Styles + { + public static readonly GUIContent defaultSprite = EditorGUIUtility.TrTextContent("Default Sprite" + , "Overrides the default Sprite for the original Rule Tile."); + public static readonly GUIContent defaultGameObject = EditorGUIUtility.TrTextContent("Default GameObject" + , "Overrides the default GameObject for the original Rule Tile."); + public static readonly GUIContent defaultCollider = EditorGUIUtility.TrTextContent("Default Collider" + , "Overrides the default Collider for the original Rule Tile."); + } + + /// <summary> + /// The AdvancedRuleOverrideTile being edited. + /// </summary> + public new AdvancedRuleOverrideTile overrideTile => target as AdvancedRuleOverrideTile; + + List<KeyValuePair<RuleTile.TilingRule, RuleTile.TilingRuleOutput>> m_Rules = new List<KeyValuePair<RuleTile.TilingRule, RuleTile.TilingRuleOutput>>(); + private ReorderableList m_RuleList; + private int m_MissingOriginalRuleIndex; + private HashSet<int> m_UniqueIds = new HashSet<int>(); + + static float k_DefaultElementHeight { get { return RuleTileEditor.k_DefaultElementHeight; } } + static float k_SingleLineHeight { get { return RuleTileEditor.k_SingleLineHeight; } } + + /// <summary> + /// OnEnable for the AdvancedRuleOverrideTileEditor + /// </summary> + public override void OnEnable() + { + if (m_RuleList == null) + { + m_RuleList = new ReorderableList(m_Rules, typeof(KeyValuePair<RuleTile.TilingRule, RuleTile.TilingRule>), false, true, false, false); + m_RuleList.drawHeaderCallback = DrawRulesHeader; + m_RuleList.drawElementCallback = DrawRuleElement; + m_RuleList.elementHeightCallback = GetRuleElementHeight; + } + } + + /// <summary> + /// Draws the Inspector GUI for the AdvancedRuleOverrideTileEditor + /// </summary> + public override void OnInspectorGUI() + { + serializedObject.UpdateIfRequiredOrScript(); + + DrawTileField(); + + EditorGUI.BeginChangeCheck(); + overrideTile.m_DefaultSprite = EditorGUILayout.ObjectField(Styles.defaultSprite, overrideTile.m_DefaultSprite, typeof(Sprite), false) as Sprite; + overrideTile.m_DefaultGameObject = EditorGUILayout.ObjectField(Styles.defaultGameObject, overrideTile.m_DefaultGameObject, typeof(GameObject), false) as GameObject; + overrideTile.m_DefaultColliderType = (Tile.ColliderType)EditorGUILayout.EnumPopup(Styles.defaultCollider, overrideTile.m_DefaultColliderType); + if (EditorGUI.EndChangeCheck()) + SaveTile(); + + DrawCustomFields(); + + if (overrideTile.m_Tile) + { + ValidateRuleTile(overrideTile.m_Tile); + overrideTile.GetOverrides(m_Rules, ref m_MissingOriginalRuleIndex); + } + + m_RuleList.DoLayoutList(); + } + + private void ValidateRuleTile(RuleTile ruleTile) + { + // Ensure that each Tiling Rule in the RuleTile has a unique ID + m_UniqueIds.Clear(); + var startId = 0; + foreach (var rule in ruleTile.m_TilingRules) + { + if (m_UniqueIds.Contains(rule.m_Id)) + { + do + { + rule.m_Id = startId++; + } while (m_UniqueIds.Contains(rule.m_Id)); + EditorUtility.SetDirty(ruleTile); + } + m_UniqueIds.Add(rule.m_Id); + startId++; + } + } + + /// <summary> + /// Draws the Header for the Rule list + /// </summary> + /// <param name="rect">Rect to draw the header in</param> + public void DrawRulesHeader(Rect rect) + { + GUI.Label(rect, "Tiling Rules", EditorStyles.label); + } + + /// <summary> + /// Draws the Rule element for the Rule list + /// </summary> + /// <param name="rect">Rect to draw the Rule Element in</param> + /// <param name="index">Index of the Rule Element to draw</param> + /// <param name="active">Whether the Rule Element is active</param> + /// <param name="focused">Whether the Rule Element is focused</param> + public void DrawRuleElement(Rect rect, int index, bool active, bool focused) + { + RuleTile.TilingRule originalRule = m_Rules[index].Key; + if (originalRule == null) + return; + + RuleTile.TilingRuleOutput overrideRule = m_Rules[index].Value; + bool isMissing = index >= m_MissingOriginalRuleIndex; + + DrawToggleInternal(new Rect(rect.xMin, rect.yMin, 16, rect.height)); + DrawRuleInternal(new Rect(rect.xMin + 16, rect.yMin, rect.width - 16, rect.height)); + + void DrawToggleInternal(Rect r) + { + EditorGUI.BeginChangeCheck(); + + bool enabled = EditorGUI.Toggle(new Rect(r.xMin, r.yMin, r.width, k_SingleLineHeight), overrideRule != null); + + if (EditorGUI.EndChangeCheck()) + { + if (enabled) + overrideTile[originalRule] = originalRule; + else + overrideTile[originalRule] = null; + + SaveTile(); + } + } + void DrawRuleInternal(Rect r) + { + EditorGUI.BeginChangeCheck(); + + DrawRule(r, overrideRule ?? originalRule, overrideRule != null, originalRule, isMissing); + + if (EditorGUI.EndChangeCheck()) + SaveTile(); + } + } + + /// <summary> + /// Draw a Rule Override for the AdvancedRuleOverrideTileEditor + /// </summary> + /// <param name="rect">Rect to draw the Rule in</param> + /// <param name="rule">The Rule Override to draw</param> + /// <param name="isOverride">Whether the original Rule is being overridden</param> + /// <param name="originalRule">Original Rule to override</param> + /// <param name="isMissing">Whether the original Rule is missing</param> + public void DrawRule(Rect rect, RuleTile.TilingRuleOutput rule, bool isOverride, RuleTile.TilingRule originalRule, bool isMissing) + { + if (isMissing) + { + EditorGUI.HelpBox(new Rect(rect.xMin, rect.yMin, rect.width, 16), "Original Tiling Rule missing", MessageType.Warning); + rect.yMin += 16; + } + + using (new EditorGUI.DisabledScope(!isOverride)) + { + float yPos = rect.yMin + 2f; + float height = rect.height - k_PaddingBetweenRules; + float matrixWidth = k_DefaultElementHeight; + + BoundsInt ruleBounds = originalRule.GetBounds(); + BoundsInt ruleGuiBounds = ruleTileEditor.GetRuleGUIBounds(ruleBounds, originalRule); + Vector2 matrixSize = ruleTileEditor.GetMatrixSize(ruleGuiBounds); + Vector2 matrixSizeRate = matrixSize / Mathf.Max(matrixSize.x, matrixSize.y); + Vector2 matrixRectSize = new Vector2(matrixWidth * matrixSizeRate.x, k_DefaultElementHeight * matrixSizeRate.y); + Vector2 matrixRectPosition = new Vector2(rect.xMax - matrixWidth * 2f - 10f, yPos); + matrixRectPosition.x += (matrixWidth - matrixRectSize.x) * 0.5f; + matrixRectPosition.y += (k_DefaultElementHeight - matrixRectSize.y) * 0.5f; + + Rect inspectorRect = new Rect(rect.xMin, yPos, rect.width - matrixWidth * 2f - 20f, height); + Rect matrixRect = new Rect(matrixRectPosition, matrixRectSize); + Rect spriteRect = new Rect(rect.xMax - matrixWidth - 5f, yPos, matrixWidth, k_DefaultElementHeight); + + ruleTileEditor.RuleInspectorOnGUI(inspectorRect, rule); + ruleTileEditor.SpriteOnGUI(spriteRect, rule); + + if (!isMissing) + using (new EditorGUI.DisabledScope(true)) + ruleTileEditor.RuleMatrixOnGUI(overrideTile.m_InstanceTile, matrixRect, ruleGuiBounds, originalRule); + } + } + + /// <summary> + /// Returns the height for an indexed Rule Element + /// </summary> + /// <param name="index">Index of the Rule Element</param> + /// <returns>Height of the indexed Rule Element</returns> + public float GetRuleElementHeight(int index) + { + var originalRule = m_Rules[index].Key; + var overrideRule = m_Rules[index].Value; + float height = overrideRule != null ? ruleTileEditor.GetElementHeight(overrideRule) : ruleTileEditor.GetElementHeight(originalRule); + + bool isMissing = index >= m_MissingOriginalRuleIndex; + if (isMissing) + height += 16; + + return height; + } + } +} diff --git a/MrBigsock/Packages/com.unity.2d.tilemap.extras/Editor/Tiles/RuleOverrideTile/AdvancedRuleOverrideTileEditor.cs.meta b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Editor/Tiles/RuleOverrideTile/AdvancedRuleOverrideTileEditor.cs.meta new file mode 100644 index 0000000000000000000000000000000000000000..a9cce8fecc501f1ee3557b4c66926c81936d51df --- /dev/null +++ b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Editor/Tiles/RuleOverrideTile/AdvancedRuleOverrideTileEditor.cs.meta @@ -0,0 +1,11 @@ +fileFormatVersion: 2 +guid: bd604afe90023492586ef97592e54ba4 +MonoImporter: + externalObjects: {} + serializedVersion: 2 + defaultReferences: [] + executionOrder: 0 + icon: {instanceID: 0} + userData: + assetBundleName: + assetBundleVariant: diff --git a/MrBigsock/Packages/com.unity.2d.tilemap.extras/Editor/Tiles/RuleOverrideTile/PopulateRuleOverrideTileWizard.cs b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Editor/Tiles/RuleOverrideTile/PopulateRuleOverrideTileWizard.cs new file mode 100644 index 0000000000000000000000000000000000000000..1b62c5ea31abc9c53519d075593414fedfacf543 --- /dev/null +++ b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Editor/Tiles/RuleOverrideTile/PopulateRuleOverrideTileWizard.cs @@ -0,0 +1,109 @@ +using UnityEngine; +using UnityEngine.Tilemaps; +using System.Linq; +using System.Text.RegularExpressions; +using UnityEngine.Scripting.APIUpdating; + +namespace UnityEditor.Tilemaps +{ + /// <summary> + /// Wizard for populating RuleOverrideTile from a SpriteSheet + /// </summary> + [MovedFrom(true, "UnityEditor")] + public class PopulateRuleOverideTileWizard : ScriptableWizard + { + [MenuItem("CONTEXT/RuleOverrideTile/Populate From Sprite Sheet")] + static void MenuOption(MenuCommand menuCommand) + { + PopulateRuleOverideTileWizard.CreateWizard(menuCommand.context as RuleOverrideTile); + } + [MenuItem("CONTEXT/RuleOverrideTile/Populate From Sprite Sheet", true)] + static bool MenuOptionValidation(MenuCommand menuCommand) + { + RuleOverrideTile tile = menuCommand.context as RuleOverrideTile; + return tile.m_Tile; + } + + /// <summary> + /// The Texture2D containing the Sprites to override with + /// </summary> + public Texture2D m_spriteSet; + + private RuleOverrideTile m_tileset; + + /// <summary> + /// Creates a wizard for the target RuleOverrideTIle + /// </summary> + /// <param name="target">The RuleOverrideTile to be edited by the wizard</param> + public static void CreateWizard(RuleOverrideTile target) { + PopulateRuleOverideTileWizard wizard = DisplayWizard<PopulateRuleOverideTileWizard>("Populate Override", "Populate"); + wizard.m_tileset = target; + } + + /// <summary> + /// Creates a new PopulateRuleOverideTileWizard and copies the settings from an existing PopulateRuleOverideTileWizard + /// </summary> + /// <param name="oldWizard">The wizard to copy settings from</param> + public static void CloneWizard(PopulateRuleOverideTileWizard oldWizard) { + PopulateRuleOverideTileWizard wizard = DisplayWizard<PopulateRuleOverideTileWizard>("Populate Override", "Populate"); + wizard.m_tileset = oldWizard.m_tileset; + wizard.m_spriteSet = oldWizard.m_spriteSet; + } + + private void OnWizardUpdate() { + isValid = m_tileset != null && m_spriteSet != null; + } + + private void OnWizardCreate() { + try { + Populate(); + } + catch(System.Exception ex) { + EditorUtility.DisplayDialog("Auto-populate failed!", ex.Message, "Ok"); + CloneWizard(this); + } + } + + /// <summary> + /// Attempts to populate the selected override tile using the chosen sprite. + /// The assumption here is that the sprite set selected by the user has the same + /// naming scheme as the original sprite. That is to say, they should both have the same number + /// of sprites, each sprite ends in an underscore followed by a number, and that they are + /// intended to be equivalent in function. + /// </summary> + private void Populate() { + string spriteSheet = AssetDatabase.GetAssetPath(m_spriteSet); + Sprite[] overrideSprites = AssetDatabase.LoadAllAssetsAtPath(spriteSheet).OfType<Sprite>().ToArray(); + + bool finished = false; + + try { + Undo.RecordObject(m_tileset, "Auto-populate " + m_tileset.name); + + foreach(RuleTile.TilingRule rule in m_tileset.m_Tile.m_TilingRules) { + foreach(Sprite originalSprite in rule.m_Sprites) { + string spriteName = originalSprite.name; + string spriteNumber = Regex.Match(spriteName, @"_\d+$").Value; + + Sprite matchingOverrideSprite = overrideSprites.First(sprite => sprite.name.EndsWith(spriteNumber)); + + m_tileset[originalSprite] = matchingOverrideSprite; + } + } + + finished = true; + } + catch(System.InvalidOperationException ex) { + throw (new System.ArgumentOutOfRangeException("Sprite sheet mismatch", ex)); + } + finally { + // We handle the undo like this in case we end up catching more exceptions. + // We want this to ALWAYS happen unless we complete the population. + if(!finished) { + Undo.PerformUndo(); + } + } + } + + } +} diff --git a/MrBigsock/Packages/com.unity.2d.tilemap.extras/Editor/Tiles/RuleOverrideTile/PopulateRuleOverrideTileWizard.cs.meta b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Editor/Tiles/RuleOverrideTile/PopulateRuleOverrideTileWizard.cs.meta new file mode 100644 index 0000000000000000000000000000000000000000..f8d7dcf41d0e1d8892141a45a20295244d5bd481 --- /dev/null +++ b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Editor/Tiles/RuleOverrideTile/PopulateRuleOverrideTileWizard.cs.meta @@ -0,0 +1,11 @@ +fileFormatVersion: 2 +guid: 4dcb91d1bb7d18847b5ce74c4cdb1921 +MonoImporter: + externalObjects: {} + serializedVersion: 2 + defaultReferences: [] + executionOrder: 0 + icon: {instanceID: 0} + userData: + assetBundleName: + assetBundleVariant: diff --git a/MrBigsock/Packages/com.unity.2d.tilemap.extras/Editor/Tiles/RuleOverrideTile/RuleOverrideTileEditor.cs b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Editor/Tiles/RuleOverrideTile/RuleOverrideTileEditor.cs new file mode 100644 index 0000000000000000000000000000000000000000..08a0ac21c2621249151c765be6f59535aff0f033 --- /dev/null +++ b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Editor/Tiles/RuleOverrideTile/RuleOverrideTileEditor.cs @@ -0,0 +1,430 @@ +using UnityEngine; +using UnityEngine.Tilemaps; +using UnityEditorInternal; +using System.Collections.Generic; + +namespace UnityEditor +{ + /// <summary> + /// The Editor for a RuleOverrideTileEditor. + /// </summary> + [CustomEditor(typeof(RuleOverrideTile))] + public class RuleOverrideTileEditor : Editor + { + private static class Styles + { + public static readonly GUIContent overrideTile = EditorGUIUtility.TrTextContent("Tile" + , "The Rule Tile to override."); + } + + /// <summary> + /// The RuleOverrideTile being edited + /// </summary> + public RuleOverrideTile overrideTile => target as RuleOverrideTile; + /// <summary> + /// The RuleTileEditor for the overridden instance of the RuleTile + /// </summary> + public RuleTileEditor ruleTileEditor + { + get + { + if (m_RuleTileEditorTarget != overrideTile.m_Tile) + { + DestroyImmediate(m_RuleTileEditor); + m_RuleTileEditor = Editor.CreateEditor(overrideTile.m_InstanceTile) as RuleTileEditor; + m_RuleTileEditorTarget = overrideTile.m_Tile; + } + return m_RuleTileEditor; + } + } + + RuleTileEditor m_RuleTileEditor; + RuleTile m_RuleTileEditorTarget; + + /// <summary> + /// List of Sprites and overriding Sprites + /// </summary> + public List<KeyValuePair<Sprite, Sprite>> m_Sprites = new List<KeyValuePair<Sprite, Sprite>>(); + /// <summary> + /// List of GameObjects and overriding GameObjects + /// </summary> + public List<KeyValuePair<GameObject, GameObject>> m_GameObjects = new List<KeyValuePair<GameObject, GameObject>>(); + private ReorderableList m_SpriteList; + private ReorderableList m_GameObjectList; + private int m_MissingOriginalSpriteIndex; + private int m_MissingOriginalGameObjectIndex; + + /// <summary> + /// Height for a Sprite Element + /// </summary> + public static float k_SpriteElementHeight = 48; + /// <summary> + /// Height for a GameObject Element + /// </summary> + public static float k_GameObjectElementHeight = 16; + /// <summary> + /// Padding between Rule Elements + /// </summary> + public static float k_PaddingBetweenRules = 4; + + /// <summary> + /// OnEnable for the RuleOverrideTileEditor + /// </summary> + public virtual void OnEnable() + { + if (m_SpriteList == null) + { + m_SpriteList = new ReorderableList(m_Sprites, typeof(KeyValuePair<Sprite, Sprite>), false, true, false, false); + m_SpriteList.drawHeaderCallback = DrawSpriteListHeader; + m_SpriteList.drawElementCallback = DrawSpriteElement; + m_SpriteList.elementHeightCallback = GetSpriteElementHeight; + } + if (m_GameObjectList == null) + { + m_GameObjectList = new ReorderableList(m_GameObjects, typeof(KeyValuePair<Sprite, Sprite>), false, true, false, false); + m_GameObjectList.drawHeaderCallback = DrawGameObjectListHeader; + m_GameObjectList.drawElementCallback = DrawGameObjectElement; + m_GameObjectList.elementHeightCallback = GetGameObjectElementHeight; + } + } + + /// <summary> + /// OnDisable for the RuleOverrideTileEditor + /// </summary> + public virtual void OnDisable() + { + DestroyImmediate(ruleTileEditor); + m_RuleTileEditorTarget = null; + } + + /// <summary> + /// Draws the Inspector GUI for the RuleOverrideTileEditor + /// </summary> + public override void OnInspectorGUI() + { + serializedObject.UpdateIfRequiredOrScript(); + + DrawTileField(); + DrawCustomFields(); + + overrideTile.GetOverrides(m_Sprites, ref m_MissingOriginalSpriteIndex); + overrideTile.GetOverrides(m_GameObjects, ref m_MissingOriginalGameObjectIndex); + + EditorGUI.BeginChangeCheck(); + m_SpriteList.DoLayoutList(); + if (EditorGUI.EndChangeCheck()) + { + overrideTile.ApplyOverrides(m_Sprites); + SaveTile(); + } + + EditorGUI.BeginChangeCheck(); + m_GameObjectList.DoLayoutList(); + if (EditorGUI.EndChangeCheck()) + { + overrideTile.ApplyOverrides(m_GameObjects); + SaveTile(); + } + } + + /// <summary> + /// Draws the header for the Sprite list + /// </summary> + /// <param name="rect">GUI Rect to draw the header at</param> + public void DrawSpriteListHeader(Rect rect) + { + float xMax = rect.xMax; + rect.xMax = rect.xMax / 2.0f; + GUI.Label(rect, "Original Sprite", EditorStyles.label); + rect.xMin = rect.xMax; + rect.xMax = xMax; + GUI.Label(rect, "Override Sprite", EditorStyles.label); + } + + /// <summary> + /// Draws the header for the GameObject list + /// </summary> + /// <param name="rect">GUI Rect to draw the header at</param> + public void DrawGameObjectListHeader(Rect rect) + { + float xMax = rect.xMax; + rect.xMax = rect.xMax / 2.0f; + GUI.Label(rect, "Original GameObject", EditorStyles.label); + rect.xMin = rect.xMax; + rect.xMax = xMax; + GUI.Label(rect, "Override GameObject", EditorStyles.label); + } + + /// <summary> + /// Gets the GUI element height for a Sprite element with the given index + /// </summary> + /// <param name="index">Index of the Sprite element</param> + /// <returns>GUI element height for the Sprite element</returns> + public float GetSpriteElementHeight(int index) + { + float height = k_SpriteElementHeight + k_PaddingBetweenRules; + + bool isMissing = index >= m_MissingOriginalSpriteIndex; + if (isMissing) + height += 16; + + return height; + } + + /// <summary> + /// Gets the GUI element height for a GameObject element with the given index + /// </summary> + /// <param name="index">Index of the GameObject element</param> + /// <returns>GUI element height for the GameObject element</returns> + public float GetGameObjectElementHeight(int index) + { + float height = k_GameObjectElementHeight + k_PaddingBetweenRules; + + bool isMissing = index >= m_MissingOriginalGameObjectIndex; + if (isMissing) + height += 16; + + return height; + } + + /// <summary> + /// Draws the Sprite element for the RuleOverride list + /// </summary> + /// <param name="rect">Rect to draw the Sprite Element in</param> + /// <param name="index">Index of the Sprite Element to draw</param> + /// <param name="active">Whether the Sprite Element is active</param> + /// <param name="focused">Whether the Sprite Element is focused</param> + public void DrawSpriteElement(Rect rect, int index, bool active, bool focused) + { + bool isMissing = index >= m_MissingOriginalSpriteIndex; + if (isMissing) + { + EditorGUI.HelpBox(new Rect(rect.xMin, rect.yMin, rect.width, 16), "Original Sprite missing", MessageType.Warning); + rect.yMin += 16; + } + + Sprite originalSprite = m_Sprites[index].Key; + Sprite overrideSprite = m_Sprites[index].Value; + + rect.y += 2; + rect.height -= k_PaddingBetweenRules; + + rect.xMax = rect.xMax / 2.0f; + using (new EditorGUI.DisabledScope(true)) + EditorGUI.ObjectField(new Rect(rect.xMin, rect.yMin, rect.height, rect.height), originalSprite, typeof(Sprite), false); + rect.xMin = rect.xMax; + rect.xMax *= 2.0f; + + EditorGUI.BeginChangeCheck(); + overrideSprite = EditorGUI.ObjectField(new Rect(rect.xMin, rect.yMin, rect.height, rect.height), overrideSprite, typeof(Sprite), false) as Sprite; + if (EditorGUI.EndChangeCheck()) + m_Sprites[index] = new KeyValuePair<Sprite, Sprite>(originalSprite, overrideSprite); + } + + /// <summary> + /// Draws the GameObject element for the RuleOverride list + /// </summary> + /// <param name="rect">Rect to draw the GameObject Element in</param> + /// <param name="index">Index of the GameObject Element to draw</param> + /// <param name="active">Whether the GameObject Element is active</param> + /// <param name="focused">Whether the GameObject Element is focused</param> + public void DrawGameObjectElement(Rect rect, int index, bool active, bool focused) + { + bool isMissing = index >= m_MissingOriginalGameObjectIndex; + if (isMissing) + { + EditorGUI.HelpBox(new Rect(rect.xMin, rect.yMin, rect.width, 16), "Original GameObject missing", MessageType.Warning); + rect.yMin += 16; + } + + GameObject originalGameObject = m_GameObjects[index].Key; + GameObject overrideGameObject = m_GameObjects[index].Value; + + rect.y += 2; + rect.height -= k_PaddingBetweenRules; + + rect.xMax = rect.xMax / 2.0f; + using (new EditorGUI.DisabledScope(true)) + EditorGUI.ObjectField(new Rect(rect.xMin, rect.yMin, rect.width, rect.height), originalGameObject, typeof(GameObject), false); + rect.xMin = rect.xMax; + rect.xMax *= 2.0f; + + EditorGUI.BeginChangeCheck(); + overrideGameObject = EditorGUI.ObjectField(new Rect(rect.xMin, rect.yMin, rect.width, rect.height), overrideGameObject, typeof(GameObject), false) as GameObject; + if (EditorGUI.EndChangeCheck()) + m_GameObjects[index] = new KeyValuePair<GameObject, GameObject>(originalGameObject, overrideGameObject); + } + + /// <summary> + /// Draws a field for the RuleTile be overridden + /// </summary> + public void DrawTileField() + { + EditorGUI.BeginChangeCheck(); + RuleTile tile = EditorGUILayout.ObjectField(Styles.overrideTile, overrideTile.m_Tile, typeof(RuleTile), false) as RuleTile; + if (EditorGUI.EndChangeCheck()) + { + if (!LoopCheck(tile)) + { + overrideTile.m_Tile = tile; + SaveTile(); + } + else + { + Debug.LogWarning("Circular tile reference"); + } + } + + bool LoopCheck(RuleTile checkTile) + { + if (!overrideTile.m_InstanceTile) + return false; + + HashSet<RuleTile> renferenceTils = new HashSet<RuleTile>(); + Add(overrideTile.m_InstanceTile); + + return renferenceTils.Contains(checkTile); + + void Add(RuleTile ruleTile) + { + if (renferenceTils.Contains(ruleTile)) + return; + + renferenceTils.Add(ruleTile); + + var overrideTiles = RuleTileEditor.FindAffectedOverrideTiles(ruleTile); + + foreach (var overrideTile in overrideTiles) + Add(overrideTile.m_InstanceTile); + } + } + } + + /// <summary> + /// Draw editor fields for custom properties for the RuleOverrideTile + /// </summary> + public void DrawCustomFields() + { + if (ruleTileEditor) + { + ruleTileEditor.target.hideFlags = HideFlags.None; + ruleTileEditor.DrawCustomFields(true); + ruleTileEditor.target.hideFlags = HideFlags.NotEditable; + } + } + + private void SaveInstanceTileAsset() + { + bool assetChanged = false; + + if (overrideTile.m_InstanceTile) + { + if (!overrideTile.m_Tile || overrideTile.m_InstanceTile.GetType() != overrideTile.m_Tile.GetType()) + { + DestroyImmediate(overrideTile.m_InstanceTile, true); + overrideTile.m_InstanceTile = null; + assetChanged = true; + } + } + if (!overrideTile.m_InstanceTile) + { + if (overrideTile.m_Tile) + { + var t = overrideTile.m_Tile.GetType(); + RuleTile instanceTile = ScriptableObject.CreateInstance(t) as RuleTile; + instanceTile.hideFlags = HideFlags.NotEditable; + AssetDatabase.AddObjectToAsset(instanceTile, overrideTile); + overrideTile.m_InstanceTile = instanceTile; + assetChanged = true; + } + } + + if (overrideTile.m_InstanceTile) + { + string instanceTileName = overrideTile.m_Tile.name + " (Override)"; + if (overrideTile.m_InstanceTile.name != instanceTileName) + { + overrideTile.m_InstanceTile.name = instanceTileName; + assetChanged = true; + } + } + + if (assetChanged) + { + EditorUtility.SetDirty(overrideTile.m_InstanceTile); + AssetDatabase.SaveAssetIfDirty(overrideTile.m_InstanceTile); + } + } + + /// <summary> + /// Saves any changes to the RuleOverrideTile + /// </summary> + public void SaveTile() + { + EditorUtility.SetDirty(target); + SceneView.RepaintAll(); + + SaveInstanceTileAsset(); + + if (overrideTile.m_InstanceTile) + { + overrideTile.Override(); + RuleTileEditor.UpdateAffectedOverrideTiles(overrideTile.m_InstanceTile); + } + + if (ruleTileEditor && ruleTileEditor.m_PreviewTilemaps != null) + { + foreach (var tilemap in ruleTileEditor.m_PreviewTilemaps) + tilemap.RefreshAllTiles(); + } + } + + /// <summary> + /// Renders a static preview Texture2D for a RuleOverrideTile asset + /// </summary> + /// <param name="assetPath">Asset path of the RuleOverrideTile</param> + /// <param name="subAssets">Arrays of assets from the given Asset path</param> + /// <param name="width">Width of the static preview</param> + /// <param name="height">Height of the static preview </param> + /// <returns>Texture2D containing static preview for the RuleOverrideTile asset</returns> + public override Texture2D RenderStaticPreview(string assetPath, Object[] subAssets, int width, int height) + { + if (ruleTileEditor) + return ruleTileEditor.RenderStaticPreview(assetPath, subAssets, width, height); + + return base.RenderStaticPreview(assetPath, subAssets, width, height); + } + + /// <summary> + /// Whether the RuleOverrideTile has a preview GUI + /// </summary> + /// <returns>True if RuleOverrideTile has a preview GUI. False if not.</returns> + public override bool HasPreviewGUI() + { + if (ruleTileEditor) + return ruleTileEditor.HasPreviewGUI(); + + return false; + } + + /// <summary> + /// Updates preview settings for the RuleOverrideTile. + /// </summary> + public override void OnPreviewSettings() + { + if (ruleTileEditor) + ruleTileEditor.OnPreviewSettings(); + } + + /// <summary> + /// Draws the preview GUI for the RuleTile + /// </summary> + /// <param name="rect">Rect to draw the preview GUI</param> + /// <param name="background">The GUIStyle of the background for the preview</param> + public override void OnPreviewGUI(Rect rect, GUIStyle background) + { + if (ruleTileEditor) + ruleTileEditor.OnPreviewGUI(rect, background); + } + } +} diff --git a/MrBigsock/Packages/com.unity.2d.tilemap.extras/Editor/Tiles/RuleOverrideTile/RuleOverrideTileEditor.cs.meta b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Editor/Tiles/RuleOverrideTile/RuleOverrideTileEditor.cs.meta new file mode 100644 index 0000000000000000000000000000000000000000..4b8d0579147f26b3750f525931fd5a835c7d3278 --- /dev/null +++ b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Editor/Tiles/RuleOverrideTile/RuleOverrideTileEditor.cs.meta @@ -0,0 +1,11 @@ +fileFormatVersion: 2 +guid: dd977390416471341b10fd1278290da0 +MonoImporter: + externalObjects: {} + serializedVersion: 2 + defaultReferences: [] + executionOrder: 0 + icon: {instanceID: 0} + userData: + assetBundleName: + assetBundleVariant: diff --git a/MrBigsock/Packages/com.unity.2d.tilemap.extras/Editor/Tiles/RuleTile.meta b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Editor/Tiles/RuleTile.meta new file mode 100644 index 0000000000000000000000000000000000000000..f0c737e0f52be959952a43e0e51969c93c26e563 --- /dev/null +++ b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Editor/Tiles/RuleTile.meta @@ -0,0 +1,8 @@ +fileFormatVersion: 2 +guid: 0fac9738c4893c34785c399d087c715d +folderAsset: yes +DefaultImporter: + externalObjects: {} + userData: + assetBundleName: + assetBundleVariant: diff --git a/MrBigsock/Packages/com.unity.2d.tilemap.extras/Editor/Tiles/RuleTile/RuleTileEditor.cs b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Editor/Tiles/RuleTile/RuleTileEditor.cs new file mode 100644 index 0000000000000000000000000000000000000000..37b2ae865ef2627ccd7ff537b795223352636def --- /dev/null +++ b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Editor/Tiles/RuleTile/RuleTileEditor.cs @@ -0,0 +1,1222 @@ +using System; +using System.Collections.Generic; +using System.Reflection; +using System.Linq; +using UnityEditorInternal; +using UnityEngine; +using UnityEngine.Tilemaps; +using Object = UnityEngine.Object; + +namespace UnityEditor +{ + /// <summary> + /// The Editor for a RuleTile. + /// </summary> + [CustomEditor(typeof(RuleTile), true)] + [CanEditMultipleObjects] + public class RuleTileEditor : Editor + { + private const string s_XIconString = "iVBORw0KGgoAAAANSUhEUgAAAA8AAAAPCAYAAAA71pVKAAAAAXNSR0IArs4c6QAAAARnQU1BAACxjwv8YQUAAAAJcEhZcwAADsMAAA7DAcdvqGQAAAAYdEVYdFNvZnR3YXJlAHBhaW50Lm5ldCA0LjAuNWWFMmUAAABoSURBVDhPnY3BDcAgDAOZhS14dP1O0x2C/LBEgiNSHvfwyZabmV0jZRUpq2zi6f0DJwdcQOEdwwDLypF0zHLMa9+NQRxkQ+ACOT2STVw/q8eY1346ZlE54sYAhVhSDrjwFymrSFnD2gTZpls2OvFUHAAAAABJRU5ErkJggg=="; + private const string s_Arrow0 = "iVBORw0KGgoAAAANSUhEUgAAAA8AAAAPCAYAAAA71pVKAAAAAXNSR0IArs4c6QAAAARnQU1BAACxjwv8YQUAAAAJcEhZcwAADsMAAA7DAcdvqGQAAAAYdEVYdFNvZnR3YXJlAHBhaW50Lm5ldCA0LjAuNWWFMmUAAACYSURBVDhPzZExDoQwDATzE4oU4QXXcgUFj+YxtETwgpMwXuFcwMFSRMVKKwzZcWzhiMg91jtg34XIntkre5EaT7yjjhI9pOD5Mw5k2X/DdUwFr3cQ7Pu23E/BiwXyWSOxrNqx+ewnsayam5OLBtbOGPUM/r93YZL4/dhpR/amwByGFBz170gNChA6w5bQQMqramBTgJ+Z3A58WuWejPCaHQAAAABJRU5ErkJggg=="; + private const string s_Arrow1 = "iVBORw0KGgoAAAANSUhEUgAAAA8AAAAPCAYAAAA71pVKAAAAAXNSR0IArs4c6QAAAARnQU1BAACxjwv8YQUAAAAJcEhZcwAADsMAAA7DAcdvqGQAAAAYdEVYdFNvZnR3YXJlAHBhaW50Lm5ldCA0LjAuNWWFMmUAAABqSURBVDhPxYzBDYAgEATpxYcd+PVr0fZ2siZrjmMhFz6STIiDs8XMlpEyi5RkO/d66TcgJUB43JfNBqRkSEYDnYjhbKD5GIUkDqRDwoH3+NgTAw+bL/aoOP4DOgH+iwECEt+IlFmkzGHlAYKAWF9R8zUnAAAAAElFTkSuQmCC"; + private const string s_Arrow2 = "iVBORw0KGgoAAAANSUhEUgAAAA8AAAAPCAYAAAA71pVKAAAAAXNSR0IArs4c6QAAAARnQU1BAACxjwv8YQUAAAAJcEhZcwAADsMAAA7DAcdvqGQAAAAYdEVYdFNvZnR3YXJlAHBhaW50Lm5ldCA0LjAuNWWFMmUAAAC0SURBVDhPjVE5EsIwDMxPKFKYF9CagoJH8xhaMskLmEGsjOSRkBzYmU2s9a58TUQUmCH1BWEHweuKP+D8tphrWcAHuIGrjPnPNY8X2+DzEWE+FzrdrkNyg2YGNNfRGlyOaZDJOxBrDhgOowaYW8UW0Vau5ZkFmXbbDr+CzOHKmLinAXMEePyZ9dZkZR+s5QX2O8DY3zZ/sgYcdDqeEVp8516o0QQV1qeMwg6C91toYoLoo+kNt/tpKQEVvFQAAAAASUVORK5CYII="; + private const string s_Arrow3 = "iVBORw0KGgoAAAANSUhEUgAAAA8AAAAPCAYAAAA71pVKAAAAAXNSR0IArs4c6QAAAARnQU1BAACxjwv8YQUAAAAJcEhZcwAADsMAAA7DAcdvqGQAAAAYdEVYdFNvZnR3YXJlAHBhaW50Lm5ldCA0LjAuNWWFMmUAAAB2SURBVDhPzY1LCoAwEEPnLi48gW5d6p31bH5SMhp0Cq0g+CCLxrzRPqMZ2pRqKG4IqzJc7JepTlbRZXYpWTg4RZE1XAso8VHFKNhQuTjKtZvHUNCEMogO4K3BhvMn9wP4EzoPZ3n0AGTW5fiBVzLAAYTP32C2Ay3agtu9V/9PAAAAAElFTkSuQmCC"; + private const string s_Arrow5 = "iVBORw0KGgoAAAANSUhEUgAAAA8AAAAPCAYAAAA71pVKAAAAAXNSR0IArs4c6QAAAARnQU1BAACxjwv8YQUAAAAJcEhZcwAADsMAAA7DAcdvqGQAAAAYdEVYdFNvZnR3YXJlAHBhaW50Lm5ldCA0LjAuNWWFMmUAAABqSURBVDhPnY3BCYBADASvFx924NevRdvbyoLBmNuDJQMDGjNxAFhK1DyUQ9fvobCdO+j7+sOKj/uSB+xYHZAxl7IR1wNTXJeVcaAVU+614uWfCT9mVUhknMlxDokd15BYsQrJFHeUQ0+MB5ErsPi/6hO1AAAAAElFTkSuQmCC"; + private const string s_Arrow6 = "iVBORw0KGgoAAAANSUhEUgAAAA8AAAAPCAYAAAA71pVKAAAAAXNSR0IArs4c6QAAAARnQU1BAACxjwv8YQUAAAAJcEhZcwAADsMAAA7DAcdvqGQAAAAYdEVYdFNvZnR3YXJlAHBhaW50Lm5ldCA0LjAuNWWFMmUAAACaSURBVDhPxZExEkAwEEVzE4UiTqClUDi0w2hlOIEZsV82xCZmQuPPfFn8t1mirLWf7S5flQOXjd64vCuEKWTKVt+6AayH3tIa7yLg6Qh2FcKFB72jBgJeziA1CMHzeaNHjkfwnAK86f3KUafU2ClHIJSzs/8HHLv09M3SaMCxS7ljw/IYJWzQABOQZ66x4h614ahTCL/WT7BSO51b5Z5hSx88AAAAAElFTkSuQmCC"; + private const string s_Arrow7 = "iVBORw0KGgoAAAANSUhEUgAAAA8AAAAPCAYAAAA71pVKAAAAAXNSR0IArs4c6QAAAARnQU1BAACxjwv8YQUAAAAJcEhZcwAADsMAAA7DAcdvqGQAAAAYdEVYdFNvZnR3YXJlAHBhaW50Lm5ldCA0LjAuNWWFMmUAAABQSURBVDhPYxh8QNle/T8U/4MKEQdAmsz2eICx6W530gygr2aQBmSMphkZYxqErAEXxusKfAYQ7XyyNMIAsgEkaYQBkAFkaYQBsjXSGDAwAAD193z4luKPrAAAAABJRU5ErkJggg=="; + private const string s_Arrow8 = "iVBORw0KGgoAAAANSUhEUgAAAA8AAAAPCAYAAAA71pVKAAAAAXNSR0IArs4c6QAAAARnQU1BAACxjwv8YQUAAAAJcEhZcwAADsMAAA7DAcdvqGQAAAAYdEVYdFNvZnR3YXJlAHBhaW50Lm5ldCA0LjAuNWWFMmUAAACYSURBVDhPxZE9DoAwCIW9iUOHegJXHRw8tIdx1egJTMSHAeMPaHSR5KVQ+KCkCRF91mdz4VDEWVzXTBgg5U1N5wahjHzXS3iFFVRxAygNVaZxJ6VHGIl2D6oUXP0ijlJuTp724FnID1Lq7uw2QM5+thoKth0N+GGyA7IA3+yM77Ag1e2zkey5gCdAg/h8csy+/89v7E+YkgUntOWeVt2SfAAAAABJRU5ErkJggg=="; + private const string s_MirrorX = "iVBORw0KGgoAAAANSUhEUgAAAA8AAAAPCAYAAAA71pVKAAAABGdBTUEAALGPC/xhBQAAAAlwSFlzAAAOwQAADsEBuJFr7QAAABh0RVh0U29mdHdhcmUAcGFpbnQubmV0IDQuMC41ZYUyZQAAAG1JREFUOE+lj9ENwCAIRB2IFdyRfRiuDSaXAF4MrR9P5eRhHGb2Gxp2oaEjIovTXSrAnPNx6hlgyCZ7o6omOdYOldGIZhAziEmOTSfigLV0RYAB9y9f/7kO8L3WUaQyhCgz0dmCL9CwCw172HgBeyG6oloC8fAAAAAASUVORK5CYII="; + private const string s_MirrorY = "iVBORw0KGgoAAAANSUhEUgAAAA8AAAAPCAYAAAA71pVKAAAABGdBTUEAALGPC/xhBQAAAAlwSFlzAAAOwgAADsIBFShKgAAAABh0RVh0U29mdHdhcmUAcGFpbnQubmV0IDQuMC41ZYUyZQAAAG9JREFUOE+djckNACEMAykoLdAjHbPyw1IOJ0L7mAejjFlm9hspyd77Kk+kBAjPOXcakJIh6QaKyOE0EB5dSPJAiUmOiL8PMVGxugsP/0OOib8vsY8yYwy6gRyC8CB5QIWgCMKBLgRSkikEUr5h6wOPWfMoCYILdgAAAABJRU5ErkJggg=="; + private const string s_MirrorXY = "iVBORw0KGgoAAAANSUhEUgAAAA8AAAAPCAYAAAA71pVKAAAABGdBTUEAALGPC/xhBQAAAAlwSFlzAAAOwgAADsIBFShKgAAAABl0RVh0U29mdHdhcmUAcGFpbnQubmV0IDQuMC4yMfEgaZUAAAHkSURBVDhPrVJLSwJRFJ4cdXwjPlrVJly1kB62cpEguElXKgYKIpaC+EIEEfGxLqI/UES1KaJlEdGmRY9ltCsIWrUJatGm0eZO3xkHIsJdH3zce+ec75z5zr3cf2MMmLdYLA/BYFA2mUyPOPvwnR+GR4PXaDQLLpfrKpVKSb1eT6bV6XTeocAS4sIw7S804BzEZ4IgsGq1ykhcr9dlj8czwPdbxJdBMyX/As/zLiz74Ar2J9lsVulcKpUYut5DnEbsHFwEx8AhtFqtGViD6BOc1ul0B5lMRhGXy2Wm1+ufkBOE/2fsL1FsQpXCiCAcQiAlk0kJRZjf7+9TRxI3Gg0WCoW+IpGISHHERBS5UKUch8n2K5WK3O125VqtpqydTkdZie12W261WjIVo73b7RZVKccZDIZ1q9XaT6fTLB6PD9BFKhQKjITFYpGFw+FBNBpVOgcCARH516pUGZYZXk5R4B3efLBxDM9f1CkWi/WR3ICtGVh6Rd4NPE+p0iEgmkSRLRoMEjYhHpA4kUiIOO8iZRU8AmnadK2/QOOfhnjPZrO95fN5Zdq5XE5yOBwvuKoNxGfBkQ8FzXkPprnj9Xrfm82mDI8fsLON3x5H/Od+RwHdLfDds9vtn0aj8QoF6QH9JzjuG3acpxmu1RgPAAAAAElFTkSuQmCC"; + private const string s_Rotated = "iVBORw0KGgoAAAANSUhEUgAAAA8AAAAPCAYAAAA71pVKAAAABGdBTUEAALGPC/xhBQAAAAlwSFlzAAAOwQAADsEBuJFr7QAAABh0RVh0U29mdHdhcmUAcGFpbnQubmV0IDQuMC41ZYUyZQAAAHdJREFUOE+djssNwCAMQxmIFdgx+2S4Vj4YxWlQgcOT8nuG5u5C732Sd3lfLlmPMR4QhXgrTQaimUlA3EtD+CJlBuQ7aUAUMjEAv9gWCQNEPhHJUkYfZ1kEpcxDzioRzGIlr0Qwi0r+Q5rTgM+AAVcygHgt7+HtBZs/2QVWP8ahAAAAAElFTkSuQmCC"; + private const string s_Fixed = "iVBORw0KGgoAAAANSUhEUgAAAA8AAAAPCAYAAAA71pVKAAAAAXNSR0IArs4c6QAAAARnQU1BAACxjwv8YQUAAAAJcEhZcwAADsMAAA7DAcdvqGQAAAAZdEVYdFNvZnR3YXJlAHBhaW50Lm5ldCA0LjAuMjHxIGmVAAAA50lEQVQ4T51Ruw6CQBCkwBYKWkIgQAs9gfgCvgb4BML/qWBM9Bdo9QPIuVOQ3JIzosVkc7Mzty9NCPE3lORaKMm1YA/LsnTXdbdhGJ6iKHoVRTEi+r4/OI6zN01Tl/XM7HneLsuyW13XU9u2ous6gYh3kiR327YPsp6ZgyDom6aZYFqiqqqJ8mdZz8xoca64BHjkZT0zY0aVcQbysp6Z4zj+Vvkp65mZttxjOSozdkEzD7KemekcxzRNHxDOHSDiQ/DIy3pmpjtuSJBThStGKMtyRKSOLnSm3DCMz3f+FUpyLZTkOgjtDSWORSDbpbmNAAAAAElFTkSuQmCC"; + + private static readonly string k_UndoName = L10n.Tr("Change RuleTile"); + + private static Texture2D[] s_Arrows; + + /// <summary> + /// Array of arrow textures used for marking positions for Rule matches + /// </summary> + public static Texture2D[] arrows + { + get + { + if (s_Arrows == null) + { + s_Arrows = new Texture2D[10]; + s_Arrows[0] = Base64ToTexture(s_Arrow0); + s_Arrows[1] = Base64ToTexture(s_Arrow1); + s_Arrows[2] = Base64ToTexture(s_Arrow2); + s_Arrows[3] = Base64ToTexture(s_Arrow3); + s_Arrows[5] = Base64ToTexture(s_Arrow5); + s_Arrows[6] = Base64ToTexture(s_Arrow6); + s_Arrows[7] = Base64ToTexture(s_Arrow7); + s_Arrows[8] = Base64ToTexture(s_Arrow8); + s_Arrows[9] = Base64ToTexture(s_XIconString); + } + return s_Arrows; + } + } + + private static Texture2D[] s_AutoTransforms; + /// <summary> + /// Arrays of textures used for marking transform Rule matches + /// </summary> + public static Texture2D[] autoTransforms + { + get + { + if (s_AutoTransforms == null) + { + s_AutoTransforms = new Texture2D[5]; + s_AutoTransforms[0] = Base64ToTexture(s_Rotated); + s_AutoTransforms[1] = Base64ToTexture(s_MirrorX); + s_AutoTransforms[2] = Base64ToTexture(s_MirrorY); + s_AutoTransforms[3] = Base64ToTexture(s_Fixed); + s_AutoTransforms[4] = Base64ToTexture(s_MirrorXY); + } + return s_AutoTransforms; + } + } + + private static class Styles + { + public static readonly GUIContent defaultSprite = EditorGUIUtility.TrTextContent("Default Sprite" + , "The default Sprite set when creating a new Rule."); + public static readonly GUIContent defaultGameObject = EditorGUIUtility.TrTextContent("Default GameObject" + , "The default GameObject set when creating a new Rule."); + public static readonly GUIContent defaultCollider = EditorGUIUtility.TrTextContent("Default Collider" + , "The default Collider Type set when creating a new Rule."); + + public static readonly GUIContent emptyRuleTileInfo = + EditorGUIUtility.TrTextContent( + "Drag Sprite or Sprite Texture assets \n" + + " to start creating a Rule Tile."); + + public static readonly GUIContent extendNeighbor = EditorGUIUtility.TrTextContent("Extend Neighbor" + , "Enabling this allows you to increase the range of neighbors beyond the 3x3 box."); + + public static readonly GUIContent numberOfTilingRules = EditorGUIUtility.TrTextContent( + "Number of Tiling Rules" + , "Change this to adjust of the number of tiling rules."); + + public static readonly GUIContent tilingRules = EditorGUIUtility.TrTextContent("Tiling Rules"); + public static readonly GUIContent tilingRulesGameObject = EditorGUIUtility.TrTextContent("GameObject" + , "The GameObject for the Tile which fits this Rule."); + public static readonly GUIContent tilingRulesCollider = EditorGUIUtility.TrTextContent("Collider" + , "The Collider Type for the Tile which fits this Rule"); + public static readonly GUIContent tilingRulesOutput = EditorGUIUtility.TrTextContent("Output" + , "The Output for the Tile which fits this Rule. Each Output type has its own properties."); + + public static readonly GUIContent tilingRulesNoise = EditorGUIUtility.TrTextContent("Noise" + , "The Perlin noise factor when placing the Tile."); + public static readonly GUIContent tilingRulesShuffle = EditorGUIUtility.TrTextContent("Shuffle" + , "The randomized transform given to the Tile when placing it."); + public static readonly GUIContent tilingRulesRandomSize = EditorGUIUtility.TrTextContent("Size" + , "The number of Sprites to randomize from."); + + public static readonly GUIContent tilingRulesMinSpeed = EditorGUIUtility.TrTextContent("Min Speed" + , "The minimum speed at which the animation is played."); + public static readonly GUIContent tilingRulesMaxSpeed = EditorGUIUtility.TrTextContent("Max Speed" + , "The maximum speed at which the animation is played."); + public static readonly GUIContent tilingRulesAnimationSize = EditorGUIUtility.TrTextContent("Size" + , "The number of Sprites in the animation."); + + public static readonly GUIStyle extendNeighborsLightStyle = new GUIStyle() + { + alignment = TextAnchor.MiddleLeft, + fontStyle = FontStyle.Bold, + fontSize = 10, + normal = new GUIStyleState() + { + textColor = Color.black + } + }; + + public static readonly GUIStyle extendNeighborsDarkStyle = new GUIStyle() + { + alignment = TextAnchor.MiddleLeft, + fontStyle = FontStyle.Bold, + fontSize = 10, + normal = new GUIStyleState() + { + textColor = Color.white + } + }; + } + + /// <summary> + /// The RuleTile being edited + /// </summary> + public RuleTile tile => target as RuleTile; + + /// <summary> + /// List of Sprites for Drag and Drop + /// </summary> + private List<Sprite> dragAndDropSprites; + + /// <summary> + /// Reorderable list for Rules + /// </summary> + private ReorderableList m_ReorderableList; + /// <summary> + /// Whether the RuleTile can extend its neighbors beyond directly adjacent ones + /// </summary> + public bool extendNeighbor; + + /// <summary> + /// Preview Utility for rendering previews + /// </summary> + public PreviewRenderUtility m_PreviewUtility; + /// <summary> + /// Grid for rendering previews + /// </summary> + public Grid m_PreviewGrid; + /// <summary> + /// List of Tilemaps for rendering previews + /// </summary> + public List<Tilemap> m_PreviewTilemaps; + /// <summary> + /// List of TilemapRenderers for rendering previews + /// </summary> + public List<TilemapRenderer> m_PreviewTilemapRenderers; + + /// <summary> + /// Default height for a Rule Element + /// </summary> + public const float k_DefaultElementHeight = 48f; + /// <summary> + /// Padding between Rule Elements + /// </summary> + public const float k_PaddingBetweenRules = 8f; + /// <summary> + /// Single line height + /// </summary> + public const float k_SingleLineHeight = 18f; + /// <summary> + /// Width for labels + /// </summary> + public const float k_LabelWidth = 80f; + + private SerializedProperty m_TilingRules; + + private MethodInfo m_ClearCacheMethod; + + /// <summary> + /// OnEnable for the RuleTileEditor + /// </summary> + public virtual void OnEnable() + { + m_ReorderableList = new ReorderableList(tile != null ? tile.m_TilingRules : null, typeof(RuleTile.TilingRule), true, true, true, true); + m_ReorderableList.drawHeaderCallback = OnDrawHeader; + m_ReorderableList.drawElementCallback = OnDrawElement; + m_ReorderableList.elementHeightCallback = GetElementHeight; + m_ReorderableList.onChangedCallback = ListUpdated; + m_ReorderableList.onAddDropdownCallback = OnAddDropdownElement; + + // Required to adjust element height changes + var rolType = GetType("UnityEditorInternal.ReorderableList"); + if (rolType != null) + { + // ClearCache was changed to InvalidateCache in newer versions of Unity. + // To maintain backwards compatibility, we will attempt to retrieve each method in order + m_ClearCacheMethod = rolType.GetMethod("InvalidateCache", BindingFlags.Instance | BindingFlags.NonPublic); + if (m_ClearCacheMethod == null) + m_ClearCacheMethod = rolType.GetMethod("ClearCache", BindingFlags.Instance | BindingFlags.NonPublic); + } + + m_TilingRules = serializedObject.FindProperty("m_TilingRules"); + } + + /// <summary> + /// OnDisable for the RuleTileEditor + /// </summary> + public virtual void OnDisable() + { + DestroyPreview(); + } + + private void UpdateTilingRuleIds() + { + var existingIdSet = new HashSet<int>(); + var usedIdSet = new HashSet<int>(); + foreach (var rule in tile.m_TilingRules) + { + existingIdSet.Add(rule.m_Id); + } + foreach (var rule in tile.m_TilingRules) + { + if (usedIdSet.Contains(rule.m_Id)) + { + while (existingIdSet.Contains(rule.m_Id)) + rule.m_Id++; + existingIdSet.Add(rule.m_Id); + } + usedIdSet.Add(rule.m_Id); + } + } + + /// <summary> + /// Get the GUI bounds for a Rule. + /// </summary> + /// <param name="bounds">Cell bounds of the Rule.</param> + /// <param name="rule">Rule to get GUI bounds for.</param> + /// <returns>The GUI bounds for a rule.</returns> + public virtual BoundsInt GetRuleGUIBounds(BoundsInt bounds, RuleTile.TilingRule rule) + { + if (extendNeighbor) + { + bounds.xMin--; + bounds.yMin--; + bounds.xMax++; + bounds.yMax++; + } + bounds.xMin = Mathf.Min(bounds.xMin, -1); + bounds.yMin = Mathf.Min(bounds.yMin, -1); + bounds.xMax = Mathf.Max(bounds.xMax, 2); + bounds.yMax = Mathf.Max(bounds.yMax, 2); + return bounds; + } + + /// <summary> + /// Callback when the Rule list is updated + /// </summary> + /// <param name="list">Reorderable list for Rules</param> + public void ListUpdated(ReorderableList list) + { + UpdateTilingRuleIds(); + } + + private float GetElementHeight(int index) + { + RuleTile.TilingRule rule = tile.m_TilingRules[index]; + return GetElementHeight(rule); + } + + /// <summary> + /// Gets the GUI element height for a TilingRule + /// </summary> + /// <param name="rule">Rule to get height for</param> + /// <returns>GUI element height for a TilingRule</returns> + public float GetElementHeight(RuleTile.TilingRule rule) + { + BoundsInt bounds = GetRuleGUIBounds(rule.GetBounds(), rule); + + float inspectorHeight = GetElementHeight(rule as RuleTile.TilingRuleOutput); + float matrixHeight = GetMatrixSize(bounds).y + 10f; + + return Mathf.Max(inspectorHeight, matrixHeight); + } + + /// <summary> + /// Gets the GUI element height for a TilingRuleOutput + /// </summary> + /// <param name="rule">Rule to get height for</param> + /// <returns>GUI element height for a TilingRuleOutput </returns> + public float GetElementHeight(RuleTile.TilingRuleOutput rule) + { + float inspectorHeight = k_DefaultElementHeight + k_PaddingBetweenRules; + + switch (rule.m_Output) + { + case RuleTile.TilingRuleOutput.OutputSprite.Random: + case RuleTile.TilingRuleOutput.OutputSprite.Animation: + inspectorHeight = k_DefaultElementHeight + k_SingleLineHeight * (rule.m_Sprites.Length + 3) + k_PaddingBetweenRules; + break; + } + + return inspectorHeight; + } + + /// <summary> + /// Gets the GUI matrix size for a Rule of a RuleTile + /// </summary> + /// <param name="bounds">Cell bounds of the Rule.</param> + /// <returns>Returns the GUI matrix size for a Rule of a RuleTile.</returns> + public virtual Vector2 GetMatrixSize(BoundsInt bounds) + { + return new Vector2(bounds.size.x * k_SingleLineHeight, bounds.size.y * k_SingleLineHeight); + } + + /// <summary> + /// Draws the Rule element for the Rule list + /// </summary> + /// <param name="rect">Rect to draw the Rule Element in</param> + /// <param name="index">Index of the Rule Element to draw</param> + /// <param name="isactive">Whether the Rule Element is active</param> + /// <param name="isfocused">Whether the Rule Element is focused</param> + protected virtual void OnDrawElement(Rect rect, int index, bool isactive, bool isfocused) + { + RuleTile.TilingRule rule = tile.m_TilingRules[index]; + BoundsInt bounds = GetRuleGUIBounds(rule.GetBounds(), rule); + + float yPos = rect.yMin + 2f; + float height = rect.height - k_PaddingBetweenRules; + Vector2 matrixSize = GetMatrixSize(bounds); + + Rect spriteRect = new Rect(rect.xMax - k_DefaultElementHeight - 5f, yPos, k_DefaultElementHeight, k_DefaultElementHeight); + Rect matrixRect = new Rect(rect.xMax - matrixSize.x - spriteRect.width - 10f, yPos, matrixSize.x, matrixSize.y); + Rect inspectorRect = new Rect(rect.xMin, yPos, rect.width - matrixSize.x - spriteRect.width - 20f, height); + + RuleInspectorOnGUI(inspectorRect, rule); + RuleMatrixOnGUI(tile, matrixRect, bounds, rule); + SpriteOnGUI(spriteRect, rule); + } + + private void OnAddElement(object obj) + { + var list = obj as ReorderableList; + RuleTile.TilingRule rule = new RuleTile.TilingRule(); + rule.m_Output = RuleTile.TilingRuleOutput.OutputSprite.Single; + rule.m_Sprites[0] = tile.m_DefaultSprite; + rule.m_GameObject = tile.m_DefaultGameObject; + rule.m_ColliderType = tile.m_DefaultColliderType; + + var count = m_TilingRules.arraySize; + ResizeRuleTileList(count + 1); + + if (list.index == -1 || list.index >= list.count) + tile.m_TilingRules[count] = rule; + else + { + tile.m_TilingRules.Insert(list.index + 1, rule); + tile.m_TilingRules.RemoveAt(count + 1); + if (list.IsSelected(list.index)) + list.index += 1; + } + UpdateTilingRuleIds(); + } + + private void OnDuplicateElement(object obj) + { + var list = obj as ReorderableList; + if (list.index < 0 || list.index >= tile.m_TilingRules.Count) + return; + + var copyRule = tile.m_TilingRules[list.index]; + var rule = copyRule.Clone(); + + var count = m_TilingRules.arraySize; + ResizeRuleTileList(count + 1); + + tile.m_TilingRules.Insert(list.index + 1, rule); + tile.m_TilingRules.RemoveAt(count + 1); + if (list.IsSelected(list.index)) + list.index += 1; + UpdateTilingRuleIds(); + } + + private void OnAddDropdownElement(Rect rect, ReorderableList list) + { + if (0 <= list.index && list.index < tile.m_TilingRules.Count && list.IsSelected(list.index)) + { + GenericMenu menu = new GenericMenu(); + menu.AddItem(EditorGUIUtility.TrTextContent("Add"), false, OnAddElement, list); + menu.AddItem(EditorGUIUtility.TrTextContent("Duplicate"), false, OnDuplicateElement, list); + menu.DropDown(rect); + } + else + { + OnAddElement(list); + } + } + + /// <summary> + /// Saves any changes to the RuleTile + /// </summary> + public void SaveTile() + { + serializedObject.ApplyModifiedProperties(); + EditorUtility.SetDirty(target); + SceneView.RepaintAll(); + + UpdateAffectedOverrideTiles(tile); + } + + /// <summary> + /// Updates all RuleOverrideTiles which override the given RUleTile + /// </summary> + /// <param name="target">RuleTile which has been updated</param> + public static void UpdateAffectedOverrideTiles(RuleTile target) + { + List<RuleOverrideTile> overrideTiles = FindAffectedOverrideTiles(target); + if (overrideTiles != null) + { + foreach (var overrideTile in overrideTiles) + { + Undo.RegisterCompleteObjectUndo(overrideTile, k_UndoName); + Undo.RecordObject(overrideTile.m_InstanceTile, k_UndoName); + overrideTile.Override(); + UpdateAffectedOverrideTiles(overrideTile.m_InstanceTile); + EditorUtility.SetDirty(overrideTile); + } + } + } + + /// <summary> + /// Gets all RuleOverrideTiles which override the given RuleTile + /// </summary> + /// <param name="target">RuleTile which has been updated</param> + /// <returns>A list of RuleOverrideTiles which override the given RuleTile</returns> + public static List<RuleOverrideTile> FindAffectedOverrideTiles(RuleTile target) + { + List<RuleOverrideTile> overrideTiles = new List<RuleOverrideTile>(); + + string[] overrideTileGuids = AssetDatabase.FindAssets("t:" + typeof(RuleOverrideTile).Name); + foreach (string overrideTileGuid in overrideTileGuids) + { + string overrideTilePath = AssetDatabase.GUIDToAssetPath(overrideTileGuid); + RuleOverrideTile overrideTile = AssetDatabase.LoadAssetAtPath<RuleOverrideTile>(overrideTilePath); + if (overrideTile.m_Tile == target) + { + overrideTiles.Add(overrideTile); + } + } + + return overrideTiles; + } + + /// <summary> + /// Draws the header for the Rule list + /// </summary> + /// <param name="rect">GUI Rect to draw the header at</param> + public void OnDrawHeader(Rect rect) + { + GUI.Label(rect, Styles.tilingRules); + + var toggleRect = new Rect(rect.xMax - rect.height, rect.y, rect.height, rect.height); + + var style = EditorGUIUtility.isProSkin ? Styles.extendNeighborsDarkStyle : Styles.extendNeighborsLightStyle; + var extendSize = style.CalcSize(Styles.extendNeighbor); + var toggleWidth = toggleRect.width + extendSize.x + 5f; + var toggleLabelRect = new Rect(rect.x + rect.width - toggleWidth, rect.y, toggleWidth, rect.height); + + EditorGUI.BeginChangeCheck(); + extendNeighbor = EditorGUI.Toggle(toggleRect, extendNeighbor); + EditorGUI.LabelField(toggleLabelRect, Styles.extendNeighbor, style); + if (EditorGUI.EndChangeCheck()) + { + if (m_ClearCacheMethod != null) + m_ClearCacheMethod.Invoke(m_ReorderableList, null); + } + } + + /// <summary> + /// Draws the Inspector GUI for the RuleTileEditor + /// </summary> + public override void OnInspectorGUI() + { + serializedObject.Update(); + Undo.RecordObject(target, k_UndoName); + + EditorGUI.BeginChangeCheck(); + + tile.m_DefaultSprite = EditorGUILayout.ObjectField(Styles.defaultSprite, tile.m_DefaultSprite, typeof(Sprite), false) as Sprite; + tile.m_DefaultGameObject = EditorGUILayout.ObjectField(Styles.defaultGameObject, tile.m_DefaultGameObject, typeof(GameObject), false) as GameObject; + tile.m_DefaultColliderType = (Tile.ColliderType)EditorGUILayout.EnumPopup(Styles.defaultCollider, tile.m_DefaultColliderType); + + DrawCustomFields(false); + + EditorGUILayout.Space(); + + EditorGUI.BeginChangeCheck(); + int count = EditorGUILayout.DelayedIntField(Styles.numberOfTilingRules, tile.m_TilingRules?.Count ?? 0); + if (count < 0) + count = 0; + if (EditorGUI.EndChangeCheck()) + ResizeRuleTileList(count); + + if (count == 0) + { + Rect rect = EditorGUILayout.GetControlRect(false, EditorGUIUtility.singleLineHeight * 5); + HandleDragAndDrop(rect); + EditorGUI.DrawRect(rect, dragAndDropActive && rect.Contains(Event.current.mousePosition) ? Color.white : Color.black); + var innerRect = new Rect(rect.x + 1, rect.y + 1, rect.width - 2, rect.height - 2); + EditorGUI.DrawRect(innerRect, EditorGUIUtility.isProSkin + ? (Color) new Color32 (56, 56, 56, 255) + : (Color) new Color32 (194, 194, 194, 255)); + DisplayClipboardText(Styles.emptyRuleTileInfo, rect); + GUILayout.Space(rect.height); + EditorGUILayout.Space(); + } + + if (m_ReorderableList != null) + m_ReorderableList.DoLayoutList(); + + if (EditorGUI.EndChangeCheck()) + SaveTile(); + + GUILayout.Space(k_DefaultElementHeight); + } + + private void ResizeRuleTileList(int count) + { + if (m_TilingRules.arraySize == count) + return; + + var isEmpty = m_TilingRules.arraySize == 0; + m_TilingRules.arraySize = count; + serializedObject.ApplyModifiedProperties(); + if (isEmpty) + { + for (int i = 0; i < count; ++i) + tile.m_TilingRules[i] = new RuleTile.TilingRule(); + } + UpdateTilingRuleIds(); + } + + /// <summary> + /// Draw editor fields for custom properties for the RuleTile + /// </summary> + /// <param name="isOverrideInstance">Whether override fields are drawn</param> + public void DrawCustomFields(bool isOverrideInstance) + { + var customFields = tile.GetCustomFields(isOverrideInstance); + + serializedObject.Update(); + EditorGUI.BeginChangeCheck(); + foreach (var field in customFields) + { + var property = serializedObject.FindProperty(field.Name); + if (property != null) + EditorGUILayout.PropertyField(property, true); + } + + if (EditorGUI.EndChangeCheck()) + { + serializedObject.ApplyModifiedProperties(); + DestroyPreview(); + CreatePreview(); + } + + } + + /// <summary> + /// Gets the index for a Rule with the RuleTile to display an arrow. + /// </summary> + /// <param name="position">The relative position of the arrow from the center.</param> + /// <returns>Returns the index for a Rule with the RuleTile to display an arrow.</returns> + public virtual int GetArrowIndex(Vector3Int position) + { + if (Mathf.Abs(position.x) == Mathf.Abs(position.y)) + { + if (position.x < 0 && position.y > 0) + return 0; + else if (position.x > 0 && position.y > 0) + return 2; + else if (position.x < 0 && position.y < 0) + return 6; + else if (position.x > 0 && position.y < 0) + return 8; + } + else if (Mathf.Abs(position.x) > Mathf.Abs(position.y)) + { + if (position.x > 0) + return 5; + else + return 3; + } + else + { + if (position.y > 0) + return 1; + else + return 7; + } + return -1; + } + + /// <summary> + /// Draws a neighbor matching rule + /// </summary> + /// <param name="rect">Rect to draw on</param> + /// <param name="position">The relative position of the arrow from the center</param> + /// <param name="neighbor">The index to the neighbor matching criteria</param> + public virtual void RuleOnGUI(Rect rect, Vector3Int position, int neighbor) + { + switch (neighbor) + { + case RuleTile.TilingRuleOutput.Neighbor.This: + GUI.DrawTexture(rect, arrows[GetArrowIndex(position)]); + break; + case RuleTile.TilingRuleOutput.Neighbor.NotThis: + GUI.DrawTexture(rect, arrows[9]); + break; + default: + var style = new GUIStyle(); + style.alignment = TextAnchor.MiddleCenter; + style.fontSize = 10; + GUI.Label(rect, neighbor.ToString(), style); + break; + } + } + + /// <summary> + /// Draws a tooltip for the neighbor matching rule + /// </summary> + /// <param name="rect">Rect to draw on</param> + /// <param name="neighbor">The index to the neighbor matching criteria</param> + public void RuleTooltipOnGUI(Rect rect, int neighbor) + { + var allConsts = tile.m_NeighborType.GetFields(BindingFlags.Public | BindingFlags.Static | BindingFlags.FlattenHierarchy); + foreach (var c in allConsts) + { + if ((int)c.GetValue(null) == neighbor) + { + GUI.Label(rect, new GUIContent("", c.Name)); + break; + } + } + } + + /// <summary> + /// Draws a transform matching rule + /// </summary> + /// <param name="rect">Rect to draw on</param> + /// <param name="ruleTransform">The transform matching criteria</param> + public virtual void RuleTransformOnGUI(Rect rect, RuleTile.TilingRuleOutput.Transform ruleTransform) + { + switch (ruleTransform) + { + case RuleTile.TilingRuleOutput.Transform.Rotated: + GUI.DrawTexture(rect, autoTransforms[0]); + break; + case RuleTile.TilingRuleOutput.Transform.MirrorX: + GUI.DrawTexture(rect, autoTransforms[1]); + break; + case RuleTile.TilingRuleOutput.Transform.MirrorY: + GUI.DrawTexture(rect, autoTransforms[2]); + break; + case RuleTile.TilingRuleOutput.Transform.Fixed: + GUI.DrawTexture(rect, autoTransforms[3]); + break; + case RuleTile.TilingRuleOutput.Transform.MirrorXY: + GUI.DrawTexture(rect, autoTransforms[4]); + break; + } + GUI.Label(rect, new GUIContent("", ruleTransform.ToString())); + } + + /// <summary> + /// Handles a neighbor matching Rule update from user mouse input + /// </summary> + /// <param name="rect">Rect containing neighbor matching Rule GUI</param> + /// <param name="tilingRule">Tiling Rule to update neighbor matching rule</param> + /// <param name="neighbors">A dictionary of neighbors</param> + /// <param name="position">The relative position of the neighbor matching Rule</param> + public void RuleNeighborUpdate(Rect rect, RuleTile.TilingRule tilingRule, Dictionary<Vector3Int, int> neighbors, Vector3Int position) + { + if (Event.current.type == EventType.MouseDown && ContainsMousePosition(rect)) + { + var allConsts = tile.m_NeighborType.GetFields(BindingFlags.Public | BindingFlags.Static | BindingFlags.FlattenHierarchy); + var neighborConsts = allConsts.Select(c => (int)c.GetValue(null)).ToList(); + neighborConsts.Sort(); + + if (neighbors.ContainsKey(position)) + { + int oldIndex = neighborConsts.IndexOf(neighbors[position]); + int newIndex = oldIndex + GetMouseChange(); + if (newIndex >= 0 && newIndex < neighborConsts.Count) + { + newIndex = (int)Mathf.Repeat(newIndex, neighborConsts.Count); + neighbors[position] = neighborConsts[newIndex]; + } + else + { + neighbors.Remove(position); + } + } + else + { + neighbors.Add(position, neighborConsts[GetMouseChange() == 1 ? 0 : (neighborConsts.Count - 1)]); + } + tilingRule.ApplyNeighbors(neighbors); + + GUI.changed = true; + Event.current.Use(); + } + } + + /// <summary> + /// Handles a transform matching Rule update from user mouse input + /// </summary> + /// <param name="rect">Rect containing transform matching Rule GUI</param> + /// <param name="tilingRule">Tiling Rule to update transform matching rule</param> + public void RuleTransformUpdate(Rect rect, RuleTile.TilingRule tilingRule) + { + if (Event.current.type == EventType.MouseDown && ContainsMousePosition(rect)) + { + tilingRule.m_RuleTransform = (RuleTile.TilingRuleOutput.Transform)(int)Mathf.Repeat((int)tilingRule.m_RuleTransform + GetMouseChange(), Enum.GetValues(typeof(RuleTile.TilingRule.Transform)).Length); + GUI.changed = true; + Event.current.Use(); + } + } + + /// <summary> + /// Determines the current mouse position is within the given Rect. + /// </summary> + /// <param name="rect">Rect to test mouse position for.</param> + /// <returns>True if the current mouse position is within the given Rect. False otherwise.</returns> + public virtual bool ContainsMousePosition(Rect rect) + { + return rect.Contains(Event.current.mousePosition); + } + + /// <summary> + /// Gets the offset change for a mouse click input + /// </summary> + /// <returns>The offset change for a mouse click input</returns> + public static int GetMouseChange() + { + return Event.current.button == 1 ? -1 : 1; + } + + /// <summary> + /// Draws a Rule Matrix for the given Rule for a RuleTile. + /// </summary> + /// <param name="tile">Tile to draw rule for.</param> + /// <param name="rect">GUI Rect to draw rule at.</param> + /// <param name="bounds">Cell bounds of the Rule.</param> + /// <param name="tilingRule">Rule to draw Rule Matrix for.</param> + public virtual void RuleMatrixOnGUI(RuleTile tile, Rect rect, BoundsInt bounds, RuleTile.TilingRule tilingRule) + { + Handles.color = EditorGUIUtility.isProSkin ? new Color(1f, 1f, 1f, 0.2f) : new Color(0f, 0f, 0f, 0.2f); + float w = rect.width / bounds.size.x; + float h = rect.height / bounds.size.y; + + for (int y = 0; y <= bounds.size.y; y++) + { + float top = rect.yMin + y * h; + Handles.DrawLine(new Vector3(rect.xMin, top), new Vector3(rect.xMax, top)); + } + for (int x = 0; x <= bounds.size.x; x++) + { + float left = rect.xMin + x * w; + Handles.DrawLine(new Vector3(left, rect.yMin), new Vector3(left, rect.yMax)); + } + Handles.color = Color.white; + + var neighbors = tilingRule.GetNeighbors(); + + for (int y = bounds.yMin; y < bounds.yMax; y++) + { + for (int x = bounds.xMin; x < bounds.xMax; x++) + { + Vector3Int pos = new Vector3Int(x, y, 0); + Rect r = new Rect(rect.xMin + (x - bounds.xMin) * w, rect.yMin + (-y + bounds.yMax - 1) * h, w - 1, h - 1); + RuleMatrixIconOnGUI(tilingRule, neighbors, pos, r); + } + } + } + + /// <summary> + /// Draws a Rule Matrix Icon for the given matching Rule for a RuleTile with the given position + /// </summary> + /// <param name="tilingRule">Tile to draw rule for.</param> + /// <param name="neighbors">A dictionary of neighbors</param> + /// <param name="position">The relative position of the neighbor matching Rule</param> + /// <param name="rect">GUI Rect to draw icon at</param> + public void RuleMatrixIconOnGUI(RuleTile.TilingRule tilingRule, Dictionary<Vector3Int, int> neighbors, Vector3Int position, Rect rect) + { + using (var check = new EditorGUI.ChangeCheckScope()) + { + if (position.x != 0 || position.y != 0) + { + if (neighbors.ContainsKey(position)) + { + RuleOnGUI(rect, position, neighbors[position]); + RuleTooltipOnGUI(rect, neighbors[position]); + } + RuleNeighborUpdate(rect, tilingRule, neighbors, position); + } + else + { + RuleTransformOnGUI(rect, tilingRule.m_RuleTransform); + RuleTransformUpdate(rect, tilingRule); + } + if (check.changed) + { + tile.UpdateNeighborPositions(); + } + } + } + + /// <summary> + /// Draws a Sprite field for the Rule + /// </summary> + /// <param name="rect">Rect to draw Sprite Inspector in</param> + /// <param name="tilingRule">Rule to draw Sprite Inspector for</param> + public virtual void SpriteOnGUI(Rect rect, RuleTile.TilingRuleOutput tilingRule) + { + tilingRule.m_Sprites[0] = EditorGUI.ObjectField(rect, tilingRule.m_Sprites[0], typeof(Sprite), false) as Sprite; + } + + /// <summary> + /// Draws an Inspector for the Rule + /// </summary> + /// <param name="rect">Rect to draw Inspector in</param> + /// <param name="tilingRule">Rule to draw Inspector for</param> + public void RuleInspectorOnGUI(Rect rect, RuleTile.TilingRuleOutput tilingRule) + { + float y = rect.yMin; + GUI.Label(new Rect(rect.xMin, y, k_LabelWidth, k_SingleLineHeight), Styles.tilingRulesGameObject); + tilingRule.m_GameObject = (GameObject)EditorGUI.ObjectField(new Rect(rect.xMin + k_LabelWidth, y, rect.width - k_LabelWidth, k_SingleLineHeight), "", tilingRule.m_GameObject, typeof(GameObject), false); + y += k_SingleLineHeight; + GUI.Label(new Rect(rect.xMin, y, k_LabelWidth, k_SingleLineHeight), Styles.tilingRulesCollider); + tilingRule.m_ColliderType = (Tile.ColliderType)EditorGUI.EnumPopup(new Rect(rect.xMin + k_LabelWidth, y, rect.width - k_LabelWidth, k_SingleLineHeight), tilingRule.m_ColliderType); + y += k_SingleLineHeight; + GUI.Label(new Rect(rect.xMin, y, k_LabelWidth, k_SingleLineHeight), Styles.tilingRulesOutput); + tilingRule.m_Output = (RuleTile.TilingRuleOutput.OutputSprite)EditorGUI.EnumPopup(new Rect(rect.xMin + k_LabelWidth, y, rect.width - k_LabelWidth, k_SingleLineHeight), tilingRule.m_Output); + y += k_SingleLineHeight; + + if (tilingRule.m_Output == RuleTile.TilingRuleOutput.OutputSprite.Animation) + { + GUI.Label(new Rect(rect.xMin, y, k_LabelWidth, k_SingleLineHeight), Styles.tilingRulesMinSpeed); + tilingRule.m_MinAnimationSpeed = EditorGUI.FloatField(new Rect(rect.xMin + k_LabelWidth, y, rect.width - k_LabelWidth, k_SingleLineHeight), tilingRule.m_MinAnimationSpeed); + y += k_SingleLineHeight; + GUI.Label(new Rect(rect.xMin, y, k_LabelWidth, k_SingleLineHeight), Styles.tilingRulesMaxSpeed); + tilingRule.m_MaxAnimationSpeed = EditorGUI.FloatField(new Rect(rect.xMin + k_LabelWidth, y, rect.width - k_LabelWidth, k_SingleLineHeight), tilingRule.m_MaxAnimationSpeed); + y += k_SingleLineHeight; + } + if (tilingRule.m_Output == RuleTile.TilingRuleOutput.OutputSprite.Random) + { + GUI.Label(new Rect(rect.xMin, y, k_LabelWidth, k_SingleLineHeight), Styles.tilingRulesNoise); + tilingRule.m_PerlinScale = EditorGUI.Slider(new Rect(rect.xMin + k_LabelWidth, y, rect.width - k_LabelWidth, k_SingleLineHeight), tilingRule.m_PerlinScale, 0.001f, 0.999f); + y += k_SingleLineHeight; + + GUI.Label(new Rect(rect.xMin, y, k_LabelWidth, k_SingleLineHeight), Styles.tilingRulesShuffle); + tilingRule.m_RandomTransform = (RuleTile.TilingRuleOutput.Transform)EditorGUI.EnumPopup(new Rect(rect.xMin + k_LabelWidth, y, rect.width - k_LabelWidth, k_SingleLineHeight), tilingRule.m_RandomTransform); + y += k_SingleLineHeight; + } + + if (tilingRule.m_Output != RuleTile.TilingRuleOutput.OutputSprite.Single) + { + GUI.Label(new Rect(rect.xMin, y, k_LabelWidth, k_SingleLineHeight) + , tilingRule.m_Output == RuleTile.TilingRuleOutput.OutputSprite.Animation ? Styles.tilingRulesAnimationSize : Styles.tilingRulesRandomSize); + EditorGUI.BeginChangeCheck(); + int newLength = EditorGUI.DelayedIntField(new Rect(rect.xMin + k_LabelWidth, y, rect.width - k_LabelWidth, k_SingleLineHeight), tilingRule.m_Sprites.Length); + if (EditorGUI.EndChangeCheck()) + Array.Resize(ref tilingRule.m_Sprites, Math.Max(newLength, 1)); + y += k_SingleLineHeight; + + for (int i = 0; i < tilingRule.m_Sprites.Length; i++) + { + tilingRule.m_Sprites[i] = EditorGUI.ObjectField(new Rect(rect.xMin + k_LabelWidth, y, rect.width - k_LabelWidth, k_SingleLineHeight), tilingRule.m_Sprites[i], typeof(Sprite), false) as Sprite; + y += k_SingleLineHeight; + } + } + } + + private void DisplayClipboardText(GUIContent clipboardText, Rect position) + { + Color old = GUI.color; + GUI.color = Color.gray; + var infoSize = GUI.skin.label.CalcSize(clipboardText); + Rect rect = new Rect(position.center.x - infoSize.x * .5f + , position.center.y - infoSize.y * .5f + , infoSize.x + , infoSize.y); + GUI.Label(rect, clipboardText); + GUI.color = old; + } + + private bool dragAndDropActive + { + get + { + return dragAndDropSprites != null + && dragAndDropSprites.Count > 0; + } + } + + private static List<Sprite> GetSpritesFromTexture(Texture2D texture) + { + string path = AssetDatabase.GetAssetPath(texture); + Object[] assets = AssetDatabase.LoadAllAssetsAtPath(path); + List<Sprite> sprites = new List<Sprite>(); + + foreach (Object asset in assets) + { + if (asset is Sprite) + { + sprites.Add(asset as Sprite); + } + } + + return sprites; + } + + private static List<Sprite> GetValidSingleSprites(Object[] objects) + { + List<Sprite> result = new List<Sprite>(); + foreach (Object obj in objects) + { + if (obj is Sprite sprite) + { + result.Add(sprite); + } + else if (obj is Texture2D texture2D) + { + List<Sprite> sprites = GetSpritesFromTexture(texture2D); + if (sprites.Count > 0) + { + result.AddRange(sprites); + } + } + } + return result; + } + + private void HandleDragAndDrop(Rect guiRect) + { + if (DragAndDrop.objectReferences.Length == 0 || !guiRect.Contains(Event.current.mousePosition)) + return; + + switch (Event.current.type) + { + case EventType.DragUpdated: + { + dragAndDropSprites = GetValidSingleSprites(DragAndDrop.objectReferences); + if (dragAndDropActive) + { + DragAndDrop.visualMode = DragAndDropVisualMode.Copy; + Event.current.Use(); + GUI.changed = true; + } + } + break; + case EventType.DragPerform: + { + if (!dragAndDropActive) + return; + + Undo.RegisterCompleteObjectUndo(tile, "Drag and Drop to Rule Tile"); + ResizeRuleTileList(dragAndDropSprites.Count); + for (int i = 0; i < dragAndDropSprites.Count; ++i) + { + tile.m_TilingRules[i].m_Sprites[0] = dragAndDropSprites[i]; + } + DragAndDropClear(); + GUI.changed = true; + EditorUtility.SetDirty(tile); + GUIUtility.ExitGUI(); + } + break; + case EventType.Repaint: + // Handled in Render() + break; + } + + if (Event.current.type == EventType.DragExited || + Event.current.type == EventType.KeyDown && Event.current.keyCode == KeyCode.Escape) + { + DragAndDropClear(); + } + } + + private void DragAndDropClear() + { + dragAndDropSprites = null; + DragAndDrop.visualMode = DragAndDropVisualMode.None; + Event.current.Use(); + } + + /// <summary> + /// Whether the RuleTile has a preview GUI + /// </summary> + /// <returns>True</returns> + public override bool HasPreviewGUI() + { + return true; + } + + /// <summary> + /// Draws the preview GUI for the RuleTile + /// </summary> + /// <param name="rect">Rect to draw the preview GUI</param> + /// <param name="background">The GUIStyle of the background for the preview</param> + public override void OnPreviewGUI(Rect rect, GUIStyle background) + { + if (m_PreviewUtility == null) + CreatePreview(); + + if (Event.current.type != EventType.Repaint) + return; + + m_PreviewUtility.BeginPreview(rect, background); + m_PreviewUtility.camera.orthographicSize = 2; + if (rect.height > rect.width) + m_PreviewUtility.camera.orthographicSize *= rect.height / rect.width; + m_PreviewUtility.camera.Render(); + m_PreviewUtility.EndAndDrawPreview(rect); + } + + /// <summary> + /// Creates a Preview for the RuleTile. + /// </summary> + protected virtual void CreatePreview() + { + m_PreviewUtility = new PreviewRenderUtility(true); + m_PreviewUtility.camera.orthographic = true; + m_PreviewUtility.camera.orthographicSize = 2; + m_PreviewUtility.camera.transform.position = new Vector3(0, 0, -10); + + var previewInstance = new GameObject(); + m_PreviewGrid = previewInstance.AddComponent<Grid>(); + m_PreviewUtility.AddSingleGO(previewInstance); + + m_PreviewTilemaps = new List<Tilemap>(); + m_PreviewTilemapRenderers = new List<TilemapRenderer>(); + + for (int i = 0; i < 4; i++) + { + var previewTilemapGo = new GameObject(); + m_PreviewTilemaps.Add(previewTilemapGo.AddComponent<Tilemap>()); + m_PreviewTilemapRenderers.Add(previewTilemapGo.AddComponent<TilemapRenderer>()); + + previewTilemapGo.transform.SetParent(previewInstance.transform, false); + } + + for (int x = -2; x <= 0; x++) + for (int y = -1; y <= 1; y++) + m_PreviewTilemaps[0].SetTile(new Vector3Int(x, y, 0), tile); + + for (int y = -1; y <= 1; y++) + m_PreviewTilemaps[1].SetTile(new Vector3Int(1, y, 0), tile); + + for (int x = -2; x <= 0; x++) + m_PreviewTilemaps[2].SetTile(new Vector3Int(x, -2, 0), tile); + + m_PreviewTilemaps[3].SetTile(new Vector3Int(1, -2, 0), tile); + } + + /// <summary> + /// Handles cleanup for the Preview GUI + /// </summary> + protected virtual void DestroyPreview() + { + if (m_PreviewUtility != null) + { + m_PreviewUtility.Cleanup(); + m_PreviewUtility = null; + m_PreviewGrid = null; + m_PreviewTilemaps = null; + m_PreviewTilemapRenderers = null; + } + } + + /// <summary> + /// Renders a static preview Texture2D for a RuleTile asset + /// </summary> + /// <param name="assetPath">Asset path of the RuleTile</param> + /// <param name="subAssets">Arrays of assets from the given Asset path</param> + /// <param name="width">Width of the static preview</param> + /// <param name="height">Height of the static preview </param> + /// <returns>Texture2D containing static preview for the RuleTile asset</returns> + public override Texture2D RenderStaticPreview(string assetPath, Object[] subAssets, int width, int height) + { + if (tile.m_DefaultSprite != null) + { + Type t = GetType("UnityEditor.SpriteUtility"); + if (t != null) + { + MethodInfo method = t.GetMethod("RenderStaticPreview", new[] { typeof(Sprite), typeof(Color), typeof(int), typeof(int) }); + if (method != null) + { + object ret = method.Invoke("RenderStaticPreview", new object[] { tile.m_DefaultSprite, Color.white, width, height }); + if (ret is Texture2D) + return ret as Texture2D; + } + } + } + return base.RenderStaticPreview(assetPath, subAssets, width, height); + } + + private static Type GetType(string typeName) + { + var type = Type.GetType(typeName); + if (type != null) + return type; + + var currentAssembly = Assembly.GetExecutingAssembly(); + var referencedAssemblies = currentAssembly.GetReferencedAssemblies(); + foreach (var assemblyName in referencedAssemblies) + { + var assembly = Assembly.Load(assemblyName); + if (assembly != null) + { + type = assembly.GetType(typeName); + if (type != null) + return type; + } + } + return null; + } + + /// <summary> + /// Converts a Base64 string to a Texture2D + /// </summary> + /// <param name="base64">Base64 string containing image data</param> + /// <returns>Texture2D containing an image from the given Base64 string</returns> + public static Texture2D Base64ToTexture(string base64) + { + Texture2D t = new Texture2D(1, 1); + t.hideFlags = HideFlags.HideAndDontSave; + t.LoadImage(Convert.FromBase64String(base64)); + return t; + } + + /// <summary> + /// Wrapper for serializing a list of Rules + /// </summary> + [Serializable] + class RuleTileRuleWrapper + { + /// <summary> + /// List of Rules to serialize + /// </summary> + [SerializeField] + public List<RuleTile.TilingRule> rules = new List<RuleTile.TilingRule>(); + } + + /// <summary> + /// Copies all Rules from a RuleTile to the clipboard + /// </summary> + /// <param name="item">MenuCommand storing the RuleTile to copy from</param> + [MenuItem("CONTEXT/RuleTile/Copy All Rules")] + public static void CopyAllRules(MenuCommand item) + { + RuleTile tile = item.context as RuleTile; + if (tile == null) + return; + + RuleTileRuleWrapper rulesWrapper = new RuleTileRuleWrapper(); + rulesWrapper.rules = tile.m_TilingRules; + var rulesJson = EditorJsonUtility.ToJson(rulesWrapper); + EditorGUIUtility.systemCopyBuffer = rulesJson; + } + /// <summary> + /// Pastes all Rules from the clipboard to a RuleTile + /// </summary> + /// <param name="item">MenuCommand storing the RuleTile to paste to</param> + [MenuItem("CONTEXT/RuleTile/Paste Rules")] + public static void PasteRules(MenuCommand item) + { + RuleTile tile = item.context as RuleTile; + if (tile == null) + return; + + try + { + RuleTileRuleWrapper rulesWrapper = new RuleTileRuleWrapper(); + EditorJsonUtility.FromJsonOverwrite(EditorGUIUtility.systemCopyBuffer, rulesWrapper); + tile.m_TilingRules.AddRange(rulesWrapper.rules); + } + catch (Exception) + { + Debug.LogError("Unable to paste rules from system copy buffer"); + } + } + } +} diff --git a/MrBigsock/Packages/com.unity.2d.tilemap.extras/Editor/Tiles/RuleTile/RuleTileEditor.cs.meta b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Editor/Tiles/RuleTile/RuleTileEditor.cs.meta new file mode 100644 index 0000000000000000000000000000000000000000..e051679806c5d7a233a78a593ff7e4239b5d846e --- /dev/null +++ b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Editor/Tiles/RuleTile/RuleTileEditor.cs.meta @@ -0,0 +1,13 @@ +fileFormatVersion: 2 +guid: 139f9377103555b49b8dcd62686df3bf +timeCreated: 1501789622 +licenseType: Pro +MonoImporter: + externalObjects: {} + serializedVersion: 2 + defaultReferences: [] + executionOrder: 0 + icon: {instanceID: 0} + userData: + assetBundleName: + assetBundleVariant: diff --git a/MrBigsock/Packages/com.unity.2d.tilemap.extras/Editor/Unity.2D.Tilemap.Extras.Editor.asmdef b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Editor/Unity.2D.Tilemap.Extras.Editor.asmdef new file mode 100644 index 0000000000000000000000000000000000000000..98197674dbf482640dd789ce46c1a5333080904a --- /dev/null +++ b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Editor/Unity.2D.Tilemap.Extras.Editor.asmdef @@ -0,0 +1,18 @@ +{ + "name": "Unity.2D.Tilemap.Extras.Editor", + "references": [ + "GUID:613783dc1674e844b87788ea74ede0f6", + "GUID:3a9781db4804a9945b9883f3a7c46d45" + ], + "optionalUnityReferences": [], + "includePlatforms": [ + "Editor" + ], + "excludePlatforms": [], + "allowUnsafeCode": false, + "overrideReferences": false, + "precompiledReferences": [], + "autoReferenced": true, + "defineConstraints": [], + "versionDefines": [] +} \ No newline at end of file diff --git a/MrBigsock/Packages/com.unity.2d.tilemap.extras/Editor/Unity.2D.Tilemap.Extras.Editor.asmdef.meta b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Editor/Unity.2D.Tilemap.Extras.Editor.asmdef.meta new file mode 100644 index 0000000000000000000000000000000000000000..f98e10d43818ab1b3485ff6e87dfe2346a1836d7 --- /dev/null +++ b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Editor/Unity.2D.Tilemap.Extras.Editor.asmdef.meta @@ -0,0 +1,7 @@ +fileFormatVersion: 2 +guid: cbdf09464b6b87745be748a67e5a21a0 +AssemblyDefinitionImporter: + externalObjects: {} + userData: + assetBundleName: + assetBundleVariant: diff --git a/MrBigsock/Packages/com.unity.2d.tilemap.extras/LICENSE.md b/MrBigsock/Packages/com.unity.2d.tilemap.extras/LICENSE.md new file mode 100644 index 0000000000000000000000000000000000000000..37fcb9bfcf8983d695e9dc01908b7379ea29c5d8 --- /dev/null +++ b/MrBigsock/Packages/com.unity.2d.tilemap.extras/LICENSE.md @@ -0,0 +1,5 @@ +2D Tilemap Extras copyright © 2020 Unity Technologies ApS + +Licensed under the Unity Companion License for Unity-dependent projects--see [Unity Companion License](http://www.unity3d.com/legal/licenses/Unity_Companion_License). + +Unless expressly provided otherwise, the Software under this license is made available strictly on an “AS IS†BASIS WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED. Please review the license for details on these and other terms and conditions. diff --git a/MrBigsock/Packages/com.unity.2d.tilemap.extras/LICENSE.md.meta b/MrBigsock/Packages/com.unity.2d.tilemap.extras/LICENSE.md.meta new file mode 100644 index 0000000000000000000000000000000000000000..8ec1bd62007d59f882bbd7f7b26b5a4ebdc02400 --- /dev/null +++ b/MrBigsock/Packages/com.unity.2d.tilemap.extras/LICENSE.md.meta @@ -0,0 +1,7 @@ +fileFormatVersion: 2 +guid: d096e666129ea0247bb05049143ba33a +TextScriptImporter: + externalObjects: {} + userData: + assetBundleName: + assetBundleVariant: diff --git a/MrBigsock/Packages/com.unity.2d.tilemap.extras/QAReport.md b/MrBigsock/Packages/com.unity.2d.tilemap.extras/QAReport.md new file mode 100644 index 0000000000000000000000000000000000000000..bd67b23406ce680a368ce81af939dfea5e8c9f9a --- /dev/null +++ b/MrBigsock/Packages/com.unity.2d.tilemap.extras/QAReport.md @@ -0,0 +1,22 @@ +# QA Report +Use this file to outline the test strategy for this package. + +## QA Owner: [*Add Name*] + +## Test strategy +*Use this section to describe how this feature was tested.* +* A link to the Test Plan (Test Rails, other) +* Results from the package's editor and runtime test suite. +* Link to automated test results (if any) +* Manual test Results, [here's an example](https://docs.google.com/spreadsheets/d/12A76U5Gf969w10KL4Ik0wC1oFIBDUoRrqIvQgD18TFo/edit#gid=0) +* Scenario test week outcome +* etc. + +## Package Status +Use this section to describe: +* package stability +* known bugs, issues +* performance metrics, +* etc + +In other words, a general feeling on the health of this package. diff --git a/MrBigsock/Packages/com.unity.2d.tilemap.extras/QAReport.md.meta b/MrBigsock/Packages/com.unity.2d.tilemap.extras/QAReport.md.meta new file mode 100644 index 0000000000000000000000000000000000000000..918e6288e17d04f1ff24ddd0e1d8b50ded21ca7a --- /dev/null +++ b/MrBigsock/Packages/com.unity.2d.tilemap.extras/QAReport.md.meta @@ -0,0 +1,7 @@ +fileFormatVersion: 2 +guid: 3ddb995fe98f35a44818e802c99f4b1e +TextScriptImporter: + externalObjects: {} + userData: + assetBundleName: + assetBundleVariant: diff --git a/MrBigsock/Packages/com.unity.2d.tilemap.extras/README.md b/MrBigsock/Packages/com.unity.2d.tilemap.extras/README.md new file mode 100644 index 0000000000000000000000000000000000000000..38a4796fcbb2a4ea44dcf9074528e90efcf51950 --- /dev/null +++ b/MrBigsock/Packages/com.unity.2d.tilemap.extras/README.md @@ -0,0 +1,93 @@ +# Notice + +## No more development on 2d-extras GitHub repository + +We are changing how we develop additional 2D Tiles and Brushes for 2D Tilemap. An important part of this change involves ensuring a single point of entry for users to get the latest tiles and brushes for Tilemap. To this end we created the Tilemap Extras package which is accessible from Package Manager. Moving forward, the 2D-extras GitHub repository will be made read-only. You will still be able to clone and pull the contents of the repository as it is right now. + +Tilemap Extras already includes Animated Tiles, RuleTile (Rectangular Isometric and Hexagonal), the Rule Override Tiles, Group Brush, Line Brush, Random Brush and GameObject Brush. It also includes 3 samples covering common use-cases of Rule Tiles and Animated Tiles. Read more about Tilemap Extras in the announcement thread. + +If there is functionality in 2d-extras that is important to your team and your product please let us know in this thread or start a discussion in the 2D forums and we will consider adding it to the Tilemap Extras package. + +For issues with the Tilemap Extras please use the standard Unity channels via the Unity Bug Reporter and/or starting a thread in our forums instead of the GitHub Repository Issues tracker. + +Thank you for all your contributions over the years. As always, let us know about your needs and project plans and we will endeavor to make Tilemap Extras an even better tool for developing 2D games in Unity! + + +# 2d-extras + +2d-extras is a repository containing helpful reusable scripts which you can use to make your games, with a slant towards 2D. Feel free to customise the behavior of the scripts to create new tools for your use case! + +Implemented examples using these scripts can be found in the sister repository [2d-techdemos](https://github.com/Unity-Technologies/2d-techdemos "2d-techdemos: Examples for 2d features"). + +All items in the repository are grouped by use for a feature and are listed below. + +## How to use this + +You can use this in two different ways: downloading this repository or adding it to your project's Package Manager manifest. + +Alternatively, you can pick and choose the scripts that you want by placing only these scripts in your project's `Assets` folder. + +### Download + +#### Setup +Download or clone this repository into your project in the folder `Packages/com.unity.2d.tilemap.extras`. + +### Package Manager Manifest + +#### Requirements +[Git](https://git-scm.com/) must be installed and added to your path. + +#### Setup +The following line needs to be added to your `Packages/manifest.json` file in your Unity Project under the `dependencies` section: + +```json +"com.unity.2d.tilemap.extras": "https://github.com/Unity-Technologies/2d-extras.git#master" +``` + +### Tilemap + +For use with Unity `2021.1.0f1` onwards. + +Please use the `2020.3` branch for Unity 2020.1-2020.3 versions. + +Please use the `1.5.0-preview` tag for Unity 2019.2-2019.4 versions. + +Please use the `2019.1` tag for Unity 2019.1 versions. + +Please use the `2018.3` branch or the `2018.3` tag for Unity 2018.3-2018.4 versions. + +Please use the `2018.2` branch or the `2018.2` tag for Unity 2018.2 versions. + +Please use the `2017` branch or the `2017` tag for earlier versions of Unity (from 2017.2 and up). + +##### Brushes + +- **Coordinate**: This Brush displays the cell coordinates it is targeting in the SceneView. Use this as an example to create brushes which have extra visualization features when painting onto a Tilemap. +- **Line**: This Brush helps draw lines of Tiles onto a Tilemap. The first click of the mouse sets the starting point of the line and the second click sets the ending point of the line and draws the lines of Tiles. Use this as an example to modify brush painting behaviour to making painting quicker with less actions. +- **Random**: This Brush helps to place random Tiles onto a Tilemap. Use this as an example to create brushes which store specific data per brush and to make brushes which randomize behaviour. +- **Prefab**: This Brush instances and places the containing Prefab onto the targeted location and parents the instanced object to the paint target. Use this as an example to quickly place an assorted type of GameObjects onto structured locations. +- **PrefabRandom**: This Brush instances and places a randomly selected Prefabs onto the targeted location and parents the instanced object to the paint target. Use this as an example to quickly place an assorted type of GameObjects onto structured locations. +- **GameObject**: This Brush instances, places and manipulates GameObjects onto the scene. Use this as an example to create brushes which targets objects other than tiles for manipulation. +- **TintBrush**: Brush to edit Tilemap per-cell tint colors. +- **TintBrushSmooth**: Advanced tint brush for interpolated tint color per-cell. Requires the use of custom shader (see TintedTilemap.shader) and helper component TileTextureGenerator. +- **Group**: This Brush helps to pick Tiles which are grouped together by position. Gaps can be set to identify if Tiles belong to a Group. Limits can be set to ensure that an over-sized Group will not be picked. Use this as an example to create brushes that have the ability to choose and pick whichever Tiles it is interested in. + +##### Tiles + +- **Animated**: Animated Tiles are tiles which run through and display a list of sprites in sequence. +- **Pipeline**: Pipeline Tiles are tiles which take into consideration its orthogonal neighboring tiles and displays a sprite depending on whether the neighboring tile is the same tile. +- **Random**: Random Tiles are tiles which pseudo-randomly pick a sprite from a given list of sprites and a target location, and displays that sprite. +- **Terrain**: Terrain Tiles, similar to Pipeline Tiles, are tiles which take into consideration its orthogonal and diagonal neighboring tiles and displays a sprite depending on whether the neighboring tile is the same tile. +- **RuleTile**: Generic visual tile for creating different tilesets like terrain, pipeline, random or animated tiles. +- **Hexagonal Rule Tile**: A Rule Tile for use with Hexagonal Grids. Enable Flat Top for Flat Top Hexagonal Grids and disable for Pointed Top Hexagonal Grids. +- **Isometric Rule Tile**: A Rule Tile for use with Isometric Grids. +- **RuleOverrideTile**: Rule Override Tiles are Tiles which can override a subset of Rules for a given Rule Tile to provide specialised behaviour while keeping most of the Rules originally set in the Rule Tile. +- **Weighted Random**: Weighted Random Tiles are tiles which randomly pick a sprite from a given list of sprites and a target location, and displays that sprite. The sprites can be weighted with a value to change its probability of appearing. + +##### Other + +- **GridInformation**: A simple MonoBehaviour that stores and provides information based on Grid positions and keywords. +- **Custom Rules for RuleTile**: This helps to create new custom Rules for the Rule Tile. Check the [Wiki](https://github.com/Unity-Technologies/2d-extras/wiki) or this great [video](https://youtu.be/FwOxLkJTXag) for more information on how to use this! + +[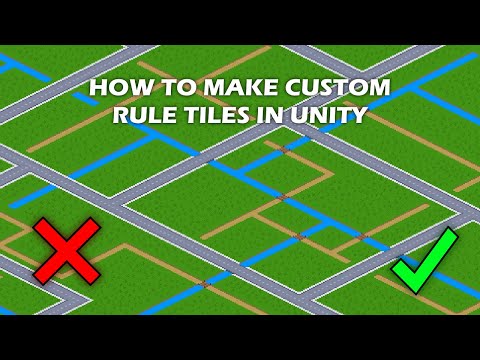](http://www.youtube.com/watch?v=FwOxLkJTXag "How to make Custom Rule Tiles in Unity") + diff --git a/MrBigsock/Packages/com.unity.2d.tilemap.extras/README.md.meta b/MrBigsock/Packages/com.unity.2d.tilemap.extras/README.md.meta new file mode 100644 index 0000000000000000000000000000000000000000..23abc72744085a03c58884d9d596fc1a8ab8fd91 --- /dev/null +++ b/MrBigsock/Packages/com.unity.2d.tilemap.extras/README.md.meta @@ -0,0 +1,7 @@ +fileFormatVersion: 2 +guid: 8a2cf010fb12d5146a68fb9a7b4d89fe +TextScriptImporter: + externalObjects: {} + userData: + assetBundleName: + assetBundleVariant: diff --git a/MrBigsock/Packages/com.unity.2d.tilemap.extras/Runtime.meta b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Runtime.meta new file mode 100644 index 0000000000000000000000000000000000000000..9f6ce745b7bea79c4d3bd619e5255ac5ca81b37c --- /dev/null +++ b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Runtime.meta @@ -0,0 +1,8 @@ +fileFormatVersion: 2 +guid: bb6e0fae761c98e4babad8a6c2188e8d +folderAsset: yes +DefaultImporter: + externalObjects: {} + userData: + assetBundleName: + assetBundleVariant: diff --git a/MrBigsock/Packages/com.unity.2d.tilemap.extras/Runtime/Brushes.meta b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Runtime/Brushes.meta new file mode 100644 index 0000000000000000000000000000000000000000..bb831abea15d59de54897036802790d90a82cfb7 --- /dev/null +++ b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Runtime/Brushes.meta @@ -0,0 +1,8 @@ +fileFormatVersion: 2 +guid: 111a320d9ff44f74c927510506ca4709 +folderAsset: yes +DefaultImporter: + externalObjects: {} + userData: + assetBundleName: + assetBundleVariant: diff --git a/MrBigsock/Packages/com.unity.2d.tilemap.extras/Runtime/Brushes/TintTextureGenerator.cs b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Runtime/Brushes/TintTextureGenerator.cs new file mode 100644 index 0000000000000000000000000000000000000000..89fa258de766e03576736e7fd8d4a34cba8497c4 --- /dev/null +++ b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Runtime/Brushes/TintTextureGenerator.cs @@ -0,0 +1,171 @@ +using System; +using UnityEngine; +using UnityEngine.Tilemaps; + +/// <summary> +/// A helper class for tinting a Grid component +/// </summary> +[ExecuteInEditMode] +public class TintTextureGenerator : MonoBehaviour +{ + /// <summary> + /// Size of the Tint map in cells + /// </summary> + public int k_TintMapSize = 256; + + /// <summary> + /// Mapping scale for cells to Tint map texture + /// </summary> + /// /// <remarks> + /// Adjust to get better definition. + /// </remarks> + public int k_ScaleFactor = 1; + + /// <summary> + /// Size of the Tint map texture in pixels + /// </summary> + public int k_TintMapTextureSize => k_TintMapSize * k_ScaleFactor; + + private Grid m_Grid; + + + /// <summary> + /// Callback when the TintTextureGenerator is loaded. + /// Refreshes the Grid Component on this GameObject. + /// </summary> + public void Start() + { + m_Grid = GetComponent<Grid>(); + Refresh(m_Grid); + } + + private Texture2D m_TintTexture; + + /// <summary> + /// Tint texture generated from Grid values + /// </summary> + public Texture2D tintTexture + { + get + { + if (m_TintTexture == null || m_TintTexture.width != k_TintMapTextureSize) + { + m_TintTexture = new Texture2D(k_TintMapTextureSize, k_TintMapTextureSize, TextureFormat.ARGB32, false); + m_TintTexture.hideFlags = HideFlags.HideAndDontSave; + m_TintTexture.wrapMode = TextureWrapMode.Clamp; + m_TintTexture.filterMode = FilterMode.Bilinear; + RefreshGlobalShaderValues(); + Refresh(m_Grid); + } + return m_TintTexture; + } + } + + /// <summary> + /// Refreshes the tint color of the Grid + /// </summary> + /// <param name="grid">Grid to refresh color</param> + public void Refresh(Grid grid) + { + if (grid == null) + return; + + var gi = GetGridInformation(grid); + int w = tintTexture.width; + int h = tintTexture.height; + for (int y = 0; y < h; y++) + { + for (int x = 0; x < w; x++) + { + Vector3 worldPos = TextureToWorld(new Vector3Int(x, y, 0)); + Vector3Int cellPos = grid.WorldToCell(worldPos); + tintTexture.SetPixel(x, y, gi.GetPositionProperty(cellPos, "Tint", Color.white)); + } + } + tintTexture.Apply(); + } + + /// <summary> + /// Refreshes the color of a position on a Grid + /// </summary> + /// <param name="grid">Grid to refresh color</param> + /// <param name="position">Position of the Grid to refresh color</param> + public void Refresh(Grid grid, Vector3Int position) + { + if (grid == null) + return; + + RefreshGlobalShaderValues(); + var worldPosition = grid.CellToWorld(position); + Vector3Int texPosition = WorldToTexture(worldPosition); + var color = GetGridInformation(grid).GetPositionProperty(position, "Tint", Color.white); + var scale = Math.Max(0, k_ScaleFactor - 2); + var radius = new Vector2Int(Mathf.RoundToInt(scale * grid.cellSize.x), Mathf.RoundToInt(scale * grid.cellSize.y)); + for (int y = -radius.y; y <= radius.y; ++y) + { + for (int x = -radius.x; x <= radius.x; ++x) + { + tintTexture.SetPixel(texPosition.x + x, texPosition.y + y, color); + } + } + tintTexture.Apply(); + } + + /// <summary> + /// Get the color of a position on a Grid + /// </summary> + /// <param name="grid">Grid to get color from</param> + /// <param name="position">Position of the Grid to get color from</param> + /// <returns>Color of a position on a Grid</returns> + public Color GetColor(Grid grid, Vector3Int position) + { + if (grid == null) + return Color.white; + + return GetGridInformation(grid).GetPositionProperty(position, "Tint", Color.white); + } + + /// <summary> + /// Set the color of a position on a Grid + /// </summary> + /// <param name="grid">Grid to set color to</param> + /// <param name="position">Position of the Grid to set color to</param> + /// <param name="color">Color to set to</param> + public void SetColor(Grid grid, Vector3Int position, Color color) + { + if (grid == null) + return; + + GetGridInformation(grid).SetPositionProperty(position, "Tint", color); + Refresh(grid, position); + } + + Vector3Int WorldToTexture(Vector3 worldPos) + { + return new Vector3Int(Mathf.FloorToInt((worldPos.x * k_ScaleFactor) + tintTexture.width / 2f) + , Mathf.FloorToInt((worldPos.y * k_ScaleFactor) + tintTexture.height / 2f), 0); + } + + Vector3 TextureToWorld(Vector3Int texPos) + { + var inv = 1 / (k_ScaleFactor == 0 ? 1 : k_ScaleFactor); + return new Vector3((texPos.x - tintTexture.width / 2) * inv + , (texPos.y - tintTexture.height / 2) * inv, 0); + } + + GridInformation GetGridInformation(Grid grid) + { + GridInformation gridInformation = grid.GetComponent<GridInformation>(); + + if (gridInformation == null) + gridInformation = grid.gameObject.AddComponent<GridInformation>(); + + return gridInformation; + } + + void RefreshGlobalShaderValues() + { + Shader.SetGlobalTexture("_TintMap", m_TintTexture); + Shader.SetGlobalFloat("_TintMapSize", k_TintMapSize); + } +} diff --git a/MrBigsock/Packages/com.unity.2d.tilemap.extras/Runtime/Brushes/TintTextureGenerator.cs.meta b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Runtime/Brushes/TintTextureGenerator.cs.meta new file mode 100644 index 0000000000000000000000000000000000000000..1c485cdc03c4c561bc7ba75d955b51a8c1c36d68 --- /dev/null +++ b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Runtime/Brushes/TintTextureGenerator.cs.meta @@ -0,0 +1,13 @@ +fileFormatVersion: 2 +guid: b41bf0cc11b1c8f419f96e8eb0adea40 +timeCreated: 1502798706 +licenseType: Pro +MonoImporter: + externalObjects: {} + serializedVersion: 2 + defaultReferences: [] + executionOrder: 0 + icon: {instanceID: 0} + userData: + assetBundleName: + assetBundleVariant: diff --git a/MrBigsock/Packages/com.unity.2d.tilemap.extras/Runtime/Brushes/TintedTilemap.shader b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Runtime/Brushes/TintedTilemap.shader new file mode 100644 index 0000000000000000000000000000000000000000..9b2fc8f1aa7de748951c35fff48a2436a1dd286a --- /dev/null +++ b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Runtime/Brushes/TintedTilemap.shader @@ -0,0 +1,62 @@ + Shader "Custom/TintedTilemap" + { + Properties + { + [PerRendererData]_MainTex ("Albedo (RGB)", 2D) = "white" {} + } + + SubShader + { + Tags { "Queue"="Transparent" "Render"="Transparent" "IgnoreProjector"="True"} + LOD 200 + + Cull Off + ZWrite Off + Blend SrcAlpha OneMinusSrcAlpha + + Pass{ + CGPROGRAM + + #pragma target 3.0 + #pragma vertex vert + #pragma fragment frag + + #include "UnityCG.cginc" + + struct appdata { + float4 vertex : POSITION; + float4 texcoord : TEXCOORD0; + }; + + sampler2D _MainTex; + sampler2D _TintMap; + float _TintMapSize; + + struct v2f { + float4 vertex : SV_POSITION; + float4 uv : TEXCOORD0; + float3 worldPos : float3; + }; + + v2f vert(appdata v) { + v2f o; + + o.worldPos = mul (unity_ObjectToWorld, v.vertex); + o.vertex = UnityObjectToClipPos(v.vertex); + o.uv = float4(v.texcoord.xy, 0, 0); + + return o; + } + + fixed4 frag(v2f i) : SV_Target { + fixed4 col = tex2D (_MainTex, i.uv); + fixed4 tint = tex2D(_TintMap, (i.worldPos.xy / _TintMapSize) + .5); + return tint * col; + } + ENDCG + } + + + } + FallBack "Diffuse" +} diff --git a/MrBigsock/Packages/com.unity.2d.tilemap.extras/Runtime/Brushes/TintedTilemap.shader.meta b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Runtime/Brushes/TintedTilemap.shader.meta new file mode 100644 index 0000000000000000000000000000000000000000..aefebd40259df4e8ca37833eebb08e8c7bf4be7c --- /dev/null +++ b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Runtime/Brushes/TintedTilemap.shader.meta @@ -0,0 +1,10 @@ +fileFormatVersion: 2 +guid: 9fcc3b710e7f7ae44bcf65125a08d5ef +timeCreated: 1502805334 +licenseType: Pro +ShaderImporter: + externalObjects: {} + defaultTextures: [] + userData: + assetBundleName: + assetBundleVariant: diff --git a/MrBigsock/Packages/com.unity.2d.tilemap.extras/Runtime/GridInformation.meta b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Runtime/GridInformation.meta new file mode 100644 index 0000000000000000000000000000000000000000..623006900cc4c3036619385bd65e2fa769e95222 --- /dev/null +++ b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Runtime/GridInformation.meta @@ -0,0 +1,8 @@ +fileFormatVersion: 2 +guid: ecefc247a9b423b4f8398f5e03d27f76 +folderAsset: yes +DefaultImporter: + externalObjects: {} + userData: + assetBundleName: + assetBundleVariant: diff --git a/MrBigsock/Packages/com.unity.2d.tilemap.extras/Runtime/GridInformation/GridInformation.cs b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Runtime/GridInformation/GridInformation.cs new file mode 100644 index 0000000000000000000000000000000000000000..da5d97b0243ef6f99c42faef820d9031ffe22c3c --- /dev/null +++ b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Runtime/GridInformation/GridInformation.cs @@ -0,0 +1,479 @@ +using System; +using System.Collections.Generic; +using System.Linq; + +namespace UnityEngine.Tilemaps +{ + [Serializable] + internal enum GridInformationType + { + Integer, + String, + Float, + Double, + UnityObject, + Color + } + + /// <summary> + /// A simple MonoBehaviour that stores and provides information based on Grid positions and keywords. + /// </summary> + [Serializable] + [HelpURL("https://docs.unity3d.com/Packages/com.unity.2d.tilemap.extras@latest/index.html?subfolder=/manual/GridInformation.html")] + [AddComponentMenu("Tilemap/Grid Information")] + public class GridInformation : MonoBehaviour, ISerializationCallbackReceiver + { + internal struct GridInformationValue + { + public GridInformationType type; + public object data; + } + + [Serializable] + internal struct GridInformationKey + { + public Vector3Int position; + public String name; + } + + private Dictionary<GridInformationKey, GridInformationValue> m_PositionProperties = new Dictionary<GridInformationKey, GridInformationValue>(); + internal Dictionary<GridInformationKey, GridInformationValue> PositionProperties + { + get { return m_PositionProperties; } + } + + [SerializeField] + [HideInInspector] + private List<GridInformationKey> m_PositionIntKeys = new List<GridInformationKey>(); + + [SerializeField] + [HideInInspector] + private List<int> m_PositionIntValues = new List<int>(); + + [SerializeField] + [HideInInspector] + private List<GridInformationKey> m_PositionStringKeys = new List<GridInformationKey>(); + + [SerializeField] + [HideInInspector] + private List<String> m_PositionStringValues = new List<String>(); + + [SerializeField] + [HideInInspector] + private List<GridInformationKey> m_PositionFloatKeys = new List<GridInformationKey>(); + + [SerializeField] + [HideInInspector] + private List<float> m_PositionFloatValues = new List<float>(); + + [SerializeField] + [HideInInspector] + private List<GridInformationKey> m_PositionDoubleKeys = new List<GridInformationKey>(); + + [SerializeField] + [HideInInspector] + private List<Double> m_PositionDoubleValues = new List<Double>(); + + [SerializeField] + [HideInInspector] + private List<GridInformationKey> m_PositionObjectKeys = new List<GridInformationKey>(); + + [SerializeField] + [HideInInspector] + private List<Object> m_PositionObjectValues = new List<Object>(); + + [SerializeField] + [HideInInspector] + private List<GridInformationKey> m_PositionColorKeys = new List<GridInformationKey>(); + + [SerializeField] + [HideInInspector] + private List<Color> m_PositionColorValues = new List<Color>(); + + /// <summary> + /// Callback before serializing this GridInformation + /// </summary> + void ISerializationCallbackReceiver.OnBeforeSerialize() + { + Grid grid = GetComponentInParent<Grid>(); + if (grid == null) + return; + + m_PositionIntKeys.Clear(); + m_PositionIntValues.Clear(); + m_PositionStringKeys.Clear(); + m_PositionStringValues.Clear(); + m_PositionFloatKeys.Clear(); + m_PositionFloatValues.Clear(); + m_PositionDoubleKeys.Clear(); + m_PositionDoubleValues.Clear(); + m_PositionObjectKeys.Clear(); + m_PositionObjectValues.Clear(); + m_PositionColorKeys.Clear(); + m_PositionColorValues.Clear(); + + foreach (var kvp in m_PositionProperties) + { + switch (kvp.Value.type) + { + case GridInformationType.Integer: + m_PositionIntKeys.Add(kvp.Key); + m_PositionIntValues.Add((int)kvp.Value.data); + break; + case GridInformationType.String: + m_PositionStringKeys.Add(kvp.Key); + m_PositionStringValues.Add(kvp.Value.data as String); + break; + case GridInformationType.Float: + m_PositionFloatKeys.Add(kvp.Key); + m_PositionFloatValues.Add((float)kvp.Value.data); + break; + case GridInformationType.Double: + m_PositionDoubleKeys.Add(kvp.Key); + m_PositionDoubleValues.Add((double)kvp.Value.data); + break; + case GridInformationType.Color: + m_PositionColorKeys.Add(kvp.Key); + m_PositionColorValues.Add((Color)kvp.Value.data); + break; + default: + m_PositionObjectKeys.Add(kvp.Key); + m_PositionObjectValues.Add(kvp.Value.data as Object); + break; + } + } + } + + /// <summary> + /// Callback after deserializing this GridInformation + /// </summary> + void ISerializationCallbackReceiver.OnAfterDeserialize() + { + m_PositionProperties.Clear(); + for (int i = 0; i != Math.Min(m_PositionIntKeys.Count, m_PositionIntValues.Count); i++) + { + GridInformationValue positionValue; + positionValue.type = GridInformationType.Integer; + positionValue.data = m_PositionIntValues[i]; + m_PositionProperties.Add(m_PositionIntKeys[i], positionValue); + } + for (int i = 0; i != Math.Min(m_PositionStringKeys.Count, m_PositionStringValues.Count); i++) + { + GridInformationValue positionValue; + positionValue.type = GridInformationType.String; + positionValue.data = m_PositionStringValues[i]; + m_PositionProperties.Add(m_PositionStringKeys[i], positionValue); + } + for (int i = 0; i != Math.Min(m_PositionFloatKeys.Count, m_PositionFloatValues.Count); i++) + { + GridInformationValue positionValue; + positionValue.type = GridInformationType.Float; + positionValue.data = m_PositionFloatValues[i]; + m_PositionProperties.Add(m_PositionFloatKeys[i], positionValue); + } + for (int i = 0; i != Math.Min(m_PositionDoubleKeys.Count, m_PositionDoubleValues.Count); i++) + { + GridInformationValue positionValue; + positionValue.type = GridInformationType.Double; + positionValue.data = m_PositionDoubleValues[i]; + m_PositionProperties.Add(m_PositionDoubleKeys[i], positionValue); + } + for (int i = 0; i != Math.Min(m_PositionObjectKeys.Count, m_PositionObjectValues.Count); i++) + { + GridInformationValue positionValue; + positionValue.type = GridInformationType.UnityObject; + positionValue.data = m_PositionObjectValues[i]; + m_PositionProperties.Add(m_PositionObjectKeys[i], positionValue); + } + for (int i = 0; i != Math.Min(m_PositionColorKeys.Count, m_PositionColorValues.Count); i++) + { + GridInformationValue positionValue; + positionValue.type = GridInformationType.Color; + positionValue.data = m_PositionColorValues[i]; + m_PositionProperties.Add(m_PositionColorKeys[i], positionValue); + } + } + + /// <summary> + /// This is not supported. + /// </summary> + /// <param name="position">Position to store information for</param> + /// <param name="name">Property name to store information for</param> + /// <param name="positionProperty">The information to be stored at the position</param> + /// <typeparam name="T">Type of the information to set</typeparam> + /// <returns>Whether the information was set</returns> + /// <exception cref="NotImplementedException">This is not implemented as only concrete Types are supported</exception> + public bool SetPositionProperty<T>(Vector3Int position, String name, T positionProperty) + { + throw new NotImplementedException("Storing this type is not accepted in GridInformation"); + } + + /// <summary> + /// Stores int information at the given position with the given property name + /// </summary> + /// <param name="position">Position to store information for</param> + /// <param name="name">Property name to store information for</param> + /// <param name="positionProperty">The information to be stored at the position</param> + /// <returns>Whether the information was set</returns> + public bool SetPositionProperty(Vector3Int position, String name, int positionProperty) + { + return SetPositionProperty(position, name, GridInformationType.Integer, positionProperty); + } + + /// <summary> + /// Stores string information at the given position with the given property name + /// </summary> + /// <param name="position">Position to store information for</param> + /// <param name="name">Property name to store information for</param> + /// <param name="positionProperty">The information to be stored at the position</param> + /// <returns>Whether the information was set</returns> + public bool SetPositionProperty(Vector3Int position, String name, string positionProperty) + { + return SetPositionProperty(position, name, GridInformationType.String, positionProperty); + } + + /// <summary> + /// Stores float information at the given position with the given property name + /// </summary> + /// <param name="position">Position to store information for</param> + /// <param name="name">Property name to store information for</param> + /// <param name="positionProperty">The information to be stored at the position</param> + /// <returns>Whether the information was set</returns> + public bool SetPositionProperty(Vector3Int position, String name, float positionProperty) + { + return SetPositionProperty(position, name, GridInformationType.Float, positionProperty); + } + + /// <summary> + /// Stores double information at the given position with the given property name + /// </summary> + /// <param name="position">Position to store information for</param> + /// <param name="name">Property name to store information for</param> + /// <param name="positionProperty">The information to be stored at the position</param> + /// <returns>Whether the information was set</returns> + public bool SetPositionProperty(Vector3Int position, String name, double positionProperty) + { + return SetPositionProperty(position, name, GridInformationType.Double, positionProperty); + } + + /// <summary> + /// Stores UnityEngine.Object information at the given position with the given property name + /// </summary> + /// <param name="position">Position to store information for</param> + /// <param name="name">Property name to store information for</param> + /// <param name="positionProperty">The information to be stored at the position</param> + /// <returns>Whether the information was set</returns> + public bool SetPositionProperty(Vector3Int position, String name, UnityEngine.Object positionProperty) + { + return SetPositionProperty(position, name, GridInformationType.UnityObject, positionProperty); + } + + /// <summary> + /// Stores color information at the given position with the given property name + /// </summary> + /// <param name="position">Position to store information for</param> + /// <param name="name">Property name to store information for</param> + /// <param name="positionProperty">The information to be stored at the position</param> + /// <returns>Whether the information was set</returns> + public bool SetPositionProperty(Vector3Int position, String name, Color positionProperty) + { + return SetPositionProperty(position, name, GridInformationType.Color, positionProperty); + } + + private bool SetPositionProperty(Vector3Int position, String name, GridInformationType dataType, System.Object positionProperty) + { + Grid grid = GetComponentInParent<Grid>(); + if (grid != null && positionProperty != null) + { + GridInformationKey positionKey; + positionKey.position = position; + positionKey.name = name; + + GridInformationValue positionValue; + positionValue.type = dataType; + positionValue.data = positionProperty; + + m_PositionProperties[positionKey] = positionValue; + return true; + } + return false; + } + + /// <summary> + /// Retrieves information stored at the given position with the given property name as the given Type + /// </summary> + /// <param name="position">Position to retrieve information for</param> + /// <param name="name">Property name to retrieve information for</param> + /// <param name="defaultValue">Default value if property does not exist at the given position</param> + /// <typeparam name="T">Type of the information to retrieve</typeparam> + /// <returns>The information stored at the position</returns> + /// <exception cref="InvalidCastException">Thrown when information to be retrieved is not the given Type</exception> + public T GetPositionProperty<T>(Vector3Int position, String name, T defaultValue) where T : UnityEngine.Object + { + GridInformationKey positionKey; + positionKey.position = position; + positionKey.name = name; + + GridInformationValue positionValue; + if (m_PositionProperties.TryGetValue(positionKey, out positionValue)) + { + if (positionValue.type != GridInformationType.UnityObject) + throw new InvalidCastException("Value stored in GridInformation is not of the right type"); + return positionValue.data as T; + } + return defaultValue; + } + + /// <summary> + /// Retrieves int information stored at the given position with the given property name + /// </summary> + /// <param name="position">Position to retrieve information for</param> + /// <param name="name">Property name to retrieve information for</param> + /// <param name="defaultValue">Default int if property does not exist at the given position</param> + /// <returns>The int stored at the position</returns> + /// <exception cref="InvalidCastException">Thrown when information to be retrieved is not a int</exception> + public int GetPositionProperty(Vector3Int position, String name, int defaultValue) + { + GridInformationKey positionKey; + positionKey.position = position; + positionKey.name = name; + + GridInformationValue positionValue; + if (m_PositionProperties.TryGetValue(positionKey, out positionValue)) + { + if (positionValue.type != GridInformationType.Integer) + throw new InvalidCastException("Value stored in GridInformation is not of the right type"); + return (int)positionValue.data; + } + return defaultValue; + } + + /// <summary> + /// Retrieves string information stored at the given position with the given property name + /// </summary> + /// <param name="position">Position to retrieve information for</param> + /// <param name="name">Property name to retrieve information for</param> + /// <param name="defaultValue">Default string if property does not exist at the given position</param> + /// <returns>The string stored at the position</returns> + /// <exception cref="InvalidCastException">Thrown when information to be retrieved is not a string</exception> + public string GetPositionProperty(Vector3Int position, String name, string defaultValue) + { + GridInformationKey positionKey; + positionKey.position = position; + positionKey.name = name; + + GridInformationValue positionValue; + if (m_PositionProperties.TryGetValue(positionKey, out positionValue)) + { + if (positionValue.type != GridInformationType.String) + throw new InvalidCastException("Value stored in GridInformation is not of the right type"); + return (string)positionValue.data; + } + return defaultValue; + } + + /// <summary> + /// Retrieves float information stored at the given position with the given property name + /// </summary> + /// <param name="position">Position to retrieve information for</param> + /// <param name="name">Property name to retrieve information for</param> + /// <param name="defaultValue">Default float if property does not exist at the given position</param> + /// <returns>The float stored at the position</returns> + /// <exception cref="InvalidCastException">Thrown when information to be retrieved is not a float</exception> + public float GetPositionProperty(Vector3Int position, String name, float defaultValue) + { + GridInformationKey positionKey; + positionKey.position = position; + positionKey.name = name; + + GridInformationValue positionValue; + if (m_PositionProperties.TryGetValue(positionKey, out positionValue)) + { + if (positionValue.type != GridInformationType.Float) + throw new InvalidCastException("Value stored in GridInformation is not of the right type"); + return (float)positionValue.data; + } + return defaultValue; + } + + /// <summary> + /// Retrieves double information stored at the given position with the given property name + /// </summary> + /// <param name="position">Position to retrieve information for</param> + /// <param name="name">Property name to retrieve information for</param> + /// <param name="defaultValue">Default double if property does not exist at the given position</param> + /// <returns>The double stored at the position</returns> + /// <exception cref="InvalidCastException">Thrown when information to be retrieved is not a double</exception> + public double GetPositionProperty(Vector3Int position, String name, double defaultValue) + { + GridInformationKey positionKey; + positionKey.position = position; + positionKey.name = name; + + GridInformationValue positionValue; + if (m_PositionProperties.TryGetValue(positionKey, out positionValue)) + { + if (positionValue.type != GridInformationType.Double) + throw new InvalidCastException("Value stored in GridInformation is not of the right type"); + return (double)positionValue.data; + } + return defaultValue; + } + + /// <summary> + /// Retrieves Color information stored at the given position with the given property name + /// </summary> + /// <param name="position">Position to retrieve information for</param> + /// <param name="name">Property name to retrieve information for</param> + /// <param name="defaultValue">Default color if property does not exist at the given position</param> + /// <returns>The color stored at the position</returns> + /// <exception cref="InvalidCastException">Thrown when information to be retrieved is not a Color</exception> + public Color GetPositionProperty(Vector3Int position, String name, Color defaultValue) + { + GridInformationKey positionKey; + positionKey.position = position; + positionKey.name = name; + + GridInformationValue positionValue; + if (m_PositionProperties.TryGetValue(positionKey, out positionValue)) + { + if (positionValue.type != GridInformationType.Color) + throw new InvalidCastException("Value stored in GridInformation is not of the right type"); + return (Color)positionValue.data; + } + return defaultValue; + } + + /// <summary> + /// Erases information stored at the given position with the given property name + /// </summary> + /// <param name="position">Position to erase</param> + /// <param name="name">Property name to erase</param> + /// <returns>Whether the information was erased</returns> + public bool ErasePositionProperty(Vector3Int position, String name) + { + GridInformationKey positionKey; + positionKey.position = position; + positionKey.name = name; + return m_PositionProperties.Remove(positionKey); + } + + /// <summary> + /// Clears all information stored + /// </summary> + public virtual void Reset() + { + m_PositionProperties.Clear(); + } + + /// <summary> + /// Gets all positions with information with the given property name + /// </summary> + /// <param name="propertyName">Property name to search for</param> + /// <returns>An array of all positions with the property name</returns> + public Vector3Int[] GetAllPositions(string propertyName) + { + return m_PositionProperties.Keys.ToList().FindAll(x => x.name == propertyName).Select(x => x.position).ToArray(); + } + } +} diff --git a/MrBigsock/Packages/com.unity.2d.tilemap.extras/Runtime/GridInformation/GridInformation.cs.meta b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Runtime/GridInformation/GridInformation.cs.meta new file mode 100644 index 0000000000000000000000000000000000000000..b40d5d6ce05363d4709f1e8c66f6dfef4b375879 --- /dev/null +++ b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Runtime/GridInformation/GridInformation.cs.meta @@ -0,0 +1,13 @@ +fileFormatVersion: 2 +guid: e7bb9acb2cffc5f45abddc238a0ad9b0 +timeCreated: 1501815409 +licenseType: Free +MonoImporter: + externalObjects: {} + serializedVersion: 2 + defaultReferences: [] + executionOrder: 0 + icon: {instanceID: 0} + userData: + assetBundleName: + assetBundleVariant: diff --git a/MrBigsock/Packages/com.unity.2d.tilemap.extras/Runtime/Tiles.meta b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Runtime/Tiles.meta new file mode 100644 index 0000000000000000000000000000000000000000..193c8be0626b91165d4bede50a15905714faa568 --- /dev/null +++ b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Runtime/Tiles.meta @@ -0,0 +1,8 @@ +fileFormatVersion: 2 +guid: 86aeedb511333af45943236d5d6be1b4 +folderAsset: yes +DefaultImporter: + externalObjects: {} + userData: + assetBundleName: + assetBundleVariant: diff --git a/MrBigsock/Packages/com.unity.2d.tilemap.extras/Runtime/Tiles/AnimatedTile.meta b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Runtime/Tiles/AnimatedTile.meta new file mode 100644 index 0000000000000000000000000000000000000000..1022ea464ca922577a002f1bf059accc42d608ba --- /dev/null +++ b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Runtime/Tiles/AnimatedTile.meta @@ -0,0 +1,8 @@ +fileFormatVersion: 2 +guid: daa079e375a6c2c49a30d83bd2d842c2 +folderAsset: yes +DefaultImporter: + externalObjects: {} + userData: + assetBundleName: + assetBundleVariant: diff --git a/MrBigsock/Packages/com.unity.2d.tilemap.extras/Runtime/Tiles/AnimatedTile/AnimatedTile.cs b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Runtime/Tiles/AnimatedTile/AnimatedTile.cs new file mode 100644 index 0000000000000000000000000000000000000000..5579bc33e0caeeffa64d62d9a9e424301266aa09 --- /dev/null +++ b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Runtime/Tiles/AnimatedTile/AnimatedTile.cs @@ -0,0 +1,387 @@ +using System; + +#if UNITY_EDITOR +using UnityEditor; +using UnityEditorInternal; +using System.Collections.Generic; +using System.Linq; +#endif + +namespace UnityEngine.Tilemaps +{ + /// <summary> + /// Animated Tiles are tiles which run through and display a list of sprites in sequence. + /// </summary> + [Serializable] + [HelpURL("https://docs.unity3d.com/Packages/com.unity.2d.tilemap.extras@latest/index.html?subfolder=/manual/AnimatedTile.html")] + public class AnimatedTile : TileBase + { + /// <summary> + /// The List of Sprites set for the Animated Tile. + /// This will be played in sequence. + /// </summary> + public Sprite[] m_AnimatedSprites; + /// <summary> + /// The minimum possible speed at which the Animation of the Tile will be played. + /// A speed value will be randomly chosen between the minimum and maximum speed. + /// </summary> + public float m_MinSpeed = 1f; + /// <summary> + /// The maximum possible speed at which the Animation of the Tile will be played. + /// A speed value will be randomly chosen between the minimum and maximum speed. + /// </summary> + public float m_MaxSpeed = 1f; + /// <summary> + /// The starting time of this Animated Tile. + /// This allows you to start the Animation from time in the list of Animated Sprites depending on the + /// Tilemap's Animation Frame Rate. + /// </summary> + public float m_AnimationStartTime; + /// <summary> + /// The starting frame of this Animated Tile. + /// This allows you to start the Animation from a particular Sprite in the list of Animated Sprites. + /// If this is set, this overrides m_AnimationStartTime. + /// </summary> + public int m_AnimationStartFrame = 0; + /// <summary> + /// The Collider Shape generated by the Tile. + /// </summary> + public Tile.ColliderType m_TileColliderType; + + /// <summary> + /// Retrieves any tile rendering data from the scripted tile. + /// </summary> + /// <param name="position">Position of the Tile on the Tilemap.</param> + /// <param name="tilemap">The Tilemap the tile is present on.</param> + /// <param name="tileData">Data to render the tile.</param> + public override void GetTileData(Vector3Int position, ITilemap tilemap, ref TileData tileData) + { + tileData.transform = Matrix4x4.identity; + tileData.color = Color.white; + if (m_AnimatedSprites != null && m_AnimatedSprites.Length > 0) + { + tileData.sprite = m_AnimatedSprites[m_AnimatedSprites.Length - 1]; + tileData.colliderType = m_TileColliderType; + } + } + + /// <summary> + /// Retrieves any tile animation data from the scripted tile. + /// </summary> + /// <param name="position">Position of the Tile on the Tilemap.</param> + /// <param name="tilemap">The Tilemap the tile is present on.</param> + /// <param name="tileAnimationData">Data to run an animation on the tile.</param> + /// <returns>Whether the call was successful.</returns> + public override bool GetTileAnimationData(Vector3Int position, ITilemap tilemap, ref TileAnimationData tileAnimationData) + { + if (m_AnimatedSprites.Length > 0) + { + tileAnimationData.animatedSprites = m_AnimatedSprites; + tileAnimationData.animationSpeed = Random.Range(m_MinSpeed, m_MaxSpeed); + tileAnimationData.animationStartTime = m_AnimationStartTime; + if (0 < m_AnimationStartFrame && m_AnimationStartFrame <= m_AnimatedSprites.Length) + { + var tilemapComponent = tilemap.GetComponent<Tilemap>(); + if (tilemapComponent != null && tilemapComponent.animationFrameRate > 0) + tileAnimationData.animationStartTime = (m_AnimationStartFrame - 1) / tilemapComponent.animationFrameRate; + } + return true; + } + return false; + } + } + +#if UNITY_EDITOR + [CustomEditor(typeof(AnimatedTile))] + public class AnimatedTileEditor : Editor + { + private static class Styles + { + public static readonly GUIContent orderAnimatedTileSpritesInfo = + EditorGUIUtility.TrTextContent("Place sprites shown based on the order of animation."); + public static readonly GUIContent emptyAnimatedTileInfo = + EditorGUIUtility.TrTextContent( + "Drag Sprite or Sprite Texture assets \n" + + " to start creating an Animated Tile."); + public static readonly GUIContent minimumSpeedLabel = EditorGUIUtility.TrTextContent("Minimum Speed", + "The minimum possible speed at which the Animation of the Tile will be played. A speed value will be randomly chosen between the minimum and maximum speed."); + public static readonly GUIContent maximumSpeedLabel = EditorGUIUtility.TrTextContent("Maximum Speed", + "The maximum possible speed at which the Animation of the Tile will be played. A speed value will be randomly chosen between the minimum and maximum speed."); + public static readonly GUIContent startTimeLabel = EditorGUIUtility.TrTextContent("Start Time", "The starting time of this Animated Tile. This allows you to start the Animation from a particular time."); + public static readonly GUIContent startFrameLabel = EditorGUIUtility.TrTextContent("Start Frame", "The starting frame of this Animated Tile. This allows you to start the Animation from a particular Sprite in the list of Animated Sprites."); + public static readonly GUIContent colliderTypeLabel = EditorGUIUtility.TrTextContent("Collider Type", "The Collider Shape generated by the Tile."); + } + + private static readonly string k_UndoName = L10n.Tr("Change AnimatedTile"); + + private SerializedProperty m_AnimatedSprites; + + private AnimatedTile tile { get { return (target as AnimatedTile); } } + + private List<Sprite> dragAndDropSprites; + + private ReorderableList reorderableList; + + private void OnEnable() + { + reorderableList = new ReorderableList(tile.m_AnimatedSprites, typeof(Sprite), true, true, true, true); + reorderableList.drawHeaderCallback = OnDrawHeader; + reorderableList.drawElementCallback = OnDrawElement; + reorderableList.elementHeightCallback = GetElementHeight; + reorderableList.onAddCallback = OnAddElement; + reorderableList.onRemoveCallback = OnRemoveElement; + reorderableList.onReorderCallback = OnReorderElement; + + m_AnimatedSprites = serializedObject.FindProperty("m_AnimatedSprites"); + } + + private void OnDrawHeader(Rect rect) + { + GUI.Label(rect, Styles.orderAnimatedTileSpritesInfo); + } + + private void OnDrawElement(Rect rect, int index, bool isActive, bool isFocused) + { + if (tile.m_AnimatedSprites != null && index < tile.m_AnimatedSprites.Length) + { + var spriteName = tile.m_AnimatedSprites[index] != null ? tile.m_AnimatedSprites[index].name : "Null"; + tile.m_AnimatedSprites[index] = (Sprite) EditorGUI.ObjectField(rect + , $"Sprite {index + 1}: {spriteName}" + , tile.m_AnimatedSprites[index] + , typeof(Sprite) + , false); + } + } + + private float GetElementHeight(int index) + { + return 3 * EditorGUI.GetPropertyHeight(SerializedPropertyType.ObjectReference, + null); + } + + private void OnAddElement(ReorderableList list) + { + var count = tile.m_AnimatedSprites != null ? tile.m_AnimatedSprites.Length + 1 : 1; + ResizeAnimatedSpriteList(count); + + if (list.index == 0 || list.index < list.count) + { + Array.Copy(tile.m_AnimatedSprites, list.index + 1, tile.m_AnimatedSprites, list.index + 2, list.count - list.index - 1); + tile.m_AnimatedSprites[list.index + 1] = null; + if (list.IsSelected(list.index)) + list.index += 1; + } + else + { + tile.m_AnimatedSprites[count - 1] = null; + } + } + + private void OnRemoveElement(ReorderableList list) + { + if (tile.m_AnimatedSprites != null && tile.m_AnimatedSprites.Length > 0 && list.index < tile.m_AnimatedSprites.Length) + { + var sprites = tile.m_AnimatedSprites.ToList(); + sprites.RemoveAt(list.index); + tile.m_AnimatedSprites = sprites.ToArray(); + } + } + + private void OnReorderElement(ReorderableList list) + { + // Fix for 2020.1, which does not track changes when reordering in the list + EditorUtility.SetDirty(tile); + } + + private void DisplayClipboardText(GUIContent clipboardText, Rect position) + { + Color old = GUI.color; + GUI.color = Color.gray; + var infoSize = GUI.skin.label.CalcSize(clipboardText); + Rect rect = new Rect(position.center.x - infoSize.x * .5f + , position.center.y - infoSize.y * .5f + , infoSize.x + , infoSize.y); + GUI.Label(rect, clipboardText); + GUI.color = old; + } + + private bool dragAndDropActive + { + get + { + return dragAndDropSprites != null + && dragAndDropSprites.Count > 0; + } + } + + private void DragAndDropClear() + { + dragAndDropSprites = null; + DragAndDrop.visualMode = DragAndDropVisualMode.None; + Event.current.Use(); + } + + private static List<Sprite> GetSpritesFromTexture(Texture2D texture) + { + string path = AssetDatabase.GetAssetPath(texture); + Object[] assets = AssetDatabase.LoadAllAssetsAtPath(path); + List<Sprite> sprites = new List<Sprite>(); + + foreach (Object asset in assets) + { + if (asset is Sprite) + { + sprites.Add(asset as Sprite); + } + } + + return sprites; + } + + private static List<Sprite> GetValidSingleSprites(Object[] objects) + { + List<Sprite> result = new List<Sprite>(); + foreach (Object obj in objects) + { + if (obj is Sprite) + { + result.Add(obj as Sprite); + } + else if (obj is Texture2D) + { + Texture2D texture = obj as Texture2D; + List<Sprite> sprites = GetSpritesFromTexture(texture); + if (sprites.Count > 0) + { + result.AddRange(sprites); + } + } + } + return result; + } + + private void HandleDragAndDrop(Rect guiRect) + { + if (DragAndDrop.objectReferences.Length == 0 || !guiRect.Contains(Event.current.mousePosition)) + return; + + switch (Event.current.type) + { + case EventType.DragUpdated: + { + dragAndDropSprites = GetValidSingleSprites(DragAndDrop.objectReferences); + if (dragAndDropActive) + { + DragAndDrop.visualMode = DragAndDropVisualMode.Copy; + Event.current.Use(); + GUI.changed = true; + } + } + break; + case EventType.DragPerform: + { + if (!dragAndDropActive) + return; + + Undo.RegisterCompleteObjectUndo(tile, "Drag and Drop to Animated Tile"); + ResizeAnimatedSpriteList(dragAndDropSprites.Count); + Array.Copy(dragAndDropSprites.ToArray(), tile.m_AnimatedSprites, dragAndDropSprites.Count); + DragAndDropClear(); + GUI.changed = true; + EditorUtility.SetDirty(tile); + GUIUtility.ExitGUI(); + } + break; + case EventType.Repaint: + // Handled in Render() + break; + } + + if (Event.current.type == EventType.DragExited || + Event.current.type == EventType.KeyDown && Event.current.keyCode == KeyCode.Escape) + { + DragAndDropClear(); + } + } + + /// <summary> + /// Draws an Inspector for the AnimatedTile. + /// </summary> + public override void OnInspectorGUI() + { + serializedObject.Update(); + + Undo.RecordObject(tile, k_UndoName); + + EditorGUI.BeginChangeCheck(); + int count = EditorGUILayout.DelayedIntField("Number of Animated Sprites", tile.m_AnimatedSprites != null ? tile.m_AnimatedSprites.Length : 0); + if (count < 0) + count = 0; + + if (tile.m_AnimatedSprites == null || tile.m_AnimatedSprites.Length != count) + ResizeAnimatedSpriteList(count); + + if (count == 0) + { + Rect rect = EditorGUILayout.GetControlRect(false, EditorGUIUtility.singleLineHeight * 5); + HandleDragAndDrop(rect); + EditorGUI.DrawRect(rect, dragAndDropActive && rect.Contains(Event.current.mousePosition) ? Color.white : Color.black); + var innerRect = new Rect(rect.x + 1, rect.y + 1, rect.width - 2, rect.height - 2); + EditorGUI.DrawRect(innerRect, EditorGUIUtility.isProSkin + ? (Color) new Color32 (56, 56, 56, 255) + : (Color) new Color32 (194, 194, 194, 255)); + DisplayClipboardText(Styles.emptyAnimatedTileInfo, rect); + GUILayout.Space(rect.height); + EditorGUILayout.Space(); + } + + if (reorderableList != null) + { + var tileCount = tile.m_AnimatedSprites != null ? tile.m_AnimatedSprites.Length : 0; + if (reorderableList.list == null || reorderableList.count != tileCount) + reorderableList.list = tile.m_AnimatedSprites; + reorderableList.DoLayoutList(); + } + + using (new EditorGUI.DisabledScope(tile.m_AnimatedSprites == null || tile.m_AnimatedSprites.Length == 0)) + { + float minSpeed = EditorGUILayout.FloatField(Styles.minimumSpeedLabel, tile.m_MinSpeed); + float maxSpeed = EditorGUILayout.FloatField(Styles.maximumSpeedLabel, tile.m_MaxSpeed); + if (minSpeed < 0.0f) + minSpeed = 0.0f; + + if (maxSpeed < 0.0f) + maxSpeed = 0.0f; + + if (maxSpeed < minSpeed) + maxSpeed = minSpeed; + + tile.m_MinSpeed = minSpeed; + tile.m_MaxSpeed = maxSpeed; + + using (new EditorGUI.DisabledScope(tile.m_AnimatedSprites == null + || (0 < tile.m_AnimationStartFrame + && tile.m_AnimationStartFrame <= tile.m_AnimatedSprites.Length))) + { + tile.m_AnimationStartTime = EditorGUILayout.FloatField(Styles.startTimeLabel, tile.m_AnimationStartTime); + } + tile.m_AnimationStartFrame = EditorGUILayout.IntField(Styles.startFrameLabel, tile.m_AnimationStartFrame); + tile.m_TileColliderType = (Tile.ColliderType) EditorGUILayout.EnumPopup(Styles.colliderTypeLabel, tile.m_TileColliderType); + } + + if (EditorGUI.EndChangeCheck()) + { + serializedObject.ApplyModifiedProperties(); + EditorUtility.SetDirty(tile); + } + } + + private void ResizeAnimatedSpriteList(int count) + { + m_AnimatedSprites.arraySize = count; + serializedObject.ApplyModifiedProperties(); + } + } +#endif +} diff --git a/MrBigsock/Packages/com.unity.2d.tilemap.extras/Runtime/Tiles/AnimatedTile/AnimatedTile.cs.meta b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Runtime/Tiles/AnimatedTile/AnimatedTile.cs.meta new file mode 100644 index 0000000000000000000000000000000000000000..5a20eba57003e79a7da2d2a60421215cf599c826 --- /dev/null +++ b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Runtime/Tiles/AnimatedTile/AnimatedTile.cs.meta @@ -0,0 +1,12 @@ +fileFormatVersion: 2 +guid: 13b75c95f34a00d4e8c04f76b73312e6 +timeCreated: 1464531813 +licenseType: Pro +MonoImporter: + serializedVersion: 2 + defaultReferences: [] + executionOrder: 0 + icon: {instanceID: 0} + userData: + assetBundleName: + assetBundleVariant: diff --git a/MrBigsock/Packages/com.unity.2d.tilemap.extras/Runtime/Tiles/HexagonalRuleTile.meta b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Runtime/Tiles/HexagonalRuleTile.meta new file mode 100644 index 0000000000000000000000000000000000000000..21968f80aa75bcab96c1492257ed38f70eb0bf1d --- /dev/null +++ b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Runtime/Tiles/HexagonalRuleTile.meta @@ -0,0 +1,8 @@ +fileFormatVersion: 2 +guid: 3d0cb420882fe5d48ba544c0bb493094 +folderAsset: yes +DefaultImporter: + externalObjects: {} + userData: + assetBundleName: + assetBundleVariant: diff --git a/MrBigsock/Packages/com.unity.2d.tilemap.extras/Runtime/Tiles/HexagonalRuleTile/HexagonalRuleTile.cs b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Runtime/Tiles/HexagonalRuleTile/HexagonalRuleTile.cs new file mode 100644 index 0000000000000000000000000000000000000000..52726e4eb97240e264fdcca601bc5947519a9d0b --- /dev/null +++ b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Runtime/Tiles/HexagonalRuleTile/HexagonalRuleTile.cs @@ -0,0 +1,196 @@ +using System; + +namespace UnityEngine +{ + /// <summary> + /// Generic visual tile for creating different tilesets like terrain, pipeline, random or animated tiles. + /// This is templated to accept a Neighbor Rule Class for Custom Rules. + /// Use this for Hexagonal Grids. + /// </summary> + /// <typeparam name="T">Neighbor Rule Class for Custom Rules</typeparam> + public class HexagonalRuleTile<T> : HexagonalRuleTile + { + /// <summary> + /// Returns the Neighbor Rule Class type for this Rule Tile. + /// </summary> + public sealed override Type m_NeighborType => typeof(T); + } + + /// <summary> + /// Generic visual tile for creating different tilesets like terrain, pipeline, random or animated tiles. + /// Use this for Hexagonal Grids. + /// </summary> + [Serializable] + [HelpURL("https://docs.unity3d.com/Packages/com.unity.2d.tilemap.extras@latest/index.html?subfolder=/manual/RuleTile.html")] + public class HexagonalRuleTile : RuleTile + { + /// <summary> + /// Angle in which the HexagonalRuleTile is rotated by for matching in Degrees. + /// </summary> + public override int m_RotationAngle => 60; + + private static float[] m_CosAngleArr1 = { + Mathf.Cos(0 * Mathf.Deg2Rad), + Mathf.Cos(-60 * Mathf.Deg2Rad), + Mathf.Cos(-120 * Mathf.Deg2Rad), + Mathf.Cos(-180 * Mathf.Deg2Rad), + Mathf.Cos(-240 * Mathf.Deg2Rad), + Mathf.Cos(-300 * Mathf.Deg2Rad), + }; + private static float[] m_SinAngleArr1 = { + Mathf.Sin(0 * Mathf.Deg2Rad), + Mathf.Sin(-60 * Mathf.Deg2Rad), + Mathf.Sin(-120 * Mathf.Deg2Rad), + Mathf.Sin(-180 * Mathf.Deg2Rad), + Mathf.Sin(-240 * Mathf.Deg2Rad), + Mathf.Sin(-300 * Mathf.Deg2Rad), + }; + private static float[] m_CosAngleArr2 = { + Mathf.Cos(0 * Mathf.Deg2Rad), + Mathf.Cos(60 * Mathf.Deg2Rad), + Mathf.Cos(120 * Mathf.Deg2Rad), + Mathf.Cos(180 * Mathf.Deg2Rad), + Mathf.Cos(240 * Mathf.Deg2Rad), + Mathf.Cos(300 * Mathf.Deg2Rad), + }; + private static float[] m_SinAngleArr2 = { + Mathf.Sin(0 * Mathf.Deg2Rad), + Mathf.Sin(60 * Mathf.Deg2Rad), + Mathf.Sin(120 * Mathf.Deg2Rad), + Mathf.Sin(180 * Mathf.Deg2Rad), + Mathf.Sin(240 * Mathf.Deg2Rad), + Mathf.Sin(300 * Mathf.Deg2Rad), + }; + + /// <summary> + /// Whether this is a flat top Hexagonal Tile + /// </summary> + [DontOverride] public bool m_FlatTop; + + static float m_TilemapToWorldYScale = Mathf.Pow(1 - Mathf.Pow(0.5f, 2f), 0.5f); + + /// <summary> + /// Converts a Tilemap Position to World Position. + /// </summary> + /// <param name="tilemapPosition">Tilemap Position to convert.</param> + /// <returns>World Position.</returns> + public static Vector3 TilemapPositionToWorldPosition(Vector3Int tilemapPosition) + { + Vector3 worldPosition = new Vector3(tilemapPosition.x, tilemapPosition.y); + if (tilemapPosition.y % 2 != 0) + worldPosition.x += 0.5f; + worldPosition.y *= m_TilemapToWorldYScale; + return worldPosition; + } + + /// <summary> + /// Converts a World Position to Tilemap Position. + /// </summary> + /// <param name="worldPosition">World Position to convert.</param> + /// <returns>Tilemap Position.</returns> + public static Vector3Int WorldPositionToTilemapPosition(Vector3 worldPosition) + { + worldPosition.y /= m_TilemapToWorldYScale; + Vector3Int tilemapPosition = new Vector3Int(); + tilemapPosition.y = Mathf.RoundToInt(worldPosition.y); + if (tilemapPosition.y % 2 != 0) + tilemapPosition.x = Mathf.RoundToInt(worldPosition.x - 0.5f); + else + tilemapPosition.x = Mathf.RoundToInt(worldPosition.x); + return tilemapPosition; + } + + /// <summary> + /// Get the offset for the given position with the given offset. + /// </summary> + /// <param name="position">Position to offset.</param> + /// <param name="offset">Offset for the position.</param> + /// <returns>The offset position.</returns> + public override Vector3Int GetOffsetPosition(Vector3Int position, Vector3Int offset) + { + Vector3Int offsetPosition = position + offset; + + if (offset.y % 2 != 0 && position.y % 2 != 0) + offsetPosition.x += 1; + + return offsetPosition; + } + + /// <summary> + /// Get the reversed offset for the given position with the given offset. + /// </summary> + /// <param name="position">Position to offset.</param> + /// <param name="offset">Offset for the position.</param> + /// <returns>The reversed offset position.</returns> + public override Vector3Int GetOffsetPositionReverse(Vector3Int position, Vector3Int offset) + { + return GetOffsetPosition(position, GetRotatedPosition(offset, 180)); + } + + /// <summary> + /// Gets a rotated position given its original position and the rotation in degrees. + /// </summary> + /// <param name="position">Original position of Tile.</param> + /// <param name="rotation">Rotation in degrees.</param> + /// <returns>Rotated position of Tile.</returns> + public override Vector3Int GetRotatedPosition(Vector3Int position, int rotation) + { + if (rotation != 0) + { + Vector3 worldPosition = TilemapPositionToWorldPosition(position); + + int index = rotation / 60; + if (m_FlatTop) + { + worldPosition = new Vector3( + worldPosition.x * m_CosAngleArr2[index] - worldPosition.y * m_SinAngleArr2[index], + worldPosition.x * m_SinAngleArr2[index] + worldPosition.y * m_CosAngleArr2[index] + ); + } + else + { + worldPosition = new Vector3( + worldPosition.x * m_CosAngleArr1[index] - worldPosition.y * m_SinAngleArr1[index], + worldPosition.x * m_SinAngleArr1[index] + worldPosition.y * m_CosAngleArr1[index] + ); + } + + position = WorldPositionToTilemapPosition(worldPosition); + } + return position; + } + + /// <summary> + /// Gets a mirrored position given its original position and the mirroring axii. + /// </summary> + /// <param name="position">Original position of Tile.</param> + /// <param name="mirrorX">Mirror in the X Axis.</param> + /// <param name="mirrorY">Mirror in the Y Axis.</param> + /// <returns>Mirrored position of Tile.</returns> + public override Vector3Int GetMirroredPosition(Vector3Int position, bool mirrorX, bool mirrorY) + { + if (mirrorX || mirrorY) + { + Vector3 worldPosition = TilemapPositionToWorldPosition(position); + + if (m_FlatTop) + { + if (mirrorX) + worldPosition.y *= -1; + if (mirrorY) + worldPosition.x *= -1; + } + else + { + if (mirrorX) + worldPosition.x *= -1; + if (mirrorY) + worldPosition.y *= -1; + } + + position = WorldPositionToTilemapPosition(worldPosition); + } + return position; + } + } +} diff --git a/MrBigsock/Packages/com.unity.2d.tilemap.extras/Runtime/Tiles/HexagonalRuleTile/HexagonalRuleTile.cs.meta b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Runtime/Tiles/HexagonalRuleTile/HexagonalRuleTile.cs.meta new file mode 100644 index 0000000000000000000000000000000000000000..84ebec292dd8f0103fd0684b52d6a0fab36f4783 --- /dev/null +++ b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Runtime/Tiles/HexagonalRuleTile/HexagonalRuleTile.cs.meta @@ -0,0 +1,11 @@ +fileFormatVersion: 2 +guid: 55f747e8af38fbe4d8819f37be6fd5aa +MonoImporter: + externalObjects: {} + serializedVersion: 2 + defaultReferences: [] + executionOrder: 0 + icon: {instanceID: 0} + userData: + assetBundleName: + assetBundleVariant: diff --git a/MrBigsock/Packages/com.unity.2d.tilemap.extras/Runtime/Tiles/IsometricRuleTile.meta b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Runtime/Tiles/IsometricRuleTile.meta new file mode 100644 index 0000000000000000000000000000000000000000..86596e8b82f518c29115ae16af7d106f34cbef1d --- /dev/null +++ b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Runtime/Tiles/IsometricRuleTile.meta @@ -0,0 +1,8 @@ +fileFormatVersion: 2 +guid: c8c72f5be5ed1d44b9a3bde2d9c137c8 +folderAsset: yes +DefaultImporter: + externalObjects: {} + userData: + assetBundleName: + assetBundleVariant: diff --git a/MrBigsock/Packages/com.unity.2d.tilemap.extras/Runtime/Tiles/IsometricRuleTile/IsometricRuleTile.cs b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Runtime/Tiles/IsometricRuleTile/IsometricRuleTile.cs new file mode 100644 index 0000000000000000000000000000000000000000..08c4041e5ad7fd2d54774b735fedeac7c21a787f --- /dev/null +++ b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Runtime/Tiles/IsometricRuleTile/IsometricRuleTile.cs @@ -0,0 +1,29 @@ +using System; + +namespace UnityEngine +{ + /// <summary> + /// Generic visual tile for creating different tilesets like terrain, pipeline, random or animated tiles. + /// This is templated to accept a Neighbor Rule Class for Custom Rules. + /// Use this for Isometric Grids. + /// </summary> + /// <typeparam name="T">Neighbor Rule Class for Custom Rules</typeparam> + public class IsometricRuleTile<T> : IsometricRuleTile + { + /// <summary> + /// Returns the Neighbor Rule Class type for this Rule Tile. + /// </summary> + public sealed override Type m_NeighborType => typeof(T); + } + + /// <summary> + /// Generic visual tile for creating different tilesets like terrain, pipeline, random or animated tiles. + /// Use this for Isometric Grids. + /// </summary> + [Serializable] + [HelpURL("https://docs.unity3d.com/Packages/com.unity.2d.tilemap.extras@latest/index.html?subfolder=/manual/RuleTile.html")] + public class IsometricRuleTile : RuleTile + { + // This has no differences with the RuleTile + } +} diff --git a/MrBigsock/Packages/com.unity.2d.tilemap.extras/Runtime/Tiles/IsometricRuleTile/IsometricRuleTile.cs.meta b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Runtime/Tiles/IsometricRuleTile/IsometricRuleTile.cs.meta new file mode 100644 index 0000000000000000000000000000000000000000..2149c7c03c59c4981f6e1c54a24dd965d15dc879 --- /dev/null +++ b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Runtime/Tiles/IsometricRuleTile/IsometricRuleTile.cs.meta @@ -0,0 +1,11 @@ +fileFormatVersion: 2 +guid: 5e6f3fbb560bd6041a202ea918fa4f23 +MonoImporter: + externalObjects: {} + serializedVersion: 2 + defaultReferences: [] + executionOrder: 0 + icon: {instanceID: 0} + userData: + assetBundleName: + assetBundleVariant: diff --git a/MrBigsock/Packages/com.unity.2d.tilemap.extras/Runtime/Tiles/PipelineTile.meta b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Runtime/Tiles/PipelineTile.meta new file mode 100644 index 0000000000000000000000000000000000000000..74b5a165c30f1b4c00871a6c3f958a0621f2caee --- /dev/null +++ b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Runtime/Tiles/PipelineTile.meta @@ -0,0 +1,8 @@ +fileFormatVersion: 2 +guid: 8cc7b6611da8a674c890494a2a21acbc +folderAsset: yes +DefaultImporter: + externalObjects: {} + userData: + assetBundleName: + assetBundleVariant: diff --git a/MrBigsock/Packages/com.unity.2d.tilemap.extras/Runtime/Tiles/PipelineTile/PipelineTile.cs b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Runtime/Tiles/PipelineTile/PipelineTile.cs new file mode 100644 index 0000000000000000000000000000000000000000..9b8abc108dde757b9979d2f1e13f2611d8d92adf --- /dev/null +++ b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Runtime/Tiles/PipelineTile/PipelineTile.cs @@ -0,0 +1,157 @@ +using System; +using System.Collections; +using System.Collections.Generic; + +#if UNITY_EDITOR +using UnityEditor; +#endif + +using UnityEngine; + +namespace UnityEngine.Tilemaps +{ + /// <summary> + /// Pipeline Tiles are tiles which take into consideration its orthogonal neighboring tiles and displays a sprite depending on whether the neighboring tile is the same tile. + /// </summary> + [Serializable] + public class PipelineTile : TileBase + { + /// <summary> + /// The Sprites used for defining the Pipeline. + /// </summary> + [SerializeField] + public Sprite[] m_Sprites; + + /// <summary> + /// This method is called when the tile is refreshed. + /// </summary> + /// <param name="position">Position of the Tile on the Tilemap.</param> + /// <param name="tilemap">The Tilemap the tile is present on.</param> + public override void RefreshTile(Vector3Int position, ITilemap tilemap) + { + for (int yd = -1; yd <= 1; yd++) + for (int xd = -1; xd <= 1; xd++) + { + Vector3Int pos = new Vector3Int(position.x + xd, position.y + yd, position.z); + if (TileValue(tilemap, pos)) + tilemap.RefreshTile(pos); + } + } + + /// <summary> + /// Retrieves any tile rendering data from the scripted tile. + /// </summary> + /// <param name="position">Position of the Tile on the Tilemap.</param> + /// <param name="tilemap">The Tilemap the tile is present on.</param> + /// <param name="tileData">Data to render the tile.</param> + public override void GetTileData(Vector3Int position, ITilemap tilemap, ref TileData tileData) + { + UpdateTile(position, tilemap, ref tileData); + } + + private void UpdateTile(Vector3Int position, ITilemap tilemap, ref TileData tileData) + { + tileData.transform = Matrix4x4.identity; + tileData.color = Color.white; + + int mask = TileValue(tilemap, position + new Vector3Int(0, 1, 0)) ? 1 : 0; + mask += TileValue(tilemap, position + new Vector3Int(1, 0, 0)) ? 2 : 0; + mask += TileValue(tilemap, position + new Vector3Int(0, -1, 0)) ? 4 : 0; + mask += TileValue(tilemap, position + new Vector3Int(-1, 0, 0)) ? 8 : 0; + + int index = GetIndex((byte)mask); + if (index >= 0 && index < m_Sprites.Length && TileValue(tilemap, position)) + { + tileData.sprite = m_Sprites[index]; + tileData.transform = GetTransform((byte)mask); + tileData.flags = TileFlags.LockTransform | TileFlags.LockColor; + tileData.colliderType = Tile.ColliderType.Sprite; + } + } + + private bool TileValue(ITilemap tileMap, Vector3Int position) + { + TileBase tile = tileMap.GetTile(position); + return (tile != null && tile == this); + } + + private int GetIndex(byte mask) + { + switch (mask) + { + case 0: return 0; + case 3: + case 6: + case 9: + case 12: return 1; + case 1: + case 2: + case 4: + case 5: + case 10: + case 8: return 2; + case 7: + case 11: + case 13: + case 14: return 3; + case 15: return 4; + } + return -1; + } + + private Matrix4x4 GetTransform(byte mask) + { + switch (mask) + { + case 9: + case 10: + case 7: + case 2: + case 8: + return Matrix4x4.TRS(Vector3.zero, Quaternion.Euler(0f, 0f, -90f), Vector3.one); + case 3: + case 14: + return Matrix4x4.TRS(Vector3.zero, Quaternion.Euler(0f, 0f, -180f), Vector3.one); + case 6: + case 13: + return Matrix4x4.TRS(Vector3.zero, Quaternion.Euler(0f, 0f, -270f), Vector3.one); + } + return Matrix4x4.identity; + } + } + +#if UNITY_EDITOR + [CustomEditor(typeof(PipelineTile))] + public class PipelineTileEditor : Editor + { + private PipelineTile tile { get { return (target as PipelineTile); } } + + /// <summary> + /// OnEnable for PipelineTile. + /// </summary> + public void OnEnable() + { + if (tile.m_Sprites == null || tile.m_Sprites.Length != 5) + tile.m_Sprites = new Sprite[5]; + } + + /// <summary> + /// Draws an Inspector for the PipelineTile. + /// </summary> + public override void OnInspectorGUI() + { + EditorGUILayout.LabelField("Place sprites shown based on the number of tiles bordering it."); + EditorGUILayout.Space(); + + EditorGUI.BeginChangeCheck(); + tile.m_Sprites[0] = (Sprite) EditorGUILayout.ObjectField("None", tile.m_Sprites[0], typeof(Sprite), false, null); + tile.m_Sprites[2] = (Sprite) EditorGUILayout.ObjectField("One", tile.m_Sprites[2], typeof(Sprite), false, null); + tile.m_Sprites[1] = (Sprite) EditorGUILayout.ObjectField("Two", tile.m_Sprites[1], typeof(Sprite), false, null); + tile.m_Sprites[3] = (Sprite) EditorGUILayout.ObjectField("Three", tile.m_Sprites[3], typeof(Sprite), false, null); + tile.m_Sprites[4] = (Sprite) EditorGUILayout.ObjectField("Four", tile.m_Sprites[4], typeof(Sprite), false, null); + if (EditorGUI.EndChangeCheck()) + EditorUtility.SetDirty(tile); + } + } +#endif +} diff --git a/MrBigsock/Packages/com.unity.2d.tilemap.extras/Runtime/Tiles/PipelineTile/PipelineTile.cs.meta b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Runtime/Tiles/PipelineTile/PipelineTile.cs.meta new file mode 100644 index 0000000000000000000000000000000000000000..e309018cdead5415a4100b75e7dc1ce15e3e6e11 --- /dev/null +++ b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Runtime/Tiles/PipelineTile/PipelineTile.cs.meta @@ -0,0 +1,12 @@ +fileFormatVersion: 2 +guid: 25192638efa881c469b1ac4d8cfd3f1b +timeCreated: 1464534747 +licenseType: Pro +MonoImporter: + serializedVersion: 2 + defaultReferences: [] + executionOrder: 0 + icon: {instanceID: 0} + userData: + assetBundleName: + assetBundleVariant: diff --git a/MrBigsock/Packages/com.unity.2d.tilemap.extras/Runtime/Tiles/RandomTile.meta b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Runtime/Tiles/RandomTile.meta new file mode 100644 index 0000000000000000000000000000000000000000..839107bae1ab200633e102747481573b56ea6ca6 --- /dev/null +++ b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Runtime/Tiles/RandomTile.meta @@ -0,0 +1,8 @@ +fileFormatVersion: 2 +guid: f459333af097b894b838c61a2fc7c220 +folderAsset: yes +DefaultImporter: + externalObjects: {} + userData: + assetBundleName: + assetBundleVariant: diff --git a/MrBigsock/Packages/com.unity.2d.tilemap.extras/Runtime/Tiles/RandomTile/RandomTile.cs b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Runtime/Tiles/RandomTile/RandomTile.cs new file mode 100644 index 0000000000000000000000000000000000000000..118795dd77290a1967fc8261d4fd560e1d62590a --- /dev/null +++ b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Runtime/Tiles/RandomTile/RandomTile.cs @@ -0,0 +1,105 @@ +using System; + +#if UNITY_EDITOR +using UnityEditor; +#endif + +namespace UnityEngine.Tilemaps +{ + /// <summary> + /// Random Tiles are tiles which pseudo-randomly pick a sprite from a given list of sprites and a target location, and displays that sprite. + /// The Sprite displayed for the Tile is randomized based on its location and will be fixed for that particular location. + /// </summary> + [Serializable] + public class RandomTile : Tile + { + /// <summary> + /// The Sprites used for randomizing output. + /// </summary> + [SerializeField] + public Sprite[] m_Sprites; + + /// <summary> + /// Retrieves any tile rendering data from the scripted tile. + /// </summary> + /// <param name="position">Position of the Tile on the Tilemap.</param> + /// <param name="tilemap">The Tilemap the tile is present on.</param> + /// <param name="tileData">Data to render the tile.</param> + public override void GetTileData(Vector3Int position, ITilemap tilemap, ref TileData tileData) + { + base.GetTileData(position, tilemap, ref tileData); + if ((m_Sprites != null) && (m_Sprites.Length > 0)) + { + long hash = position.x; + hash = (hash + 0xabcd1234) + (hash << 15); + hash = (hash + 0x0987efab) ^ (hash >> 11); + hash ^= position.y; + hash = (hash + 0x46ac12fd) + (hash << 7); + hash = (hash + 0xbe9730af) ^ (hash << 11); + var oldState = Random.state; + Random.InitState((int)hash); + tileData.sprite = m_Sprites[(int) (m_Sprites.Length * Random.value)]; + Random.state = oldState; + } + } + } + +#if UNITY_EDITOR + [CustomEditor(typeof(RandomTile))] + public class RandomTileEditor : Editor + { + private SerializedProperty m_Color; + private SerializedProperty m_ColliderType; + + private RandomTile tile { get { return (target as RandomTile); } } + + /// <summary> + /// OnEnable for RandomTile. + /// </summary> + public void OnEnable() + { + m_Color = serializedObject.FindProperty("m_Color"); + m_ColliderType = serializedObject.FindProperty("m_ColliderType"); + } + + /// <summary> + /// Draws an Inspector for the RandomTile. + /// </summary> + public override void OnInspectorGUI() + { + serializedObject.Update(); + + EditorGUI.BeginChangeCheck(); + int count = EditorGUILayout.DelayedIntField("Number of Sprites", tile.m_Sprites != null ? tile.m_Sprites.Length : 0); + if (count < 0) + count = 0; + if (tile.m_Sprites == null || tile.m_Sprites.Length != count) + { + Array.Resize<Sprite>(ref tile.m_Sprites, count); + } + + if (count == 0) + return; + + EditorGUILayout.LabelField("Place random sprites."); + EditorGUILayout.Space(); + + for (int i = 0; i < count; i++) + { + tile.m_Sprites[i] = (Sprite) EditorGUILayout.ObjectField("Sprite " + (i+1), tile.m_Sprites[i], typeof(Sprite), false, null); + } + + EditorGUILayout.Space(); + + EditorGUILayout.PropertyField(m_Color); + EditorGUILayout.PropertyField(m_ColliderType); + + if (EditorGUI.EndChangeCheck()) + { + EditorUtility.SetDirty(tile); + serializedObject.ApplyModifiedProperties(); + } + } + } +#endif +} diff --git a/MrBigsock/Packages/com.unity.2d.tilemap.extras/Runtime/Tiles/RandomTile/RandomTile.cs.meta b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Runtime/Tiles/RandomTile/RandomTile.cs.meta new file mode 100644 index 0000000000000000000000000000000000000000..4eeb80f912a74421511cbd231eeb2db10d263b53 --- /dev/null +++ b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Runtime/Tiles/RandomTile/RandomTile.cs.meta @@ -0,0 +1,12 @@ +fileFormatVersion: 2 +guid: 535f8e525ff367c4ba67961e201a05ed +timeCreated: 1445235751 +licenseType: Pro +MonoImporter: + serializedVersion: 2 + defaultReferences: [] + executionOrder: 0 + icon: {instanceID: 0} + userData: + assetBundleName: + assetBundleVariant: diff --git a/MrBigsock/Packages/com.unity.2d.tilemap.extras/Runtime/Tiles/RuleOverrideTile.meta b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Runtime/Tiles/RuleOverrideTile.meta new file mode 100644 index 0000000000000000000000000000000000000000..2a09dbbcf7348bc6a70118e69e47e0521df1d922 --- /dev/null +++ b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Runtime/Tiles/RuleOverrideTile.meta @@ -0,0 +1,8 @@ +fileFormatVersion: 2 +guid: 3404b3ef5c8133646b532504aef95120 +folderAsset: yes +DefaultImporter: + externalObjects: {} + userData: + assetBundleName: + assetBundleVariant: diff --git a/MrBigsock/Packages/com.unity.2d.tilemap.extras/Runtime/Tiles/RuleOverrideTile/AdvancedRuleOverrideTile.cs b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Runtime/Tiles/RuleOverrideTile/AdvancedRuleOverrideTile.cs new file mode 100644 index 0000000000000000000000000000000000000000..b1bbaf73476a63e8a7a71f1ef9a157d6e6b4a790 --- /dev/null +++ b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Runtime/Tiles/RuleOverrideTile/AdvancedRuleOverrideTile.cs @@ -0,0 +1,141 @@ +using System; +using System.Collections.Generic; +using UnityEngine.Scripting.APIUpdating; + +namespace UnityEngine.Tilemaps +{ + /// <summary> + /// Rule Override Tiles are Tiles which can override a subset of Rules for a given Rule Tile to provide specialised behaviour while keeping most of the Rules originally set in the Rule Tile. + /// </summary> + [MovedFrom(true, "UnityEngine")] + [Serializable] + [HelpURL("https://docs.unity3d.com/Packages/com.unity.2d.tilemap.extras@latest/index.html?subfolder=/manual/RuleOverrideTile.html")] + public class AdvancedRuleOverrideTile : RuleOverrideTile + { + + /// <summary> + /// Gets the overriding TilingRuleOutput of a given TilingRule. + /// </summary> + /// <param name="originalRule">The original TilingRule that is overridden</param> + public RuleTile.TilingRuleOutput this[RuleTile.TilingRule originalRule] + { + get + { + foreach (var overrideRule in m_OverrideTilingRules) + if (overrideRule.m_Id == originalRule.m_Id) + return overrideRule; + + return null; + } + set + { + for (int i = m_OverrideTilingRules.Count - 1; i >= 0; i--) + { + if (m_OverrideTilingRules[i].m_Id == originalRule.m_Id) + { + m_OverrideTilingRules.RemoveAt(i); + break; + } + } + if (value != null) + { + var json = JsonUtility.ToJson(value); + var overrideRule = JsonUtility.FromJson<RuleTile.TilingRuleOutput>(json); + m_OverrideTilingRules.Add(overrideRule); + } + } + } + + /// <summary> + /// The Default Sprite set when creating a new Rule override. + /// </summary> + public Sprite m_DefaultSprite; + /// <summary> + /// The Default GameObject set when creating a new Rule override. + /// </summary> + public GameObject m_DefaultGameObject; + /// <summary> + /// The Default Collider Type set when creating a new Rule override. + /// </summary> + public Tile.ColliderType m_DefaultColliderType = Tile.ColliderType.Sprite; + + /// <summary> + /// A list of TilingRule Overrides + /// </summary> + public List<RuleTile.TilingRuleOutput> m_OverrideTilingRules = new List<RuleTile.TilingRuleOutput>(); + + /// <summary> + /// Applies overrides to this + /// </summary> + /// <param name="overrides">A list of overrides to apply</param> + /// <exception cref="ArgumentNullException">The input overrides list is not valid</exception> + public void ApplyOverrides(IList<KeyValuePair<RuleTile.TilingRule, RuleTile.TilingRuleOutput>> overrides) + { + if (overrides == null) + throw new System.ArgumentNullException("overrides"); + + for (int i = 0; i < overrides.Count; i++) + this[overrides[i].Key] = overrides[i].Value; + } + + /// <summary> + /// Gets overrides for this + /// </summary> + /// <param name="overrides">A list of overrides to fill</param> + /// <param name="validCount">Returns the number of valid overrides for Rules</param> + /// <exception cref="ArgumentNullException">The input overrides list is not valid</exception> + public void GetOverrides(List<KeyValuePair<RuleTile.TilingRule, RuleTile.TilingRuleOutput>> overrides, ref int validCount) + { + if (overrides == null) + throw new System.ArgumentNullException("overrides"); + + overrides.Clear(); + + if (m_Tile) + { + foreach (var originalRule in m_Tile.m_TilingRules) + { + RuleTile.TilingRuleOutput overrideRule = this[originalRule]; + overrides.Add(new KeyValuePair<RuleTile.TilingRule, RuleTile.TilingRuleOutput>(originalRule, overrideRule)); + } + } + + validCount = overrides.Count; + + foreach (var overrideRule in m_OverrideTilingRules) + { + if (!overrides.Exists(o => o.Key.m_Id == overrideRule.m_Id)) + { + var originalRule = new RuleTile.TilingRule() { m_Id = overrideRule.m_Id }; + overrides.Add(new KeyValuePair<RuleTile.TilingRule, RuleTile.TilingRuleOutput>(originalRule, overrideRule)); + } + } + } + + /// <summary> + /// Updates the Rules with the Overrides set for this AdvancedRuleOverrideTile + /// </summary> + public override void Override() + { + if (!m_Tile || !m_InstanceTile) + return; + + PrepareOverride(); + + var tile = m_InstanceTile; + + tile.m_DefaultSprite = m_DefaultSprite; + tile.m_DefaultGameObject = m_DefaultGameObject; + tile.m_DefaultColliderType = m_DefaultColliderType; + + foreach (var rule in tile.m_TilingRules) + { + var overrideRule = this[rule]; + if (overrideRule != null) + { + JsonUtility.FromJsonOverwrite(JsonUtility.ToJson(overrideRule), rule); + } + } + } + } +} diff --git a/MrBigsock/Packages/com.unity.2d.tilemap.extras/Runtime/Tiles/RuleOverrideTile/AdvancedRuleOverrideTile.cs.meta b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Runtime/Tiles/RuleOverrideTile/AdvancedRuleOverrideTile.cs.meta new file mode 100644 index 0000000000000000000000000000000000000000..1dd56c98d9b1c5366256453bc475abac81e06e63 --- /dev/null +++ b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Runtime/Tiles/RuleOverrideTile/AdvancedRuleOverrideTile.cs.meta @@ -0,0 +1,11 @@ +fileFormatVersion: 2 +guid: 9ff2caf20a1df406d9ab8f2f5a57f734 +MonoImporter: + externalObjects: {} + serializedVersion: 2 + defaultReferences: [] + executionOrder: 0 + icon: {instanceID: 0} + userData: + assetBundleName: + assetBundleVariant: diff --git a/MrBigsock/Packages/com.unity.2d.tilemap.extras/Runtime/Tiles/RuleOverrideTile/RuleOverrideTile.cs b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Runtime/Tiles/RuleOverrideTile/RuleOverrideTile.cs new file mode 100644 index 0000000000000000000000000000000000000000..6bbca647af0af8fd9ac4889704a2058855eefe4f --- /dev/null +++ b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Runtime/Tiles/RuleOverrideTile/RuleOverrideTile.cs @@ -0,0 +1,381 @@ +using System; +using System.Collections.Generic; +using System.Linq; +using UnityEngine.Scripting.APIUpdating; + +#if UNITY_EDITOR +using UnityEditor; +#endif + +namespace UnityEngine.Tilemaps +{ + /// <summary> + /// Rule Override Tiles are Tiles which can override a subset of Rules for a given Rule Tile to provide specialised behaviour while keeping most of the Rules originally set in the Rule Tile. + /// </summary> + [MovedFrom(true, "UnityEngine")] + [Serializable] + [HelpURL("https://docs.unity3d.com/Packages/com.unity.2d.tilemap.extras@latest/index.html?subfolder=/manual/RuleOverrideTile.html")] + public class RuleOverrideTile : TileBase + { + + /// <summary> + /// A data structure storing the Sprite overriding the original RuleTile Sprite + /// </summary> + [Serializable] + public class TileSpritePair + { + /// <summary> + /// Original Sprite from the original RuleTile. + /// </summary> + public Sprite m_OriginalSprite; + /// <summary> + /// Overriding Sprite for the Original Sprite. + /// </summary> + public Sprite m_OverrideSprite; + } + + /// <summary> + /// A data structure storing the GameObject overriding the original RuleTile GameObject + /// </summary> + [Serializable] + public class TileGameObjectPair + { + /// <summary> + /// Original GameObject from the original RuleTile. + /// </summary> + public GameObject m_OriginalGameObject; + /// <summary> + /// Overriding GameObject for the Original Sprite. + /// </summary> + public GameObject m_OverrideGameObject; + } + + /// <summary> + /// Gets the overriding Sprite of a given Sprite. + /// </summary> + /// <param name="originalSprite">The original Sprite that is overridden</param> + public Sprite this[Sprite originalSprite] + { + get + { + foreach (TileSpritePair spritePair in m_Sprites) + { + if (spritePair.m_OriginalSprite == originalSprite) + { + return spritePair.m_OverrideSprite; + } + } + return null; + } + set + { + if (value == null) + { + m_Sprites = m_Sprites.Where(spritePair => spritePair.m_OriginalSprite != originalSprite).ToList(); + } + else + { + foreach (TileSpritePair spritePair in m_Sprites) + { + if (spritePair.m_OriginalSprite == originalSprite) + { + spritePair.m_OverrideSprite = value; + return; + } + } + m_Sprites.Add(new TileSpritePair() + { + m_OriginalSprite = originalSprite, + m_OverrideSprite = value, + }); + } + } + } + + /// <summary> + /// Gets the overriding GameObject of a given GameObject. + /// </summary> + /// <param name="originalGameObject">The original GameObject that is overridden</param> + public GameObject this[GameObject originalGameObject] + { + get + { + foreach (TileGameObjectPair gameObjectPair in m_GameObjects) + { + if (gameObjectPair.m_OriginalGameObject == originalGameObject) + { + return gameObjectPair.m_OverrideGameObject; + } + } + return null; + } + set + { + if (value == null) + { + m_GameObjects = m_GameObjects.Where(gameObjectPair => gameObjectPair.m_OriginalGameObject != originalGameObject).ToList(); + } + else + { + foreach (TileGameObjectPair gameObjectPair in m_GameObjects) + { + if (gameObjectPair.m_OriginalGameObject == originalGameObject) + { + gameObjectPair.m_OverrideGameObject = value; + return; + } + } + m_GameObjects.Add(new TileGameObjectPair() + { + m_OriginalGameObject = originalGameObject, + m_OverrideGameObject = value, + }); + } + } + } + + /// <summary> + /// The RuleTile to override + /// </summary> + public RuleTile m_Tile; + /// <summary> + /// A list of Sprite Overrides + /// </summary> + public List<TileSpritePair> m_Sprites = new List<TileSpritePair>(); + /// <summary> + /// A list of GameObject Overrides + /// </summary> + public List<TileGameObjectPair> m_GameObjects = new List<TileGameObjectPair>(); + + /// <summary> + /// Returns the Rule Tile for retrieving TileData + /// </summary> + [HideInInspector] public RuleTile m_InstanceTile; + + private void CreateInstanceTile() + { + var t = m_Tile.GetType(); + RuleTile instanceTile = CreateInstance(t) as RuleTile; + instanceTile.hideFlags = HideFlags.NotEditable; + instanceTile.name = m_Tile.name + " (Override)"; + m_InstanceTile = instanceTile; + +#if UNITY_EDITOR + if(AssetDatabase.Contains(this)) + AssetDatabase.AddObjectToAsset(instanceTile, this); + EditorUtility.SetDirty(this); +#endif + } + + /// <summary> + /// Applies overrides to this + /// </summary> + /// <param name="overrides">A list of overrides to apply</param> + /// <exception cref="ArgumentNullException">The input overrides list is not valid</exception> + public void ApplyOverrides(IList<KeyValuePair<Sprite, Sprite>> overrides) + { + if (overrides == null) + throw new ArgumentNullException("overrides"); + + for (int i = 0; i < overrides.Count; i++) + this[overrides[i].Key] = overrides[i].Value; + } + + /// <summary> + /// Applies overrides to this + /// </summary> + /// <param name="overrides">A list of overrides to apply</param> + /// <exception cref="ArgumentNullException">The input overrides list is not valid</exception> + public void ApplyOverrides(IList<KeyValuePair<GameObject, GameObject>> overrides) + { + if (overrides == null) + throw new ArgumentNullException("overrides"); + + for (int i = 0; i < overrides.Count; i++) + this[overrides[i].Key] = overrides[i].Value; + } + + /// <summary> + /// Gets overrides for this + /// </summary> + /// <param name="overrides">A list of overrides to fill</param> + /// <param name="validCount">Returns the number of valid overrides for Sprites</param> + /// <exception cref="ArgumentNullException">The input overrides list is not valid</exception> + public void GetOverrides(List<KeyValuePair<Sprite, Sprite>> overrides, ref int validCount) + { + if (overrides == null) + throw new ArgumentNullException("overrides"); + + overrides.Clear(); + + List<Sprite> originalSprites = new List<Sprite>(); + + if (m_Tile) + { + if (m_Tile.m_DefaultSprite) + originalSprites.Add(m_Tile.m_DefaultSprite); + + foreach (RuleTile.TilingRule rule in m_Tile.m_TilingRules) + foreach (Sprite sprite in rule.m_Sprites) + if (sprite && !originalSprites.Contains(sprite)) + originalSprites.Add(sprite); + } + + validCount = originalSprites.Count; + + foreach (var pair in m_Sprites) + if (!originalSprites.Contains(pair.m_OriginalSprite)) + originalSprites.Add(pair.m_OriginalSprite); + + foreach (Sprite sprite in originalSprites) + overrides.Add(new KeyValuePair<Sprite, Sprite>(sprite, this[sprite])); + } + + /// <summary> + /// Gets overrides for this + /// </summary> + /// <param name="overrides">A list of overrides to fill</param> + /// <param name="validCount">Returns the number of valid overrides for GameObjects</param> + /// <exception cref="ArgumentNullException">The input overrides list is not valid</exception> + public void GetOverrides(List<KeyValuePair<GameObject, GameObject>> overrides, ref int validCount) + { + if (overrides == null) + throw new ArgumentNullException("overrides"); + + overrides.Clear(); + + List<GameObject> originalGameObjects = new List<GameObject>(); + + if (m_Tile) + { + if (m_Tile.m_DefaultGameObject) + originalGameObjects.Add(m_Tile.m_DefaultGameObject); + + foreach (RuleTile.TilingRule rule in m_Tile.m_TilingRules) + if (rule.m_GameObject && !originalGameObjects.Contains(rule.m_GameObject)) + originalGameObjects.Add(rule.m_GameObject); + } + + validCount = originalGameObjects.Count; + + foreach (var pair in m_GameObjects) + if (!originalGameObjects.Contains(pair.m_OriginalGameObject)) + originalGameObjects.Add(pair.m_OriginalGameObject); + + foreach (GameObject gameObject in originalGameObjects) + overrides.Add(new KeyValuePair<GameObject, GameObject>(gameObject, this[gameObject])); + } + + /// <summary> + /// Updates the Rules with the Overrides set for this RuleOverrideTile + /// </summary> + public virtual void Override() + { + if (!m_Tile) + return; + + if (!m_InstanceTile) + CreateInstanceTile(); + + PrepareOverride(); + + var tile = m_InstanceTile; + + tile.m_DefaultSprite = this[tile.m_DefaultSprite] ?? tile.m_DefaultSprite; + tile.m_DefaultGameObject = this[tile.m_DefaultGameObject] ?? tile.m_DefaultGameObject; + + foreach (var rule in tile.m_TilingRules) + { + for (int i = 0; i < rule.m_Sprites.Length; i++) + { + Sprite sprite = rule.m_Sprites[i]; + rule.m_Sprites[i] = this[sprite] ?? sprite; + } + + rule.m_GameObject = this[rule.m_GameObject] ?? rule.m_GameObject; + } + } + + /// <summary> + /// Prepares the Overrides set for this RuleOverrideTile + /// </summary> + public void PrepareOverride() + { + // Create clone of instanceTile to keep data from collections being overridden by JsonUtility + var tempTile = Instantiate(m_InstanceTile); + + var customData = m_InstanceTile.GetCustomFields(true) + .ToDictionary(field => field, field => field.GetValue(tempTile)); + + JsonUtility.FromJsonOverwrite(JsonUtility.ToJson(m_Tile), m_InstanceTile); + + foreach (var kvp in customData) + kvp.Key.SetValue(m_InstanceTile, kvp.Value); + } + + /// <summary> + /// Retrieves any tile animation data from the scripted tile. + /// </summary> + /// <param name="position">Position of the Tile on the Tilemap.</param> + /// <param name="tilemap">The Tilemap the tile is present on.</param> + /// <param name="tileAnimationData">Data to run an animation on the tile.</param> + /// <returns>Whether the call was successful.</returns> + public override bool GetTileAnimationData(Vector3Int position, ITilemap tilemap, ref TileAnimationData tileAnimationData) + { + if (!m_InstanceTile) + return false; + return m_InstanceTile.GetTileAnimationData(position, tilemap, ref tileAnimationData); + } + + /// <summary> + /// Retrieves any tile rendering data from the scripted tile. + /// </summary> + /// <param name="position">Position of the Tile on the Tilemap.</param> + /// <param name="tilemap">The Tilemap the tile is present on.</param> + /// <param name="tileData">Data to render the tile.</param> + public override void GetTileData(Vector3Int position, ITilemap tilemap, ref TileData tileData) + { + if (!m_InstanceTile) + return; + m_InstanceTile.GetTileData(position, tilemap, ref tileData); + } + + /// <summary> + /// This method is called when the tile is refreshed. + /// </summary> + /// <param name="position">Position of the Tile on the Tilemap.</param> + /// <param name="tilemap">The Tilemap the tile is present on.</param> + public override void RefreshTile(Vector3Int position, ITilemap tilemap) + { + if (!m_InstanceTile) + return; + m_InstanceTile.RefreshTile(position, tilemap); + } + + /// <summary> + /// StartUp is called on the first frame of the running Scene. + /// </summary> + /// <param name="position">Position of the Tile on the Tilemap.</param> + /// <param name="tilemap">The Tilemap the tile is present on.</param> + /// <param name="go">The GameObject instantiated for the Tile.</param> + /// <returns>Whether StartUp was successful</returns> + public override bool StartUp(Vector3Int position, ITilemap tilemap, GameObject go) + { + if (!m_InstanceTile) + return true; + return m_InstanceTile.StartUp(position, tilemap, go); + } + + /// <summary> + /// Callback when the tile is enabled + /// </summary> + public void OnEnable() + { + if (m_Tile == null) + return; + + if (m_InstanceTile == null) + Override(); + } + } +} diff --git a/MrBigsock/Packages/com.unity.2d.tilemap.extras/Runtime/Tiles/RuleOverrideTile/RuleOverrideTile.cs.meta b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Runtime/Tiles/RuleOverrideTile/RuleOverrideTile.cs.meta new file mode 100644 index 0000000000000000000000000000000000000000..96ce07800699e8bdad5b0cba9c48beab333cdad0 --- /dev/null +++ b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Runtime/Tiles/RuleOverrideTile/RuleOverrideTile.cs.meta @@ -0,0 +1,11 @@ +fileFormatVersion: 2 +guid: 4c779848d9dd029409ca676b49e10a18 +MonoImporter: + externalObjects: {} + serializedVersion: 2 + defaultReferences: [] + executionOrder: 0 + icon: {instanceID: 0} + userData: + assetBundleName: + assetBundleVariant: diff --git a/MrBigsock/Packages/com.unity.2d.tilemap.extras/Runtime/Tiles/RuleTile.meta b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Runtime/Tiles/RuleTile.meta new file mode 100644 index 0000000000000000000000000000000000000000..ed5ce77eddbfe2b9429df89af0819977b930343a --- /dev/null +++ b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Runtime/Tiles/RuleTile.meta @@ -0,0 +1,8 @@ +fileFormatVersion: 2 +guid: ba757fb3d4cc7e641ab2b91814856b68 +folderAsset: yes +DefaultImporter: + externalObjects: {} + userData: + assetBundleName: + assetBundleVariant: diff --git a/MrBigsock/Packages/com.unity.2d.tilemap.extras/Runtime/Tiles/RuleTile/RuleTile.cs b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Runtime/Tiles/RuleTile/RuleTile.cs new file mode 100644 index 0000000000000000000000000000000000000000..21a1dcc4f4700b21d21214cb7ffcb91020646446 --- /dev/null +++ b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Runtime/Tiles/RuleTile/RuleTile.cs @@ -0,0 +1,829 @@ +using System; +using System.Linq; +using System.Reflection; +using System.Collections.Generic; +using UnityEngine.Tilemaps; +using UnityEngine.Serialization; + +namespace UnityEngine +{ + /// <summary> + /// Generic visual tile for creating different tilesets like terrain, pipeline, random or animated tiles. + /// This is templated to accept a Neighbor Rule Class for Custom Rules. + /// </summary> + /// <typeparam name="T">Neighbor Rule Class for Custom Rules</typeparam> + public class RuleTile<T> : RuleTile + { + /// <summary> + /// Returns the Neighbor Rule Class type for this Rule Tile. + /// </summary> + public sealed override Type m_NeighborType => typeof(T); + } + + /// <summary> + /// Generic visual tile for creating different tilesets like terrain, pipeline, random or animated tiles. + /// </summary> + [Serializable] + [HelpURL("https://docs.unity3d.com/Packages/com.unity.2d.tilemap.extras@latest/index.html?subfolder=/manual/RuleTile.html")] + public class RuleTile : TileBase + { + /// <summary> + /// Returns the default Neighbor Rule Class type. + /// </summary> + public virtual Type m_NeighborType => typeof(TilingRuleOutput.Neighbor); + + /// <summary> + /// The Default Sprite set when creating a new Rule. + /// </summary> + public Sprite m_DefaultSprite; + /// <summary> + /// The Default GameObject set when creating a new Rule. + /// </summary> + public GameObject m_DefaultGameObject; + /// <summary> + /// The Default Collider Type set when creating a new Rule. + /// </summary> + public Tile.ColliderType m_DefaultColliderType = Tile.ColliderType.Sprite; + + /// <summary> + /// Angle in which the RuleTile is rotated by for matching in Degrees. + /// </summary> + public virtual int m_RotationAngle => 90; + + /// <summary> + /// Number of rotations the RuleTile can be rotated by for matching. + /// </summary> + public int m_RotationCount => 360 / m_RotationAngle; + + /// <summary> + /// The data structure holding the Rule information for matching Rule Tiles with + /// its neighbors. + /// </summary> + [Serializable] + public class TilingRuleOutput + { + /// <summary> + /// Id for this Rule. + /// </summary> + public int m_Id; + /// <summary> + /// The output Sprites for this Rule. + /// </summary> + public Sprite[] m_Sprites = new Sprite[1]; + /// <summary> + /// The output GameObject for this Rule. + /// </summary> + public GameObject m_GameObject; + /// <summary> + /// The output minimum Animation Speed for this Rule. + /// </summary> + [FormerlySerializedAs("m_AnimationSpeed")] + public float m_MinAnimationSpeed = 1f; + /// <summary> + /// The output maximum Animation Speed for this Rule. + /// </summary> + [FormerlySerializedAs("m_AnimationSpeed")] + public float m_MaxAnimationSpeed = 1f; + /// <summary> + /// The perlin scale factor for this Rule. + /// </summary> + public float m_PerlinScale = 0.5f; + /// <summary> + /// The output type for this Rule. + /// </summary> + public OutputSprite m_Output = OutputSprite.Single; + /// <summary> + /// The output Collider Type for this Rule. + /// </summary> + public Tile.ColliderType m_ColliderType = Tile.ColliderType.Sprite; + /// <summary> + /// The randomized transform output for this Rule. + /// </summary> + public Transform m_RandomTransform; + + /// <summary> + /// The enumeration for matching Neighbors when matching Rule Tiles + /// </summary> + public class Neighbor + { + /// <summary> + /// The Rule Tile will check if the contents of the cell in that direction is an instance of this Rule Tile. + /// If not, the rule will fail. + /// </summary> + public const int This = 1; + /// <summary> + /// The Rule Tile will check if the contents of the cell in that direction is not an instance of this Rule Tile. + /// If it is, the rule will fail. + /// </summary> + public const int NotThis = 2; + } + + /// <summary> + /// The enumeration for the transform rule used when matching Rule Tiles. + /// </summary> + public enum Transform + { + /// <summary> + /// The Rule Tile will match Tiles exactly as laid out in its neighbors. + /// </summary> + Fixed, + /// <summary> + /// The Rule Tile will rotate and match its neighbors. + /// </summary> + Rotated, + /// <summary> + /// The Rule Tile will mirror in the X axis and match its neighbors. + /// </summary> + MirrorX, + /// <summary> + /// The Rule Tile will mirror in the Y axis and match its neighbors. + /// </summary> + MirrorY, + /// <summary> + /// The Rule Tile will mirror in the X or Y axis and match its neighbors. + /// </summary> + MirrorXY + } + + /// <summary> + /// The Output for the Tile which fits this Rule. + /// </summary> + public enum OutputSprite + { + /// <summary> + /// A Single Sprite will be output. + /// </summary> + Single, + /// <summary> + /// A Random Sprite will be output. + /// </summary> + Random, + /// <summary> + /// A Sprite Animation will be output. + /// </summary> + Animation + } + } + + /// <summary> + /// The data structure holding the Rule information for matching Rule Tiles with + /// its neighbors. + /// </summary> + [Serializable] + public class TilingRule : TilingRuleOutput + { + /// <summary> + /// The matching Rule conditions for each of its neighboring Tiles. + /// </summary> + public List<int> m_Neighbors = new List<int>(); + /// <summary> + /// * Preset this list to RuleTile backward compatible, but not support for HexagonalRuleTile backward compatible. + /// </summary> + public List<Vector3Int> m_NeighborPositions = new List<Vector3Int>() + { + new Vector3Int(-1, 1, 0), + new Vector3Int(0, 1, 0), + new Vector3Int(1, 1, 0), + new Vector3Int(-1, 0, 0), + new Vector3Int(1, 0, 0), + new Vector3Int(-1, -1, 0), + new Vector3Int(0, -1, 0), + new Vector3Int(1, -1, 0), + }; + /// <summary> + /// The transform matching Rule for this Rule. + /// </summary> + public Transform m_RuleTransform; + + /// <summary> + /// This clones a copy of the TilingRule. + /// </summary> + /// <returns>A copy of the TilingRule.</returns> + public TilingRule Clone() + { + TilingRule rule = new TilingRule + { + m_Neighbors = new List<int>(m_Neighbors), + m_NeighborPositions = new List<Vector3Int>(m_NeighborPositions), + m_RuleTransform = m_RuleTransform, + m_Sprites = new Sprite[m_Sprites.Length], + m_GameObject = m_GameObject, + m_MinAnimationSpeed = m_MinAnimationSpeed, + m_MaxAnimationSpeed = m_MaxAnimationSpeed, + m_PerlinScale = m_PerlinScale, + m_Output = m_Output, + m_ColliderType = m_ColliderType, + m_RandomTransform = m_RandomTransform, + }; + Array.Copy(m_Sprites, rule.m_Sprites, m_Sprites.Length); + return rule; + } + + /// <summary> + /// Returns all neighbors of this Tile as a dictionary + /// </summary> + /// <returns>A dictionary of neighbors for this Tile</returns> + public Dictionary<Vector3Int, int> GetNeighbors() + { + Dictionary<Vector3Int, int> dict = new Dictionary<Vector3Int, int>(); + + for (int i = 0; i < m_Neighbors.Count && i < m_NeighborPositions.Count; i++) + dict.Add(m_NeighborPositions[i], m_Neighbors[i]); + + return dict; + } + + /// <summary> + /// Applies the values from the given dictionary as this Tile's neighbors + /// </summary> + /// <param name="dict">Dictionary to apply values from</param> + public void ApplyNeighbors(Dictionary<Vector3Int, int> dict) + { + m_NeighborPositions = dict.Keys.ToList(); + m_Neighbors = dict.Values.ToList(); + } + + /// <summary> + /// Gets the cell bounds of the TilingRule. + /// </summary> + /// <returns>Returns the cell bounds of the TilingRule.</returns> + public BoundsInt GetBounds() + { + BoundsInt bounds = new BoundsInt(Vector3Int.zero, Vector3Int.one); + foreach (var neighbor in GetNeighbors()) + { + bounds.xMin = Mathf.Min(bounds.xMin, neighbor.Key.x); + bounds.yMin = Mathf.Min(bounds.yMin, neighbor.Key.y); + bounds.xMax = Mathf.Max(bounds.xMax, neighbor.Key.x + 1); + bounds.yMax = Mathf.Max(bounds.yMax, neighbor.Key.y + 1); + } + return bounds; + } + } + + /// <summary> + /// Attribute which marks a property which cannot be overridden by a RuleOverrideTile + /// </summary> + public class DontOverride : Attribute { } + + /// <summary> + /// A list of Tiling Rules for the Rule Tile. + /// </summary> + [HideInInspector] public List<TilingRule> m_TilingRules = new List<RuleTile.TilingRule>(); + + /// <summary> + /// Returns a set of neighboring positions for this RuleTile + /// </summary> + public HashSet<Vector3Int> neighborPositions + { + get + { + if (m_NeighborPositions.Count == 0) + UpdateNeighborPositions(); + + return m_NeighborPositions; + } + } + + private HashSet<Vector3Int> m_NeighborPositions = new HashSet<Vector3Int>(); + + /// <summary> + /// Updates the neighboring positions of this RuleTile + /// </summary> + public void UpdateNeighborPositions() + { + m_CacheTilemapsNeighborPositions.Clear(); + + HashSet<Vector3Int> positions = m_NeighborPositions; + positions.Clear(); + + foreach (TilingRule rule in m_TilingRules) + { + foreach (var neighbor in rule.GetNeighbors()) + { + Vector3Int position = neighbor.Key; + positions.Add(position); + + // Check rule against rotations of 0, 90, 180, 270 + if (rule.m_RuleTransform == TilingRuleOutput.Transform.Rotated) + { + for (int angle = m_RotationAngle; angle < 360; angle += m_RotationAngle) + { + positions.Add(GetRotatedPosition(position, angle)); + } + } + // Check rule against x-axis, y-axis mirror + else if (rule.m_RuleTransform == TilingRuleOutput.Transform.MirrorXY) + { + positions.Add(GetMirroredPosition(position, true, true)); + positions.Add(GetMirroredPosition(position, true, false)); + positions.Add(GetMirroredPosition(position, false, true)); + } + // Check rule against x-axis mirror + else if (rule.m_RuleTransform == TilingRuleOutput.Transform.MirrorX) + { + positions.Add(GetMirroredPosition(position, true, false)); + } + // Check rule against y-axis mirror + else if (rule.m_RuleTransform == TilingRuleOutput.Transform.MirrorY) + { + positions.Add(GetMirroredPosition(position, false, true)); + } + } + } + } + + /// <summary> + /// StartUp is called on the first frame of the running Scene. + /// </summary> + /// <param name="position">Position of the Tile on the Tilemap.</param> + /// <param name="tilemap">The Tilemap the tile is present on.</param> + /// <param name="instantiatedGameObject">The GameObject instantiated for the Tile.</param> + /// <returns>Whether StartUp was successful</returns> + public override bool StartUp(Vector3Int position, ITilemap tilemap, GameObject instantiatedGameObject) + { + if (instantiatedGameObject != null) + { + Tilemap tmpMap = tilemap.GetComponent<Tilemap>(); + Matrix4x4 orientMatrix = tmpMap.orientationMatrix; + + var iden = Matrix4x4.identity; + Vector3 gameObjectTranslation = new Vector3(); + Quaternion gameObjectRotation = new Quaternion(); + Vector3 gameObjectScale = new Vector3(); + + bool ruleMatched = false; + Matrix4x4 transform = iden; + foreach (TilingRule rule in m_TilingRules) + { + if (RuleMatches(rule, position, tilemap, ref transform)) + { + transform = orientMatrix * transform; + + // Converts the tile's translation, rotation, & scale matrix to values to be used by the instantiated GameObject + gameObjectTranslation = new Vector3(transform.m03, transform.m13, transform.m23); + gameObjectRotation = Quaternion.LookRotation(new Vector3(transform.m02, transform.m12, transform.m22), new Vector3(transform.m01, transform.m11, transform.m21)); + gameObjectScale = transform.lossyScale; + + ruleMatched = true; + break; + } + } + if (!ruleMatched) + { + // Fallback to just using the orientMatrix for the translation, rotation, & scale values. + gameObjectTranslation = new Vector3(orientMatrix.m03, orientMatrix.m13, orientMatrix.m23); + gameObjectRotation = Quaternion.LookRotation(new Vector3(orientMatrix.m02, orientMatrix.m12, orientMatrix.m22), new Vector3(orientMatrix.m01, orientMatrix.m11, orientMatrix.m21)); + gameObjectScale = orientMatrix.lossyScale; + } + + instantiatedGameObject.transform.localPosition = gameObjectTranslation + tmpMap.CellToLocalInterpolated(position + tmpMap.tileAnchor); + instantiatedGameObject.transform.localRotation = gameObjectRotation; + instantiatedGameObject.transform.localScale = gameObjectScale; + } + + return true; + } + + /// <summary> + /// Retrieves any tile rendering data from the scripted tile. + /// </summary> + /// <param name="position">Position of the Tile on the Tilemap.</param> + /// <param name="tilemap">The Tilemap the tile is present on.</param> + /// <param name="tileData">Data to render the tile.</param> + public override void GetTileData(Vector3Int position, ITilemap tilemap, ref TileData tileData) + { + var iden = Matrix4x4.identity; + + tileData.sprite = m_DefaultSprite; + tileData.gameObject = m_DefaultGameObject; + tileData.colliderType = m_DefaultColliderType; + tileData.flags = TileFlags.LockTransform; + tileData.transform = iden; + + Matrix4x4 transform = iden; + foreach (TilingRule rule in m_TilingRules) + { + if (RuleMatches(rule, position, tilemap, ref transform)) + { + switch (rule.m_Output) + { + case TilingRuleOutput.OutputSprite.Single: + case TilingRuleOutput.OutputSprite.Animation: + tileData.sprite = rule.m_Sprites[0]; + break; + case TilingRuleOutput.OutputSprite.Random: + int index = Mathf.Clamp(Mathf.FloorToInt(GetPerlinValue(position, rule.m_PerlinScale, 100000f) * rule.m_Sprites.Length), 0, rule.m_Sprites.Length - 1); + tileData.sprite = rule.m_Sprites[index]; + if (rule.m_RandomTransform != TilingRuleOutput.Transform.Fixed) + transform = ApplyRandomTransform(rule.m_RandomTransform, transform, rule.m_PerlinScale, position); + break; + } + tileData.transform = transform; + tileData.gameObject = rule.m_GameObject; + tileData.colliderType = rule.m_ColliderType; + break; + } + } + } + + /// <summary> + /// Returns a Perlin Noise value based on the given inputs. + /// </summary> + /// <param name="position">Position of the Tile on the Tilemap.</param> + /// <param name="scale">The Perlin Scale factor of the Tile.</param> + /// <param name="offset">Offset of the Tile on the Tilemap.</param> + /// <returns>A Perlin Noise value based on the given inputs.</returns> + public static float GetPerlinValue(Vector3Int position, float scale, float offset) + { + return Mathf.PerlinNoise((position.x + offset) * scale, (position.y + offset) * scale); + } + + static Dictionary<Tilemap, KeyValuePair<HashSet<TileBase>, HashSet<Vector3Int>>> m_CacheTilemapsNeighborPositions = new Dictionary<Tilemap, KeyValuePair<HashSet<TileBase>, HashSet<Vector3Int>>>(); + static TileBase[] m_AllocatedUsedTileArr = Array.Empty<TileBase>(); + + static bool IsTilemapUsedTilesChange(Tilemap tilemap, out KeyValuePair<HashSet<TileBase>, HashSet<Vector3Int>> hashSet) + { + if (!m_CacheTilemapsNeighborPositions.TryGetValue(tilemap, out hashSet)) + return true; + + var oldUsedTiles = hashSet.Key; + int newUsedTilesCount = tilemap.GetUsedTilesCount(); + if (newUsedTilesCount != oldUsedTiles.Count) + return true; + + if (m_AllocatedUsedTileArr.Length < newUsedTilesCount) + Array.Resize(ref m_AllocatedUsedTileArr, newUsedTilesCount); + + tilemap.GetUsedTilesNonAlloc(m_AllocatedUsedTileArr); + for (int i = 0; i < newUsedTilesCount; i++) + { + TileBase newUsedTile = m_AllocatedUsedTileArr[i]; + if (!oldUsedTiles.Contains(newUsedTile)) + return true; + } + + return false; + } + + static KeyValuePair<HashSet<TileBase>, HashSet<Vector3Int>> CachingTilemapNeighborPositions(Tilemap tilemap) + { + int usedTileCount = tilemap.GetUsedTilesCount(); + HashSet<TileBase> usedTiles = new HashSet<TileBase>(); + HashSet<Vector3Int> neighborPositions = new HashSet<Vector3Int>(); + + if (m_AllocatedUsedTileArr.Length < usedTileCount) + Array.Resize(ref m_AllocatedUsedTileArr, usedTileCount); + + tilemap.GetUsedTilesNonAlloc(m_AllocatedUsedTileArr); + + for (int i = 0; i < usedTileCount; i++) + { + TileBase tile = m_AllocatedUsedTileArr[i]; + usedTiles.Add(tile); + RuleTile ruleTile = null; + + if (tile is RuleTile rt) + ruleTile = rt; + else if (tile is RuleOverrideTile ot) + ruleTile = ot.m_Tile; + + if (ruleTile) + foreach (Vector3Int neighborPosition in ruleTile.neighborPositions) + neighborPositions.Add(neighborPosition); + } + + var value = new KeyValuePair<HashSet<TileBase>, HashSet<Vector3Int>>(usedTiles, neighborPositions); + m_CacheTilemapsNeighborPositions[tilemap] = value; + return value; + } + + static bool NeedRelease() + { + foreach (var keypair in m_CacheTilemapsNeighborPositions) + { + if (keypair.Key == null) + { + return true; + } + } + return false; + } + + static void ReleaseDestroyedTilemapCacheData() + { + if (!NeedRelease()) + return; + + var hasCleared = false; + var keys = m_CacheTilemapsNeighborPositions.Keys.ToArray(); + foreach (var key in keys) + { + if (key == null && m_CacheTilemapsNeighborPositions.Remove(key)) + hasCleared = true; + } + if (hasCleared) + { + // TrimExcess + m_CacheTilemapsNeighborPositions = new Dictionary<Tilemap, KeyValuePair<HashSet<TileBase>, HashSet<Vector3Int>>>(m_CacheTilemapsNeighborPositions); + } + } + + /// <summary> + /// Retrieves any tile animation data from the scripted tile. + /// </summary> + /// <param name="position">Position of the Tile on the Tilemap.</param> + /// <param name="tilemap">The Tilemap the tile is present on.</param> + /// <param name="tileAnimationData">Data to run an animation on the tile.</param> + /// <returns>Whether the call was successful.</returns> + public override bool GetTileAnimationData(Vector3Int position, ITilemap tilemap, ref TileAnimationData tileAnimationData) + { + Matrix4x4 transform = Matrix4x4.identity; + foreach (TilingRule rule in m_TilingRules) + { + if (rule.m_Output == TilingRuleOutput.OutputSprite.Animation) + { + if (RuleMatches(rule, position, tilemap, ref transform)) + { + tileAnimationData.animatedSprites = rule.m_Sprites; + tileAnimationData.animationSpeed = Random.Range( rule.m_MinAnimationSpeed, rule.m_MaxAnimationSpeed); + return true; + } + } + } + return false; + } + + /// <summary> + /// This method is called when the tile is refreshed. + /// </summary> + /// <param name="position">Position of the Tile on the Tilemap.</param> + /// <param name="tilemap">The Tilemap the tile is present on.</param> + public override void RefreshTile(Vector3Int position, ITilemap tilemap) + { + base.RefreshTile(position, tilemap); + + Tilemap baseTilemap = tilemap.GetComponent<Tilemap>(); + + ReleaseDestroyedTilemapCacheData(); // Prevent memory leak + + if (IsTilemapUsedTilesChange(baseTilemap, out var neighborPositionsSet)) + neighborPositionsSet = CachingTilemapNeighborPositions(baseTilemap); + + var neighborPositionsRuleTile = neighborPositionsSet.Value; + foreach (Vector3Int offset in neighborPositionsRuleTile) + { + Vector3Int offsetPosition = GetOffsetPositionReverse(position, offset); + TileBase tile = tilemap.GetTile(offsetPosition); + RuleTile ruleTile = null; + + if (tile is RuleTile rt) + ruleTile = rt; + else if (tile is RuleOverrideTile ot) + ruleTile = ot.m_Tile; + + if (ruleTile != null) + if (ruleTile == this || ruleTile.neighborPositions.Contains(offset)) + base.RefreshTile(offsetPosition, tilemap); + } + } + + /// <summary> + /// Does a Rule Match given a Tiling Rule and neighboring Tiles. + /// </summary> + /// <param name="rule">The Tiling Rule to match with.</param> + /// <param name="position">Position of the Tile on the Tilemap.</param> + /// <param name="tilemap">The tilemap to match with.</param> + /// <param name="transform">A transform matrix which will match the Rule.</param> + /// <returns>True if there is a match, False if not.</returns> + public virtual bool RuleMatches(TilingRule rule, Vector3Int position, ITilemap tilemap, ref Matrix4x4 transform) + { + if (RuleMatches(rule, position, tilemap, 0)) + { + transform = Matrix4x4.TRS(Vector3.zero, Quaternion.Euler(0f, 0f, 0f), Vector3.one); + return true; + } + + // Check rule against rotations of 0, 90, 180, 270 + if (rule.m_RuleTransform == TilingRuleOutput.Transform.Rotated) + { + for (int angle = m_RotationAngle; angle < 360; angle += m_RotationAngle) + { + if (RuleMatches(rule, position, tilemap, angle)) + { + transform = Matrix4x4.TRS(Vector3.zero, Quaternion.Euler(0f, 0f, -angle), Vector3.one); + return true; + } + } + } + // Check rule against x-axis, y-axis mirror + else if (rule.m_RuleTransform == TilingRuleOutput.Transform.MirrorXY) + { + if (RuleMatches(rule, position, tilemap, true, true)) + { + transform = Matrix4x4.TRS(Vector3.zero, Quaternion.identity, new Vector3(-1f, -1f, 1f)); + return true; + } + if (RuleMatches(rule, position, tilemap, true, false)) + { + transform = Matrix4x4.TRS(Vector3.zero, Quaternion.identity, new Vector3(-1f, 1f, 1f)); + return true; + } + if (RuleMatches(rule, position, tilemap, false, true)) + { + transform = Matrix4x4.TRS(Vector3.zero, Quaternion.identity, new Vector3(1f, -1f, 1f)); + return true; + } + } + // Check rule against x-axis mirror + else if (rule.m_RuleTransform == TilingRuleOutput.Transform.MirrorX) + { + if (RuleMatches(rule, position, tilemap, true, false)) + { + transform = Matrix4x4.TRS(Vector3.zero, Quaternion.identity, new Vector3(-1f, 1f, 1f)); + return true; + } + } + // Check rule against y-axis mirror + else if (rule.m_RuleTransform == TilingRuleOutput.Transform.MirrorY) + { + if (RuleMatches(rule, position, tilemap, false, true)) + { + transform = Matrix4x4.TRS(Vector3.zero, Quaternion.identity, new Vector3(1f, -1f, 1f)); + return true; + } + } + + return false; + } + + /// <summary> + /// Returns a random transform matrix given the random transform rule. + /// </summary> + /// <param name="type">Random transform rule.</param> + /// <param name="original">The original transform matrix.</param> + /// <param name="perlinScale">The Perlin Scale factor of the Tile.</param> + /// <param name="position">Position of the Tile on the Tilemap.</param> + /// <returns>A random transform matrix.</returns> + public virtual Matrix4x4 ApplyRandomTransform(TilingRuleOutput.Transform type, Matrix4x4 original, float perlinScale, Vector3Int position) + { + float perlin = GetPerlinValue(position, perlinScale, 200000f); + switch (type) + { + case TilingRuleOutput.Transform.MirrorXY: + return original * Matrix4x4.TRS(Vector3.zero, Quaternion.identity, new Vector3(Math.Abs(perlin - 0.5) > 0.25 ? 1f : -1f, perlin < 0.5 ? 1f : -1f, 1f)); + case TilingRuleOutput.Transform.MirrorX: + return original * Matrix4x4.TRS(Vector3.zero, Quaternion.identity, new Vector3(perlin < 0.5 ? 1f : -1f, 1f, 1f)); + case TilingRuleOutput.Transform.MirrorY: + return original * Matrix4x4.TRS(Vector3.zero, Quaternion.identity, new Vector3(1f, perlin < 0.5 ? 1f : -1f, 1f)); + case TilingRuleOutput.Transform.Rotated: + int angle = Mathf.Clamp(Mathf.FloorToInt(perlin * m_RotationCount), 0, m_RotationCount - 1) * m_RotationAngle; + return Matrix4x4.TRS(Vector3.zero, Quaternion.Euler(0f, 0f, -angle), Vector3.one); + } + return original; + } + + /// <summary> + /// Returns custom fields for this RuleTile + /// </summary> + /// <param name="isOverrideInstance">Whether override fields are returned</param> + /// <returns>Custom fields for this RuleTile</returns> + public FieldInfo[] GetCustomFields(bool isOverrideInstance) + { + return this.GetType().GetFields(BindingFlags.Instance | BindingFlags.Static | BindingFlags.Public | BindingFlags.NonPublic) + .Where(field => typeof(RuleTile).GetField(field.Name) == null) + .Where(field => field.IsPublic || field.IsDefined(typeof(SerializeField))) + .Where(field => !field.IsDefined(typeof(HideInInspector))) + .Where(field => !isOverrideInstance || !field.IsDefined(typeof(DontOverride))) + .ToArray(); + } + + /// <summary> + /// Checks if there is a match given the neighbor matching rule and a Tile. + /// </summary> + /// <param name="neighbor">Neighbor matching rule.</param> + /// <param name="other">Tile to match.</param> + /// <returns>True if there is a match, False if not.</returns> + public virtual bool RuleMatch(int neighbor, TileBase other) + { + if (other is RuleOverrideTile ot) + other = ot.m_InstanceTile; + + switch (neighbor) + { + case TilingRuleOutput.Neighbor.This: return other == this; + case TilingRuleOutput.Neighbor.NotThis: return other != this; + } + return true; + } + + /// <summary> + /// Checks if there is a match given the neighbor matching rule and a Tile with a rotation angle. + /// </summary> + /// <param name="rule">Neighbor matching rule.</param> + /// <param name="position">Position of the Tile on the Tilemap.</param> + /// <param name="tilemap">Tilemap to match.</param> + /// <param name="angle">Rotation angle for matching.</param> + /// <returns>True if there is a match, False if not.</returns> + public bool RuleMatches(TilingRule rule, Vector3Int position, ITilemap tilemap, int angle) + { + var minCount = Math.Min(rule.m_Neighbors.Count, rule.m_NeighborPositions.Count); + for (int i = 0; i < minCount ; i++) + { + int neighbor = rule.m_Neighbors[i]; + Vector3Int positionOffset = GetRotatedPosition(rule.m_NeighborPositions[i], angle); + TileBase other = tilemap.GetTile(GetOffsetPosition(position, positionOffset)); + if (!RuleMatch(neighbor, other)) + { + return false; + } + } + return true; + } + + /// <summary> + /// Checks if there is a match given the neighbor matching rule and a Tile with mirrored axii. + /// </summary> + /// <param name="rule">Neighbor matching rule.</param> + /// <param name="position">Position of the Tile on the Tilemap.</param> + /// <param name="tilemap">Tilemap to match.</param> + /// <param name="mirrorX">Mirror X Axis for matching.</param> + /// <param name="mirrorY">Mirror Y Axis for matching.</param> + /// <returns>True if there is a match, False if not.</returns> + public bool RuleMatches(TilingRule rule, Vector3Int position, ITilemap tilemap, bool mirrorX, bool mirrorY) + { + var minCount = Math.Min(rule.m_Neighbors.Count, rule.m_NeighborPositions.Count); + for (int i = 0; i < minCount; i++) + { + int neighbor = rule.m_Neighbors[i]; + Vector3Int positionOffset = GetMirroredPosition(rule.m_NeighborPositions[i], mirrorX, mirrorY); + TileBase other = tilemap.GetTile(GetOffsetPosition(position, positionOffset)); + if (!RuleMatch(neighbor, other)) + { + return false; + } + } + return true; + } + + /// <summary> + /// Gets a rotated position given its original position and the rotation in degrees. + /// </summary> + /// <param name="position">Original position of Tile.</param> + /// <param name="rotation">Rotation in degrees.</param> + /// <returns>Rotated position of Tile.</returns> + public virtual Vector3Int GetRotatedPosition(Vector3Int position, int rotation) + { + switch (rotation) + { + case 0: + return position; + case 90: + return new Vector3Int(position.y, -position.x, 0); + case 180: + return new Vector3Int(-position.x, -position.y, 0); + case 270: + return new Vector3Int(-position.y, position.x, 0); + } + return position; + } + + /// <summary> + /// Gets a mirrored position given its original position and the mirroring axii. + /// </summary> + /// <param name="position">Original position of Tile.</param> + /// <param name="mirrorX">Mirror in the X Axis.</param> + /// <param name="mirrorY">Mirror in the Y Axis.</param> + /// <returns>Mirrored position of Tile.</returns> + public virtual Vector3Int GetMirroredPosition(Vector3Int position, bool mirrorX, bool mirrorY) + { + if (mirrorX) + position.x *= -1; + if (mirrorY) + position.y *= -1; + return position; + } + + /// <summary> + /// Get the offset for the given position with the given offset. + /// </summary> + /// <param name="position">Position to offset.</param> + /// <param name="offset">Offset for the position.</param> + /// <returns>The offset position.</returns> + public virtual Vector3Int GetOffsetPosition(Vector3Int position, Vector3Int offset) + { + return position + offset; + } + + /// <summary> + /// Get the reversed offset for the given position with the given offset. + /// </summary> + /// <param name="position">Position to offset.</param> + /// <param name="offset">Offset for the position.</param> + /// <returns>The reversed offset position.</returns> + public virtual Vector3Int GetOffsetPositionReverse(Vector3Int position, Vector3Int offset) + { + return position - offset; + } + } +} diff --git a/MrBigsock/Packages/com.unity.2d.tilemap.extras/Runtime/Tiles/RuleTile/RuleTile.cs.meta b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Runtime/Tiles/RuleTile/RuleTile.cs.meta new file mode 100644 index 0000000000000000000000000000000000000000..d3ce2de9ea421bfbb5322ce77fc04db2a12a9a83 --- /dev/null +++ b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Runtime/Tiles/RuleTile/RuleTile.cs.meta @@ -0,0 +1,13 @@ +fileFormatVersion: 2 +guid: 9d1514134bc4fbd41bb739b1b9a49231 +timeCreated: 1501789622 +licenseType: Pro +MonoImporter: + externalObjects: {} + serializedVersion: 2 + defaultReferences: [] + executionOrder: 0 + icon: {instanceID: 0} + userData: + assetBundleName: + assetBundleVariant: diff --git a/MrBigsock/Packages/com.unity.2d.tilemap.extras/Runtime/Tiles/TerrainTile.meta b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Runtime/Tiles/TerrainTile.meta new file mode 100644 index 0000000000000000000000000000000000000000..677327dc6d845c4621e6872d4399931aaa471c54 --- /dev/null +++ b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Runtime/Tiles/TerrainTile.meta @@ -0,0 +1,8 @@ +fileFormatVersion: 2 +guid: bb65a41a206451e419f4513e440069d3 +folderAsset: yes +DefaultImporter: + externalObjects: {} + userData: + assetBundleName: + assetBundleVariant: diff --git a/MrBigsock/Packages/com.unity.2d.tilemap.extras/Runtime/Tiles/TerrainTile/TerrainTile.cs b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Runtime/Tiles/TerrainTile/TerrainTile.cs new file mode 100644 index 0000000000000000000000000000000000000000..c6442a2af972b9efcae7e5bce7c5214f20999c29 --- /dev/null +++ b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Runtime/Tiles/TerrainTile/TerrainTile.cs @@ -0,0 +1,236 @@ +using System; + +#if UNITY_EDITOR +using UnityEditor; +#endif + +namespace UnityEngine.Tilemaps +{ + /// <summary> + /// Terrain Tiles, similar to Pipeline Tiles, are tiles which take into consideration its orthogonal and diagonal neighboring tiles and displays a sprite depending on whether the neighboring tile is the same tile. + /// </summary> + [Serializable] + public class TerrainTile : TileBase + { + /// <summary> + /// The Sprites used for defining the Terrain. + /// </summary> + [SerializeField] + public Sprite[] m_Sprites; + + /// <summary> + /// This method is called when the tile is refreshed. + /// </summary> + /// <param name="position">Position of the Tile on the Tilemap.</param> + /// <param name="tilemap">The Tilemap the tile is present on.</param> + public override void RefreshTile(Vector3Int position, ITilemap tilemap) + { + for (int yd = -1; yd <= 1; yd++) + for (int xd = -1; xd <= 1; xd++) + { + Vector3Int pos = new Vector3Int(position.x + xd, position.y + yd, position.z); + if (TileValue(tilemap, pos)) + tilemap.RefreshTile(pos); + } + } + + /// <summary> + /// Retrieves any tile rendering data from the scripted tile. + /// </summary> + /// <param name="position">Position of the Tile on the Tilemap.</param> + /// <param name="tilemap">The Tilemap the tile is present on.</param> + /// <param name="tileData">Data to render the tile.</param> + public override void GetTileData(Vector3Int position, ITilemap tilemap, ref TileData tileData) + { + UpdateTile(position, tilemap, ref tileData); + } + + private void UpdateTile(Vector3Int location, ITilemap tileMap, ref TileData tileData) + { + tileData.transform = Matrix4x4.identity; + tileData.color = Color.white; + + int mask = TileValue(tileMap, location + new Vector3Int(0, 1, 0)) ? 1 : 0; + mask += TileValue(tileMap, location + new Vector3Int(1, 1, 0)) ? 2 : 0; + mask += TileValue(tileMap, location + new Vector3Int(1, 0, 0)) ? 4 : 0; + mask += TileValue(tileMap, location + new Vector3Int(1, -1, 0)) ? 8 : 0; + mask += TileValue(tileMap, location + new Vector3Int(0, -1, 0)) ? 16 : 0; + mask += TileValue(tileMap, location + new Vector3Int(-1, -1, 0)) ? 32 : 0; + mask += TileValue(tileMap, location + new Vector3Int(-1, 0, 0)) ? 64 : 0; + mask += TileValue(tileMap, location + new Vector3Int(-1, 1, 0)) ? 128 : 0; + + byte original = (byte)mask; + if ((original | 254) < 255) { mask = mask & 125; } + if ((original | 251) < 255) { mask = mask & 245; } + if ((original | 239) < 255) { mask = mask & 215; } + if ((original | 191) < 255) { mask = mask & 95; } + + int index = GetIndex((byte)mask); + if (index >= 0 && index < m_Sprites.Length && TileValue(tileMap, location)) + { + tileData.sprite = m_Sprites[index]; + tileData.transform = GetTransform((byte)mask); + tileData.color = Color.white; + tileData.flags = TileFlags.LockTransform | TileFlags.LockColor; + tileData.colliderType = Tile.ColliderType.Sprite; + } + } + + private bool TileValue(ITilemap tileMap, Vector3Int position) + { + TileBase tile = tileMap.GetTile(position); + return (tile != null && tile == this); + } + + private int GetIndex(byte mask) + { + switch (mask) + { + case 0: return 0; + case 1: + case 4: + case 16: + case 64: return 1; + case 5: + case 20: + case 80: + case 65: return 2; + case 7: + case 28: + case 112: + case 193: return 3; + case 17: + case 68: return 4; + case 21: + case 84: + case 81: + case 69: return 5; + case 23: + case 92: + case 113: + case 197: return 6; + case 29: + case 116: + case 209: + case 71: return 7; + case 31: + case 124: + case 241: + case 199: return 8; + case 85: return 9; + case 87: + case 93: + case 117: + case 213: return 10; + case 95: + case 125: + case 245: + case 215: return 11; + case 119: + case 221: return 12; + case 127: + case 253: + case 247: + case 223: return 13; + case 255: return 14; + } + return -1; + } + + private Matrix4x4 GetTransform(byte mask) + { + switch (mask) + { + case 4: + case 20: + case 28: + case 68: + case 84: + case 92: + case 116: + case 124: + case 93: + case 125: + case 221: + case 253: + return Matrix4x4.TRS(Vector3.zero, Quaternion.Euler(0f, 0f, -90f), Vector3.one); + case 16: + case 80: + case 112: + case 81: + case 113: + case 209: + case 241: + case 117: + case 245: + case 247: + return Matrix4x4.TRS(Vector3.zero, Quaternion.Euler(0f, 0f, -180f), Vector3.one); + case 64: + case 65: + case 193: + case 69: + case 197: + case 71: + case 199: + case 213: + case 215: + case 223: + return Matrix4x4.TRS(Vector3.zero, Quaternion.Euler(0f, 0f, -270f), Vector3.one); + } + return Matrix4x4.identity; + } + } + +#if UNITY_EDITOR + [CustomEditor(typeof(TerrainTile))] + public class TerrainTileEditor : Editor + { + private TerrainTile tile { get { return (target as TerrainTile); } } + + /// <summary> + /// OnEnable for TerrainTile. + /// </summary> + public void OnEnable() + { + if (tile.m_Sprites == null || tile.m_Sprites.Length != 15) + { + tile.m_Sprites = new Sprite[15]; + EditorUtility.SetDirty(tile); + } + } + + /// <summary> + /// Draws an Inspector for the Terrain Tile. + /// </summary> + public override void OnInspectorGUI() + { + EditorGUILayout.LabelField("Place sprites shown based on the contents of the sprite."); + EditorGUILayout.Space(); + + float oldLabelWidth = EditorGUIUtility.labelWidth; + EditorGUIUtility.labelWidth = 210; + + EditorGUI.BeginChangeCheck(); + tile.m_Sprites[0] = (Sprite) EditorGUILayout.ObjectField("Filled", tile.m_Sprites[0], typeof(Sprite), false, null); + tile.m_Sprites[1] = (Sprite) EditorGUILayout.ObjectField("Three Sides", tile.m_Sprites[1], typeof(Sprite), false, null); + tile.m_Sprites[2] = (Sprite) EditorGUILayout.ObjectField("Two Sides and One Corner", tile.m_Sprites[2], typeof(Sprite), false, null); + tile.m_Sprites[3] = (Sprite) EditorGUILayout.ObjectField("Two Adjacent Sides", tile.m_Sprites[3], typeof(Sprite), false, null); + tile.m_Sprites[4] = (Sprite) EditorGUILayout.ObjectField("Two Opposite Sides", tile.m_Sprites[4], typeof(Sprite), false, null); + tile.m_Sprites[5] = (Sprite) EditorGUILayout.ObjectField("One Side and Two Corners", tile.m_Sprites[5], typeof(Sprite), false, null); + tile.m_Sprites[6] = (Sprite) EditorGUILayout.ObjectField("One Side and One Lower Corner", tile.m_Sprites[6], typeof(Sprite), false, null); + tile.m_Sprites[7] = (Sprite) EditorGUILayout.ObjectField("One Side and One Upper Corner", tile.m_Sprites[7], typeof(Sprite), false, null); + tile.m_Sprites[8] = (Sprite) EditorGUILayout.ObjectField("One Side", tile.m_Sprites[8], typeof(Sprite), false, null); + tile.m_Sprites[9] = (Sprite) EditorGUILayout.ObjectField("Four Corners", tile.m_Sprites[9], typeof(Sprite), false, null); + tile.m_Sprites[10] = (Sprite) EditorGUILayout.ObjectField("Three Corners", tile.m_Sprites[10], typeof(Sprite), false, null); + tile.m_Sprites[11] = (Sprite) EditorGUILayout.ObjectField("Two Adjacent Corners", tile.m_Sprites[11], typeof(Sprite), false, null); + tile.m_Sprites[12] = (Sprite) EditorGUILayout.ObjectField("Two Opposite Corners", tile.m_Sprites[12], typeof(Sprite), false, null); + tile.m_Sprites[13] = (Sprite) EditorGUILayout.ObjectField("One Corner", tile.m_Sprites[13], typeof(Sprite), false, null); + tile.m_Sprites[14] = (Sprite) EditorGUILayout.ObjectField("Empty", tile.m_Sprites[14], typeof(Sprite), false, null); + if (EditorGUI.EndChangeCheck()) + EditorUtility.SetDirty(tile); + + EditorGUIUtility.labelWidth = oldLabelWidth; + } + } +#endif +} diff --git a/MrBigsock/Packages/com.unity.2d.tilemap.extras/Runtime/Tiles/TerrainTile/TerrainTile.cs.meta b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Runtime/Tiles/TerrainTile/TerrainTile.cs.meta new file mode 100644 index 0000000000000000000000000000000000000000..c0a64655884c5a2431960b8608cec3aa5043ec6f --- /dev/null +++ b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Runtime/Tiles/TerrainTile/TerrainTile.cs.meta @@ -0,0 +1,12 @@ +fileFormatVersion: 2 +guid: f6e4e4fc705376343a3e65b25d94f0e2 +timeCreated: 1464534739 +licenseType: Pro +MonoImporter: + serializedVersion: 2 + defaultReferences: [] + executionOrder: 0 + icon: {instanceID: 0} + userData: + assetBundleName: + assetBundleVariant: diff --git a/MrBigsock/Packages/com.unity.2d.tilemap.extras/Runtime/Tiles/WeightedRandomTile.meta b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Runtime/Tiles/WeightedRandomTile.meta new file mode 100644 index 0000000000000000000000000000000000000000..bc6af0950e8dcc7dc79d847cfd16de9c345c5607 --- /dev/null +++ b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Runtime/Tiles/WeightedRandomTile.meta @@ -0,0 +1,8 @@ +fileFormatVersion: 2 +guid: c1ac7817487f7984ab80d00618cf091a +folderAsset: yes +DefaultImporter: + externalObjects: {} + userData: + assetBundleName: + assetBundleVariant: diff --git a/MrBigsock/Packages/com.unity.2d.tilemap.extras/Runtime/Tiles/WeightedRandomTile/WeightedRandomTile.cs b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Runtime/Tiles/WeightedRandomTile/WeightedRandomTile.cs new file mode 100644 index 0000000000000000000000000000000000000000..a3e2843509ea67cb064354a3cdd6d33fbdfeb910 --- /dev/null +++ b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Runtime/Tiles/WeightedRandomTile/WeightedRandomTile.cs @@ -0,0 +1,140 @@ +using System; + +#if UNITY_EDITOR +using UnityEditor; +#endif + +namespace UnityEngine.Tilemaps +{ + /// <summary> + /// A Sprite with a Weight value for randomization. + /// </summary> + [Serializable] + public struct WeightedSprite + { + /// <summary> + /// Sprite. + /// </summary> + public Sprite Sprite; + /// <summary> + /// Weight of the Sprite. + /// </summary> + public int Weight; + } + + /// <summary> + /// Weighted Random Tiles are tiles which randomly pick a sprite from a given list of sprites and a target location, and displays that sprite. + /// The sprites can be weighted with a value to change its probability of appearing. The Sprite displayed for the Tile is randomized based on its location and will be fixed for that particular location. + /// </summary> + [Serializable] + public class WeightedRandomTile : Tile + { + /// <summary> + /// The Sprites used for randomizing output. + /// </summary> + [SerializeField] public WeightedSprite[] Sprites; + + /// <summary> + /// Retrieves any tile rendering data from the scripted tile. + /// </summary> + /// <param name="position">Position of the Tile on the Tilemap.</param> + /// <param name="tilemap">The Tilemap the tile is present on.</param> + /// <param name="tileData">Data to render the tile.</param> + public override void GetTileData(Vector3Int position, ITilemap tilemap, ref TileData tileData) + { + base.GetTileData(position, tilemap, ref tileData); + + if (Sprites == null || Sprites.Length <= 0) return; + + var oldState = Random.state; + long hash = position.x; + hash = hash + 0xabcd1234 + (hash << 15); + hash = hash + 0x0987efab ^ (hash >> 11); + hash ^= position.y; + hash = hash + 0x46ac12fd + (hash << 7); + hash = hash + 0xbe9730af ^ (hash << 11); + Random.InitState((int) hash); + + // Get the cumulative weight of the sprites + var cumulativeWeight = 0; + foreach (var spriteInfo in Sprites) cumulativeWeight += spriteInfo.Weight; + + // Pick a random weight and choose a sprite depending on it + var randomWeight = Random.Range(0, cumulativeWeight); + foreach (var spriteInfo in Sprites) + { + randomWeight -= spriteInfo.Weight; + if (randomWeight < 0) + { + tileData.sprite = spriteInfo.Sprite; + break; + } + } + Random.state = oldState; + } + } + +#if UNITY_EDITOR + [CustomEditor(typeof(WeightedRandomTile))] + public class WeightedRandomTileEditor : Editor + { + private SerializedProperty m_Color; + private SerializedProperty m_ColliderType; + + private WeightedRandomTile Tile { + get { return target as WeightedRandomTile; } + } + + /// <summary> + /// OnEnable for WeightedRandomTile. + /// </summary> + public void OnEnable() + { + m_Color = serializedObject.FindProperty("m_Color"); + m_ColliderType = serializedObject.FindProperty("m_ColliderType"); + } + + /// <summary> + /// Draws an Inspector for the WeightedRandomTile. + /// </summary> + public override void OnInspectorGUI() + { + serializedObject.Update(); + + EditorGUI.BeginChangeCheck(); + + int count = EditorGUILayout.DelayedIntField("Number of Sprites", Tile.Sprites != null ? Tile.Sprites.Length : 0); + if (count < 0) + count = 0; + + if (Tile.Sprites == null || Tile.Sprites.Length != count) + { + Array.Resize(ref Tile.Sprites, count); + } + + if (count == 0) + return; + + EditorGUILayout.LabelField("Place random sprites."); + EditorGUILayout.Space(); + + for (int i = 0; i < count; i++) + { + Tile.Sprites[i].Sprite = (Sprite) EditorGUILayout.ObjectField("Sprite " + (i + 1), Tile.Sprites[i].Sprite, typeof(Sprite), false, null); + Tile.Sprites[i].Weight = EditorGUILayout.IntField("Weight " + (i + 1), Tile.Sprites[i].Weight); + } + + EditorGUILayout.Space(); + + EditorGUILayout.PropertyField(m_Color); + EditorGUILayout.PropertyField(m_ColliderType); + + if (EditorGUI.EndChangeCheck()) + { + EditorUtility.SetDirty(Tile); + serializedObject.ApplyModifiedProperties(); + } + } + } +#endif +} diff --git a/MrBigsock/Packages/com.unity.2d.tilemap.extras/Runtime/Tiles/WeightedRandomTile/WeightedRandomTile.cs.meta b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Runtime/Tiles/WeightedRandomTile/WeightedRandomTile.cs.meta new file mode 100644 index 0000000000000000000000000000000000000000..6fa092a9345d39be4a8774ba9e9bbb5f4f860906 --- /dev/null +++ b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Runtime/Tiles/WeightedRandomTile/WeightedRandomTile.cs.meta @@ -0,0 +1,11 @@ +fileFormatVersion: 2 +guid: 52f942ca9511f354a8efbf325740f4ab +MonoImporter: + externalObjects: {} + serializedVersion: 2 + defaultReferences: [] + executionOrder: 0 + icon: {instanceID: 0} + userData: + assetBundleName: + assetBundleVariant: diff --git a/MrBigsock/Packages/com.unity.2d.tilemap.extras/Runtime/Unity.2D.Tilemap.Extras.asmdef b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Runtime/Unity.2D.Tilemap.Extras.asmdef new file mode 100644 index 0000000000000000000000000000000000000000..3eff8e194ec7be4836793f904262e0ccc77887a3 --- /dev/null +++ b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Runtime/Unity.2D.Tilemap.Extras.asmdef @@ -0,0 +1,3 @@ +{ + "name": "Unity.2D.Tilemap.Extras" +} diff --git a/MrBigsock/Packages/com.unity.2d.tilemap.extras/Runtime/Unity.2D.Tilemap.Extras.asmdef.meta b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Runtime/Unity.2D.Tilemap.Extras.asmdef.meta new file mode 100644 index 0000000000000000000000000000000000000000..c6b2bd9e96d43a35cef3b7d7ff75d15e312dce72 --- /dev/null +++ b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Runtime/Unity.2D.Tilemap.Extras.asmdef.meta @@ -0,0 +1,7 @@ +fileFormatVersion: 2 +guid: 613783dc1674e844b87788ea74ede0f6 +AssemblyDefinitionImporter: + externalObjects: {} + userData: + assetBundleName: + assetBundleVariant: diff --git a/MrBigsock/Packages/com.unity.2d.tilemap.extras/Samples~/DungeonRuleTile/.sample.json b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Samples~/DungeonRuleTile/.sample.json new file mode 100644 index 0000000000000000000000000000000000000000..12da29743e5a9fbd0b91aa82896bb05f74566ec1 --- /dev/null +++ b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Samples~/DungeonRuleTile/.sample.json @@ -0,0 +1,5 @@ +{ + "displayName": "Dungeon Rule Tile", + "interactiveImport": "false", + "description": "An example implementation of a Rule Tile with rules matching eight-way orthogonal and diagonal neighbors.", +} \ No newline at end of file diff --git a/MrBigsock/Packages/com.unity.2d.tilemap.extras/Samples~/DungeonRuleTile/Sprites/Dungeon.png b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Samples~/DungeonRuleTile/Sprites/Dungeon.png new file mode 100644 index 0000000000000000000000000000000000000000..dc498102953321bac9ceea148fa1d42c9b4c2784 Binary files /dev/null and b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Samples~/DungeonRuleTile/Sprites/Dungeon.png differ diff --git a/MrBigsock/Packages/com.unity.2d.tilemap.extras/Samples~/DungeonRuleTile/Sprites/Dungeon.png.meta b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Samples~/DungeonRuleTile/Sprites/Dungeon.png.meta new file mode 100644 index 0000000000000000000000000000000000000000..46db90db882dbfe9ccaec9946827bc03bd3cd4e6 --- /dev/null +++ b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Samples~/DungeonRuleTile/Sprites/Dungeon.png.meta @@ -0,0 +1,526 @@ +fileFormatVersion: 2 +guid: 4e794009527fa49a7a0e11880aaa3a50 +TextureImporter: + internalIDToNameTable: + - first: + 213: 8074338581418846440 + second: Dungeon_00 + - first: + 213: 4519664136019108208 + second: Dungeon_01 + - first: + 213: -6337999885091750218 + second: Dungeon_02 + - first: + 213: -5779282676337348671 + second: Dungeon_03 + - first: + 213: -1238933728272178944 + second: Dungeon_04 + - first: + 213: -4637172546458061636 + second: Dungeon_05 + - first: + 213: 1404196382344981941 + second: Dungeon_06 + - first: + 213: -6537453368205885854 + second: Dungeon_07 + - first: + 213: -3932762474534642510 + second: Dungeon_08 + - first: + 213: -38647862416911262 + second: Dungeon_09 + - first: + 213: 9158922217335370285 + second: Dungeon_10 + - first: + 213: -2913927307954446974 + second: Dungeon_11 + - first: + 213: -8314697479797604436 + second: Dungeon_12 + - first: + 213: -8560909203809222747 + second: Dungeon_13 + - first: + 213: -2860982009417361707 + second: Dungeon_14 + - first: + 213: 833101472512629755 + second: Dungeon_15 + externalObjects: {} + serializedVersion: 11 + mipmaps: + mipMapMode: 0 + enableMipMap: 0 + sRGBTexture: 1 + linearTexture: 0 + fadeOut: 0 + borderMipMap: 0 + mipMapsPreserveCoverage: 0 + alphaTestReferenceValue: 0.5 + mipMapFadeDistanceStart: 1 + mipMapFadeDistanceEnd: 3 + bumpmap: + convertToNormalMap: 0 + externalNormalMap: 0 + heightScale: 0.25 + normalMapFilter: 0 + isReadable: 0 + streamingMipmaps: 0 + streamingMipmapsPriority: 0 + vTOnly: 0 + grayScaleToAlpha: 0 + generateCubemap: 6 + cubemapConvolution: 0 + seamlessCubemap: 0 + textureFormat: 1 + maxTextureSize: 2048 + textureSettings: + serializedVersion: 2 + filterMode: -1 + aniso: -1 + mipBias: -100 + wrapU: 1 + wrapV: 1 + wrapW: -1 + nPOTScale: 0 + lightmap: 0 + compressionQuality: 50 + spriteMode: 2 + spriteExtrude: 1 + spriteMeshType: 1 + alignment: 0 + spritePivot: {x: 0.5, y: 0.5} + spritePixelsToUnits: 128 + spriteBorder: {x: 0, y: 0, z: 0, w: 0} + spriteGenerateFallbackPhysicsShape: 1 + alphaUsage: 1 + alphaIsTransparency: 1 + spriteTessellationDetail: -1 + textureType: 8 + textureShape: 1 + singleChannelComponent: 0 + maxTextureSizeSet: 0 + compressionQualitySet: 0 + textureFormatSet: 0 + ignorePngGamma: 0 + applyGammaDecoding: 0 + platformSettings: + - serializedVersion: 3 + buildTarget: DefaultTexturePlatform + maxTextureSize: 2048 + resizeAlgorithm: 0 + textureFormat: -1 + textureCompression: 2 + compressionQuality: 50 + crunchedCompression: 0 + allowsAlphaSplitting: 0 + overridden: 0 + androidETC2FallbackOverride: 0 + forceMaximumCompressionQuality_BC6H_BC7: 0 + - serializedVersion: 3 + buildTarget: Standalone + maxTextureSize: 2048 + resizeAlgorithm: 0 + textureFormat: -1 + textureCompression: 2 + compressionQuality: 50 + crunchedCompression: 0 + allowsAlphaSplitting: 0 + overridden: 0 + androidETC2FallbackOverride: 0 + forceMaximumCompressionQuality_BC6H_BC7: 0 + - serializedVersion: 3 + buildTarget: iPhone + maxTextureSize: 2048 + resizeAlgorithm: 0 + textureFormat: -1 + textureCompression: 2 + compressionQuality: 50 + crunchedCompression: 0 + allowsAlphaSplitting: 0 + overridden: 0 + androidETC2FallbackOverride: 0 + forceMaximumCompressionQuality_BC6H_BC7: 0 + - serializedVersion: 3 + buildTarget: Android + maxTextureSize: 2048 + resizeAlgorithm: 0 + textureFormat: -1 + textureCompression: 2 + compressionQuality: 50 + crunchedCompression: 0 + allowsAlphaSplitting: 0 + overridden: 0 + androidETC2FallbackOverride: 0 + forceMaximumCompressionQuality_BC6H_BC7: 0 + - serializedVersion: 3 + buildTarget: WebGL + maxTextureSize: 2048 + resizeAlgorithm: 0 + textureFormat: -1 + textureCompression: 2 + compressionQuality: 50 + crunchedCompression: 0 + allowsAlphaSplitting: 0 + overridden: 0 + androidETC2FallbackOverride: 0 + forceMaximumCompressionQuality_BC6H_BC7: 0 + spriteSheet: + serializedVersion: 2 + sprites: + - serializedVersion: 2 + name: Dungeon_00 + rect: + serializedVersion: 2 + x: 8 + y: 440 + width: 128 + height: 128 + alignment: 0 + pivot: {x: 0, y: 0} + border: {x: 0, y: 0, z: 0, w: 0} + outline: [] + physicsShape: [] + tessellationDetail: 0 + bones: [] + spriteID: 8e8a3bdf820dd0070800000000000000 + internalID: 8074338581418846440 + vertices: [] + indices: + edges: [] + weights: [] + - serializedVersion: 2 + name: Dungeon_01 + rect: + serializedVersion: 2 + x: 152 + y: 440 + width: 128 + height: 128 + alignment: 0 + pivot: {x: 0, y: 0} + border: {x: 0, y: 0, z: 0, w: 0} + outline: [] + physicsShape: [] + tessellationDetail: 0 + bones: [] + spriteID: 071ed73e59219be30800000000000000 + internalID: 4519664136019108208 + vertices: [] + indices: + edges: [] + weights: [] + - serializedVersion: 2 + name: Dungeon_02 + rect: + serializedVersion: 2 + x: 296 + y: 440 + width: 128 + height: 128 + alignment: 0 + pivot: {x: 0, y: 0} + border: {x: 0, y: 0, z: 0, w: 0} + outline: [] + physicsShape: [] + tessellationDetail: 0 + bones: [] + spriteID: 6beb3a64fa6ea08a0800000000000000 + internalID: -6337999885091750218 + vertices: [] + indices: + edges: [] + weights: [] + - serializedVersion: 2 + name: Dungeon_03 + rect: + serializedVersion: 2 + x: 440 + y: 440 + width: 128 + height: 128 + alignment: 0 + pivot: {x: 0, y: 0} + border: {x: 0, y: 0, z: 0, w: 0} + outline: [] + physicsShape: [] + tessellationDetail: 0 + bones: [] + spriteID: 1cfe75c960ddbcfa0800000000000000 + internalID: -5779282676337348671 + vertices: [] + indices: + edges: [] + weights: [] + - serializedVersion: 2 + name: Dungeon_04 + rect: + serializedVersion: 2 + x: 8 + y: 296 + width: 128 + height: 128 + alignment: 0 + pivot: {x: 0, y: 0} + border: {x: 0, y: 0, z: 0, w: 0} + outline: [] + physicsShape: [] + tessellationDetail: 0 + bones: [] + spriteID: 001261a965c6ecee0800000000000000 + internalID: -1238933728272178944 + vertices: [] + indices: + edges: [] + weights: [] + - serializedVersion: 2 + name: Dungeon_05 + rect: + serializedVersion: 2 + x: 152 + y: 296 + width: 128 + height: 128 + alignment: 0 + pivot: {x: 0, y: 0} + border: {x: 0, y: 0, z: 0, w: 0} + outline: [] + physicsShape: [] + tessellationDetail: 0 + bones: [] + spriteID: cb47c26432475afb0800000000000000 + internalID: -4637172546458061636 + vertices: [] + indices: + edges: [] + weights: [] + - serializedVersion: 2 + name: Dungeon_06 + rect: + serializedVersion: 2 + x: 296 + y: 296 + width: 128 + height: 128 + alignment: 0 + pivot: {x: 0, y: 0} + border: {x: 0, y: 0, z: 0, w: 0} + outline: [] + physicsShape: [] + tessellationDetail: 0 + bones: [] + spriteID: 5bdaf848b25bc7310800000000000000 + internalID: 1404196382344981941 + vertices: [] + indices: + edges: [] + weights: [] + - serializedVersion: 2 + name: Dungeon_07 + rect: + serializedVersion: 2 + x: 440 + y: 296 + width: 128 + height: 128 + alignment: 0 + pivot: {x: 0, y: 0} + border: {x: 0, y: 0, z: 0, w: 0} + outline: [] + physicsShape: [] + tessellationDetail: 0 + bones: [] + spriteID: 26e386c6ccc4645a0800000000000000 + internalID: -6537453368205885854 + vertices: [] + indices: + edges: [] + weights: [] + - serializedVersion: 2 + name: Dungeon_08 + rect: + serializedVersion: 2 + x: 8 + y: 152 + width: 128 + height: 128 + alignment: 0 + pivot: {x: 0, y: 0} + border: {x: 0, y: 0, z: 0, w: 0} + outline: [] + physicsShape: [] + tessellationDetail: 0 + bones: [] + spriteID: 2bc5067bd550c69c0800000000000000 + internalID: -3932762474534642510 + vertices: [] + indices: + edges: [] + weights: [] + - serializedVersion: 2 + name: Dungeon_09 + rect: + serializedVersion: 2 + x: 152 + y: 152 + width: 128 + height: 128 + alignment: 0 + pivot: {x: 0, y: 0} + border: {x: 0, y: 0, z: 0, w: 0} + outline: [] + physicsShape: [] + tessellationDetail: 0 + bones: [] + spriteID: 264df4159f1b67ff0800000000000000 + internalID: -38647862416911262 + vertices: [] + indices: + edges: [] + weights: [] + - serializedVersion: 2 + name: Dungeon_10 + rect: + serializedVersion: 2 + x: 296 + y: 152 + width: 128 + height: 128 + alignment: 0 + pivot: {x: 0, y: 0} + border: {x: 0, y: 0, z: 0, w: 0} + outline: [] + physicsShape: [] + tessellationDetail: 0 + bones: [] + spriteID: d2235b90b370b1f70800000000000000 + internalID: 9158922217335370285 + vertices: [] + indices: + edges: [] + weights: [] + - serializedVersion: 2 + name: Dungeon_11 + rect: + serializedVersion: 2 + x: 440 + y: 152 + width: 128 + height: 128 + alignment: 0 + pivot: {x: 0, y: 0} + border: {x: 0, y: 0, z: 0, w: 0} + outline: [] + physicsShape: [] + tessellationDetail: 0 + bones: [] + spriteID: 28d90145d86af87d0800000000000000 + internalID: -2913927307954446974 + vertices: [] + indices: + edges: [] + weights: [] + - serializedVersion: 2 + name: Dungeon_12 + rect: + serializedVersion: 2 + x: 8 + y: 8 + width: 128 + height: 128 + alignment: 0 + pivot: {x: 0, y: 0} + border: {x: 0, y: 0, z: 0, w: 0} + outline: [] + physicsShape: [] + tessellationDetail: 0 + bones: [] + spriteID: caff3ddf1b24c9c80800000000000000 + internalID: -8314697479797604436 + vertices: [] + indices: + edges: [] + weights: [] + - serializedVersion: 2 + name: Dungeon_13 + rect: + serializedVersion: 2 + x: 152 + y: 8 + width: 128 + height: 128 + alignment: 0 + pivot: {x: 0, y: 0} + border: {x: 0, y: 0, z: 0, w: 0} + outline: [] + physicsShape: [] + tessellationDetail: 0 + bones: [] + spriteID: 5af3490df6a813980800000000000000 + internalID: -8560909203809222747 + vertices: [] + indices: + edges: [] + weights: [] + - serializedVersion: 2 + name: Dungeon_14 + rect: + serializedVersion: 2 + x: 296 + y: 8 + width: 128 + height: 128 + alignment: 0 + pivot: {x: 0, y: 0} + border: {x: 0, y: 0, z: 0, w: 0} + outline: [] + physicsShape: [] + tessellationDetail: 0 + bones: [] + spriteID: 5d615e34500cb48d0800000000000000 + internalID: -2860982009417361707 + vertices: [] + indices: + edges: [] + weights: [] + - serializedVersion: 2 + name: Dungeon_15 + rect: + serializedVersion: 2 + x: 440 + y: 8 + width: 128 + height: 128 + alignment: 0 + pivot: {x: 0, y: 0} + border: {x: 0, y: 0, z: 0, w: 0} + outline: [] + physicsShape: [] + tessellationDetail: 0 + bones: [] + spriteID: bfb58f21065cf8b00800000000000000 + internalID: 833101472512629755 + vertices: [] + indices: + edges: [] + weights: [] + outline: [] + physicsShape: [] + bones: [] + spriteID: 5e97eb03825dee720800000000000000 + internalID: 0 + vertices: [] + indices: + edges: [] + weights: [] + secondaryTextures: [] + spritePackingTag: + pSDRemoveMatte: 0 + pSDShowRemoveMatteOption: 1 + userData: + assetBundleName: + assetBundleVariant: diff --git a/MrBigsock/Packages/com.unity.2d.tilemap.extras/Samples~/DungeonRuleTile/Tiles/Dungeon.asset b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Samples~/DungeonRuleTile/Tiles/Dungeon.asset new file mode 100644 index 0000000000000000000000000000000000000000..d74d729994d06a0049835801cb2d2527c44c159c --- /dev/null +++ b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Samples~/DungeonRuleTile/Tiles/Dungeon.asset @@ -0,0 +1,246 @@ +%YAML 1.1 +%TAG !u! tag:unity3d.com,2011: +--- !u!114 &11400000 +MonoBehaviour: + m_ObjectHideFlags: 0 + m_CorrespondingSourceObject: {fileID: 0} + m_PrefabInstance: {fileID: 0} + m_PrefabAsset: {fileID: 0} + m_GameObject: {fileID: 0} + m_Enabled: 1 + m_EditorHideFlags: 0 + m_Script: {fileID: 11500000, guid: 9d1514134bc4fbd41bb739b1b9a49231, type: 3} + m_Name: Dungeon + m_EditorClassIdentifier: + m_DefaultSprite: {fileID: -2860982009417361707, guid: 4e794009527fa49a7a0e11880aaa3a50, type: 3} + m_DefaultGameObject: {fileID: 0} + m_DefaultColliderType: 1 + m_TilingRules: + - m_Id: 0 + m_Sprites: + - {fileID: 8074338581418846440, guid: 4e794009527fa49a7a0e11880aaa3a50, type: 3} + m_GameObject: {fileID: 0} + m_AnimationSpeed: 1 + m_PerlinScale: 0.915 + m_Output: 0 + m_ColliderType: 1 + m_RandomTransform: 0 + m_Neighbors: 0100000001000000010000000100000001000000010000000100000001000000 + m_NeighborPositions: + - {x: -1, y: 1, z: 0} + - {x: 0, y: 1, z: 0} + - {x: 1, y: 1, z: 0} + - {x: -1, y: 0, z: 0} + - {x: 1, y: 0, z: 0} + - {x: -1, y: -1, z: 0} + - {x: 0, y: -1, z: 0} + - {x: 1, y: -1, z: 0} + m_RuleTransform: 0 + - m_Id: 13 + m_Sprites: + - {fileID: 4519664136019108208, guid: 4e794009527fa49a7a0e11880aaa3a50, type: 3} + m_GameObject: {fileID: 0} + m_AnimationSpeed: 1 + m_PerlinScale: 0.5 + m_Output: 0 + m_ColliderType: 1 + m_RandomTransform: 0 + m_Neighbors: 01000000010000000100000001000000010000000100000001000000 + m_NeighborPositions: + - {x: 0, y: 1, z: 0} + - {x: 1, y: 1, z: 0} + - {x: -1, y: 0, z: 0} + - {x: 1, y: 0, z: 0} + - {x: -1, y: -1, z: 0} + - {x: 0, y: -1, z: 0} + - {x: 1, y: -1, z: 0} + m_RuleTransform: 1 + - m_Id: 11 + m_Sprites: + - {fileID: -6337999885091750218, guid: 4e794009527fa49a7a0e11880aaa3a50, type: 3} + m_GameObject: {fileID: 0} + m_AnimationSpeed: 1 + m_PerlinScale: 0.5 + m_Output: 0 + m_ColliderType: 1 + m_RandomTransform: 0 + m_Neighbors: 010000000100000001000000010000000100000001000000 + m_NeighborPositions: + - {x: 0, y: 1, z: 0} + - {x: -1, y: 0, z: 0} + - {x: 1, y: 0, z: 0} + - {x: 0, y: -1, z: 0} + - {x: -1, y: -1, z: 0} + - {x: 1, y: -1, z: 0} + m_RuleTransform: 1 + - m_Id: 12 + m_Sprites: + - {fileID: -5779282676337348671, guid: 4e794009527fa49a7a0e11880aaa3a50, type: 3} + m_GameObject: {fileID: 0} + m_AnimationSpeed: 1 + m_PerlinScale: 0.5 + m_Output: 0 + m_ColliderType: 1 + m_RandomTransform: 0 + m_Neighbors: 010000000100000001000000010000000100000001000000 + m_NeighborPositions: + - {x: 0, y: 1, z: 0} + - {x: 1, y: 1, z: 0} + - {x: -1, y: 0, z: 0} + - {x: 1, y: 0, z: 0} + - {x: -1, y: -1, z: 0} + - {x: 0, y: -1, z: 0} + m_RuleTransform: 1 + - m_Id: 10 + m_Sprites: + - {fileID: -1238933728272178944, guid: 4e794009527fa49a7a0e11880aaa3a50, type: 3} + m_GameObject: {fileID: 0} + m_AnimationSpeed: 1 + m_PerlinScale: 0.5 + m_Output: 0 + m_ColliderType: 1 + m_RandomTransform: 0 + m_Neighbors: 0100000001000000010000000100000001000000 + m_NeighborPositions: + - {x: 0, y: 1, z: 0} + - {x: -1, y: 0, z: 0} + - {x: 1, y: 0, z: 0} + - {x: 0, y: -1, z: 0} + - {x: -1, y: -1, z: 0} + m_RuleTransform: 1 + - m_Id: 9 + m_Sprites: + - {fileID: -4637172546458061636, guid: 4e794009527fa49a7a0e11880aaa3a50, type: 3} + m_GameObject: {fileID: 0} + m_AnimationSpeed: 1 + m_PerlinScale: 0.5 + m_Output: 0 + m_ColliderType: 1 + m_RandomTransform: 0 + m_Neighbors: 01000000010000000100000001000000 + m_NeighborPositions: + - {x: 0, y: 1, z: 0} + - {x: -1, y: 0, z: 0} + - {x: 1, y: 0, z: 0} + - {x: 0, y: -1, z: 0} + m_RuleTransform: 0 + - m_Id: 8 + m_Sprites: + - {fileID: 1404196382344981941, guid: 4e794009527fa49a7a0e11880aaa3a50, type: 3} + m_GameObject: {fileID: 0} + m_AnimationSpeed: 1 + m_PerlinScale: 0.5 + m_Output: 0 + m_ColliderType: 1 + m_RandomTransform: 0 + m_Neighbors: 0100000001000000010000000100000001000000 + m_NeighborPositions: + - {x: 1, y: 0, z: 0} + - {x: -1, y: 0, z: 0} + - {x: 1, y: -1, z: 0} + - {x: 0, y: -1, z: 0} + - {x: -1, y: -1, z: 0} + m_RuleTransform: 1 + - m_Id: 7 + m_Sprites: + - {fileID: -6537453368205885854, guid: 4e794009527fa49a7a0e11880aaa3a50, type: 3} + m_GameObject: {fileID: 0} + m_AnimationSpeed: 1 + m_PerlinScale: 0.5 + m_Output: 0 + m_ColliderType: 1 + m_RandomTransform: 0 + m_Neighbors: 01000000010000000100000001000000 + m_NeighborPositions: + - {x: 1, y: 0, z: 0} + - {x: -1, y: 0, z: 0} + - {x: 0, y: -1, z: 0} + - {x: -1, y: -1, z: 0} + m_RuleTransform: 1 + - m_Id: 6 + m_Sprites: + - {fileID: -3932762474534642510, guid: 4e794009527fa49a7a0e11880aaa3a50, type: 3} + m_GameObject: {fileID: 0} + m_AnimationSpeed: 1 + m_PerlinScale: 0.5 + m_Output: 0 + m_ColliderType: 1 + m_RandomTransform: 0 + m_Neighbors: 01000000010000000100000001000000 + m_NeighborPositions: + - {x: 1, y: 0, z: 0} + - {x: -1, y: 0, z: 0} + - {x: 1, y: -1, z: 0} + - {x: 0, y: -1, z: 0} + m_RuleTransform: 1 + - m_Id: 3 + m_Sprites: + - {fileID: -38647862416911262, guid: 4e794009527fa49a7a0e11880aaa3a50, type: 3} + m_GameObject: {fileID: 0} + m_AnimationSpeed: 1 + m_PerlinScale: 0.5 + m_Output: 0 + m_ColliderType: 1 + m_RandomTransform: 0 + m_Neighbors: 010000000100000001000000 + m_NeighborPositions: + - {x: -1, y: 0, z: 0} + - {x: 1, y: 0, z: 0} + - {x: 0, y: -1, z: 0} + m_RuleTransform: 1 + - m_Id: 5 + m_Sprites: + - {fileID: 9158922217335370285, guid: 4e794009527fa49a7a0e11880aaa3a50, type: 3} + m_GameObject: {fileID: 0} + m_AnimationSpeed: 1 + m_PerlinScale: 0.5 + m_Output: 0 + m_ColliderType: 1 + m_RandomTransform: 0 + m_Neighbors: 0100000001000000 + m_NeighborPositions: + - {x: 1, y: 0, z: 0} + - {x: -1, y: 0, z: 0} + m_RuleTransform: 1 + - m_Id: 4 + m_Sprites: + - {fileID: -2913927307954446974, guid: 4e794009527fa49a7a0e11880aaa3a50, type: 3} + m_GameObject: {fileID: 0} + m_AnimationSpeed: 1 + m_PerlinScale: 0.5 + m_Output: 0 + m_ColliderType: 1 + m_RandomTransform: 0 + m_Neighbors: 010000000100000001000000 + m_NeighborPositions: + - {x: 1, y: 0, z: 0} + - {x: 0, y: -1, z: 0} + - {x: 1, y: -1, z: 0} + m_RuleTransform: 1 + - m_Id: 2 + m_Sprites: + - {fileID: -8314697479797604436, guid: 4e794009527fa49a7a0e11880aaa3a50, type: 3} + m_GameObject: {fileID: 0} + m_AnimationSpeed: 1 + m_PerlinScale: 0.5 + m_Output: 0 + m_ColliderType: 1 + m_RandomTransform: 0 + m_Neighbors: 0100000001000000 + m_NeighborPositions: + - {x: 1, y: 0, z: 0} + - {x: 0, y: -1, z: 0} + m_RuleTransform: 1 + - m_Id: 1 + m_Sprites: + - {fileID: -8560909203809222747, guid: 4e794009527fa49a7a0e11880aaa3a50, type: 3} + m_GameObject: {fileID: 0} + m_AnimationSpeed: 1 + m_PerlinScale: 0.5 + m_Output: 0 + m_ColliderType: 1 + m_RandomTransform: 0 + m_Neighbors: 01000000 + m_NeighborPositions: + - {x: -1, y: 0, z: 0} + m_RuleTransform: 1 diff --git a/MrBigsock/Packages/com.unity.2d.tilemap.extras/Samples~/DungeonRuleTile/Tiles/Dungeon.asset.meta b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Samples~/DungeonRuleTile/Tiles/Dungeon.asset.meta new file mode 100644 index 0000000000000000000000000000000000000000..978ed8f25b74d055a8b44880bfb7388da37ddaa7 --- /dev/null +++ b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Samples~/DungeonRuleTile/Tiles/Dungeon.asset.meta @@ -0,0 +1,8 @@ +fileFormatVersion: 2 +guid: 08002d404144b40bf842342dda8fa3e4 +NativeFormatImporter: + externalObjects: {} + mainObjectFileID: 0 + userData: + assetBundleName: + assetBundleVariant: diff --git a/MrBigsock/Packages/com.unity.2d.tilemap.extras/Samples~/PipeRuleTile/.sample.json b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Samples~/PipeRuleTile/.sample.json new file mode 100644 index 0000000000000000000000000000000000000000..1a5601672dfe5c77d9e589f2cf513889930859a1 --- /dev/null +++ b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Samples~/PipeRuleTile/.sample.json @@ -0,0 +1,5 @@ +{ + "displayName": "Pipe Rule Tile", + "interactiveImport": "false", + "description": "An example implementation of a Rule Tile with rules matching four-way orthogonal neighbors.", +} \ No newline at end of file diff --git a/MrBigsock/Packages/com.unity.2d.tilemap.extras/Samples~/PipeRuleTile/Sprites/Pipes.png b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Samples~/PipeRuleTile/Sprites/Pipes.png new file mode 100644 index 0000000000000000000000000000000000000000..f6eb1da36ac4c9147ccf860b01994c2bdfb6e759 Binary files /dev/null and b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Samples~/PipeRuleTile/Sprites/Pipes.png differ diff --git a/MrBigsock/Packages/com.unity.2d.tilemap.extras/Samples~/PipeRuleTile/Sprites/Pipes.png.meta b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Samples~/PipeRuleTile/Sprites/Pipes.png.meta new file mode 100644 index 0000000000000000000000000000000000000000..ea1ddbbb1b6f5157fe67bb845449b6ed68211aa6 --- /dev/null +++ b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Samples~/PipeRuleTile/Sprites/Pipes.png.meta @@ -0,0 +1,286 @@ +fileFormatVersion: 2 +guid: 1ebc1aff258f04220b2ce4e1240442fa +TextureImporter: + internalIDToNameTable: + - first: + 213: -3350344413380223241 + second: Pipes_00 + - first: + 213: -982495737459262880 + second: Pipes_01 + - first: + 213: 6001099972171207910 + second: Pipes_02 + - first: + 213: 4376287821753702255 + second: Pipes_03 + - first: + 213: -8656142213013974842 + second: Pipes_04 + - first: + 213: 8455133356877028895 + second: Pipes_05 + externalObjects: {} + serializedVersion: 11 + mipmaps: + mipMapMode: 0 + enableMipMap: 0 + sRGBTexture: 1 + linearTexture: 0 + fadeOut: 0 + borderMipMap: 0 + mipMapsPreserveCoverage: 0 + alphaTestReferenceValue: 0.5 + mipMapFadeDistanceStart: 1 + mipMapFadeDistanceEnd: 3 + bumpmap: + convertToNormalMap: 0 + externalNormalMap: 0 + heightScale: 0.25 + normalMapFilter: 0 + isReadable: 0 + streamingMipmaps: 0 + streamingMipmapsPriority: 0 + vTOnly: 0 + grayScaleToAlpha: 0 + generateCubemap: 6 + cubemapConvolution: 0 + seamlessCubemap: 0 + textureFormat: 1 + maxTextureSize: 2048 + textureSettings: + serializedVersion: 2 + filterMode: -1 + aniso: -1 + mipBias: -100 + wrapU: 1 + wrapV: 1 + wrapW: -1 + nPOTScale: 0 + lightmap: 0 + compressionQuality: 50 + spriteMode: 2 + spriteExtrude: 1 + spriteMeshType: 1 + alignment: 0 + spritePivot: {x: 0.5, y: 0.5} + spritePixelsToUnits: 128 + spriteBorder: {x: 0, y: 0, z: 0, w: 0} + spriteGenerateFallbackPhysicsShape: 1 + alphaUsage: 1 + alphaIsTransparency: 1 + spriteTessellationDetail: -1 + textureType: 8 + textureShape: 1 + singleChannelComponent: 0 + maxTextureSizeSet: 0 + compressionQualitySet: 0 + textureFormatSet: 0 + ignorePngGamma: 0 + applyGammaDecoding: 0 + platformSettings: + - serializedVersion: 3 + buildTarget: DefaultTexturePlatform + maxTextureSize: 2048 + resizeAlgorithm: 0 + textureFormat: -1 + textureCompression: 2 + compressionQuality: 50 + crunchedCompression: 0 + allowsAlphaSplitting: 0 + overridden: 0 + androidETC2FallbackOverride: 0 + forceMaximumCompressionQuality_BC6H_BC7: 0 + - serializedVersion: 3 + buildTarget: Standalone + maxTextureSize: 2048 + resizeAlgorithm: 0 + textureFormat: -1 + textureCompression: 2 + compressionQuality: 50 + crunchedCompression: 0 + allowsAlphaSplitting: 0 + overridden: 0 + androidETC2FallbackOverride: 0 + forceMaximumCompressionQuality_BC6H_BC7: 0 + - serializedVersion: 3 + buildTarget: iPhone + maxTextureSize: 2048 + resizeAlgorithm: 0 + textureFormat: -1 + textureCompression: 2 + compressionQuality: 50 + crunchedCompression: 0 + allowsAlphaSplitting: 0 + overridden: 0 + androidETC2FallbackOverride: 0 + forceMaximumCompressionQuality_BC6H_BC7: 0 + - serializedVersion: 3 + buildTarget: Android + maxTextureSize: 2048 + resizeAlgorithm: 0 + textureFormat: -1 + textureCompression: 2 + compressionQuality: 50 + crunchedCompression: 0 + allowsAlphaSplitting: 0 + overridden: 0 + androidETC2FallbackOverride: 0 + forceMaximumCompressionQuality_BC6H_BC7: 0 + - serializedVersion: 3 + buildTarget: WebGL + maxTextureSize: 2048 + resizeAlgorithm: 0 + textureFormat: -1 + textureCompression: 2 + compressionQuality: 50 + crunchedCompression: 0 + allowsAlphaSplitting: 0 + overridden: 0 + androidETC2FallbackOverride: 0 + forceMaximumCompressionQuality_BC6H_BC7: 0 + spriteSheet: + serializedVersion: 2 + sprites: + - serializedVersion: 2 + name: Pipes_00 + rect: + serializedVersion: 2 + x: 8 + y: 152 + width: 128 + height: 128 + alignment: 0 + pivot: {x: 0.5, y: 0.5} + border: {x: 0, y: 0, z: 0, w: 0} + outline: [] + physicsShape: [] + tessellationDetail: 0 + bones: [] + spriteID: 7fed145c18f2181d0800000000000000 + internalID: -3350344413380223241 + vertices: [] + indices: + edges: [] + weights: [] + - serializedVersion: 2 + name: Pipes_01 + rect: + serializedVersion: 2 + x: 152 + y: 152 + width: 128 + height: 128 + alignment: 0 + pivot: {x: 0.5, y: 0.5} + border: {x: 0, y: 0, z: 0, w: 0} + outline: [] + physicsShape: [] + tessellationDetail: 0 + bones: [] + spriteID: 06ab76f05597d52f0800000000000000 + internalID: -982495737459262880 + vertices: [] + indices: + edges: [] + weights: [] + - serializedVersion: 2 + name: Pipes_02 + rect: + serializedVersion: 2 + x: 296 + y: 152 + width: 128 + height: 128 + alignment: 0 + pivot: {x: 0.5, y: 0.5} + border: {x: 0, y: 0, z: 0, w: 0} + outline: [] + physicsShape: [] + tessellationDetail: 0 + bones: [] + spriteID: 6e41fd621a0384350800000000000000 + internalID: 6001099972171207910 + vertices: [] + indices: + edges: [] + weights: [] + - serializedVersion: 2 + name: Pipes_03 + rect: + serializedVersion: 2 + x: 8 + y: 8 + width: 128 + height: 128 + alignment: 0 + pivot: {x: 0.5, y: 0.5} + border: {x: 0, y: 0, z: 0, w: 0} + outline: [] + physicsShape: [] + tessellationDetail: 0 + bones: [] + spriteID: f675f7a5692bbbc30800000000000000 + internalID: 4376287821753702255 + vertices: [] + indices: + edges: [] + weights: [] + - serializedVersion: 2 + name: Pipes_04 + rect: + serializedVersion: 2 + x: 152 + y: 8 + width: 128 + height: 128 + alignment: 0 + pivot: {x: 0.5, y: 0.5} + border: {x: 0, y: 0, z: 0, w: 0} + outline: [] + physicsShape: [] + tessellationDetail: 0 + bones: [] + spriteID: 6c0d90cf4843fd780800000000000000 + internalID: -8656142213013974842 + vertices: [] + indices: + edges: [] + weights: [] + - serializedVersion: 2 + name: Pipes_05 + rect: + serializedVersion: 2 + x: 296 + y: 8 + width: 128 + height: 128 + alignment: 0 + pivot: {x: 0.5, y: 0.5} + border: {x: 0, y: 0, z: 0, w: 0} + outline: [] + physicsShape: [] + tessellationDetail: 0 + bones: [] + spriteID: f1e1639adfaa65570800000000000000 + internalID: 8455133356877028895 + vertices: [] + indices: + edges: [] + weights: [] + outline: [] + physicsShape: [] + bones: [] + spriteID: a10240c83e0c94dbaa728f513a672cd2 + internalID: 0 + vertices: [] + indices: + edges: [] + weights: [] + secondaryTextures: [] + spritePackingTag: + pSDRemoveMatte: 0 + pSDShowRemoveMatteOption: 1 + userData: + assetBundleName: + assetBundleVariant: diff --git a/MrBigsock/Packages/com.unity.2d.tilemap.extras/Samples~/PipeRuleTile/Tiles/Pipes.asset b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Samples~/PipeRuleTile/Tiles/Pipes.asset new file mode 100644 index 0000000000000000000000000000000000000000..350141506538a44b522a090d76b340d7af82a125 --- /dev/null +++ b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Samples~/PipeRuleTile/Tiles/Pipes.asset @@ -0,0 +1,90 @@ +%YAML 1.1 +%TAG !u! tag:unity3d.com,2011: +--- !u!114 &11400000 +MonoBehaviour: + m_ObjectHideFlags: 0 + m_CorrespondingSourceObject: {fileID: 0} + m_PrefabInstance: {fileID: 0} + m_PrefabAsset: {fileID: 0} + m_GameObject: {fileID: 0} + m_Enabled: 1 + m_EditorHideFlags: 0 + m_Script: {fileID: 11500000, guid: 9d1514134bc4fbd41bb739b1b9a49231, type: 3} + m_Name: Pipes + m_EditorClassIdentifier: + m_DefaultSprite: {fileID: -3350344413380223241, guid: 1ebc1aff258f04220b2ce4e1240442fa, type: 3} + m_DefaultGameObject: {fileID: 0} + m_DefaultColliderType: 1 + m_TilingRules: + - m_Id: 4 + m_Sprites: + - {fileID: -982495737459262880, guid: 1ebc1aff258f04220b2ce4e1240442fa, type: 3} + m_GameObject: {fileID: 0} + m_AnimationSpeed: 1 + m_PerlinScale: 0.5 + m_Output: 0 + m_ColliderType: 1 + m_RandomTransform: 0 + m_Neighbors: 01000000010000000100000001000000 + m_NeighborPositions: + - {x: 0, y: 1, z: 0} + - {x: -1, y: 0, z: 0} + - {x: 0, y: -1, z: 0} + - {x: 1, y: 0, z: 0} + m_RuleTransform: 0 + - m_Id: 3 + m_Sprites: + - {fileID: 6001099972171207910, guid: 1ebc1aff258f04220b2ce4e1240442fa, type: 3} + m_GameObject: {fileID: 0} + m_AnimationSpeed: 1 + m_PerlinScale: 0.5 + m_Output: 0 + m_ColliderType: 1 + m_RandomTransform: 0 + m_Neighbors: 010000000100000001000000 + m_NeighborPositions: + - {x: 0, y: 1, z: 0} + - {x: -1, y: 0, z: 0} + - {x: 1, y: 0, z: 0} + m_RuleTransform: 1 + - m_Id: 1 + m_Sprites: + - {fileID: 4376287821753702255, guid: 1ebc1aff258f04220b2ce4e1240442fa, type: 3} + m_GameObject: {fileID: 0} + m_AnimationSpeed: 1 + m_PerlinScale: 0.5 + m_Output: 0 + m_ColliderType: 1 + m_RandomTransform: 0 + m_Neighbors: 0100000001000000 + m_NeighborPositions: + - {x: -1, y: 0, z: 0} + - {x: 1, y: 0, z: 0} + m_RuleTransform: 1 + - m_Id: 2 + m_Sprites: + - {fileID: -8656142213013974842, guid: 1ebc1aff258f04220b2ce4e1240442fa, type: 3} + m_GameObject: {fileID: 0} + m_AnimationSpeed: 1 + m_PerlinScale: 0.5 + m_Output: 0 + m_ColliderType: 1 + m_RandomTransform: 0 + m_Neighbors: 0100000001000000 + m_NeighborPositions: + - {x: -1, y: 0, z: 0} + - {x: 0, y: 1, z: 0} + m_RuleTransform: 1 + - m_Id: 0 + m_Sprites: + - {fileID: 8455133356877028895, guid: 1ebc1aff258f04220b2ce4e1240442fa, type: 3} + m_GameObject: {fileID: 0} + m_AnimationSpeed: 1 + m_PerlinScale: 0.5 + m_Output: 0 + m_ColliderType: 1 + m_RandomTransform: 0 + m_Neighbors: 01000000 + m_NeighborPositions: + - {x: -1, y: 0, z: 0} + m_RuleTransform: 1 diff --git a/MrBigsock/Packages/com.unity.2d.tilemap.extras/Samples~/PipeRuleTile/Tiles/Pipes.asset.meta b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Samples~/PipeRuleTile/Tiles/Pipes.asset.meta new file mode 100644 index 0000000000000000000000000000000000000000..113ca2686b1d2624af88e200bc9b70c8cfb4ed9e --- /dev/null +++ b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Samples~/PipeRuleTile/Tiles/Pipes.asset.meta @@ -0,0 +1,8 @@ +fileFormatVersion: 2 +guid: 3866e832eb1cd4493870232771332091 +NativeFormatImporter: + externalObjects: {} + mainObjectFileID: 0 + userData: + assetBundleName: + assetBundleVariant: diff --git a/MrBigsock/Packages/com.unity.2d.tilemap.extras/Samples~/WaterfallAnimatedTile/.sample.json b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Samples~/WaterfallAnimatedTile/.sample.json new file mode 100644 index 0000000000000000000000000000000000000000..9c09d6030ce2915dcda3ade421f7e7a68ee2a3ce --- /dev/null +++ b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Samples~/WaterfallAnimatedTile/.sample.json @@ -0,0 +1,5 @@ +{ + "displayName": "Waterfall Animated Tile", + "interactiveImport": "false", + "description": "An example implementation of an Animated Tile.", +} \ No newline at end of file diff --git a/MrBigsock/Packages/com.unity.2d.tilemap.extras/Samples~/WaterfallAnimatedTile/Sprites/Waterfall.png b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Samples~/WaterfallAnimatedTile/Sprites/Waterfall.png new file mode 100644 index 0000000000000000000000000000000000000000..8ff66124cf693446191197548fa491b7469be247 Binary files /dev/null and b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Samples~/WaterfallAnimatedTile/Sprites/Waterfall.png differ diff --git a/MrBigsock/Packages/com.unity.2d.tilemap.extras/Samples~/WaterfallAnimatedTile/Sprites/Waterfall.png.meta b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Samples~/WaterfallAnimatedTile/Sprites/Waterfall.png.meta new file mode 100644 index 0000000000000000000000000000000000000000..9e8d94315fdb686191d249fca95738e4ca134c03 --- /dev/null +++ b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Samples~/WaterfallAnimatedTile/Sprites/Waterfall.png.meta @@ -0,0 +1,472 @@ +fileFormatVersion: 2 +guid: ea3d66a7edd9d46d38e40be89ae8d3cf +TextureImporter: + internalIDToNameTable: + - first: + 213: 4721293536888397434 + second: Waterfall_0 + - first: + 213: 5364352939883245045 + second: Waterfall_1 + - first: + 213: 7196270143389622831 + second: Waterfall_2 + - first: + 213: 6029957480157117390 + second: Waterfall_3 + - first: + 213: -2507161886035769597 + second: Waterfall_4 + - first: + 213: 1725676371340164549 + second: Waterfall_5 + - first: + 213: 2872686455240326431 + second: Waterfall_6 + - first: + 213: 3786080894526622295 + second: Waterfall_7 + - first: + 213: 5045660051849922305 + second: Waterfall_8 + - first: + 213: 5612536237972652912 + second: Waterfall_9 + - first: + 213: -3250777559591057548 + second: Waterfall_10 + - first: + 213: 5957929059053060748 + second: Waterfall_11 + - first: + 213: 7109957084653153026 + second: Waterfall_12 + - first: + 213: 477525649501392199 + second: Waterfall_13 + - first: + 213: -8431399023861082550 + second: Waterfall_14 + - first: + 213: -5115951240437522786 + second: Waterfall_15 + - first: + 213: 447768722618067419 + second: Waterfall_16 + - first: + 213: 8730975675779852889 + second: Waterfall_17 + - first: + 213: -3300933181597420981 + second: Waterfall_18 + - first: + 213: 7541503168263187259 + second: Waterfall_19 + - first: + 213: -5806308423563604605 + second: Waterfall_20 + - first: + 213: -675073502400653780 + second: Waterfall_21 + - first: + 213: 8203429098523291671 + second: Waterfall_22 + - first: + 213: 219257364728720650 + second: Waterfall_23 + - first: + 213: 8458781440006651279 + second: Waterfall_24 + - first: + 213: 8715392851287823110 + second: Waterfall_25 + - first: + 213: 3020409149263879807 + second: Waterfall_26 + - first: + 213: -9102966082491327057 + second: Waterfall_27 + - first: + 213: 5664645608148975165 + second: Waterfall_28 + - first: + 213: -8527461141348136443 + second: Waterfall_29 + - first: + 213: -908826963640821454 + second: Waterfall_30 + - first: + 213: -5617403070256607301 + second: Waterfall_31 + - first: + 213: 5275563956605968481 + second: Waterfall_32 + - first: + 213: -8289717914693220806 + second: Waterfall_33 + - first: + 213: 4951532302442582524 + second: Waterfall_34 + - first: + 213: -3006427023634639824 + second: Waterfall_35 + - first: + 213: 7534001321458047645 + second: Waterfall_36 + - first: + 213: 5834154964668067101 + second: Waterfall_37 + - first: + 213: -3291032407325874115 + second: Waterfall_38 + - first: + 213: -6674891174622030782 + second: Waterfall_39 + externalObjects: {} + serializedVersion: 11 + mipmaps: + mipMapMode: 0 + enableMipMap: 0 + sRGBTexture: 1 + linearTexture: 0 + fadeOut: 0 + borderMipMap: 0 + mipMapsPreserveCoverage: 0 + alphaTestReferenceValue: 0.5 + mipMapFadeDistanceStart: 1 + mipMapFadeDistanceEnd: 3 + bumpmap: + convertToNormalMap: 0 + externalNormalMap: 0 + heightScale: 0.25 + normalMapFilter: 0 + isReadable: 0 + streamingMipmaps: 0 + streamingMipmapsPriority: 0 + vTOnly: 0 + grayScaleToAlpha: 0 + generateCubemap: 6 + cubemapConvolution: 0 + seamlessCubemap: 0 + textureFormat: 1 + maxTextureSize: 2048 + textureSettings: + serializedVersion: 2 + filterMode: -1 + aniso: -1 + mipBias: -100 + wrapU: 1 + wrapV: 1 + wrapW: -1 + nPOTScale: 0 + lightmap: 0 + compressionQuality: 50 + spriteMode: 2 + spriteExtrude: 1 + spriteMeshType: 1 + alignment: 0 + spritePivot: {x: 0.5, y: 0.5} + spritePixelsToUnits: 62 + spriteBorder: {x: 0, y: 0, z: 0, w: 0} + spriteGenerateFallbackPhysicsShape: 1 + alphaUsage: 1 + alphaIsTransparency: 1 + spriteTessellationDetail: -1 + textureType: 8 + textureShape: 1 + singleChannelComponent: 0 + maxTextureSizeSet: 0 + compressionQualitySet: 0 + textureFormatSet: 0 + ignorePngGamma: 0 + applyGammaDecoding: 0 + platformSettings: + - serializedVersion: 3 + buildTarget: DefaultTexturePlatform + maxTextureSize: 2048 + resizeAlgorithm: 0 + textureFormat: -1 + textureCompression: 1 + compressionQuality: 50 + crunchedCompression: 0 + allowsAlphaSplitting: 0 + overridden: 0 + androidETC2FallbackOverride: 0 + forceMaximumCompressionQuality_BC6H_BC7: 0 + - serializedVersion: 3 + buildTarget: Standalone + maxTextureSize: 2048 + resizeAlgorithm: 0 + textureFormat: -1 + textureCompression: 1 + compressionQuality: 50 + crunchedCompression: 0 + allowsAlphaSplitting: 0 + overridden: 0 + androidETC2FallbackOverride: 0 + forceMaximumCompressionQuality_BC6H_BC7: 0 + - serializedVersion: 3 + buildTarget: iPhone + maxTextureSize: 2048 + resizeAlgorithm: 0 + textureFormat: -1 + textureCompression: 1 + compressionQuality: 50 + crunchedCompression: 0 + allowsAlphaSplitting: 0 + overridden: 0 + androidETC2FallbackOverride: 0 + forceMaximumCompressionQuality_BC6H_BC7: 0 + - serializedVersion: 3 + buildTarget: Android + maxTextureSize: 2048 + resizeAlgorithm: 0 + textureFormat: -1 + textureCompression: 1 + compressionQuality: 50 + crunchedCompression: 0 + allowsAlphaSplitting: 0 + overridden: 0 + androidETC2FallbackOverride: 0 + forceMaximumCompressionQuality_BC6H_BC7: 0 + - serializedVersion: 3 + buildTarget: WebGL + maxTextureSize: 2048 + resizeAlgorithm: 0 + textureFormat: -1 + textureCompression: 1 + compressionQuality: 50 + crunchedCompression: 0 + allowsAlphaSplitting: 0 + overridden: 0 + androidETC2FallbackOverride: 0 + forceMaximumCompressionQuality_BC6H_BC7: 0 + spriteSheet: + serializedVersion: 2 + sprites: + - serializedVersion: 2 + name: Waterfall_0 + rect: + serializedVersion: 2 + x: 4 + y: 72 + width: 64 + height: 64 + alignment: 0 + pivot: {x: 0, y: 0} + border: {x: 0, y: 0, z: 0, w: 0} + outline: [] + physicsShape: [] + tessellationDetail: 0 + bones: [] + spriteID: a7e94921577658140800000000000000 + internalID: 4721293536888397434 + vertices: [] + indices: + edges: [] + weights: [] + - serializedVersion: 2 + name: Waterfall_1 + rect: + serializedVersion: 2 + x: 72 + y: 72 + width: 64 + height: 64 + alignment: 0 + pivot: {x: 0, y: 0} + border: {x: 0, y: 0, z: 0, w: 0} + outline: [] + physicsShape: [] + tessellationDetail: 0 + bones: [] + spriteID: 5fd2097c392027a40800000000000000 + internalID: 5364352939883245045 + vertices: [] + indices: + edges: [] + weights: [] + - serializedVersion: 2 + name: Waterfall_2 + rect: + serializedVersion: 2 + x: 140 + y: 72 + width: 64 + height: 64 + alignment: 0 + pivot: {x: 0, y: 0} + border: {x: 0, y: 0, z: 0, w: 0} + outline: [] + physicsShape: [] + tessellationDetail: 0 + bones: [] + spriteID: f22ec7461994ed360800000000000000 + internalID: 7196270143389622831 + vertices: [] + indices: + edges: [] + weights: [] + - serializedVersion: 2 + name: Waterfall_3 + rect: + serializedVersion: 2 + x: 208 + y: 72 + width: 64 + height: 64 + alignment: 0 + pivot: {x: 0, y: 0} + border: {x: 0, y: 0, z: 0, w: 0} + outline: [] + physicsShape: [] + tessellationDetail: 0 + bones: [] + spriteID: ecb797f4166bea350800000000000000 + internalID: 6029957480157117390 + vertices: [] + indices: + edges: [] + weights: [] + - serializedVersion: 2 + name: Waterfall_4 + rect: + serializedVersion: 2 + x: 276 + y: 72 + width: 64 + height: 64 + alignment: 0 + pivot: {x: 0, y: 0} + border: {x: 0, y: 0, z: 0, w: 0} + outline: [] + physicsShape: [] + tessellationDetail: 0 + bones: [] + spriteID: 30780745c85c43dd0800000000000000 + internalID: -2507161886035769597 + vertices: [] + indices: + edges: [] + weights: [] + - serializedVersion: 2 + name: Waterfall_5 + rect: + serializedVersion: 2 + x: 4 + y: 4 + width: 64 + height: 64 + alignment: 0 + pivot: {x: 0, y: 0} + border: {x: 0, y: 0, z: 0, w: 0} + outline: [] + physicsShape: [] + tessellationDetail: 0 + bones: [] + spriteID: 5cdf3074485d2f710800000000000000 + internalID: 1725676371340164549 + vertices: [] + indices: + edges: [] + weights: [] + - serializedVersion: 2 + name: Waterfall_6 + rect: + serializedVersion: 2 + x: 72 + y: 4 + width: 64 + height: 64 + alignment: 0 + pivot: {x: 0, y: 0} + border: {x: 0, y: 0, z: 0, w: 0} + outline: [] + physicsShape: [] + tessellationDetail: 0 + bones: [] + spriteID: f1dfca36c15ddd720800000000000000 + internalID: 2872686455240326431 + vertices: [] + indices: + edges: [] + weights: [] + - serializedVersion: 2 + name: Waterfall_7 + rect: + serializedVersion: 2 + x: 140 + y: 4 + width: 64 + height: 64 + alignment: 0 + pivot: {x: 0, y: 0} + border: {x: 0, y: 0, z: 0, w: 0} + outline: [] + physicsShape: [] + tessellationDetail: 0 + bones: [] + spriteID: 75a0729938cda8430800000000000000 + internalID: 3786080894526622295 + vertices: [] + indices: + edges: [] + weights: [] + - serializedVersion: 2 + name: Waterfall_8 + rect: + serializedVersion: 2 + x: 208 + y: 4 + width: 64 + height: 64 + alignment: 0 + pivot: {x: 0, y: 0} + border: {x: 0, y: 0, z: 0, w: 0} + outline: [] + physicsShape: [] + tessellationDetail: 0 + bones: [] + spriteID: 107c4e78519c50640800000000000000 + internalID: 5045660051849922305 + vertices: [] + indices: + edges: [] + weights: [] + - serializedVersion: 2 + name: Waterfall_9 + rect: + serializedVersion: 2 + x: 276 + y: 4 + width: 64 + height: 64 + alignment: 0 + pivot: {x: 0, y: 0} + border: {x: 0, y: 0, z: 0, w: 0} + outline: [] + physicsShape: [] + tessellationDetail: 0 + bones: [] + spriteID: 0730e51d8fbb3ed40800000000000000 + internalID: 5612536237972652912 + vertices: [] + indices: + edges: [] + weights: [] + outline: [] + physicsShape: [] + bones: [] + spriteID: 5e97eb03825dee720800000000000000 + internalID: 0 + vertices: [] + indices: + edges: [] + weights: [] + secondaryTextures: [] + spritePackingTag: + pSDRemoveMatte: 0 + pSDShowRemoveMatteOption: 0 + userData: + assetBundleName: + assetBundleVariant: diff --git a/MrBigsock/Packages/com.unity.2d.tilemap.extras/Samples~/WaterfallAnimatedTile/Tiles/Waterfall.asset b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Samples~/WaterfallAnimatedTile/Tiles/Waterfall.asset new file mode 100644 index 0000000000000000000000000000000000000000..45e252ece6673ffb7f5c3a6c9320b98363735e16 --- /dev/null +++ b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Samples~/WaterfallAnimatedTile/Tiles/Waterfall.asset @@ -0,0 +1,25 @@ +%YAML 1.1 +%TAG !u! tag:unity3d.com,2011: +--- !u!114 &11400000 +MonoBehaviour: + m_ObjectHideFlags: 0 + m_CorrespondingSourceObject: {fileID: 0} + m_PrefabInstance: {fileID: 0} + m_PrefabAsset: {fileID: 0} + m_GameObject: {fileID: 0} + m_Enabled: 1 + m_EditorHideFlags: 0 + m_Script: {fileID: 11500000, guid: 13b75c95f34a00d4e8c04f76b73312e6, type: 3} + m_Name: Waterfall + m_EditorClassIdentifier: + m_AnimatedSprites: + - {fileID: 4721293536888397434, guid: ea3d66a7edd9d46d38e40be89ae8d3cf, type: 3} + - {fileID: 5364352939883245045, guid: ea3d66a7edd9d46d38e40be89ae8d3cf, type: 3} + - {fileID: 7196270143389622831, guid: ea3d66a7edd9d46d38e40be89ae8d3cf, type: 3} + - {fileID: 6029957480157117390, guid: ea3d66a7edd9d46d38e40be89ae8d3cf, type: 3} + - {fileID: -2507161886035769597, guid: ea3d66a7edd9d46d38e40be89ae8d3cf, type: 3} + m_MinSpeed: 1 + m_MaxSpeed: 1 + m_AnimationStartTime: 0 + m_AnimationStartFrame: 0 + m_TileColliderType: 0 diff --git a/MrBigsock/Packages/com.unity.2d.tilemap.extras/Samples~/WaterfallAnimatedTile/Tiles/Waterfall.asset.meta b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Samples~/WaterfallAnimatedTile/Tiles/Waterfall.asset.meta new file mode 100644 index 0000000000000000000000000000000000000000..1409f8fda38cca49239bd1b26e288ad39e63fd77 --- /dev/null +++ b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Samples~/WaterfallAnimatedTile/Tiles/Waterfall.asset.meta @@ -0,0 +1,8 @@ +fileFormatVersion: 2 +guid: 9eb6880a443e94253bbdc5b52fa3e1d3 +NativeFormatImporter: + externalObjects: {} + mainObjectFileID: 0 + userData: + assetBundleName: + assetBundleVariant: diff --git a/MrBigsock/Packages/com.unity.2d.tilemap.extras/Samples~/WaterfallAnimatedTile/Tiles/Waterfall_Bottom.asset b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Samples~/WaterfallAnimatedTile/Tiles/Waterfall_Bottom.asset new file mode 100644 index 0000000000000000000000000000000000000000..d475cdc4e5e917e40d049824be4c74b83388e14a --- /dev/null +++ b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Samples~/WaterfallAnimatedTile/Tiles/Waterfall_Bottom.asset @@ -0,0 +1,25 @@ +%YAML 1.1 +%TAG !u! tag:unity3d.com,2011: +--- !u!114 &11400000 +MonoBehaviour: + m_ObjectHideFlags: 0 + m_CorrespondingSourceObject: {fileID: 0} + m_PrefabInstance: {fileID: 0} + m_PrefabAsset: {fileID: 0} + m_GameObject: {fileID: 0} + m_Enabled: 1 + m_EditorHideFlags: 0 + m_Script: {fileID: 11500000, guid: 13b75c95f34a00d4e8c04f76b73312e6, type: 3} + m_Name: Waterfall_Bottom + m_EditorClassIdentifier: + m_AnimatedSprites: + - {fileID: 1725676371340164549, guid: ea3d66a7edd9d46d38e40be89ae8d3cf, type: 3} + - {fileID: 2872686455240326431, guid: ea3d66a7edd9d46d38e40be89ae8d3cf, type: 3} + - {fileID: 3786080894526622295, guid: ea3d66a7edd9d46d38e40be89ae8d3cf, type: 3} + - {fileID: 5045660051849922305, guid: ea3d66a7edd9d46d38e40be89ae8d3cf, type: 3} + - {fileID: 5612536237972652912, guid: ea3d66a7edd9d46d38e40be89ae8d3cf, type: 3} + m_MinSpeed: 1 + m_MaxSpeed: 1 + m_AnimationStartTime: 0 + m_AnimationStartFrame: 0 + m_TileColliderType: 0 diff --git a/MrBigsock/Packages/com.unity.2d.tilemap.extras/Samples~/WaterfallAnimatedTile/Tiles/Waterfall_Bottom.asset.meta b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Samples~/WaterfallAnimatedTile/Tiles/Waterfall_Bottom.asset.meta new file mode 100644 index 0000000000000000000000000000000000000000..e6ff766afe94e2d295853550218cf32f105e9517 --- /dev/null +++ b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Samples~/WaterfallAnimatedTile/Tiles/Waterfall_Bottom.asset.meta @@ -0,0 +1,8 @@ +fileFormatVersion: 2 +guid: 6cac02ba5b9814dbcb8988ddc06da6bb +NativeFormatImporter: + externalObjects: {} + mainObjectFileID: 0 + userData: + assetBundleName: + assetBundleVariant: diff --git a/MrBigsock/Packages/com.unity.2d.tilemap.extras/Tests.meta b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Tests.meta new file mode 100644 index 0000000000000000000000000000000000000000..351719576093c304983fc0915f2a8d45dd763619 --- /dev/null +++ b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Tests.meta @@ -0,0 +1,8 @@ +fileFormatVersion: 2 +guid: 7e501fe6c6911484c907a1a3b00692dd +folderAsset: yes +DefaultImporter: + externalObjects: {} + userData: + assetBundleName: + assetBundleVariant: diff --git a/MrBigsock/Packages/com.unity.2d.tilemap.extras/Tests/Editor.meta b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Tests/Editor.meta new file mode 100644 index 0000000000000000000000000000000000000000..cd7fe02c75f87bfb24df6bf5c05f75b94388ae7d --- /dev/null +++ b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Tests/Editor.meta @@ -0,0 +1,8 @@ +fileFormatVersion: 2 +guid: b679ec8b3957ea64ebd2626843d0a81e +folderAsset: yes +DefaultImporter: + externalObjects: {} + userData: + assetBundleName: + assetBundleVariant: diff --git a/MrBigsock/Packages/com.unity.2d.tilemap.extras/Tests/Editor/PlaceholderTests.cs b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Tests/Editor/PlaceholderTests.cs new file mode 100644 index 0000000000000000000000000000000000000000..8762ea2284f57eff7d4c4b5df6c73a869c0d2d6a --- /dev/null +++ b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Tests/Editor/PlaceholderTests.cs @@ -0,0 +1,10 @@ +using NUnit.Framework; + +internal class U2DExtrasPlaceholder +{ + [Test] + public void PlaceHolderTest() + { + Assert.Pass("2D Extras tests are in a separate package."); + } +} diff --git a/MrBigsock/Packages/com.unity.2d.tilemap.extras/Tests/Editor/PlaceholderTests.cs.meta b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Tests/Editor/PlaceholderTests.cs.meta new file mode 100644 index 0000000000000000000000000000000000000000..adddad8fb2fa1daff5c04bb0f67907e9bfdb9842 --- /dev/null +++ b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Tests/Editor/PlaceholderTests.cs.meta @@ -0,0 +1,11 @@ +fileFormatVersion: 2 +guid: 1570cfa747cfb0647a5afa98d8babd66 +MonoImporter: + externalObjects: {} + serializedVersion: 2 + defaultReferences: [] + executionOrder: 0 + icon: {instanceID: 0} + userData: + assetBundleName: + assetBundleVariant: diff --git a/MrBigsock/Packages/com.unity.2d.tilemap.extras/Tests/Editor/Unity.2D.Tilemap.Extras.EditorTests.asmdef b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Tests/Editor/Unity.2D.Tilemap.Extras.EditorTests.asmdef new file mode 100644 index 0000000000000000000000000000000000000000..2e5ebd78128dd077269860131edc54ff7161bb0b --- /dev/null +++ b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Tests/Editor/Unity.2D.Tilemap.Extras.EditorTests.asmdef @@ -0,0 +1,22 @@ +{ + "name": "Unity.2D.Tilemap.Extras.Placeholder.EditorTests", + "references": [ + "UnityEngine.TestRunner", + "UnityEditor.TestRunner" + ], + "includePlatforms": [ + "Editor" + ], + "excludePlatforms": [], + "allowUnsafeCode": false, + "overrideReferences": true, + "precompiledReferences": [ + "nunit.framework.dll" + ], + "autoReferenced": false, + "defineConstraints": [ + "UNITY_INCLUDE_TESTS" + ], + "versionDefines": [], + "noEngineReferences": false +} \ No newline at end of file diff --git a/MrBigsock/Packages/com.unity.2d.tilemap.extras/Tests/Editor/Unity.2D.Tilemap.Extras.EditorTests.asmdef.meta b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Tests/Editor/Unity.2D.Tilemap.Extras.EditorTests.asmdef.meta new file mode 100644 index 0000000000000000000000000000000000000000..c574c3a5473e8ab04f1ab3c7544fd00e1076eed7 --- /dev/null +++ b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Tests/Editor/Unity.2D.Tilemap.Extras.EditorTests.asmdef.meta @@ -0,0 +1,7 @@ +fileFormatVersion: 2 +guid: 6e1c163768a31b44ba01cc5392db861e +AssemblyDefinitionImporter: + externalObjects: {} + userData: + assetBundleName: + assetBundleVariant: diff --git a/MrBigsock/Packages/com.unity.2d.tilemap.extras/Third Party Notices.md b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Third Party Notices.md new file mode 100644 index 0000000000000000000000000000000000000000..40ded99ffcdbe9b2058a8a30b88c2b89e75a7707 --- /dev/null +++ b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Third Party Notices.md @@ -0,0 +1,21 @@ +This package contains third-party software components governed by the license(s) indicated below: +--------- + +Component Name: Line Brush +public static IEnumerable<Vector2Int> GetPointsOnLine(Vector2Int p1, Vector2Int p2) +http://ericw.ca/notes/bresenhams-line-algorithm-in-csharp.html + +License Type: MIT + +Copyright (c) 2020 Eric Woroshow + +Permission is hereby granted, free of charge, to any person obtaining a copy of this software and associated documentation files (the "Software"), to deal in the Software without restriction, including without limitation the rights to use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is furnished to do so, subject to the following conditions: + +The above copyright notice and this permission notice shall be included in all copies or substantial portions of the Software. + +THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. + +ericw.ca / Content © 2009-2020 Eric Woroshow. All rights reserved. +Code under MIT license unless otherwise noted. + +--------- diff --git a/MrBigsock/Packages/com.unity.2d.tilemap.extras/Third Party Notices.md.meta b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Third Party Notices.md.meta new file mode 100644 index 0000000000000000000000000000000000000000..8747dd0455ce85a1056e5ad1ffeccdeef58b93ce --- /dev/null +++ b/MrBigsock/Packages/com.unity.2d.tilemap.extras/Third Party Notices.md.meta @@ -0,0 +1,7 @@ +fileFormatVersion: 2 +guid: d131a2d0c5c33464b90253c59096277f +TextScriptImporter: + externalObjects: {} + userData: + assetBundleName: + assetBundleVariant: diff --git a/MrBigsock/Packages/com.unity.2d.tilemap.extras/package.json b/MrBigsock/Packages/com.unity.2d.tilemap.extras/package.json new file mode 100644 index 0000000000000000000000000000000000000000..153051607339e1c31a959aacc0c3b5676410cf00 --- /dev/null +++ b/MrBigsock/Packages/com.unity.2d.tilemap.extras/package.json @@ -0,0 +1,35 @@ +{ + "name": "com.unity.2d.tilemap.extras", + "displayName": "2D Tilemap Extras", + "version": "2.2.3", + "unity": "2021.1", + "description": "2D Tilemap Extras is a package that contains extra scripts for use with 2D Tilemap features in Unity. These include custom Tiles and Brushes for the Tilemap feature.\n\nThe following are included in the package:\nBrushes: GameObject Brush, Group Brush, Line Brush, Random Brush\nTiles: Animated Tile, Rule Tile, Rule Override Tile\nOther: Grid Information, Custom Rules for Rule Tile", + "keywords": ["2d"], + "category": "2D", + "dependencies": { + "com.unity.modules.tilemap": "1.0.0", + "com.unity.2d.tilemap": "1.0.0", + "com.unity.ugui": "1.0.0", + "com.unity.modules.jsonserialize": "1.0.0" + }, + "relatedPackages": { + "com.unity.2d.tilemap.extras.tests": "2.2.3" + }, + "samples": [ + { + "displayName": "Waterfall Animated Tile", + "description": "An example implementation of an Animated Tile.", + "path": "Samples~/WaterfallAnimatedTile" + }, + { + "displayName": "Pipe Rule Tile", + "description": "An example implementation of a Rule Tile with rules matching four-way orthogonal neighbors.", + "path": "Samples~/PipeRuleTile" + }, + { + "displayName": "Dungeon Rule Tile", + "description": "An example implementation of a Rule Tile with rules matching eight-way orthogonal and diagonal neighbors.", + "path": "Samples~/DungeonRuleTile" + } + ] +} diff --git a/MrBigsock/Packages/com.unity.2d.tilemap.extras/package.json.meta b/MrBigsock/Packages/com.unity.2d.tilemap.extras/package.json.meta new file mode 100644 index 0000000000000000000000000000000000000000..aa0617ac8743588a144c17ce573bafbbf1c9edaf --- /dev/null +++ b/MrBigsock/Packages/com.unity.2d.tilemap.extras/package.json.meta @@ -0,0 +1,7 @@ +fileFormatVersion: 2 +guid: a9f15b7c9363d9944a7d69248fd279ec +PackageManifestImporter: + externalObjects: {} + userData: + assetBundleName: + assetBundleVariant: diff --git a/MrBigsock/Packages/manifest.json b/MrBigsock/Packages/manifest.json index b2f01d0914f828e12edd79135052e61d819631e0..da3ca66bd2c7d4fc92eb139bc34fda755cf4dcfb 100644 --- a/MrBigsock/Packages/manifest.json +++ b/MrBigsock/Packages/manifest.json @@ -5,6 +5,7 @@ "com.unity.ide.rider": "3.0.15", "com.unity.ide.visualstudio": "2.0.16", "com.unity.ide.vscode": "1.2.5", + "com.unity.inputsystem": "1.4.2", "com.unity.test-framework": "1.1.31", "com.unity.textmeshpro": "3.0.6", "com.unity.timeline": "1.6.4", diff --git a/MrBigsock/Packages/packages-lock.json b/MrBigsock/Packages/packages-lock.json index 6a53eabe1eafa4b01b1bf46763133eaa86705763..352a86fc4c82b80d2f268be33a3e68fa874fae87 100644 --- a/MrBigsock/Packages/packages-lock.json +++ b/MrBigsock/Packages/packages-lock.json @@ -74,16 +74,15 @@ "dependencies": {} }, "com.unity.2d.tilemap.extras": { - "version": "2.2.3", - "depth": 1, - "source": "registry", + "version": "file:com.unity.2d.tilemap.extras", + "depth": 0, + "source": "embedded", "dependencies": { "com.unity.modules.tilemap": "1.0.0", "com.unity.2d.tilemap": "1.0.0", "com.unity.ugui": "1.0.0", "com.unity.modules.jsonserialize": "1.0.0" - }, - "url": "https://packages.unity.com" + } }, "com.unity.burst": { "version": "1.6.6", @@ -149,6 +148,15 @@ "dependencies": {}, "url": "https://packages.unity.com" }, + "com.unity.inputsystem": { + "version": "1.4.2", + "depth": 0, + "source": "registry", + "dependencies": { + "com.unity.modules.uielements": "1.0.0" + }, + "url": "https://packages.unity.com" + }, "com.unity.mathematics": { "version": "1.2.6", "depth": 2, diff --git a/MrBigsock/ProjectSettings/ProjectSettings.asset b/MrBigsock/ProjectSettings/ProjectSettings.asset index 557564cbdce085574b632223c4d490b68b891730..210c5ac20666f8e454f07677b68ed531f064c1fa 100644 --- a/MrBigsock/ProjectSettings/ProjectSettings.asset +++ b/MrBigsock/ProjectSettings/ProjectSettings.asset @@ -656,7 +656,7 @@ PlayerSettings: m_VersionCode: 1 m_VersionName: apiCompatibilityLevel: 6 - activeInputHandler: 0 + activeInputHandler: 2 cloudProjectId: framebufferDepthMemorylessMode: 0 qualitySettingsNames: [] diff --git a/MrBigsock/ProjectSettings/SceneTemplateSettings.json b/MrBigsock/ProjectSettings/SceneTemplateSettings.json new file mode 100644 index 0000000000000000000000000000000000000000..6f3e60fd8b7178b90a74640de7a6006d7a2b6565 --- /dev/null +++ b/MrBigsock/ProjectSettings/SceneTemplateSettings.json @@ -0,0 +1,167 @@ +{ + "templatePinStates": [], + "dependencyTypeInfos": [ + { + "userAdded": false, + "type": "UnityEngine.AnimationClip", + "ignore": false, + "defaultInstantiationMode": 0, + "supportsModification": true + }, + { + "userAdded": false, + "type": "UnityEditor.Animations.AnimatorController", + "ignore": false, + "defaultInstantiationMode": 0, + "supportsModification": true + }, + { + "userAdded": false, + "type": "UnityEngine.AnimatorOverrideController", + "ignore": false, + "defaultInstantiationMode": 0, + "supportsModification": true + }, + { + "userAdded": false, + "type": "UnityEditor.Audio.AudioMixerController", + "ignore": false, + "defaultInstantiationMode": 0, + "supportsModification": true + }, + { + "userAdded": false, + "type": "UnityEngine.ComputeShader", + "ignore": true, + "defaultInstantiationMode": 1, + "supportsModification": true + }, + { + "userAdded": false, + "type": "UnityEngine.Cubemap", + "ignore": false, + "defaultInstantiationMode": 0, + "supportsModification": true + }, + { + "userAdded": false, + "type": "UnityEngine.GameObject", + "ignore": false, + "defaultInstantiationMode": 0, + "supportsModification": true + }, + { + "userAdded": false, + "type": "UnityEditor.LightingDataAsset", + "ignore": false, + "defaultInstantiationMode": 0, + "supportsModification": false + }, + { + "userAdded": false, + "type": "UnityEngine.LightingSettings", + "ignore": false, + "defaultInstantiationMode": 0, + "supportsModification": true + }, + { + "userAdded": false, + "type": "UnityEngine.Material", + "ignore": false, + "defaultInstantiationMode": 0, + "supportsModification": true + }, + { + "userAdded": false, + "type": "UnityEditor.MonoScript", + "ignore": true, + "defaultInstantiationMode": 1, + "supportsModification": true + }, + { + "userAdded": false, + "type": "UnityEngine.PhysicMaterial", + "ignore": false, + "defaultInstantiationMode": 0, + "supportsModification": true + }, + { + "userAdded": false, + "type": "UnityEngine.PhysicsMaterial2D", + "ignore": false, + "defaultInstantiationMode": 0, + "supportsModification": true + }, + { + "userAdded": false, + "type": "UnityEngine.Rendering.PostProcessing.PostProcessProfile", + "ignore": false, + "defaultInstantiationMode": 0, + "supportsModification": true + }, + { + "userAdded": false, + "type": "UnityEngine.Rendering.PostProcessing.PostProcessResources", + "ignore": false, + "defaultInstantiationMode": 0, + "supportsModification": true + }, + { + "userAdded": false, + "type": "UnityEngine.Rendering.VolumeProfile", + "ignore": false, + "defaultInstantiationMode": 0, + "supportsModification": true + }, + { + "userAdded": false, + "type": "UnityEditor.SceneAsset", + "ignore": false, + "defaultInstantiationMode": 0, + "supportsModification": false + }, + { + "userAdded": false, + "type": "UnityEngine.Shader", + "ignore": true, + "defaultInstantiationMode": 1, + "supportsModification": true + }, + { + "userAdded": false, + "type": "UnityEngine.ShaderVariantCollection", + "ignore": true, + "defaultInstantiationMode": 1, + "supportsModification": true + }, + { + "userAdded": false, + "type": "UnityEngine.Texture", + "ignore": false, + "defaultInstantiationMode": 0, + "supportsModification": true + }, + { + "userAdded": false, + "type": "UnityEngine.Texture2D", + "ignore": false, + "defaultInstantiationMode": 0, + "supportsModification": true + }, + { + "userAdded": false, + "type": "UnityEngine.Timeline.TimelineAsset", + "ignore": false, + "defaultInstantiationMode": 0, + "supportsModification": true + } + ], + "defaultDependencyTypeInfo": { + "userAdded": false, + "type": "<default_scene_template_dependencies>", + "ignore": false, + "defaultInstantiationMode": 1, + "supportsModification": true + }, + "newSceneOverride": 0 +} \ No newline at end of file